# quantum-viz
`quantum-viz` is the Python package companion of [quantum-viz.js](https://github.com/microsoft/quantum-viz.js), a JavaScript package that supports visualizing any arbitrary quantum gate, classical control logic and collapsed grouped blocks of gates using JSON-formatted input data. `quantum-viz` contains a Jupyter widget and will also include support for translating quantum circuits written in common quantum programming libraries to JSON using the `quantum-viz.js` JSON schema.
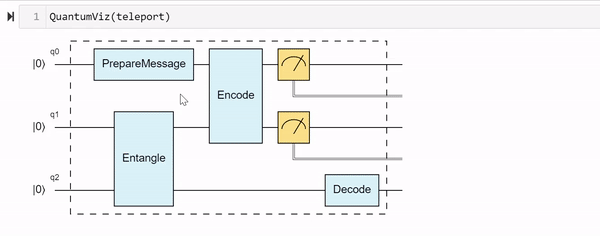
## Installation
You can install the *quantum-viz.js widget* via `pip` from PyPI:
```bash
pip install quantum-viz
```
## Example
To use the quantum-viz widget, run the below example code in a [Jupyter notebook](https://jupyter.org/) cell:
```python
from quantum_viz import Viewer
# Create a quantum circuit that prepares a Bell state
circuit = {
"qubits": [{ "id": 0 }, { "id": 1, "numChildren": 1 }],
"operations": [
{
"gate": 'H',
"targets": [{ "qId": 0 }],
},
{
"gate": 'X',
"isControlled": "True",
"controls": [{ "qId": 0 }],
"targets": [{ "qId": 1 }],
},
{
"gate": 'Measure',
"isMeasurement": "True",
"controls": [{ "qId": 1 }],
"targets": [{ "type": 1, "qId": 1, "cId": 0 }],
},
],
}
widget = Viewer(circuit)
widget # Display the widget
```
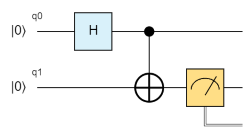
## Qiskit Integration
By installing the optional `[qiskit]` dependency, you can leverage Qiskit's `QuantumCircuit` APIs
to define the circuit and render it using the `Viewer` widget on Jupyter, for example:
```python
from qiskit import QuantumRegister, ClassicalRegister, QuantumCircuit
from quantum_viz import Viewer
qr = QuantumRegister(3, 'q')
anc = QuantumRegister(1, 'ancilla')
cr = ClassicalRegister(3, 'c')
qc = QuantumCircuit(qr, anc, cr)
qc.h(qr[0:3])
qc.x(anc[0])
qc.h(anc[0])
qc.cx(qr[0:3], anc[0])
qc.h(qr[0:3])
qc.barrier(qr)
qc.measure(qr, cr)
Viewer(qc)
```
Optionally, you can also import the `display` method from `quantum_viz.utils` to render the circuit on a new browser window:
```python
from quantum_viz.utils import display
display(qc)
```
## Contributing
Check out our [contributing guidelines](https://github.com/microsoft/quantum-viz.js/blob/main/quantum-viz/CONTRIBUTING.md) to find out how you can contribute to quantum-viz.
Raw data
{
"_id": null,
"home_page": "https://github.com/microsoft/quantum-viz.js",
"name": "quantum-viz",
"maintainer": "",
"docs_url": null,
"requires_python": ">=3.8,<4.0",
"maintainer_email": "",
"keywords": "",
"author": "Microsoft Corporation",
"author_email": "que-contacts@microsoft.com",
"download_url": "https://files.pythonhosted.org/packages/a1/d6/289988da97b49b6bf169e3eb75491a1c1f3c08d4966d0805d1a6e6903cc5/quantum-viz-1.0.5.tar.gz",
"platform": null,
"description": "# quantum-viz\n\n`quantum-viz` is the Python package companion of [quantum-viz.js](https://github.com/microsoft/quantum-viz.js), a JavaScript package that supports visualizing any arbitrary quantum gate, classical control logic and collapsed grouped blocks of gates using JSON-formatted input data. `quantum-viz` contains a Jupyter widget and will also include support for translating quantum circuits written in common quantum programming libraries to JSON using the `quantum-viz.js` JSON schema.\n\n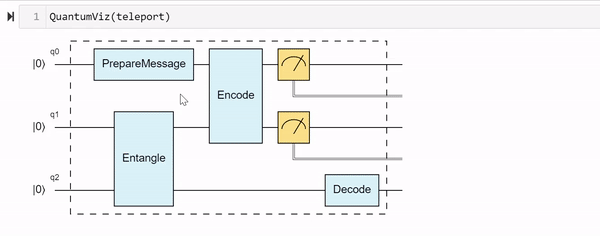\n\n## Installation\n\nYou can install the *quantum-viz.js widget* via `pip` from PyPI:\n\n```bash\npip install quantum-viz\n```\n\n## Example\n\nTo use the quantum-viz widget, run the below example code in a [Jupyter notebook](https://jupyter.org/) cell:\n\n```python\nfrom quantum_viz import Viewer\n\n# Create a quantum circuit that prepares a Bell state\ncircuit = {\n \"qubits\": [{ \"id\": 0 }, { \"id\": 1, \"numChildren\": 1 }],\n \"operations\": [\n {\n \"gate\": 'H',\n \"targets\": [{ \"qId\": 0 }],\n },\n {\n \"gate\": 'X',\n \"isControlled\": \"True\",\n \"controls\": [{ \"qId\": 0 }],\n \"targets\": [{ \"qId\": 1 }],\n },\n {\n \"gate\": 'Measure',\n \"isMeasurement\": \"True\",\n \"controls\": [{ \"qId\": 1 }],\n \"targets\": [{ \"type\": 1, \"qId\": 1, \"cId\": 0 }],\n },\n ],\n}\n\nwidget = Viewer(circuit)\nwidget # Display the widget\n```\n\n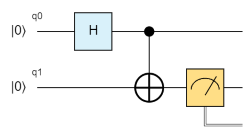\n\n## Qiskit Integration\n\nBy installing the optional `[qiskit]` dependency, you can leverage Qiskit's `QuantumCircuit` APIs\nto define the circuit and render it using the `Viewer` widget on Jupyter, for example:\n\n```python\nfrom qiskit import QuantumRegister, ClassicalRegister, QuantumCircuit\nfrom quantum_viz import Viewer\n\nqr = QuantumRegister(3, 'q')\nanc = QuantumRegister(1, 'ancilla')\ncr = ClassicalRegister(3, 'c')\nqc = QuantumCircuit(qr, anc, cr)\n\n\nqc.h(qr[0:3])\nqc.x(anc[0])\nqc.h(anc[0])\nqc.cx(qr[0:3], anc[0])\nqc.h(qr[0:3])\nqc.barrier(qr)\nqc.measure(qr, cr)\n\nViewer(qc)\n```\n\nOptionally, you can also import the `display` method from `quantum_viz.utils` to render the circuit on a new browser window:\n\n```python\nfrom quantum_viz.utils import display\ndisplay(qc)\n```\n\n## Contributing\n\nCheck out our [contributing guidelines](https://github.com/microsoft/quantum-viz.js/blob/main/quantum-viz/CONTRIBUTING.md) to find out how you can contribute to quantum-viz.\n\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "quantum-viz.js Python tools",
"version": "1.0.5",
"split_keywords": [],
"urls": [
{
"comment_text": "",
"digests": {
"md5": "c0755793235c2b37aef6ef31df000368",
"sha256": "f3df8132577c1640277b167949f7418cd766074a79ee20dca4bdc265dca7853b"
},
"downloads": -1,
"filename": "quantum_viz-1.0.5-py3-none-any.whl",
"has_sig": false,
"md5_digest": "c0755793235c2b37aef6ef31df000368",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.8,<4.0",
"size": 10467,
"upload_time": "2022-12-29T19:15:47",
"upload_time_iso_8601": "2022-12-29T19:15:47.506783Z",
"url": "https://files.pythonhosted.org/packages/5d/ee/11b61a3434aa0bf65fed834496a23235f8de63bd133261942f6c9d828797/quantum_viz-1.0.5-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"md5": "ccec0066d61c712abbca6ff13e401b1f",
"sha256": "0e6214317e5dcc370b732613e2bfb895b864d1740a2a9dd70c3e09551d75f6dd"
},
"downloads": -1,
"filename": "quantum-viz-1.0.5.tar.gz",
"has_sig": false,
"md5_digest": "ccec0066d61c712abbca6ff13e401b1f",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.8,<4.0",
"size": 10064,
"upload_time": "2022-12-29T19:15:49",
"upload_time_iso_8601": "2022-12-29T19:15:49.269301Z",
"url": "https://files.pythonhosted.org/packages/a1/d6/289988da97b49b6bf169e3eb75491a1c1f3c08d4966d0805d1a6e6903cc5/quantum-viz-1.0.5.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2022-12-29 19:15:49",
"github": true,
"gitlab": false,
"bitbucket": false,
"github_user": "microsoft",
"github_project": "quantum-viz.js",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "quantum-viz"
}