# Simple Django Rest Framework
Simple Django Rest Framework is django based app used to provide abstraction that combines both django rest framework with drf_yasg a swagger generator to implement good looking and well documented apis using djang
## Quick Start
### Install
1. assuming you already have a django project that you need to add this app to you need to start with installing the package using
```
pip install sdrf
```
2. install required apps to INSTALLED_APPS in django settings
```python
INSTALLED_APPS = [
...
"rest_framework",
"drf_yasg",
'sdrf',
]
```
3. add swagger docs url to your project urls
```python
from django.contrib import admin
from django.urls import path,include
urlpatterns = [
...
path('',include('sdrf.urls'))
]
```
4. check out if everything is okay by visting default swagger docs url at ``http://<your-project-url>/rest``
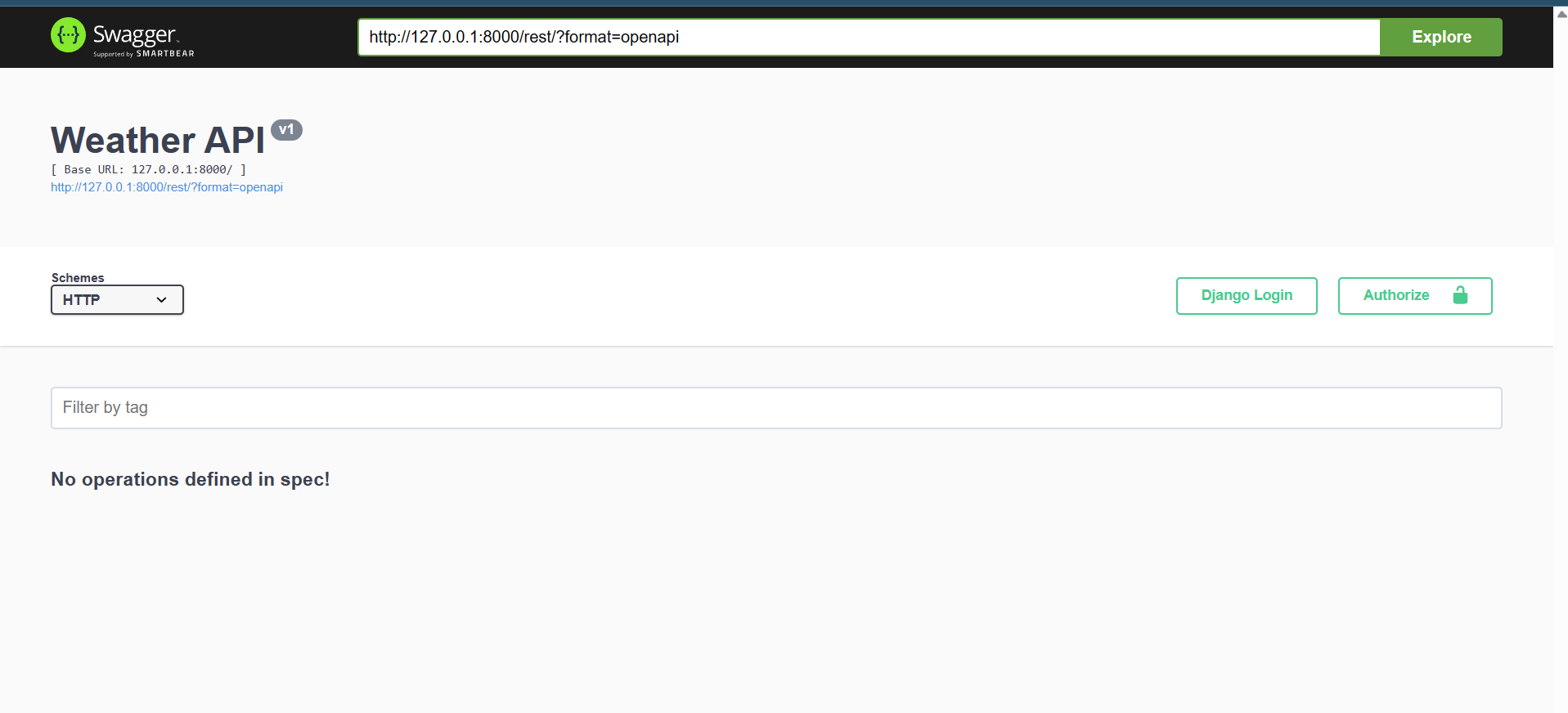
### Configure
now you have an rest app up and running you can configure it as you want. addtionally for django rest framework configuration and drf_yasg configuration you have our sdrf configs you can make with these default values
| config variable name | default value | description |
| ------- | ------ | ----- |
| REST_APP_NAME | `Weather API` | the app name used in swagger docs view |
| REST_APP_VERSION | `v1` | the api version used in swagger docs view |
| REST_APP_CREATOR | ``{'name': '','email': '','url': ''}`` | the contact information for communicating the rest app creator used in swagger docs view |
| REST_APP_BASE_URL | `rest/` | the base url that the swagger docs will be viewd on and a prefix for default api endpoint |
## Usage
### Build Single Method API Endpoints
1. assuming you have an django app that already exsist create and an API endpoint class that inheiret APIEndPoint class for example see out hello world here
```python
from sdrf.api_endpoint import APIEndpoint
from sdrf.endpoint_config import APIEndpointConfig
class HelloEndPoint(APIEndpoint):
def configure(self, config: APIEndpointConfig) -> APIEndpointConfig:
...
return config
@staticmethod
def execute(request: Request, *args, **kwargs) -> Response:
name = request.query_params.get('name')
return HttpResponse(f'Hello {name}')
```
2. configure your api endpoint using configure method, in this method you have all sort of configuration that you can configure for your rest api even the auhentication and authorization configurations, http method, routing, and swagger view configs simplified with easy and nice looking config code
```python
from sdrf.api_endpoint import APIEndpoint
from sdrf.endpoint_config import APIEndpointConfig
class HelloEndPoint(APIEndpoint):
def configure(self, config: APIEndpointConfig) -> APIEndpointConfig:
config.name = 'Hello Rest!'
config.description= """
First Well documented API endpoint that says hello to name that you send
"""
config.endpoint= 'hello'
config.http_method = 'GET'
config.set_response(200,'hello [name]')
config.add_parameter('name',self.DataType.STRING,self.ParameterTypes.QUERY_PARAM)
config.add_tag('First API')
return config
@staticmethod
def execute(request: Request, *args, **kwargs) -> Response:
name = request.query_params.get('name')
return HttpResponse(f'Hello {name}')
```
3. finally add your api endpoint to your app urls using as_url static method
```python
from .views import HelloEndPoint
urlpatterns = [
HelloEndPoint.as_url()
]
```
4. check your output and test your api in the swagger view
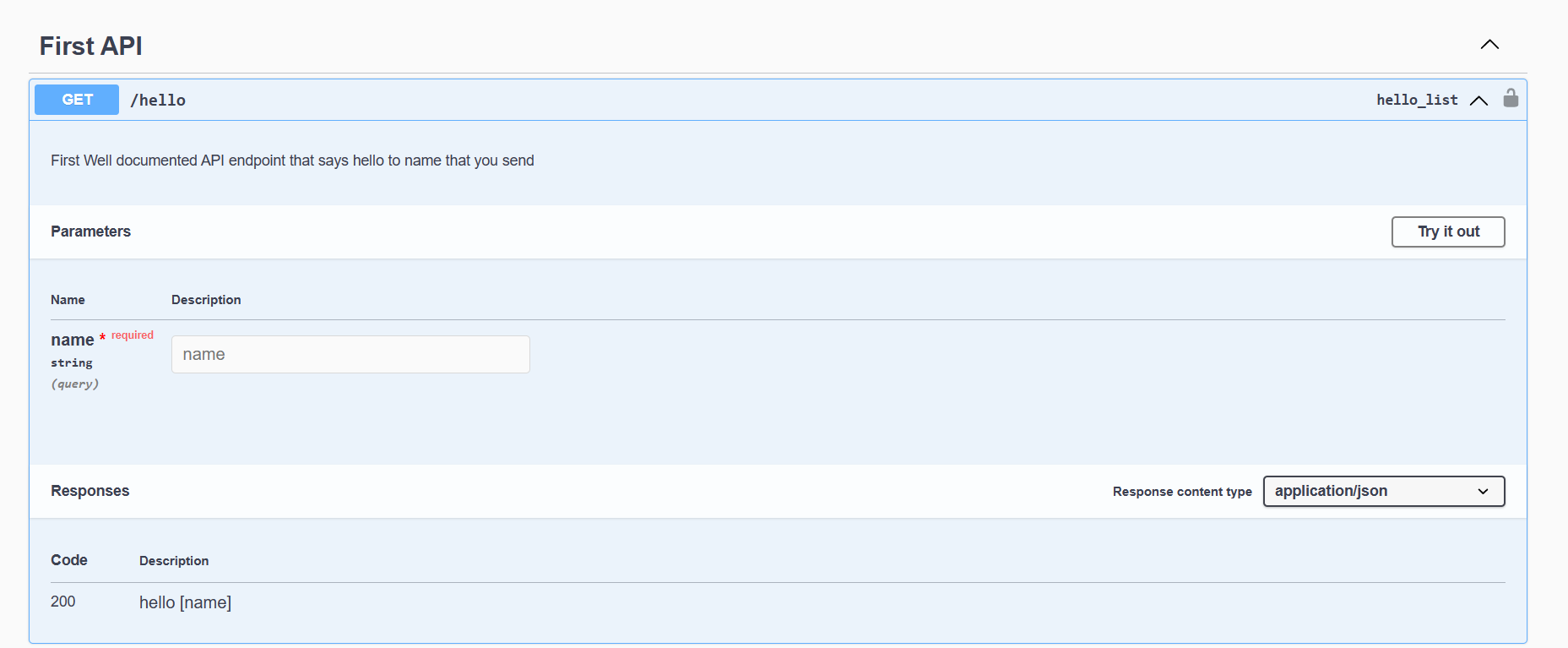
### Build Model API EndPoint
just like you would do in normal django rest framework and make `ViewSet` you don`t need to learn anything new to that but instead of inherting `rest_framework.viewsets.ModelViewSet` you will inherit our `ModelEndPoint`like that
```python
from .models import Person,PersonSeralizer
from sdrf.model_endpoint import ModelEndPoint
...
class PersonEndPoint(ModelEndPoint):
queryset = Person.objects.all()
serializer_class = PersonSeralizer
```
then simply make it as urls in `urls.py`
```python
from .views import PersonEndPoint
urlpatterns = [
...
PersonEndPoint.as_urls(),
]
```
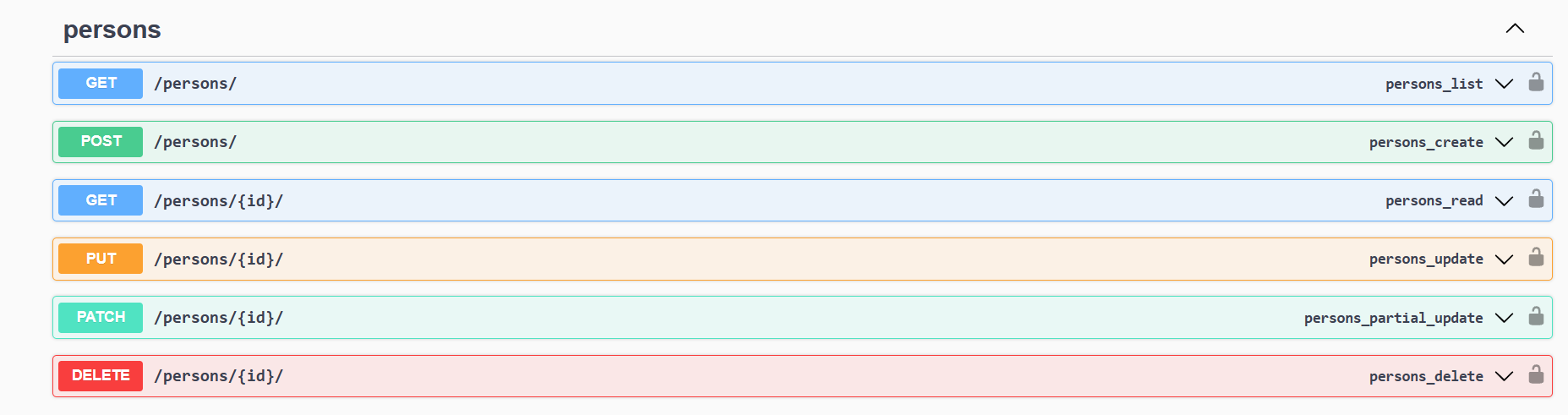
Raw data
{
"_id": null,
"home_page": "https://github.com/hus201/sdrf",
"name": "sdrf",
"maintainer": "",
"docs_url": null,
"requires_python": ">=3.8, <4",
"maintainer_email": "",
"keywords": "django,rest apis,abstraction",
"author": "Hussein Anabtawi",
"author_email": "Hussein Anabtawi <husainanabtawi2001@hotmail.com>",
"download_url": "https://files.pythonhosted.org/packages/d4/fb/09ac90dbcef4ed01d20cb88bd77e838c0ff6a5de9fc1c43db5480e12f395/sdrf-0.1.0.tar.gz",
"platform": null,
"description": "# Simple Django Rest Framework\n\nSimple Django Rest Framework is django based app used to provide abstraction that combines both django rest framework with drf_yasg a swagger generator to implement good looking and well documented apis using djang\n\n\n## Quick Start\n\n### Install\n1. assuming you already have a django project that you need to add this app to you need to start with installing the package using\n```\npip install sdrf\n```\n2. install required apps to INSTALLED_APPS in django settings\n```python\nINSTALLED_APPS = [\n ...\n \"rest_framework\",\n \"drf_yasg\",\n 'sdrf',\n]\n```\n3. add swagger docs url to your project urls\n```python\nfrom django.contrib import admin\nfrom django.urls import path,include\n\nurlpatterns = [\n ...\n path('',include('sdrf.urls'))\n]\n```\n4. check out if everything is okay by visting default swagger docs url at ``http://<your-project-url>/rest``\n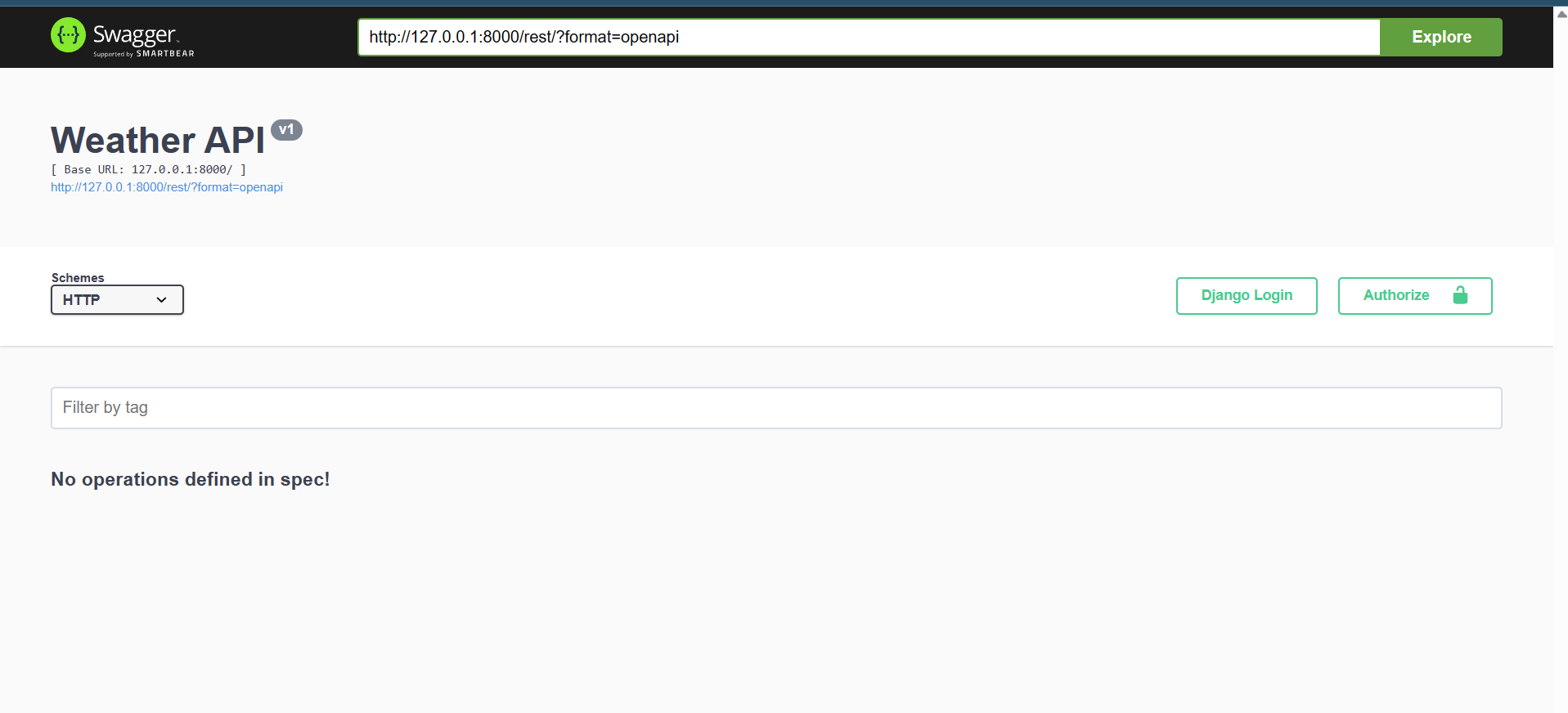\n\n### Configure\nnow you have an rest app up and running you can configure it as you want. addtionally for django rest framework configuration and drf_yasg configuration you have our sdrf configs you can make with these default values\n\n\n| config variable name | default value | description |\n| ------- | ------ | ----- |\n| REST_APP_NAME | `Weather API` | the app name used in swagger docs view |\n| REST_APP_VERSION | `v1` | the api version used in swagger docs view |\n| REST_APP_CREATOR | ``{'name': '','email': '','url': ''}`` | the contact information for communicating the rest app creator used in swagger docs view |\n| REST_APP_BASE_URL | `rest/` | the base url that the swagger docs will be viewd on and a prefix for default api endpoint |\n\n## Usage\n\n### Build Single Method API Endpoints\n1. assuming you have an django app that already exsist create and an API endpoint class that inheiret APIEndPoint class for example see out hello world here\n```python\nfrom sdrf.api_endpoint import APIEndpoint\nfrom sdrf.endpoint_config import APIEndpointConfig\n\n\nclass HelloEndPoint(APIEndpoint):\n def configure(self, config: APIEndpointConfig) -> APIEndpointConfig:\n ...\n return config\n\n @staticmethod\n def execute(request: Request, *args, **kwargs) -> Response:\n name = request.query_params.get('name')\n return HttpResponse(f'Hello {name}')\n\n```\n2. configure your api endpoint using configure method, in this method you have all sort of configuration that you can configure for your rest api even the auhentication and authorization configurations, http method, routing, and swagger view configs simplified with easy and nice looking config code\n```python\nfrom sdrf.api_endpoint import APIEndpoint\nfrom sdrf.endpoint_config import APIEndpointConfig\n\n\nclass HelloEndPoint(APIEndpoint):\n def configure(self, config: APIEndpointConfig) -> APIEndpointConfig:\n config.name = 'Hello Rest!'\n config.description= \"\"\"\n First Well documented API endpoint that says hello to name that you send\n \"\"\"\n config.endpoint= 'hello'\n config.http_method = 'GET'\n config.set_response(200,'hello [name]')\n config.add_parameter('name',self.DataType.STRING,self.ParameterTypes.QUERY_PARAM)\n config.add_tag('First API')\n return config\n\n @staticmethod\n def execute(request: Request, *args, **kwargs) -> Response:\n name = request.query_params.get('name')\n return HttpResponse(f'Hello {name}')\n\n```\n3. finally add your api endpoint to your app urls using as_url static method\n```python\nfrom .views import HelloEndPoint\n\nurlpatterns = [\n HelloEndPoint.as_url()\n]\n```\n\n4. check your output and test your api in the swagger view\n\n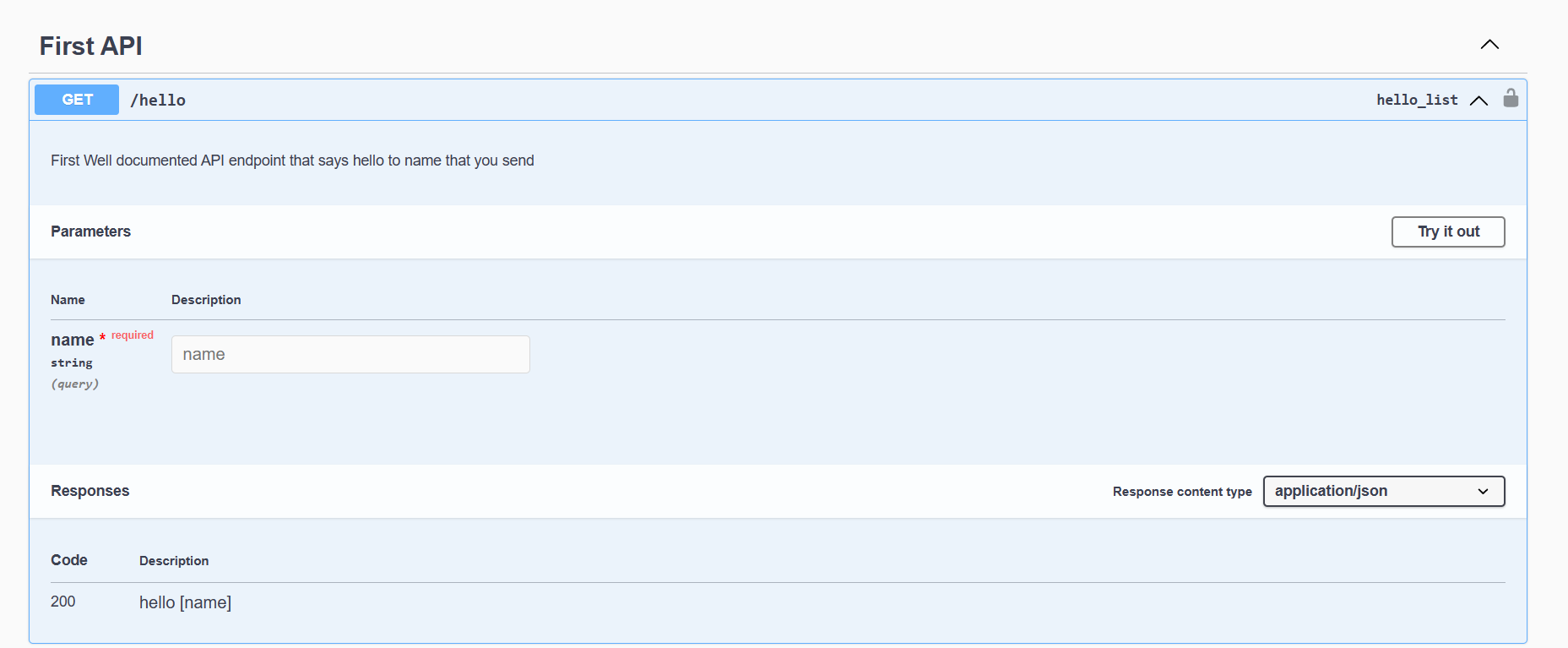\n\n\n### Build Model API EndPoint\n\njust like you would do in normal django rest framework and make `ViewSet` you don`t need to learn anything new to that but instead of inherting `rest_framework.viewsets.ModelViewSet` you will inherit our `ModelEndPoint`like that\n\n```python\nfrom .models import Person,PersonSeralizer\nfrom sdrf.model_endpoint import ModelEndPoint\n...\nclass PersonEndPoint(ModelEndPoint):\n queryset = Person.objects.all()\n serializer_class = PersonSeralizer\n```\nthen simply make it as urls in `urls.py`\n```python\nfrom .views import PersonEndPoint\n\nurlpatterns = [\n ...\n PersonEndPoint.as_urls(),\n]\n\n```\n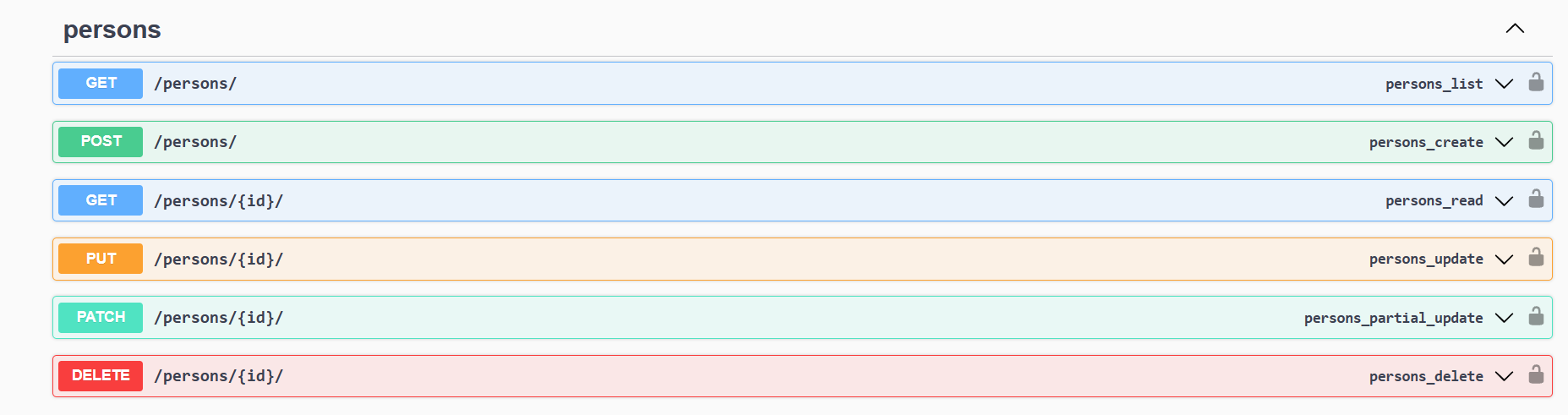\n",
"bugtrack_url": null,
"license": "",
"summary": "Simple rest framework is an abstraction for building rest api with well written documentations",
"version": "0.1.0",
"project_urls": {
"Bug Tracker": "https://github.com/hus201/sdrf/issues",
"Homepage": "https://github.com/hus201/sdrf"
},
"split_keywords": [
"django",
"rest apis",
"abstraction"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "df661e7518ed2583c765f4f2a46ae0591ef721239534fbab6fe615e8c9aee503",
"md5": "f5c83c7e0c9c27c8f37cebef3da5b943",
"sha256": "fbe29325cf5ab1000f4b442d7f99865153ba6b8dc91a31ec18ee36cbabb91292"
},
"downloads": -1,
"filename": "sdrf-0.1.0-py3-none-any.whl",
"has_sig": false,
"md5_digest": "f5c83c7e0c9c27c8f37cebef3da5b943",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.8, <4",
"size": 9118,
"upload_time": "2023-07-21T13:24:32",
"upload_time_iso_8601": "2023-07-21T13:24:32.177087Z",
"url": "https://files.pythonhosted.org/packages/df/66/1e7518ed2583c765f4f2a46ae0591ef721239534fbab6fe615e8c9aee503/sdrf-0.1.0-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "d4fb09ac90dbcef4ed01d20cb88bd77e838c0ff6a5de9fc1c43db5480e12f395",
"md5": "d931a3de0ba9ea532682d07ab81a352a",
"sha256": "c0f51234ae2574c5a489ca78a6a8f1f0ada151df8bfd1e319a5de637a87d8d67"
},
"downloads": -1,
"filename": "sdrf-0.1.0.tar.gz",
"has_sig": false,
"md5_digest": "d931a3de0ba9ea532682d07ab81a352a",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.8, <4",
"size": 8677,
"upload_time": "2023-07-21T13:24:33",
"upload_time_iso_8601": "2023-07-21T13:24:33.823341Z",
"url": "https://files.pythonhosted.org/packages/d4/fb/09ac90dbcef4ed01d20cb88bd77e838c0ff6a5de9fc1c43db5480e12f395/sdrf-0.1.0.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-07-21 13:24:33",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "hus201",
"github_project": "sdrf",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "sdrf"
}