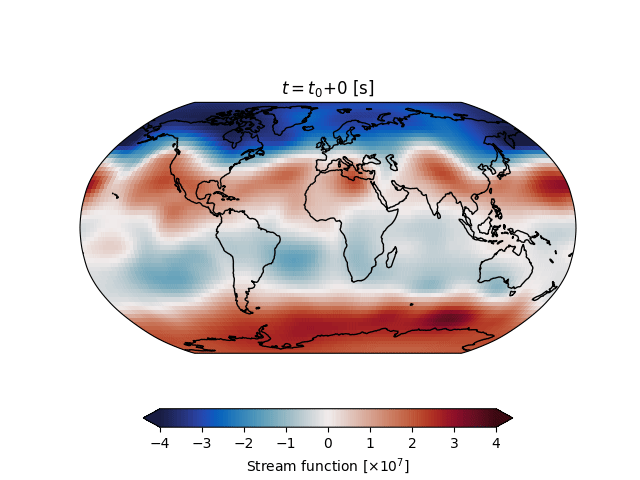
# pyshqg
[](https://pyshqg.readthedocs.io/en/latest/?badge=latest)
`pyshqg` is a python solver for the [Marshal and Molteni (1993) quasi-geostrophic (QG) model](https://doi.org/10.1175/1520-0469(1993)050%3C1792:TADUOP%3E2.0.CO;2).
QG models express the conservation of potential vorticity over time and are
meant to describe the large-scale circulation of the atmosphere under specific hypotheses.
This QG model is very special, because it is expressed in spherical harmonics and
because it encapsulates complex physical processes.
- [Documentation](https://pyshqg.readthedocs.io)
- [Source code](https://github.com/cerea-daml/pyshqg)
- [Issue tracker](https://github.com/cerea-daml/pyshqg/issues)
## Installation
Install using pip:
$ pip install pyshqg
More details can be found on this [page](https://pyshqg.readthedocs.io/en/latest/pages/installation.html).
## Usage
Here is a sneak peak at how to use the package.
First import all the machinery.
>>> import numpy as np
>>>
>>> from pyshqg.backend.numpy_backend import NumpyBackend as Backend
>>> from pyshqg.preprocessing.reference_data import load_test_data
>>> from pyshqg.core.constructors import construct_model, construct_integrator
Load the data and create the model and integrator.
>>> ds_test = load_test_data(internal_truncature=21, grid_truncature=31)
>>> backend = Backend(floatx='float64')
>>> model = construct_model(backend, ds_test.config)
>>> rk4 = construct_integrator(ds_test.config['rk4_integration'], model)
Perform one integration step and test against the test data.
>>> state = model.model_state(ds_test.spec_q.to_numpy())
>>> fwd_state = rk4.forward(state)
>>>
>>> def rms(x):
... return np.sqrt(np.mean(np.square(x)))
>>>
>>> def rmse(x, y):
... return rms(x-y)
>>>
>>> rms_ref = rms(ds_test.spec_rk4.to_numpy())
>>> rms_diff = rmse(
... backend.to_numpy(fwd_state['spec_q']),
... ds_test.spec_rk4.to_numpy(),
... )
>>> assert rms_diff < 1e-6 * rms_ref
Run the model for a full trajectory and convert the output to an ``xarray.Dataset``.
>>> state = model.model_state(ds_test.spec_q.to_numpy())
>>> trajectory = rk4.run(
... state,
... t_start=0,
... num_snapshots=8,
... num_steps_per_snapshot=10,
... variables=('q', 'psi'),
... )
>>> trajectory
<xarray.Dataset> Size: 14MB
Dimensions: (time: 9, batch: 16, level: 3, lat: 32, lon: 64)
Coordinates:
* time (time) int64 72B 0 18000 36000 54000 ... 90000 108000 126000 144000
* lat (lat) float64 256B -85.76 -80.27 -74.74 ... 74.74 80.27 85.76
* lon (lon) float64 512B 180.0 185.6 191.2 196.9 ... 163.1 168.8 174.4
Dimensions without coordinates: batch, level
Data variables:
q (time, batch, level, lat, lon) float64 7MB -0.000298 ... -0.0001269
psi (time, batch, level, lat, lon) float64 7MB 1.445e+08 ... -1.38e+07
More details can be found on this [page](https://pyshqg.readthedocs.io/en/latest/pages/examples.html).
## Aknowledgements
This python package is based on an original implementation of the model
in Fortran written at LMD by XXX.
## Todo-list
- make higher resolution gif animation
- make more example notebooks (see list below)
- fill in the documentation sections
- fill in docstrings, in particular with the convention used in the docs
- publish first version on pypi and conda-forge
- write pytorch/jax backends
- include unit tests in github CI
- apply linting and include it in github CI
Potential example notebooks:
- one notebook for each term of the QG model
- how to compute the forecast skill T15 vs T31 (as illustration)
- how to use tf backend to compute the grad
- gradient test for tf backend
- adjoint test for tf backend
- how to define a NN-based model error correction for the T21 and train it with dummy data
Raw data
{
"_id": null,
"home_page": "",
"name": "pyshqg",
"maintainer": "",
"docs_url": null,
"requires_python": ">=3.9",
"maintainer_email": "",
"keywords": "quasi-geostrophic,numerical model,atmosphere,spherical harmonics",
"author": "",
"author_email": "Alban Farchi <alban.farchi@enpc.fr>, Marc Bocquet <marc.bocquet@enpc.fr>, Quentin Malartic <quentin.malartic@enpc.fr>, Fabio D'Andrea <fabio.dandrea@lmd.ipsl.fr>",
"download_url": "https://files.pythonhosted.org/packages/07/b1/220190e402ce57a39454b1f8932d01312963219dbf451802bd232d9d0e7b/pyshqg-1.0.1.tar.gz",
"platform": null,
"description": "\n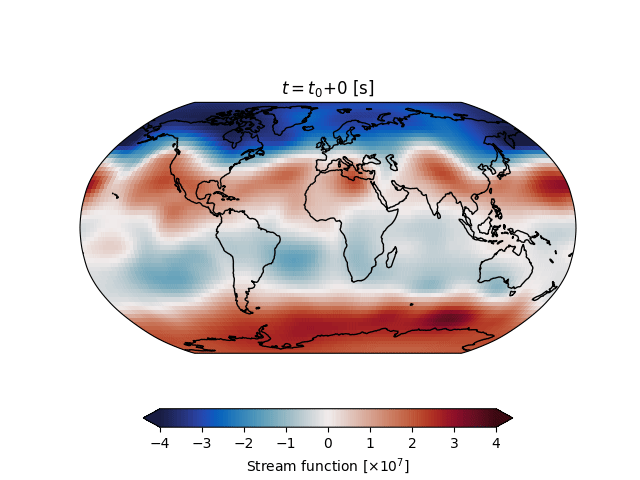\n\n# pyshqg\n\n[](https://pyshqg.readthedocs.io/en/latest/?badge=latest)\n\n`pyshqg` is a python solver for the [Marshal and Molteni (1993) quasi-geostrophic (QG) model](https://doi.org/10.1175/1520-0469(1993)050%3C1792:TADUOP%3E2.0.CO;2).\nQG models express the conservation of potential vorticity over time and are\nmeant to describe the large-scale circulation of the atmosphere under specific hypotheses.\nThis QG model is very special, because it is expressed in spherical harmonics and\nbecause it encapsulates complex physical processes.\n\n- [Documentation](https://pyshqg.readthedocs.io)\n- [Source code](https://github.com/cerea-daml/pyshqg)\n- [Issue tracker](https://github.com/cerea-daml/pyshqg/issues)\n\n## Installation\n\nInstall using pip:\n\n $ pip install pyshqg\n\nMore details can be found on this [page](https://pyshqg.readthedocs.io/en/latest/pages/installation.html).\n\n## Usage\n\nHere is a sneak peak at how to use the package.\nFirst import all the machinery.\n\n >>> import numpy as np\n >>>\n >>> from pyshqg.backend.numpy_backend import NumpyBackend as Backend\n >>> from pyshqg.preprocessing.reference_data import load_test_data\n >>> from pyshqg.core.constructors import construct_model, construct_integrator\n\nLoad the data and create the model and integrator.\n\n >>> ds_test = load_test_data(internal_truncature=21, grid_truncature=31)\n >>> backend = Backend(floatx='float64')\n >>> model = construct_model(backend, ds_test.config)\n >>> rk4 = construct_integrator(ds_test.config['rk4_integration'], model)\n\nPerform one integration step and test against the test data.\n\n >>> state = model.model_state(ds_test.spec_q.to_numpy())\n >>> fwd_state = rk4.forward(state)\n >>>\n >>> def rms(x):\n ... return np.sqrt(np.mean(np.square(x)))\n >>>\n >>> def rmse(x, y):\n ... return rms(x-y)\n >>>\n >>> rms_ref = rms(ds_test.spec_rk4.to_numpy())\n >>> rms_diff = rmse(\n ... backend.to_numpy(fwd_state['spec_q']),\n ... ds_test.spec_rk4.to_numpy(),\n ... )\n >>> assert rms_diff < 1e-6 * rms_ref\n\nRun the model for a full trajectory and convert the output to an ``xarray.Dataset``.\n\n >>> state = model.model_state(ds_test.spec_q.to_numpy())\n >>> trajectory = rk4.run(\n ... state,\n ... t_start=0,\n ... num_snapshots=8,\n ... num_steps_per_snapshot=10,\n ... variables=('q', 'psi'),\n ... )\n >>> trajectory\n <xarray.Dataset> Size: 14MB\n Dimensions: (time: 9, batch: 16, level: 3, lat: 32, lon: 64)\n Coordinates:\n * time (time) int64 72B 0 18000 36000 54000 ... 90000 108000 126000 144000\n * lat (lat) float64 256B -85.76 -80.27 -74.74 ... 74.74 80.27 85.76\n * lon (lon) float64 512B 180.0 185.6 191.2 196.9 ... 163.1 168.8 174.4\n Dimensions without coordinates: batch, level\n Data variables:\n q (time, batch, level, lat, lon) float64 7MB -0.000298 ... -0.0001269\n psi (time, batch, level, lat, lon) float64 7MB 1.445e+08 ... -1.38e+07\n\nMore details can be found on this [page](https://pyshqg.readthedocs.io/en/latest/pages/examples.html).\n\n## Aknowledgements\n\nThis python package is based on an original implementation of the model\nin Fortran written at LMD by XXX.\n\n## Todo-list\n\n- make higher resolution gif animation\n- make more example notebooks (see list below)\n- fill in the documentation sections\n- fill in docstrings, in particular with the convention used in the docs\n- publish first version on pypi and conda-forge\n- write pytorch/jax backends\n- include unit tests in github CI\n- apply linting and include it in github CI\n\nPotential example notebooks:\n\n- one notebook for each term of the QG model\n- how to compute the forecast skill T15 vs T31 (as illustration)\n- how to use tf backend to compute the grad\n- gradient test for tf backend\n- adjoint test for tf backend\n- how to define a NN-based model error correction for the T21 and train it with dummy data\n\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "QG Model in spherical harmonics",
"version": "1.0.1",
"project_urls": {
"Documentation": "https://pyshqg.readthedocs.io",
"Issues": "https://github.com/cerea-daml/pyshqg/issues",
"Source": "https://github.com/cerea-daml/pyshqg"
},
"split_keywords": [
"quasi-geostrophic",
"numerical model",
"atmosphere",
"spherical harmonics"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "df7ca130c9cc54f044b166fe698cdc6ecc7f1f33d2d295e61221d5c2910f42ea",
"md5": "b12b440029c28a1376ff596e4c72caf5",
"sha256": "1fdc32ad22ccd5940e94ab834cd4e90b676e9fbd5aa448e04b324090d44c9fda"
},
"downloads": -1,
"filename": "pyshqg-1.0.1-py3-none-any.whl",
"has_sig": false,
"md5_digest": "b12b440029c28a1376ff596e4c72caf5",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.9",
"size": 32526764,
"upload_time": "2024-03-13T11:23:04",
"upload_time_iso_8601": "2024-03-13T11:23:04.610640Z",
"url": "https://files.pythonhosted.org/packages/df/7c/a130c9cc54f044b166fe698cdc6ecc7f1f33d2d295e61221d5c2910f42ea/pyshqg-1.0.1-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "07b1220190e402ce57a39454b1f8932d01312963219dbf451802bd232d9d0e7b",
"md5": "60a25f0fced5850b5658befbae37e3c0",
"sha256": "07c849b64cf351de5cf8b8afc946fd30741304bfc54d744aae013c3b7be981f8"
},
"downloads": -1,
"filename": "pyshqg-1.0.1.tar.gz",
"has_sig": false,
"md5_digest": "60a25f0fced5850b5658befbae37e3c0",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.9",
"size": 32536658,
"upload_time": "2024-03-13T11:23:13",
"upload_time_iso_8601": "2024-03-13T11:23:13.429170Z",
"url": "https://files.pythonhosted.org/packages/07/b1/220190e402ce57a39454b1f8932d01312963219dbf451802bd232d9d0e7b/pyshqg-1.0.1.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-03-13 11:23:13",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "cerea-daml",
"github_project": "pyshqg",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"lcname": "pyshqg"
}