# The Official Python Library for the ShuttleAI API

[](https://pypi.org/project/shuttleai/)
[](https://pepy.tech/project/shuttleai)
[](https://pepy.tech/project/shuttleai)
[](https://pypi.org/project/shuttleai/)
The ShuttleAI Python library provides easy access to the ShuttleAI REST API for Python 3.9+ applications. It includes type definitions for all request parameters and response fields, offering both synchronous and asynchronous clients powered by [httpx](https://github.com/encode/httpx) and [aiohttp](https://github.com/aio-libs/aiohttp), respectively.
We prioritize performance optimizations across the library. Beyond using orjson for near-instant JSON processing, we implement various techniques to reduce overhead and enhance speed, ensuring efficient and swift API interactions. These optimizations include minimizing built-in library usage, leveraging reusable aiohttp client sessions, and incorporating several small adjustments to streamline operations.
## Installation
```s
pip install shuttleai
```
*We recommend using `--upgrade` or `-U` to ensure you have the latest version of the library.*
### From Source
This client uses `poetry` as a dependency and virtual environment manager.
You can install poetry with
```bash
pip install poetry
```
`poetry` will set up a virtual environment and install dependencies with the following command:
```bash
poetry install
```
## Getting Started
Below is a non-streamed sync and async example for the ShuttleAI API. You can find more examples in the `examples/` directory.
### Synchronous Client
```python
from shuttleai import ShuttleAI
shuttleai = ShuttleAI()
response = shuttleai.chat.completions.create(
model="shuttle-2.5",
messages=[{"role": "user", "content": "What day is today?"}],
internet=True
)
print(chunk.choices[0].message.content)
```
### Asynchronous Client
```python
import asyncio
from shuttleai import AsyncShuttleAI
async def main():
shuttleai = AsyncShuttleAI()
response = await shuttleai.chat.completions.create(
model="shuttle-2.5",
messages=[{"role": "user", "content": "Imagine an AI like no other, its name is ShuttleAI."}],
)
print(response.choices[0].message.content)
asyncio.run(main())
```
### ShuttleAI CLI
Scroll down to the [Scripts](#scripts) section for more information.
## Run examples
You can run the examples in the `examples/` directory using `poetry run` or by entering the virtual environment using `poetry shell`.
### Using poetry run
```bash
cd examples
poetry run python chat_no_streaming.py
```
### Using poetry shell
```bash
poetry shell
cd examples
>> python chat_no_streaming.py
```
## API Key Setup
To use the ShuttleAI API, you need to have an API key.
You can get a **FREE** API key by signing up at
[shuttleai.com](https://shuttleai.com) and heading to
the [key management page](https://shuttleai.com/keys).
After you have an API key, you can set it as an environment variable:
### Windows
```s
setx SHUTTLEAI_API_KEY "<your_api_key>"
```
> [!Note]
> This will only work in the current terminal session. To set it permanently, you can use the `setx` command with the `/m` flag.
```s
setx SHUTTLEAI_API_KEY "<your_api_key>" /m
```
### macOS/Linux
```bash
export SHUTTLEAI_API_KEY=<your_api_key>
```
## Contribution
We welcome and appreciate contributions to the ShuttleAI API Python SDK.
Please see the [contribution guide](CONTRIBUTING.md) for more information.
*Benefits may apply! :smile:*
## Scripts
### Formatting/Checks
- `poetry run ruff check shuttleai` - Check ruff
- `poetry run black shuttleai --diff --color` - Check black
- `poetry run black shuttleai` - Format code
- `poetry run mypy shuttleai` - Check for type errors
### Tools
- `poetry run clean` - Clean up the project directory
- `poetry run key` - Display your default API key (if set by environment variable)
- `poetry run contr` - Display contributors
### ShuttleAI CLI
- `poetry run shuttleai` or `shuttleai` - Run the ShuttleAI CLI.
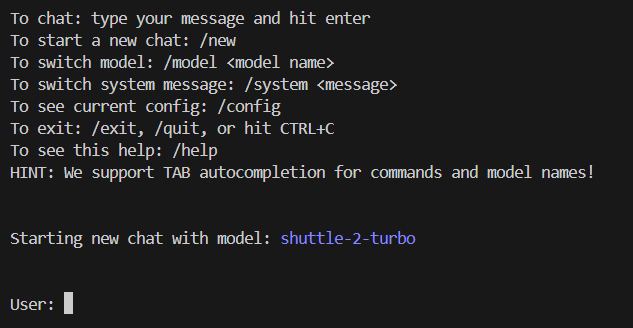
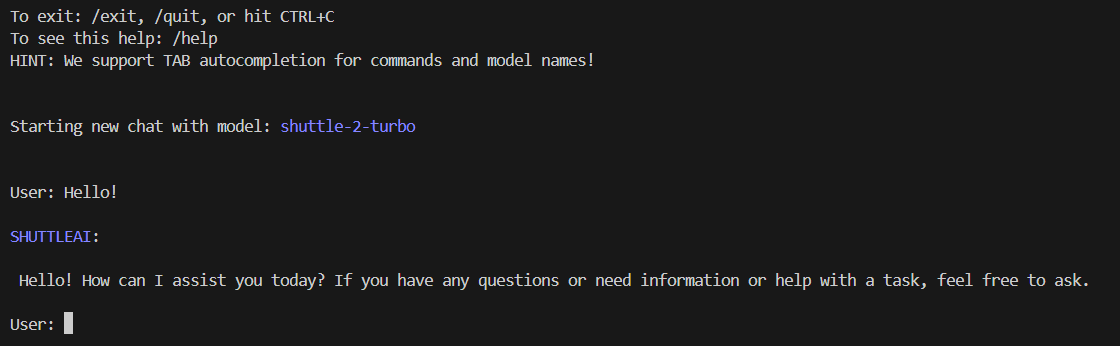
> [!Important]
> We support auto TAB completion of commands and model names! Just press `TAB`!

Raw data
{
"_id": null,
"home_page": "https://github.com/shuttleai",
"name": "shuttleai",
"maintainer": "Christian \"Thoth\" Heru",
"docs_url": null,
"requires_python": "<4.0,>=3.9",
"maintainer_email": "chris@shuttleai.app",
"keywords": "shuttleai, ai, gpt, claude, api, free, chatgpt, gpt-4",
"author": "Tristan Liu",
"author_email": "tristan@shuttleai.app",
"download_url": "https://files.pythonhosted.org/packages/76/fc/0d0b36d3447e706e058c1798474da69dfa9af94bb11dabe478025fcabfca/shuttleai-4.7.2.tar.gz",
"platform": null,
"description": "# The Official Python Library for the ShuttleAI API\n\n\n[](https://pypi.org/project/shuttleai/)\n[](https://pepy.tech/project/shuttleai)\n[](https://pepy.tech/project/shuttleai)\n[](https://pypi.org/project/shuttleai/)\n\nThe ShuttleAI Python library provides easy access to the ShuttleAI REST API for Python 3.9+ applications. It includes type definitions for all request parameters and response fields, offering both synchronous and asynchronous clients powered by [httpx](https://github.com/encode/httpx) and [aiohttp](https://github.com/aio-libs/aiohttp), respectively.\n\nWe prioritize performance optimizations across the library. Beyond using orjson for near-instant JSON processing, we implement various techniques to reduce overhead and enhance speed, ensuring efficient and swift API interactions. These optimizations include minimizing built-in library usage, leveraging reusable aiohttp client sessions, and incorporating several small adjustments to streamline operations.\n\n## Installation\n\n```s\npip install shuttleai\n```\n*We recommend using `--upgrade` or `-U` to ensure you have the latest version of the library.*\n\n### From Source\n\nThis client uses `poetry` as a dependency and virtual environment manager.\n\nYou can install poetry with\n\n```bash\npip install poetry\n```\n\n`poetry` will set up a virtual environment and install dependencies with the following command:\n\n```bash\npoetry install\n```\n\n## Getting Started\n\nBelow is a non-streamed sync and async example for the ShuttleAI API. You can find more examples in the `examples/` directory.\n\n### Synchronous Client\n\n```python\nfrom shuttleai import ShuttleAI\n\nshuttleai = ShuttleAI()\n\nresponse = shuttleai.chat.completions.create(\n model=\"shuttle-2.5\",\n messages=[{\"role\": \"user\", \"content\": \"What day is today?\"}],\n internet=True\n)\n\nprint(chunk.choices[0].message.content)\n```\n\n### Asynchronous Client\n\n```python\nimport asyncio\nfrom shuttleai import AsyncShuttleAI\n\nasync def main():\n shuttleai = AsyncShuttleAI()\n\n response = await shuttleai.chat.completions.create(\n model=\"shuttle-2.5\",\n messages=[{\"role\": \"user\", \"content\": \"Imagine an AI like no other, its name is ShuttleAI.\"}],\n )\n\n print(response.choices[0].message.content)\n\nasyncio.run(main())\n```\n\n### ShuttleAI CLI\n\nScroll down to the [Scripts](#scripts) section for more information.\n\n## Run examples\n\nYou can run the examples in the `examples/` directory using `poetry run` or by entering the virtual environment using `poetry shell`.\n\n### Using poetry run\n\n```bash\ncd examples\npoetry run python chat_no_streaming.py\n```\n\n### Using poetry shell\n\n```bash\npoetry shell\ncd examples\n\n>> python chat_no_streaming.py\n```\n\n## API Key Setup\n\nTo use the ShuttleAI API, you need to have an API key. \nYou can get a **FREE** API key by signing up at \n[shuttleai.com](https://shuttleai.com) and heading to \nthe [key management page](https://shuttleai.com/keys).\n\nAfter you have an API key, you can set it as an environment variable:\n\n### Windows\n\n```s\nsetx SHUTTLEAI_API_KEY \"<your_api_key>\"\n```\n> [!Note]\n> This will only work in the current terminal session. To set it permanently, you can use the `setx` command with the `/m` flag.\n\n```s\nsetx SHUTTLEAI_API_KEY \"<your_api_key>\" /m\n```\n\n### macOS/Linux\n\n```bash\nexport SHUTTLEAI_API_KEY=<your_api_key>\n```\n\n## Contribution\nWe welcome and appreciate contributions to the ShuttleAI API Python SDK.\nPlease see the [contribution guide](CONTRIBUTING.md) for more information.\n*Benefits may apply! :smile:*\n\n## Scripts\n### Formatting/Checks\n- `poetry run ruff check shuttleai` - Check ruff\n- `poetry run black shuttleai --diff --color` - Check black\n- `poetry run black shuttleai` - Format code\n- `poetry run mypy shuttleai` - Check for type errors\n\n### Tools\n- `poetry run clean` - Clean up the project directory\n- `poetry run key` - Display your default API key (if set by environment variable)\n- `poetry run contr` - Display contributors\n\n### ShuttleAI CLI\n- `poetry run shuttleai` or `shuttleai` - Run the ShuttleAI CLI.\n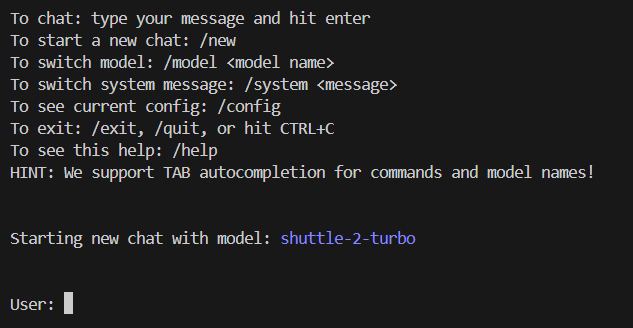\n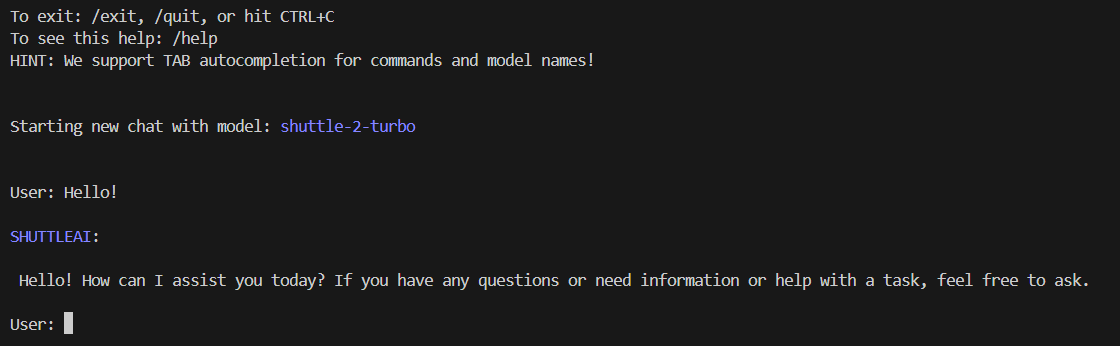\n> [!Important]\n> We support auto TAB completion of commands and model names! Just press `TAB`!\n\n",
"bugtrack_url": null,
"license": null,
"summary": "Access Shuttle AI's API via an easy-to-use Python wrapper. Dashboard: https://shuttleai.com Discord: https://discord.gg/shuttleai",
"version": "4.7.2",
"project_urls": {
"Dashboard": "https://shuttleai.com",
"Discord": "https://discord.gg/shuttleai",
"Documentation": "https://docs.shuttleai.com",
"Homepage": "https://github.com/shuttleai",
"PyPI": "https://pypi.org/project/shuttleai",
"Repository": "https://github.com/shuttleai/shuttleai-python"
},
"split_keywords": [
"shuttleai",
" ai",
" gpt",
" claude",
" api",
" free",
" chatgpt",
" gpt-4"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "1ae3a4b91bbc6a6e5c6b9064aa34f3807969bcc4c9f4b72a073043ea835104d8",
"md5": "9414cc85db5569fe04d7272c4e832a27",
"sha256": "c66dcbd04ae50547b296781cf32cd632dbec7051e5547ffa5f1838ab5376046a"
},
"downloads": -1,
"filename": "shuttleai-4.7.2-py3-none-any.whl",
"has_sig": false,
"md5_digest": "9414cc85db5569fe04d7272c4e832a27",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": "<4.0,>=3.9",
"size": 34920,
"upload_time": "2024-09-22T02:53:04",
"upload_time_iso_8601": "2024-09-22T02:53:04.582751Z",
"url": "https://files.pythonhosted.org/packages/1a/e3/a4b91bbc6a6e5c6b9064aa34f3807969bcc4c9f4b72a073043ea835104d8/shuttleai-4.7.2-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "76fc0d0b36d3447e706e058c1798474da69dfa9af94bb11dabe478025fcabfca",
"md5": "497df64aeb2c27aa596fa937a40d9e4b",
"sha256": "4ae94c7f3e508ae1ec7b5fbb2e01d01c0ede4efb1dae8baf66ef0b5dafce2622"
},
"downloads": -1,
"filename": "shuttleai-4.7.2.tar.gz",
"has_sig": false,
"md5_digest": "497df64aeb2c27aa596fa937a40d9e4b",
"packagetype": "sdist",
"python_version": "source",
"requires_python": "<4.0,>=3.9",
"size": 23984,
"upload_time": "2024-09-22T02:53:06",
"upload_time_iso_8601": "2024-09-22T02:53:06.046350Z",
"url": "https://files.pythonhosted.org/packages/76/fc/0d0b36d3447e706e058c1798474da69dfa9af94bb11dabe478025fcabfca/shuttleai-4.7.2.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-09-22 02:53:06",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "shuttleai",
"github_project": "shuttleai-python",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "shuttleai"
}