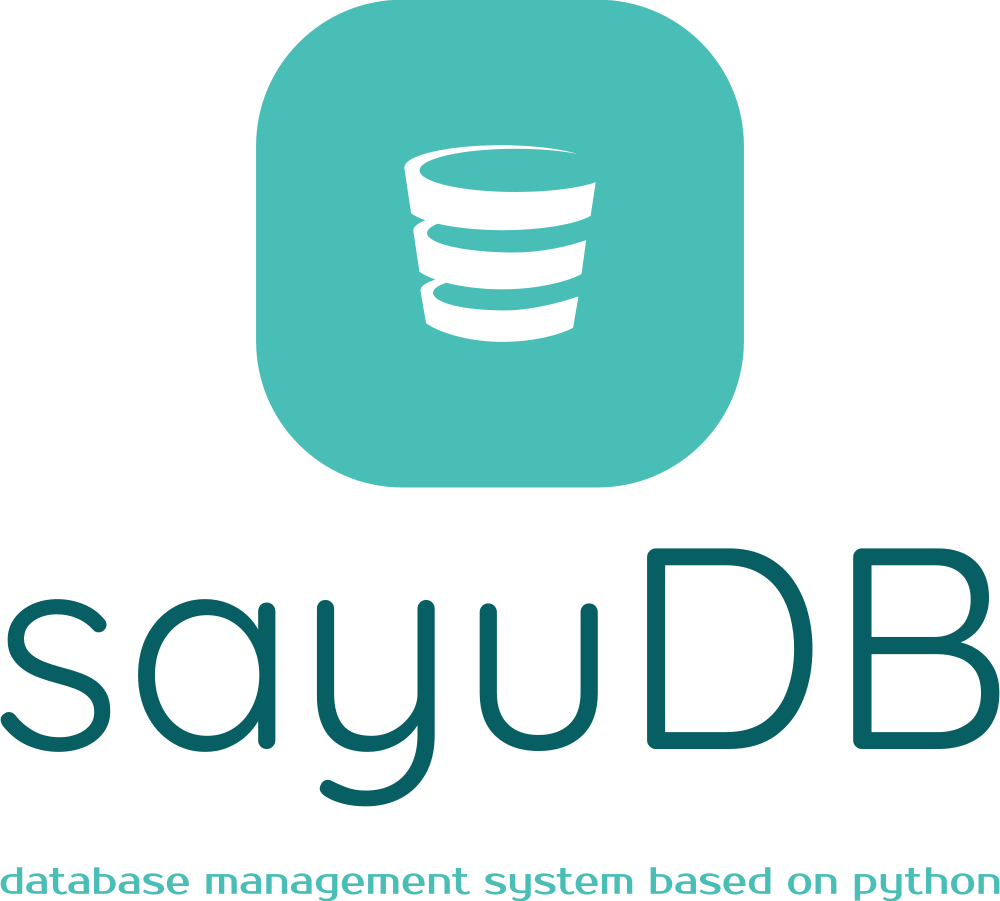
---
# Preface
### What is sayuDB
sayuDB is an database management system based on python and JSON. Developed at Clee Ltd. This project actually for personal purpose only but for some reason I publish it.
It supports a large part of the SQL standard feature
# Tutorial
##### Table of contents
- [Installation](#installation)
- [Create or remove user](#Create_or_remove_user)
- [Grant privileges to user](#Grant_privileges_to_user)
- [Create and drop database](#Create_and_drop_database)
- [Import and export database](#Import_and_export_database)
- [Activate or deactivate server](#Activate_or_deactivate_server)
- [Using the sayuDB](#Using_the_sayuDB)
- [Create table](#Create_table)
- [Drop table](#Drop_table)
- [Show table](#Show_table)
- [Insert](#Insert)
- [Select](#Select)
- [Where](#Where)
- [Update](#Update)
- [Delete](#Delete)
- [Alter table](#Alter_table)
- [Clear table](#Clear_table)
---
### Installation
```shell
> pip3 install sayuDB
```
and if you want to show help menu just
```
> python3 -m sayuDB --h
```
### Create or remove user
To create a user you can command by
```shell
> python3 -m sayuDB create user <username> <password>
```
and if you want to remove user you can command by
```shell
> python3 -m sayuDB remove user <username>
```
Note that creating or removing user only can be user when you have root/local access.
the user is use for remote database. if you want to user this database locally, you didn't need this.
And for show user, you can command by
```shell
> python3 -m sayuDB show users
```
### Grant privileges to user
Ofcourse you need to grant user to some database first before use it remotely.
Grant it by command
```shell
> python3 -m sayuDB grant user <username> <database_name>
```
### Create and drop database
To create a database, just command by
```shell
> python3 create database <database_name>
```
And to drop/delete it, just command by
```shell
> python3 -m sayuDB drop database <database_name>
```
Anyway, you can also show databases by
```shell
> python3 -m sayuDB show databases
```
### Import and export database
To import a database, just command by
```shell
> python3 -m sayuDB import database <path_to_ezdb_file>
```
And for export it, just command by
```shell
> python3 -m sayuDB export database <database_name> <output_path>
```
### Activate or deactivate server
If you want the database remotely online. just activate server by
```shell
> python3 -m sayuDB activate server <port>
```
_`Note: I recomended use 8787 as port`_
then acces it within your server IP.
if you didn't see something, try make the server public by
```shell
> python3 -m sayuDB setup public server <server_name> <port>
```
The `server_name` is your server IP or a domain that already set A record to your server IP.
And the `port` must be same when you activate it.
If you want to deactivate it, just command by
```shell
> python3 -m sayuDB deactivate server
```
---
### Using the sayuDB
To user sayu db in python, just define it first by
```python
import sayuDB # Importing the sayuDB
db = sayuDB.sayuDB("testdb") # call the class
```
The parameters are
|parameter|status|description|
|-|-|-|
`database`|required|the database name
`username`|optional|the username for you sayuDB account if you access it remotely
`password`|optional|ofc the password
`host`|optional|the host of your database hosted in
`as_json`|optional|this is the boolean if you want to return the database as json or just printed table.
### Create table
To create a table use this following function
```python
db.create_table("<table_name>", col=[
["number","int"],
["name","str"],
["age","int"],
["address","str"]
])
```
The parameters are
|parameter|status|description|
|-|-|-|
`name`|required|the name of table you want to create
`col`|required|a lists that contain list wich contain the column name and datatypes `[ ["<column_name>","<datatypes>"] ]`
> Note: supported datatypes `str`,`float`,`int`,`dict`
### Drop table
You can drop table by use this dollowing function
```python
db.drop_table("<table_name>")
```
The parameters are
|parameter|status|description|
|-|-|-|
`name`|required|the name of the table you want to drop/delete
### Show table
you can show the table by use this following funtion.
```python
db.show_table("<table_name>")
```
it will returned
```shell
number name age address
-------- ------ ----- ---------
```
### Insert
To insert some row to the table, you can use this following function
```python
db.insert_row(table="<table_name>", col="number,name,age,address", contents=[1,"Arsybai",23,"Solo, Indonesia"])
```
The parameters are
|parameter|status|description|
|-|-|-|
`table`|required|The name of table you want to insert a row
`col`|required|the column that you want to insert. separate by comma or you can use list
`contents`|required|the contents/value for each column
and now if you show the table it will look like this
```shell
number name age address
-------- ------- ----- ---------------
1 Arsybai 23 Solo, Indonesia
```
and you can also insert it with some function. as example I want to insert moepoi as number 2. but I want the number automatically increased.
```python
db.insert_row(table="<table_name>", col="number,name,age,address", contents=["increase()","Moepoi",20,"Jakarta, Indonesia"])
```
So, I use '`increase()' function for it. then the table will look like this
```shell
number name age address
-------- ------- ----- ------------------
1 Arsybai 23 Solo, Indonesia
2 Moepoi 20 Jakarta, Indonesia
```
Table of functions
|name|description|
|-|-|
`today()`|Generate today datetime (only work in str typedata)
`index()`|Indexing the number of row. it can be use as id too (only work in int typedata)
`genID()`|Generate random 5 digits ID
`increase()`|Increase from the last row value (Only work in int typedata)
### Select
To select a table, use this following function
```python
db.select_row(table="<table_name>", col="name,age")
```
|parameter|status|description|
|-|-|-|
`table`:`str`|required|the name of table that you want to select
`col`:`str`|required|the column name that you want to select. it separated by comma. or you can use `col="*"` if you want to select all comuns
`where`:`str`|optional|select the specific row.
`as_json`:`bool`|optional|if you already define it in the `sayuDB` class, you didn't need to do it again. the default is `False`
### Where
Now, if you want to select specific row, you can use `where` parameter for it
##### Equal or not equal.
as example, I want to select `Arsybai` from table.
```python
db.select_row(table="<table_name>", col="*", where="name=Arsybai")
```
Otherwise, if you want to select row that isn't `Arsybai` use `where="name!=Arsybai"`
The return will look like this
```shell
number name age address
-------- ------- ----- ---------------
1 Arsybai 23 Solo, Indonesia
1 Rows
```
##### contains
If you want to select row that contain someting. you can use ` contain `.
as example I want to select name that contain `poi`
```python
db.select_row(table="<table_name>", col="*", where="name contain poi")
```
The return will look like this
```shell
number name age address
-------- ------ ----- ------------------
2 Moepoi 20 Jakarta, Indonesia
1 Rows
```
##### Using multiple condition
as example I want to select `Arsybai` with age `20`
```python
db.select_row(table="<table_name>", col="*", where="name=Arsybai && age=20")
```
The return will look like this
```shell
number name age address
-------- ------ ----- ---------
0 Rows
```
> Note : You can use `AND ( && )`, `OR ( || )` in multiple condition. also the space between && or || is required
### Update
If you want to update a row, use this following function
```python
db.update_row(table="<table_name>", set_="<column_name>=<value>", where="<column_name>=<value>")
```
Note that update row isn't support multiple condition.
Also you can update a row more efficienly with `update_row_json`
```python
db.update_row_json(table="<table_name>", set_={"<col1_name>":"<value1>","<col2_name>":"<value2>"}, where="<col_name>=<value>")
```
### Delete
If you want to delete some row, use this following function
```python
#to delete specific data that equal with some value
db.delete_row(table="<table_name>", where="<col_name>=<value>")
#to delete specific data that contain some value
db.delete_row(table="<table_name>", where="<col_name> contain <value>")
#to delete specific data with row number
db.delete_row(table="<table_name>", where="row <number_of_row>")
#to delete specific data between row number
db.delete_row(table="<table_name>", where="row between <number_of_rowX>-<number_of_rowY>")
```
### Alter table
To alter or updating some table, use this following function
```python
# to add a column
db.alter_table_add_column(table="<table_name>", col_name="<col_name>", datatypes="<datatype>")
# to remove a column
db.alter_table_drop_column(table="<table_name>", col_name="<col_name>")
# to rename a column
db.alter_table_rename_column(table="<table_name>", col="<column_to_rename>", to_="<rename_to>")
```
### Clear table
To clear table use this following function
```python
db.clear_table(table="<table_name>")
```
This will erase all rows data in the table.
Raw data
{
"_id": null,
"home_page": "https://github.com/Arsybai/sayuDB",
"name": "sayuDB",
"maintainer": "",
"docs_url": null,
"requires_python": "",
"maintainer_email": "",
"keywords": "database",
"author": "Arsybai",
"author_email": "me@arsybai.com",
"download_url": "https://files.pythonhosted.org/packages/92/2a/1a4ea0f487aff9b004c261f7b48a9764b62c9bd71b333e9982cefc08e7ce/sayuDB-1.1.4.tar.gz",
"platform": null,
"description": "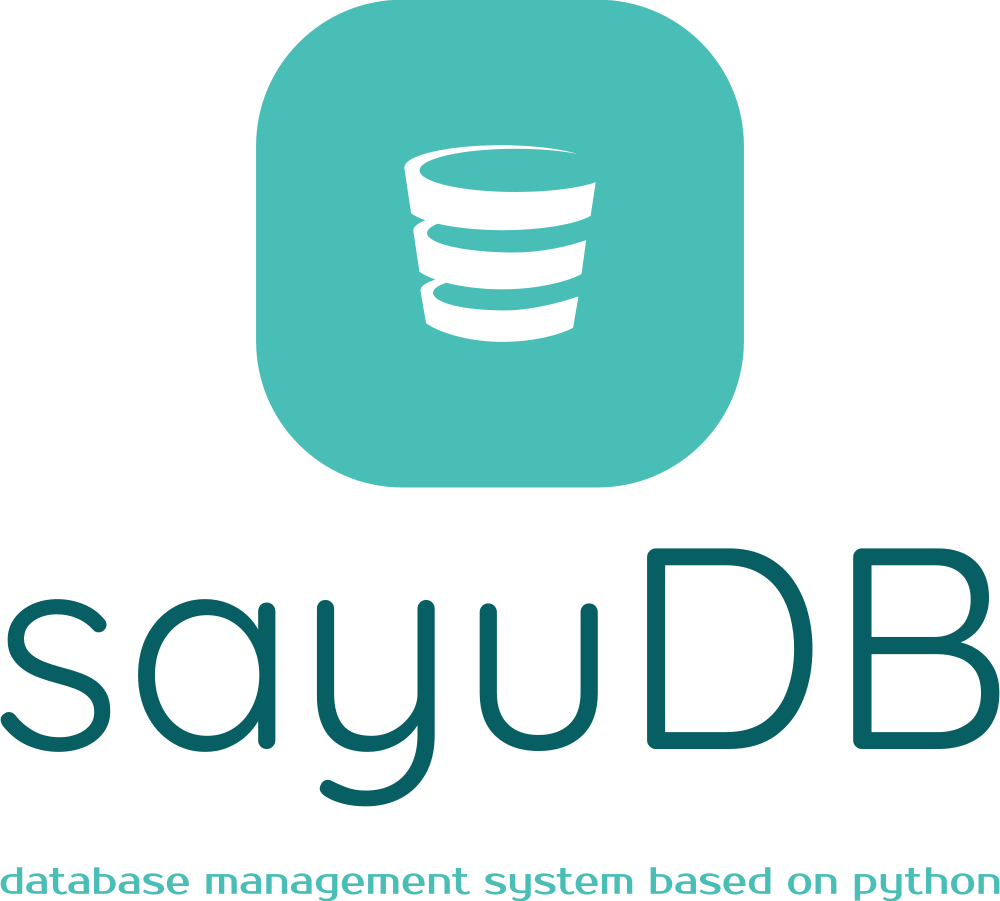\r\n---\r\n# Preface\r\n### What is sayuDB\r\nsayuDB is an database management system based on python and JSON. Developed at Clee Ltd. This project actually for personal purpose only but for some reason I publish it.\r\nIt supports a large part of the SQL standard feature\r\n\r\n# Tutorial\r\n##### Table of contents\r\n\r\n- [Installation](#installation)\r\n- [Create or remove user](#Create_or_remove_user)\r\n- [Grant privileges to user](#Grant_privileges_to_user)\r\n- [Create and drop database](#Create_and_drop_database)\r\n- [Import and export database](#Import_and_export_database)\r\n- [Activate or deactivate server](#Activate_or_deactivate_server)\r\n- [Using the sayuDB](#Using_the_sayuDB)\r\n- [Create table](#Create_table)\r\n- [Drop table](#Drop_table)\r\n- [Show table](#Show_table)\r\n- [Insert](#Insert)\r\n- [Select](#Select)\r\n- [Where](#Where)\r\n- [Update](#Update)\r\n- [Delete](#Delete)\r\n- [Alter table](#Alter_table)\r\n- [Clear table](#Clear_table)\r\n---\r\n\r\n### Installation\r\n```shell\r\n> pip3 install sayuDB\r\n```\r\nand if you want to show help menu just \r\n```\r\n> python3 -m sayuDB --h\r\n```\r\n\r\n### Create or remove user\r\nTo create a user you can command by\r\n```shell\r\n> python3 -m sayuDB create user <username> <password>\r\n```\r\nand if you want to remove user you can command by\r\n```shell\r\n> python3 -m sayuDB remove user <username>\r\n```\r\nNote that creating or removing user only can be user when you have root/local access.\r\nthe user is use for remote database. if you want to user this database locally, you didn't need this.\r\n\r\nAnd for show user, you can command by\r\n```shell\r\n> python3 -m sayuDB show users\r\n```\r\n\r\n### Grant privileges to user\r\nOfcourse you need to grant user to some database first before use it remotely.\r\nGrant it by command\r\n```shell\r\n> python3 -m sayuDB grant user <username> <database_name>\r\n```\r\n### Create and drop database\r\nTo create a database, just command by\r\n```shell\r\n> python3 create database <database_name>\r\n```\r\nAnd to drop/delete it, just command by\r\n```shell\r\n> python3 -m sayuDB drop database <database_name>\r\n```\r\nAnyway, you can also show databases by\r\n```shell\r\n> python3 -m sayuDB show databases\r\n```\r\n### Import and export database\r\nTo import a database, just command by\r\n```shell\r\n> python3 -m sayuDB import database <path_to_ezdb_file>\r\n```\r\nAnd for export it, just command by\r\n```shell\r\n> python3 -m sayuDB export database <database_name> <output_path>\r\n```\r\n### Activate or deactivate server\r\nIf you want the database remotely online. just activate server by\r\n```shell\r\n> python3 -m sayuDB activate server <port>\r\n```\r\n_`Note: I recomended use 8787 as port`_\r\nthen acces it within your server IP.\r\nif you didn't see something, try make the server public by\r\n```shell\r\n> python3 -m sayuDB setup public server <server_name> <port>\r\n```\r\nThe `server_name` is your server IP or a domain that already set A record to your server IP.\r\nAnd the `port` must be same when you activate it.\r\n\r\nIf you want to deactivate it, just command by\r\n```shell\r\n> python3 -m sayuDB deactivate server\r\n```\r\n---\r\n### Using the sayuDB\r\nTo user sayu db in python, just define it first by\r\n```python\r\nimport sayuDB # Importing the sayuDB\r\ndb = sayuDB.sayuDB(\"testdb\") # call the class\r\n```\r\nThe parameters are\r\n|parameter|status|description|\r\n|-|-|-|\r\n`database`|required|the database name\r\n`username`|optional|the username for you sayuDB account if you access it remotely\r\n`password`|optional|ofc the password\r\n`host`|optional|the host of your database hosted in\r\n`as_json`|optional|this is the boolean if you want to return the database as json or just printed table.\r\n### Create table\r\nTo create a table use this following function\r\n```python\r\ndb.create_table(\"<table_name>\", col=[\r\n [\"number\",\"int\"],\r\n [\"name\",\"str\"],\r\n [\"age\",\"int\"],\r\n [\"address\",\"str\"]\r\n])\r\n```\r\nThe parameters are\r\n|parameter|status|description|\r\n|-|-|-|\r\n`name`|required|the name of table you want to create\r\n`col`|required|a lists that contain list wich contain the column name and datatypes `[ [\"<column_name>\",\"<datatypes>\"] ]`\r\n\r\n> Note: supported datatypes `str`,`float`,`int`,`dict`\r\n\r\n### Drop table\r\nYou can drop table by use this dollowing function\r\n```python\r\ndb.drop_table(\"<table_name>\")\r\n```\r\nThe parameters are\r\n|parameter|status|description|\r\n|-|-|-|\r\n`name`|required|the name of the table you want to drop/delete\r\n\r\n### Show table\r\nyou can show the table by use this following funtion.\r\n```python\r\ndb.show_table(\"<table_name>\")\r\n```\r\nit will returned\r\n```shell\r\nnumber name age address\r\n-------- ------ ----- ---------\r\n```\r\n### Insert\r\nTo insert some row to the table, you can use this following function\r\n```python\r\ndb.insert_row(table=\"<table_name>\", col=\"number,name,age,address\", contents=[1,\"Arsybai\",23,\"Solo, Indonesia\"])\r\n```\r\nThe parameters are\r\n|parameter|status|description|\r\n|-|-|-|\r\n`table`|required|The name of table you want to insert a row\r\n`col`|required|the column that you want to insert. separate by comma or you can use list\r\n`contents`|required|the contents/value for each column\r\n\r\nand now if you show the table it will look like this\r\n```shell\r\n number name age address\r\n-------- ------- ----- ---------------\r\n 1 Arsybai 23 Solo, Indonesia\r\n```\r\n\r\nand you can also insert it with some function. as example I want to insert moepoi as number 2. but I want the number automatically increased.\r\n```python\r\ndb.insert_row(table=\"<table_name>\", col=\"number,name,age,address\", contents=[\"increase()\",\"Moepoi\",20,\"Jakarta, Indonesia\"])\r\n```\r\nSo, I use '`increase()' function for it. then the table will look like this\r\n```shell\r\n number name age address\r\n-------- ------- ----- ------------------\r\n 1 Arsybai 23 Solo, Indonesia\r\n 2 Moepoi 20 Jakarta, Indonesia\r\n```\r\nTable of functions\r\n|name|description|\r\n|-|-|\r\n`today()`|Generate today datetime (only work in str typedata)\r\n`index()`|Indexing the number of row. it can be use as id too (only work in int typedata)\r\n`genID()`|Generate random 5 digits ID\r\n`increase()`|Increase from the last row value (Only work in int typedata)\r\n\r\n### Select\r\nTo select a table, use this following function\r\n```python\r\ndb.select_row(table=\"<table_name>\", col=\"name,age\")\r\n```\r\n|parameter|status|description|\r\n|-|-|-|\r\n`table`:`str`|required|the name of table that you want to select\r\n`col`:`str`|required|the column name that you want to select. it separated by comma. or you can use `col=\"*\"` if you want to select all comuns\r\n`where`:`str`|optional|select the specific row.\r\n`as_json`:`bool`|optional|if you already define it in the `sayuDB` class, you didn't need to do it again. the default is `False`\r\n\r\n### Where\r\nNow, if you want to select specific row, you can use `where` parameter for it\r\n##### Equal or not equal.\r\nas example, I want to select `Arsybai` from table.\r\n```python\r\ndb.select_row(table=\"<table_name>\", col=\"*\", where=\"name=Arsybai\")\r\n```\r\nOtherwise, if you want to select row that isn't `Arsybai` use `where=\"name!=Arsybai\"`\r\nThe return will look like this\r\n```shell\r\n number name age address\r\n-------- ------- ----- ---------------\r\n 1 Arsybai 23 Solo, Indonesia\r\n\r\n1 Rows\r\n```\r\n##### contains\r\nIf you want to select row that contain someting. you can use ` contain `.\r\nas example I want to select name that contain `poi`\r\n```python\r\ndb.select_row(table=\"<table_name>\", col=\"*\", where=\"name contain poi\")\r\n```\r\nThe return will look like this\r\n```shell\r\n number name age address\r\n-------- ------ ----- ------------------\r\n 2 Moepoi 20 Jakarta, Indonesia\r\n\r\n1 Rows\r\n```\r\n##### Using multiple condition\r\nas example I want to select `Arsybai` with age `20`\r\n```python\r\ndb.select_row(table=\"<table_name>\", col=\"*\", where=\"name=Arsybai && age=20\")\r\n```\r\nThe return will look like this\r\n```shell\r\nnumber name age address\r\n-------- ------ ----- ---------\r\n\r\n0 Rows\r\n```\r\n> Note : You can use `AND ( && )`, `OR ( || )` in multiple condition. also the space between && or || is required\r\n\r\n### Update\r\nIf you want to update a row, use this following function\r\n```python\r\ndb.update_row(table=\"<table_name>\", set_=\"<column_name>=<value>\", where=\"<column_name>=<value>\")\r\n```\r\nNote that update row isn't support multiple condition.\r\n\r\nAlso you can update a row more efficienly with `update_row_json`\r\n```python\r\ndb.update_row_json(table=\"<table_name>\", set_={\"<col1_name>\":\"<value1>\",\"<col2_name>\":\"<value2>\"}, where=\"<col_name>=<value>\")\r\n```\r\n\r\n### Delete\r\nIf you want to delete some row, use this following function\r\n```python\r\n#to delete specific data that equal with some value\r\ndb.delete_row(table=\"<table_name>\", where=\"<col_name>=<value>\")\r\n\r\n#to delete specific data that contain some value\r\ndb.delete_row(table=\"<table_name>\", where=\"<col_name> contain <value>\")\r\n\r\n#to delete specific data with row number\r\ndb.delete_row(table=\"<table_name>\", where=\"row <number_of_row>\")\r\n\r\n#to delete specific data between row number\r\ndb.delete_row(table=\"<table_name>\", where=\"row between <number_of_rowX>-<number_of_rowY>\")\r\n```\r\n### Alter table\r\nTo alter or updating some table, use this following function\r\n```python\r\n# to add a column\r\ndb.alter_table_add_column(table=\"<table_name>\", col_name=\"<col_name>\", datatypes=\"<datatype>\")\r\n\r\n# to remove a column\r\ndb.alter_table_drop_column(table=\"<table_name>\", col_name=\"<col_name>\")\r\n\r\n# to rename a column\r\ndb.alter_table_rename_column(table=\"<table_name>\", col=\"<column_to_rename>\", to_=\"<rename_to>\")\r\n```\r\n### Clear table\r\nTo clear table use this following function\r\n```python\r\ndb.clear_table(table=\"<table_name>\")\r\n```\r\nThis will erase all rows data in the table.\r\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "Database management system based on python and JSON.",
"version": "1.1.4",
"project_urls": {
"Homepage": "https://github.com/Arsybai/sayuDB"
},
"split_keywords": [
"database"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "922a1a4ea0f487aff9b004c261f7b48a9764b62c9bd71b333e9982cefc08e7ce",
"md5": "0edf02cfcab84e51d81046fd3b5f61f3",
"sha256": "e4dd460bc965ecfd67e254490c4f8e889f4b9e92572580357ea70fda71ee1e8b"
},
"downloads": -1,
"filename": "sayuDB-1.1.4.tar.gz",
"has_sig": false,
"md5_digest": "0edf02cfcab84e51d81046fd3b5f61f3",
"packagetype": "sdist",
"python_version": "source",
"requires_python": null,
"size": 15046,
"upload_time": "2023-05-18T13:50:41",
"upload_time_iso_8601": "2023-05-18T13:50:41.791406Z",
"url": "https://files.pythonhosted.org/packages/92/2a/1a4ea0f487aff9b004c261f7b48a9764b62c9bd71b333e9982cefc08e7ce/sayuDB-1.1.4.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-05-18 13:50:41",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "Arsybai",
"github_project": "sayuDB",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"lcname": "sayudb"
}