# pystardog
[](https://badge.fury.io/py/pystardog)
a Python wrapper for communicating with the Stardog HTTP server.
**Docs**: [http://pystardog.readthedocs.io](http://pystardog.readthedocs.io)
**Requirements**: Python 3.8+
## What is it?
This library wraps all the functionality of a client for the Stardog
Knowledge Graph, and provides access to a full set of functions such
as executing SPARQL queries and many administrative tasks.
The implementation uses the HTTP protocol, since most of Stardog
functionality is available using this protocol. For more information,
see [HTTP
Programming](https://docs.stardog.com/developing/http-api)
in Stardog's documentation.
## Installation
pystardog is on [PyPI](https://pypi.org/project/pystardog/). To install:
```shell
pip install pystardog
```
## Quick Example
```python
import stardog
conn_details = {
'endpoint': 'http://localhost:5820',
'username': 'admin',
'password': 'admin'
}
with stardog.Admin(**conn_details) as admin:
db = admin.new_database('db')
with stardog.Connection('db', **conn_details) as conn:
conn.begin()
conn.add(stardog.content.File('./test/data/example.ttl'))
conn.commit()
results = conn.select('select * { ?a ?p ?o }')
db.drop()
```
## Interactive Tutorial
There is a Jupyter notebook and instructions in the [`notebooks`](./notebooks)
directory of this repository.
## Documentation
Documentation is available at [http://pystardog.readthedocs.io](http://pystardog.readthedocs.io)
### Build the Docs Locally
The docs can be built locally using [Sphinx](https://www.sphinx-doc.org/en/master/):
```shell
cd docs
pip install -r requirements.txt
make html
```
#### Autodoc Type Hints
The docs use [`sphinx-autodoc-typehints`](https://github.com/tox-dev/sphinx-autodoc-typehints) which allows you to omit types when documenting argument/returns types of functions. For example:
The following function:
```python
def database(self, name: str) -> "Database":
"""Retrieves an object representing a database.
:param name: The database name
:return: the database
"""
return Database(name, self.client)
```
will yield the following documentation after Sphinx processes it:
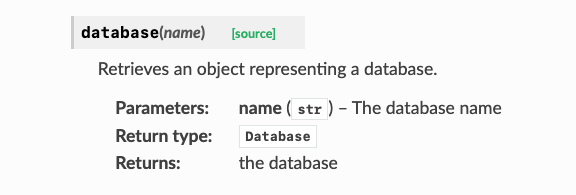
> **Note**
> Only arguments that have an existing `:param:` directive in the docstring get their
> respective `:type:` directives added. The `:rtype:` directive is added if and only if no existing `:rtype:` is found.
> See the [docs](https://github.com/tox-dev/sphinx-autodoc-typehints) for additional information on how the extension works.
#### Auto Build
Docs can be rebuilt automatically when saving a Python file by utilizing [`sphinx-autobuild`](https://github.com/executablebooks/sphinx-autobuild)
```shell
cd docs
pip install -r requirements.txt requirements-dev.txt
make livehtml
```
This should make the docs available at [http://localhost:8000](http://localhost:8000).
Example output after running `make livehtml`:
```text
❯ make livehtml
sphinx-autobuild "." "_build" --watch ../stardog/
[sphinx-autobuild] > sphinx-build /Users/frodo/projects/pystardog/docs /Users/frodo/projects/pystardog/docs/_build
Running Sphinx v6.2.1
loading pickled environment... done
building [mo]: targets for 0 po files that are out of date
writing output...
building [html]: targets for 0 source files that are out of date
updating environment: 0 added, 0 changed, 0 removed
reading sources...
looking for now-outdated files... none found
no targets are out of date.
build succeeded.
The HTML pages are in _build.
[I 230710 15:26:18 server:335] Serving on http://127.0.0.1:8000
[I 230710 15:26:18 handlers:62] Start watching changes
[I 230710 15:26:18 handlers:64] Start detecting changes
```
## Contributing and Development
Contrbutions are always welcome to pystardog.
To make a contribution:
1. Create a new branch off of `main`. There is no set naming convention for branches but try and keep it descriptive.
```bash
git checkout -b feature/add-support-for-X
```
2. Make your changes. If you are making substantive changes to pystardog, tests should be added to ensure your changes are working as expected. See [Running Tests](#running-tests) for additional information
about running tests.
3. Format your code. All Python code should be formatted using [Black](https://pypi.org/project/black/). See [Formatting Your Code](#formatting-your-code) for additional information.
4. Commit and push your code. Similar to branch names, there is no set structure for commit messages but try and keep your commit messages succinct and on topic.
```bash
git commit -am "feat: adds support for feature X"
git push origin feature/add-support-for-x
```
5. Create a pull request against `main`. All CircleCI checks should be passing in order to merge your PR. CircleCI will run tests against all supported versions of Python, single node and cluster tests for pystardog, as well as do some static analysis of the code.
### Running Tests
#### Requirements:
- [Docker](https://docs.docker.com/)
- [Docker Compose](https://docs.docker.com/compose/)
- Valid Stardog License
To run the tests locally, a valid Stardog license is required and placed at `dockerfiles/stardog-license-key.bin`.
1. Bring a stardog instance using docker-compose. For testing about 90% of the pystardog features, just a single node is sufficient,
although we also provide a cluster set up for further testing.
```shell
# Bring a single node instance plus a bunch of Virtual Graphs for testing (Recommended).
docker-compose -f docker-compose.single-node.yml up -d
# A cluster setup is also provided, if cluster only features are to be implemented and tested.
docker-compose -f docker-compose.cluster.yml up -d
```
2. Create a virtual environment with the necessary dependencies:
```shell
# Create a virtualenv and activate it
virtualenv -p $(which python3) venv
source venv/bin/activate
# Install dependencies
pip install -r requirements.txt -r test-requirements.txt
```
3. Run the test suite:
```shell
# Run the basic test suite (covers most of the pystardog functionalities)
pytest test/test_admin_basic.py test/test_connection.py test/test_utils.py -s
```
> **Note**
> Tests can be targeted against a specific Stardog endpoint by specifying an `--endpoint` option to `pytest`. Please note, that the tests will make modifications
> to the Stardog instance like deleting users, roles, databases, etc. By default, the `--endpoint` is set to `http://localhost:5820`,
> which is where the Dockerized Stardog (defined in the Docker compose files) is configured to be available at.
>
> ```bash
> pytest test/test_connection.py -k test_queries -s --endpoint https://my-other-stardog:5820
> ```
### Formatting your code
To format all the Python code:
```shell
# make sure black is install
virtualenv -p $(which python3) venv
. venv/bin/activate
pip install -r test-requirements.txt
# run black formatter
black .
```
Raw data
{
"_id": null,
"home_page": "https://github.com/stardog-union/pystardog",
"name": "pystardog",
"maintainer": "",
"docs_url": null,
"requires_python": "",
"maintainer_email": "",
"keywords": "",
"author": "Stardog Union",
"author_email": "support@stardog.com",
"download_url": "",
"platform": null,
"description": "# pystardog\n[](https://badge.fury.io/py/pystardog)\n\na Python wrapper for communicating with the Stardog HTTP server.\n\n**Docs**: [http://pystardog.readthedocs.io](http://pystardog.readthedocs.io)\n\n**Requirements**: Python 3.8+\n\n## What is it?\n\nThis library wraps all the functionality of a client for the Stardog\nKnowledge Graph, and provides access to a full set of functions such\nas executing SPARQL queries and many administrative tasks.\n\nThe implementation uses the HTTP protocol, since most of Stardog\nfunctionality is available using this protocol. For more information,\nsee [HTTP\nProgramming](https://docs.stardog.com/developing/http-api)\nin Stardog's documentation.\n\n## Installation\n\npystardog is on [PyPI](https://pypi.org/project/pystardog/). To install:\n\n```shell\npip install pystardog\n```\n\n## Quick Example\n\n```python\nimport stardog\n\nconn_details = {\n 'endpoint': 'http://localhost:5820',\n 'username': 'admin',\n 'password': 'admin'\n}\n\nwith stardog.Admin(**conn_details) as admin:\n db = admin.new_database('db')\n\n with stardog.Connection('db', **conn_details) as conn:\n conn.begin()\n conn.add(stardog.content.File('./test/data/example.ttl'))\n conn.commit()\n results = conn.select('select * { ?a ?p ?o }')\n\n db.drop()\n```\n\n## Interactive Tutorial\n\nThere is a Jupyter notebook and instructions in the [`notebooks`](./notebooks)\ndirectory of this repository.\n\n## Documentation\n\nDocumentation is available at [http://pystardog.readthedocs.io](http://pystardog.readthedocs.io)\n\n### Build the Docs Locally\n\nThe docs can be built locally using [Sphinx](https://www.sphinx-doc.org/en/master/):\n\n ```shell\n cd docs\n pip install -r requirements.txt\n make html\n ```\n\n#### Autodoc Type Hints\n\nThe docs use [`sphinx-autodoc-typehints`](https://github.com/tox-dev/sphinx-autodoc-typehints) which allows you to omit types when documenting argument/returns types of functions. For example:\n\nThe following function:\n\n```python\ndef database(self, name: str) -> \"Database\":\n \"\"\"Retrieves an object representing a database.\n\n :param name: The database name\n\n :return: the database\n \"\"\"\n return Database(name, self.client)\n```\n\nwill yield the following documentation after Sphinx processes it:\n\n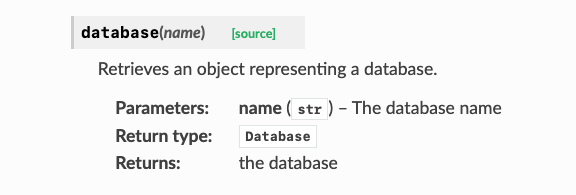\n\n> **Note**\n> Only arguments that have an existing `:param:` directive in the docstring get their\n> respective `:type:` directives added. The `:rtype:` directive is added if and only if no existing `:rtype:` is found.\n> See the [docs](https://github.com/tox-dev/sphinx-autodoc-typehints) for additional information on how the extension works.\n\n#### Auto Build \n\nDocs can be rebuilt automatically when saving a Python file by utilizing [`sphinx-autobuild`](https://github.com/executablebooks/sphinx-autobuild)\n\n```shell\ncd docs\npip install -r requirements.txt requirements-dev.txt\nmake livehtml\n```\n\nThis should make the docs available at [http://localhost:8000](http://localhost:8000).\n\nExample output after running `make livehtml`:\n\n```text\n\u276f make livehtml\nsphinx-autobuild \".\" \"_build\" --watch ../stardog/\n[sphinx-autobuild] > sphinx-build /Users/frodo/projects/pystardog/docs /Users/frodo/projects/pystardog/docs/_build\nRunning Sphinx v6.2.1\nloading pickled environment... done\nbuilding [mo]: targets for 0 po files that are out of date\nwriting output...\nbuilding [html]: targets for 0 source files that are out of date\nupdating environment: 0 added, 0 changed, 0 removed\nreading sources...\nlooking for now-outdated files... none found\nno targets are out of date.\nbuild succeeded.\n\nThe HTML pages are in _build.\n[I 230710 15:26:18 server:335] Serving on http://127.0.0.1:8000\n[I 230710 15:26:18 handlers:62] Start watching changes\n[I 230710 15:26:18 handlers:64] Start detecting changes\n```\n\n## Contributing and Development\n\nContrbutions are always welcome to pystardog.\n\nTo make a contribution:\n\n1. Create a new branch off of `main`. There is no set naming convention for branches but try and keep it descriptive.\n\n ```bash\n git checkout -b feature/add-support-for-X\n ```\n\n2. Make your changes. If you are making substantive changes to pystardog, tests should be added to ensure your changes are working as expected. See [Running Tests](#running-tests) for additional information\nabout running tests.\n\n3. Format your code. All Python code should be formatted using [Black](https://pypi.org/project/black/). See [Formatting Your Code](#formatting-your-code) for additional information.\n\n4. Commit and push your code. Similar to branch names, there is no set structure for commit messages but try and keep your commit messages succinct and on topic.\n\n ```bash\n git commit -am \"feat: adds support for feature X\"\n git push origin feature/add-support-for-x\n ```\n\n5. Create a pull request against `main`. All CircleCI checks should be passing in order to merge your PR. CircleCI will run tests against all supported versions of Python, single node and cluster tests for pystardog, as well as do some static analysis of the code.\n\n### Running Tests\n\n#### Requirements:\n\n- [Docker](https://docs.docker.com/)\n- [Docker Compose](https://docs.docker.com/compose/)\n- Valid Stardog License\n\nTo run the tests locally, a valid Stardog license is required and placed at `dockerfiles/stardog-license-key.bin`. \n\n1. Bring a stardog instance using docker-compose. For testing about 90% of the pystardog features, just a single node is sufficient,\nalthough we also provide a cluster set up for further testing. \n\n ```shell\n # Bring a single node instance plus a bunch of Virtual Graphs for testing (Recommended).\n docker-compose -f docker-compose.single-node.yml up -d\n\n # A cluster setup is also provided, if cluster only features are to be implemented and tested.\n docker-compose -f docker-compose.cluster.yml up -d\n ```\n\n2. Create a virtual environment with the necessary dependencies:\n\n ```shell\n # Create a virtualenv and activate it\n virtualenv -p $(which python3) venv\n source venv/bin/activate\n\n # Install dependencies\n pip install -r requirements.txt -r test-requirements.txt \n ```\n\n3. Run the test suite:\n\n ```shell\n # Run the basic test suite (covers most of the pystardog functionalities)\n pytest test/test_admin_basic.py test/test_connection.py test/test_utils.py -s \n ```\n\n > **Note**\n > Tests can be targeted against a specific Stardog endpoint by specifying an `--endpoint` option to `pytest`. Please note, that the tests will make modifications\n > to the Stardog instance like deleting users, roles, databases, etc. By default, the `--endpoint` is set to `http://localhost:5820`,\n > which is where the Dockerized Stardog (defined in the Docker compose files) is configured to be available at.\n >\n > ```bash\n > pytest test/test_connection.py -k test_queries -s --endpoint https://my-other-stardog:5820\n > ```\n\n### Formatting your code\n\nTo format all the Python code:\n\n ```shell\n # make sure black is install\n virtualenv -p $(which python3) venv\n . venv/bin/activate\n pip install -r test-requirements.txt\n\n # run black formatter\n black .\n ```\n",
"bugtrack_url": null,
"license": "",
"summary": "Use Stardog with Python!",
"version": "0.17.0",
"project_urls": {
"Homepage": "https://github.com/stardog-union/pystardog"
},
"split_keywords": [],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "01a4214a862aa7b548bd0a976333ab16b2dd96f003ef2b65f8200cef1c0141ce",
"md5": "49408500d7fe700505f46df706e83081",
"sha256": "f5afa9492bf073dc9c4c00d64cbefc6e4f274130090541f4dab4504b738285e7"
},
"downloads": -1,
"filename": "pystardog-0.17.0-py3-none-any.whl",
"has_sig": false,
"md5_digest": "49408500d7fe700505f46df706e83081",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": null,
"size": 62774,
"upload_time": "2023-09-18T20:01:04",
"upload_time_iso_8601": "2023-09-18T20:01:04.679975Z",
"url": "https://files.pythonhosted.org/packages/01/a4/214a862aa7b548bd0a976333ab16b2dd96f003ef2b65f8200cef1c0141ce/pystardog-0.17.0-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-09-18 20:01:04",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "stardog-union",
"github_project": "pystardog",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"circle": true,
"requirements": [],
"tox": true,
"lcname": "pystardog"
}