## Selenium-recaptcha-solver
You can use this package to solve ReCAPTCHA challenges with selenium.
It supports single-step and multi-step audio solving for ReCAPTCHA V2 with >90% success rate.
Please use this package responsibly and for non-exploitative ends.
## Requirements
Python 3.7+
Main dependencies:
<ul>
<li>SpeechRecognition python package to transcribe speech</li>
<li>Pydub for file conversions</li>
<li>Requests for HTTP requests</li>
<li>Selenium for web driver </li>
</ul>
If you're getting an error related to FFmpeg not being installed or in your PATH, get it here: https://ffmpeg.org/download.html
If the error persists, make sure FFmpeg is properly installed for your OS and in your PATH.
## Installation
```bash
python -m pip install selenium-recaptcha-solver
```
## Usage example with ReCAPTCHA demo site
```python
from selenium_recaptcha_solver import RecaptchaSolver
from selenium.webdriver.common.by import By
from selenium import webdriver
from selenium.webdriver.chrome.options import Options
test_ua = 'Mozilla/5.0 (Windows NT 4.0; WOW64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/37.0.2049.0 Safari/537.36'
options = Options()
options.add_argument("--headless") # Remove this if you want to see the browser (Headless makes the chromedriver not have a GUI)
options.add_argument("--window-size=1920,1080")
options.add_argument(f'--user-agent={test_ua}')
options.add_argument('--no-sandbox')
options.add_argument("--disable-extensions")
test_driver = webdriver.Chrome(options=options)
solver = RecaptchaSolver(driver=test_driver)
test_driver.get('https://www.google.com/recaptcha/api2/demo')
recaptcha_iframe = test_driver.find_element(By.XPATH, '//iframe[@title="reCAPTCHA"]')
solver.click_recaptcha_v2(iframe=recaptcha_iframe)
```
You can check a detailed use case in the tests folder of this project (Its execution is shown below in the demonstration chapter).
## Demonstration
[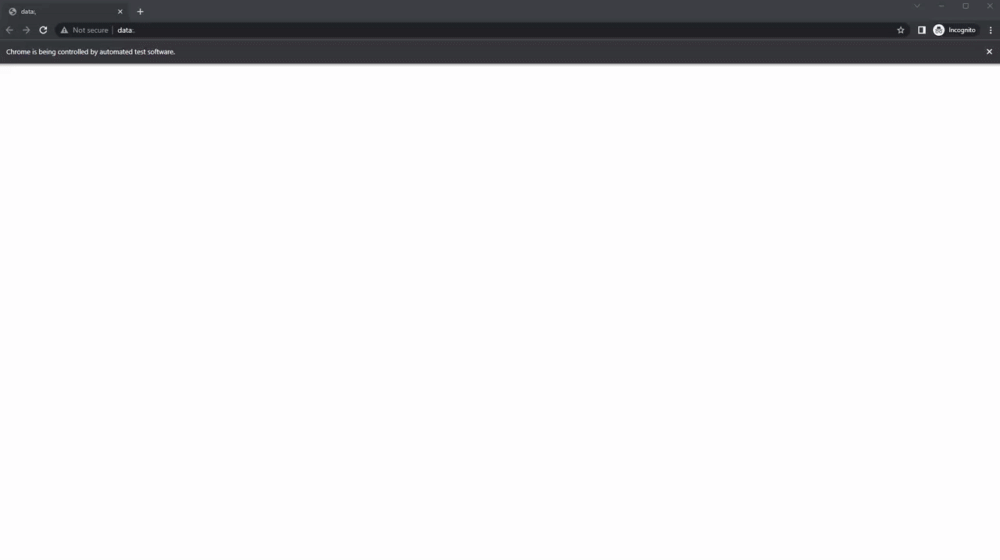](https://gyazo.com/858ceb5df9f43f6aafadf69e233cd2d1)
## Avoiding detection
To decrease your chances of ReCAPTCHA detecting automated queries, try the following:
- Use a custom user-agent header (Make sure it's not a headless user-agent!)
- Use a hard-to-detect web driver
- Use proxies to avoid IP blacklisting
An example of a good user-agent: Mozilla/5.0 (Windows NT 4.0; WOW64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/37.0.2049.0 Safari/537.36
An example of a bad user-agent: are Mozilla/5.0 (X11; Linux x86_64) AppleWebKit/537.36 (KHTML, like Gecko) HeadlessChrome/92.0.4512.0 Safari/537.36
Note the last part of the user-agent; the headless specification is usually there.
## Questions
If the documentation hasn't covered something, or you have questions about how to use the package or how it works, please reach out.
Raw data
{
"_id": null,
"home_page": "https://github.com/thicccat688/selenium-recaptcha-solver",
"name": "selenium-recaptcha-solver",
"maintainer": "",
"docs_url": null,
"requires_python": "",
"maintainer_email": "",
"keywords": "python,captcha,speech recognition,selenium,web automation",
"author": "Tom\u00e1s Perestrelo",
"author_email": "tomasperestrelo21@gmail.com",
"download_url": "https://files.pythonhosted.org/packages/1a/54/142e1abf30916d3457ced3b7037a3f39ee6df0ca129e72898e28f29213ea/selenium-recaptcha-solver-1.9.0.tar.gz",
"platform": null,
"description": "## Selenium-recaptcha-solver\r\n\r\nYou can use this package to solve ReCAPTCHA challenges with selenium.\r\n\r\nIt supports single-step and multi-step audio solving for ReCAPTCHA V2 with >90% success rate.\r\n\r\nPlease use this package responsibly and for non-exploitative ends.\r\n\r\n## Requirements \r\n\r\nPython 3.7+\r\n\r\nMain dependencies:\r\n<ul>\r\n <li>SpeechRecognition python package to transcribe speech</li>\r\n <li>Pydub for file conversions</li>\r\n <li>Requests for HTTP requests</li>\r\n <li>Selenium for web driver </li>\r\n</ul>\r\n\r\nIf you're getting an error related to FFmpeg not being installed or in your PATH, get it here: https://ffmpeg.org/download.html\r\n\r\nIf the error persists, make sure FFmpeg is properly installed for your OS and in your PATH.\r\n\r\n## Installation\r\n\r\n```bash\r\npython -m pip install selenium-recaptcha-solver\r\n```\r\n\r\n## Usage example with ReCAPTCHA demo site\r\n\r\n```python\r\nfrom selenium_recaptcha_solver import RecaptchaSolver\r\nfrom selenium.webdriver.common.by import By\r\nfrom selenium import webdriver\r\nfrom selenium.webdriver.chrome.options import Options\r\n\r\n\r\ntest_ua = 'Mozilla/5.0 (Windows NT 4.0; WOW64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/37.0.2049.0 Safari/537.36'\r\n\r\noptions = Options()\r\n\r\noptions.add_argument(\"--headless\") # Remove this if you want to see the browser (Headless makes the chromedriver not have a GUI)\r\noptions.add_argument(\"--window-size=1920,1080\")\r\n\r\noptions.add_argument(f'--user-agent={test_ua}')\r\n\r\noptions.add_argument('--no-sandbox')\r\noptions.add_argument(\"--disable-extensions\")\r\n\r\ntest_driver = webdriver.Chrome(options=options)\r\n\r\nsolver = RecaptchaSolver(driver=test_driver)\r\n\r\ntest_driver.get('https://www.google.com/recaptcha/api2/demo')\r\n\r\nrecaptcha_iframe = test_driver.find_element(By.XPATH, '//iframe[@title=\"reCAPTCHA\"]')\r\n\r\nsolver.click_recaptcha_v2(iframe=recaptcha_iframe)\r\n```\r\n\r\nYou can check a detailed use case in the tests folder of this project (Its execution is shown below in the demonstration chapter).\r\n\r\n## Demonstration\r\n[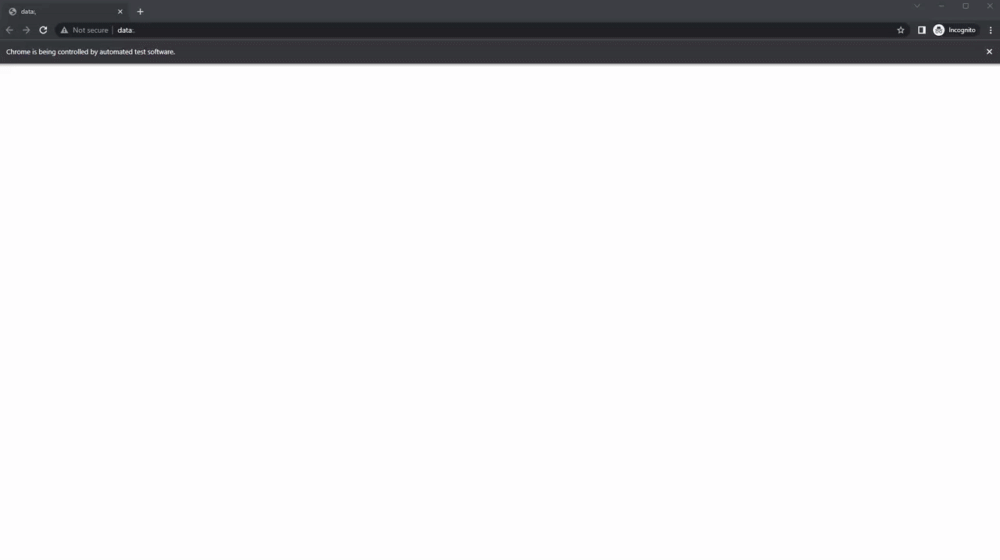](https://gyazo.com/858ceb5df9f43f6aafadf69e233cd2d1)\r\n\r\n## Avoiding detection\r\n\r\nTo decrease your chances of ReCAPTCHA detecting automated queries, try the following:\r\n- Use a custom user-agent header (Make sure it's not a headless user-agent!)\r\n- Use a hard-to-detect web driver \r\n- Use proxies to avoid IP blacklisting\r\n\r\nAn example of a good user-agent: Mozilla/5.0 (Windows NT 4.0; WOW64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/37.0.2049.0 Safari/537.36\r\n\r\nAn example of a bad user-agent: are Mozilla/5.0 (X11; Linux x86_64) AppleWebKit/537.36 (KHTML, like Gecko) HeadlessChrome/92.0.4512.0 Safari/537.36\r\n\r\nNote the last part of the user-agent; the headless specification is usually there.\r\n\r\n## Questions\r\nIf the documentation hasn't covered something, or you have questions about how to use the package or how it works, please reach out.\r\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "",
"version": "1.9.0",
"project_urls": {
"Download": "https://pypi.org/project/selenium-recaptcha-solver",
"Homepage": "https://github.com/thicccat688/selenium-recaptcha-solver"
},
"split_keywords": [
"python",
"captcha",
"speech recognition",
"selenium",
"web automation"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "c9da7e40d891bb0660af97f8ac852854cba3086d0be9eb68dd269cedb9bf908c",
"md5": "07e72fcc6acaa49ef04778bfcc7ef857",
"sha256": "39dcab67ff562fa31f9ab53c8b23eff62446dbdde93cb8642b516771a6ab8629"
},
"downloads": -1,
"filename": "selenium_recaptcha_solver-1.9.0-py3-none-any.whl",
"has_sig": false,
"md5_digest": "07e72fcc6acaa49ef04778bfcc7ef857",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": null,
"size": 8320,
"upload_time": "2023-07-09T16:52:56",
"upload_time_iso_8601": "2023-07-09T16:52:56.238595Z",
"url": "https://files.pythonhosted.org/packages/c9/da/7e40d891bb0660af97f8ac852854cba3086d0be9eb68dd269cedb9bf908c/selenium_recaptcha_solver-1.9.0-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "1a54142e1abf30916d3457ced3b7037a3f39ee6df0ca129e72898e28f29213ea",
"md5": "04313d972e2c6bd7b78caf6f094d45a7",
"sha256": "8ca6b6fd8a96ee96066ab94ade920f8902ef80dd4ee4dfb2a1e5f9a96c68977c"
},
"downloads": -1,
"filename": "selenium-recaptcha-solver-1.9.0.tar.gz",
"has_sig": false,
"md5_digest": "04313d972e2c6bd7b78caf6f094d45a7",
"packagetype": "sdist",
"python_version": "source",
"requires_python": null,
"size": 7301,
"upload_time": "2023-07-09T16:52:58",
"upload_time_iso_8601": "2023-07-09T16:52:58.816126Z",
"url": "https://files.pythonhosted.org/packages/1a/54/142e1abf30916d3457ced3b7037a3f39ee6df0ca129e72898e28f29213ea/selenium-recaptcha-solver-1.9.0.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-07-09 16:52:58",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "thicccat688",
"github_project": "selenium-recaptcha-solver",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"requirements": [],
"lcname": "selenium-recaptcha-solver"
}