# 📦 PyTONAPI
[](https://ton.org)
[](https://pypi.python.org/pypi/pytonapi)

[](https://github.com/tonkeeper/pytonapi/blob/main/LICENSE)
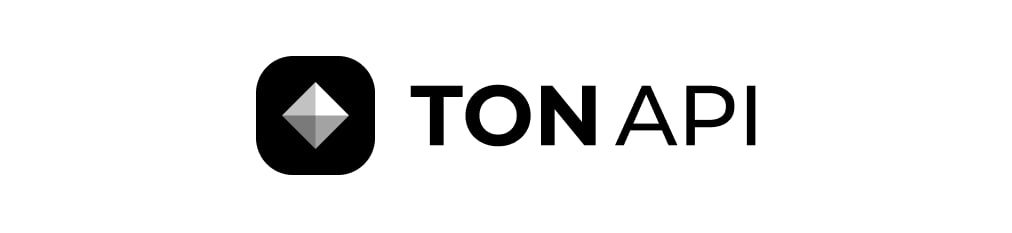



Python SDK for [tonapi.io](https://tonapi.io).\
Information about the API can be found in the [documentation](https://docs.tonconsole.com/tonapi/api-v2).\
To use the API **you need an API key**, you can get it here [tonconsole.com](https://tonconsole.com/).
<blockquote>
For creating wallets, transferring TON, Jetton, NFTs, and other operations, recommend using <a href="https://github.com/nessshon/tonutils">tonutils</a> in combination with <code>TonapiClient</code>. For more information, refer to the library documentation.
</blockquote>
## Usage
### Installation
```bash
pip install pytonapi
```
### Examples
```python
from pytonapi import AsyncTonapi
# Declare an asynchronous function for using await
async def main():
# Create a new Tonapi object with the provided API key
tonapi = AsyncTonapi(api_key="Your API key")
# Specify the account ID
account_id = "EQC-3ilVr-W0Uc3pLrGJElwSaFxvhXXfkiQA3EwdVBHNNess" # noqa
# Retrieve account information asynchronously
account = await tonapi.accounts.get_info(account_id=account_id)
# Print account details
print(f"Account Address (raw): {account.address.to_raw()}")
print(f"Account Address (userfriendly): {account.address.to_userfriendly(is_bounceable=True)}")
print(f"Account Balance (nanoton): {account.balance.to_nano()}")
print(f"Account Balance (amount): {account.balance.to_amount()}")
if __name__ == '__main__':
import asyncio
# Run the asynchronous function
asyncio.run(main())
```
* **Additional examples** can be found [examples](https://github.com/tonkeeper/pytonapi/tree/main/examples) folder.
## Donations
**TON** - `UQCDrgGaI6gWK-qlyw69xWZosurGxrpRgIgSkVsgahUtxZR0`
**USDT** (TRC-20) - `TGKmm9H3FApFw8xcgRcZDHSku68vozAjo9`
## Contribution
We welcome your contributions! If you have ideas for improvement or have identified a bug, please create an issue or
submit a pull request.
## Support
Supported by [TONAPI](https://tonapi.io) and [TON Society](https://github.com/ton-society/grants-and-bounties) (Grants
and Bounties program).
## License
This repository is distributed under the [MIT License](https://github.com/tonkeeper/pytonapi/blob/main/LICENSE). Feel
free to use, modify, and distribute the code in accordance
with the terms of the license.
Raw data
{
"_id": null,
"home_page": "https://github.com/tonkeeper/pytonapi/",
"name": "pytonapi",
"maintainer": null,
"docs_url": null,
"requires_python": null,
"maintainer_email": null,
"keywords": null,
"author": "nessshon",
"author_email": null,
"download_url": "https://files.pythonhosted.org/packages/46/cc/5e8558dd4b0d00035359036142a135527297eb71d01aca7eafb61197c4c7/pytonapi-0.4.5.tar.gz",
"platform": null,
"description": "# \ud83d\udce6 PyTONAPI\n\n[](https://ton.org)\n[](https://pypi.python.org/pypi/pytonapi)\n\n[](https://github.com/tonkeeper/pytonapi/blob/main/LICENSE)\n\n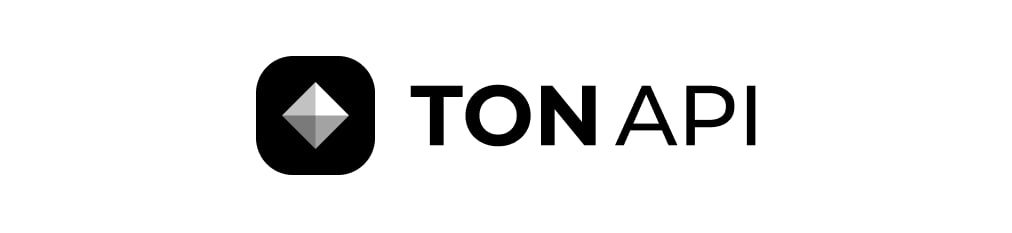\n\n\n\n\n\nPython SDK for [tonapi.io](https://tonapi.io).\\\nInformation about the API can be found in the [documentation](https://docs.tonconsole.com/tonapi/api-v2).\\\nTo use the API **you need an API key**, you can get it here [tonconsole.com](https://tonconsole.com/).\n\n<blockquote>\nFor creating wallets, transferring TON, Jetton, NFTs, and other operations, recommend using <a href=\"https://github.com/nessshon/tonutils\">tonutils</a> in combination with <code>TonapiClient</code>. For more information, refer to the library documentation.\n</blockquote>\n\n## Usage\n\n### Installation\n\n```bash\npip install pytonapi\n```\n\n### Examples\n\n\n```python\nfrom pytonapi import AsyncTonapi\n\n\n# Declare an asynchronous function for using await\nasync def main():\n # Create a new Tonapi object with the provided API key\n tonapi = AsyncTonapi(api_key=\"Your API key\")\n\n # Specify the account ID\n account_id = \"EQC-3ilVr-W0Uc3pLrGJElwSaFxvhXXfkiQA3EwdVBHNNess\" # noqa\n\n # Retrieve account information asynchronously\n account = await tonapi.accounts.get_info(account_id=account_id)\n\n # Print account details\n print(f\"Account Address (raw): {account.address.to_raw()}\")\n print(f\"Account Address (userfriendly): {account.address.to_userfriendly(is_bounceable=True)}\")\n print(f\"Account Balance (nanoton): {account.balance.to_nano()}\")\n print(f\"Account Balance (amount): {account.balance.to_amount()}\")\n\n\nif __name__ == '__main__':\n import asyncio\n\n # Run the asynchronous function\n asyncio.run(main())\n\n```\n\n* **Additional examples** can be found [examples](https://github.com/tonkeeper/pytonapi/tree/main/examples) folder.\n\n## Donations\n\n**TON** - `UQCDrgGaI6gWK-qlyw69xWZosurGxrpRgIgSkVsgahUtxZR0`\n\n**USDT** (TRC-20) - `TGKmm9H3FApFw8xcgRcZDHSku68vozAjo9`\n\n## Contribution\n\nWe welcome your contributions! If you have ideas for improvement or have identified a bug, please create an issue or\nsubmit a pull request.\n\n## Support\n\nSupported by [TONAPI](https://tonapi.io) and [TON Society](https://github.com/ton-society/grants-and-bounties) (Grants\nand Bounties program).\n\n## License\n\nThis repository is distributed under the [MIT License](https://github.com/tonkeeper/pytonapi/blob/main/LICENSE). Feel\nfree to use, modify, and distribute the code in accordance\nwith the terms of the license.\n\n",
"bugtrack_url": null,
"license": null,
"summary": "Provide access to indexed TON blockchain.",
"version": "0.4.5",
"project_urls": {
"Homepage": "https://github.com/tonkeeper/pytonapi/"
},
"split_keywords": [],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "2e1754e92652e361e172f4bf060c1d8a666f2e33f2f79cf3b385b6bc299dad0f",
"md5": "7d91482b92d5439e5e76cb0a6277ccda",
"sha256": "2d75d5b3db49fa5fd3b83bd379c5682ad9e7e80f1da4ed5e7505fa687ba95919"
},
"downloads": -1,
"filename": "pytonapi-0.4.5-py3-none-any.whl",
"has_sig": false,
"md5_digest": "7d91482b92d5439e5e76cb0a6277ccda",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": null,
"size": 45248,
"upload_time": "2024-12-19T00:39:56",
"upload_time_iso_8601": "2024-12-19T00:39:56.949175Z",
"url": "https://files.pythonhosted.org/packages/2e/17/54e92652e361e172f4bf060c1d8a666f2e33f2f79cf3b385b6bc299dad0f/pytonapi-0.4.5-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "46cc5e8558dd4b0d00035359036142a135527297eb71d01aca7eafb61197c4c7",
"md5": "7205d665da0174cbedb69944b7ec7b80",
"sha256": "9d45a57077dd66ace92f6465b4556886daa6692a5c8845902cd403c6aeb30c5c"
},
"downloads": -1,
"filename": "pytonapi-0.4.5.tar.gz",
"has_sig": false,
"md5_digest": "7205d665da0174cbedb69944b7ec7b80",
"packagetype": "sdist",
"python_version": "source",
"requires_python": null,
"size": 34757,
"upload_time": "2024-12-19T00:39:59",
"upload_time_iso_8601": "2024-12-19T00:39:59.989985Z",
"url": "https://files.pythonhosted.org/packages/46/cc/5e8558dd4b0d00035359036142a135527297eb71d01aca7eafb61197c4c7/pytonapi-0.4.5.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-12-19 00:39:59",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "tonkeeper",
"github_project": "pytonapi",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"requirements": [
{
"name": "aiohttp",
"specs": [
[
"~=",
"3.11.11"
]
]
},
{
"name": "pydantic",
"specs": [
[
"~=",
"2.10.4"
]
]
}
],
"lcname": "pytonapi"
}