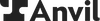
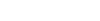
# Anvil Encryption
[](https://pypi.org/project/python-anvil-encryption)
[](https://pypi.org/project/python-anvil-encryption)
This library is a small wrapper around the [`pypa/cryptography` library](https://cryptography.io/en/latest/).
It offers convenience methods for encrypting and decrypting arbitrary payloads in both AES and RSA.
For use encrypting / decrypting payloads in Anvil, you can generate an RSA keypair from
your [organization's settings page](https://www.useanvil.com/docs/api/getting-started#encryption).
[Anvil](www.useanvil.com/developers) provides easy APIs for all things paperwork.
1. [PDF filling API](https://www.useanvil.com/products/pdf-filling-api/) - fill out a PDF template with a web request
and structured JSON data.
2. [PDF generation API](https://www.useanvil.com/products/pdf-generation-api/) - send markdown or HTML and Anvil will
render it to a PDF.
3. [Etch E-sign with API](https://www.useanvil.com/products/etch/) - customizable, embeddable, e-signature platform with
an API to control the signing process end-to-end.
4. [Anvil Workflows (w/ API)](https://www.useanvil.com/products/workflows/) - Webforms + PDF + E-sign with a powerful
no-code builder. Easily collect structured data, generate PDFs, and request signatures.
Learn more about Anvil on our [Anvil developer page](https://www.useanvil.com/developers).
## Setup
### Requirements
* Python 3.7+
### Installation
Install it directly into an activated virtual environment:
```text
$ pip install python-anvil-encryption
```
or add it to your [Poetry](https://poetry.eustace.io/) project:
```text
$ poetry add python-anvil-encryption
```
## Usage
This usage example is also in a runnable form in the `examples/` directory.
```python
import os
from python_anvil_encryption import encryption
CURRENT_PATH = os.path.dirname(os.path.realpath(__file__))
# Keys could be read from a file (or preferably from environment variables)
public_key = None
private_key = None
with open(os.path.join(CURRENT_PATH, "keys/public.pub"), "rb") as pub_file:
public_key = pub_file.read()
with open(os.path.join(CURRENT_PATH, "./keys/private.pem"), "rb") as priv_file:
private_key = priv_file.read()
# RSA
message = b"Super secret message"
encrypted_message = encryption.encrypt_rsa(public_key, message)
decrypted_message = encryption.decrypt_rsa(private_key, encrypted_message)
assert decrypted_message == message
print(f"Are equal? {decrypted_message == message}")
# AES
aes_key = encryption.generate_aes_key()
aes_encrypted_message = encryption.encrypt_aes(aes_key, message)
# The aes key in the first parameter is required to be in a hex
# byte string format.
decrypted_message = encryption.decrypt_aes(
aes_key.hex().encode(),
aes_encrypted_message
)
assert decrypted_message == message
print(f"Are equal? {decrypted_message == message}")
```
Raw data
{
"_id": null,
"home_page": "https://pypi.org/project/python-anvil-encryption",
"name": "python-anvil-encryption",
"maintainer": "",
"docs_url": null,
"requires_python": ">=3.7.2,<3.12",
"maintainer_email": "",
"keywords": "anvil,api,pdf,signing,encryption",
"author": "Anvil Foundry Inc.",
"author_email": "developers@useanvil.com",
"download_url": "https://files.pythonhosted.org/packages/62/38/e5759a23d0104f5aa9e3e63d4ce03cf5cb2d77258bd016fef94cbd87c007/python_anvil_encryption-0.1.tar.gz",
"platform": null,
"description": "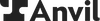\n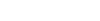\n\n# Anvil Encryption\n\n[](https://pypi.org/project/python-anvil-encryption)\n[](https://pypi.org/project/python-anvil-encryption)\n\nThis library is a small wrapper around the [`pypa/cryptography` library](https://cryptography.io/en/latest/).\nIt offers convenience methods for encrypting and decrypting arbitrary payloads in both AES and RSA.\n\nFor use encrypting / decrypting payloads in Anvil, you can generate an RSA keypair from\nyour [organization's settings page](https://www.useanvil.com/docs/api/getting-started#encryption).\n\n[Anvil](www.useanvil.com/developers) provides easy APIs for all things paperwork.\n\n1. [PDF filling API](https://www.useanvil.com/products/pdf-filling-api/) - fill out a PDF template with a web request\n and structured JSON data.\n2. [PDF generation API](https://www.useanvil.com/products/pdf-generation-api/) - send markdown or HTML and Anvil will\n render it to a PDF.\n3. [Etch E-sign with API](https://www.useanvil.com/products/etch/) - customizable, embeddable, e-signature platform with\n an API to control the signing process end-to-end.\n4. [Anvil Workflows (w/ API)](https://www.useanvil.com/products/workflows/) - Webforms + PDF + E-sign with a powerful\n no-code builder. Easily collect structured data, generate PDFs, and request signatures.\n\nLearn more about Anvil on our [Anvil developer page](https://www.useanvil.com/developers).\n\n## Setup\n\n### Requirements\n\n* Python 3.7+\n\n### Installation\n\nInstall it directly into an activated virtual environment:\n\n```text\n$ pip install python-anvil-encryption\n```\n\nor add it to your [Poetry](https://poetry.eustace.io/) project:\n\n```text\n$ poetry add python-anvil-encryption\n```\n\n## Usage\n\nThis usage example is also in a runnable form in the `examples/` directory.\n\n```python\nimport os\nfrom python_anvil_encryption import encryption\n\nCURRENT_PATH = os.path.dirname(os.path.realpath(__file__))\n\n# Keys could be read from a file (or preferably from environment variables)\npublic_key = None\nprivate_key = None\nwith open(os.path.join(CURRENT_PATH, \"keys/public.pub\"), \"rb\") as pub_file:\n public_key = pub_file.read()\n\nwith open(os.path.join(CURRENT_PATH, \"./keys/private.pem\"), \"rb\") as priv_file:\n private_key = priv_file.read()\n\n# RSA\nmessage = b\"Super secret message\"\nencrypted_message = encryption.encrypt_rsa(public_key, message)\ndecrypted_message = encryption.decrypt_rsa(private_key, encrypted_message)\nassert decrypted_message == message\nprint(f\"Are equal? {decrypted_message == message}\")\n\n# AES\naes_key = encryption.generate_aes_key()\naes_encrypted_message = encryption.encrypt_aes(aes_key, message)\n# The aes key in the first parameter is required to be in a hex\n# byte string format.\ndecrypted_message = encryption.decrypt_aes(\n aes_key.hex().encode(),\n aes_encrypted_message\n)\nassert decrypted_message == message\nprint(f\"Are equal? {decrypted_message == message}\")\n```\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "Encrypt and decrypt payloads in Anvil (https://www.useanvil.com/)",
"version": "0.1",
"project_urls": {
"Documentation": "https://python-anvil-encryption.readthedocs.io",
"Homepage": "https://pypi.org/project/python-anvil-encryption",
"Repository": "https://github.com/anvilco/python-anvil-encryption"
},
"split_keywords": [
"anvil",
"api",
"pdf",
"signing",
"encryption"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "108ff82755c45072ddd280cfa737e114fd99936b2db5daf356e9918eb78f0743",
"md5": "427c61e9a6e31d5d5e693aefd8218ae8",
"sha256": "55382d4d00b91ac0fb7716add07880b46d920fbdd5ada3a563fa45b0c06c0c80"
},
"downloads": -1,
"filename": "python_anvil_encryption-0.1-py3-none-any.whl",
"has_sig": false,
"md5_digest": "427c61e9a6e31d5d5e693aefd8218ae8",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.7.2,<3.12",
"size": 21192,
"upload_time": "2023-09-01T20:27:23",
"upload_time_iso_8601": "2023-09-01T20:27:23.669787Z",
"url": "https://files.pythonhosted.org/packages/10/8f/f82755c45072ddd280cfa737e114fd99936b2db5daf356e9918eb78f0743/python_anvil_encryption-0.1-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "6238e5759a23d0104f5aa9e3e63d4ce03cf5cb2d77258bd016fef94cbd87c007",
"md5": "2f71ffea7aae465c5e5aa9d7314bc6f9",
"sha256": "565c3feb44d93fb6cf96c0363ded997bad23ee433219270ad67ff576b9a1b9b1"
},
"downloads": -1,
"filename": "python_anvil_encryption-0.1.tar.gz",
"has_sig": false,
"md5_digest": "2f71ffea7aae465c5e5aa9d7314bc6f9",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.7.2,<3.12",
"size": 21313,
"upload_time": "2023-09-01T20:27:25",
"upload_time_iso_8601": "2023-09-01T20:27:25.051109Z",
"url": "https://files.pythonhosted.org/packages/62/38/e5759a23d0104f5aa9e3e63d4ce03cf5cb2d77258bd016fef94cbd87c007/python_anvil_encryption-0.1.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-09-01 20:27:25",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "anvilco",
"github_project": "python-anvil-encryption",
"travis_ci": false,
"coveralls": true,
"github_actions": true,
"appveyor": true,
"lcname": "python-anvil-encryption"
}