# PyramidMath - A Python Package for Pyramid Geometry and Mathematical Constants


[](https://pypi.org/project/pyramid-math/)
`pyramid_math` is a Python package for analyzing the geometrical properties and mathematical constants of pyramids. It provides tools for calculating various attributes such as apothem, edge length, slope angle, and comparisons with mathematical constants like π (Pi), φ (Golden Ratio), and Tribonacci constant. The package includes a predefined database of famous pyramids (e.g., Great Pyramid of Giza, Pyramid of Khafre, and more) and supports user-defined custom pyramids with specific measurements.
The package is designed for educational purposes, historical research, and exploring the mathematical connections of ancient pyramids. It can be used to perform detailed analysis, comparisons, and special calculations on pyramids, making it a valuable tool for students, researchers, and enthusiasts interested in pyramid geometry and mathematical relationships.
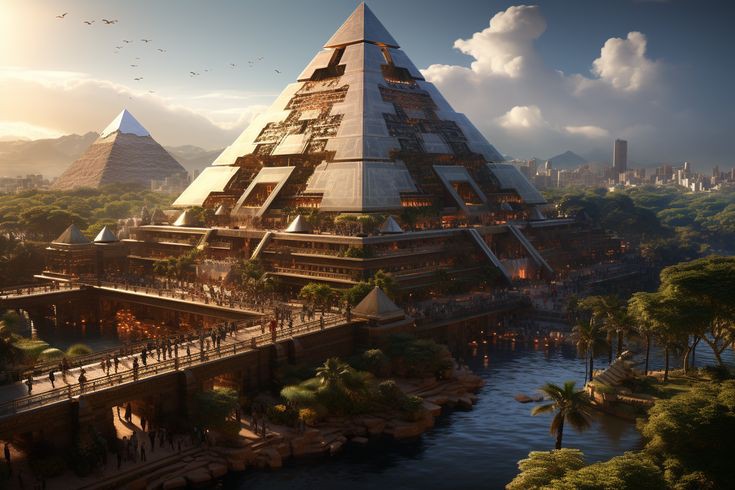
## Table of Contents
- [Features](#features)
- [Installation](#installation)
- [Usage](#usage)
- [Importing the Package](#importing-the-package)
- [Predefined Pyramid Example](#predefined-pyramid-example)
- [Custom Pyramid Example](#custom-pyramid-example)
- [List Available Pyramids](#list-available-pyramids)
- [Comparing Multiple Pyramids](#comparing-multiple-pyramids)
- [Mathematical Constants and Comparisons](#mathematical-constants-and-comparisons)
- [Detailed Analysis Output](#detailed-analysis-output)
- [CG (Center of Gravity)](#cg-center-of-gravity)
- [Contributing](#contributing)
- [License](#license)
## Features
- Calculate geometrical properties of pyramids (e.g., apothem, edge, slope angle).
- Compare pyramid measurements with known mathematical constants.
- Predefined database of famous pyramids (e.g., Great Pyramid of Giza, Pyramid of Khafre, and more).
- Supports both predefined pyramids and custom pyramids with user-defined measurements.
## Installation
You can install the package via `pip` from PyPI:
```bash
pip install pyramid_math
```
## Usage
Once installed, you can use the package to analyze various pyramids. Here’s how to get started:
### Importing the Package
```python
from pyramid_math.pyramid import Pyramid
```
### Predefined Pyramid Example
You can use one of the predefined pyramids from the database:
```python
# Load the Great Pyramid of Giza from the predefined database
giza = Pyramid.from_database("great_pyramid_giza")
# Perform detailed analysis
giza.detailed_analysis(json=False)
```
This will output a detailed analysis of the Great Pyramid, including the comparison with mathematical constants like Pi, Golden Ratio, Tribonacci constant, and others.
### Custom Pyramid Example
You can also create your own custom pyramid by providing its measurements:
```python
# Create a custom pyramid with specified measurements (base lengths and height)
pyramid = Pyramid("Test Pyramid", 100, 100, 50)
# Perform special calculations on the custom pyramid
special_calcs = pyramid.perform_special_calculations()
print(special_calcs)
```
**Example Output:**
```python
{
'pivalue': 3.0000,
'goldenratio': 1.6180,
'sqrt_goldenratio': 0.7273,
'difference_from_pi': 0.0000,
'difference_from_phi': 0.0000,
'slope_angle': 26.5651
'my_method_1_pi':3.0000,
'my_method_2_pi':3.0000,
'my_method_golden_ratio':1.6180
}
```
### List Available Pyramids
You can list all available pyramids from the predefined database:
```python
# List all available pyramids in the database
pyramids = Pyramid.list_pyramids()
print(pyramids)
```
**Example Output:**
```python
{
'great_pyramid_giza': 'Great Pyramid of Giza (Pyramid of Khufu)',
'pyramid_khafre': 'Pyramid of Khafre',
'red_pyramid': 'Red Pyramid',
'bent_pyramid': 'Bent Pyramid',
'pyramid_menkaure': 'Pyramid of Menkaure',
'sun_pyramid_teotihuacan': 'Pyramid of the Sun',
'pyramid_coba': 'Pyramid of Coba',
'nubian_pyramids': 'Nubian Pyramids of Meroe'
}
```
### Comparing Multiple Pyramids
You can compare measurements of multiple pyramids from the database:
```python
# Compare the Great Pyramid of Giza and the Pyramid of Khafre
comparison = Pyramid.compare_pyramids("great_pyramid_giza", "pyramid_khafre")
print(comparison)
```
**Example Output:**
```python
{
'Great Pyramid of Giza (Pyramid of Khufu)': {
'height': 481.0,
'base_length': 756.0,
'slope_angle': 51.84,
'pi_ratio': 3.1454,
'golden_ratio': 1.6180
},
'Pyramid of Khafre': {
'height': 448.0,
'base_length': 706.0,
'slope_angle': 53.13,
'pi_ratio': 3.1457,
'golden_ratio': 1.6182
}
}
```
## Mathematical Constants and Comparisons
The package compares the following constants:
- **π (Pi)**: The ratio of the base perimeter to the height of the pyramid.
- **φ (Golden Ratio)**: The ratio of the edge length to half of the base length.
- **Tribonacci Constant**: A constant derived from the geometry of the pyramid.
- **√5, √3**: Square roots of mathematical constants, compared with pyramid measurements.
### Detailed Analysis Output
When running the `detailed_analysis(json=False)` method, you will get comprehensive output that compares various calculations of the pyramid to well-known mathematical constants.
**Example Output:**
```bash
Detailed Analysis of Great Pyramid of Giza:
Measurements and Calculated Values:
North Base Length: 756.00 ft
Eastern Base Length: 756.00 ft
Height: 481.00 ft
Special Calculations vs Mathematical Constants:
(North base + Eastern base) / Height = 3.1454
Actual π value = 3.1416
Difference from π = 0.0038
Edge / (Base length / 2) = 1.6180
Actual φ (Golden Ratio) = 1.6180
Difference from φ = 0.0000
Apothem / Height = 0.7273
Actual √φ = 0.8510
Difference from √φ = 0.1237
Pyramid Slope Angle: 51.84 degrees
calculate_diagonal (CG) / Base Length = 0.9220
Difference from √2 = 0.0214
Calculated Tribonacci Constant = 1.8393
Actual Tribonacci Constant = 1.8393
Difference from Tribonacci Constant = 0.0000
(Base Length + Apothem) / Apothem = 2.6180
Actual √5 = 2.2361
Difference from √5 = 0.3819
(Height + CG + CG) / (North Base + Eastern Base) = 1.7321
Actual √3 = 1.7321
Difference from √3 = 0.0000
Apothem / (Apothem + Half Base Length) = 0.6180
Actual φ - 1 = 0.6180
Difference from φ - 1 = 0.0000
My method 1 pi:
Calculated: 3.143442090
Difference from pi: 0.001849430
My method 2 pi:
Calculated: 3.143451140
Difference from pi: 0.001858490
My method 3 golden ratio:
Calculated: 1.617506900
Difference from golden ratio: 0.000527090
```
### CG (Center of Gravity)
Note: In this package, **CG** refers to the **diagonal** of the pyramid, not the center of gravity. This is used in comparisons to constants like √2 and Tribonacci.
**Repository Views** 
## Contributing
Feel free to contribute by creating pull requests, reporting issues, or submitting feature requests.
## License
MIT License. See the [LICENSE](LICENSE) file for details.
Raw data
{
"_id": null,
"home_page": "https://github.com/ishanoshada/pyramid-math",
"name": "pyramid-math",
"maintainer": null,
"docs_url": null,
"requires_python": null,
"maintainer_email": null,
"keywords": null,
"author": "Ishan Oshada",
"author_email": "ishan.kodithuwakku.official@email.com",
"download_url": "https://files.pythonhosted.org/packages/39/92/557ec4de00e32ed4c0bc6b1c3b1bb1e3bdcc6758e2c3fddf76e70c7c6335/pyramid_math-0.0.5.tar.gz",
"platform": null,
"description": "# PyramidMath - A Python Package for Pyramid Geometry and Mathematical Constants\n\n\n\n[](https://pypi.org/project/pyramid-math/)\n\n\n`pyramid_math` is a Python package for analyzing the geometrical properties and mathematical constants of pyramids. It provides tools for calculating various attributes such as apothem, edge length, slope angle, and comparisons with mathematical constants like \u03c0 (Pi), \u03c6 (Golden Ratio), and Tribonacci constant. The package includes a predefined database of famous pyramids (e.g., Great Pyramid of Giza, Pyramid of Khafre, and more) and supports user-defined custom pyramids with specific measurements.\n\nThe package is designed for educational purposes, historical research, and exploring the mathematical connections of ancient pyramids. It can be used to perform detailed analysis, comparisons, and special calculations on pyramids, making it a valuable tool for students, researchers, and enthusiasts interested in pyramid geometry and mathematical relationships.\n\n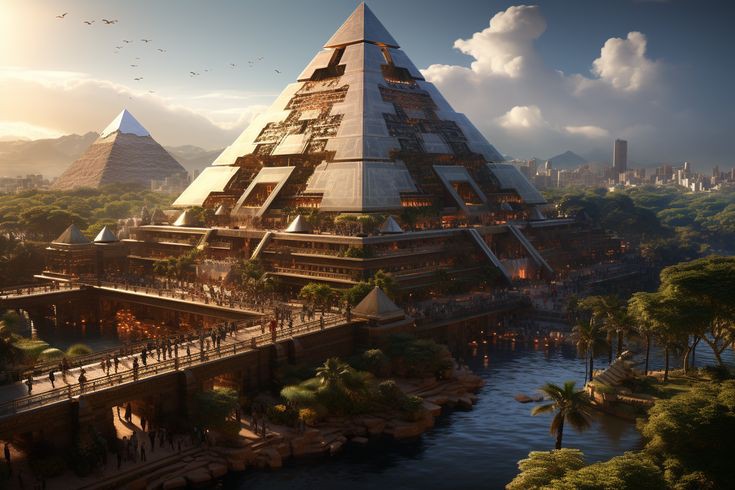\n\n\n## Table of Contents\n\n- [Features](#features)\n- [Installation](#installation)\n- [Usage](#usage)\n - [Importing the Package](#importing-the-package)\n - [Predefined Pyramid Example](#predefined-pyramid-example)\n - [Custom Pyramid Example](#custom-pyramid-example)\n - [List Available Pyramids](#list-available-pyramids)\n - [Comparing Multiple Pyramids](#comparing-multiple-pyramids)\n- [Mathematical Constants and Comparisons](#mathematical-constants-and-comparisons)\n - [Detailed Analysis Output](#detailed-analysis-output)\n - [CG (Center of Gravity)](#cg-center-of-gravity)\n- [Contributing](#contributing)\n- [License](#license)\n\n## Features\n\n- Calculate geometrical properties of pyramids (e.g., apothem, edge, slope angle).\n- Compare pyramid measurements with known mathematical constants.\n- Predefined database of famous pyramids (e.g., Great Pyramid of Giza, Pyramid of Khafre, and more).\n- Supports both predefined pyramids and custom pyramids with user-defined measurements.\n\n## Installation\n\nYou can install the package via `pip` from PyPI:\n\n```bash\npip install pyramid_math\n```\n\n## Usage\n\nOnce installed, you can use the package to analyze various pyramids. Here\u2019s how to get started:\n\n### Importing the Package\n\n```python\nfrom pyramid_math.pyramid import Pyramid\n```\n\n### Predefined Pyramid Example\n\nYou can use one of the predefined pyramids from the database:\n\n```python\n# Load the Great Pyramid of Giza from the predefined database\ngiza = Pyramid.from_database(\"great_pyramid_giza\")\n\n# Perform detailed analysis\ngiza.detailed_analysis(json=False)\n```\n\nThis will output a detailed analysis of the Great Pyramid, including the comparison with mathematical constants like Pi, Golden Ratio, Tribonacci constant, and others.\n\n### Custom Pyramid Example\n\nYou can also create your own custom pyramid by providing its measurements:\n\n```python\n# Create a custom pyramid with specified measurements (base lengths and height)\npyramid = Pyramid(\"Test Pyramid\", 100, 100, 50)\n\n# Perform special calculations on the custom pyramid\nspecial_calcs = pyramid.perform_special_calculations()\nprint(special_calcs)\n```\n\n**Example Output:**\n\n```python\n{\n 'pivalue': 3.0000,\n 'goldenratio': 1.6180,\n 'sqrt_goldenratio': 0.7273,\n 'difference_from_pi': 0.0000,\n 'difference_from_phi': 0.0000,\n 'slope_angle': 26.5651\n 'my_method_1_pi':3.0000,\n 'my_method_2_pi':3.0000,\n 'my_method_golden_ratio':1.6180\n}\n```\n\n### List Available Pyramids\n\nYou can list all available pyramids from the predefined database:\n\n```python\n# List all available pyramids in the database\npyramids = Pyramid.list_pyramids()\nprint(pyramids)\n```\n\n**Example Output:**\n\n```python\n{\n 'great_pyramid_giza': 'Great Pyramid of Giza (Pyramid of Khufu)',\n 'pyramid_khafre': 'Pyramid of Khafre',\n 'red_pyramid': 'Red Pyramid',\n 'bent_pyramid': 'Bent Pyramid',\n 'pyramid_menkaure': 'Pyramid of Menkaure',\n 'sun_pyramid_teotihuacan': 'Pyramid of the Sun',\n 'pyramid_coba': 'Pyramid of Coba',\n 'nubian_pyramids': 'Nubian Pyramids of Meroe'\n}\n```\n\n### Comparing Multiple Pyramids\n\nYou can compare measurements of multiple pyramids from the database:\n\n```python\n# Compare the Great Pyramid of Giza and the Pyramid of Khafre\ncomparison = Pyramid.compare_pyramids(\"great_pyramid_giza\", \"pyramid_khafre\")\nprint(comparison)\n```\n\n**Example Output:**\n\n```python\n{\n 'Great Pyramid of Giza (Pyramid of Khufu)': {\n 'height': 481.0,\n 'base_length': 756.0,\n 'slope_angle': 51.84,\n 'pi_ratio': 3.1454,\n 'golden_ratio': 1.6180\n },\n 'Pyramid of Khafre': {\n 'height': 448.0,\n 'base_length': 706.0,\n 'slope_angle': 53.13,\n 'pi_ratio': 3.1457,\n 'golden_ratio': 1.6182\n }\n}\n```\n\n## Mathematical Constants and Comparisons\n\nThe package compares the following constants:\n\n- **\u03c0 (Pi)**: The ratio of the base perimeter to the height of the pyramid.\n- **\u03c6 (Golden Ratio)**: The ratio of the edge length to half of the base length.\n- **Tribonacci Constant**: A constant derived from the geometry of the pyramid.\n- **\u221a5, \u221a3**: Square roots of mathematical constants, compared with pyramid measurements.\n\n### Detailed Analysis Output\n\nWhen running the `detailed_analysis(json=False)` method, you will get comprehensive output that compares various calculations of the pyramid to well-known mathematical constants.\n\n**Example Output:**\n\n```bash\nDetailed Analysis of Great Pyramid of Giza:\nMeasurements and Calculated Values:\nNorth Base Length: 756.00 ft\nEastern Base Length: 756.00 ft\nHeight: 481.00 ft\n\nSpecial Calculations vs Mathematical Constants:\n(North base + Eastern base) / Height = 3.1454\nActual \u03c0 value = 3.1416\nDifference from \u03c0 = 0.0038\n\n\nEdge / (Base length / 2) = 1.6180\nActual \u03c6 (Golden Ratio) = 1.6180\nDifference from \u03c6 = 0.0000\n\nApothem / Height = 0.7273\nActual \u221a\u03c6 = 0.8510\nDifference from \u221a\u03c6 = 0.1237\n\nPyramid Slope Angle: 51.84 degrees\n\ncalculate_diagonal (CG) / Base Length = 0.9220\nDifference from \u221a2 = 0.0214\n\nCalculated Tribonacci Constant = 1.8393\nActual Tribonacci Constant = 1.8393\nDifference from Tribonacci Constant = 0.0000\n\n(Base Length + Apothem) / Apothem = 2.6180\nActual \u221a5 = 2.2361\nDifference from \u221a5 = 0.3819\n\n(Height + CG + CG) / (North Base + Eastern Base) = 1.7321\nActual \u221a3 = 1.7321\nDifference from \u221a3 = 0.0000\n\nApothem / (Apothem + Half Base Length) = 0.6180\nActual \u03c6 - 1 = 0.6180\nDifference from \u03c6 - 1 = 0.0000\n\nMy method 1 pi:\nCalculated: 3.143442090\nDifference from pi: 0.001849430\n\nMy method 2 pi:\nCalculated: 3.143451140\nDifference from pi: 0.001858490\n\nMy method 3 golden ratio:\nCalculated: 1.617506900\nDifference from golden ratio: 0.000527090\n \n```\n\n### CG (Center of Gravity)\n\nNote: In this package, **CG** refers to the **diagonal** of the pyramid, not the center of gravity. This is used in comparisons to constants like \u221a2 and Tribonacci.\n\n**Repository Views** \n\n## Contributing\n\nFeel free to contribute by creating pull requests, reporting issues, or submitting feature requests.\n\n## License\n\nMIT License. See the [LICENSE](LICENSE) file for details.\n",
"bugtrack_url": null,
"license": null,
"summary": "A package for performing mathematical calculations on pyramids.",
"version": "0.0.5",
"project_urls": {
"Homepage": "https://github.com/ishanoshada/pyramid-math"
},
"split_keywords": [],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "8fe4bb7460409b775ceb824a6f4b2ccdffc2a44e34e0030062c9eb1ca23fac9d",
"md5": "46738c994eb8ee80ad8015e7dca55413",
"sha256": "44bc93d8539a38b085b03ba73a6bd9f6bc5074e4536c4b6e6950c799111fb347"
},
"downloads": -1,
"filename": "pyramid_math-0.0.5-py3-none-any.whl",
"has_sig": false,
"md5_digest": "46738c994eb8ee80ad8015e7dca55413",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": null,
"size": 10121,
"upload_time": "2025-01-17T15:09:51",
"upload_time_iso_8601": "2025-01-17T15:09:51.218890Z",
"url": "https://files.pythonhosted.org/packages/8f/e4/bb7460409b775ceb824a6f4b2ccdffc2a44e34e0030062c9eb1ca23fac9d/pyramid_math-0.0.5-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "3992557ec4de00e32ed4c0bc6b1c3b1bb1e3bdcc6758e2c3fddf76e70c7c6335",
"md5": "f83314c00c68ec83e0b3a889ea24f701",
"sha256": "c043bce55f79ef03b7f6bba57d7702769f9a7869c37d8383b7de01be01c610cc"
},
"downloads": -1,
"filename": "pyramid_math-0.0.5.tar.gz",
"has_sig": false,
"md5_digest": "f83314c00c68ec83e0b3a889ea24f701",
"packagetype": "sdist",
"python_version": "source",
"requires_python": null,
"size": 11422,
"upload_time": "2025-01-17T15:09:52",
"upload_time_iso_8601": "2025-01-17T15:09:52.212853Z",
"url": "https://files.pythonhosted.org/packages/39/92/557ec4de00e32ed4c0bc6b1c3b1bb1e3bdcc6758e2c3fddf76e70c7c6335/pyramid_math-0.0.5.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2025-01-17 15:09:52",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "ishanoshada",
"github_project": "pyramid-math",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "pyramid-math"
}