[](https://choosealicense.com/licenses/mit/)
[](https://badge.fury.io/py/rclone-python)
# rclone-python βοΈ
A python wrapper for rclone that makes rclone's functionality usable in python.
rclone needs to be installed on the system for the wrapper to work.
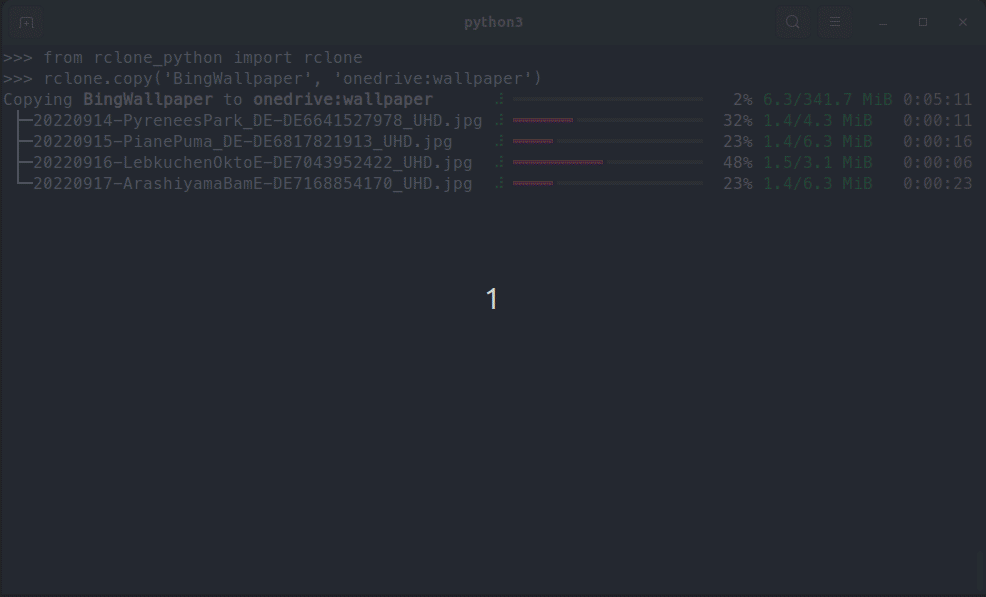
## Features βοΈ
- Copy, move and sync files between remotes
- Delete and prune files/directories
- List files in a directory including properties of the files.
- List available remotes.
- Generate hashes from files or validate them with their hashsum.
- Create new remotes
- Check available remotes
- Create and manage public links.
- Check the currently installed rclone versions and if updates are available.
## Installation πΎ
_rclone_python_ can be installed using pip
```shell
pip install rclone-python
```
or by cloning this repository and running from within the root of the project
```shell
pip install .
```
## How to use π‘
All functionally of this wrapper is accessible through `rclone`.
The following example checks if rclone is installed.
```python
from rclone_python import rclone
print(rclone.is_installed())
```
```console
True
```
### Create new remote
Create a new rclone remote connection with rclone's default client-id and client-secret.
```python
from rclone_python import rclone
from rclone_python.remote_types import RemoteTypes
rclone.create_remote('onedrive', RemoteTypes.onedrive)
```
Additionally, client-id and client-secret can be used with many cloud providers.
```python
from rclone_python import rclone
from rclone_python.remote_types import RemoteTypes
rclone.create_remote('onedrive', RemoteTypes.onedrive, client_id='YOUR_CLIENT_ID', client_secret='YOUR_CLIENT_SECRET')
```
### Copy
```python
from rclone_python import rclone
# copy all file in the test_dir on OneDrive to the local data folder.
rclone.copy('onedrive:data', 'data', ignore_existing=True, args=['--create-empty-src-dirs'])
```
```console
Copying onedrive:data to data β Έ βββββββββββββββββββΈβββββββββββββββββββββ 47% 110.0/236.5 MiB 0:00:04
ββvideo1.webm β Έ βββββββββββββΊβββββββββββββββββββββββββββ 31% 24.4/78.8 MiB 0:00:06
ββvideo2.webm β Έ βββββββββββββββββββΊβββββββββββββββββββββ 45% 35.5/78.8 MiB 0:00:03
ββvideo3.webm β Έ ββββββββββββββΈββββββββββββββββββββββββββ 35% 27.6/78.8 MiB 0:00:05
```
### Delete
Delete a file or a directory. When deleting a directory, only the files in the directory (and all it's subdirectories)
are deleted, but the folders remain.
```python
from rclone_python import rclone
# delete a specific file on onedrive
rclone.delete('onedrive:data/video1.mp4')
```
### Prune
```python
from rclone_python import rclone
# remove the entire test_dir folder (and all files contained in it and it's subdirectories) on onedrive
rclone.purge('onedrive:test_dir')
```
### Get Hash
```python
from rclone_python import rclone
from rclone_python.hash_types import HashTypes
print(rclone.hash(HashTypes.sha1, "box:data")
```
```console
{'video1.webm': '3ef08d895f25e8b7d84d3a1ac58f8f302e33058b', 'video3.webm': '3ef08d895f25e8b7d84d3a1ac58f8f302e33058b', 'video2.webm': '3ef08d895f25e8b7d84d3a1ac58f8f302e33058b'}
```
### Check
Checks the files in the source and destination match.
- "=" path means path was found in source and destination and was identical
- "-" path means path was missing on the source, so only in the destination
- "+" path means path was missing on the destination, so only in the source
- "*" path means path was present in source and destination but different.
- "!" path means there was an error reading or hashing the source or dest.
```python
from rclone_python import rclone
print(rclone.check("data", "box:data"))
```
```console
(False, [('*', 'video1.webm'), ('=', 'video2.webm'), ('=', 'video2.webm')])
```
## Custom Progressbar
You can use your own rich progressbar with all transfer operations.
This allows you to customize the columns to be displayed.
A list of all rich-progress columns can be found [here](https://rich.readthedocs.io/en/stable/progress.html#columns).
```python
from rclone_python import rclone
from rich.progress import (
Progress,
TextColumn,
BarColumn,
TaskProgressColumn,
TransferSpeedColumn,
)
pbar = Progress(
TextColumn("[progress.description]{task.description}"),
BarColumn(),
TaskProgressColumn(),
TransferSpeedColumn(),
)
rclone.copy("data", "box:rclone_test/data1", pbar=pbar)
```
```console
Copying data to data1 βββββββΈβββββββββββββββββββββββββββββββββ 17% 5.3 MB/s
ββvideo1.mp4 ββββββββββββββββΊββββββββββββββββββββββββ 38% 4.2 MB/s
ββvideo2.mp4 ββΈββββββββββββββββββββββββββββββββββββββ 5% 1.6 MB/s
ββanother.mp4 ββΈββββββββββββββββββββββββββββββββββββββ 4% 1.4 MB/s
```
## Set the log level
The log level can be set using:
```python
rclone.set_log_level(logging.DEBUG)
```
This will make the wrapper print the raw rclone progress.
## Star History
[](https://star-history.com/#Johannes11833/rclone_python&Date)
Raw data
{
"_id": null,
"home_page": "https://github.com/Johannes11833/rclone_python",
"name": "rclone-python",
"maintainer": null,
"docs_url": null,
"requires_python": ">=3.6",
"maintainer_email": null,
"keywords": "rclone, wrapper, cloud sync",
"author": "Johannes Gundlach",
"author_email": null,
"download_url": "https://files.pythonhosted.org/packages/e3/06/ad0ebd8d7a767934a797daa6950bc91f072603a73bb529556d18d17e3c54/rclone_python-0.1.21.tar.gz",
"platform": null,
"description": "[](https://choosealicense.com/licenses/mit/)\n[](https://badge.fury.io/py/rclone-python)\n\n# rclone-python \u2601\ufe0f\n\nA python wrapper for rclone that makes rclone's functionality usable in python.\nrclone needs to be installed on the system for the wrapper to work.\n\n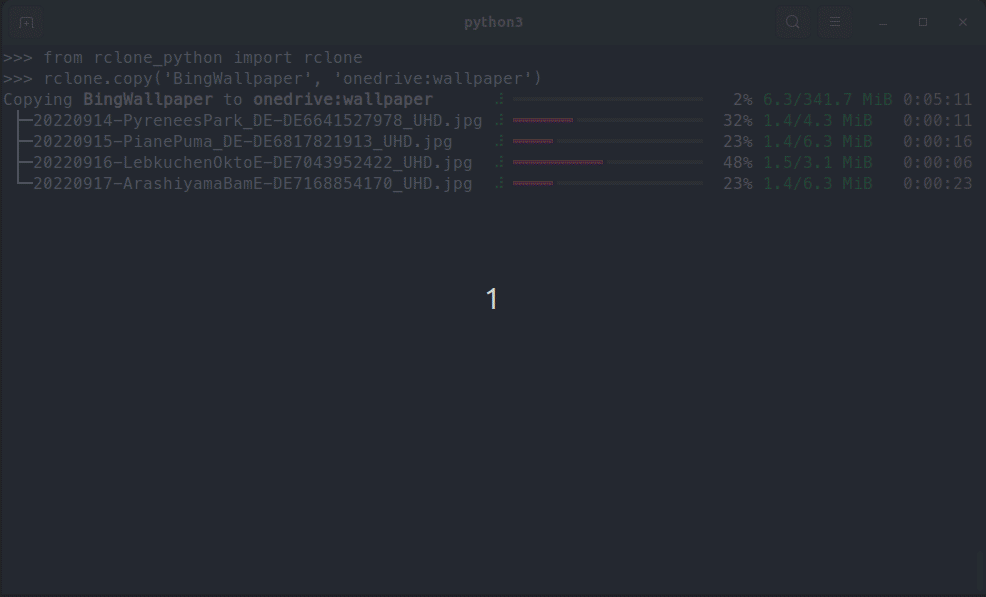\n\n## Features \u2692\ufe0f\n\n- Copy, move and sync files between remotes\n- Delete and prune files/directories\n- List files in a directory including properties of the files.\n- List available remotes.\n- Generate hashes from files or validate them with their hashsum.\n- Create new remotes\n- Check available remotes\n- Create and manage public links.\n- Check the currently installed rclone versions and if updates are available.\n\n## Installation \ud83d\udcbe\n\n_rclone_python_ can be installed using pip\n\n```shell\npip install rclone-python\n```\n\nor by cloning this repository and running from within the root of the project\n\n```shell\npip install .\n```\n\n## How to use \ud83d\udca1\n\nAll functionally of this wrapper is accessible through `rclone`.\nThe following example checks if rclone is installed.\n\n```python\nfrom rclone_python import rclone\n\nprint(rclone.is_installed())\n```\n\n```console\nTrue\n```\n### Create new remote\n\nCreate a new rclone remote connection with rclone's default client-id and client-secret.\n\n```python\nfrom rclone_python import rclone\nfrom rclone_python.remote_types import RemoteTypes\n\nrclone.create_remote('onedrive', RemoteTypes.onedrive)\n```\n\nAdditionally, client-id and client-secret can be used with many cloud providers.\n\n```python\nfrom rclone_python import rclone\nfrom rclone_python.remote_types import RemoteTypes\n\nrclone.create_remote('onedrive', RemoteTypes.onedrive, client_id='YOUR_CLIENT_ID', client_secret='YOUR_CLIENT_SECRET')\n```\n\n### Copy\n\n```python\nfrom rclone_python import rclone\n\n# copy all file in the test_dir on OneDrive to the local data folder.\nrclone.copy('onedrive:data', 'data', ignore_existing=True, args=['--create-empty-src-dirs'])\n```\n\n\n```console\nCopying onedrive:data to data \u2838 \u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2578\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501 47% 110.0/236.5 MiB 0:00:04\n \u251c\u2500video1.webm \u2838 \u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u257a\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501 31% 24.4/78.8 MiB 0:00:06\n \u251c\u2500video2.webm \u2838 \u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u257a\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501 45% 35.5/78.8 MiB 0:00:03\n \u2514\u2500video3.webm \u2838 \u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2578\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501 35% 27.6/78.8 MiB 0:00:05\n```\n\n### Delete\n\nDelete a file or a directory. When deleting a directory, only the files in the directory (and all it's subdirectories)\nare deleted, but the folders remain.\n\n```python\nfrom rclone_python import rclone\n\n# delete a specific file on onedrive\nrclone.delete('onedrive:data/video1.mp4')\n\n```\n\n### Prune\n\n```python\nfrom rclone_python import rclone\n\n# remove the entire test_dir folder (and all files contained in it and it's subdirectories) on onedrive\nrclone.purge('onedrive:test_dir')\n```\n\n### Get Hash\n```python\nfrom rclone_python import rclone\nfrom rclone_python.hash_types import HashTypes\n\nprint(rclone.hash(HashTypes.sha1, \"box:data\")\n```\n```console\n{'video1.webm': '3ef08d895f25e8b7d84d3a1ac58f8f302e33058b', 'video3.webm': '3ef08d895f25e8b7d84d3a1ac58f8f302e33058b', 'video2.webm': '3ef08d895f25e8b7d84d3a1ac58f8f302e33058b'}\n```\n\n### Check\nChecks the files in the source and destination match.\n - \"=\" path means path was found in source and destination and was identical\n - \"-\" path means path was missing on the source, so only in the destination\n - \"+\" path means path was missing on the destination, so only in the source\n - \"*\" path means path was present in source and destination but different.\n - \"!\" path means there was an error reading or hashing the source or dest.\n```python\nfrom rclone_python import rclone\n\nprint(rclone.check(\"data\", \"box:data\"))\n```\n```console\n(False, [('*', 'video1.webm'), ('=', 'video2.webm'), ('=', 'video2.webm')])\n```\n\n## Custom Progressbar\nYou can use your own rich progressbar with all transfer operations.\nThis allows you to customize the columns to be displayed.\nA list of all rich-progress columns can be found [here](https://rich.readthedocs.io/en/stable/progress.html#columns).\n\n```python\nfrom rclone_python import rclone\n\nfrom rich.progress import (\n Progress,\n TextColumn,\n BarColumn,\n TaskProgressColumn,\n TransferSpeedColumn,\n)\n\npbar = Progress(\n TextColumn(\"[progress.description]{task.description}\"),\n BarColumn(),\n TaskProgressColumn(),\n TransferSpeedColumn(),\n)\nrclone.copy(\"data\", \"box:rclone_test/data1\", pbar=pbar)\n```\n\n```console\nCopying data to data1 \u2501\u2501\u2501\u2501\u2501\u2501\u2578\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501 17% 5.3 MB/s \n \u251c\u2500video1.mp4 \u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u257a\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501 38% 4.2 MB/s \n \u251c\u2500video2.mp4 \u2501\u2578\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501 5% 1.6 MB/s\n \u2514\u2500another.mp4 \u2501\u2578\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501\u2501 4% 1.4 MB/s\n```\n\n## Set the log level\nThe log level can be set using: \n```python\nrclone.set_log_level(logging.DEBUG)\n```\nThis will make the wrapper print the raw rclone progress. \n\n\n## Star History\n\n[](https://star-history.com/#Johannes11833/rclone_python&Date)\n",
"bugtrack_url": null,
"license": null,
"summary": "A python wrapper for rclone.",
"version": "0.1.21",
"project_urls": {
"Homepage": "https://github.com/Johannes11833/rclone_python"
},
"split_keywords": [
"rclone",
" wrapper",
" cloud sync"
],
"urls": [
{
"comment_text": null,
"digests": {
"blake2b_256": "efc2a8830c22dd3f86a9b1a39fc3e4bc63fa62e98401ffaa25bb762669336292",
"md5": "f78850ad94a1f2109ce9d576fcc2ca31",
"sha256": "22860edcd0484bd375b34b4a821f9e9837588f8e277cc1b67326dd950b1a7144"
},
"downloads": -1,
"filename": "rclone_python-0.1.21-py3-none-any.whl",
"has_sig": false,
"md5_digest": "f78850ad94a1f2109ce9d576fcc2ca31",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.6",
"size": 14925,
"upload_time": "2025-01-28T13:40:50",
"upload_time_iso_8601": "2025-01-28T13:40:50.174660Z",
"url": "https://files.pythonhosted.org/packages/ef/c2/a8830c22dd3f86a9b1a39fc3e4bc63fa62e98401ffaa25bb762669336292/rclone_python-0.1.21-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "e306ad0ebd8d7a767934a797daa6950bc91f072603a73bb529556d18d17e3c54",
"md5": "2943061d16e5c74533177d2ebbe0cc9e",
"sha256": "c19c9496da38254879b505e58ef832a556b691bd08a897ee27f4e885dac25970"
},
"downloads": -1,
"filename": "rclone_python-0.1.21.tar.gz",
"has_sig": false,
"md5_digest": "2943061d16e5c74533177d2ebbe0cc9e",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.6",
"size": 21071,
"upload_time": "2025-01-28T13:40:51",
"upload_time_iso_8601": "2025-01-28T13:40:51.837926Z",
"url": "https://files.pythonhosted.org/packages/e3/06/ad0ebd8d7a767934a797daa6950bc91f072603a73bb529556d18d17e3c54/rclone_python-0.1.21.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2025-01-28 13:40:51",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "Johannes11833",
"github_project": "rclone_python",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"requirements": [
{
"name": "rich",
"specs": []
}
],
"lcname": "rclone-python"
}