# <img src="https://raw.githubusercontent.com/cykim8811/pyx-react/main/assets/pyx.svg" width="30" height="30"> PyX Framework
## Introduction
PyX Framework is a framework that enables Python objects to be easily rendered on a web server. This allows Python developers to integrate their backend logic with web interfaces seamlessly.
## Features
- **Automatic Rendering**: Implement `__render__(self, user)` in Python objects to display them as web components.
- **Event Handling**: Easily handle user interactions with Python methods.
- **Dynamic Updates**: Changes in Python object states are automatically reflected in the web interface.
## Installation
To install PyX Framework, use pip:
```bash
pip install pyx-react
```
or clone this repository:
```bash
git clone https://github.com/cykim8811/pyx-react.git
cd pyx-react
python setup.py install
```
To install vscode extension, search for `pyx-react` in vscode extension marketplace.
## Usage
Here's a simple example of how to use PyX Framework:
- Create a .pyx file
```python
from pyx import createElement, App
class Counter:
def __init__(self):
self.count = 8
def increment(self, e):
if e.button == 0:
self.count += 1
def __render__(self, user):
return (
<div>
<div>count: {self.count}</div>
<button onClick={self.increment}>Increment</button>
</div>
)
app = App(Counter())
app.run(host='0.0.0.0', port=8080)
```
- Run pyx transpiler
```bash
pyx file.pyx
```
- Run transpiled .py file
```bash
python3 file.x.py
```
### Result
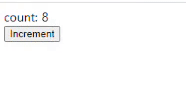
## Documentation
No documentation is available at the moment. Please refer to the examples for more information.
## Contributing
Contributions to PyX Framework are welcome!
## License
PyX Framework is licensed under MIT License.
## Contact
cykim8811@snu.ac.kr
Raw data
{
"_id": null,
"home_page": "https://github.com/cykim8811/pyx-react",
"name": "pyx-react",
"maintainer": "",
"docs_url": null,
"requires_python": "",
"maintainer_email": "",
"keywords": "pyx,react,python,visualization,web",
"author": "Kim Changyeon",
"author_email": "cykim8811@snu.ac.kr",
"download_url": "https://files.pythonhosted.org/packages/d4/d7/086cf0135f72dcf1e4d5476bea169b5f8de85a285eb2f5393de20ce7ede7/pyx-react-0.1.4.tar.gz",
"platform": null,
"description": "\n# <img src=\"https://raw.githubusercontent.com/cykim8811/pyx-react/main/assets/pyx.svg\" width=\"30\" height=\"30\"> PyX Framework\n\n\n## Introduction\nPyX Framework is a framework that enables Python objects to be easily rendered on a web server. This allows Python developers to integrate their backend logic with web interfaces seamlessly.\n\n## Features\n- **Automatic Rendering**: Implement `__render__(self, user)` in Python objects to display them as web components.\n- **Event Handling**: Easily handle user interactions with Python methods.\n- **Dynamic Updates**: Changes in Python object states are automatically reflected in the web interface.\n\n## Installation\nTo install PyX Framework, use pip:\n\n```bash\npip install pyx-react\n```\n\nor clone this repository:\n\n```bash\ngit clone https://github.com/cykim8811/pyx-react.git\ncd pyx-react\npython setup.py install\n```\n\nTo install vscode extension, search for `pyx-react` in vscode extension marketplace.\n\n## Usage\nHere's a simple example of how to use PyX Framework:\n\n- Create a .pyx file\n```python\n\nfrom pyx import createElement, App\n\nclass Counter:\n def __init__(self):\n self.count = 8\n\n def increment(self, e):\n if e.button == 0:\n self.count += 1\n\n def __render__(self, user):\n return (\n <div>\n <div>count: {self.count}</div>\n <button onClick={self.increment}>Increment</button>\n </div>\n )\n\n\napp = App(Counter())\napp.run(host='0.0.0.0', port=8080)\n\n\n```\n\n- Run pyx transpiler\n\n```bash\npyx file.pyx\n```\n\n- Run transpiled .py file\n\n```bash\npython3 file.x.py\n```\n\n\n### Result\n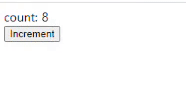\n\n## Documentation\nNo documentation is available at the moment. Please refer to the examples for more information.\n\n## Contributing\nContributions to PyX Framework are welcome!\n\n## License\nPyX Framework is licensed under MIT License.\n\n## Contact\ncykim8811@snu.ac.kr\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "A framework that enables Python objects to be easily rendered on a web server",
"version": "0.1.4",
"project_urls": {
"Homepage": "https://github.com/cykim8811/pyx-react"
},
"split_keywords": [
"pyx",
"react",
"python",
"visualization",
"web"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "6debf03ca7c9198bfd5dc94d6fcf673db051b10837839bbda0c4b94d14c982b8",
"md5": "d84b106e60c1b5962a7e1b507437cbab",
"sha256": "7edbe7f3c8c1c4a6c79b533b54ab1c44af95abd3280feb96880f37bd50118ec9"
},
"downloads": -1,
"filename": "pyx_react-0.1.4-py3-none-any.whl",
"has_sig": false,
"md5_digest": "d84b106e60c1b5962a7e1b507437cbab",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": null,
"size": 96347,
"upload_time": "2024-01-14T14:17:54",
"upload_time_iso_8601": "2024-01-14T14:17:54.476912Z",
"url": "https://files.pythonhosted.org/packages/6d/eb/f03ca7c9198bfd5dc94d6fcf673db051b10837839bbda0c4b94d14c982b8/pyx_react-0.1.4-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "d4d7086cf0135f72dcf1e4d5476bea169b5f8de85a285eb2f5393de20ce7ede7",
"md5": "0c0f9a1c8b6252e4cb0f84a182c35662",
"sha256": "f195e17831525e09ad95a2e4dd7be02d8484480349119bc9f3618814997c3f1f"
},
"downloads": -1,
"filename": "pyx-react-0.1.4.tar.gz",
"has_sig": false,
"md5_digest": "0c0f9a1c8b6252e4cb0f84a182c35662",
"packagetype": "sdist",
"python_version": "source",
"requires_python": null,
"size": 99582,
"upload_time": "2024-01-14T14:17:56",
"upload_time_iso_8601": "2024-01-14T14:17:56.519627Z",
"url": "https://files.pythonhosted.org/packages/d4/d7/086cf0135f72dcf1e4d5476bea169b5f8de85a285eb2f5393de20ce7ede7/pyx-react-0.1.4.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-01-14 14:17:56",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "cykim8811",
"github_project": "pyx-react",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"requirements": [],
"lcname": "pyx-react"
}