# PyVista xarray
[](https://pypi.org/project/pyvista-xarray/)
[](https://codecov.io/gh/pyvista/pyvista-xarray)
[](https://mybinder.org/v2/gh/pyvista/pyvista-xarray/HEAD)
xarray DataArray accessors for PyVista to visualize datasets in 3D
## 🚀 Usage
You must `import pvxarray` in order to register the `DataArray` accessor with
xarray. After which, a `pyvista` namespace of accessors will be available.
Try on MyBinder: https://mybinder.org/v2/gh/pyvista/pyvista-xarray/HEAD
The following is an example to visualize a `RectilinearGrid` with PyVista:
```py
import pvxarray
import xarray as xr
ds = xr.tutorial.load_dataset("air_temperature")
da = ds.air[dict(time=0)] # Select DataArray for a timestep
# Plot in 3D
da.pyvista.plot(x="lon", y="lat", show_edges=True, cpos='xy')
# Or grab the mesh object for use with PyVista
mesh = da.pyvista.mesh(x="lon", y="lat")
```
<!-- notebook=0, off_screen=1, screenshot='imgs/air_temperature.png' -->
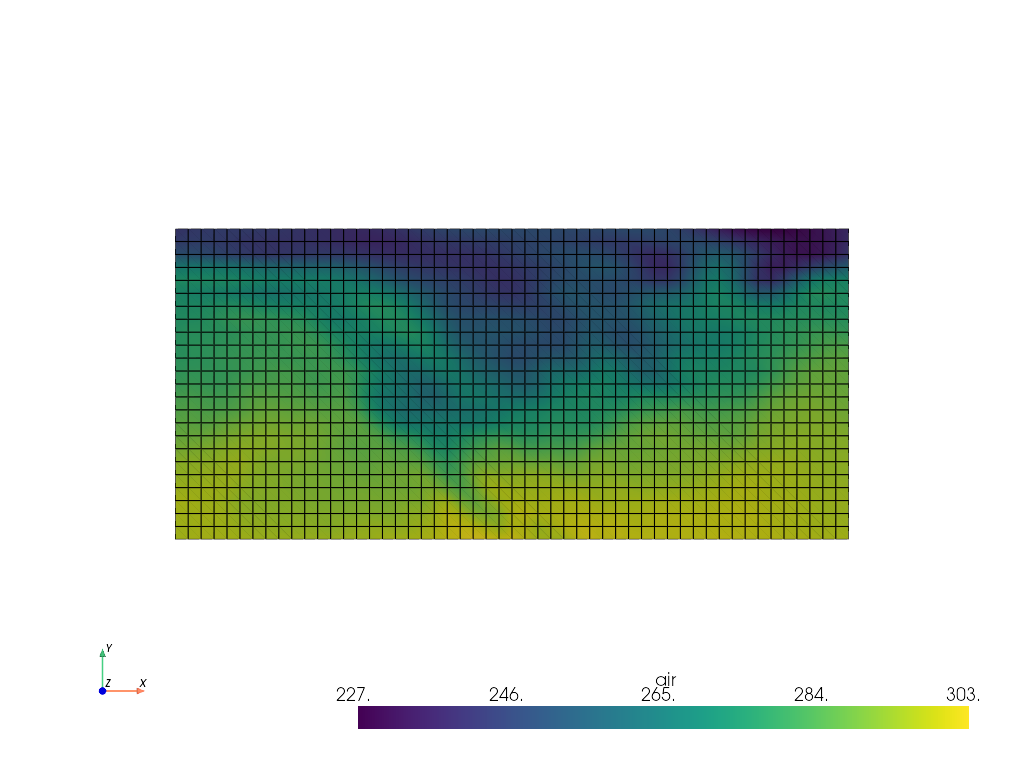
Or you can read VTK meshes directly to xarray:
```py
import xarray as xr
ds = xr.open_dataset("data.vtk", engine="pyvista")
ds["data array"].pyvista.plot(x="x", y="y", z="z")
```
## ⬇️ Installation
### 🐍 Installing with `conda`
Conda makes managing `pyvista-xarray`'s dependencies across platforms quite
easy and this is the recommended method to install:
```bash
conda install -c conda-forge pyvista-xarray
```
### 🎡 Installing with `pip`
If you prefer pip, then you can install from PyPI: https://pypi.org/project/pyvista-xarray/
```bash
pip install pyvista-xarray
```
### Upstream Work
Many of the examples leverage brand new features in PyVista `v0.38.1` and
GeoVista which may not yet be released when you're reading this. Here is a list
of pull requests needed for some of the examples:
- GeoVista algorithm support: https://github.com/bjlittle/geovista/pull/127
Work that was required and merged:
- https://github.com/pyvista/pyvista/pull/2698
- https://github.com/pyvista/pyvista/pull/2697
- https://github.com/pyvista/pyvista/pull/3318
- https://github.com/pyvista/pyvista/pull/3556
- https://github.com/pyvista/pyvista/pull/3385
## 💭 Feedback
Please share your thoughts and questions on the Discussions board. If you would
like to report any bugs or make feature requests, please open an issue.
If filing a bug report, please share a scooby Report:
```py
import pvxarray
print(pvxarray.Report())
```
## 🏏 Further Examples
The following are a few simple examples taken from the xarray and
rioxarray documentation. There are also more sophisticated examples
in the `examples/` directory in this repository.
### Simple RectilinearGrid
```py
import numpy as np
import pvxarray
import xarray as xr
lon = np.array([-99.83, -99.32])
lat = np.array([42.25, 42.21])
z = np.array([0, 10])
temp = 15 + 8 * np.random.randn(2, 2, 2)
ds = xr.Dataset(
{
"temperature": (["z", "x", "y"], temp),
},
coords={
"lon": (["x"], lon),
"lat": (["y"], lat),
"z": (["z"], z),
},
)
mesh = ds.temperature.pyvista.mesh(x="lon", y="lat", z="z")
mesh.plot()
```
## Raster with rioxarray
```py
import pvxarray
import rioxarray
import xarray as xr
da = rioxarray.open_rasterio("TC_NG_SFBay_US_Geo_COG.tif")
da = da.rio.reproject("EPSG:3857")
# Grab the mesh object for use with PyVista
mesh = da.pyvista.mesh(x="x", y="y", component="band")
mesh.plot(scalars="data", cpos='xy', rgb=True)
```
<!-- notebook=0, off_screen=1, screenshot='imgs/raster.png' -->
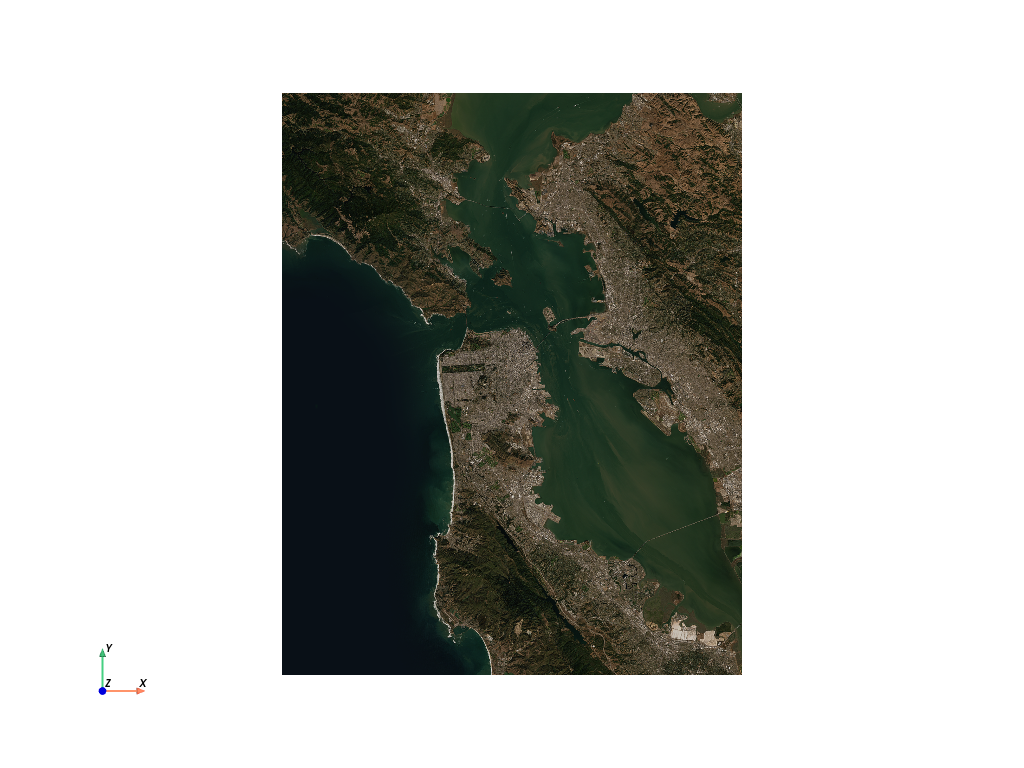
```py
import pvxarray
import rioxarray
da = rioxarray.open_rasterio("Elevation.tif")
da = da.rio.reproject("EPSG:3857")
# Grab the mesh object for use with PyVista
mesh = da.pyvista.mesh(x="x", y="y")
# Warp top and plot in 3D
mesh.warp_by_scalar().plot()
```
<!-- notebook=0, off_screen=1, screenshot='imgs/topo.png' -->
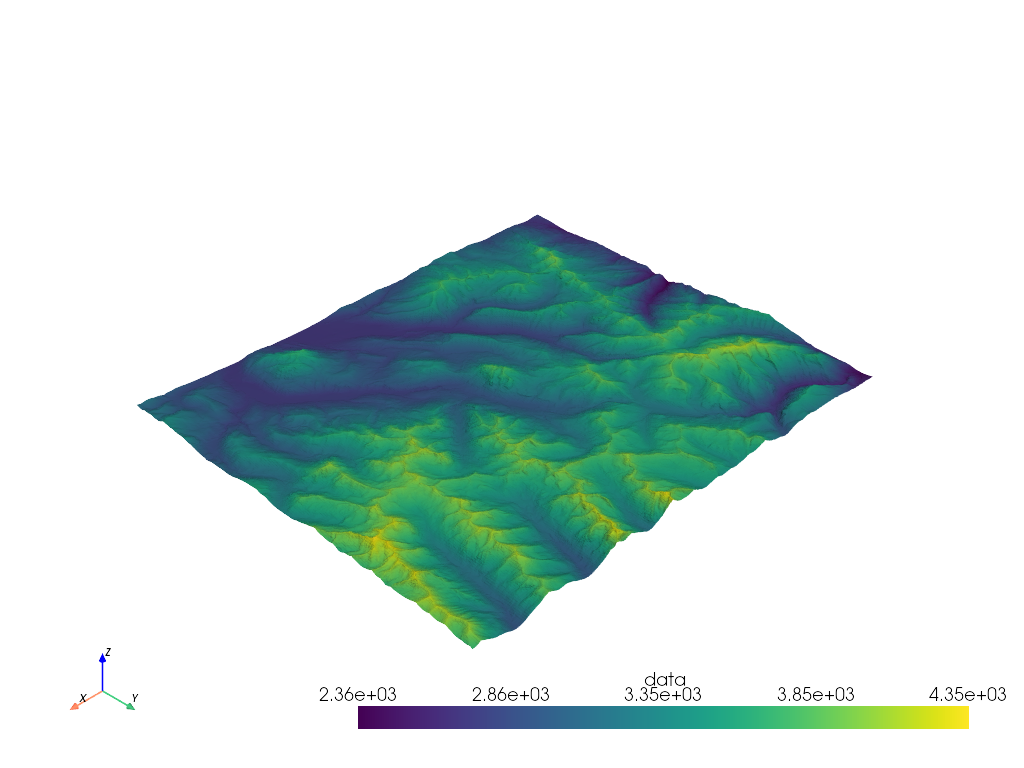
## StructuredGrid
```py
import pvxarray
import pyvista as pv
import xarray as xr
ds = xr.tutorial.open_dataset("ROMS_example.nc", chunks={"ocean_time": 1})
if ds.Vtransform == 1:
Zo_rho = ds.hc * (ds.s_rho - ds.Cs_r) + ds.Cs_r * ds.h
z_rho = Zo_rho + ds.zeta * (1 + Zo_rho / ds.h)
elif ds.Vtransform == 2:
Zo_rho = (ds.hc * ds.s_rho + ds.Cs_r * ds.h) / (ds.hc + ds.h)
z_rho = ds.zeta + (ds.zeta + ds.h) * Zo_rho
ds.coords["z_rho"] = z_rho.transpose() # needing transpose seems to be an xarray bug
da = ds.salt[dict(ocean_time=0)]
# Make array ordering consistent
da = da.transpose("s_rho", "xi_rho", "eta_rho", transpose_coords=False)
# Grab StructuredGrid mesh
mesh = da.pyvista.mesh(x="lon_rho", y="lat_rho", z="z_rho")
# Plot in 3D
p = pv.Plotter()
p.add_mesh(mesh, lighting=False, cmap='plasma', clim=[0, 35])
p.view_vector([1, -1, 1])
p.set_scale(zscale=0.001)
p.show()
```
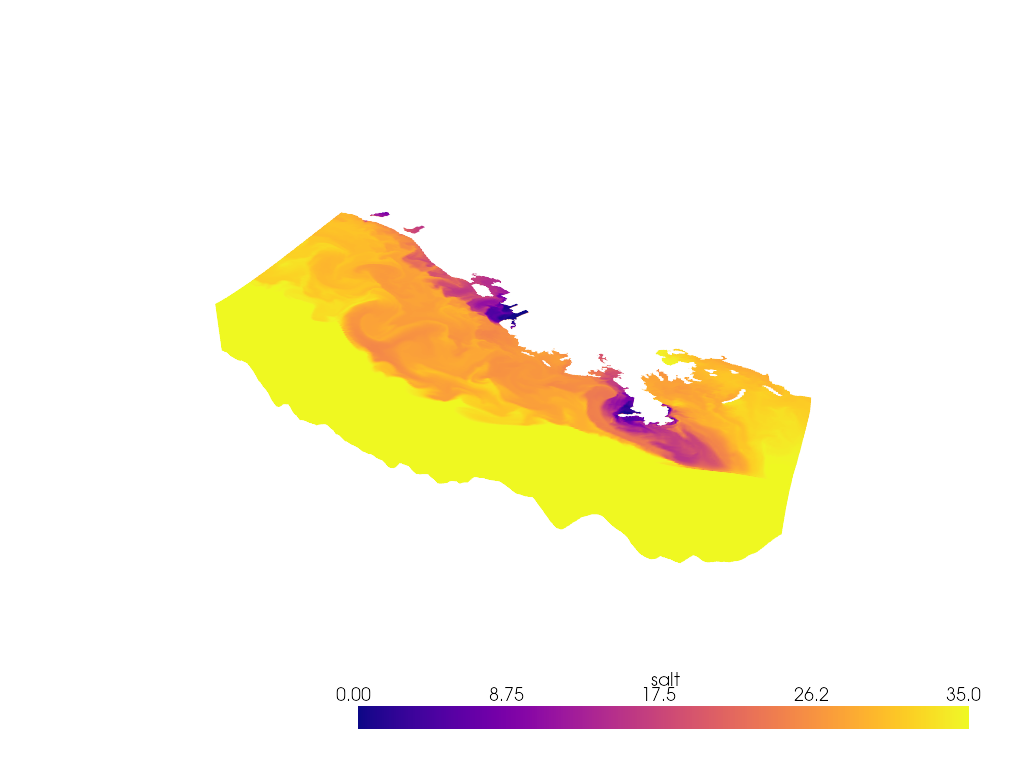
Raw data
{
"_id": null,
"home_page": "https://github.com/pyvista/pyvista-xarray",
"name": "pyvista-xarray",
"maintainer": null,
"docs_url": null,
"requires_python": ">=3.9",
"maintainer_email": null,
"keywords": null,
"author": "Kitware, Inc.",
"author_email": "kitware@kitware.com",
"download_url": "https://files.pythonhosted.org/packages/2c/62/a12321431df9f849349b489f6cfcdb66d33e9b6447360d6d44dc512de4a9/pyvista-xarray-0.1.6.tar.gz",
"platform": null,
"description": "# PyVista xarray\n\n[](https://pypi.org/project/pyvista-xarray/)\n[](https://codecov.io/gh/pyvista/pyvista-xarray)\n[](https://mybinder.org/v2/gh/pyvista/pyvista-xarray/HEAD)\n\nxarray DataArray accessors for PyVista to visualize datasets in 3D\n\n\n## \ud83d\ude80 Usage\n\nYou must `import pvxarray` in order to register the `DataArray` accessor with\nxarray. After which, a `pyvista` namespace of accessors will be available.\n\nTry on MyBinder: https://mybinder.org/v2/gh/pyvista/pyvista-xarray/HEAD\n\nThe following is an example to visualize a `RectilinearGrid` with PyVista:\n\n```py\nimport pvxarray\nimport xarray as xr\n\nds = xr.tutorial.load_dataset(\"air_temperature\")\nda = ds.air[dict(time=0)] # Select DataArray for a timestep\n\n# Plot in 3D\nda.pyvista.plot(x=\"lon\", y=\"lat\", show_edges=True, cpos='xy')\n\n# Or grab the mesh object for use with PyVista\nmesh = da.pyvista.mesh(x=\"lon\", y=\"lat\")\n```\n\n<!-- notebook=0, off_screen=1, screenshot='imgs/air_temperature.png' -->\n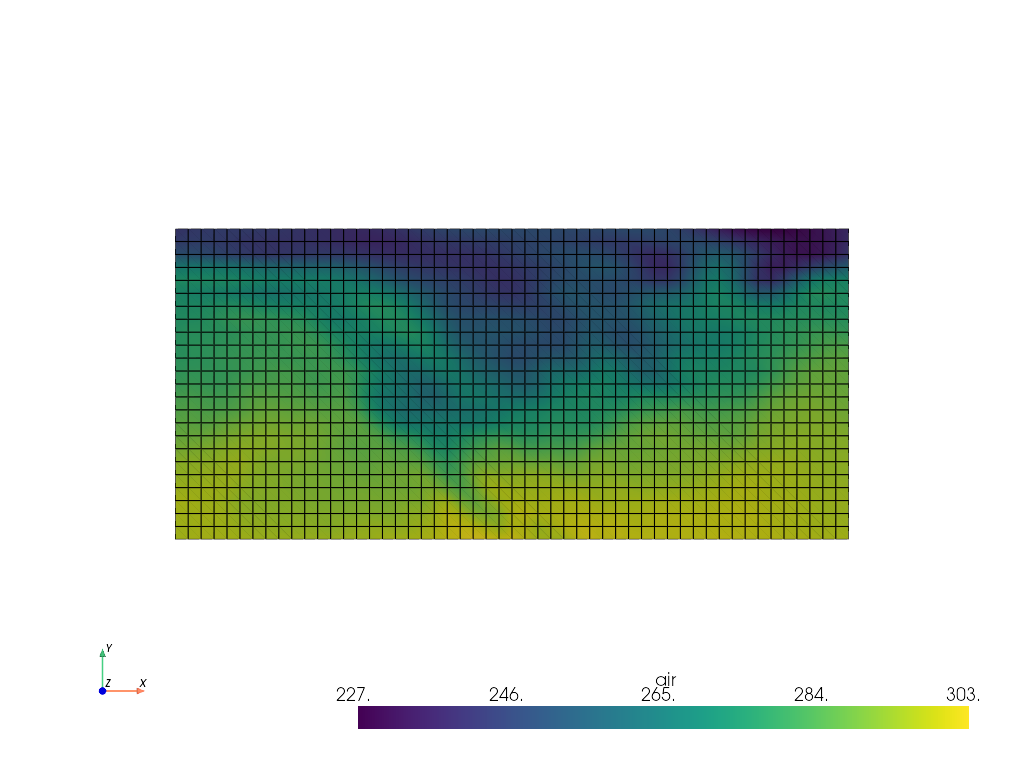\n\n\nOr you can read VTK meshes directly to xarray:\n\n```py\nimport xarray as xr\n\nds = xr.open_dataset(\"data.vtk\", engine=\"pyvista\")\nds[\"data array\"].pyvista.plot(x=\"x\", y=\"y\", z=\"z\")\n```\n\n## \u2b07\ufe0f Installation\n\n### \ud83d\udc0d Installing with `conda`\n\nConda makes managing `pyvista-xarray`'s dependencies across platforms quite\neasy and this is the recommended method to install:\n\n```bash\nconda install -c conda-forge pyvista-xarray\n```\n\n### \ud83c\udfa1 Installing with `pip`\n\nIf you prefer pip, then you can install from PyPI: https://pypi.org/project/pyvista-xarray/\n\n```bash\npip install pyvista-xarray\n```\n\n### Upstream Work\n\nMany of the examples leverage brand new features in PyVista `v0.38.1` and\nGeoVista which may not yet be released when you're reading this. Here is a list\nof pull requests needed for some of the examples:\n\n- GeoVista algorithm support: https://github.com/bjlittle/geovista/pull/127\n\nWork that was required and merged:\n\n- https://github.com/pyvista/pyvista/pull/2698\n- https://github.com/pyvista/pyvista/pull/2697\n- https://github.com/pyvista/pyvista/pull/3318\n- https://github.com/pyvista/pyvista/pull/3556\n- https://github.com/pyvista/pyvista/pull/3385\n\n## \ud83d\udcad Feedback\nPlease share your thoughts and questions on the Discussions board. If you would\nlike to report any bugs or make feature requests, please open an issue.\n\nIf filing a bug report, please share a scooby Report:\n\n```py\nimport pvxarray\nprint(pvxarray.Report())\n```\n\n\n## \ud83c\udfcf Further Examples\n\nThe following are a few simple examples taken from the xarray and\nrioxarray documentation. There are also more sophisticated examples\nin the `examples/` directory in this repository.\n\n### Simple RectilinearGrid\n\n```py\nimport numpy as np\nimport pvxarray\nimport xarray as xr\n\nlon = np.array([-99.83, -99.32])\nlat = np.array([42.25, 42.21])\nz = np.array([0, 10])\ntemp = 15 + 8 * np.random.randn(2, 2, 2)\n\nds = xr.Dataset(\n {\n \"temperature\": ([\"z\", \"x\", \"y\"], temp),\n },\n coords={\n \"lon\": ([\"x\"], lon),\n \"lat\": ([\"y\"], lat),\n \"z\": ([\"z\"], z),\n },\n)\n\nmesh = ds.temperature.pyvista.mesh(x=\"lon\", y=\"lat\", z=\"z\")\nmesh.plot()\n```\n\n\n## Raster with rioxarray\n\n```py\nimport pvxarray\nimport rioxarray\nimport xarray as xr\n\nda = rioxarray.open_rasterio(\"TC_NG_SFBay_US_Geo_COG.tif\")\nda = da.rio.reproject(\"EPSG:3857\")\n\n# Grab the mesh object for use with PyVista\nmesh = da.pyvista.mesh(x=\"x\", y=\"y\", component=\"band\")\n\nmesh.plot(scalars=\"data\", cpos='xy', rgb=True)\n```\n\n<!-- notebook=0, off_screen=1, screenshot='imgs/raster.png' -->\n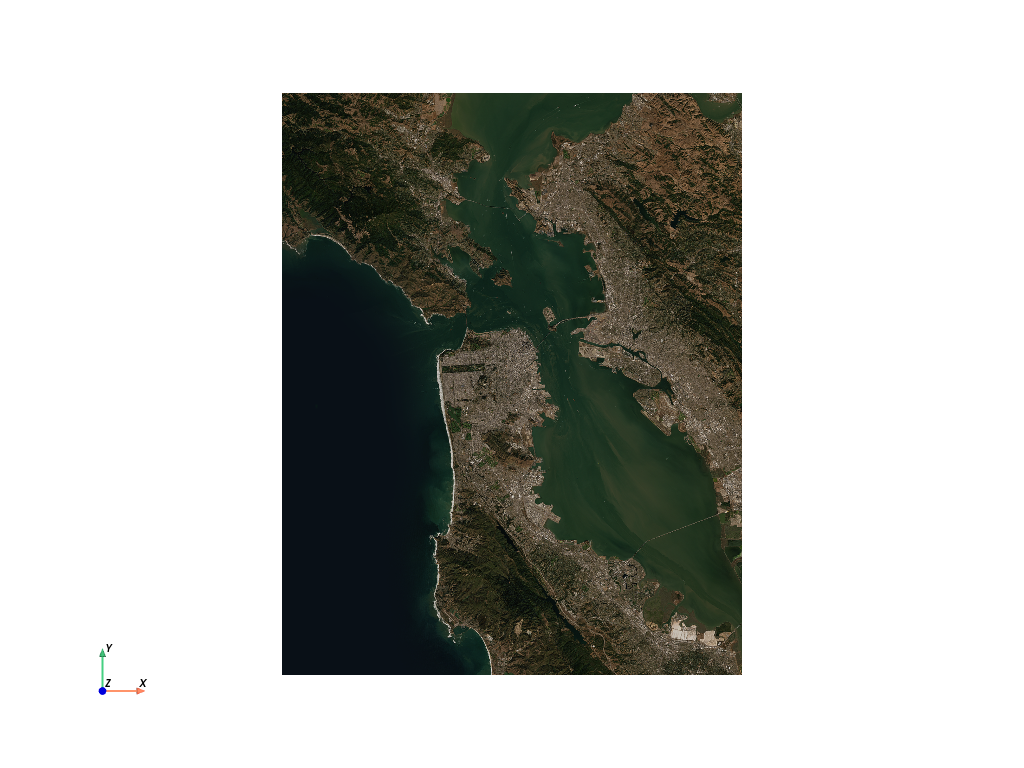\n\n```py\nimport pvxarray\nimport rioxarray\n\nda = rioxarray.open_rasterio(\"Elevation.tif\")\nda = da.rio.reproject(\"EPSG:3857\")\n\n# Grab the mesh object for use with PyVista\nmesh = da.pyvista.mesh(x=\"x\", y=\"y\")\n\n# Warp top and plot in 3D\nmesh.warp_by_scalar().plot()\n```\n\n<!-- notebook=0, off_screen=1, screenshot='imgs/topo.png' -->\n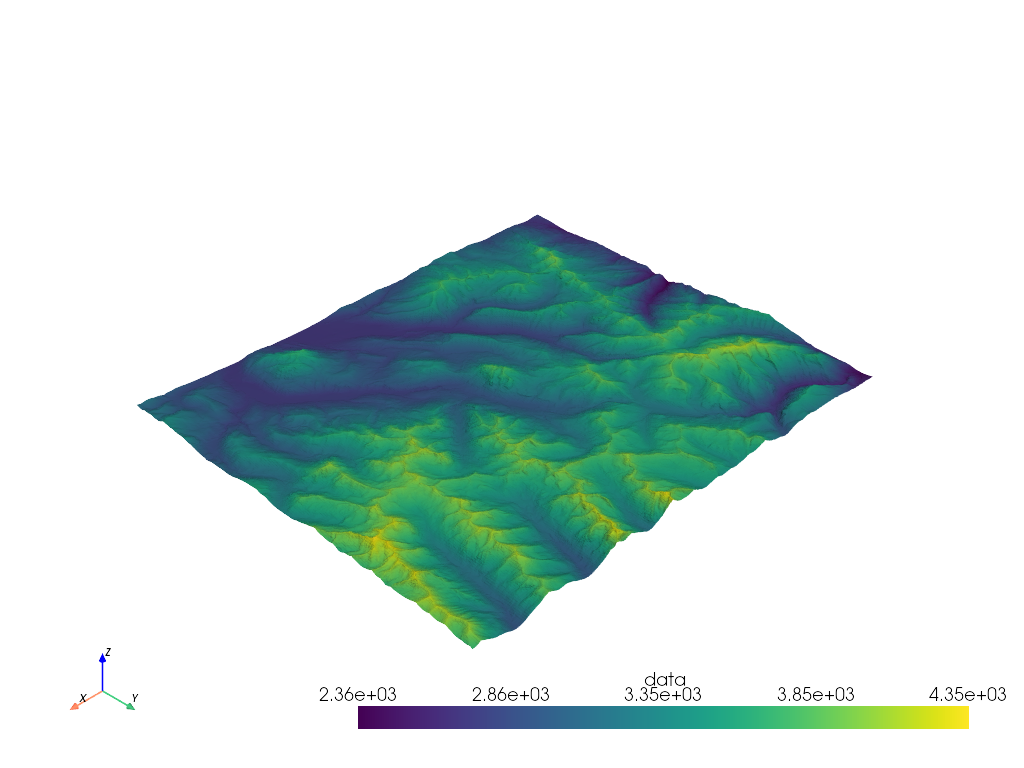\n\n\n## StructuredGrid\n\n```py\nimport pvxarray\nimport pyvista as pv\nimport xarray as xr\n\nds = xr.tutorial.open_dataset(\"ROMS_example.nc\", chunks={\"ocean_time\": 1})\n\nif ds.Vtransform == 1:\n Zo_rho = ds.hc * (ds.s_rho - ds.Cs_r) + ds.Cs_r * ds.h\n z_rho = Zo_rho + ds.zeta * (1 + Zo_rho / ds.h)\nelif ds.Vtransform == 2:\n Zo_rho = (ds.hc * ds.s_rho + ds.Cs_r * ds.h) / (ds.hc + ds.h)\n z_rho = ds.zeta + (ds.zeta + ds.h) * Zo_rho\n\nds.coords[\"z_rho\"] = z_rho.transpose() # needing transpose seems to be an xarray bug\n\nda = ds.salt[dict(ocean_time=0)]\n\n# Make array ordering consistent\nda = da.transpose(\"s_rho\", \"xi_rho\", \"eta_rho\", transpose_coords=False)\n\n# Grab StructuredGrid mesh\nmesh = da.pyvista.mesh(x=\"lon_rho\", y=\"lat_rho\", z=\"z_rho\")\n\n# Plot in 3D\np = pv.Plotter()\np.add_mesh(mesh, lighting=False, cmap='plasma', clim=[0, 35])\np.view_vector([1, -1, 1])\np.set_scale(zscale=0.001)\np.show()\n```\n\n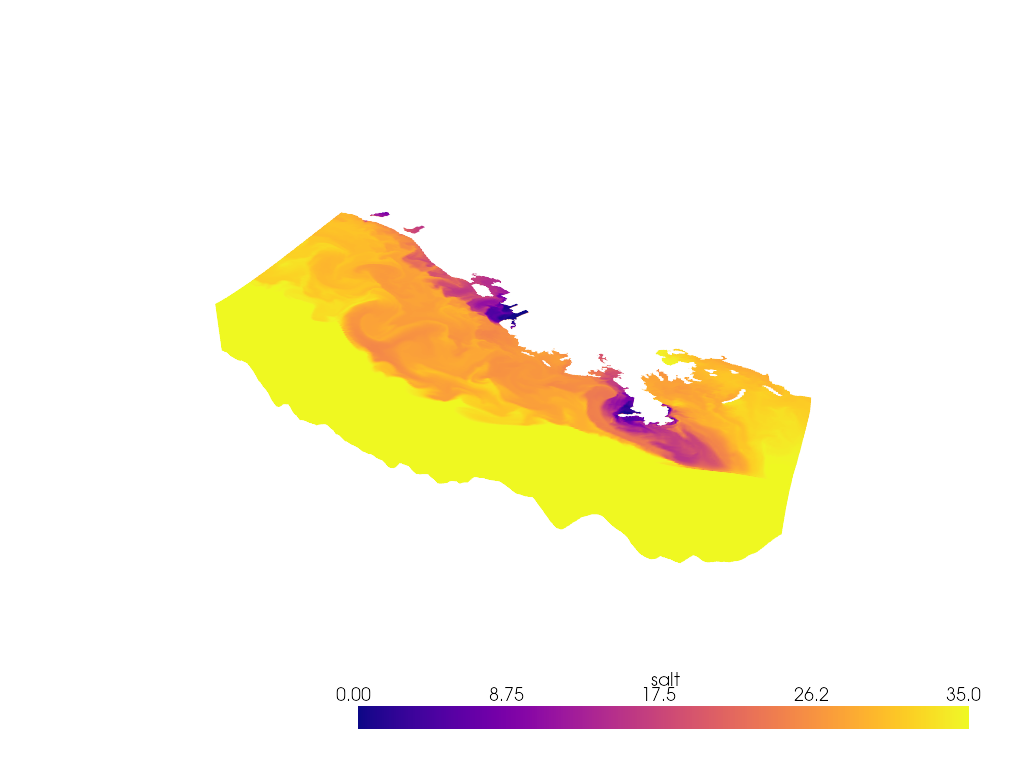\n\n\n",
"bugtrack_url": null,
"license": null,
"summary": "xarray DataArray accessors for PyVista",
"version": "0.1.6",
"project_urls": {
"Homepage": "https://github.com/pyvista/pyvista-xarray"
},
"split_keywords": [],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "5fc9be8b673f83742ba62373724ad1d03830be3e89ef50c3995bb4a3c7504b02",
"md5": "f0afffaa495a44bbb92e3ed4ed461d3a",
"sha256": "44ccdf162b4b7b04b8c6506739f59ec1b7b66003522a2c4286de269d29d930ea"
},
"downloads": -1,
"filename": "pyvista_xarray-0.1.6-py3-none-any.whl",
"has_sig": false,
"md5_digest": "f0afffaa495a44bbb92e3ed4ed461d3a",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.9",
"size": 17736,
"upload_time": "2024-05-03T17:42:03",
"upload_time_iso_8601": "2024-05-03T17:42:03.775732Z",
"url": "https://files.pythonhosted.org/packages/5f/c9/be8b673f83742ba62373724ad1d03830be3e89ef50c3995bb4a3c7504b02/pyvista_xarray-0.1.6-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "2c62a12321431df9f849349b489f6cfcdb66d33e9b6447360d6d44dc512de4a9",
"md5": "df23d090b9178c828109a4dd900e43c5",
"sha256": "02e6359da75fc9dff55572b07b9eb46befd3f4168820bdf43438e7a8542d621d"
},
"downloads": -1,
"filename": "pyvista-xarray-0.1.6.tar.gz",
"has_sig": false,
"md5_digest": "df23d090b9178c828109a4dd900e43c5",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.9",
"size": 26292263,
"upload_time": "2024-05-03T17:42:07",
"upload_time_iso_8601": "2024-05-03T17:42:07.001919Z",
"url": "https://files.pythonhosted.org/packages/2c/62/a12321431df9f849349b489f6cfcdb66d33e9b6447360d6d44dc512de4a9/pyvista-xarray-0.1.6.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-05-03 17:42:07",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "pyvista",
"github_project": "pyvista-xarray",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"requirements": [],
"lcname": "pyvista-xarray"
}