# Rocket-FFT
[](https://pypi.org/project/rocket-fft/)
[](https://opensource.org/licenses/BSD-3-Clause)
[](https://pypi.org/project/rocket-fft/)
[](https://pypi.org/project/rocket-fft/)
[](https://pypi.org/project/rocket-fft/)
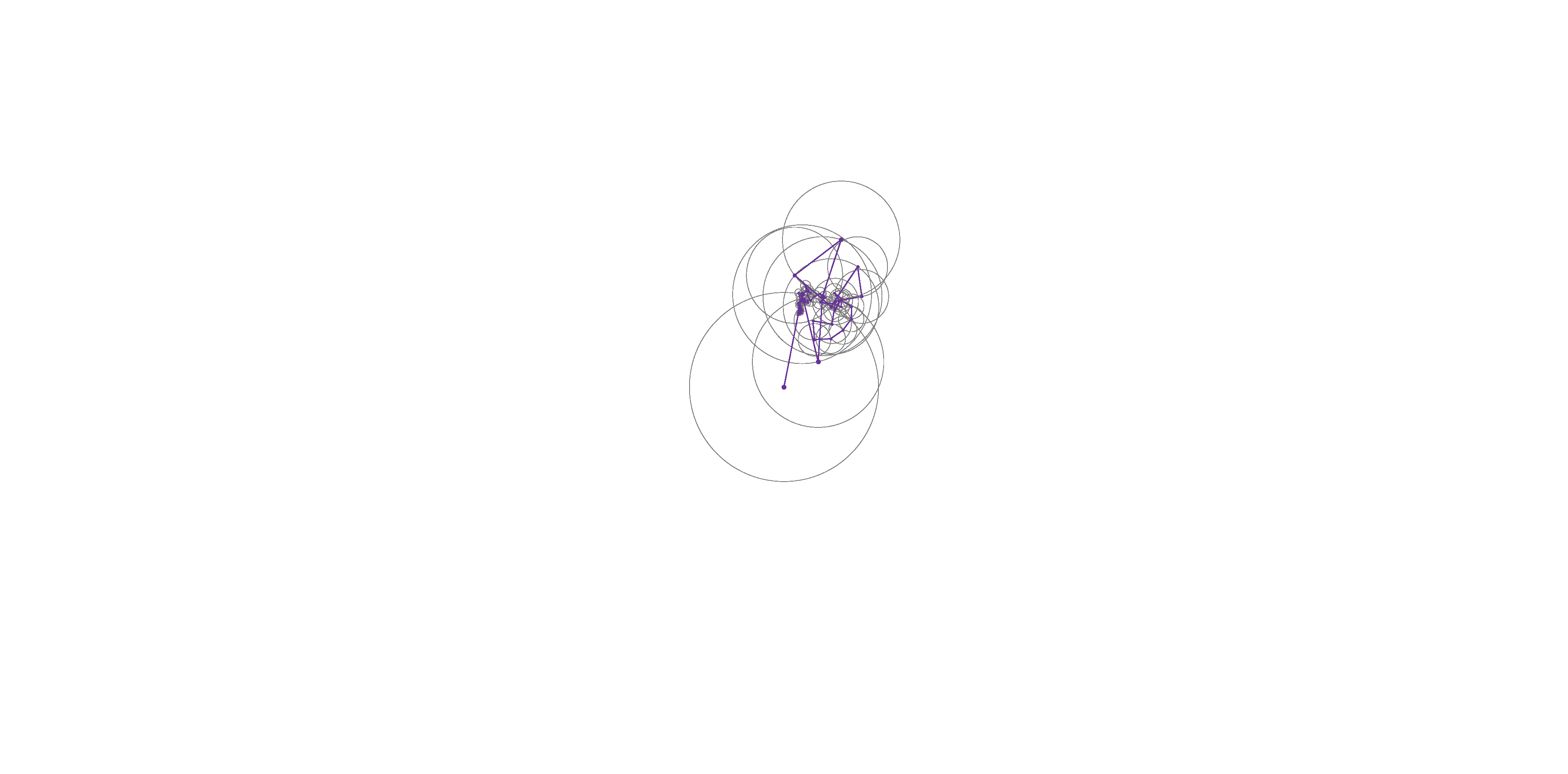
Rocket-FFT makes [Numba](https://numba.pydata.org/) aware of `numpy.fft` and `scipy.fft`. It takes its name from the [PocketFFT](https://github.com/mreineck/pocketfft) Fast Fourier Transformation library that powers it, and Numba's goal of making your scientific Python code blazingly fast - like a rocket.
## Getting Started
The easiest way to get Rocket-FFT is to:
```
$ pip install rocket-fft
```
Alternatively, you can build it from source:
```
$ git clone https://github.com/styfenschaer/rocket-fft.git
$ cd rocket-fft
$ python setup.py install
```
The latter requires a C++ compiler compatible with your Python installation.
Once installed successfully, the following will work (no import required):
```python
import numba as nb
import numpy as np
@nb.njit
def jit_fft(x):
return np.fft.fft(x)
a = np.array([1, 6, 1, 8, 0, 3, 3, 9])
jit_fft(a)
```
## Performance Tip
Rocket-FFT makes extensive use of Numba's polymorphic dispatching to achieve both flexible function signatures similar to SciPy and NumPy, and low compilation times. Compilation takes only a few hundred milliseconds in most cases. Calls with default arguments follow a fast path and compile fastest.
## NumPy-like and SciPy-like interfaces
NumPy and SciPy show subtle differences in how they convert types<sup>1</sup> and handle the `axes` argument in some functions<sup>2</sup>. Rocket-FFT implements both ways and lets its users choose between them.
You can set the interface by using the `scipy_like` or `numpy_like` function from the `rocket_fft` namespace:
```python
from rocket_fft import numpy_like, scipy_like
numpy_like()
```
Both functions can be used regardless of whether SciPy is installed<sup>3</sup>. By default, Rocket-FFT uses the SciPy-like interface if SciPy is installed, and the NumPy-like interface otherwise. Note that the interface cannot be changed after the compilation of Rocket-FFT's internals.
<sup>1</sup>NumPy converts all types to either `float64` or `complex128` whereas SciPy takes a more fine-grained approach
<br/>
<sup>2</sup>NumPy allows duplicate axes in `fft2`, `ifft2`, `fftn` and `ifftn`, whereas SciPy doesn't
<br/>
<sup>3</sup>SciPy is an optional runtime dependency
## Low-Level Interface
Rocket-FFT also provides a low-level interface to the PocketFFT library. Using the low-level interface can significantly reduce compile time, minimize overhead and give more flexibility to the user. It also provides some functions that are not available through the SciPy-like and NumPy-like interfaces. You can import its functions from the `rocket_fft` namespace:
```python
from rocket_fft import c2c, dct, ...
```
The low-level interface includes the following functions:
```python
def c2c(ain: NDArray[c8] | NDArray[c16], aout: NDArray[c8] | NDArray[c16], axes: NDArray[i8], forward: b1, fct: f4 | f8, nthreads: i8) -> None: ...
def r2c(ain: NDArray[f4] | NDArray[f8], aout: NDArray[c8] | NDArray[c16], axes: NDArray[i8], forward: b1, fct: f4 | f8, nthreads: i8) -> None: ...
def c2r(ain: NDArray[c8] | NDArray[c16], aout: NDArray[f4] | NDArray[f8], axes: NDArray[i8], forward: b1, fct: f4 | f8, nthreads: i8) -> None: ...
def c2c_sym(ain: NDArray[f4] | NDArray[f8], aout: NDArray[c8] | NDArray[c16], axes: NDArray[i8], forward: b1, fct: f4 | f8, nthreads: i8) -> None: ...
def dst(ain: NDArray[f4] | NDArray[f8], aout: NDArray[f4] | NDArray[f8], axes: NDArray[i8], type: i8, fct: f4 | f8, ortho: b1, nthreads: i8) -> None: ...
def dct(ain: NDArray[f4] | NDArray[f8], aout: NDArray[f4] | NDArray[f8], axes: NDArray[i8], type: i8, fct: f4 | f8, ortho: b1, nthreads: i8) -> None: ...
def r2r_separable_hartley(ain: NDArray[f4] | NDArray[f8], aout: NDArray[f4] | NDArray[f8], axes: NDArray[i8], fct: f4 | f8, nthreads: i8) -> None: ...
def r2r_genuine_hartley(ain: NDArray[f4] | NDArray[f8], aout: NDArray[f4] | NDArray[f8], axes: NDArray[i8], fct: f4 | f8, nthreads: i8) -> None: ...
def r2r_fftpack(ain: NDArray[f4] | NDArray[f8], aout: NDArray[f4] | NDArray[f8], axes: NDArray[i8], real2hermitian: b1, forward: b1, fct: f4 | f8, nthreads: i8) -> None: ...
def good_size(target: i8, real: b1) -> i8: ...
```
Note that the low-level interface provides a lower level of safety and convenience compared to the SciPy-like and NumPy-like interfaces.
There is almost no safety net, and it is up to the user to ensure proper usage. You may want to consult the original [PocketFFT](https://github.com/mreineck/pocketfft) C++ implementation before using it.
Raw data
{
"_id": null,
"home_page": "https://github.com/styfenschaer/rocket-fft",
"name": "rocket-fft",
"maintainer": "",
"docs_url": null,
"requires_python": "",
"maintainer_email": "",
"keywords": "FFT,Fourier,Numba,SciPy,NumPy",
"author": "Styfen Sch\u00e4r",
"author_email": "styfen.schaer.blog@gmail.com",
"download_url": "https://files.pythonhosted.org/packages/7a/52/b2447835d93fee2424bc5e7b1a5f1a9a8c75c27fd954ee3b81d581bb889e/rocket-fft-0.2.5.tar.gz",
"platform": null,
"description": "# Rocket-FFT\n[](https://pypi.org/project/rocket-fft/)\n[](https://opensource.org/licenses/BSD-3-Clause)\n[](https://pypi.org/project/rocket-fft/)\n[](https://pypi.org/project/rocket-fft/)\n[](https://pypi.org/project/rocket-fft/)\n\n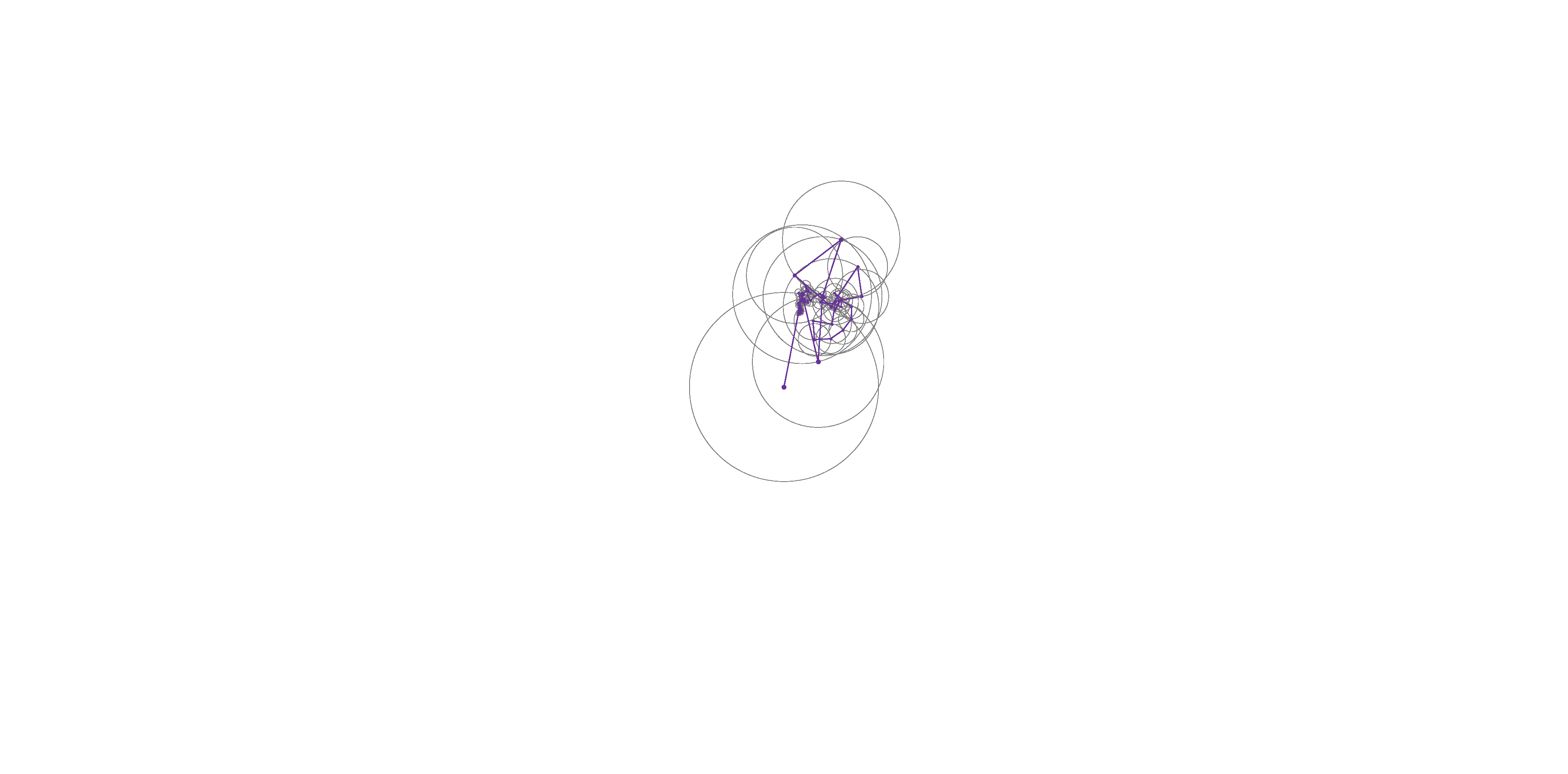\n\nRocket-FFT makes [Numba](https://numba.pydata.org/) aware of `numpy.fft` and `scipy.fft`. It takes its name from the [PocketFFT](https://github.com/mreineck/pocketfft) Fast Fourier Transformation library that powers it, and Numba's goal of making your scientific Python code blazingly fast - like a rocket. \n\n## Getting Started\nThe easiest way to get Rocket-FFT is to:\n```\n$ pip install rocket-fft\n```\nAlternatively, you can build it from source:\n```\n$ git clone https://github.com/styfenschaer/rocket-fft.git\n$ cd rocket-fft\n$ python setup.py install\n``` \nThe latter requires a C++ compiler compatible with your Python installation.\n\nOnce installed successfully, the following will work (no import required):\n```python\nimport numba as nb\nimport numpy as np\n\n@nb.njit\ndef jit_fft(x):\n return np.fft.fft(x)\n\na = np.array([1, 6, 1, 8, 0, 3, 3, 9])\njit_fft(a)\n```\n\n## Performance Tip\nRocket-FFT makes extensive use of Numba's polymorphic dispatching to achieve both flexible function signatures similar to SciPy and NumPy, and low compilation times. Compilation takes only a few hundred milliseconds in most cases. Calls with default arguments follow a fast path and compile fastest.\n\n## NumPy-like and SciPy-like interfaces\nNumPy and SciPy show subtle differences in how they convert types<sup>1</sup> and handle the `axes` argument in some functions<sup>2</sup>. Rocket-FFT implements both ways and lets its users choose between them.\n\nYou can set the interface by using the `scipy_like` or `numpy_like` function from the `rocket_fft` namespace:\n```python\nfrom rocket_fft import numpy_like, scipy_like\n\nnumpy_like()\n```\nBoth functions can be used regardless of whether SciPy is installed<sup>3</sup>. By default, Rocket-FFT uses the SciPy-like interface if SciPy is installed, and the NumPy-like interface otherwise. Note that the interface cannot be changed after the compilation of Rocket-FFT's internals.\n\n<sup>1</sup>NumPy converts all types to either `float64` or `complex128` whereas SciPy takes a more fine-grained approach\n<br/>\n<sup>2</sup>NumPy allows duplicate axes in `fft2`, `ifft2`, `fftn` and `ifftn`, whereas SciPy doesn't\n<br/>\n<sup>3</sup>SciPy is an optional runtime dependency\n\n## Low-Level Interface\nRocket-FFT also provides a low-level interface to the PocketFFT library. Using the low-level interface can significantly reduce compile time, minimize overhead and give more flexibility to the user. It also provides some functions that are not available through the SciPy-like and NumPy-like interfaces. You can import its functions from the `rocket_fft` namespace:\n```python\nfrom rocket_fft import c2c, dct, ...\n```\nThe low-level interface includes the following functions:\n```python\ndef c2c(ain: NDArray[c8] | NDArray[c16], aout: NDArray[c8] | NDArray[c16], axes: NDArray[i8], forward: b1, fct: f4 | f8, nthreads: i8) -> None: ...\ndef r2c(ain: NDArray[f4] | NDArray[f8], aout: NDArray[c8] | NDArray[c16], axes: NDArray[i8], forward: b1, fct: f4 | f8, nthreads: i8) -> None: ...\ndef c2r(ain: NDArray[c8] | NDArray[c16], aout: NDArray[f4] | NDArray[f8], axes: NDArray[i8], forward: b1, fct: f4 | f8, nthreads: i8) -> None: ...\ndef c2c_sym(ain: NDArray[f4] | NDArray[f8], aout: NDArray[c8] | NDArray[c16], axes: NDArray[i8], forward: b1, fct: f4 | f8, nthreads: i8) -> None: ...\ndef dst(ain: NDArray[f4] | NDArray[f8], aout: NDArray[f4] | NDArray[f8], axes: NDArray[i8], type: i8, fct: f4 | f8, ortho: b1, nthreads: i8) -> None: ...\ndef dct(ain: NDArray[f4] | NDArray[f8], aout: NDArray[f4] | NDArray[f8], axes: NDArray[i8], type: i8, fct: f4 | f8, ortho: b1, nthreads: i8) -> None: ...\ndef r2r_separable_hartley(ain: NDArray[f4] | NDArray[f8], aout: NDArray[f4] | NDArray[f8], axes: NDArray[i8], fct: f4 | f8, nthreads: i8) -> None: ...\ndef r2r_genuine_hartley(ain: NDArray[f4] | NDArray[f8], aout: NDArray[f4] | NDArray[f8], axes: NDArray[i8], fct: f4 | f8, nthreads: i8) -> None: ...\ndef r2r_fftpack(ain: NDArray[f4] | NDArray[f8], aout: NDArray[f4] | NDArray[f8], axes: NDArray[i8], real2hermitian: b1, forward: b1, fct: f4 | f8, nthreads: i8) -> None: ...\ndef good_size(target: i8, real: b1) -> i8: ...\n```\nNote that the low-level interface provides a lower level of safety and convenience compared to the SciPy-like and NumPy-like interfaces. \nThere is almost no safety net, and it is up to the user to ensure proper usage. You may want to consult the original [PocketFFT](https://github.com/mreineck/pocketfft) C++ implementation before using it.\n",
"bugtrack_url": null,
"license": "BSD",
"summary": "Rocket-FFT extends Numba by scipy.fft and numpy.fft",
"version": "0.2.5",
"project_urls": {
"Download": "https://github.com/styfenschaer/rocket-fft",
"Homepage": "https://github.com/styfenschaer/rocket-fft"
},
"split_keywords": [
"fft",
"fourier",
"numba",
"scipy",
"numpy"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "212838a38940b97d2ecb47dda2d46f3df72d546086ecada8523634d4dc4d24f4",
"md5": "2b2420db95f2e8f6769255c492722ea6",
"sha256": "b752e2b0337afe138f1bf0cd46c4d391964c480aeaf42ddf97366d68b8e5e412"
},
"downloads": -1,
"filename": "rocket_fft-0.2.5-cp310-cp310-macosx_10_9_x86_64.whl",
"has_sig": false,
"md5_digest": "2b2420db95f2e8f6769255c492722ea6",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": null,
"size": 290342,
"upload_time": "2024-03-15T09:43:11",
"upload_time_iso_8601": "2024-03-15T09:43:11.401873Z",
"url": "https://files.pythonhosted.org/packages/21/28/38a38940b97d2ecb47dda2d46f3df72d546086ecada8523634d4dc4d24f4/rocket_fft-0.2.5-cp310-cp310-macosx_10_9_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "a93d10b2061d7d07b5965251503d85144b5a6307e6a99d3fea945b18ba40e43d",
"md5": "d91251aaa5422b068db6d175e63cbaa0",
"sha256": "ee839203b95010bf62f09d77cd722025a16596de5da4d87b44f650238d5ab0d2"
},
"downloads": -1,
"filename": "rocket_fft-0.2.5-cp310-cp310-macosx_11_0_arm64.whl",
"has_sig": false,
"md5_digest": "d91251aaa5422b068db6d175e63cbaa0",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": null,
"size": 250232,
"upload_time": "2024-03-15T09:43:12",
"upload_time_iso_8601": "2024-03-15T09:43:12.828526Z",
"url": "https://files.pythonhosted.org/packages/a9/3d/10b2061d7d07b5965251503d85144b5a6307e6a99d3fea945b18ba40e43d/rocket_fft-0.2.5-cp310-cp310-macosx_11_0_arm64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "561bff754484e83be4f4e720828f5b47f3ba7026dc092a30d61c54bbec200177",
"md5": "df8662d0d269a0cce78f660e2e47c110",
"sha256": "dfdb1abd653e2ef831fec8d73fc4996b9ae8a22f2ef9054a100814ad4fe08462"
},
"downloads": -1,
"filename": "rocket_fft-0.2.5-cp310-cp310-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"has_sig": false,
"md5_digest": "df8662d0d269a0cce78f660e2e47c110",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": null,
"size": 2132997,
"upload_time": "2024-03-15T09:43:14",
"upload_time_iso_8601": "2024-03-15T09:43:14.149077Z",
"url": "https://files.pythonhosted.org/packages/56/1b/ff754484e83be4f4e720828f5b47f3ba7026dc092a30d61c54bbec200177/rocket_fft-0.2.5-cp310-cp310-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "5374bbdc3260a60a52c8485f1c178a32af2b64faa66d0b2626ca943c5ef8d98c",
"md5": "6770e7ae46b98c7b946abcd17e4c2cdd",
"sha256": "1c2c90a47ead16a00751d232aacf0ddcb66e37222770aab9e90b5551d6c3a39e"
},
"downloads": -1,
"filename": "rocket_fft-0.2.5-cp310-cp310-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"has_sig": false,
"md5_digest": "6770e7ae46b98c7b946abcd17e4c2cdd",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": null,
"size": 2233682,
"upload_time": "2024-03-15T09:43:15",
"upload_time_iso_8601": "2024-03-15T09:43:15.583737Z",
"url": "https://files.pythonhosted.org/packages/53/74/bbdc3260a60a52c8485f1c178a32af2b64faa66d0b2626ca943c5ef8d98c/rocket_fft-0.2.5-cp310-cp310-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "5f7e53c88cf0a8ae4eade287fad38efedb120318db1245873625e10f228cd118",
"md5": "92f8c332d388e456c4d7c1e91fcb2e67",
"sha256": "dada3fab0ef686a31ff627f2e28635feb8d2ccc198dc14259673e413a6d0d2da"
},
"downloads": -1,
"filename": "rocket_fft-0.2.5-cp310-cp310-win_amd64.whl",
"has_sig": false,
"md5_digest": "92f8c332d388e456c4d7c1e91fcb2e67",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": null,
"size": 192697,
"upload_time": "2024-03-15T09:43:16",
"upload_time_iso_8601": "2024-03-15T09:43:16.898089Z",
"url": "https://files.pythonhosted.org/packages/5f/7e/53c88cf0a8ae4eade287fad38efedb120318db1245873625e10f228cd118/rocket_fft-0.2.5-cp310-cp310-win_amd64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "ec8c44b8afb623c7055aedd0ef4f4b8af5f6b19d07cce8b307639b3b596cdcb8",
"md5": "573132fc71a765e7ddd511804da0063b",
"sha256": "61f3e0ab86409638348cdcb0c7ec3c81d6c1358c67ee5ff34959accad38289f7"
},
"downloads": -1,
"filename": "rocket_fft-0.2.5-cp311-cp311-macosx_10_9_x86_64.whl",
"has_sig": false,
"md5_digest": "573132fc71a765e7ddd511804da0063b",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": null,
"size": 290346,
"upload_time": "2024-03-15T09:43:18",
"upload_time_iso_8601": "2024-03-15T09:43:18.765751Z",
"url": "https://files.pythonhosted.org/packages/ec/8c/44b8afb623c7055aedd0ef4f4b8af5f6b19d07cce8b307639b3b596cdcb8/rocket_fft-0.2.5-cp311-cp311-macosx_10_9_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "5faa8e2403114bf03bba8dd7513ff9419c822fc9193e846e98b756a5c95c8538",
"md5": "f6610581fe07589285cc713a2980053c",
"sha256": "e28a8a381c785b528ef23d96139aeefffc9f549d5edb13a9fbddd7a62c636f8a"
},
"downloads": -1,
"filename": "rocket_fft-0.2.5-cp311-cp311-macosx_11_0_arm64.whl",
"has_sig": false,
"md5_digest": "f6610581fe07589285cc713a2980053c",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": null,
"size": 250229,
"upload_time": "2024-03-15T09:43:20",
"upload_time_iso_8601": "2024-03-15T09:43:20.476727Z",
"url": "https://files.pythonhosted.org/packages/5f/aa/8e2403114bf03bba8dd7513ff9419c822fc9193e846e98b756a5c95c8538/rocket_fft-0.2.5-cp311-cp311-macosx_11_0_arm64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "701d3ff2f912d5b132aa2acb6e48077cc27026bcde01b4705502800e000a487e",
"md5": "8832fe462d336b6bb66dcd60907a5563",
"sha256": "7db08c74814462c50375dc890ad884ad97100ccc243da02f45b1ea9592b3fa11"
},
"downloads": -1,
"filename": "rocket_fft-0.2.5-cp311-cp311-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"has_sig": false,
"md5_digest": "8832fe462d336b6bb66dcd60907a5563",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": null,
"size": 2133282,
"upload_time": "2024-03-15T09:43:21",
"upload_time_iso_8601": "2024-03-15T09:43:21.788365Z",
"url": "https://files.pythonhosted.org/packages/70/1d/3ff2f912d5b132aa2acb6e48077cc27026bcde01b4705502800e000a487e/rocket_fft-0.2.5-cp311-cp311-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "2235636fead6e189dfac59296dc1cbd08d991813819d5fd6b0b73cb9857f6c9e",
"md5": "6cd8c4e4dc70445d262ac0e01eb61aa6",
"sha256": "3c343b9cd17ce9da60022256aabfb39588628de150210250e3f7c75adca369dd"
},
"downloads": -1,
"filename": "rocket_fft-0.2.5-cp311-cp311-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"has_sig": false,
"md5_digest": "6cd8c4e4dc70445d262ac0e01eb61aa6",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": null,
"size": 2233730,
"upload_time": "2024-03-15T09:43:23",
"upload_time_iso_8601": "2024-03-15T09:43:23.261348Z",
"url": "https://files.pythonhosted.org/packages/22/35/636fead6e189dfac59296dc1cbd08d991813819d5fd6b0b73cb9857f6c9e/rocket_fft-0.2.5-cp311-cp311-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "7178e676232bd61624ef9c63ef337e132982f6136a13e96e6cc455c1b9435fca",
"md5": "acc9dd9d44c1e68188643abcdafea728",
"sha256": "7c2300964ab8aa69a77c42a2495d26c2e19b0a601a26c5565e4cbaeda980625c"
},
"downloads": -1,
"filename": "rocket_fft-0.2.5-cp311-cp311-win_amd64.whl",
"has_sig": false,
"md5_digest": "acc9dd9d44c1e68188643abcdafea728",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": null,
"size": 192694,
"upload_time": "2024-03-15T09:43:24",
"upload_time_iso_8601": "2024-03-15T09:43:24.567671Z",
"url": "https://files.pythonhosted.org/packages/71/78/e676232bd61624ef9c63ef337e132982f6136a13e96e6cc455c1b9435fca/rocket_fft-0.2.5-cp311-cp311-win_amd64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "abd07b18c050d772e599158158447dcbf3d32c9fb6e6a431222430c403656f6a",
"md5": "678a8bb80f36ae2916d3093e05422d04",
"sha256": "c76f189535e2cda8970a4679966ac320f7486071f0a59a8f13b48884c44325a0"
},
"downloads": -1,
"filename": "rocket_fft-0.2.5-cp312-cp312-macosx_10_9_x86_64.whl",
"has_sig": false,
"md5_digest": "678a8bb80f36ae2916d3093e05422d04",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": null,
"size": 290369,
"upload_time": "2024-03-15T09:43:26",
"upload_time_iso_8601": "2024-03-15T09:43:26.347880Z",
"url": "https://files.pythonhosted.org/packages/ab/d0/7b18c050d772e599158158447dcbf3d32c9fb6e6a431222430c403656f6a/rocket_fft-0.2.5-cp312-cp312-macosx_10_9_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "df5ca7799631012d51c8d63ff391e922f7214300c41c70eda01aa0c1e553c092",
"md5": "23d6c3c893aff317850b34e32c611ade",
"sha256": "7b63e6e8e7b925e9a8cce75bf30559c6507fe7ce92156acbe42d5481ada81d1c"
},
"downloads": -1,
"filename": "rocket_fft-0.2.5-cp312-cp312-macosx_11_0_arm64.whl",
"has_sig": false,
"md5_digest": "23d6c3c893aff317850b34e32c611ade",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": null,
"size": 250228,
"upload_time": "2024-03-15T09:43:28",
"upload_time_iso_8601": "2024-03-15T09:43:28.256677Z",
"url": "https://files.pythonhosted.org/packages/df/5c/a7799631012d51c8d63ff391e922f7214300c41c70eda01aa0c1e553c092/rocket_fft-0.2.5-cp312-cp312-macosx_11_0_arm64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "902eab61028c898c9de1addb1a3bb7ca685ba4c49e8f280e8d16cbb57d71f529",
"md5": "b07a8994749f51e7a06ff567050d69d5",
"sha256": "b38877592f7704fc328d47103e7cf4fdca24f59e2b1c97b3e773c6e60580af4a"
},
"downloads": -1,
"filename": "rocket_fft-0.2.5-cp312-cp312-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"has_sig": false,
"md5_digest": "b07a8994749f51e7a06ff567050d69d5",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": null,
"size": 2130703,
"upload_time": "2024-03-15T09:43:29",
"upload_time_iso_8601": "2024-03-15T09:43:29.639557Z",
"url": "https://files.pythonhosted.org/packages/90/2e/ab61028c898c9de1addb1a3bb7ca685ba4c49e8f280e8d16cbb57d71f529/rocket_fft-0.2.5-cp312-cp312-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "6a7b33383d05947c8d2ff0464a38d56222539e56c6c5d2495cbc4dde7c52b724",
"md5": "3a18e9dea8ef5319eb2f46b189fe16d0",
"sha256": "beaf5a064af017f940d24a0b9a94cb4696c8722fda9c1726fea645a41344ea6e"
},
"downloads": -1,
"filename": "rocket_fft-0.2.5-cp312-cp312-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"has_sig": false,
"md5_digest": "3a18e9dea8ef5319eb2f46b189fe16d0",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": null,
"size": 2232653,
"upload_time": "2024-03-15T09:43:30",
"upload_time_iso_8601": "2024-03-15T09:43:30.993760Z",
"url": "https://files.pythonhosted.org/packages/6a/7b/33383d05947c8d2ff0464a38d56222539e56c6c5d2495cbc4dde7c52b724/rocket_fft-0.2.5-cp312-cp312-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "4c83b18b211ec172bb20dd5dcf77aee7a2517edf5db795be893a2545e8d73ab8",
"md5": "8f472751b4d045bd6aba4abe6ec15677",
"sha256": "d8a6b6b0ad5b7769aab9b37cad2f17f14f778bff205ba46aee6e71ca1f273662"
},
"downloads": -1,
"filename": "rocket_fft-0.2.5-cp312-cp312-win_amd64.whl",
"has_sig": false,
"md5_digest": "8f472751b4d045bd6aba4abe6ec15677",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": null,
"size": 192707,
"upload_time": "2024-03-15T09:43:32",
"upload_time_iso_8601": "2024-03-15T09:43:32.291508Z",
"url": "https://files.pythonhosted.org/packages/4c/83/b18b211ec172bb20dd5dcf77aee7a2517edf5db795be893a2545e8d73ab8/rocket_fft-0.2.5-cp312-cp312-win_amd64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "162a1e042302d199f6634b3e31721aea4cc6db5064faa4166d043880426a7646",
"md5": "a4d536e5bc1da50a7b34241b258a3f63",
"sha256": "d9eddb716b8601d6728c621c94b1a5f1ab8053763e9010e684320d3bef9361b4"
},
"downloads": -1,
"filename": "rocket_fft-0.2.5-cp38-cp38-macosx_10_9_x86_64.whl",
"has_sig": false,
"md5_digest": "a4d536e5bc1da50a7b34241b258a3f63",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": null,
"size": 290324,
"upload_time": "2024-03-15T09:43:33",
"upload_time_iso_8601": "2024-03-15T09:43:33.479413Z",
"url": "https://files.pythonhosted.org/packages/16/2a/1e042302d199f6634b3e31721aea4cc6db5064faa4166d043880426a7646/rocket_fft-0.2.5-cp38-cp38-macosx_10_9_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "324b9d4f5a02f24b36bcc529abc6d01d579ec3a939831024e541d91234603df0",
"md5": "cca64c56d6b30e67b1485b3573b0c737",
"sha256": "19c4f11f3fa3f0acb2f81d7c5f16c1077998ce04ad1c9a069e0b6877906cd8d6"
},
"downloads": -1,
"filename": "rocket_fft-0.2.5-cp38-cp38-macosx_11_0_arm64.whl",
"has_sig": false,
"md5_digest": "cca64c56d6b30e67b1485b3573b0c737",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": null,
"size": 250235,
"upload_time": "2024-03-15T09:43:34",
"upload_time_iso_8601": "2024-03-15T09:43:34.693397Z",
"url": "https://files.pythonhosted.org/packages/32/4b/9d4f5a02f24b36bcc529abc6d01d579ec3a939831024e541d91234603df0/rocket_fft-0.2.5-cp38-cp38-macosx_11_0_arm64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "4d47a3fb7878ccefbabcd1d49cf4cee0d6a4a03b888d4577fae7b7999ad4f6b1",
"md5": "d9ef781ecddf12698fe42f9dd66e45ac",
"sha256": "63a19be485d95d3497c32913d87328d5427ffdb78f871398bb060f632346548c"
},
"downloads": -1,
"filename": "rocket_fft-0.2.5-cp38-cp38-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"has_sig": false,
"md5_digest": "d9ef781ecddf12698fe42f9dd66e45ac",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": null,
"size": 2132819,
"upload_time": "2024-03-15T09:43:35",
"upload_time_iso_8601": "2024-03-15T09:43:35.981662Z",
"url": "https://files.pythonhosted.org/packages/4d/47/a3fb7878ccefbabcd1d49cf4cee0d6a4a03b888d4577fae7b7999ad4f6b1/rocket_fft-0.2.5-cp38-cp38-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "0419c70c17de2e1c0c4d86594b9dab318edc6d019ab8c630f16dd5533ad6247c",
"md5": "7cdce7e17062f9771f252bf2091af3ff",
"sha256": "cc2c62bc5943795cae010d61efe7b2383c00814e7f0f8e0678ffb515756bbb4b"
},
"downloads": -1,
"filename": "rocket_fft-0.2.5-cp38-cp38-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"has_sig": false,
"md5_digest": "7cdce7e17062f9771f252bf2091af3ff",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": null,
"size": 2233512,
"upload_time": "2024-03-15T09:43:37",
"upload_time_iso_8601": "2024-03-15T09:43:37.507458Z",
"url": "https://files.pythonhosted.org/packages/04/19/c70c17de2e1c0c4d86594b9dab318edc6d019ab8c630f16dd5533ad6247c/rocket_fft-0.2.5-cp38-cp38-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "feb7e2020f135c3511b98a20fe754db24e9c91af08a38c53c87f69ba81b3a8a1",
"md5": "bfbec5a0a6f59123a196567c4e6fd60b",
"sha256": "6dd1f1a04b56f258d7f34d6d1bb9815be5c563400a81609c997e10ea77e466a1"
},
"downloads": -1,
"filename": "rocket_fft-0.2.5-cp38-cp38-win_amd64.whl",
"has_sig": false,
"md5_digest": "bfbec5a0a6f59123a196567c4e6fd60b",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": null,
"size": 192691,
"upload_time": "2024-03-15T09:43:38",
"upload_time_iso_8601": "2024-03-15T09:43:38.851248Z",
"url": "https://files.pythonhosted.org/packages/fe/b7/e2020f135c3511b98a20fe754db24e9c91af08a38c53c87f69ba81b3a8a1/rocket_fft-0.2.5-cp38-cp38-win_amd64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "538b9019b669fdf3229ce3ac63aad1b599014f89b3f9997a8b6adbdb3a644a1b",
"md5": "9edf1687e5f33901146f96968fa345c5",
"sha256": "ae4ed289f68fb9d30386b427d0d886b6bcacd6831340b693e72ee098b72864c6"
},
"downloads": -1,
"filename": "rocket_fft-0.2.5-cp39-cp39-macosx_10_9_x86_64.whl",
"has_sig": false,
"md5_digest": "9edf1687e5f33901146f96968fa345c5",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": null,
"size": 290330,
"upload_time": "2024-03-15T09:43:40",
"upload_time_iso_8601": "2024-03-15T09:43:40.103302Z",
"url": "https://files.pythonhosted.org/packages/53/8b/9019b669fdf3229ce3ac63aad1b599014f89b3f9997a8b6adbdb3a644a1b/rocket_fft-0.2.5-cp39-cp39-macosx_10_9_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "ab5e6f7b4e6f32133c7654fd4a415bff251932b87938c9fc04a62d0ff8f0f033",
"md5": "e88d525f50510760ed4945737fb1f543",
"sha256": "0c51219c3cb38fa7c9ff8641642ac8a5de22f9fa033252bbc2ea557bf34e9637"
},
"downloads": -1,
"filename": "rocket_fft-0.2.5-cp39-cp39-macosx_11_0_arm64.whl",
"has_sig": false,
"md5_digest": "e88d525f50510760ed4945737fb1f543",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": null,
"size": 250233,
"upload_time": "2024-03-15T09:43:41",
"upload_time_iso_8601": "2024-03-15T09:43:41.275462Z",
"url": "https://files.pythonhosted.org/packages/ab/5e/6f7b4e6f32133c7654fd4a415bff251932b87938c9fc04a62d0ff8f0f033/rocket_fft-0.2.5-cp39-cp39-macosx_11_0_arm64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "66cb7ff8393ea3bbe80ea1c34dc92250e5a4f2b7d84a349b09101f7f76a438b7",
"md5": "229318946e36eb33010000462f93e160",
"sha256": "27848e7e74c84dd9eae3a683260dbf060fde56714a1112438f84cbd310847444"
},
"downloads": -1,
"filename": "rocket_fft-0.2.5-cp39-cp39-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"has_sig": false,
"md5_digest": "229318946e36eb33010000462f93e160",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": null,
"size": 2132826,
"upload_time": "2024-03-15T09:43:42",
"upload_time_iso_8601": "2024-03-15T09:43:42.573057Z",
"url": "https://files.pythonhosted.org/packages/66/cb/7ff8393ea3bbe80ea1c34dc92250e5a4f2b7d84a349b09101f7f76a438b7/rocket_fft-0.2.5-cp39-cp39-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "2480fb502b216489d993c14bfd8431a99e5f70ce6062db502b3af4a83a37fcad",
"md5": "dddb77020d90a92587604ef527a7e713",
"sha256": "989739a8bb4432b7f4059c19bdede7fde98c76cb70fa62d7a299a8606be5619c"
},
"downloads": -1,
"filename": "rocket_fft-0.2.5-cp39-cp39-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"has_sig": false,
"md5_digest": "dddb77020d90a92587604ef527a7e713",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": null,
"size": 2233463,
"upload_time": "2024-03-15T09:43:44",
"upload_time_iso_8601": "2024-03-15T09:43:44.009628Z",
"url": "https://files.pythonhosted.org/packages/24/80/fb502b216489d993c14bfd8431a99e5f70ce6062db502b3af4a83a37fcad/rocket_fft-0.2.5-cp39-cp39-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "234f79abd9f7467b79120411b9c764d142260297bbc58e994e521486bc2472b0",
"md5": "8b0851bab697416c67d18a4029dfc933",
"sha256": "c9a674a7434cbb2d11691d1ad9cbae24ff8d7db10a70cc60004efc5308410360"
},
"downloads": -1,
"filename": "rocket_fft-0.2.5-cp39-cp39-win_amd64.whl",
"has_sig": false,
"md5_digest": "8b0851bab697416c67d18a4029dfc933",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": null,
"size": 192694,
"upload_time": "2024-03-15T09:43:45",
"upload_time_iso_8601": "2024-03-15T09:43:45.481913Z",
"url": "https://files.pythonhosted.org/packages/23/4f/79abd9f7467b79120411b9c764d142260297bbc58e994e521486bc2472b0/rocket_fft-0.2.5-cp39-cp39-win_amd64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "7a52b2447835d93fee2424bc5e7b1a5f1a9a8c75c27fd954ee3b81d581bb889e",
"md5": "c10c1dd8d87729c36078f4e6e5b03418",
"sha256": "9c8ab3fbfaf322344d6de83315bb73626b8467b5aa93ef26aadc3346c2630a28"
},
"downloads": -1,
"filename": "rocket-fft-0.2.5.tar.gz",
"has_sig": false,
"md5_digest": "c10c1dd8d87729c36078f4e6e5b03418",
"packagetype": "sdist",
"python_version": "source",
"requires_python": null,
"size": 44302,
"upload_time": "2024-03-15T09:43:47",
"upload_time_iso_8601": "2024-03-15T09:43:47.219755Z",
"url": "https://files.pythonhosted.org/packages/7a/52/b2447835d93fee2424bc5e7b1a5f1a9a8c75c27fd954ee3b81d581bb889e/rocket-fft-0.2.5.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-03-15 09:43:47",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "styfenschaer",
"github_project": "rocket-fft",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "rocket-fft"
}