[](https://pypi.python.org/pypi/pysnoo2)
[](https://pypi.python.org/pypi/pysnoo2)
[](https://pypi.python.org/pypi/pysnoo2)
# pysnoo2
pysnoo2 is a python library to interact with the SNOO Smart Sleeper Bassinet. pysnoo2 is based on pysnoo by @rado0x54
[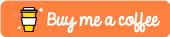](https://www.buymeacoffee.com/danpatten0)
## Disclaimer
Please use this library at your own risk and make sure that you do not violate the
[Terms of Service of HappiestBaby](https://www.happiestbaby.com/pages/terms-of-service). Note that happiest baby can change their API any anytime and may cause this library to break.
## Installation
```shell
pip install pysnoo2
```
## Known Issues
1. Refresh tokens are not currently working, access tokens are instead granted every 3 hours as needed.
## To Do
1. Save pubnub tokens off similar to how access tokens are saved for re-use
## Programmatic Usage
Programatically, the project provides two main class inferfaces. The Snoo API Client interface
[snoo.py](https://github.com/rado0x54/pysnoo/blob/master/pysnoo/snoo.py) and the Snoo PubNub
interface [pubnub.py](https://github.com/rado0x54/pysnoo/blob/master/pysnoo/pubnub.py).
Here's a short example to setup both. It uses the Snoo API Interface to get the Snoo serial number,
and access token, and pubnub access token which are required to initialize the PubNub interface. More usage examples can be
found by looking at the [CLI Tool](https://github.com/rado0x54/pysnoo/blob/master/scripts/snoo)
```python
async with SnooAuthSession(token, token_updater) as auth:
if not auth.authorized:
# Init Auth
new_token = await auth.fetch_token()
token_updater(new_token)
# Snoo API Interface
snoo = Snoo(auth)
devices = await snoo.get_devices()
if not devices:
# No devices
print('There is no Snoo connected to that account!')
else:
# Snoo PubNub Interface
pubnubToken = await snoo.pubnub_auth()
pubnub = SnooPubNub(pubnubToken,
snoo.pubnub_auth,
devices[0].serial_number,
f'pn-pysnoo-{devices[0].serial_number}')
last_activity_state = (await pubnub.history())[0]
if last_activity_state.state_machine.state == SessionLevel.ONLINE:
# Start
await pubnub.publish_start()
else:
# Stop
await pubnub.publish_goto_state(SessionLevel.ONLINE)
await pubnub.stop()
```
## CLI Usage
The pysnoo package contains the `snoo` CLI tool:
```shell
# snoo -h
Snoo Smart Bassinett
positional arguments:
{user,device,baby,last_session,status,sessions,session_avg,total,monitor,history,toggle,toggle_hold,up,down}
optional arguments:
-h, --help show this help message and exit
-u USERNAME, --username USERNAME
username for Snoo account
-p PASSWORD, --password PASSWORD
username for Snoo account
-t file, --token_file file
Cached token file to read and write an existing OAuth Token to.
-d DATETIME, --datetime DATETIME
Datetime in ISO8601 fromat. Used for some commands.
-l level, --level SessionLevel
Used to set the level with the level command. See SessionLevel for valid values.
-v, --verbose Show verbose logging.
```
### Credentials / Token / First Run
If the tool cannot find a valid oauth token in `--token_file` (`./.snoo_token.txt` by default), it will automatically query for
a username and password on the first run. After a successful authentication a token will be placed in the specified
file. Subsequent calls that have access to the token will not query for credentials any longer.
```shell
# snoo status
Username: snoo-user@gmail.com
Password:
awake (since: 0:34:54.891556)
# ls .snoo_token.txt
.snoo_token.txt
# snoo status
awake (since: 0:36:27.266366)
.snoo_token.txt
```
### Snoo CLI Commands
#### status
`snoo status` returns the current status of the snoo.
#### monitor
`snoo monitor` monitors the live events emited by the Snoo Bassinet. Monitoring can be cancelled
via `CTRL-C`. The command always returns the last historic event when launching.
```shell
# snoo monitor
{
"left_safety_clip": 1,
"rx_signal": {
"rssi": -45,
"strength": 99
},
"right_safety_clip": 1,
"sw_version": "v1.14.22",
"event_time_ms": 1698816595667,
"state_machine": {
"up_transition": "NONE",
"since_session_start_ms": -1,
"sticky_white_noise": "off",
"weaning": "off",
"time_left": -1,
"session_id": "0",
"state": "ONLINE",
"is_active_session": "false",
"down_transition": "NONE",
"hold": "off",
"audio": "on"
},
"system_state": "normal",
"event": "status_requested"
}
```
#### toggle
`snoo toggle` toggle the Snoo Bassinet between an active and a paused state.
#### toggle_hold
`snoo toggle_hold` will toggle the hold option on or off.
#### up
`snoo up` will transition the Snoo Bassinet one level up (if available)
#### down
`snoo down` will transition the Snoo Bassinet one level down (if available)
#### history
`snoo history` will return the last 100 events from the Snoo Bassinet.
```shell
# snoo history
{'event': 'cry',
'event_time': '2021-02-13T16:02:23.025Z',
'left_safety_clip': True,
'right_safety_clip': True,
'rx_signal': {'rssi': -45, 'strength': 100},
'state_machine': {'audio': True,
'down_transition': 'LEVEL1',
'hold': False,
'is_active_session': True,
'session_id': '1696520262',
'since_session_start': '1:01:40.420000',
'state': 'LEVEL2',
'sticky_white_noise': False,
'time_left': '0:06:00',
'up_transition': 'LEVEL3',
'weaning': False},
'sw_version': 'v1.14.12',
'system_state': 'normal'}
{'event': 'activity',
'event_time': '2021-02-13T16:02:40.628Z',
'left_safety_clip': True,
'right_safety_clip': True,
'rx_signal': {'rssi': -45, 'strength': 100},
'state_machine': {'audio': True,
'down_transition': 'NONE',
'hold': False,
'is_active_session': False,
'session_id': '0',
'since_session_start': None,
'state': 'ONLINE',
'sticky_white_noise': False,
'time_left': None,
'up_transition': 'NONE',
'weaning': False},
'sw_version': 'v1.14.12',
'system_state': 'normal'}
...
```
#### baby
`snoo baby` returns data about the baby and the settings of the linked Snoo Bassinet.
```shell
{'baby': 'ID',
'babyName': 'BABY_NAME',
'birthDate': '2021-01-17',
'createdAt': '2020-11-18T16:12:08.064Z',
'disabledLimiter': False,
'pictures': [{'encoded': False,
'id': 'PICTURE_ID',
'mime': 'image/png',
'updatedAt': '2021-01-21T10:45:07.542Z'}],
'preemie': None,
'settings': {'carRideMode': False,
'daytimeStart': 7,
'minimalLevel': 'baseline',
'minimalLevelVolume': 'lvl-1',
'motionLimiter': True,
'offlineLock': False,
'responsivenessLevel': 'lvl0',
'soothingLevelVolume': 'lvl0',
'weaning': False},
'sex': 'Male',
'updatedAt': '2021-02-02T22:23:10.770Z',
'updatedByUserAt': '2021-02-02T21:35:45.665Z'}
```
Some of these settings can be upgraded programmatically.
#### device
`snoo device` returns information around the linked Snoo Bassinet:
```shell
{'baby': 'BABY_ID',
'createdAt': '2019-02-19T19:03:13.544Z',
'firmwareUpdateDate': '2021-01-14T17:36:51.149Z',
'firmwareVersion': 'v1.14.12',
'lastProvisionSuccess': '2020-11-18T16:25:57.973Z',
'lastSSID': {'name': 'SSID-Network', 'updatedAt': '2021-01-28T22:04:21.151Z'},
'serialNumber': 'SERIAL_NUMBER',
'timezone': 'America/New_York',
'updatedAt': '2021-02-13T16:50:16.685Z'}
```
#### user
`snoo user` returns account related information:
```shell
{'email': 'EMAIL',
'givenName': 'GIVEN_NAME',
'region': 'US',
'surname': 'SURNAME',
'userId': 'USER_ID'}
```
#### last_session
`snoo last_session` returns information around the last or currently active session.
```shell
{'currentStatus': 'awake',
'currentStatusDuration': '0:51:04.121661',
'endTime': '2021-02-13T16:02:40.635Z',
'levels': ['BASELINE', 'LEVEL1', 'LEVEL2', 'ONLINE'],
'startTime': '2021-02-13T15:00:42.604Z'}
```
#### session_avg
`snoo session_avg -d DATETIME` returns the average values for the week starting from `DATETIME`.
```shell
# snoo session_avg -d 2021-01-30T07:00:00
{'daySleepAVG': '5:38:43',
'days': {'daySleep': ['4:46:18',
'5:06:47',
'5:32:30',
'7:41:16',
'6:15:46',
'5:39:27',
'4:28:57'],
'longestSleep': ['3:25:44',
'3:35:49',
'3:32:16',
'3:56:58',
'3:11:14',
'4:02:54',
'3:12:44'],
'nightSleep': ['8:12:50',
'8:30:22',
'6:40:29',
'6:42:13',
'8:46:07',
'6:24:24',
'8:14:42'],
'nightWakings': [4, 3, 6, 5, 4, 3, 3],
'totalSleep': ['12:59:08',
'13:37:09',
'12:12:59',
'14:23:29',
'15:01:53',
'12:03:51',
'12:43:39']},
'longestSleepAVG': '3:33:57',
'nightSleepAVG': '7:38:44',
'nightWakingsAVG': 4,
'totalSleepAVG': '13:17:27'}
```
#### total
`snoo total` returns the total time the Snoo Bassinet has been in operation.
```shell
# snoo total
11 days, 9:13:32
```
Raw data
{
"_id": null,
"home_page": "https://github.com/DanPatten/pysnoo2",
"name": "pysnoo2",
"maintainer": "",
"docs_url": null,
"requires_python": ">=3.7, <4",
"maintainer_email": "",
"keywords": "baby,snoo,happiest baby,home automation",
"author": "Dan Patten",
"author_email": "dan@danpatten.com",
"download_url": "https://files.pythonhosted.org/packages/ed/97/4f673dcd25029c3e4a09adbab5294d344c6995394bb58032e6d688272921/pysnoo2-0.3.3.tar.gz",
"platform": null,
"description": "[](https://pypi.python.org/pypi/pysnoo2)\r\n[](https://pypi.python.org/pypi/pysnoo2)\r\n[](https://pypi.python.org/pypi/pysnoo2)\r\n# pysnoo2\r\npysnoo2 is a python library to interact with the SNOO Smart Sleeper Bassinet. pysnoo2 is based on pysnoo by @rado0x54\r\n\r\n[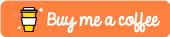](https://www.buymeacoffee.com/danpatten0)\r\n\r\n## Disclaimer\r\nPlease use this library at your own risk and make sure that you do not violate the\r\n[Terms of Service of HappiestBaby](https://www.happiestbaby.com/pages/terms-of-service). Note that happiest baby can change their API any anytime and may cause this library to break.\r\n\r\n## Installation\r\n```shell\r\npip install pysnoo2\r\n```\r\n\r\n## Known Issues\r\n1. Refresh tokens are not currently working, access tokens are instead granted every 3 hours as needed.\r\n\r\n## To Do\r\n1. Save pubnub tokens off similar to how access tokens are saved for re-use\r\n\r\n## Programmatic Usage\r\nProgramatically, the project provides two main class inferfaces. The Snoo API Client interface\r\n[snoo.py](https://github.com/rado0x54/pysnoo/blob/master/pysnoo/snoo.py) and the Snoo PubNub \r\ninterface [pubnub.py](https://github.com/rado0x54/pysnoo/blob/master/pysnoo/pubnub.py).\r\n\r\nHere's a short example to setup both. It uses the Snoo API Interface to get the Snoo serial number,\r\nand access token, and pubnub access token which are required to initialize the PubNub interface. More usage examples can be\r\nfound by looking at the [CLI Tool](https://github.com/rado0x54/pysnoo/blob/master/scripts/snoo)\r\n```python\r\nasync with SnooAuthSession(token, token_updater) as auth:\r\n\r\n if not auth.authorized:\r\n # Init Auth\r\n new_token = await auth.fetch_token()\r\n token_updater(new_token)\r\n\r\n # Snoo API Interface\r\n snoo = Snoo(auth)\r\n devices = await snoo.get_devices()\r\n if not devices:\r\n # No devices\r\n print('There is no Snoo connected to that account!')\r\n else:\r\n # Snoo PubNub Interface\r\n pubnubToken = await snoo.pubnub_auth()\r\n pubnub = SnooPubNub(pubnubToken,\r\n snoo.pubnub_auth,\r\n devices[0].serial_number,\r\n f'pn-pysnoo-{devices[0].serial_number}')\r\n \r\n last_activity_state = (await pubnub.history())[0]\r\n if last_activity_state.state_machine.state == SessionLevel.ONLINE:\r\n # Start\r\n await pubnub.publish_start()\r\n else:\r\n # Stop\r\n await pubnub.publish_goto_state(SessionLevel.ONLINE)\r\n \r\n await pubnub.stop()\r\n \r\n\r\n```\r\n\r\n## CLI Usage\r\nThe pysnoo package contains the `snoo` CLI tool:\r\n\r\n```shell\r\n# snoo -h\r\nSnoo Smart Bassinett\r\n\r\npositional arguments:\r\n {user,device,baby,last_session,status,sessions,session_avg,total,monitor,history,toggle,toggle_hold,up,down}\r\n\r\noptional arguments:\r\n -h, --help show this help message and exit\r\n -u USERNAME, --username USERNAME\r\n username for Snoo account\r\n -p PASSWORD, --password PASSWORD\r\n username for Snoo account\r\n -t file, --token_file file\r\n Cached token file to read and write an existing OAuth Token to.\r\n -d DATETIME, --datetime DATETIME\r\n Datetime in ISO8601 fromat. Used for some commands.\r\n -l level, --level SessionLevel\r\n Used to set the level with the level command. See SessionLevel for valid values.\r\n -v, --verbose Show verbose logging.\r\n```\r\n\r\n### Credentials / Token / First Run\r\nIf the tool cannot find a valid oauth token in `--token_file` (`./.snoo_token.txt` by default), it will automatically query for\r\na username and password on the first run. After a successful authentication a token will be placed in the specified\r\nfile. Subsequent calls that have access to the token will not query for credentials any longer.\r\n\r\n```shell\r\n# snoo status\r\nUsername: snoo-user@gmail.com\r\nPassword: \r\nawake (since: 0:34:54.891556)\r\n# ls .snoo_token.txt\r\n.snoo_token.txt\r\n# snoo status\r\nawake (since: 0:36:27.266366)\r\n.snoo_token.txt\r\n```\r\n### Snoo CLI Commands\r\n\r\n#### status\r\n`snoo status` returns the current status of the snoo.\r\n\r\n#### monitor\r\n`snoo monitor` monitors the live events emited by the Snoo Bassinet. Monitoring can be cancelled\r\nvia `CTRL-C`. The command always returns the last historic event when launching.\r\n```shell\r\n# snoo monitor\r\n{\r\n \"left_safety_clip\": 1,\r\n \"rx_signal\": {\r\n \"rssi\": -45,\r\n \"strength\": 99\r\n },\r\n \"right_safety_clip\": 1,\r\n \"sw_version\": \"v1.14.22\",\r\n \"event_time_ms\": 1698816595667,\r\n \"state_machine\": {\r\n \"up_transition\": \"NONE\",\r\n \"since_session_start_ms\": -1,\r\n \"sticky_white_noise\": \"off\",\r\n \"weaning\": \"off\",\r\n \"time_left\": -1,\r\n \"session_id\": \"0\",\r\n \"state\": \"ONLINE\",\r\n \"is_active_session\": \"false\",\r\n \"down_transition\": \"NONE\",\r\n \"hold\": \"off\",\r\n \"audio\": \"on\"\r\n },\r\n \"system_state\": \"normal\",\r\n \"event\": \"status_requested\"\r\n}\r\n```\r\n\r\n#### toggle\r\n`snoo toggle` toggle the Snoo Bassinet between an active and a paused state.\r\n\r\n#### toggle_hold\r\n`snoo toggle_hold` will toggle the hold option on or off.\r\n\r\n#### up\r\n`snoo up` will transition the Snoo Bassinet one level up (if available)\r\n\r\n#### down\r\n`snoo down` will transition the Snoo Bassinet one level down (if available)\r\n\r\n#### history\r\n`snoo history` will return the last 100 events from the Snoo Bassinet.\r\n\r\n```shell\r\n# snoo history\r\n{'event': 'cry',\r\n 'event_time': '2021-02-13T16:02:23.025Z',\r\n 'left_safety_clip': True,\r\n 'right_safety_clip': True,\r\n 'rx_signal': {'rssi': -45, 'strength': 100},\r\n 'state_machine': {'audio': True,\r\n 'down_transition': 'LEVEL1',\r\n 'hold': False,\r\n 'is_active_session': True,\r\n 'session_id': '1696520262',\r\n 'since_session_start': '1:01:40.420000',\r\n 'state': 'LEVEL2',\r\n 'sticky_white_noise': False,\r\n 'time_left': '0:06:00',\r\n 'up_transition': 'LEVEL3',\r\n 'weaning': False},\r\n 'sw_version': 'v1.14.12',\r\n 'system_state': 'normal'}\r\n{'event': 'activity',\r\n 'event_time': '2021-02-13T16:02:40.628Z',\r\n 'left_safety_clip': True,\r\n 'right_safety_clip': True,\r\n 'rx_signal': {'rssi': -45, 'strength': 100},\r\n 'state_machine': {'audio': True,\r\n 'down_transition': 'NONE',\r\n 'hold': False,\r\n 'is_active_session': False,\r\n 'session_id': '0',\r\n 'since_session_start': None,\r\n 'state': 'ONLINE',\r\n 'sticky_white_noise': False,\r\n 'time_left': None,\r\n 'up_transition': 'NONE',\r\n 'weaning': False},\r\n 'sw_version': 'v1.14.12',\r\n 'system_state': 'normal'}\r\n ...\r\n```\r\n\r\n#### baby\r\n`snoo baby` returns data about the baby and the settings of the linked Snoo Bassinet.\r\n\r\n```shell\r\n{'baby': 'ID',\r\n 'babyName': 'BABY_NAME',\r\n 'birthDate': '2021-01-17',\r\n 'createdAt': '2020-11-18T16:12:08.064Z',\r\n 'disabledLimiter': False,\r\n 'pictures': [{'encoded': False,\r\n 'id': 'PICTURE_ID',\r\n 'mime': 'image/png',\r\n 'updatedAt': '2021-01-21T10:45:07.542Z'}],\r\n 'preemie': None,\r\n 'settings': {'carRideMode': False,\r\n 'daytimeStart': 7,\r\n 'minimalLevel': 'baseline',\r\n 'minimalLevelVolume': 'lvl-1',\r\n 'motionLimiter': True,\r\n 'offlineLock': False,\r\n 'responsivenessLevel': 'lvl0',\r\n 'soothingLevelVolume': 'lvl0',\r\n 'weaning': False},\r\n 'sex': 'Male',\r\n 'updatedAt': '2021-02-02T22:23:10.770Z',\r\n 'updatedByUserAt': '2021-02-02T21:35:45.665Z'}\r\n```\r\nSome of these settings can be upgraded programmatically.\r\n\r\n#### device\r\n`snoo device` returns information around the linked Snoo Bassinet:\r\n```shell\r\n{'baby': 'BABY_ID',\r\n 'createdAt': '2019-02-19T19:03:13.544Z',\r\n 'firmwareUpdateDate': '2021-01-14T17:36:51.149Z',\r\n 'firmwareVersion': 'v1.14.12',\r\n 'lastProvisionSuccess': '2020-11-18T16:25:57.973Z',\r\n 'lastSSID': {'name': 'SSID-Network', 'updatedAt': '2021-01-28T22:04:21.151Z'},\r\n 'serialNumber': 'SERIAL_NUMBER',\r\n 'timezone': 'America/New_York',\r\n 'updatedAt': '2021-02-13T16:50:16.685Z'}\r\n```\r\n\r\n#### user\r\n`snoo user` returns account related information:\r\n```shell\r\n{'email': 'EMAIL',\r\n 'givenName': 'GIVEN_NAME',\r\n 'region': 'US',\r\n 'surname': 'SURNAME',\r\n 'userId': 'USER_ID'}\r\n```\r\n\r\n#### last_session\r\n`snoo last_session` returns information around the last or currently active session.\r\n```shell\r\n{'currentStatus': 'awake',\r\n 'currentStatusDuration': '0:51:04.121661',\r\n 'endTime': '2021-02-13T16:02:40.635Z',\r\n 'levels': ['BASELINE', 'LEVEL1', 'LEVEL2', 'ONLINE'],\r\n 'startTime': '2021-02-13T15:00:42.604Z'}\r\n```\r\n\r\n#### session_avg\r\n`snoo session_avg -d DATETIME` returns the average values for the week starting from `DATETIME`.\r\n\r\n```shell\r\n# snoo session_avg -d 2021-01-30T07:00:00\r\n{'daySleepAVG': '5:38:43',\r\n 'days': {'daySleep': ['4:46:18',\r\n '5:06:47',\r\n '5:32:30',\r\n '7:41:16',\r\n '6:15:46',\r\n '5:39:27',\r\n '4:28:57'],\r\n 'longestSleep': ['3:25:44',\r\n '3:35:49',\r\n '3:32:16',\r\n '3:56:58',\r\n '3:11:14',\r\n '4:02:54',\r\n '3:12:44'],\r\n 'nightSleep': ['8:12:50',\r\n '8:30:22',\r\n '6:40:29',\r\n '6:42:13',\r\n '8:46:07',\r\n '6:24:24',\r\n '8:14:42'],\r\n 'nightWakings': [4, 3, 6, 5, 4, 3, 3],\r\n 'totalSleep': ['12:59:08',\r\n '13:37:09',\r\n '12:12:59',\r\n '14:23:29',\r\n '15:01:53',\r\n '12:03:51',\r\n '12:43:39']},\r\n 'longestSleepAVG': '3:33:57',\r\n 'nightSleepAVG': '7:38:44',\r\n 'nightWakingsAVG': 4,\r\n 'totalSleepAVG': '13:17:27'}\r\n```\r\n\r\n#### total\r\n`snoo total` returns the total time the Snoo Bassinet has been in operation.\r\n```shell\r\n# snoo total\r\n11 days, 9:13:32\r\n```\r\n\r\n\r\n\r\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "A Python library and CLI to communicate with the Snoo Smart Baby Sleeper and Bassinet from Happiest Baby",
"version": "0.3.3",
"project_urls": {
"Homepage": "https://github.com/DanPatten/pysnoo2"
},
"split_keywords": [
"baby",
"snoo",
"happiest baby",
"home automation"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "b51cdc2091bd18c85c54500dacb24f74d7814f4aec816e46eca676265ba52137",
"md5": "ed7b589752bb2fdc06fb57c7a7bf9d9a",
"sha256": "60f6a50a2c3d840ce360f78d8127ca4b6fd696740c9b4a104570da82412344bb"
},
"downloads": -1,
"filename": "pysnoo2-0.3.3-py2.py3-none-any.whl",
"has_sig": false,
"md5_digest": "ed7b589752bb2fdc06fb57c7a7bf9d9a",
"packagetype": "bdist_wheel",
"python_version": "py2.py3",
"requires_python": ">=3.7, <4",
"size": 25275,
"upload_time": "2023-11-06T05:42:27",
"upload_time_iso_8601": "2023-11-06T05:42:27.882733Z",
"url": "https://files.pythonhosted.org/packages/b5/1c/dc2091bd18c85c54500dacb24f74d7814f4aec816e46eca676265ba52137/pysnoo2-0.3.3-py2.py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "ed974f673dcd25029c3e4a09adbab5294d344c6995394bb58032e6d688272921",
"md5": "46accf39bacb47cd657a35bc5dc4c5d8",
"sha256": "95eb0fcd96b376e43e92c75362ea2aeb1070ea83be83ec657a8aef9dd8e98bdb"
},
"downloads": -1,
"filename": "pysnoo2-0.3.3.tar.gz",
"has_sig": false,
"md5_digest": "46accf39bacb47cd657a35bc5dc4c5d8",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.7, <4",
"size": 27220,
"upload_time": "2023-11-06T05:42:29",
"upload_time_iso_8601": "2023-11-06T05:42:29.444334Z",
"url": "https://files.pythonhosted.org/packages/ed/97/4f673dcd25029c3e4a09adbab5294d344c6995394bb58032e6d688272921/pysnoo2-0.3.3.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-11-06 05:42:29",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "DanPatten",
"github_project": "pysnoo2",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"requirements": [],
"lcname": "pysnoo2"
}