Name | reactable JSON |
Version |
0.1.4
JSON |
| download |
home_page | None |
Summary | Interactive tables for Python. |
upload_time | 2024-11-22 18:28:28 |
maintainer | None |
docs_url | None |
author | None |
requires_python | >=3.9 |
license | None |
keywords |
tables
|
VCS |
 |
bugtrack_url |
|
requirements |
No requirements were recorded.
|
Travis-CI |
No Travis.
|
coveralls test coverage |
No coveralls.
|
# reactable-py
reactable generates interactive tables in Python.
It's a port of the R package [reactable](https://github.com/glin/reactable) by [@glin](https://github.com/glin).
See these handy documentation pages:
- [📚 User guide](https://machow.github.io/reactable-py/get-started)
- [🧩 Examples](https://machow.github.io/reactable-py/demos/)
## Features
- **controls**: sorting, filtering, and pagination.
- **structure**: grouping, aggregating, expanding rows.
- **format**: represent numbers, dates, currencies.
- **style**: conditional styling for headers, cell values, and more.
## Installing
```bash
pip install reactable
```
## Basic use
Use with jupyter notebooks or quarto documents (.qmd) to display tables.
```python
from reactable import Reactable, embed_css
from reactable.data import sleep
embed_css()
Reactable(sleep)
```
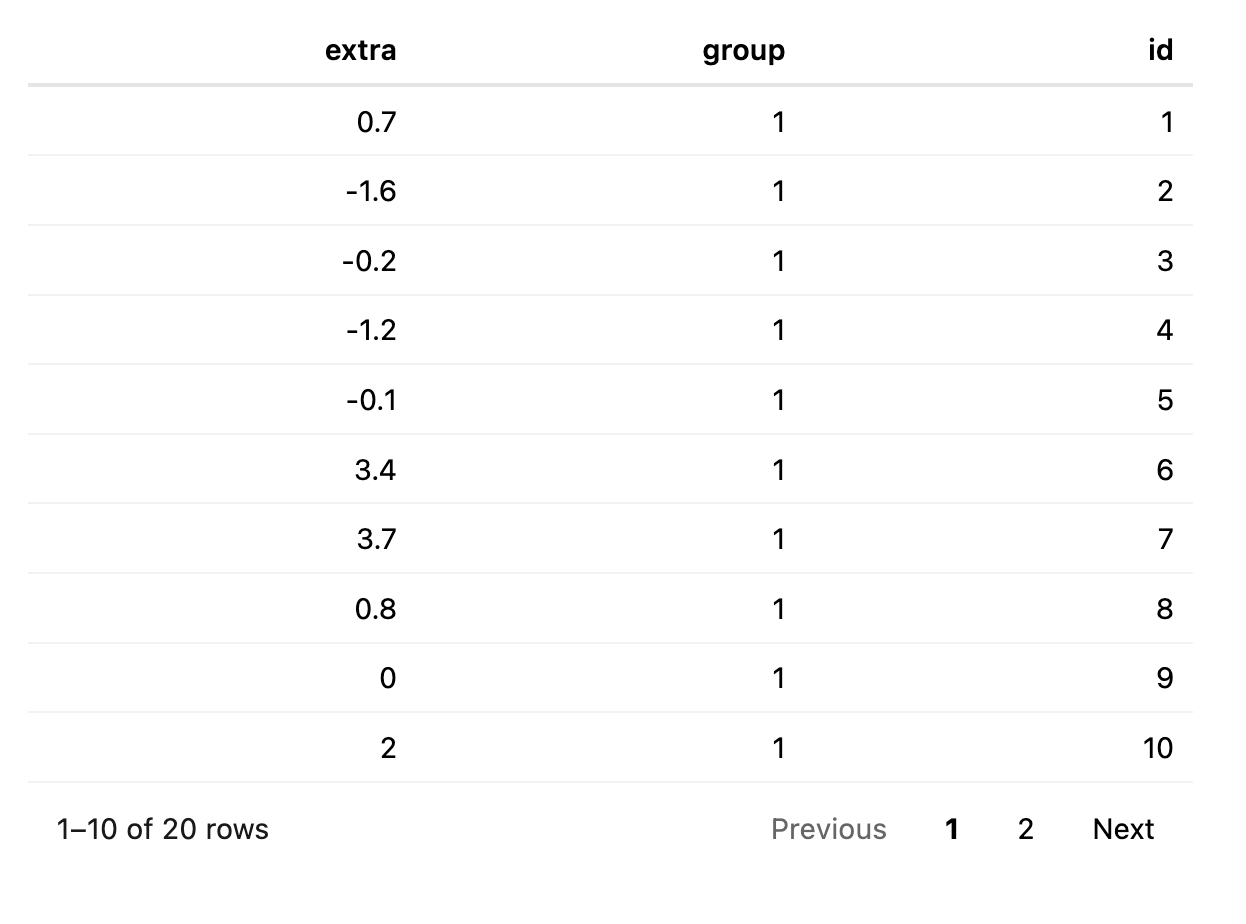
## Learn more
**Essentials**
- [Code basics](https://machow.github.io/reactable-py/get-started/code-structure.html)
- [Displaying tables](https://machow.github.io/reactable-py/get-started/display-export.html)
**Controls**
- [Sorting](https://machow.github.io/reactable-py/get-started/controls-sorting.html)
- [Filtering](https://machow.github.io/reactable-py/get-started/controls-filtering.html)
- [Searching](https://machow.github.io/reactable-py/get-started/controls-searching.html)
- [Pagination](https://machow.github.io/reactable-py/get-started/controls-pagination.html)
- [Column resizing](https://machow.github.io/reactable-py/get-started/controls-resizing.html)
- [Cell click actions](https://machow.github.io/reactable-py/get-started/controls-click-actions.html)
**Structure**
- [Column headers](https://machow.github.io/reactable-py/get-started/structure-headers.html)
- [Row groups and aggregation](https://machow.github.io/reactable-py/get-started/structure-grouping.html)
- [Expandable details](https://machow.github.io/reactable-py/get-started/structure-details.html)
- [Footers](https://machow.github.io/reactable-py/get-started/structure-footers.html)
- [Rownames](https://machow.github.io/reactable-py/get-started/structure-rownames.html)
**Format**
- [Column formatting](https://machow.github.io/reactable-py/get-started/format-columns.html)
- [Column aggregated cells](https://machow.github.io/reactable-py/get-started/format-aggregated.html)
- [Rendering cells](https://machow.github.io/reactable-py/get-started/format-cell.html)
- [Rendering header and footer](https://machow.github.io/reactable-py/get-started/format-header-footer.html)
- [Rendering details](https://machow.github.io/reactable-py/get-started/format-details.html)
**Style**
- [Table styling](https://machow.github.io/reactable-py/get-started/style-table.html)
- [Conditional styling (python)](https://machow.github.io/reactable-py/get-started/style-conditional.html)
- [Custom sort indicators](https://machow.github.io/reactable-py/get-started/style-custom-sort-indicators.html)
- [Theming](https://machow.github.io/reactable-py/get-started/style-theming.html)
**Extra**
- [Javascript formatters](https://machow.github.io/reactable-py/get-started/format-custom-rendering.html)
- [Javascript styling](https://machow.github.io/reactable-py/get-started/style-conditional-js.html)
- [Javascript filters](https://machow.github.io/reactable-py/get-started/extra-advanced-filters.html)
- [Using reactable with htmltools](https://machow.github.io/reactable-py/get-started/extra-htmltools.html)
Raw data
{
"_id": null,
"home_page": null,
"name": "reactable",
"maintainer": null,
"docs_url": null,
"requires_python": ">=3.9",
"maintainer_email": null,
"keywords": "tables",
"author": null,
"author_email": "Michael Chow <michael.chow@posit.co>",
"download_url": "https://files.pythonhosted.org/packages/b4/ab/06a24782da9509147e0258ada4cd4ba05c2c25eaacab373c4d8428fa3186/reactable-0.1.4.tar.gz",
"platform": null,
"description": "# reactable-py\n\nreactable generates interactive tables in Python.\nIt's a port of the R package [reactable](https://github.com/glin/reactable) by [@glin](https://github.com/glin).\n\nSee these handy documentation pages:\n\n- [\ud83d\udcda User guide](https://machow.github.io/reactable-py/get-started)\n- [\ud83e\udde9 Examples](https://machow.github.io/reactable-py/demos/)\n\n## Features\n\n- **controls**: sorting, filtering, and pagination.\n- **structure**: grouping, aggregating, expanding rows.\n- **format**: represent numbers, dates, currencies.\n- **style**: conditional styling for headers, cell values, and more.\n\n## Installing\n\n```bash\npip install reactable\n```\n\n## Basic use\n\nUse with jupyter notebooks or quarto documents (.qmd) to display tables.\n\n```python\nfrom reactable import Reactable, embed_css\nfrom reactable.data import sleep\n\nembed_css()\n\nReactable(sleep)\n```\n\n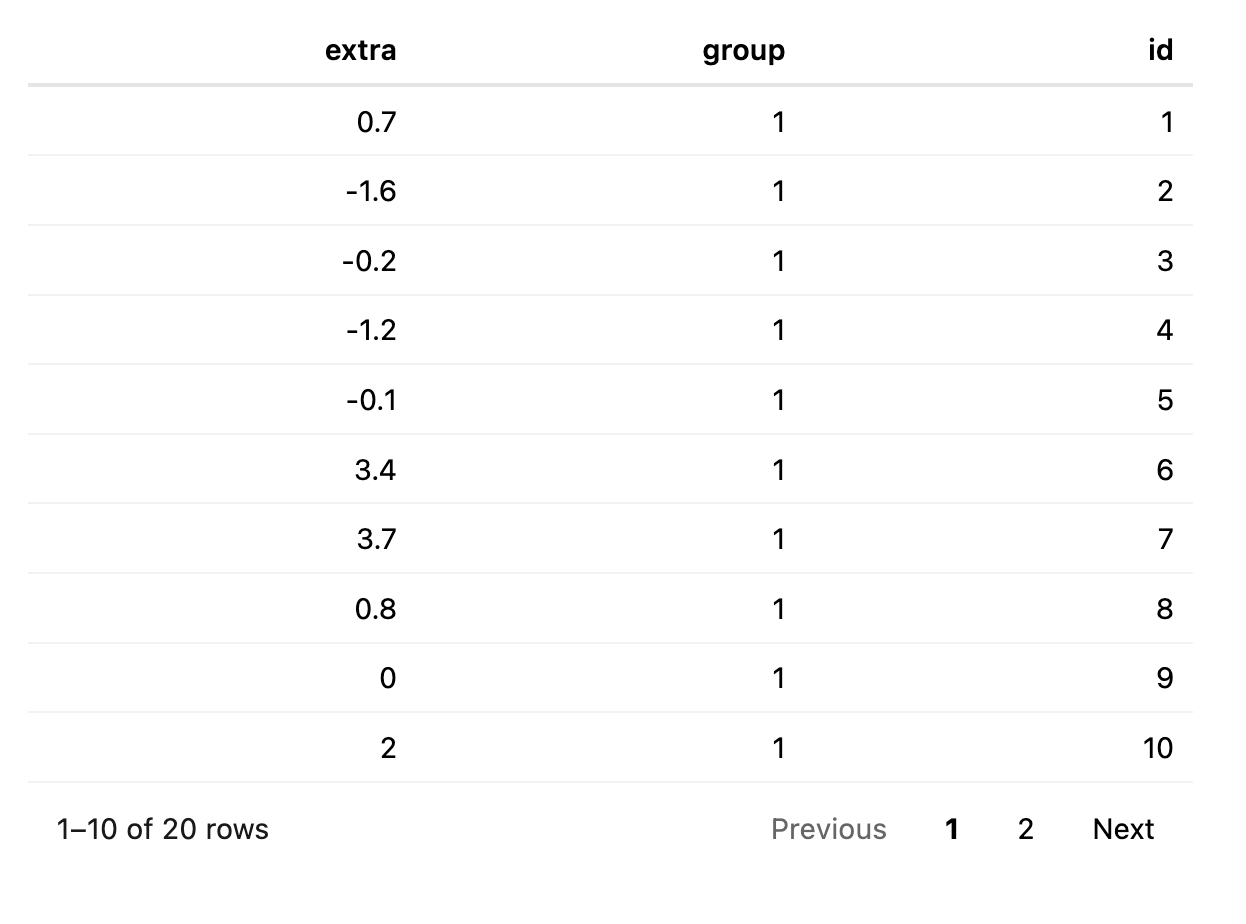\n\n## Learn more\n\n**Essentials**\n\n- [Code basics](https://machow.github.io/reactable-py/get-started/code-structure.html)\n- [Displaying tables](https://machow.github.io/reactable-py/get-started/display-export.html)\n\n**Controls**\n\n- [Sorting](https://machow.github.io/reactable-py/get-started/controls-sorting.html)\n- [Filtering](https://machow.github.io/reactable-py/get-started/controls-filtering.html)\n- [Searching](https://machow.github.io/reactable-py/get-started/controls-searching.html)\n- [Pagination](https://machow.github.io/reactable-py/get-started/controls-pagination.html)\n- [Column resizing](https://machow.github.io/reactable-py/get-started/controls-resizing.html)\n- [Cell click actions](https://machow.github.io/reactable-py/get-started/controls-click-actions.html)\n\n**Structure**\n\n- [Column headers](https://machow.github.io/reactable-py/get-started/structure-headers.html)\n- [Row groups and aggregation](https://machow.github.io/reactable-py/get-started/structure-grouping.html)\n- [Expandable details](https://machow.github.io/reactable-py/get-started/structure-details.html)\n- [Footers](https://machow.github.io/reactable-py/get-started/structure-footers.html)\n- [Rownames](https://machow.github.io/reactable-py/get-started/structure-rownames.html)\n\n**Format**\n\n- [Column formatting](https://machow.github.io/reactable-py/get-started/format-columns.html)\n- [Column aggregated cells](https://machow.github.io/reactable-py/get-started/format-aggregated.html)\n- [Rendering cells](https://machow.github.io/reactable-py/get-started/format-cell.html)\n- [Rendering header and footer](https://machow.github.io/reactable-py/get-started/format-header-footer.html)\n- [Rendering details](https://machow.github.io/reactable-py/get-started/format-details.html)\n\n**Style**\n\n- [Table styling](https://machow.github.io/reactable-py/get-started/style-table.html)\n- [Conditional styling (python)](https://machow.github.io/reactable-py/get-started/style-conditional.html)\n- [Custom sort indicators](https://machow.github.io/reactable-py/get-started/style-custom-sort-indicators.html)\n- [Theming](https://machow.github.io/reactable-py/get-started/style-theming.html)\n\n**Extra**\n\n- [Javascript formatters](https://machow.github.io/reactable-py/get-started/format-custom-rendering.html)\n- [Javascript styling](https://machow.github.io/reactable-py/get-started/style-conditional-js.html)\n- [Javascript filters](https://machow.github.io/reactable-py/get-started/extra-advanced-filters.html)\n- [Using reactable with htmltools](https://machow.github.io/reactable-py/get-started/extra-htmltools.html)\n",
"bugtrack_url": null,
"license": null,
"summary": "Interactive tables for Python.",
"version": "0.1.4",
"project_urls": {
"documentation": "https://machow.github.io/reactable-py",
"homepage": "https://github.com/machow/reactable-py"
},
"split_keywords": [
"tables"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "7a444baa041175bf010d40a07a2d12ea2f4d854fa7f1df16ad9d44571a192488",
"md5": "343aeb3d98b8ce6f59292ad496e07cd5",
"sha256": "105f36821dd388dbe1f178d67ca4703c990b9a1fae3196ab04abc70b250e6f4d"
},
"downloads": -1,
"filename": "reactable-0.1.4-py3-none-any.whl",
"has_sig": false,
"md5_digest": "343aeb3d98b8ce6f59292ad496e07cd5",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.9",
"size": 116064,
"upload_time": "2024-11-22T18:28:26",
"upload_time_iso_8601": "2024-11-22T18:28:26.626051Z",
"url": "https://files.pythonhosted.org/packages/7a/44/4baa041175bf010d40a07a2d12ea2f4d854fa7f1df16ad9d44571a192488/reactable-0.1.4-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "b4ab06a24782da9509147e0258ada4cd4ba05c2c25eaacab373c4d8428fa3186",
"md5": "5bb08e3ed02417c7c522622a0d9f04b6",
"sha256": "06faeb3d8ddcefd7ee3d869e3cec43fbaf8c25bb0a7629189184285bfd1c788c"
},
"downloads": -1,
"filename": "reactable-0.1.4.tar.gz",
"has_sig": false,
"md5_digest": "5bb08e3ed02417c7c522622a0d9f04b6",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.9",
"size": 937856,
"upload_time": "2024-11-22T18:28:28",
"upload_time_iso_8601": "2024-11-22T18:28:28.476991Z",
"url": "https://files.pythonhosted.org/packages/b4/ab/06a24782da9509147e0258ada4cd4ba05c2c25eaacab373c4d8428fa3186/reactable-0.1.4.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-11-22 18:28:28",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "machow",
"github_project": "reactable-py",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "reactable"
}