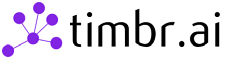
[](https://app.fossa.com/projects/custom%2B50508%2Fgithub.com%2FWPSemantix%2Ftimbr_python_SQLAlchemy?ref=badge_shield&issueType=license)
[](https://app.fossa.com/projects/custom%2B50508%2Fgithub.com%2FWPSemantix%2Ftimbr_python_SQLAlchemy?ref=badge_shield&issueType=security)
[](https://www.python.org/downloads/release/python-3713/)
[](https://www.python.org/downloads/release/python-3820/)
[](https://www.python.org/downloads/release/python-3921/)
[](https://badge.fury.io/py/pytimbr-sqla)
# timbr Python connector using SQLAlchemy
This project is a python connector to timbr using SQLAlchemy.
## Dependencies
- Access to a timbr-server
- Python from 3.7.13 or newer
- Support SQLAlchemy 1.4.36 or newer but not version 2.x yet.
- For <b>Linux</b> based machines only install those dependencies first:
- gcc
- heimdal-dev
- krb5
- python-devel
- python-dev
- python-all-dev
- libsasl2-dev
- Ubuntu example:
- apt install gcc, heimdal-dev, krb5, python-devel, python-dev, python-all-dev, libsasl2-dev
## Installation
- Install as clone repository:
- Install Python: https://www.python.org/downloads/release/python-3713/
- Run the following command to install the Python dependencies: `pip install -r requirements.txt`
- Install using pip and git:
- `pip install git+https://github.com/WPSemantix/timbr_python_SQLAlchemy`
- Install using pip:
- `pip install pytimbr-sqla`
## Known issues
If you encounter a problem installing `PyHive` with sasl dependencies on windows, install the following wheel (for 64bit Windows) by running:
`pip install https://download.lfd.uci.edu/pythonlibs/archived/cp37/sasl-0.3.1-cp37-cp37m-win_amd64.whl`
For Python 3.9:
`pip install https://download.lfd.uci.edu/pythonlibs/archived/sasl-0.3.1-cp39-cp39-win_amd64.whl`
## Sample usage
- For an example of how to use the Python SQLAlchemy connector for timbr, follow this [example file](examples/example.py)
- For an example of how to use the Python SQLAlchemy connector with 'PyHive' as async query for timbr, follow this [example file](examples/pyhive_async_example.py)
- For an example of how to use the Python SQLAlchemy connector with 'PyHive' as sync query for timbr, follow this [example file](examples/pyhive_sync_example.py)
## Connection parameters
### General example
```python
hostname = '<TIMBR_IP/HOST>'
port = '<TIMBR_PORT>'
ontology = '<ONTOLOGY_NAME>'
protocol = '<http/https>'
username = '<TIMBR_USER/token>'
password = '<TIMBR_PASSWORD/TOKEN_VALUE>'
# hostname - The IP / Hostname of the Timbr server (not necessarily the hostname of the Timbr platform).
# port - The port to connect to in the Timbr server. Timbr's default port with enabled_ssl is 443 without SSL is 11000.
# ontology = The name of the ontology (knowledge graph) to connect.
# protocol - Connection protocol can be 'http' or 'https'.
# username - Use 'token' as the username when connecting using a Timbr token, otherwise use the user name.
# password - If using a token as a username then the pass is the token value, otherwise its the user's password.
```
### HTTP example with dummy data
#### Username and password
```python
hostname = 'mytimbrenv.com'
port = '11000'
ontology = 'my_ontology'
protocol = 'http'
username = 'timbr'
password = 'StrongPassword'
```
#### Timbr token
```python
hostname = 'mytimbrenv.com'
port = '11000'
ontology = 'my_ontology'
protocol = 'http'
username = 'token'
password = '<TOKEN_VALUE>'
```
### HTTPS example with dummy data
#### Username and password
```python
hostname = 'mytimbrenv.com'
port = '443'
ontology = 'my_ontology'
protocol = 'https'
username = 'timbr'
password = 'StrongPassword'
```
#### Timbr token
```python
hostname = 'mytimbrenv.com'
port = '443'
ontology = 'my_ontology'
protocol = 'https'
username = 'token'
password = '<TOKEN_VALUE>'
```
## Connect options
### Connect using 'pytimbr_sqla' and 'SQLAlchemy' packages
```python
from sqlalchemy import create_engine
# Declare the connection variables
# General example
hostname = '<TIMBR_IP/HOST>'
port = '<TIMBR_PORT>'
ontology = '<ONTOLOGY_NAME>'
protocol = '<http/https>'
username = '<TIMBR_USER/token>'
password = '<TIMBR_PASSWORD/TOKEN_VALUE>'
# hostname - The IP / Hostname of the Timbr server (not necessarily the hostname of the Timbr platform).
# port - The port to connect to in the Timbr server. Timbr's default port with enabled_ssl is 443 without SSL is 11000.
# ontology = The name of the ontology (knowledge graph) to connect.
# protocol - Connection protocol can be 'http' or 'https'.
# username - Use 'token' as the username when connecting using a Timbr token, otherwise use the user name.
# password - If using a token as a username then the pass is the token value, otherwise its the user's password.
# Create new sqlalchemy connection
engine = create_engine(f"timbr+{protocol}://{username}@{ontology}:{password}@{hostname}:{port}")
# Connect to the created engine
conn = engine.connect()
# Execute a query
query = "SHOW CONCEPTS"
res_obj = conn.execute(query)
results_headers = [(desc[0], desc[1]) for desc in res_obj.cursor.description]
results = res_obj.fetchall()
# Print the columns name
for name, col_type in results_headers:
print(f"{name} - {col_type}")
# Print the results
for result in results:
print(result)
```
### Attention:
### timbr works only as async when running a query, if you want to use standard PyHive you have two options
### Connect using 'PyHive' and 'SQLAlchemy' packages
#### Connect using PyHive Async Query
```python
from sqlalchemy import create_engine
from TCLIService.ttypes import TOperationState
# Declare the connection variables
# General example
hostname = '<TIMBR_IP/HOST>'
port = '<TIMBR_PORT>'
ontology = '<ONTOLOGY_NAME>'
protocol = '<http/https>'
username = '<TIMBR_USER/token>'
password = '<TIMBR_PASSWORD/TOKEN_VALUE>'
connect_args = {
'configuration': {
'set:hiveconf:hiveMetadata': 'true',
'set:hiveconf:active_datasource': '<datasource_name>',
'set:hiveconf:queryTimeout': '<TIMEOUT_IN_SECONDS>',
},
}
# hostname - The IP / Hostname of the Timbr server (not necessarily the hostname of the Timbr platform).
# port - The port to connect to in the Timbr server. Timbr's default port with enabled_ssl is 443 without SSL is 11000.
# ontology = The name of the ontology (knowledge graph) to connect.
# protocol - Connection protocol can be 'http' or 'https'.
# username - Use 'token' as the username when connecting using a Timbr token, otherwise use the user name.
# password - If using a token as a username then the pass is the token value, otherwise its the user's password.
# connect_args - The connection special arguments for extra customization. The only argument you must have is the first one (set:hiveconf:hiveMetadata) the others are optional.
# Create new sqlalchemy connection
engine = create_engine(f"hive+{protocol}://{username}@{ontology}:{password}@{hostname}:{port}", connect_args = connect_args)
# Connect to the created engine
conn = engine.connect()
dbapi_conn = engine.raw_connection()
cursor = dbapi_conn.cursor()
# Execute a query
query = "SHOW CONCEPTS"
cursor.execute(query)
# Check the status of this execution
status = cursor.poll().operationState
while status in (TOperationState.INITIALIZED_STATE, TOperationState.RUNNING_STATE):
status = cursor.poll().operationState
# Get the results of the execution
results_headers = [(desc[0], desc[1]) for desc in cursor.description]
results = cursor.fetchall()
# Display the results of the execution
# Print the columns name
for name, col_type in results_headers:
print(f"{name} - {col_type}")
# Print the results
for result in results:
print(result)
```
#### Connect using PyHive Sync Query
```python
from sqlalchemy import create_engine
from TCLIService.ttypes import TOperationState
# Declare the connection variables
# General example
hostname = '<TIMBR_IP/HOST>'
port = '<TIMBR_PORT>'
ontology = '<ONTOLOGY_NAME>'
protocol = '<http/https>'
username = '<TIMBR_USER/token>'
password = '<TIMBR_PASSWORD/TOKEN_VALUE>'
connect_args = {
'configuration': {
'set:hiveconf:async': 'false',
'set:hiveconf:hiveMetadata': 'true',
'set:hiveconf:active_datasource': '<datasource_name>',
'set:hiveconf:queryTimeout': '<TIMEOUT_IN_SECONDS>',
},
}
# hostname - The IP / Hostname of the Timbr server (not necessarily the hostname of the Timbr platform).
# port - The port to connect to in the Timbr server. Timbr's default port with enabled_ssl is 443 without SSL is 11000.
# ontology = The name of the ontology (knowledge graph) to connect.
# protocol - Connection protocol can be 'http' or 'https'.
# username - Use 'token' as the username when connecting using a Timbr token, otherwise use the user name.
# password - If using a token as a username then the pass is the token value, otherwise its the user's password.
# connect_args - The connection special arguments for extra customization. The only 2 arguments you must have are the first and the second one (set:hiveconf:async, set:hiveconf:hiveMetadata) the others are optional.
# Create new sqlalchemy connection
engine = create_engine(f"hive+{protocol}://{username}@{ontology}:{password}@{hostname}:{port}", connect_args = connect_args)
# Connect to the created engine
conn = engine.connect()
# Execute a query
query = "SHOW CONCEPTS"
res_obj = conn.execute(query)
results_headers = [(desc[0], desc[1]) for desc in res_obj.cursor.description]
results = res_obj.fetchall()
# Print the columns name
for name, col_type in results_headers:
print(f"{name} - {col_type}")
# Print the results
for result in results:
print(result)
```
Raw data
{
"_id": null,
"home_page": "https://github.com/WPSemantix/timbr_python_SQLAlchemy",
"name": "pytimbr-sqla",
"maintainer": null,
"docs_url": null,
"requires_python": null,
"maintainer_email": null,
"keywords": "timbr, timbr-python, timbr-connector, python-connector, PyTimbr, pytimbr, py-timbr, Py-Timbr, pytimbr_sqla, pytimbr_Sqla, PyTimbr_Sqla, pytimbr_SQla, PyTimbr_SQla, pytimbr_SQLa, PyTimbr_SQLa, pytimbr_SQlA, PyTimbr_SQLA, pytimbrsqlalchemy, PyTimbrSqlalchemy, PyTimbrSQlalchemy, PyTimbrSQLalchemy, PyTimbrSQLAlchemy, Py-TimbrSQLAlchemy, py-timbrsqlalchemy, Py-Timbr-SQLAlchemy, py-timbr-sqlalchemy",
"author": "timbr",
"author_email": "contact@timbr.ai",
"download_url": "https://files.pythonhosted.org/packages/bd/a9/7da9f41bfcbde0b56c89d6deadc2fd5b6de6566331b5120c5fa1c5060682/pytimbr_sqla-1.0.6.tar.gz",
"platform": null,
"description": "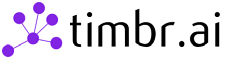\r\n\r\n[](https://app.fossa.com/projects/custom%2B50508%2Fgithub.com%2FWPSemantix%2Ftimbr_python_SQLAlchemy?ref=badge_shield&issueType=license)\r\n[](https://app.fossa.com/projects/custom%2B50508%2Fgithub.com%2FWPSemantix%2Ftimbr_python_SQLAlchemy?ref=badge_shield&issueType=security)\r\n\r\n[](https://www.python.org/downloads/release/python-3713/)\r\n[](https://www.python.org/downloads/release/python-3820/)\r\n[](https://www.python.org/downloads/release/python-3921/)\r\n\r\n[](https://badge.fury.io/py/pytimbr-sqla)\r\n\r\n# timbr Python connector using SQLAlchemy\r\nThis project is a python connector to timbr using SQLAlchemy.\r\n\r\n## Dependencies\r\n- Access to a timbr-server\r\n- Python from 3.7.13 or newer\r\n- Support SQLAlchemy 1.4.36 or newer but not version 2.x yet.\r\n- For <b>Linux</b> based machines only install those dependencies first:\r\n - gcc\r\n - heimdal-dev\r\n - krb5\r\n - python-devel\r\n - python-dev\r\n - python-all-dev\r\n - libsasl2-dev\r\n- Ubuntu example:\r\n - apt install gcc, heimdal-dev, krb5, python-devel, python-dev, python-all-dev, libsasl2-dev\r\n\r\n\r\n## Installation\r\n- Install as clone repository:\r\n - Install Python: https://www.python.org/downloads/release/python-3713/\r\n - Run the following command to install the Python dependencies: `pip install -r requirements.txt`\r\n\r\n- Install using pip and git:\r\n - `pip install git+https://github.com/WPSemantix/timbr_python_SQLAlchemy`\r\n\r\n- Install using pip:\r\n - `pip install pytimbr-sqla`\r\n\r\n## Known issues\r\nIf you encounter a problem installing `PyHive` with sasl dependencies on windows, install the following wheel (for 64bit Windows) by running:\r\n\r\n`pip install https://download.lfd.uci.edu/pythonlibs/archived/cp37/sasl-0.3.1-cp37-cp37m-win_amd64.whl`\r\n\r\nFor Python 3.9:\r\n\r\n`pip install https://download.lfd.uci.edu/pythonlibs/archived/sasl-0.3.1-cp39-cp39-win_amd64.whl`\r\n\r\n## Sample usage\r\n- For an example of how to use the Python SQLAlchemy connector for timbr, follow this [example file](examples/example.py)\r\n- For an example of how to use the Python SQLAlchemy connector with 'PyHive' as async query for timbr, follow this [example file](examples/pyhive_async_example.py)\r\n- For an example of how to use the Python SQLAlchemy connector with 'PyHive' as sync query for timbr, follow this [example file](examples/pyhive_sync_example.py)\r\n\r\n## Connection parameters\r\n\r\n### General example\r\n```python\r\n hostname = '<TIMBR_IP/HOST>'\r\n port = '<TIMBR_PORT>'\r\n ontology = '<ONTOLOGY_NAME>'\r\n protocol = '<http/https>'\r\n username = '<TIMBR_USER/token>'\r\n password = '<TIMBR_PASSWORD/TOKEN_VALUE>'\r\n\r\n # hostname - The IP / Hostname of the Timbr server (not necessarily the hostname of the Timbr platform).\r\n # port - The port to connect to in the Timbr server. Timbr's default port with enabled_ssl is 443 without SSL is 11000.\r\n # ontology = The name of the ontology (knowledge graph) to connect.\r\n # protocol - Connection protocol can be 'http' or 'https'.\r\n # username - Use 'token' as the username when connecting using a Timbr token, otherwise use the user name.\r\n # password - If using a token as a username then the pass is the token value, otherwise its the user's password.\r\n```\r\n\r\n### HTTP example with dummy data\r\n\r\n#### Username and password\r\n```python\r\n hostname = 'mytimbrenv.com'\r\n port = '11000'\r\n ontology = 'my_ontology'\r\n protocol = 'http'\r\n username = 'timbr'\r\n password = 'StrongPassword'\r\n```\r\n\r\n#### Timbr token\r\n```python\r\n hostname = 'mytimbrenv.com'\r\n port = '11000'\r\n ontology = 'my_ontology'\r\n protocol = 'http'\r\n username = 'token'\r\n password = '<TOKEN_VALUE>'\r\n```\r\n\r\n### HTTPS example with dummy data\r\n\r\n#### Username and password\r\n```python\r\n hostname = 'mytimbrenv.com'\r\n port = '443'\r\n ontology = 'my_ontology'\r\n protocol = 'https'\r\n username = 'timbr'\r\n password = 'StrongPassword'\r\n```\r\n\r\n#### Timbr token\r\n```python\r\n hostname = 'mytimbrenv.com'\r\n port = '443'\r\n ontology = 'my_ontology'\r\n protocol = 'https'\r\n username = 'token'\r\n password = '<TOKEN_VALUE>'\r\n```\r\n## Connect options\r\n\r\n### Connect using 'pytimbr_sqla' and 'SQLAlchemy' packages\r\n```python\r\n from sqlalchemy import create_engine\r\n\r\n # Declare the connection variables\r\n # General example\r\n hostname = '<TIMBR_IP/HOST>'\r\n port = '<TIMBR_PORT>'\r\n ontology = '<ONTOLOGY_NAME>'\r\n protocol = '<http/https>'\r\n username = '<TIMBR_USER/token>'\r\n password = '<TIMBR_PASSWORD/TOKEN_VALUE>'\r\n\r\n # hostname - The IP / Hostname of the Timbr server (not necessarily the hostname of the Timbr platform).\r\n # port - The port to connect to in the Timbr server. Timbr's default port with enabled_ssl is 443 without SSL is 11000.\r\n # ontology = The name of the ontology (knowledge graph) to connect.\r\n # protocol - Connection protocol can be 'http' or 'https'.\r\n # username - Use 'token' as the username when connecting using a Timbr token, otherwise use the user name.\r\n # password - If using a token as a username then the pass is the token value, otherwise its the user's password.\r\n\r\n # Create new sqlalchemy connection\r\n engine = create_engine(f\"timbr+{protocol}://{username}@{ontology}:{password}@{hostname}:{port}\")\r\n\r\n # Connect to the created engine\r\n conn = engine.connect()\r\n\r\n # Execute a query\r\n query = \"SHOW CONCEPTS\"\r\n res_obj = conn.execute(query)\r\n results_headers = [(desc[0], desc[1]) for desc in res_obj.cursor.description]\r\n results = res_obj.fetchall()\r\n\r\n # Print the columns name\r\n for name, col_type in results_headers:\r\n print(f\"{name} - {col_type}\")\r\n # Print the results\r\n for result in results:\r\n print(result)\r\n```\r\n### Attention:\r\n### timbr works only as async when running a query, if you want to use standard PyHive you have two options\r\n\r\n### Connect using 'PyHive' and 'SQLAlchemy' packages\r\n\r\n#### Connect using PyHive Async Query\r\n```python\r\n from sqlalchemy import create_engine\r\n from TCLIService.ttypes import TOperationState\r\n\r\n # Declare the connection variables\r\n # General example\r\n hostname = '<TIMBR_IP/HOST>'\r\n port = '<TIMBR_PORT>'\r\n ontology = '<ONTOLOGY_NAME>'\r\n protocol = '<http/https>'\r\n username = '<TIMBR_USER/token>'\r\n password = '<TIMBR_PASSWORD/TOKEN_VALUE>'\r\n connect_args = {\r\n 'configuration': {\r\n 'set:hiveconf:hiveMetadata': 'true',\r\n 'set:hiveconf:active_datasource': '<datasource_name>',\r\n 'set:hiveconf:queryTimeout': '<TIMEOUT_IN_SECONDS>',\r\n },\r\n }\r\n\r\n # hostname - The IP / Hostname of the Timbr server (not necessarily the hostname of the Timbr platform).\r\n # port - The port to connect to in the Timbr server. Timbr's default port with enabled_ssl is 443 without SSL is 11000.\r\n # ontology = The name of the ontology (knowledge graph) to connect.\r\n # protocol - Connection protocol can be 'http' or 'https'.\r\n # username - Use 'token' as the username when connecting using a Timbr token, otherwise use the user name.\r\n # password - If using a token as a username then the pass is the token value, otherwise its the user's password.\r\n # connect_args - The connection special arguments for extra customization. The only argument you must have is the first one (set:hiveconf:hiveMetadata) the others are optional.\r\n\r\n # Create new sqlalchemy connection\r\n engine = create_engine(f\"hive+{protocol}://{username}@{ontology}:{password}@{hostname}:{port}\", connect_args = connect_args)\r\n\r\n # Connect to the created engine\r\n conn = engine.connect()\r\n dbapi_conn = engine.raw_connection()\r\n cursor = dbapi_conn.cursor()\r\n\r\n # Execute a query\r\n query = \"SHOW CONCEPTS\"\r\n cursor.execute(query)\r\n\r\n # Check the status of this execution\r\n status = cursor.poll().operationState\r\n while status in (TOperationState.INITIALIZED_STATE, TOperationState.RUNNING_STATE):\r\n status = cursor.poll().operationState\r\n\r\n # Get the results of the execution\r\n results_headers = [(desc[0], desc[1]) for desc in cursor.description]\r\n results = cursor.fetchall()\r\n\r\n # Display the results of the execution\r\n # Print the columns name\r\n for name, col_type in results_headers:\r\n print(f\"{name} - {col_type}\")\r\n # Print the results\r\n for result in results:\r\n print(result)\r\n```\r\n\r\n#### Connect using PyHive Sync Query\r\n```python\r\n from sqlalchemy import create_engine\r\n from TCLIService.ttypes import TOperationState\r\n\r\n # Declare the connection variables\r\n # General example\r\n hostname = '<TIMBR_IP/HOST>'\r\n port = '<TIMBR_PORT>'\r\n ontology = '<ONTOLOGY_NAME>'\r\n protocol = '<http/https>'\r\n username = '<TIMBR_USER/token>'\r\n password = '<TIMBR_PASSWORD/TOKEN_VALUE>'\r\n connect_args = {\r\n 'configuration': {\r\n 'set:hiveconf:async': 'false',\r\n 'set:hiveconf:hiveMetadata': 'true',\r\n 'set:hiveconf:active_datasource': '<datasource_name>',\r\n 'set:hiveconf:queryTimeout': '<TIMEOUT_IN_SECONDS>',\r\n },\r\n }\r\n\r\n # hostname - The IP / Hostname of the Timbr server (not necessarily the hostname of the Timbr platform).\r\n # port - The port to connect to in the Timbr server. Timbr's default port with enabled_ssl is 443 without SSL is 11000.\r\n # ontology = The name of the ontology (knowledge graph) to connect.\r\n # protocol - Connection protocol can be 'http' or 'https'.\r\n # username - Use 'token' as the username when connecting using a Timbr token, otherwise use the user name.\r\n # password - If using a token as a username then the pass is the token value, otherwise its the user's password.\r\n # connect_args - The connection special arguments for extra customization. The only 2 arguments you must have are the first and the second one (set:hiveconf:async, set:hiveconf:hiveMetadata) the others are optional.\r\n\r\n # Create new sqlalchemy connection\r\n engine = create_engine(f\"hive+{protocol}://{username}@{ontology}:{password}@{hostname}:{port}\", connect_args = connect_args)\r\n\r\n # Connect to the created engine\r\n conn = engine.connect()\r\n\r\n # Execute a query\r\n query = \"SHOW CONCEPTS\"\r\n res_obj = conn.execute(query)\r\n results_headers = [(desc[0], desc[1]) for desc in res_obj.cursor.description]\r\n results = res_obj.fetchall()\r\n\r\n # Print the columns name\r\n for name, col_type in results_headers:\r\n print(f\"{name} - {col_type}\")\r\n # Print the results\r\n for result in results:\r\n print(result)\r\n```\r\n\r\n\r\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "Timbr Python SQLAlchemy connector",
"version": "1.0.6",
"project_urls": {
"Bug Tracker": "https://github.com/WPSemantix/timbr_python_SQLAlchemy/issues",
"Download": "https://github.com/WPSemantix/timbr_python_SQLAlchemy/archive/refs/tags/v1.0.6.tar.gz",
"Homepage": "https://github.com/WPSemantix/timbr_python_SQLAlchemy"
},
"split_keywords": [
"timbr",
" timbr-python",
" timbr-connector",
" python-connector",
" pytimbr",
" pytimbr",
" py-timbr",
" py-timbr",
" pytimbr_sqla",
" pytimbr_sqla",
" pytimbr_sqla",
" pytimbr_sqla",
" pytimbr_sqla",
" pytimbr_sqla",
" pytimbr_sqla",
" pytimbr_sqla",
" pytimbr_sqla",
" pytimbrsqlalchemy",
" pytimbrsqlalchemy",
" pytimbrsqlalchemy",
" pytimbrsqlalchemy",
" pytimbrsqlalchemy",
" py-timbrsqlalchemy",
" py-timbrsqlalchemy",
" py-timbr-sqlalchemy",
" py-timbr-sqlalchemy"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "bda97da9f41bfcbde0b56c89d6deadc2fd5b6de6566331b5120c5fa1c5060682",
"md5": "d602b488a1169e730c8568d90b4f5879",
"sha256": "031d09d2a55627e8c943a8ef31278ab52c2131ff8a929f584901b24bef436d62"
},
"downloads": -1,
"filename": "pytimbr_sqla-1.0.6.tar.gz",
"has_sig": false,
"md5_digest": "d602b488a1169e730c8568d90b4f5879",
"packagetype": "sdist",
"python_version": "source",
"requires_python": null,
"size": 45446,
"upload_time": "2025-01-02T19:31:29",
"upload_time_iso_8601": "2025-01-02T19:31:29.527895Z",
"url": "https://files.pythonhosted.org/packages/bd/a9/7da9f41bfcbde0b56c89d6deadc2fd5b6de6566331b5120c5fa1c5060682/pytimbr_sqla-1.0.6.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2025-01-02 19:31:29",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "WPSemantix",
"github_project": "timbr_python_SQLAlchemy",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"requirements": [],
"lcname": "pytimbr-sqla"
}