# pypme – Python package for PME (Public Market Equivalent) calculation
Based on the [Modified PME
method](https://en.wikipedia.org/wiki/Public_Market_Equivalent#Modified_PME).
## Example
```python
from pypme import verbose_xpme
from datetime import date
pmeirr, assetirr, df = verbose_xpme(
dates=[date(2015, 1, 1), date(2015, 6, 12), date(2016, 2, 15)],
cashflows=[-10000, 7500],
prices=[100, 120, 100],
pme_prices=[100, 150, 100],
)
```
Will return `0.5525698793027238` and `0.19495150355969598` for the IRRs and produce this
dataframe:
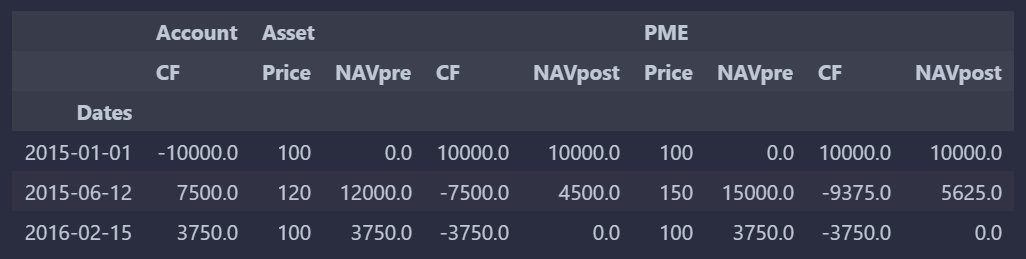
Notes:
- The `cashflows` are interpreted from a transaction account that is used to buy from an
asset at price `prices`.
- The corresponding prices for the PME are `pme_prices`.
- The `cashflows` is extended with one element representing the remaining value, that's
why all the other lists (`dates`, `prices`, `pme_prices`) need to be exactly 1 element
longer than `cashflows`.
## Variants
- `xpme`: Calculate PME for unevenly spaced / scheduled cashflows and return the PME IRR
only. In this case, the IRR is always annual.
- `verbose_xpme`: Calculate PME for unevenly spaced / scheduled cashflows and return
vebose information.
- `pme`: Calculate PME for evenly spaced cashflows and return the PME IRR only. In this
case, the IRR is for the underlying period.
- `verbose_pme`: Calculate PME for evenly spaced cashflows and return vebose
information.
- `tessa_xpme` and `tessa_verbose_xpme`: Use live price information via the tessa
library. See below.
## tessa examples – using tessa to retrieve PME prices online
Use `tessa_xpme` and `tessa_verbose_xpme` to get live prices via the [tessa
library](https://github.com/ymyke/tessa) and use those prices as the PME. Like so:
```python
from datetime import datetime, timezone
from pypme import tessa_xpme
common_args = {
"dates": [
datetime(2012, 1, 1, tzinfo=timezone.utc),
datetime(2013, 1, 1, tzinfo=timezone.utc)
],
"cashflows": [-100],
"prices": [1, 1],
}
print(tessa_xpme(pme_ticker="LIT", **common_args)) # source will default to "yahoo"
print(tessa_xpme(pme_ticker="bitcoin", pme_source="coingecko", **common_args))
print(tessa_xpme(pme_ticker="SREN.SW", pme_source="yahoo", **common_args))
```
Note that the dates need to be timezone-aware for these functions.
## Garbage in, garbage out
Note that the package will only perform essential sanity checks and otherwise just works
with what it gets, also with nonsensical data. E.g.:
```python
from pypme import verbose_pme
pmeirr, assetirr, df = verbose_pme(
cashflows=[-10, 500], prices=[1, 1, 1], pme_prices=[1, 1, 1]
)
```
Results in this df and IRRs of 0:
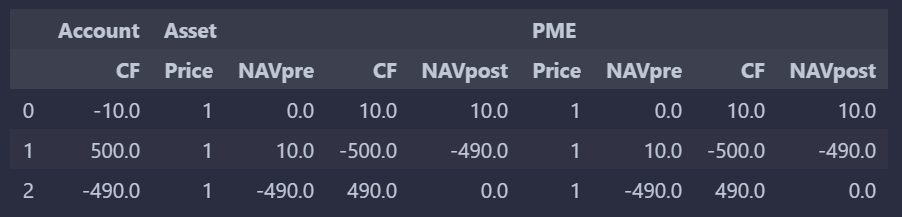
## Other noteworthy libraries
- [tessa](https://github.com/ymyke/tessa): Find financial assets and get their price history without worrying about different APIs or rate limiting.
- [strela](https://github.com/ymyke/strela): A python package for financial alerts.
## References
- [Google Sheet w/ reference calculation](https://docs.google.com/spreadsheets/d/1LMSBU19oWx8jw1nGoChfimY5asUA4q6Vzh7jRZ_7_HE/edit#gid=0)
- [Modified PME on Wikipedia](https://en.wikipedia.org/wiki/Public_Market_Equivalent#Modified_PME)
Raw data
{
"_id": null,
"home_page": "https://github.com/ymyke/pypme",
"name": "pypme",
"maintainer": "",
"docs_url": null,
"requires_python": ">=3.9",
"maintainer_email": "",
"keywords": "python,finance,investing,financial-analysis,pme,investment-analysis",
"author": "ymyke",
"author_email": "",
"download_url": "https://files.pythonhosted.org/packages/68/2d/0b0cbf757fb243b80ad124d70fce8888f90eaeb4da0235e70ffa26733970/pypme-0.6.1.tar.gz",
"platform": null,
"description": "# pypme \u2013 Python package for PME (Public Market Equivalent) calculation\n\nBased on the [Modified PME\nmethod](https://en.wikipedia.org/wiki/Public_Market_Equivalent#Modified_PME).\n\n## Example\n\n```python\nfrom pypme import verbose_xpme\nfrom datetime import date\n\npmeirr, assetirr, df = verbose_xpme(\n dates=[date(2015, 1, 1), date(2015, 6, 12), date(2016, 2, 15)],\n cashflows=[-10000, 7500],\n prices=[100, 120, 100],\n pme_prices=[100, 150, 100],\n)\n```\n\nWill return `0.5525698793027238` and `0.19495150355969598` for the IRRs and produce this\ndataframe:\n\n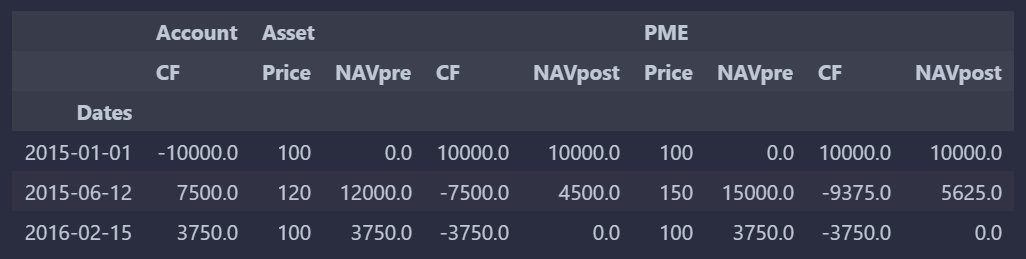\n\nNotes:\n- The `cashflows` are interpreted from a transaction account that is used to buy from an\n asset at price `prices`.\n- The corresponding prices for the PME are `pme_prices`.\n- The `cashflows` is extended with one element representing the remaining value, that's\n why all the other lists (`dates`, `prices`, `pme_prices`) need to be exactly 1 element\n longer than `cashflows`.\n\n## Variants\n\n- `xpme`: Calculate PME for unevenly spaced / scheduled cashflows and return the PME IRR\n only. In this case, the IRR is always annual.\n- `verbose_xpme`: Calculate PME for unevenly spaced / scheduled cashflows and return\n vebose information.\n- `pme`: Calculate PME for evenly spaced cashflows and return the PME IRR only. In this\n case, the IRR is for the underlying period.\n- `verbose_pme`: Calculate PME for evenly spaced cashflows and return vebose\n information.\n- `tessa_xpme` and `tessa_verbose_xpme`: Use live price information via the tessa\n library. See below.\n\n## tessa examples \u2013 using tessa to retrieve PME prices online\n\nUse `tessa_xpme` and `tessa_verbose_xpme` to get live prices via the [tessa\nlibrary](https://github.com/ymyke/tessa) and use those prices as the PME. Like so:\n\n```python\nfrom datetime import datetime, timezone\nfrom pypme import tessa_xpme\n\ncommon_args = {\n \"dates\": [\n datetime(2012, 1, 1, tzinfo=timezone.utc), \n datetime(2013, 1, 1, tzinfo=timezone.utc)\n ],\n \"cashflows\": [-100],\n \"prices\": [1, 1],\n}\nprint(tessa_xpme(pme_ticker=\"LIT\", **common_args)) # source will default to \"yahoo\"\nprint(tessa_xpme(pme_ticker=\"bitcoin\", pme_source=\"coingecko\", **common_args))\nprint(tessa_xpme(pme_ticker=\"SREN.SW\", pme_source=\"yahoo\", **common_args))\n```\n\nNote that the dates need to be timezone-aware for these functions.\n\n\n## Garbage in, garbage out\n\nNote that the package will only perform essential sanity checks and otherwise just works\nwith what it gets, also with nonsensical data. E.g.:\n\n```python\nfrom pypme import verbose_pme\n\npmeirr, assetirr, df = verbose_pme(\n cashflows=[-10, 500], prices=[1, 1, 1], pme_prices=[1, 1, 1]\n)\n```\n\nResults in this df and IRRs of 0:\n\n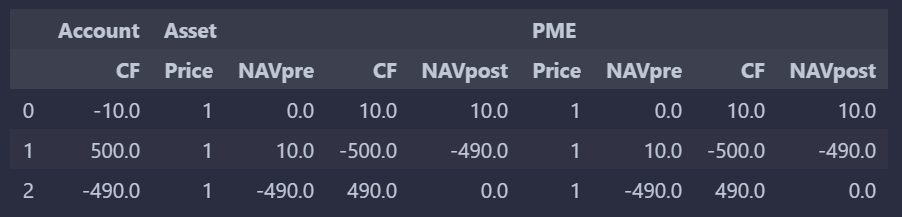\n\n\n## Other noteworthy libraries\n\n- [tessa](https://github.com/ymyke/tessa): Find financial assets and get their price history without worrying about different APIs or rate limiting.\n- [strela](https://github.com/ymyke/strela): A python package for financial alerts.\n\n\n## References\n\n- [Google Sheet w/ reference calculation](https://docs.google.com/spreadsheets/d/1LMSBU19oWx8jw1nGoChfimY5asUA4q6Vzh7jRZ_7_HE/edit#gid=0)\n- [Modified PME on Wikipedia](https://en.wikipedia.org/wiki/Public_Market_Equivalent#Modified_PME)\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "Python package for PME (Public Market Equivalent) calculation",
"version": "0.6.1",
"project_urls": {
"Homepage": "https://github.com/ymyke/pypme",
"Repository": "https://github.com/ymyke/pypme"
},
"split_keywords": [
"python",
"finance",
"investing",
"financial-analysis",
"pme",
"investment-analysis"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "23031a10a6aea37ed4d4fc3bdbf31b4ab5cde124a7ae26f98f85daeaac55fa91",
"md5": "63ee1f7ffa470cad46a298dd4795c28b",
"sha256": "729bb0741852140ed9f2b71e76cd2b5c553496eaf636fe83cc5539a6f2b1d365"
},
"downloads": -1,
"filename": "pypme-0.6.1-py3-none-any.whl",
"has_sig": false,
"md5_digest": "63ee1f7ffa470cad46a298dd4795c28b",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.9",
"size": 6021,
"upload_time": "2023-06-27T12:59:04",
"upload_time_iso_8601": "2023-06-27T12:59:04.604836Z",
"url": "https://files.pythonhosted.org/packages/23/03/1a10a6aea37ed4d4fc3bdbf31b4ab5cde124a7ae26f98f85daeaac55fa91/pypme-0.6.1-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "682d0b0cbf757fb243b80ad124d70fce8888f90eaeb4da0235e70ffa26733970",
"md5": "7e71d260d4727645b6d29e502fc5aee8",
"sha256": "3cf46a82f9f08258179901445c45911ef8146fbf01b8a2c7b1f4be577d2786d2"
},
"downloads": -1,
"filename": "pypme-0.6.1.tar.gz",
"has_sig": false,
"md5_digest": "7e71d260d4727645b6d29e502fc5aee8",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.9",
"size": 5861,
"upload_time": "2023-06-27T12:59:06",
"upload_time_iso_8601": "2023-06-27T12:59:06.223459Z",
"url": "https://files.pythonhosted.org/packages/68/2d/0b0cbf757fb243b80ad124d70fce8888f90eaeb4da0235e70ffa26733970/pypme-0.6.1.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-06-27 12:59:06",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "ymyke",
"github_project": "pypme",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"lcname": "pypme"
}