[](https://github.com/averyanalex/quantpiler/actions/workflows/ci.yml)
[](https://opensource.org/license/agpl-v3)
[](https://pypi.org/project/quantpiler/)
# Сompiler of classical algorithms into oracles for quantum computing
## Achievements:
- CRC32 hash function (4 byte input) - 318 qubits.
## Architecture:
1. Building expression.
2. Optimizing expression.
3. Constructing list of logical gates (logical expressions) for each bit of
optimized expression.
4. Logical gates optimization (minimizing unique logic operations and qubit
allocations).
5. Generation of a quantum circuit from a DAG of logical gates.
## Authors:
- Alexander Averyanov - author
- Evgeny Kiktenko - mentor
- Dmitry Ershov - helped with the optimizer design
## Example:
```python
import quantpiler
x_len = 4
x = quantpiler.argument("x", x_len)
a = 6
# N = 2**4
prod = 1
for i in range(x_len):
prod = ((x >> i) & 1).ternary(prod * a**(2**i), prod) & 0b1111
circ = prod.compile()
qc = quantpiler.circuit_to_qiskit(circ)
qc.draw("mpl")
```
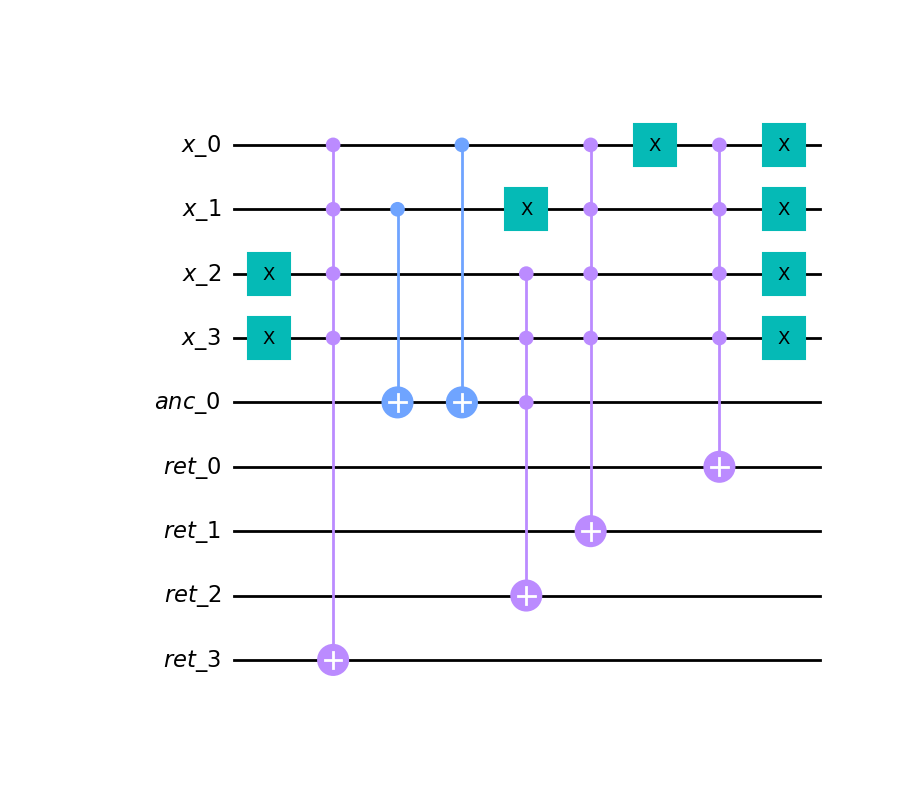
```python
# returning ancillas and arguments to their original state
rqc = quantpiler.circuit_to_qiskit(circ, rev=True)
rqc.draw("mpl")
```
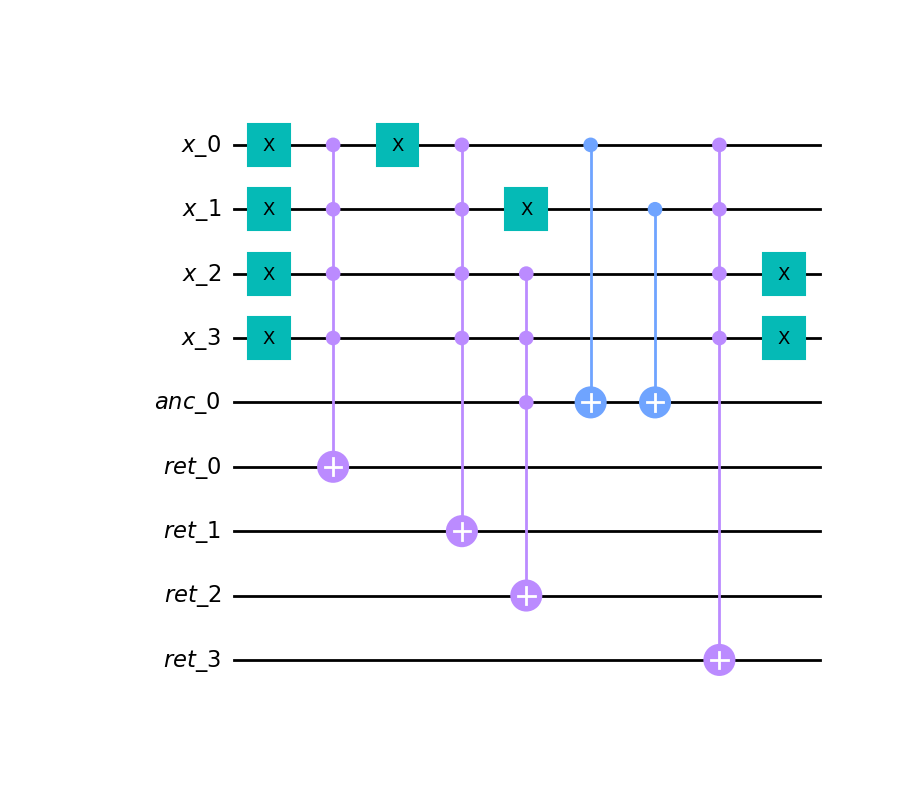
## User guide
### Installation
```shell
pip install quantpiler
```
Binary releases on PyPI only available for Windows (x86, x86_64) and
GNU/Linux (x86_64).
Now you can import library in Python:
```python
import quantpiler
```
### Creating input variables
```python
a = quantpiler.argument("a", 2)
b = quantpiler.argument("b", 4)
```
This will create argument "a" with length of 2 qubits and "b" with length of 4
qubits. You can't use arguments with same name but with
different lengths.
### Expressions
Any argument variable, constant, or combination thereof is an expression.
Expressions are actually lists of logic gates representing each bit. For
example, `a ^ b` is effectively `[[a[0] ^ b[0], [a[1] ^ b[1], b[2], b[3]]`.
#### Output expression lengths
Let's `a` -- length of first operand, `b` -- length (value for bitshifts) of
second operand.
| Name | Notation | Length |
| -------------- | -------- | ------------- |
| Binary invert | ~ | a |
| Bitwise XOR | ^ | max(a, b) |
| Bitwise OR | \| | max(a, b) |
| Bitwise AND | & | min(a, b) |
| Sum | + | max(a, b) + 1 |
| Product | \* | a + b |
| Right bitshift | >> | a - b |
| Left bitshift | << | a + b |
Length is the **maximum possible** length of result. Actual length depends on
optimizer decitions: for example, the length of `a ^ a` will be 0 (no qubits,
empty expression).
#### Estimating length of expression:
```python
# you can use builtin python's len to get estimated expression's length
length = len(a ^ b + 1)
```
Please note that this is an **estimated** length as actual length may vary depending
optimizer solutions.
#### Debugging expression:
```python
print(str(a ^ b + 1))
```
or, for jupyter:
```python
a ^ b + 1
```
This will print `(^ "a(2)" (+ 1 "4(2)"))` for `a` of length 2 and `b` of length 4.
### Bitwise binary operations
```python
r = ~a
r = a ^ b
r = a | b
r = a & b
```
You can also do this with constants:
```python
r = 0b101 ^ a
b |= 1
r = b & 0b11
```
### Bit shifting
```python
r = a << 2
r = b >> 3
```
Please note that only constant distance shifting is supported at this time.
### Arithmetic operations
```python
r = a + b
r = 2 * a * b
```
### Ternary operations
If you want to emulate if statements, i.e.
```python
if cond:
r = a + b
else:
r = b & 0b11
```
you can use ternary operators:
```python
r = cond.ternary(a + b, b & 0b11)
```
Note that cond must be an expression exactly 1 qubit long. You can
achieve this by using bitwise and with 1.
### Compiling
Let's compile something:
```python
r = a ^ b + 3
# internal circuit representation
circ = r.compile()
# QuantumCircuit from qiskit
qc = quantpiler.circuit_to_qiskit(circ)
# let's draw our circuit
qc.draw("mpl")
```
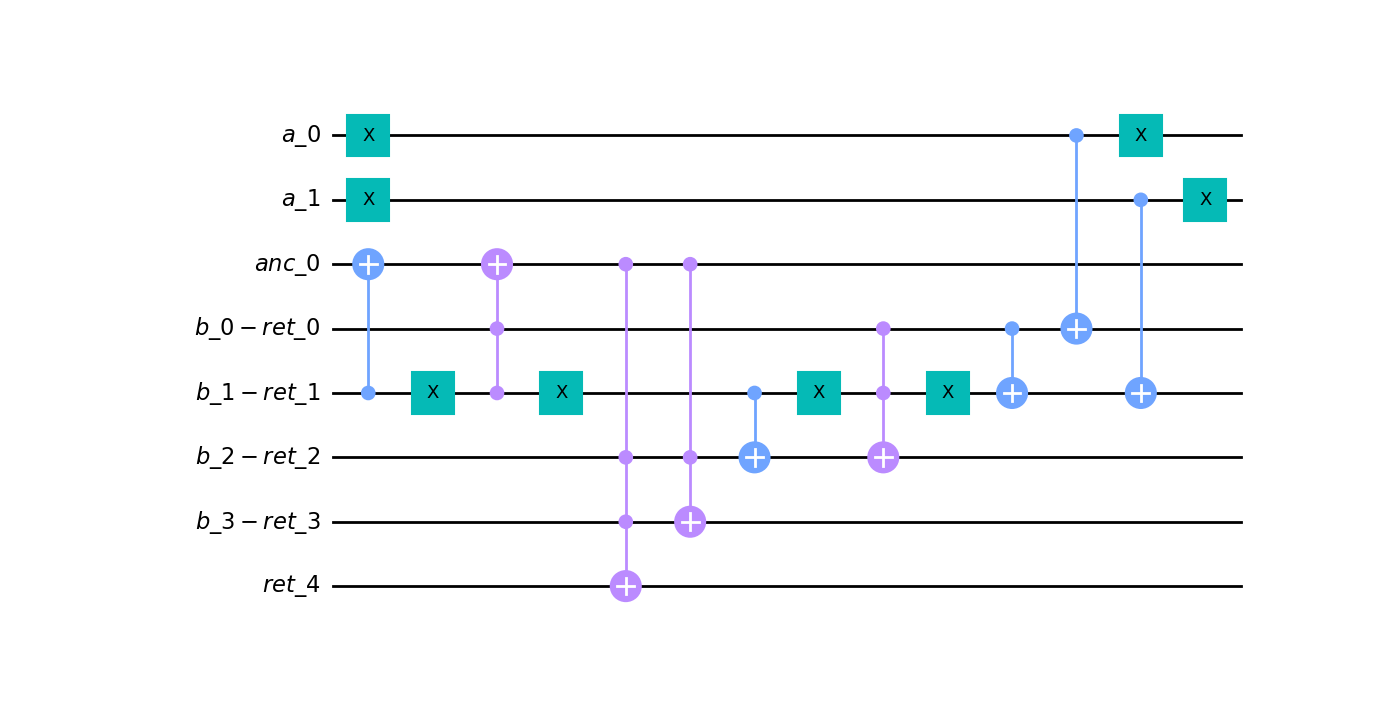
Raw data
{
"_id": null,
"home_page": null,
"name": "quantpiler",
"maintainer": null,
"docs_url": null,
"requires_python": ">=3.8",
"maintainer_email": null,
"keywords": "qiskit,quantum",
"author": "Alexander Averyanov <alex@averyan.ru>",
"author_email": "Alexander Averyanov <alex@averyan.ru>",
"download_url": "https://files.pythonhosted.org/packages/35/9e/5431bc679b612802daf4cba52766327ece0624c5bb5014816343056ffef8/quantpiler-0.3.5.tar.gz",
"platform": null,
"description": "[](https://github.com/averyanalex/quantpiler/actions/workflows/ci.yml)\n[](https://opensource.org/license/agpl-v3)\n[](https://pypi.org/project/quantpiler/)\n\n# \u0421ompiler of classical algorithms into oracles for quantum computing\n\n## Achievements:\n\n- CRC32 hash function (4 byte input) - 318 qubits.\n\n## Architecture:\n\n1. Building expression.\n2. Optimizing expression.\n3. Constructing list of logical gates (logical expressions) for each bit of\n optimized expression.\n4. Logical gates optimization (minimizing unique logic operations and qubit\n allocations).\n5. Generation of a quantum circuit from a DAG of logical gates.\n\n## Authors:\n\n- Alexander Averyanov - author\n- Evgeny Kiktenko - mentor\n- Dmitry Ershov - helped with the optimizer design\n\n## Example:\n\n```python\nimport quantpiler\n\nx_len = 4\nx = quantpiler.argument(\"x\", x_len)\n\na = 6\n# N = 2**4\n\nprod = 1\nfor i in range(x_len):\n prod = ((x >> i) & 1).ternary(prod * a**(2**i), prod) & 0b1111\n\ncirc = prod.compile()\nqc = quantpiler.circuit_to_qiskit(circ)\n\nqc.draw(\"mpl\")\n```\n\n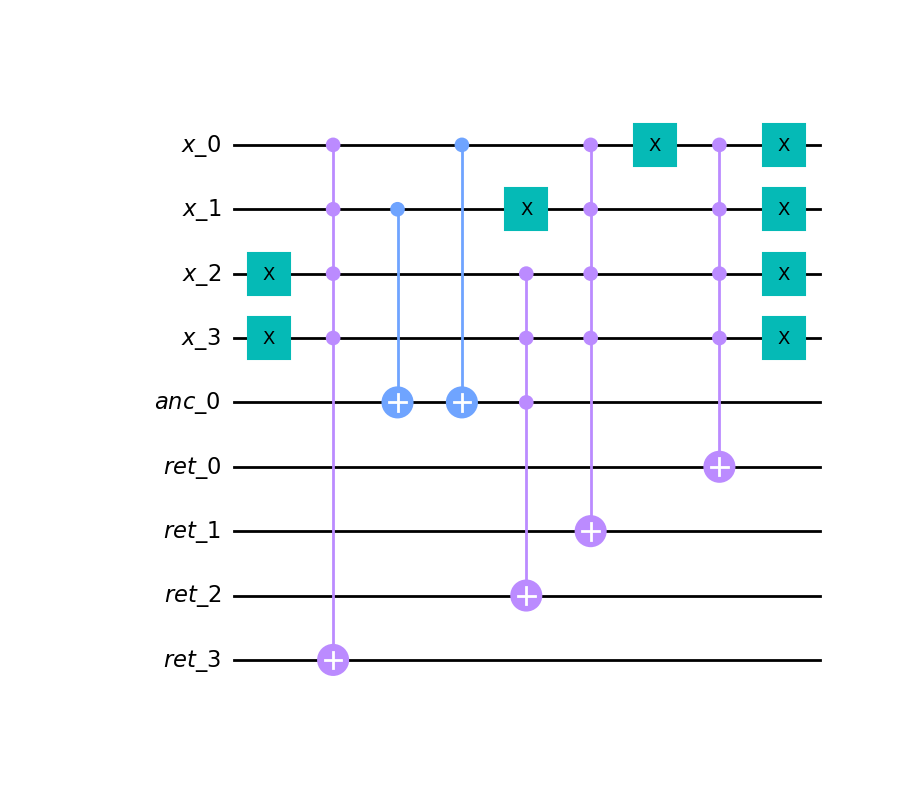\n\n```python\n# returning ancillas and arguments to their original state\nrqc = quantpiler.circuit_to_qiskit(circ, rev=True)\n\nrqc.draw(\"mpl\")\n```\n\n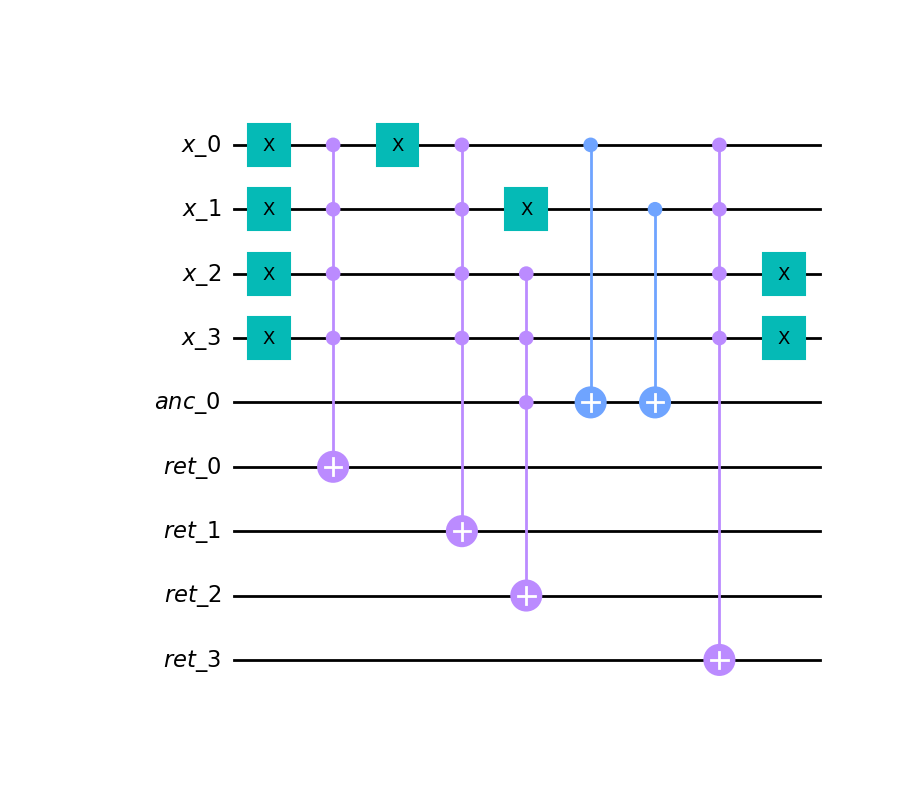\n\n## User guide\n\n### Installation\n\n```shell\npip install quantpiler\n```\n\nBinary releases on PyPI only available for Windows (x86, x86_64) and\nGNU/Linux (x86_64).\n\nNow you can import library in Python:\n\n```python\nimport quantpiler\n```\n\n### Creating input variables\n\n```python\na = quantpiler.argument(\"a\", 2)\nb = quantpiler.argument(\"b\", 4)\n```\n\nThis will create argument \"a\" with length of 2 qubits and \"b\" with length of 4\nqubits. You can't use arguments with same name but with\ndifferent lengths.\n\n### Expressions\n\nAny argument variable, constant, or combination thereof is an expression.\nExpressions are actually lists of logic gates representing each bit. For\nexample, `a ^ b` is effectively `[[a[0] ^ b[0], [a[1] ^ b[1], b[2], b[3]]`.\n\n#### Output expression lengths\n\nLet's `a` -- length of first operand, `b` -- length (value for bitshifts) of\nsecond operand.\n\n| Name | Notation | Length |\n| -------------- | -------- | ------------- |\n| Binary invert | ~ | a |\n| Bitwise XOR | ^ | max(a, b) |\n| Bitwise OR | \\| | max(a, b) |\n| Bitwise AND | & | min(a, b) |\n| Sum | + | max(a, b) + 1 |\n| Product | \\* | a + b |\n| Right bitshift | >> | a - b |\n| Left bitshift | << | a + b |\n\nLength is the **maximum possible** length of result. Actual length depends on\noptimizer decitions: for example, the length of `a ^ a` will be 0 (no qubits,\nempty expression).\n\n#### Estimating length of expression:\n\n```python\n# you can use builtin python's len to get estimated expression's length\nlength = len(a ^ b + 1)\n```\n\nPlease note that this is an **estimated** length as actual length may vary depending\noptimizer solutions.\n\n#### Debugging expression:\n\n```python\nprint(str(a ^ b + 1))\n```\n\nor, for jupyter:\n\n```python\na ^ b + 1\n```\n\nThis will print `(^ \"a(2)\" (+ 1 \"4(2)\"))` for `a` of length 2 and `b` of length 4.\n\n### Bitwise binary operations\n\n```python\nr = ~a\nr = a ^ b\nr = a | b\nr = a & b\n```\n\nYou can also do this with constants:\n\n```python\nr = 0b101 ^ a\nb |= 1\nr = b & 0b11\n```\n\n### Bit shifting\n\n```python\nr = a << 2\nr = b >> 3\n```\n\nPlease note that only constant distance shifting is supported at this time.\n\n### Arithmetic operations\n\n```python\nr = a + b\nr = 2 * a * b\n```\n\n### Ternary operations\n\nIf you want to emulate if statements, i.e.\n\n```python\nif cond:\n r = a + b\nelse:\n r = b & 0b11\n```\n\nyou can use ternary operators:\n\n```python\nr = cond.ternary(a + b, b & 0b11)\n```\n\nNote that cond must be an expression exactly 1 qubit long. You can\nachieve this by using bitwise and with 1.\n\n### Compiling\n\nLet's compile something:\n\n```python\nr = a ^ b + 3\n\n# internal circuit representation\ncirc = r.compile()\n\n# QuantumCircuit from qiskit\nqc = quantpiler.circuit_to_qiskit(circ)\n\n# let's draw our circuit\nqc.draw(\"mpl\")\n```\n\n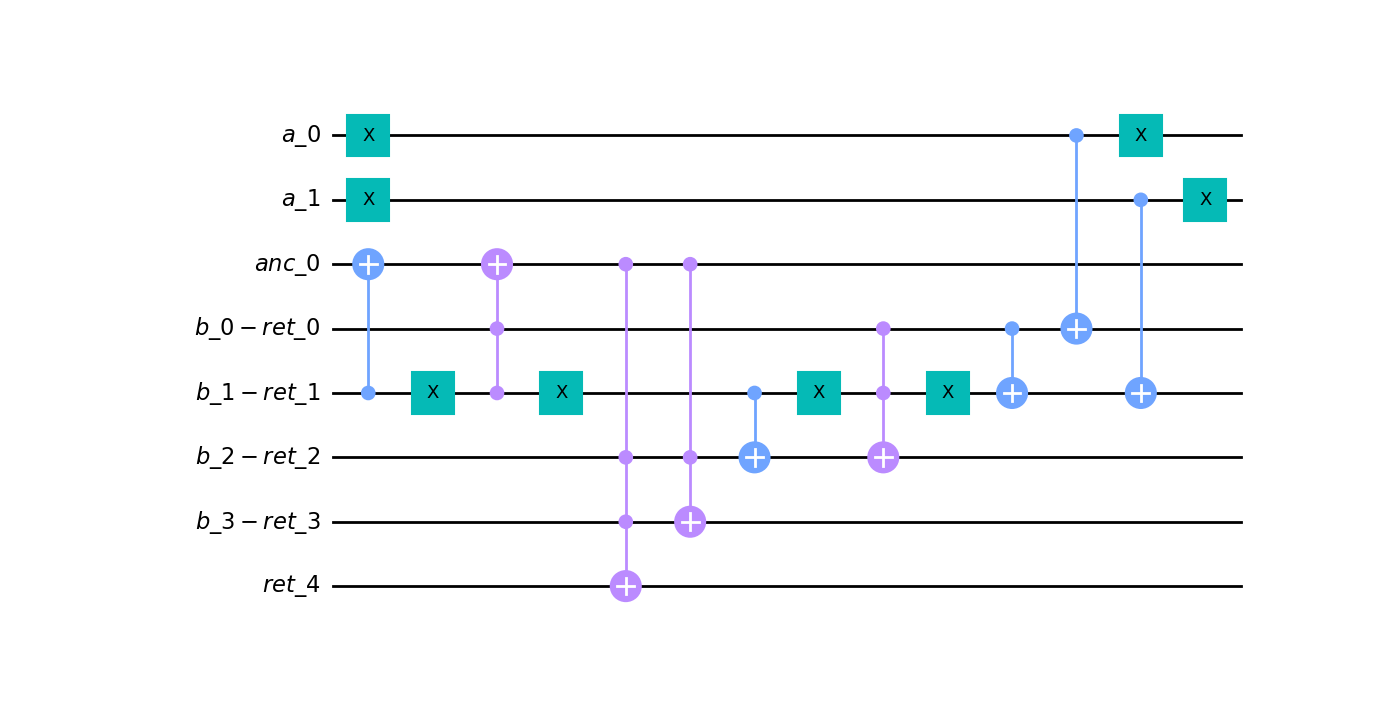\n\n",
"bugtrack_url": null,
"license": "AGPL-3.0-or-later",
"summary": "\u0421ompiler of classical algorithms into oracles for quantum computing",
"version": "0.3.5",
"project_urls": {
"Source Code": "https://github.com/averyanalex/quantpiler"
},
"split_keywords": [
"qiskit",
"quantum"
],
"urls": [
{
"comment_text": null,
"digests": {
"blake2b_256": "517a00eba2ee3b8dfbceace2de757a8b295e48b991fac01b2b8f1e9f80f30b4b",
"md5": "227c9a8426af6f0ddca2f8eb77d1320f",
"sha256": "b6721ff8b19047af2046fa134ad69b1fb1360ca1715ce6d933c344a8369fb4c9"
},
"downloads": -1,
"filename": "quantpiler-0.3.5-cp310-cp310-manylinux_2_28_x86_64.whl",
"has_sig": false,
"md5_digest": "227c9a8426af6f0ddca2f8eb77d1320f",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": ">=3.8",
"size": 1104872,
"upload_time": "2023-12-23T20:49:21",
"upload_time_iso_8601": "2023-12-23T20:49:21.839477Z",
"url": "https://files.pythonhosted.org/packages/51/7a/00eba2ee3b8dfbceace2de757a8b295e48b991fac01b2b8f1e9f80f30b4b/quantpiler-0.3.5-cp310-cp310-manylinux_2_28_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "2e8673e6feff03dd9026db70154760d24843b30b4e2ff07758b686a51a9c1a39",
"md5": "7e4704763b59771d22588445f4d8db13",
"sha256": "e662671ab7e011b5a8a308e9b5ed4b9a8bca3147925ae207da0a56e9af831751"
},
"downloads": -1,
"filename": "quantpiler-0.3.5-cp310-none-win32.whl",
"has_sig": false,
"md5_digest": "7e4704763b59771d22588445f4d8db13",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": ">=3.8",
"size": 466948,
"upload_time": "2023-12-23T20:49:23",
"upload_time_iso_8601": "2023-12-23T20:49:23.934847Z",
"url": "https://files.pythonhosted.org/packages/2e/86/73e6feff03dd9026db70154760d24843b30b4e2ff07758b686a51a9c1a39/quantpiler-0.3.5-cp310-none-win32.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "e04fe96c1c93ff9af30a5ca40ea515f1311033d75df16b5908f5f7bb548239c0",
"md5": "37130b168efa5c50ed9e01f73aa0d47b",
"sha256": "609f23537d48800f4dfcbe1a4b17f8f9f5e3bca588b00ad0bc2069f045451dc0"
},
"downloads": -1,
"filename": "quantpiler-0.3.5-cp310-none-win_amd64.whl",
"has_sig": false,
"md5_digest": "37130b168efa5c50ed9e01f73aa0d47b",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": ">=3.8",
"size": 516608,
"upload_time": "2023-12-23T20:49:25",
"upload_time_iso_8601": "2023-12-23T20:49:25.226423Z",
"url": "https://files.pythonhosted.org/packages/e0/4f/e96c1c93ff9af30a5ca40ea515f1311033d75df16b5908f5f7bb548239c0/quantpiler-0.3.5-cp310-none-win_amd64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "97aa5e340750cf9f45903a062fc3cd1808db1bf9eba7ecd4156611824eb2edbd",
"md5": "fe32dfbfb7ad4dde20b625bc1a2fba97",
"sha256": "01f6fd8f4152da243f9b540e63eeebf37355ec66d1c4f38cefdb552f299fc1f3"
},
"downloads": -1,
"filename": "quantpiler-0.3.5-cp311-cp311-manylinux_2_28_x86_64.whl",
"has_sig": false,
"md5_digest": "fe32dfbfb7ad4dde20b625bc1a2fba97",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": ">=3.8",
"size": 1104942,
"upload_time": "2023-12-23T20:49:27",
"upload_time_iso_8601": "2023-12-23T20:49:27.170588Z",
"url": "https://files.pythonhosted.org/packages/97/aa/5e340750cf9f45903a062fc3cd1808db1bf9eba7ecd4156611824eb2edbd/quantpiler-0.3.5-cp311-cp311-manylinux_2_28_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "c9e10fae8350dfa4e4f8487a91629125a82705af1643a99f1dcfe531db777f4a",
"md5": "c3701afe12774c97215ad59b4a77d8e1",
"sha256": "509d17f0a49f7f3fa551c1b8e9b6ce79537d00b1898671d98f2951224518d083"
},
"downloads": -1,
"filename": "quantpiler-0.3.5-cp311-none-win32.whl",
"has_sig": false,
"md5_digest": "c3701afe12774c97215ad59b4a77d8e1",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": ">=3.8",
"size": 466780,
"upload_time": "2023-12-23T20:49:29",
"upload_time_iso_8601": "2023-12-23T20:49:29.335792Z",
"url": "https://files.pythonhosted.org/packages/c9/e1/0fae8350dfa4e4f8487a91629125a82705af1643a99f1dcfe531db777f4a/quantpiler-0.3.5-cp311-none-win32.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "c0cfc3c784b8c198c683918ded03ac651ec465366d09e3edea69667bc58e2abf",
"md5": "75a61cec8379b2f19469643278deea4b",
"sha256": "24e6f2a56da1284d03c53f7c7e21a10243f6806de0bf3125a35072b252f8ee06"
},
"downloads": -1,
"filename": "quantpiler-0.3.5-cp311-none-win_amd64.whl",
"has_sig": false,
"md5_digest": "75a61cec8379b2f19469643278deea4b",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": ">=3.8",
"size": 516538,
"upload_time": "2023-12-23T20:49:31",
"upload_time_iso_8601": "2023-12-23T20:49:31.222119Z",
"url": "https://files.pythonhosted.org/packages/c0/cf/c3c784b8c198c683918ded03ac651ec465366d09e3edea69667bc58e2abf/quantpiler-0.3.5-cp311-none-win_amd64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "7331b86a60cb51dd6f521c7ed05d9de44bb39d0efd649cb1080bb5e30212e02f",
"md5": "383805863a103ea33acf5f690eb7e5bc",
"sha256": "856774670ce5c4f303498db961e3d28f6c1f0cd37d01d6b856a058f129f742e9"
},
"downloads": -1,
"filename": "quantpiler-0.3.5-cp312-cp312-manylinux_2_28_x86_64.whl",
"has_sig": false,
"md5_digest": "383805863a103ea33acf5f690eb7e5bc",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": ">=3.8",
"size": 1105511,
"upload_time": "2023-12-23T20:49:33",
"upload_time_iso_8601": "2023-12-23T20:49:33.090475Z",
"url": "https://files.pythonhosted.org/packages/73/31/b86a60cb51dd6f521c7ed05d9de44bb39d0efd649cb1080bb5e30212e02f/quantpiler-0.3.5-cp312-cp312-manylinux_2_28_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "5706b524ca12a254816004eb0781df796bebed89cfc180bec6718ccd789e7770",
"md5": "15fb0b277567a8a2336fb4058f7e9dde",
"sha256": "526a3a6517482ac4570fdff72a1456dd257a17dce2878fa708240f1c8c35f8d8"
},
"downloads": -1,
"filename": "quantpiler-0.3.5-cp312-none-win32.whl",
"has_sig": false,
"md5_digest": "15fb0b277567a8a2336fb4058f7e9dde",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": ">=3.8",
"size": 465003,
"upload_time": "2023-12-23T20:49:34",
"upload_time_iso_8601": "2023-12-23T20:49:34.472425Z",
"url": "https://files.pythonhosted.org/packages/57/06/b524ca12a254816004eb0781df796bebed89cfc180bec6718ccd789e7770/quantpiler-0.3.5-cp312-none-win32.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "423eff632fdaf068b808c708539da0948b62244d5cc53d7b206aa595dd143c1a",
"md5": "b903649cf835eb70c0c112dc2f61bb1b",
"sha256": "13cc96d33b503d4df44402bfef87bf283b48ee696fa081109692ab327e4c2e44"
},
"downloads": -1,
"filename": "quantpiler-0.3.5-cp312-none-win_amd64.whl",
"has_sig": false,
"md5_digest": "b903649cf835eb70c0c112dc2f61bb1b",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": ">=3.8",
"size": 521145,
"upload_time": "2023-12-23T20:49:36",
"upload_time_iso_8601": "2023-12-23T20:49:36.363309Z",
"url": "https://files.pythonhosted.org/packages/42/3e/ff632fdaf068b808c708539da0948b62244d5cc53d7b206aa595dd143c1a/quantpiler-0.3.5-cp312-none-win_amd64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "d2d98b6412e135ff391bad3378cd6719b0ad1ccdd6a8e6c217c712387b78bcd3",
"md5": "a821207ec5ab6f1ba66755eb46a607eb",
"sha256": "73ba24f431e47b4827654d9d6d3c083f4194fcc8d379287b8202ee51f304f427"
},
"downloads": -1,
"filename": "quantpiler-0.3.5-cp38-cp38-manylinux_2_28_x86_64.whl",
"has_sig": false,
"md5_digest": "a821207ec5ab6f1ba66755eb46a607eb",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": ">=3.8",
"size": 1104139,
"upload_time": "2023-12-23T20:49:37",
"upload_time_iso_8601": "2023-12-23T20:49:37.755362Z",
"url": "https://files.pythonhosted.org/packages/d2/d9/8b6412e135ff391bad3378cd6719b0ad1ccdd6a8e6c217c712387b78bcd3/quantpiler-0.3.5-cp38-cp38-manylinux_2_28_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "1e04bc3d09682551a9b0eb615bd7197b77934f98c7861831301ba224291c5dbf",
"md5": "aeae91f90956da4071c55a4125249563",
"sha256": "da71dd6dc59489640a5140d01b1602ae3578097219eb79219f22c33a27d74610"
},
"downloads": -1,
"filename": "quantpiler-0.3.5-cp38-none-win32.whl",
"has_sig": false,
"md5_digest": "aeae91f90956da4071c55a4125249563",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": ">=3.8",
"size": 467432,
"upload_time": "2023-12-23T20:49:39",
"upload_time_iso_8601": "2023-12-23T20:49:39.732431Z",
"url": "https://files.pythonhosted.org/packages/1e/04/bc3d09682551a9b0eb615bd7197b77934f98c7861831301ba224291c5dbf/quantpiler-0.3.5-cp38-none-win32.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "60e88e4a7331a1fd0c216980750f57301b672a5d1c05af2476aefa5d60e2181e",
"md5": "97039845a65f3a2144e5fe8ab424bf24",
"sha256": "bd7e696eae8982106949fddb327d37cc4f0bafba0fef755f12afe3682c28fcc6"
},
"downloads": -1,
"filename": "quantpiler-0.3.5-cp38-none-win_amd64.whl",
"has_sig": false,
"md5_digest": "97039845a65f3a2144e5fe8ab424bf24",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": ">=3.8",
"size": 516374,
"upload_time": "2023-12-23T20:49:41",
"upload_time_iso_8601": "2023-12-23T20:49:41.654911Z",
"url": "https://files.pythonhosted.org/packages/60/e8/8e4a7331a1fd0c216980750f57301b672a5d1c05af2476aefa5d60e2181e/quantpiler-0.3.5-cp38-none-win_amd64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "24b6c6a026f285a58b335b5dfb8cac50e2989da51981fa1e829763e852da183d",
"md5": "b695c77730a6fd9d863b9b1ba68b6f64",
"sha256": "fd12909d3172a81ba6b3c880fdd51b9422dd35618569f69b6c74af32ab53dd6b"
},
"downloads": -1,
"filename": "quantpiler-0.3.5-cp39-cp39-manylinux_2_28_x86_64.whl",
"has_sig": false,
"md5_digest": "b695c77730a6fd9d863b9b1ba68b6f64",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": ">=3.8",
"size": 1104471,
"upload_time": "2023-12-23T20:49:43",
"upload_time_iso_8601": "2023-12-23T20:49:43.517641Z",
"url": "https://files.pythonhosted.org/packages/24/b6/c6a026f285a58b335b5dfb8cac50e2989da51981fa1e829763e852da183d/quantpiler-0.3.5-cp39-cp39-manylinux_2_28_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "eaf1272858ff3cc43a81d8a86f5cf80c4915ad1284531d6238ad0636cbbf3f1d",
"md5": "14f7259a07e9d66684212f9fcc51ad97",
"sha256": "f25627729fa4b7b9c1f1ffab76f50c974f90ccfedab0586b510da656359f632e"
},
"downloads": -1,
"filename": "quantpiler-0.3.5-cp39-none-win32.whl",
"has_sig": false,
"md5_digest": "14f7259a07e9d66684212f9fcc51ad97",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": ">=3.8",
"size": 466839,
"upload_time": "2023-12-23T20:49:45",
"upload_time_iso_8601": "2023-12-23T20:49:45.492871Z",
"url": "https://files.pythonhosted.org/packages/ea/f1/272858ff3cc43a81d8a86f5cf80c4915ad1284531d6238ad0636cbbf3f1d/quantpiler-0.3.5-cp39-none-win32.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "8837b128a1098ab63a8721bf525ff44da1d69e1fd398770a09f99745f6674f99",
"md5": "ed8ce9195fa0835a1b5c95deb4deb9b6",
"sha256": "c57b3219a234386db2bcc55bbc3ec0a3f40828a61ed3bd0f44764f12f3e71200"
},
"downloads": -1,
"filename": "quantpiler-0.3.5-cp39-none-win_amd64.whl",
"has_sig": false,
"md5_digest": "ed8ce9195fa0835a1b5c95deb4deb9b6",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": ">=3.8",
"size": 517166,
"upload_time": "2023-12-23T20:49:47",
"upload_time_iso_8601": "2023-12-23T20:49:47.393136Z",
"url": "https://files.pythonhosted.org/packages/88/37/b128a1098ab63a8721bf525ff44da1d69e1fd398770a09f99745f6674f99/quantpiler-0.3.5-cp39-none-win_amd64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "37fa32eb2a4983b0278617d644215fb4767dee87f35d32416e6401190ba6710a",
"md5": "522727b4d04de7f5184b4f1b40123500",
"sha256": "661b72d1fcaddd376af8f0f02d91792cfef13c0646c1b6b7bea52e0c0458a021"
},
"downloads": -1,
"filename": "quantpiler-0.3.5-pp310-pypy310_pp73-manylinux_2_28_x86_64.whl",
"has_sig": false,
"md5_digest": "522727b4d04de7f5184b4f1b40123500",
"packagetype": "bdist_wheel",
"python_version": "pp310",
"requires_python": ">=3.8",
"size": 1102802,
"upload_time": "2023-12-23T20:49:48",
"upload_time_iso_8601": "2023-12-23T20:49:48.583464Z",
"url": "https://files.pythonhosted.org/packages/37/fa/32eb2a4983b0278617d644215fb4767dee87f35d32416e6401190ba6710a/quantpiler-0.3.5-pp310-pypy310_pp73-manylinux_2_28_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "9f005d9e0bcf5b2fb5b6401987a5d41720b437ed188af19f4012eb0de3865a18",
"md5": "d7ceb670ad96d7e8b04c73ca983293fe",
"sha256": "cc0ac3779b4fb5c5f36279d2182f035f8457e6ae80829b149c74d9464e7537c8"
},
"downloads": -1,
"filename": "quantpiler-0.3.5-pp38-pypy38_pp73-manylinux_2_28_x86_64.whl",
"has_sig": false,
"md5_digest": "d7ceb670ad96d7e8b04c73ca983293fe",
"packagetype": "bdist_wheel",
"python_version": "pp38",
"requires_python": ">=3.8",
"size": 1103705,
"upload_time": "2023-12-23T20:49:49",
"upload_time_iso_8601": "2023-12-23T20:49:49.848848Z",
"url": "https://files.pythonhosted.org/packages/9f/00/5d9e0bcf5b2fb5b6401987a5d41720b437ed188af19f4012eb0de3865a18/quantpiler-0.3.5-pp38-pypy38_pp73-manylinux_2_28_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "7d09033b6ccb6650b9c89f1c6e5ef08f85b1a5194722f42b1f0320875bcd32e0",
"md5": "acdf5878a1bf06f1a826e6f625c08e5a",
"sha256": "fa4c3ff0a8c2e017690b50531ed129f4688e6f80cfdf7de55142069558bfed8c"
},
"downloads": -1,
"filename": "quantpiler-0.3.5-pp39-pypy39_pp73-manylinux_2_28_x86_64.whl",
"has_sig": false,
"md5_digest": "acdf5878a1bf06f1a826e6f625c08e5a",
"packagetype": "bdist_wheel",
"python_version": "pp39",
"requires_python": ">=3.8",
"size": 1102875,
"upload_time": "2023-12-23T20:49:51",
"upload_time_iso_8601": "2023-12-23T20:49:51.182140Z",
"url": "https://files.pythonhosted.org/packages/7d/09/033b6ccb6650b9c89f1c6e5ef08f85b1a5194722f42b1f0320875bcd32e0/quantpiler-0.3.5-pp39-pypy39_pp73-manylinux_2_28_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "359e5431bc679b612802daf4cba52766327ece0624c5bb5014816343056ffef8",
"md5": "1aea4e51a457fa60f6d3f92a4c57a699",
"sha256": "ec91eb86061310c5c96143e3a2169fc5afb1a4ecefea8629e467e12efb2bf752"
},
"downloads": -1,
"filename": "quantpiler-0.3.5.tar.gz",
"has_sig": false,
"md5_digest": "1aea4e51a457fa60f6d3f92a4c57a699",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.8",
"size": 116380,
"upload_time": "2023-12-23T20:49:52",
"upload_time_iso_8601": "2023-12-23T20:49:52.579740Z",
"url": "https://files.pythonhosted.org/packages/35/9e/5431bc679b612802daf4cba52766327ece0624c5bb5014816343056ffef8/quantpiler-0.3.5.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-12-23 20:49:52",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "averyanalex",
"github_project": "quantpiler",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "quantpiler"
}