Name | qcio JSON |
Version |
0.11.14
JSON |
| download |
home_page | https://github.com/coltonbh/qcio |
Summary | Beautiful and user friendly data structures for quantum chemistry. |
upload_time | 2024-10-16 20:42:43 |
maintainer | None |
docs_url | None |
author | Colton Hicks |
requires_python | <4.0,>=3.8 |
license | MIT |
keywords |
|
VCS |
 |
bugtrack_url |
|
requirements |
No requirements were recorded.
|
Travis-CI |
No Travis.
|
coveralls test coverage |
No coveralls.
|
# Quantum Chemistry I/O
[](https://pypi.python.org/pypi/qcio)
[](https://pypi.python.org/pypi/qcio)
[](https://pypi.python.org/pypi/qcio)
[](https://github.com/coltonbh/qcio/actions)
[](https://github.com/coltonbh/qcio/actions)
[](https://github.com/charliermarsh/ruff)
Elegant and intuitive data structures for quantum chemistry, featuring seamless Jupyter Notebook visualizations. Also featuring NIST/CODATA2022 core physical constants and a Periodic Table.
Inspired by [QCElemental](https://github.com/MolSSI/QCElemental). Built for consistency and rapid development.
`qcio` works in harmony with a suite of other quantum chemistry tools for fast, structured, and interoperable quantum chemistry.
## The QC Suite of Programs
- [qcio](https://github.com/coltonbh/qcio) - Elegant and intuitive data structures for quantum chemistry, featuring seamless Jupyter Notebook visualizations. [Documentation](https://qcio.coltonhicks.com)
- [qcparse](https://github.com/coltonbh/qcparse) - A library for efficient parsing of quantum chemistry data into structured `qcio` objects.
- [qcop](https://github.com/coltonbh/qcop) - A package for operating quantum chemistry programs using `qcio` standardized data structures. Compatible with `TeraChem`, `psi4`, `QChem`, `NWChem`, `ORCA`, `Molpro`, `geomeTRIC` and many more.
- [BigChem](https://github.com/mtzgroup/bigchem) - A distributed application for running quantum chemistry calculations at scale across clusters of computers or the cloud. Bring multi-node scaling to your favorite quantum chemistry program.
- `ChemCloud` - A [web application](https://github.com/mtzgroup/chemcloud-server) and associated [Python client](https://github.com/mtzgroup/chemcloud-client) for exposing a BigChem cluster securely over the internet.
## Installation
```bash
pip install qcio
```
## Quickstart
`qcio` is built around a simple mental model: `Input` objects are used to define inputs for a quantum chemistry program, and `Output` objects are used to capture the outputs from a quantum chemistry program.
All `qcio` objects can be serialized and saved to disk by calling `.save("filename.json")` and loaded from disk by calling `.open("filename.json")`. `qcio` supports `json`, `yaml`, and `toml` file formats. Binary data will be automatically base64 encoded and decoded when saving and loading.
### Input Objects
#### ProgramInput - Core input object for a single QC program.
```python
from qcio import Structure, ProgramInput
# xyz files or saved Structure objects can be opened from disk
caffeine = Structure.open("caffeine.xyz")
# Define the program input
prog_input = ProgramInput(
structure=caffeine,
calctype="energy",
keywords={"purify": "no", "restricted": False},
model={"method": "hf", "basis": "sto-3g"},
extras={"comment": "This is a comment"}, # Anything extra not in the schema
)
# Binary or other files used as input can be added
prog_input.add_file("wfn.dat")
prog_input.keywords["initial_guess"] = "wfn.dat"
# Save the input to disk in json, yaml, or toml format
prog_input.save("input.json")
# Open the input from disk
prog_input = ProgramInput.open("input.json")
```
`Structure` objects can be opened from and saved as xyz files or saved to disk as `.json`, `.yaml`, or `.toml` formats by changing the extension of the file. For `.xyz` files precision can be controlled by passing the `precision` argument to the `save` method.
```python
caffeine = Structure.open("caffeine.xyz")
caffeine.save("caffeine2.json", precision=6)
caffeine.save("caffeine.toml")
```
#### DualProgramInput - Input object for a workflow that uses multiple QC programs.
`DualProgramInput` objects can be used to power workflows that require multiple QC programs. For example, a geometry optimization workflow might use `geomeTRIC` to power the optimization and use `terachem` to compute the energies and gradients.
```python
from qcio import Structure, DualProgramInput
# xyz files or saved Structure objects can be opened from disk
caffeine = Structure.open("caffeine.xyz")
# Define the program input
prog_input = DualProgramInput(
structure=caffeine,
calctype="optimization",
keywords={"maxiter": 250},
subprogram="terachem",
subprogram_args = {
"model": {"method": "hf", "basis": "sto-3g"},
"keywords": {"purify": "no", "restricted": False},
},
extras={"comment": "This is a comment"}, # Anything extra not in the schema
)
```
#### FileInput - Input object for a QC programs using native file formats.
`qcio` also supports the native file formats of each QC program with a `FileInput` object. Assume you have a directory like this with your input files for `psi4`:
```
psi4/
input.dat
geometry.xyz
wfn.dat
```
You can collect these native files and any associated command line arguments needed to specify a calculation into a `FileInput` object like this:
```python
from qcio import FileInput
psi4_input = FileInput.from_directory("psi4")
# All input files will be loaded into the `files` attribute
psi4_input.files
# {'input.dat': '...', 'geometry.xyz': '...', 'wfn.dat': '...'}
# Add psi4 command line args to the input
psi4_input.cmdline_args.extend(["-n", "4"])
# Files can be dumped to a directory for a calculation
psi4_input.save_files("psi4")
```
#### Modifying Input Objects
Objects are immutable by default so if you want to modify an object cast it to a dictionary, make the desired modification, and then instantiate a new object. This prevents accidentally modifying objects that may already be referenced in other calculations--perhaps as `.input_data` on an `Output` object.
```python
# Cast to a dictionary and modify
new_input_dict = prog_input.model_dumps()
new_input_dict["model"]["method"] = "b3lyp"
# Instantiate a new object
new_prog_input = ProgramInput(**new_input_dict)
```
### Output Objects
#### ProgramOutput
All computations result in a `ProgramOutput` object that encapsulates the core results, files, stdout, and additional details of the calculation. A `ProgramOutput` object has the following attributes:
```python
output.input_data # Input data used by the QC program
output.success # Whether the calculation succeeded or failed
output.results # All structured results from the calculation
output.results.files # Any files returned by the calculation
output.stdout # Stdout log from the calculation
output.pstdout # Shortcut to print out the stdout in human readable format
output.provenance # Provenance information about the calculation
output.extras # Any extra information not in the schema
```
The `.results` attribute on a `ProgramOutput` is polymorphic and may be either `Files`, `SinglePointResults` or `OptimizationResults` depending on the type of calculation requested. Available attributes for each result type can be found by calling `dir()` on the object.
```python
dir(output.results)
```
Results can be saved to disk in json, yaml, or toml format by calling `.save("filename.{json/yaml/toml}")` and loaded from disk by calling `.open("filename.{json/yaml/toml}")`.
## ✨ Visualization ✨
Visualize all your results with a single line of code!
First install the visualization module:
```sh
pip install qcio[view]
```
or if your shell requires `''` around arguments with brackets:
```sh
pip install 'qcio[view]'
```
Then in a Jupyter notebook import the `qcio` view module and call `view.view(...)` passing it one or any number of `qcio` objects you want to visualizing including `Structure` objects or any `ProgramOutput` object. You may also pass an array of `titles` and/or `subtitles` to add additional information to the molecular structure display. If no titles are passed `qcio` with look for `Structure` identifiers such as a name or SMILES to label the `Structure`.
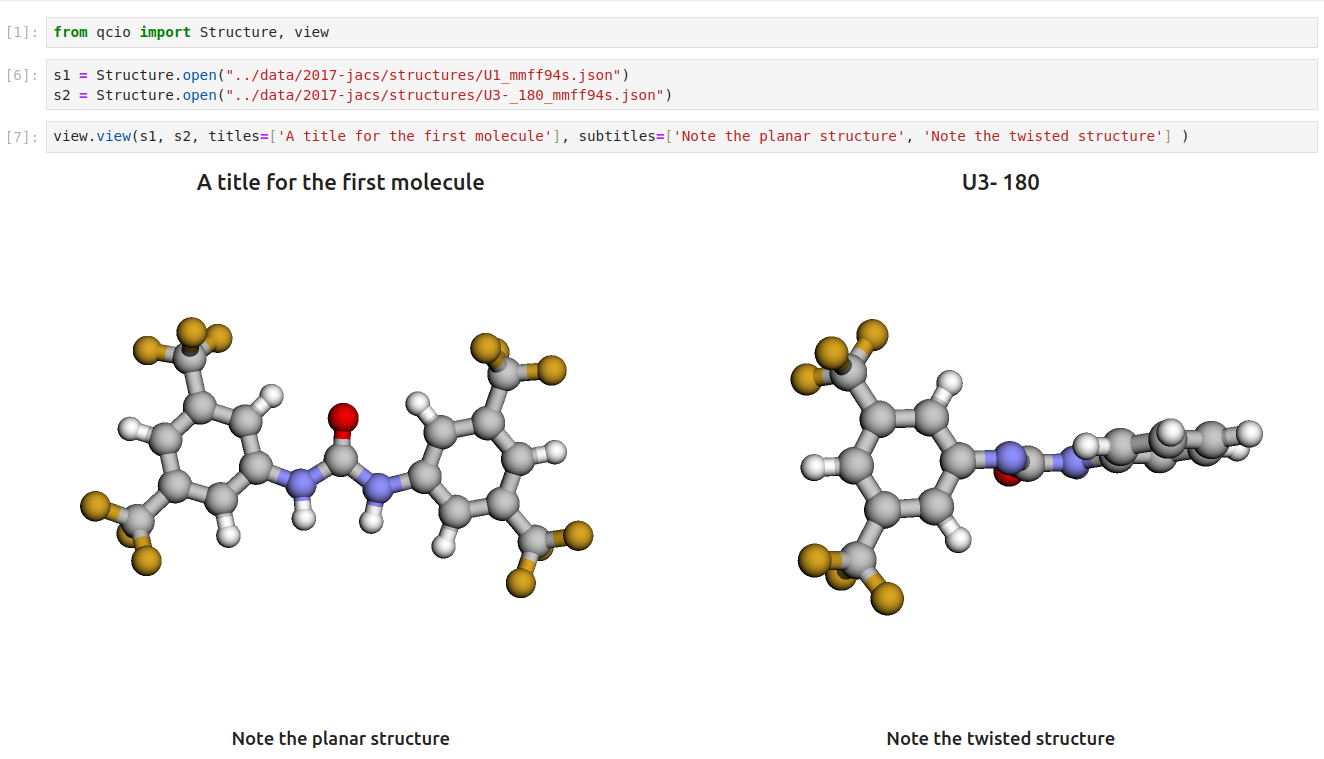
Seamless visualizations for `ProgramOutput` objects make results analysis easy!
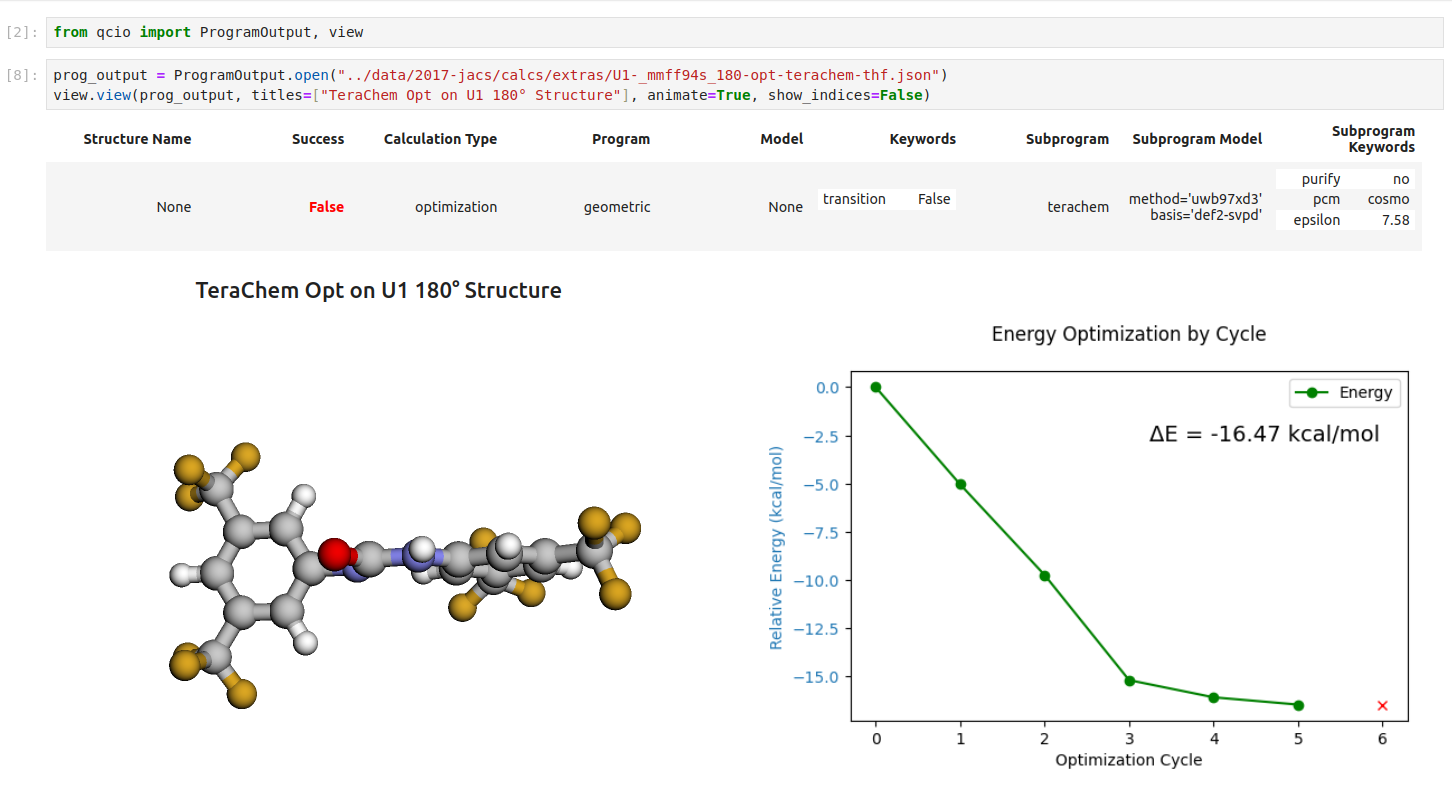
Single point calculations display their results in a table.
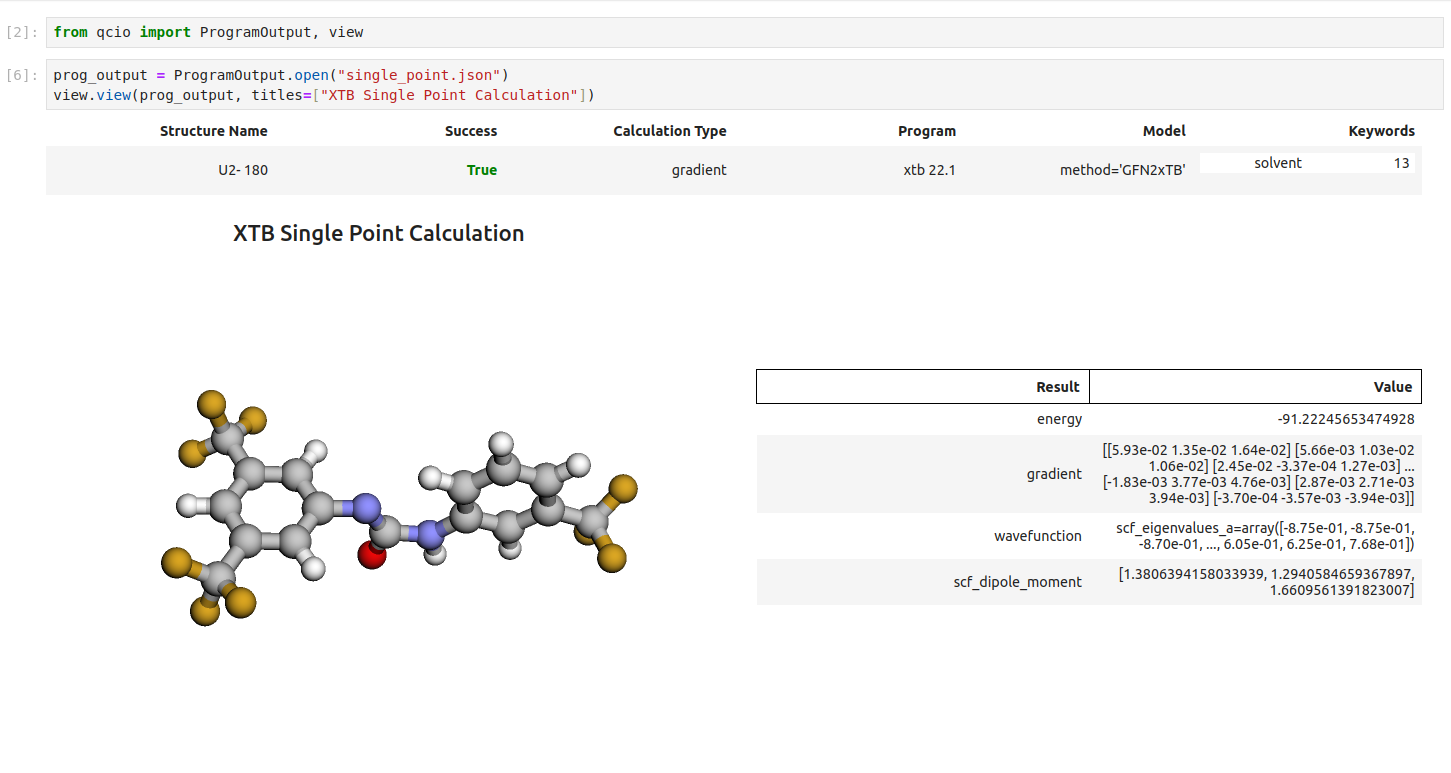
If you want to use the HTML generated by the viewer to build your own dashboards use the functions inside of `qcio.view.py` that begin with the word `generate_` to create HTML you can insert into any dashboard.
## Constants and Periodic Table
Core physical constants are available in the `constants` module. The source of all values is documented and kept up-to-date with the latest NIST/CODATA2022 values.
```python
from qcio import constants
constants.BOHR_TO_ANGSTROM
```
Periodic Table is also available from the `constants` module.
```python
from qcio.constants import periodic_table as pt
pt.He.symbol
pt.He.number
pt.He.name
pt.Ht.mass
pt.He.group
pt.He.period
pt.He.block
pt.He.electron_confg
group_6 = pt.group(6)
period_3 = pt.period(3)
# Check Data Source
pt.data_source
pt.data_url
```
## Support
If you have any issues with `qcio` or would like to request a feature, please open an [issue](https://github.com/coltonbh/qcio/issues).
Raw data
{
"_id": null,
"home_page": "https://github.com/coltonbh/qcio",
"name": "qcio",
"maintainer": null,
"docs_url": null,
"requires_python": "<4.0,>=3.8",
"maintainer_email": null,
"keywords": null,
"author": "Colton Hicks",
"author_email": "github@coltonhicks.com",
"download_url": "https://files.pythonhosted.org/packages/d9/2e/92034cb2f912989a817cb3be93b9cd68bf5a7676b5bd7cb44a90ce010416/qcio-0.11.14.tar.gz",
"platform": null,
"description": "# Quantum Chemistry I/O\n\n[](https://pypi.python.org/pypi/qcio)\n[](https://pypi.python.org/pypi/qcio)\n[](https://pypi.python.org/pypi/qcio)\n[](https://github.com/coltonbh/qcio/actions)\n[](https://github.com/coltonbh/qcio/actions)\n[](https://github.com/charliermarsh/ruff)\n\nElegant and intuitive data structures for quantum chemistry, featuring seamless Jupyter Notebook visualizations. Also featuring NIST/CODATA2022 core physical constants and a Periodic Table.\n\nInspired by [QCElemental](https://github.com/MolSSI/QCElemental). Built for consistency and rapid development.\n\n`qcio` works in harmony with a suite of other quantum chemistry tools for fast, structured, and interoperable quantum chemistry.\n\n## The QC Suite of Programs\n\n- [qcio](https://github.com/coltonbh/qcio) - Elegant and intuitive data structures for quantum chemistry, featuring seamless Jupyter Notebook visualizations. [Documentation](https://qcio.coltonhicks.com)\n- [qcparse](https://github.com/coltonbh/qcparse) - A library for efficient parsing of quantum chemistry data into structured `qcio` objects.\n- [qcop](https://github.com/coltonbh/qcop) - A package for operating quantum chemistry programs using `qcio` standardized data structures. Compatible with `TeraChem`, `psi4`, `QChem`, `NWChem`, `ORCA`, `Molpro`, `geomeTRIC` and many more.\n- [BigChem](https://github.com/mtzgroup/bigchem) - A distributed application for running quantum chemistry calculations at scale across clusters of computers or the cloud. Bring multi-node scaling to your favorite quantum chemistry program.\n- `ChemCloud` - A [web application](https://github.com/mtzgroup/chemcloud-server) and associated [Python client](https://github.com/mtzgroup/chemcloud-client) for exposing a BigChem cluster securely over the internet.\n\n## Installation\n\n```bash\npip install qcio\n```\n\n## Quickstart\n\n`qcio` is built around a simple mental model: `Input` objects are used to define inputs for a quantum chemistry program, and `Output` objects are used to capture the outputs from a quantum chemistry program.\n\nAll `qcio` objects can be serialized and saved to disk by calling `.save(\"filename.json\")` and loaded from disk by calling `.open(\"filename.json\")`. `qcio` supports `json`, `yaml`, and `toml` file formats. Binary data will be automatically base64 encoded and decoded when saving and loading.\n\n### Input Objects\n\n#### ProgramInput - Core input object for a single QC program.\n\n```python\nfrom qcio import Structure, ProgramInput\n# xyz files or saved Structure objects can be opened from disk\ncaffeine = Structure.open(\"caffeine.xyz\")\n# Define the program input\nprog_input = ProgramInput(\n structure=caffeine,\n calctype=\"energy\",\n keywords={\"purify\": \"no\", \"restricted\": False},\n model={\"method\": \"hf\", \"basis\": \"sto-3g\"},\n extras={\"comment\": \"This is a comment\"}, # Anything extra not in the schema\n)\n# Binary or other files used as input can be added\nprog_input.add_file(\"wfn.dat\")\nprog_input.keywords[\"initial_guess\"] = \"wfn.dat\"\n\n# Save the input to disk in json, yaml, or toml format\nprog_input.save(\"input.json\")\n\n# Open the input from disk\nprog_input = ProgramInput.open(\"input.json\")\n```\n\n`Structure` objects can be opened from and saved as xyz files or saved to disk as `.json`, `.yaml`, or `.toml` formats by changing the extension of the file. For `.xyz` files precision can be controlled by passing the `precision` argument to the `save` method.\n\n```python\ncaffeine = Structure.open(\"caffeine.xyz\")\ncaffeine.save(\"caffeine2.json\", precision=6)\ncaffeine.save(\"caffeine.toml\")\n```\n\n#### DualProgramInput - Input object for a workflow that uses multiple QC programs.\n\n`DualProgramInput` objects can be used to power workflows that require multiple QC programs. For example, a geometry optimization workflow might use `geomeTRIC` to power the optimization and use `terachem` to compute the energies and gradients.\n\n```python\nfrom qcio import Structure, DualProgramInput\n# xyz files or saved Structure objects can be opened from disk\ncaffeine = Structure.open(\"caffeine.xyz\")\n# Define the program input\nprog_input = DualProgramInput(\n structure=caffeine,\n calctype=\"optimization\",\n keywords={\"maxiter\": 250},\n subprogram=\"terachem\",\n subprogram_args = {\n \"model\": {\"method\": \"hf\", \"basis\": \"sto-3g\"},\n \"keywords\": {\"purify\": \"no\", \"restricted\": False},\n },\n extras={\"comment\": \"This is a comment\"}, # Anything extra not in the schema\n)\n```\n\n#### FileInput - Input object for a QC programs using native file formats.\n\n`qcio` also supports the native file formats of each QC program with a `FileInput` object. Assume you have a directory like this with your input files for `psi4`:\n\n```\npsi4/\n input.dat\n geometry.xyz\n wfn.dat\n```\n\nYou can collect these native files and any associated command line arguments needed to specify a calculation into a `FileInput` object like this:\n\n```python\nfrom qcio import FileInput\npsi4_input = FileInput.from_directory(\"psi4\")\n\n# All input files will be loaded into the `files` attribute\npsi4_input.files\n# {'input.dat': '...', 'geometry.xyz': '...', 'wfn.dat': '...'}\n\n# Add psi4 command line args to the input\npsi4_input.cmdline_args.extend([\"-n\", \"4\"])\n\n# Files can be dumped to a directory for a calculation\npsi4_input.save_files(\"psi4\")\n```\n\n#### Modifying Input Objects\n\nObjects are immutable by default so if you want to modify an object cast it to a dictionary, make the desired modification, and then instantiate a new object. This prevents accidentally modifying objects that may already be referenced in other calculations--perhaps as `.input_data` on an `Output` object.\n\n```python\n# Cast to a dictionary and modify\nnew_input_dict = prog_input.model_dumps()\nnew_input_dict[\"model\"][\"method\"] = \"b3lyp\"\n# Instantiate a new object\nnew_prog_input = ProgramInput(**new_input_dict)\n```\n\n### Output Objects\n\n#### ProgramOutput\n\nAll computations result in a `ProgramOutput` object that encapsulates the core results, files, stdout, and additional details of the calculation. A `ProgramOutput` object has the following attributes:\n\n```python\noutput.input_data # Input data used by the QC program\noutput.success # Whether the calculation succeeded or failed\noutput.results # All structured results from the calculation\noutput.results.files # Any files returned by the calculation\noutput.stdout # Stdout log from the calculation\noutput.pstdout # Shortcut to print out the stdout in human readable format\noutput.provenance # Provenance information about the calculation\noutput.extras # Any extra information not in the schema\n```\n\nThe `.results` attribute on a `ProgramOutput` is polymorphic and may be either `Files`, `SinglePointResults` or `OptimizationResults` depending on the type of calculation requested. Available attributes for each result type can be found by calling `dir()` on the object.\n\n```python\ndir(output.results)\n```\n\nResults can be saved to disk in json, yaml, or toml format by calling `.save(\"filename.{json/yaml/toml}\")` and loaded from disk by calling `.open(\"filename.{json/yaml/toml}\")`.\n\n## \u2728 Visualization \u2728\n\nVisualize all your results with a single line of code!\n\nFirst install the visualization module:\n\n```sh\npip install qcio[view]\n```\n\nor if your shell requires `''` around arguments with brackets:\n\n```sh\npip install 'qcio[view]'\n```\n\nThen in a Jupyter notebook import the `qcio` view module and call `view.view(...)` passing it one or any number of `qcio` objects you want to visualizing including `Structure` objects or any `ProgramOutput` object. You may also pass an array of `titles` and/or `subtitles` to add additional information to the molecular structure display. If no titles are passed `qcio` with look for `Structure` identifiers such as a name or SMILES to label the `Structure`.\n\n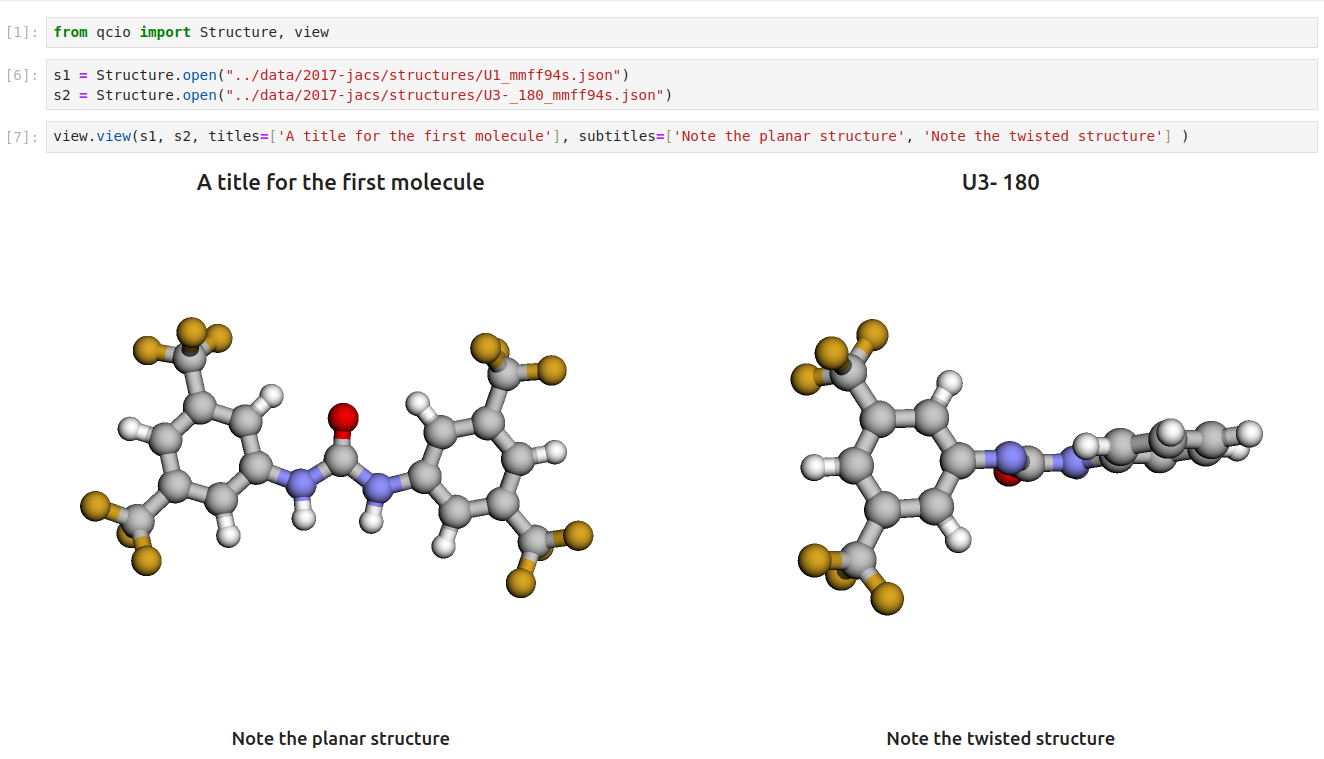\n\nSeamless visualizations for `ProgramOutput` objects make results analysis easy!\n\n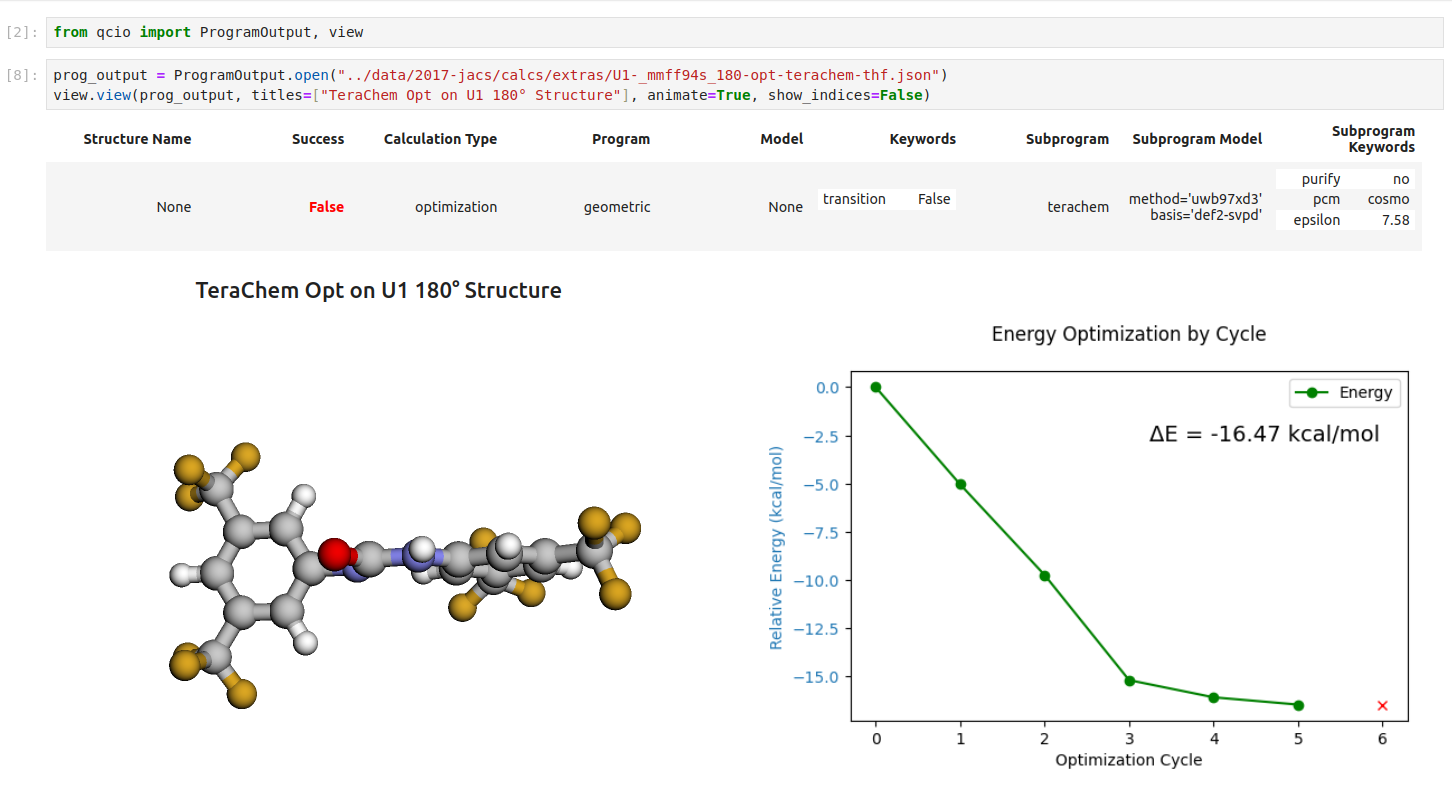\n\nSingle point calculations display their results in a table.\n\n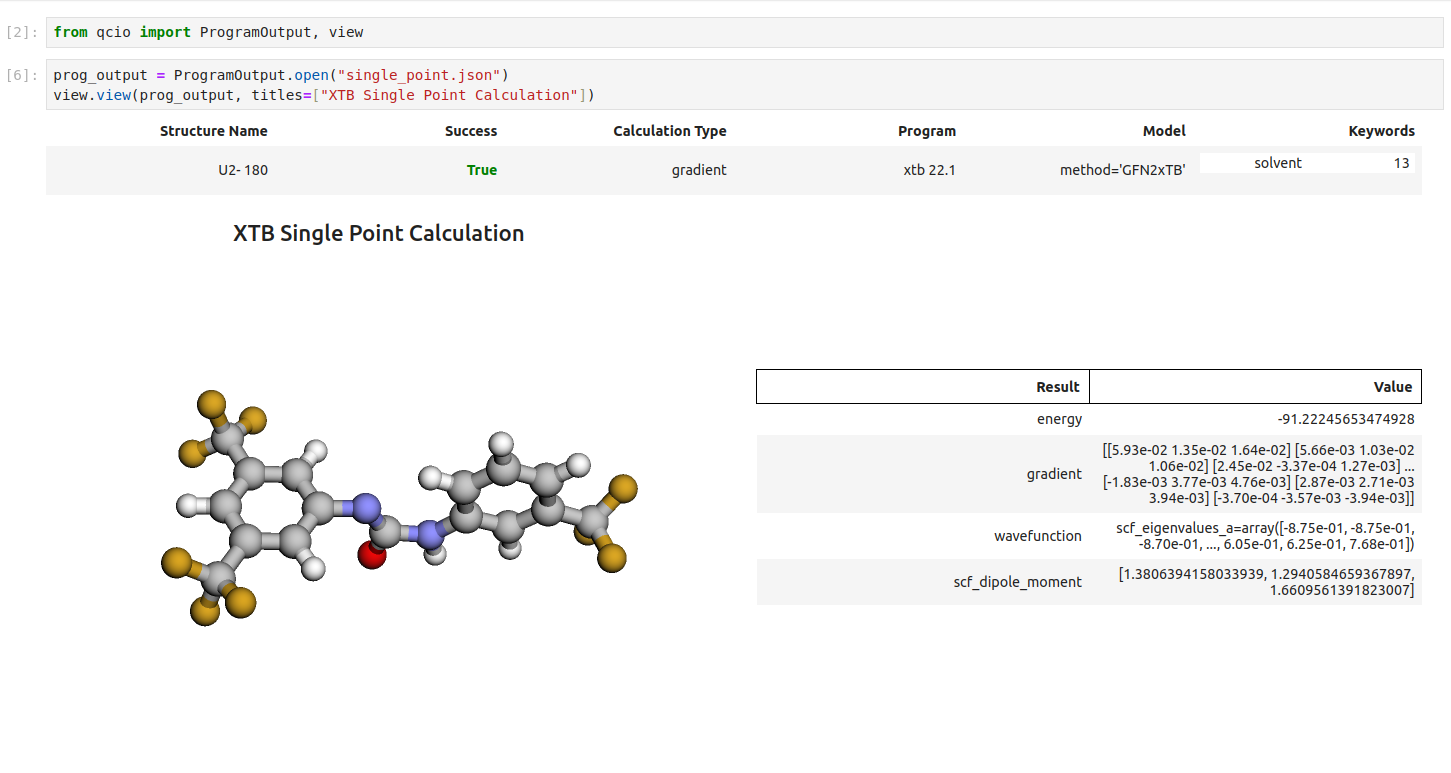\n\nIf you want to use the HTML generated by the viewer to build your own dashboards use the functions inside of `qcio.view.py` that begin with the word `generate_` to create HTML you can insert into any dashboard.\n\n## Constants and Periodic Table\n\nCore physical constants are available in the `constants` module. The source of all values is documented and kept up-to-date with the latest NIST/CODATA2022 values.\n\n```python\nfrom qcio import constants\n\nconstants.BOHR_TO_ANGSTROM\n```\n\nPeriodic Table is also available from the `constants` module.\n\n```python\nfrom qcio.constants import periodic_table as pt\n\npt.He.symbol\npt.He.number\npt.He.name\npt.Ht.mass\npt.He.group\npt.He.period\npt.He.block\npt.He.electron_confg\n\ngroup_6 = pt.group(6)\nperiod_3 = pt.period(3)\n\n# Check Data Source\npt.data_source\npt.data_url\n```\n\n## Support\n\nIf you have any issues with `qcio` or would like to request a feature, please open an [issue](https://github.com/coltonbh/qcio/issues).\n\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "Beautiful and user friendly data structures for quantum chemistry.",
"version": "0.11.14",
"project_urls": {
"Documentation": "https://qcio.coltonhicks.com",
"Homepage": "https://github.com/coltonbh/qcio",
"Repository": "https://github.com/coltonbh/qcio"
},
"split_keywords": [],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "8f3b27558bcb014e6db8150c5cdcbea46f414c612e94a34fed77772a8be5e503",
"md5": "3e3021dc1be5f7423fbf8f3d7bbd7b42",
"sha256": "9f99a278b03e3ee468c15ad829eb9b58cd6b242e1fc73c9765260fede44e2ec4"
},
"downloads": -1,
"filename": "qcio-0.11.14-py3-none-any.whl",
"has_sig": false,
"md5_digest": "3e3021dc1be5f7423fbf8f3d7bbd7b42",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": "<4.0,>=3.8",
"size": 44777,
"upload_time": "2024-10-16T20:42:41",
"upload_time_iso_8601": "2024-10-16T20:42:41.026060Z",
"url": "https://files.pythonhosted.org/packages/8f/3b/27558bcb014e6db8150c5cdcbea46f414c612e94a34fed77772a8be5e503/qcio-0.11.14-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "d92e92034cb2f912989a817cb3be93b9cd68bf5a7676b5bd7cb44a90ce010416",
"md5": "9c0962dc61ecef5b4890474a6865d1c0",
"sha256": "d18c09d30f7d15a7e7723055ba753d671ec78c01cda9068dc25bb8cab8d7dd71"
},
"downloads": -1,
"filename": "qcio-0.11.14.tar.gz",
"has_sig": false,
"md5_digest": "9c0962dc61ecef5b4890474a6865d1c0",
"packagetype": "sdist",
"python_version": "source",
"requires_python": "<4.0,>=3.8",
"size": 42574,
"upload_time": "2024-10-16T20:42:43",
"upload_time_iso_8601": "2024-10-16T20:42:43.016850Z",
"url": "https://files.pythonhosted.org/packages/d9/2e/92034cb2f912989a817cb3be93b9cd68bf5a7676b5bd7cb44a90ce010416/qcio-0.11.14.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-10-16 20:42:43",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "coltonbh",
"github_project": "qcio",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "qcio"
}