<img src="https://github.com/AtsushiSakai/PyRoombaAdapter/raw/master/docs/icon.png?raw=true" align="right" width="300"/>
# PyRoombaAdapter
A Python library for Roomba Open Interface
[](https://pepy.tech/project/pyroombaadapter)
[](https://pepy.tech/project/pyroombaadapter)
[](https://pepy.tech/project/pyroombaadapter)
# What is this?
This is a python library for Roomba Open Interface(ROI)
This module is based on the document:
- [iRobot® Roomba 500 Open Interface (OI) Specification](https://www.irobot.lv/uploaded_files/File/iRobot_Roomba_500_Open_Interface_Spec.pdf)
It aims to control a Roomba easily.
This module is only tested on Roomba 690 model.
# Install
You can use pip to install it.
$ pip install pyroombaadapter
- [pyroombaadapter · PyPI](https://pypi.org/project/pyroombaadapter/)
# Requirements
- Python 3.6.x or higher (2.7 is not supported)
- [pyserial](https://pythonhosted.org/pyserial/)
# Documentation
Please check the document for all API and usages.
- [Welcome to PyRoombaAdapter’s documentation\!](https://atsushisakai.github.io/PyRoombaAdapter/)
# Usage examples
All examples are in examples directory.
Click each image to see each example movie.
## Go and back example
[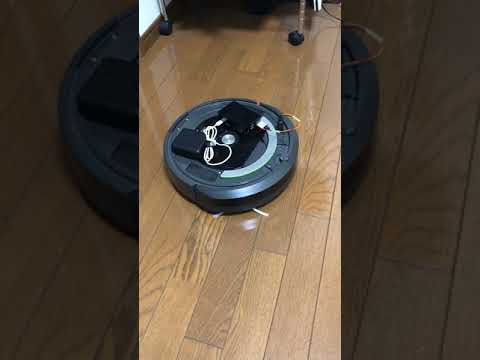](https://www.youtube.com/watch?v=rGppIKN-roE)
This example uses "move" API.
- [move API](https://atsushisakai.github.io/PyRoombaAdapter/API.html#pyroombaadapter.PyRoombaAdapter.move)
```python
"""
Go and back example with roomba
"""
from time import sleep
import math
from pyroombaadapter import PyRoombaAdapter
PORT = "/dev/ttyUSB0"
adapter = PyRoombaAdapter(PORT)
adapter.move(0.2, math.radians(0.0)) # go straight
sleep(1.0)
adapter.move(0, math.radians(-20)) # turn right
sleep(6.0)
adapter.move(0.2, math.radians(0.0)) # go straight
sleep(1.0)
adapter.move(0, math.radians(20)) # turn left
sleep(6.0)
#Print the total distance traveled
print(f"distance: {adapter.request_distance()} mm, angle: {adapter.request_angle()} rad")
```
## Play song1
[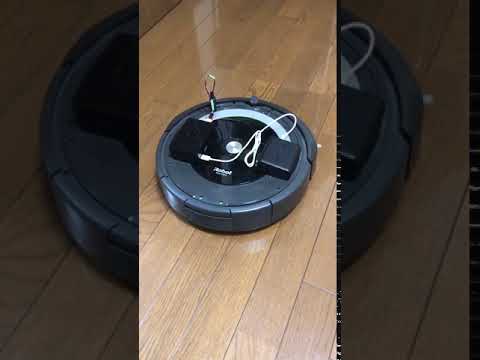](https://www.youtube.com/watch?v=0XqpQq7PQ8I)
This example uses "send_song_cmd" and "send_play_cmd" API.
- [send_song_cmd API](https://atsushisakai.github.io/PyRoombaAdapter/API.html#pyroombaadapter.PyRoombaAdapter.send_song_cmd)
- [send_play_cmd API](https://atsushisakai.github.io/PyRoombaAdapter/API.html#pyroombaadapter.PyRoombaAdapter.send_play_cmd)
```python
"""
Play Darth Vader song
"""
from time import sleep
from pyroombaadapter import PyRoombaAdapter
PORT = "/dev/ttyUSB0"
adapter = PyRoombaAdapter(PORT)
adapter.send_song_cmd(0, 9,
[69, 69, 69, 65, 72, 69, 65, 72, 69],
[40, 40, 40, 30, 10, 40, 30, 10, 80])
adapter.send_play_cmd(0)
sleep(10.0)
```
## Play song2
[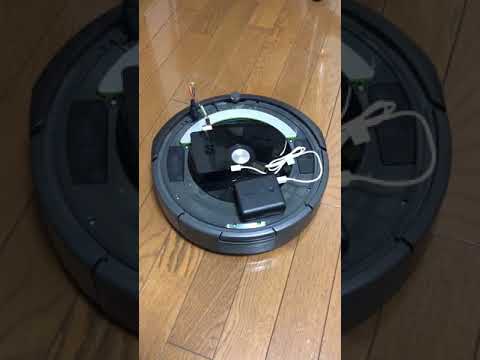](https://www.youtube.com/watch?v=nYstniMkJo0)
This example uses "send_song_cmd" and "send_play_cmd" API.
- [send_song_cmd API](https://atsushisakai.github.io/PyRoombaAdapter/API.html#pyroombaadapter.PyRoombaAdapter.send_song_cmd)
- [send_play_cmd API](https://atsushisakai.github.io/PyRoombaAdapter/API.html#pyroombaadapter.PyRoombaAdapter.send_play_cmd)
```python
"""
Play namidaga kirari by spitz
"""
from time import sleep
from pyroombaadapter import PyRoombaAdapter
PORT = "/dev/ttyUSB0"
adapter = PyRoombaAdapter(PORT)
adapter.send_song_cmd(0, 10,
[66, 67, 69, 67, 66, 62, 64, 66, 67, 66],
[16, 16, 16, 32, 32, 16, 16, 16, 16, 64])
sleep(1.0)
adapter.send_song_cmd(1, 9,
[66, 67, 69, 67, 66, 71, 59, 62, 61],
[16, 16, 16, 32, 32, 32, 16, 16, 64])
sleep(1.0)
adapter.send_song_cmd(2, 13,
[62, 64, 61, 62, 64, 66, 62, 64, 66, 67, 64, 66, 71],
[16, 16, 16, 16, 16, 16, 16, 16, 16, 16, 16, 16, 16])
sleep(1.0)
adapter.send_song_cmd(3, 7,
[71, 67, 64, 62, 61, 62, 62],
[16, 16, 16, 16, 48, 16, 64])
sleep(3.0)
adapter.send_play_cmd(0)
sleep(4.0)
adapter.send_play_cmd(1)
sleep(4.0)
adapter.send_play_cmd(0)
sleep(4.0)
adapter.send_play_cmd(1)
sleep(4.0)
adapter.send_play_cmd(2)
sleep(4.0)
adapter.send_play_cmd(3)
sleep(4.0)
```
## Read sensors
There are two ways how to read sensor values. Request manually on demand:
```python
"""
Read Roomba sensors
"""
from time import sleep
from pyroombaadapter import PyRoombaAdapter
PORT = "/dev/ttyUSB0"
adapter = PyRoombaAdapter(PORT)
adapter.change_mode_to_passive()
# Request sensor value manually
print(adapter.request_charging_state())
print(adapter.request_voltage())
print(adapter.request_current())
print(adapter.request_temperature())
print(adapter.request_charge())
print(adapter.request_capacity())
print(adapter.request_oi_mode())
print(adapter.request_distance())
print(adapter.request_angle())
```
Start a data stream:
```python
"""
Read Roomba sensors
"""
from time import sleep
from pyroombaadapter import PyRoombaAdapter
PORT = "/dev/ttyUSB0"
adapter = PyRoombaAdapter(PORT)
adapter.change_mode_to_passive()
# Read sensor value from data stream
adapter.data_stream_start(
["Charging State", "Voltage", "Current", "Temperature", "Battery Charge", "Battery Capacity", "OI Mode"])
sleep(1)
print(adapter.data_stream_read())
sleep(1)
print(adapter.data_stream_read())
sleep(1)
print(adapter.data_stream_read())
sleep(1)
adapter.data_stream_stop()
```
# Contribution
Any contributions to this project are welcome!
Feel free to make an issue and a PR to improve this OSS.
# License
MIT
# Authors
- [Atsushi Sakai](https://github.com/AtsushiSakai/)
Raw data
{
"_id": null,
"home_page": "https://github.com/AtsushiSakai/PyRoombaAdapter",
"name": "pyroombaadapter",
"maintainer": "Atsushi Sakai",
"docs_url": null,
"requires_python": ">3.6.0",
"maintainer_email": "asakaig@gmail.com",
"keywords": "python roomba",
"author": "Atsushi Sakai",
"author_email": "asakaig@gmail.com",
"download_url": "https://files.pythonhosted.org/packages/5a/49/cf35fec9abaf8186726abf7d141933aba055d5462b6bd79c844c5196ac27/pyroombaadapter-0.3.0.tar.gz",
"platform": null,
"description": "<img src=\"https://github.com/AtsushiSakai/PyRoombaAdapter/raw/master/docs/icon.png?raw=true\" align=\"right\" width=\"300\"/>\n\n# PyRoombaAdapter\n\nA Python library for Roomba Open Interface\n\n[](https://pepy.tech/project/pyroombaadapter)\n[](https://pepy.tech/project/pyroombaadapter)\n[](https://pepy.tech/project/pyroombaadapter)\n\n# What is this?\n\nThis is a python library for Roomba Open Interface(ROI)\n\nThis module is based on the document:\n\n- [iRobot\u00ae Roomba 500 Open Interface (OI) Specification](https://www.irobot.lv/uploaded_files/File/iRobot_Roomba_500_Open_Interface_Spec.pdf)\n\nIt aims to control a Roomba easily.\n\nThis module is only tested on Roomba 690 model. \n\n# Install\n\nYou can use pip to install it.\n\n $ pip install pyroombaadapter\n\n- [pyroombaadapter \u00b7 PyPI](https://pypi.org/project/pyroombaadapter/)\n\n# Requirements\n\n- Python 3.6.x or higher (2.7 is not supported)\n\n- [pyserial](https://pythonhosted.org/pyserial/)\n\n# Documentation\n\nPlease check the document for all API and usages.\n\n- [Welcome to PyRoombaAdapter\u2019s documentation\\!](https://atsushisakai.github.io/PyRoombaAdapter/)\n\n# Usage examples\n\nAll examples are in examples directory.\n\nClick each image to see each example movie.\n\n## Go and back example\n\n[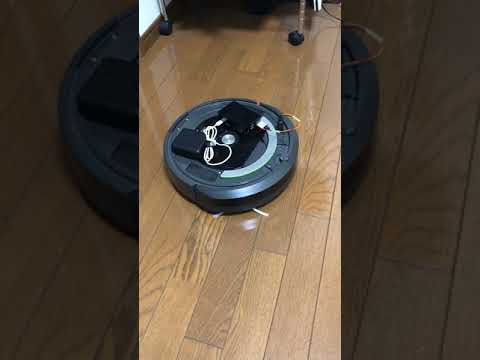](https://www.youtube.com/watch?v=rGppIKN-roE)\n\nThis example uses \"move\" API.\n\n- [move API](https://atsushisakai.github.io/PyRoombaAdapter/API.html#pyroombaadapter.PyRoombaAdapter.move)\n\n```python\n\"\"\"\n Go and back example with roomba\n\"\"\"\nfrom time import sleep\nimport math\nfrom pyroombaadapter import PyRoombaAdapter\n\nPORT = \"/dev/ttyUSB0\"\nadapter = PyRoombaAdapter(PORT)\nadapter.move(0.2, math.radians(0.0)) # go straight\nsleep(1.0)\nadapter.move(0, math.radians(-20)) # turn right\nsleep(6.0)\nadapter.move(0.2, math.radians(0.0)) # go straight\nsleep(1.0)\nadapter.move(0, math.radians(20)) # turn left\nsleep(6.0)\n\n#Print the total distance traveled\nprint(f\"distance: {adapter.request_distance()} mm, angle: {adapter.request_angle()} rad\")\n```\n\n## Play song1 \n\n[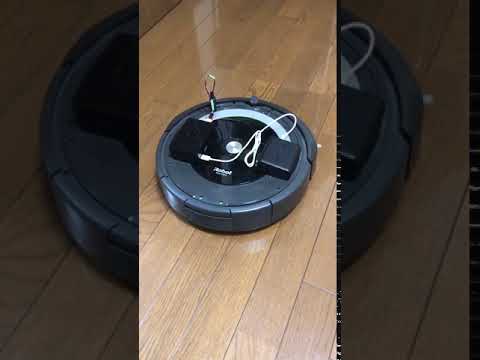](https://www.youtube.com/watch?v=0XqpQq7PQ8I)\n\nThis example uses \"send_song_cmd\" and \"send_play_cmd\" API.\n\n- [send_song_cmd API](https://atsushisakai.github.io/PyRoombaAdapter/API.html#pyroombaadapter.PyRoombaAdapter.send_song_cmd)\n\n- [send_play_cmd API](https://atsushisakai.github.io/PyRoombaAdapter/API.html#pyroombaadapter.PyRoombaAdapter.send_play_cmd)\n\n```python\n\"\"\"\n Play Darth Vader song\n\"\"\"\nfrom time import sleep\n\nfrom pyroombaadapter import PyRoombaAdapter\n\nPORT = \"/dev/ttyUSB0\"\nadapter = PyRoombaAdapter(PORT)\n\nadapter.send_song_cmd(0, 9,\n [69, 69, 69, 65, 72, 69, 65, 72, 69],\n [40, 40, 40, 30, 10, 40, 30, 10, 80])\nadapter.send_play_cmd(0)\nsleep(10.0)\n```\n\n## Play song2 \n\n[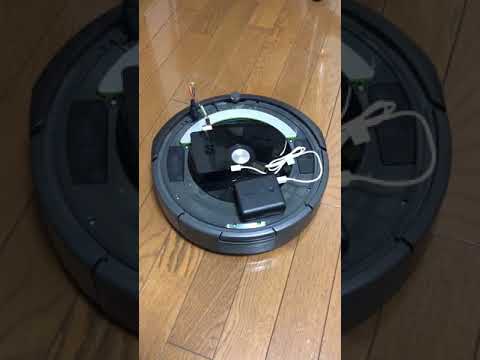](https://www.youtube.com/watch?v=nYstniMkJo0)\n\nThis example uses \"send_song_cmd\" and \"send_play_cmd\" API.\n\n- [send_song_cmd API](https://atsushisakai.github.io/PyRoombaAdapter/API.html#pyroombaadapter.PyRoombaAdapter.send_song_cmd)\n\n- [send_play_cmd API](https://atsushisakai.github.io/PyRoombaAdapter/API.html#pyroombaadapter.PyRoombaAdapter.send_play_cmd)\n\n```python\n\"\"\"\n Play namidaga kirari by spitz\n\"\"\"\nfrom time import sleep\n\nfrom pyroombaadapter import PyRoombaAdapter\n\nPORT = \"/dev/ttyUSB0\"\nadapter = PyRoombaAdapter(PORT)\n\nadapter.send_song_cmd(0, 10,\n [66, 67, 69, 67, 66, 62, 64, 66, 67, 66],\n [16, 16, 16, 32, 32, 16, 16, 16, 16, 64])\n\nsleep(1.0)\nadapter.send_song_cmd(1, 9,\n [66, 67, 69, 67, 66, 71, 59, 62, 61],\n [16, 16, 16, 32, 32, 32, 16, 16, 64])\n\nsleep(1.0)\nadapter.send_song_cmd(2, 13,\n [62, 64, 61, 62, 64, 66, 62, 64, 66, 67, 64, 66, 71],\n [16, 16, 16, 16, 16, 16, 16, 16, 16, 16, 16, 16, 16])\nsleep(1.0)\nadapter.send_song_cmd(3, 7,\n [71, 67, 64, 62, 61, 62, 62],\n [16, 16, 16, 16, 48, 16, 64])\n\nsleep(3.0)\nadapter.send_play_cmd(0)\nsleep(4.0)\nadapter.send_play_cmd(1)\nsleep(4.0)\nadapter.send_play_cmd(0)\nsleep(4.0)\nadapter.send_play_cmd(1)\nsleep(4.0)\nadapter.send_play_cmd(2)\nsleep(4.0)\nadapter.send_play_cmd(3)\nsleep(4.0)\n```\n\n## Read sensors\nThere are two ways how to read sensor values. Request manually on demand:\n\n```python\n\"\"\"\n Read Roomba sensors\n\"\"\"\nfrom time import sleep\nfrom pyroombaadapter import PyRoombaAdapter\n\nPORT = \"/dev/ttyUSB0\"\nadapter = PyRoombaAdapter(PORT)\nadapter.change_mode_to_passive()\n\n# Request sensor value manually\nprint(adapter.request_charging_state())\nprint(adapter.request_voltage())\nprint(adapter.request_current())\nprint(adapter.request_temperature())\nprint(adapter.request_charge())\nprint(adapter.request_capacity())\nprint(adapter.request_oi_mode())\nprint(adapter.request_distance())\nprint(adapter.request_angle())\n```\n\nStart a data stream:\n\n```python\n\"\"\"\n Read Roomba sensors\n\"\"\"\nfrom time import sleep\nfrom pyroombaadapter import PyRoombaAdapter\n\nPORT = \"/dev/ttyUSB0\"\nadapter = PyRoombaAdapter(PORT)\nadapter.change_mode_to_passive()\n\n# Read sensor value from data stream\nadapter.data_stream_start(\n [\"Charging State\", \"Voltage\", \"Current\", \"Temperature\", \"Battery Charge\", \"Battery Capacity\", \"OI Mode\"])\nsleep(1)\nprint(adapter.data_stream_read())\nsleep(1)\nprint(adapter.data_stream_read())\nsleep(1)\nprint(adapter.data_stream_read())\nsleep(1)\nadapter.data_stream_stop()\n```\n\n# Contribution\n\nAny contributions to this project are welcome!\n\nFeel free to make an issue and a PR to improve this OSS.\n\n# License\n\nMIT\n\n# Authors\n\n- [Atsushi Sakai](https://github.com/AtsushiSakai/)\n\n\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "A Python library for Roomba Open Interface",
"version": "0.3.0",
"project_urls": {
"Homepage": "https://github.com/AtsushiSakai/PyRoombaAdapter"
},
"split_keywords": [
"python",
"roomba"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "5a49cf35fec9abaf8186726abf7d141933aba055d5462b6bd79c844c5196ac27",
"md5": "808188a934846f786502b6b886872c47",
"sha256": "ac74c9052013ef5aef759924dd374567b73eedb8de74ca8c4dbe679962026017"
},
"downloads": -1,
"filename": "pyroombaadapter-0.3.0.tar.gz",
"has_sig": false,
"md5_digest": "808188a934846f786502b6b886872c47",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">3.6.0",
"size": 277444,
"upload_time": "2024-02-18T08:03:51",
"upload_time_iso_8601": "2024-02-18T08:03:51.239798Z",
"url": "https://files.pythonhosted.org/packages/5a/49/cf35fec9abaf8186726abf7d141933aba055d5462b6bd79c844c5196ac27/pyroombaadapter-0.3.0.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-02-18 08:03:51",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "AtsushiSakai",
"github_project": "PyRoombaAdapter",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "pyroombaadapter"
}