Name | pyswitchbee JSON |
Version |
1.8.3
JSON |
| download |
home_page | None |
Summary | SwitchBee Python Integration. |
upload_time | 2024-07-27 19:19:58 |
maintainer | None |
docs_url | None |
author | None |
requires_python | >=3.9 |
license | None |
keywords |
home
automation
switchbee
smart
|
VCS |
 |
bugtrack_url |
|
requirements |
No requirements were recorded.
|
Travis-CI |
No Travis.
|
coveralls test coverage |
No coveralls.
|
# pySwitchbee
A Python module library to control [SwitchBee](https://www.switchbee.com) smart home devices.


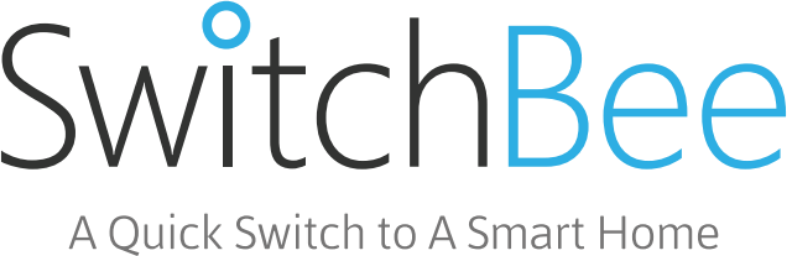
## Example code usage:
```python
from asyncio import get_event_loop
from aiohttp import ClientSession, ClientTimeout, TCPConnector
from switchbee.api import CentralUnitAPI
from switchbee.device import ApiStateCommand, DeviceType
async def main():
session = ClientSession(
connector=TCPConnector(ssl=False),
timeout=ClientTimeout(total=3),
)
cu = CentralUnitAPI("192.168.50.2", "user", "pass", session)
await cu.connect()
print(f"Central Unit Name: {cu.name}")
print(f"Central Unit MAC: {cu.mac}")
print(f"Central Unit Version: {cu.version}")
devices = await cu.devices
for device in devices:
# set the dimmer lights to 50% brightness
if device.type == DeviceType.Dimmer:
print(
"Discovered Dimmer device called {device.name}"
" current brightness is {device.brigt}"
)
await cu.set_state(device.id, 50)
# set the shutters position to 30% opened
if device.type == DeviceType.Shutter:
print(
"Discovered Shutter device called {device.name}"
" current position is {device.position}"
)
await cu.set_state(device.id, 30)
# turn off switches
if device.type == DeviceType.Switch:
print(
"Discovered Switch device called {device.name}"
" current state is {device.state}"
)
await cu.set_state(device.id, ApiStateCommand.OFF)
# set timer switch on for 10 minutes
if device.type == DeviceType.TimedPower:
print(
"Discovered Timed Power device called {device.name}"
" current state is {device.state} with {device.minutes_left} "
"minutes left until shutdown"
)
await cu.set_state(device.id, 10)
session.close()
if __name__ == "__main__":
get_event_loop().run_until_complete(main())
exit()
```
## Using the CLI tool:
Alternatively, it is possible to control [SwitchBee](https://www.switchbee.com) devices using the cli tool `switchbee_cli.py` as following:
To list devices that currently on:
`python switchbee_cli.py -i 192.168.50.2 -u USERNAME -p PASSWORD get_states --only-on`
```
'_state': 'ON',
'hardware': <HardwareType.Switch: 'DIMMABLE_SWITCH'>,
'id': 311,
'name': 'Ceiling',
'type': <DeviceType.Switch: 'SWITCH'>,
'zone': 'Outdoo Storage'}
{ '_state': 'ON',
'hardware': <HardwareType.Switch: 'DIMMABLE_SWITCH'>,
'id': 142,
'name': 'Spotlights',
'type': <DeviceType.Switch: 'SWITCH'>,
'zone': 'Porch'}
```
To set shutter with device id 392 position 50%:
`python switchbee_cli.py -i 192.168.50.2 -u USERNAME -p PASSWORD set_state --device-id 392 --state 50`
To turn on Power Timed Switch with device id 450 for 30 minutes:
`python switchbee_cli.py -i 192.168.50.2 -u USERNAME -p PASSWORD set_state --device-id 450 --state 30`
To turn off light with device id 122:
`python switchbee_cli.py -i 192.168.50.2 -u USERNAME -p PASSWORD set_state --device-id 122 --state OFF`
Raw data
{
"_id": null,
"home_page": null,
"name": "pyswitchbee",
"maintainer": null,
"docs_url": null,
"requires_python": ">=3.9",
"maintainer_email": null,
"keywords": "home, automation, switchbee, smart",
"author": null,
"author_email": "Jafar Atili <at.jafar@outlook.com>",
"download_url": "https://files.pythonhosted.org/packages/fe/2c/d6434e4c6c173733a0976907925d44380b08d00d616afb59b7ded94f8719/pyswitchbee-1.8.3.tar.gz",
"platform": null,
"description": "# pySwitchbee\n\nA Python module library to control [SwitchBee](https://www.switchbee.com) smart home devices.\n\n\n\n\n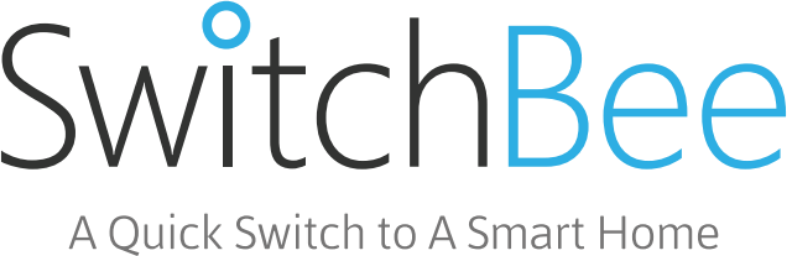\n\n\n\n## Example code usage:\n\n```python\nfrom asyncio import get_event_loop\n\nfrom aiohttp import ClientSession, ClientTimeout, TCPConnector\n\nfrom switchbee.api import CentralUnitAPI\nfrom switchbee.device import ApiStateCommand, DeviceType\n\n\nasync def main():\n session = ClientSession(\n connector=TCPConnector(ssl=False),\n timeout=ClientTimeout(total=3),\n )\n\n cu = CentralUnitAPI(\"192.168.50.2\", \"user\", \"pass\", session)\n await cu.connect()\n\n print(f\"Central Unit Name: {cu.name}\")\n print(f\"Central Unit MAC: {cu.mac}\")\n print(f\"Central Unit Version: {cu.version}\")\n\n devices = await cu.devices\n\n for device in devices:\n # set the dimmer lights to 50% brightness\n if device.type == DeviceType.Dimmer:\n print(\n \"Discovered Dimmer device called {device.name}\"\n \" current brightness is {device.brigt}\"\n )\n await cu.set_state(device.id, 50)\n\n # set the shutters position to 30% opened\n if device.type == DeviceType.Shutter:\n print(\n \"Discovered Shutter device called {device.name}\"\n \" current position is {device.position}\"\n )\n await cu.set_state(device.id, 30)\n\n # turn off switches\n if device.type == DeviceType.Switch:\n print(\n \"Discovered Switch device called {device.name}\"\n \" current state is {device.state}\"\n )\n await cu.set_state(device.id, ApiStateCommand.OFF)\n\n # set timer switch on for 10 minutes\n if device.type == DeviceType.TimedPower:\n print(\n \"Discovered Timed Power device called {device.name}\"\n \" current state is {device.state} with {device.minutes_left} \"\n \"minutes left until shutdown\"\n )\n await cu.set_state(device.id, 10)\n\n session.close()\n\n\nif __name__ == \"__main__\":\n\n get_event_loop().run_until_complete(main())\n exit()\n```\n\n## Using the CLI tool:\n\nAlternatively, it is possible to control [SwitchBee](https://www.switchbee.com) devices using the cli tool `switchbee_cli.py` as following:\n\nTo list devices that currently on:\n\n`python switchbee_cli.py -i 192.168.50.2 -u USERNAME -p PASSWORD get_states --only-on`\n\n```\n '_state': 'ON',\n 'hardware': <HardwareType.Switch: 'DIMMABLE_SWITCH'>,\n 'id': 311,\n 'name': 'Ceiling',\n 'type': <DeviceType.Switch: 'SWITCH'>,\n 'zone': 'Outdoo Storage'}\n\n{ '_state': 'ON',\n 'hardware': <HardwareType.Switch: 'DIMMABLE_SWITCH'>,\n 'id': 142,\n 'name': 'Spotlights',\n 'type': <DeviceType.Switch: 'SWITCH'>,\n 'zone': 'Porch'}\n```\n\nTo set shutter with device id 392 position 50%:\n\n`python switchbee_cli.py -i 192.168.50.2 -u USERNAME -p PASSWORD set_state --device-id 392 --state 50`\n\n\nTo turn on Power Timed Switch with device id 450 for 30 minutes:\n\n`python switchbee_cli.py -i 192.168.50.2 -u USERNAME -p PASSWORD set_state --device-id 450 --state 30`\n\n\nTo turn off light with device id 122:\n\n`python switchbee_cli.py -i 192.168.50.2 -u USERNAME -p PASSWORD set_state --device-id 122 --state OFF`\n",
"bugtrack_url": null,
"license": null,
"summary": "SwitchBee Python Integration.",
"version": "1.8.3",
"project_urls": {
"homepage": "https://pypi.org/project/pyswitchbee/",
"repository": "https://github.com/jafar-atili/pySwitchbee/"
},
"split_keywords": [
"home",
" automation",
" switchbee",
" smart"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "8c492948120ee1366434122439c738d81ab7fe791628d030d89e8887550bd0cb",
"md5": "71ca943e68fd783167ebc617556b9adc",
"sha256": "668ac21d1f74b08cd8aafb86e7ba442b27fc90f2923ad32acb2999e3450e1b8f"
},
"downloads": -1,
"filename": "pyswitchbee-1.8.3-py3-none-any.whl",
"has_sig": false,
"md5_digest": "71ca943e68fd783167ebc617556b9adc",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.9",
"size": 19646,
"upload_time": "2024-07-27T19:19:56",
"upload_time_iso_8601": "2024-07-27T19:19:56.595850Z",
"url": "https://files.pythonhosted.org/packages/8c/49/2948120ee1366434122439c738d81ab7fe791628d030d89e8887550bd0cb/pyswitchbee-1.8.3-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "fe2cd6434e4c6c173733a0976907925d44380b08d00d616afb59b7ded94f8719",
"md5": "81464e1ffa646f4e71ec3c51aa31670f",
"sha256": "62b4599b84f4b71f46369f9e7c57defb725812db99987d55eafde4efdc4df0ec"
},
"downloads": -1,
"filename": "pyswitchbee-1.8.3.tar.gz",
"has_sig": false,
"md5_digest": "81464e1ffa646f4e71ec3c51aa31670f",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.9",
"size": 16875,
"upload_time": "2024-07-27T19:19:58",
"upload_time_iso_8601": "2024-07-27T19:19:58.577381Z",
"url": "https://files.pythonhosted.org/packages/fe/2c/d6434e4c6c173733a0976907925d44380b08d00d616afb59b7ded94f8719/pyswitchbee-1.8.3.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-07-27 19:19:58",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "jafar-atili",
"github_project": "pySwitchbee",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"lcname": "pyswitchbee"
}