## Pytest Plugin to Show Unit Coverage
**Code** coverage tools like
[coverage.py](https://coverage.readthedocs.io/en/7.0.1/) show you the
instrumented code coverage of your tests, however it won't tell you if you've
written specific unit tests for each of your code's **units** (here unit meaning
function).
This package implements a mechanism for measuring and reporting unit coverage.
Instead of instrumenting your code you will need to mark tests with a
**pointer** to the unit that it is covering.
For example if you have in your code this module `mypackage/widget.py`:
``` python
def foo(in):
return in * 3
```
then in your test suite you would write a unit test for this function and mark it as relating to that unit, e.g. in `tests/test_widget.py`:
``` python
from mypackage.widget import foo
@pytest.mark.pointer(foo)
def test_foo():
assert foo(3) == 9
```
This package works by collecting all of the pointed-to units during test
execution and persists these to the pytest cache (typically somewhere under
`.pytest_cache`). Then in subsequent runs you need only report the results.
### Invocation
This package adds a couple new options to the `pytest` CLI:
`--pointers-collect=STR` (default `src`)
This explicitly indicates to collect unit coverage results. If not specified,
but `--pointers-report` is given results will be collected using the default.
`--pointers-report` (default `False`)
When this flag is given a textual report will be given at the end of the test
run. Note that even if this is not given the coverage checks will still be run.
`--pointers-func-min-pass=INT` (default `2`)
This flag controls the number of unit test pointer marks are needed to get a
"passing" unit. In the report units with 0 pointers are shown as red, passing
numbers are green, and anything in between is blue.
`--pointers-fail-under=FLOAT` (default `0.0`)
This flag controls the percentage of passing units are needed for the entire
coverage check to pass. The percentage is always displayed even without
`--pointers-report`. If this test is failed then the test process exits with
code 1, which is useful for things like CI.
#### Example
Here is an example with source code under the `src` folder, requiring 1 pointer
test per collected unit in the code, for all functions.
```
pytest --pointers-report --pointers-collect=src --pointers-func-min-pass=1 --pointers-fail-under=100 tests
```
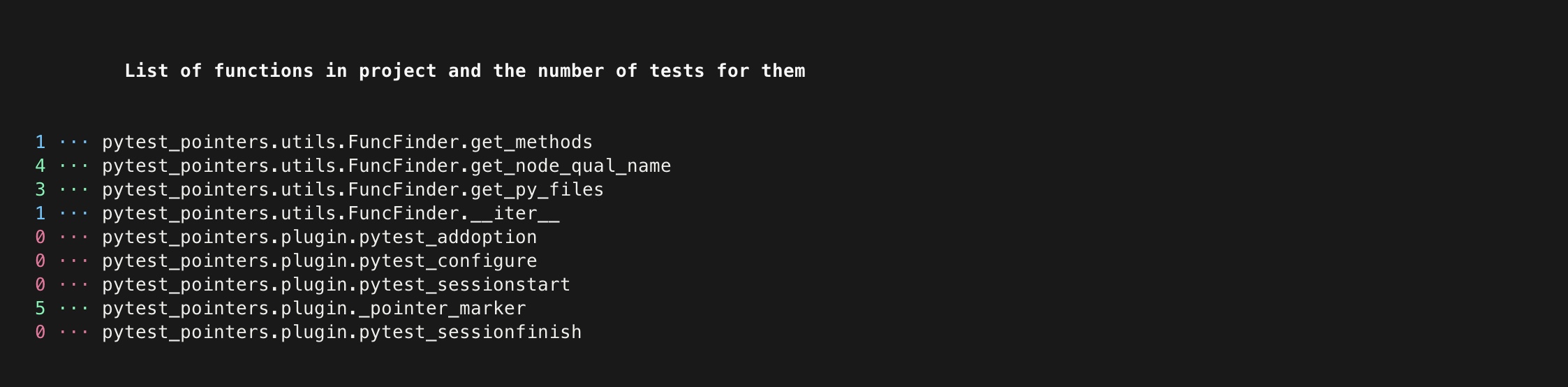
### Installation
``` shell
pip install pytest_pointers
```
Raw data
{
"_id": null,
"home_page": "https://github.com/jaklimoff/pytest-pointers",
"name": "pytest-pointers",
"maintainer": "",
"docs_url": null,
"requires_python": ">=3.7,<4.0",
"maintainer_email": "",
"keywords": "plugin,pytest",
"author": "Jack Klimov",
"author_email": "jaklimoff@gmail.com",
"download_url": "https://files.pythonhosted.org/packages/08/f1/d87614d1dd90394ac66a938aca7b4dd19f6b29a9bc47f44726fe11fe6bf1/pytest-pointers-0.3.1.tar.gz",
"platform": null,
"description": "## Pytest Plugin to Show Unit Coverage\n\n**Code** coverage tools like\n[coverage.py](https://coverage.readthedocs.io/en/7.0.1/) show you the\ninstrumented code coverage of your tests, however it won't tell you if you've\nwritten specific unit tests for each of your code's **units** (here unit meaning\nfunction).\n\nThis package implements a mechanism for measuring and reporting unit coverage.\nInstead of instrumenting your code you will need to mark tests with a\n**pointer** to the unit that it is covering.\n\nFor example if you have in your code this module `mypackage/widget.py`:\n\n``` python\ndef foo(in):\n return in * 3\n```\n\nthen in your test suite you would write a unit test for this function and mark it as relating to that unit, e.g. in `tests/test_widget.py`:\n\n``` python\n\nfrom mypackage.widget import foo\n\n@pytest.mark.pointer(foo)\ndef test_foo():\n assert foo(3) == 9\n```\n\nThis package works by collecting all of the pointed-to units during test\nexecution and persists these to the pytest cache (typically somewhere under\n`.pytest_cache`). Then in subsequent runs you need only report the results.\n\n### Invocation\n\nThis package adds a couple new options to the `pytest` CLI:\n\n`--pointers-collect=STR` (default `src`)\n\nThis explicitly indicates to collect unit coverage results. If not specified,\nbut `--pointers-report` is given results will be collected using the default.\n\n`--pointers-report` (default `False`)\n\nWhen this flag is given a textual report will be given at the end of the test\nrun. Note that even if this is not given the coverage checks will still be run.\n\n`--pointers-func-min-pass=INT` (default `2`)\n\nThis flag controls the number of unit test pointer marks are needed to get a\n\"passing\" unit. In the report units with 0 pointers are shown as red, passing\nnumbers are green, and anything in between is blue.\n\n`--pointers-fail-under=FLOAT` (default `0.0`)\n\nThis flag controls the percentage of passing units are needed for the entire\ncoverage check to pass. The percentage is always displayed even without\n`--pointers-report`. If this test is failed then the test process exits with\ncode 1, which is useful for things like CI.\n\n#### Example\n\nHere is an example with source code under the `src` folder, requiring 1 pointer\ntest per collected unit in the code, for all functions.\n\n```\npytest --pointers-report --pointers-collect=src --pointers-func-min-pass=1 --pointers-fail-under=100 tests\n```\n\n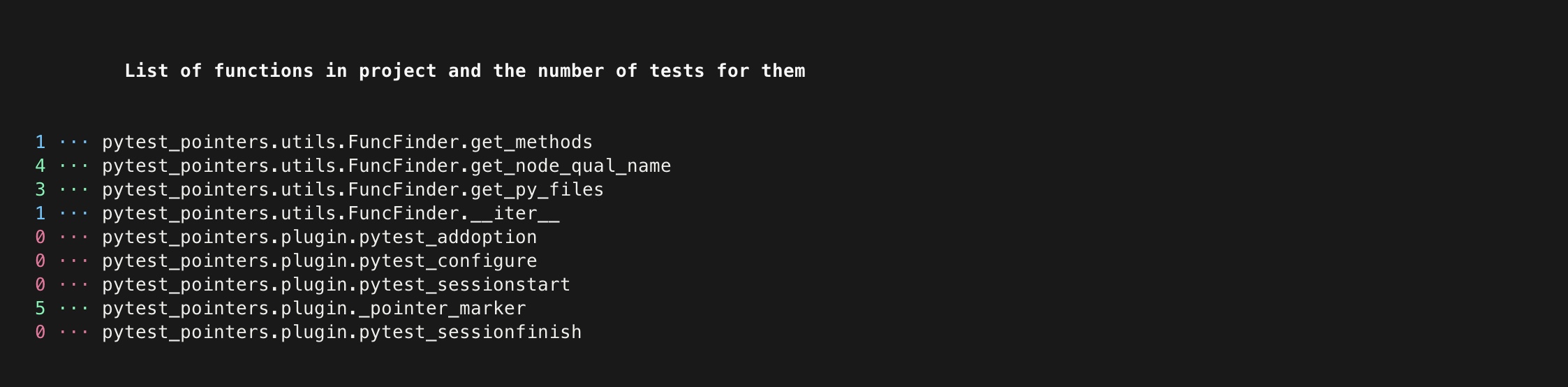\n\n### Installation\n\n``` shell\npip install pytest_pointers\n```\n\n\n\n",
"bugtrack_url": null,
"license": "",
"summary": "Pytest plugin to define functions you test with special marks for better navigation and reports",
"version": "0.3.1",
"split_keywords": [
"plugin",
"pytest"
],
"urls": [
{
"comment_text": "",
"digests": {
"md5": "579dd227ca2e6c8393eed27e94d57896",
"sha256": "27d37ccd2b78862805142c693e39e43a1b4dbb12b8e4ef9b9baaad599dc10aba"
},
"downloads": -1,
"filename": "pytest_pointers-0.3.1-py3-none-any.whl",
"has_sig": false,
"md5_digest": "579dd227ca2e6c8393eed27e94d57896",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.7,<4.0",
"size": 8701,
"upload_time": "2022-12-26T16:40:27",
"upload_time_iso_8601": "2022-12-26T16:40:27.220255Z",
"url": "https://files.pythonhosted.org/packages/e4/ef/73d1bec20a39a5b519fe1bcc3a2797dc10b31ea2701c9260bf9d294bd55c/pytest_pointers-0.3.1-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"md5": "0ef1b813a96ef56bbb295c835d8e699e",
"sha256": "1fd3ad896ce344bbac58c32b4fffda6533f84869e6d2b1b4049a92a3184d71c4"
},
"downloads": -1,
"filename": "pytest-pointers-0.3.1.tar.gz",
"has_sig": false,
"md5_digest": "0ef1b813a96ef56bbb295c835d8e699e",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.7,<4.0",
"size": 8451,
"upload_time": "2022-12-26T16:40:25",
"upload_time_iso_8601": "2022-12-26T16:40:25.410141Z",
"url": "https://files.pythonhosted.org/packages/08/f1/d87614d1dd90394ac66a938aca7b4dd19f6b29a9bc47f44726fe11fe6bf1/pytest-pointers-0.3.1.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2022-12-26 16:40:25",
"github": true,
"gitlab": false,
"bitbucket": false,
"github_user": "jaklimoff",
"github_project": "pytest-pointers",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "pytest-pointers"
}