Name | signalrcoreplus JSON |
Version |
0.10.3
JSON |
| download |
home_page | https://github.com/dcmeglio/signalrcore |
Summary | A Python SignalR Core client(json and messagepack), with invocation auth and two way streaming. Compatible with azure / serverless functions. Also with automatic reconnect and manually reconnect. |
upload_time | 2022-12-29 19:15:45 |
maintainer | |
docs_url | None |
author | mandrewcito |
requires_python | |
license | |
keywords |
signalr
core
client
3.1
|
VCS |
 |
bugtrack_url |
|
requirements |
No requirements were recorded.
|
Travis-CI |
|
coveralls test coverage |
No coveralls.
|
# SignalR core client
[](https://www.paypal.me/mandrewcito/1)

[](https://pepy.tech/project/signalrcore/month)
[](https://pepy.tech/project/signalrcore)



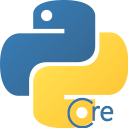
# Links
* [Dev to posts with library examples and implementation](https://dev.to/mandrewcito/singlar-core-python-client-58e7)
* [Pypi](https://pypi.org/project/signalrcore/)
* [Wiki - This Doc](https://mandrewcito.github.io/signalrcore/)
# Develop
Test server will be avaiable in [here](https://github.com/mandrewcito/signalrcore-containertestservers) and docker compose is required.
```bash
git clone https://github.com/mandrewcito/signalrcore-containertestservers
cd signalrcore-containertestservers
docker-compose up
cd ../signalrcore
make tests
```
## Known Issues
Issues related with closing sockets are inherited from the websocket-client library. Due to these problems i can't update the library to versions higher than websocket-client 0.54.0.
I'm working to solve it but for now its patched (Error number 1. Raises an exception, and then exception is treated for prevent errors).
If I update the websocket library I fall into error number 2, on local machine I can't reproduce it but travis builds fail (sometimes and randomly :()
* [1. Closing socket error](https://github.com/slackapi/python-slackclient/issues/171)
* [2. Random errors closing socket](https://github.com/websocket-client/websocket-client/issues/449)
# A Tiny How To
## Connect to a server without auth
```python
hub_connection = HubConnectionBuilder()\
.with_url(server_url)\
.configure_logging(logging.DEBUG)\
.with_automatic_reconnect({
"type": "raw",
"keep_alive_interval": 10,
"reconnect_interval": 5,
"max_attempts": 5
}).build()
```
## Connect to a server with auth
login_function must provide auth token
```python
hub_connection = HubConnectionBuilder()\
.with_url(server_url,
options={
"access_token_factory": login_function,
"headers": {
"mycustomheader": "mycustomheadervalue"
}
})\
.configure_logging(logging.DEBUG)\
.with_automatic_reconnect({
"type": "raw",
"keep_alive_interval": 10,
"reconnect_interval": 5,
"max_attempts": 5
}).build()
```
### Unauthorized errors
A login function must provide an error controller if authorization fails. When connection starts, if authorization fails exception will be propagated.
```python
def login(self):
response = requests.post(
self.login_url,
json={
"username": self.email,
"password": self.password
},verify=False)
if response.status_code == 200:
return response.json()["token"]
raise requests.exceptions.ConnectionError()
hub_connection.start() # this code will raise requests.exceptions.ConnectionError() if auth fails
```
## Configure logging
```python
HubConnectionBuilder()\
.with_url(server_url,
.configure_logging(logging.DEBUG)
...
```
## Configure socket trace
```python
HubConnectionBuilder()\
.with_url(server_url,
.configure_logging(logging.DEBUG, socket_trace=True)
...
```
## Configure your own handler
```python
import logging
handler = logging.StreamHandler()
handler.setLevel(logging.DEBUG)
hub_connection = HubConnectionBuilder()\
.with_url(server_url, options={"verify_ssl": False}) \
.configure_logging(logging.DEBUG, socket_trace=True, handler=handler)
...
```
## Configuring reconnection
After reaching max_attempts an exeption will be thrown and on_disconnect event will be fired.
```python
hub_connection = HubConnectionBuilder()\
.with_url(server_url)\
...
.build()
```
## Configuring additional headers
```python
hub_connection = HubConnectionBuilder()\
.with_url(server_url,
options={
"headers": {
"mycustomheader": "mycustomheadervalue"
}
})
...
.build()
```
## Configuring additional querystring parameters
```python
server_url ="http.... /?myquerystringparam=134&foo=bar"
connection = HubConnectionBuilder()\
.with_url(server_url,
options={
})\
.build()
```
## Congfiguring skip negotiation
```python
hub_connection = HubConnectionBuilder() \
.with_url("ws://"+server_url, options={
"verify_ssl": False,
"skip_negotiation": False,
"headers": {
}
}) \
.configure_logging(logging.DEBUG, socket_trace=True, handler=handler) \
.build()
```
## Configuring ping(keep alive)
keep_alive_interval sets the seconds of ping message
```python
hub_connection = HubConnectionBuilder()\
.with_url(server_url)\
.configure_logging(logging.DEBUG)\
.with_automatic_reconnect({
"type": "raw",
"keep_alive_interval": 10,
"reconnect_interval": 5,
"max_attempts": 5
}).build()
```
## Configuring logging
```python
hub_connection = HubConnectionBuilder()\
.with_url(server_url)\
.configure_logging(logging.DEBUG)\
.with_automatic_reconnect({
"type": "raw",
"keep_alive_interval": 10,
"reconnect_interval": 5,
"max_attempts": 5
}).build()
```
## Configure messagepack
```python
from signalrcore.protocol.messagepack_protocol import MessagePackHubProtocol
HubConnectionBuilder()\
.with_url(self.server_url, options={"verify_ssl":False})\
...
.with_hub_protocol(MessagePackHubProtocol())\
...
.build()
```
## Events
### On Connect / On Disconnect
on_open - fires when connection is opened and ready to send messages
on_close - fires when connection is closed
```python
hub_connection.on_open(lambda: print("connection opened and handshake received ready to send messages"))
hub_connection.on_close(lambda: print("connection closed"))
```
### On Hub Error (Hub Exceptions ...)
```
hub_connection.on_error(lambda data: print(f"An exception was thrown closed{data.error}"))
```
### Register an operation
ReceiveMessage - signalr method
print - function that has as parameters args of signalr method
```python
hub_connection.on("ReceiveMessage", print)
```
## Sending messages
SendMessage - signalr method
username, message - parameters of signalrmethod
```python
hub_connection.send("SendMessage", [username, message])
```
## Sending messages with callback
SendMessage - signalr method
username, message - parameters of signalrmethod
```python
send_callback_received = threading.Lock()
send_callback_received.acquire()
self.connection.send(
"SendMessage", # Method
[self.username, self.message], # Params
lambda m: send_callback_received.release()) # Callback
if not send_callback_received.acquire(timeout=1):
raise ValueError("CALLBACK NOT RECEIVED")
```
## Requesting streaming (Server to client)
```python
hub_connection.stream(
"Counter",
[len(self.items), 500]).subscribe({
"next": self.on_next,
"complete": self.on_complete,
"error": self.on_error
})
```
## Client side Streaming
```python
from signalrcore.subject import Subject
subject = Subject()
# Start Streaming
hub_connection.send("UploadStream", subject)
# Each iteration
subject.next(str(iteration))
# End streaming
subject.complete()
```
# Full Examples
Examples will be avaiable [here](https://github.com/mandrewcito/signalrcore/tree/master/test/examples)
It were developed using package from [aspnet core - SignalRChat](https://codeload.github.com/aspnet/Docs/zip/master)
## Chat example
A mini example could be something like this:
```python
import logging
import sys
from signalrcore.hub_connection_builder import HubConnectionBuilder
def input_with_default(input_text, default_value):
value = input(input_text.format(default_value))
return default_value if value is None or value.strip() == "" else value
server_url = input_with_default('Enter your server url(default: {0}): ', "wss://localhost:44376/chatHub")
username = input_with_default('Enter your username (default: {0}): ', "mandrewcito")
handler = logging.StreamHandler()
handler.setLevel(logging.DEBUG)
hub_connection = HubConnectionBuilder()\
.with_url(server_url, options={"verify_ssl": False}) \
.configure_logging(logging.DEBUG, socket_trace=True, handler=handler) \
.with_automatic_reconnect({
"type": "interval",
"keep_alive_interval": 10,
"intervals": [1, 3, 5, 6, 7, 87, 3]
}).build()
hub_connection.on_open(lambda: print("connection opened and handshake received ready to send messages"))
hub_connection.on_close(lambda: print("connection closed"))
hub_connection.on("ReceiveMessage", print)
hub_connection.start()
message = None
# Do login
while message != "exit()":
message = input(">> ")
if message is not None and message != "" and message != "exit()":
hub_connection.send("SendMessage", [username, message])
hub_connection.stop()
sys.exit(0)
```
Raw data
{
"_id": null,
"home_page": "https://github.com/dcmeglio/signalrcore",
"name": "signalrcoreplus",
"maintainer": "",
"docs_url": null,
"requires_python": "",
"maintainer_email": "",
"keywords": "signalr core client 3.1",
"author": "mandrewcito",
"author_email": "anbaalo@gmail.com",
"download_url": "https://files.pythonhosted.org/packages/64/ea/5498299c0832a581b068c7e7cb86dc4ef580b61f414dbef010acf84f9cfd/signalrcoreplus-0.10.3.tar.gz",
"platform": null,
"description": "# SignalR core client\r\n[](https://www.paypal.me/mandrewcito/1)\r\n\r\n[](https://pepy.tech/project/signalrcore/month)\r\n[](https://pepy.tech/project/signalrcore)\r\n\r\n\r\n\r\n\r\n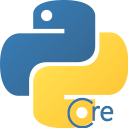\r\n\r\n\r\n# Links \r\n\r\n* [Dev to posts with library examples and implementation](https://dev.to/mandrewcito/singlar-core-python-client-58e7)\r\n\r\n* [Pypi](https://pypi.org/project/signalrcore/)\r\n\r\n* [Wiki - This Doc](https://mandrewcito.github.io/signalrcore/)\r\n\r\n# Develop\r\n\r\nTest server will be avaiable in [here](https://github.com/mandrewcito/signalrcore-containertestservers) and docker compose is required.\r\n\r\n```bash\r\ngit clone https://github.com/mandrewcito/signalrcore-containertestservers\r\ncd signalrcore-containertestservers\r\ndocker-compose up\r\ncd ../signalrcore\r\nmake tests\r\n```\r\n\r\n## Known Issues\r\n\r\nIssues related with closing sockets are inherited from the websocket-client library. Due to these problems i can't update the library to versions higher than websocket-client 0.54.0. \r\nI'm working to solve it but for now its patched (Error number 1. Raises an exception, and then exception is treated for prevent errors). \r\nIf I update the websocket library I fall into error number 2, on local machine I can't reproduce it but travis builds fail (sometimes and randomly :()\r\n* [1. Closing socket error](https://github.com/slackapi/python-slackclient/issues/171)\r\n* [2. Random errors closing socket](https://github.com/websocket-client/websocket-client/issues/449)\r\n\r\n# A Tiny How To\r\n\r\n## Connect to a server without auth\r\n\r\n```python\r\nhub_connection = HubConnectionBuilder()\\\r\n .with_url(server_url)\\\r\n .configure_logging(logging.DEBUG)\\\r\n .with_automatic_reconnect({\r\n \"type\": \"raw\",\r\n \"keep_alive_interval\": 10,\r\n \"reconnect_interval\": 5,\r\n \"max_attempts\": 5\r\n }).build()\r\n```\r\n## Connect to a server with auth\r\n\r\nlogin_function must provide auth token\r\n\r\n```python\r\nhub_connection = HubConnectionBuilder()\\\r\n .with_url(server_url,\r\n options={\r\n \"access_token_factory\": login_function,\r\n \"headers\": {\r\n \"mycustomheader\": \"mycustomheadervalue\"\r\n }\r\n })\\\r\n .configure_logging(logging.DEBUG)\\\r\n .with_automatic_reconnect({\r\n \"type\": \"raw\",\r\n \"keep_alive_interval\": 10,\r\n \"reconnect_interval\": 5,\r\n \"max_attempts\": 5\r\n }).build()\r\n```\r\n### Unauthorized errors\r\nA login function must provide an error controller if authorization fails. When connection starts, if authorization fails exception will be propagated.\r\n\r\n```python\r\n def login(self):\r\n response = requests.post(\r\n self.login_url,\r\n json={\r\n \"username\": self.email,\r\n \"password\": self.password\r\n },verify=False)\r\n if response.status_code == 200:\r\n return response.json()[\"token\"]\r\n raise requests.exceptions.ConnectionError()\r\n\r\n hub_connection.start() # this code will raise requests.exceptions.ConnectionError() if auth fails\r\n```\r\n## Configure logging\r\n\r\n```python\r\nHubConnectionBuilder()\\\r\n .with_url(server_url,\r\n .configure_logging(logging.DEBUG)\r\n ...\r\n```\r\n## Configure socket trace\r\n```python \r\nHubConnectionBuilder()\\\r\n .with_url(server_url,\r\n .configure_logging(logging.DEBUG, socket_trace=True) \r\n ... \r\n ```\r\n## Configure your own handler\r\n```python\r\n import logging\r\nhandler = logging.StreamHandler()\r\nhandler.setLevel(logging.DEBUG)\r\nhub_connection = HubConnectionBuilder()\\\r\n .with_url(server_url, options={\"verify_ssl\": False}) \\\r\n .configure_logging(logging.DEBUG, socket_trace=True, handler=handler)\r\n ...\r\n ```\r\n## Configuring reconnection\r\nAfter reaching max_attempts an exeption will be thrown and on_disconnect event will be fired.\r\n```python\r\nhub_connection = HubConnectionBuilder()\\\r\n .with_url(server_url)\\\r\n ...\r\n .build()\r\n```\r\n## Configuring additional headers\r\n```python\r\nhub_connection = HubConnectionBuilder()\\\r\n .with_url(server_url,\r\n options={\r\n \"headers\": {\r\n \"mycustomheader\": \"mycustomheadervalue\"\r\n }\r\n })\r\n ...\r\n .build()\r\n```\r\n## Configuring additional querystring parameters\r\n```python\r\nserver_url =\"http.... /?myquerystringparam=134&foo=bar\"\r\nconnection = HubConnectionBuilder()\\\r\n .with_url(server_url,\r\n options={\r\n })\\\r\n .build()\r\n```\r\n## Congfiguring skip negotiation\r\n```python\r\nhub_connection = HubConnectionBuilder() \\\r\n .with_url(\"ws://\"+server_url, options={\r\n \"verify_ssl\": False,\r\n \"skip_negotiation\": False,\r\n \"headers\": {\r\n }\r\n }) \\\r\n .configure_logging(logging.DEBUG, socket_trace=True, handler=handler) \\\r\n .build()\r\n\r\n```\r\n## Configuring ping(keep alive)\r\n\r\nkeep_alive_interval sets the seconds of ping message\r\n\r\n```python\r\nhub_connection = HubConnectionBuilder()\\\r\n .with_url(server_url)\\\r\n .configure_logging(logging.DEBUG)\\\r\n .with_automatic_reconnect({\r\n \"type\": \"raw\",\r\n \"keep_alive_interval\": 10,\r\n \"reconnect_interval\": 5,\r\n \"max_attempts\": 5\r\n }).build()\r\n```\r\n## Configuring logging\r\n```python\r\nhub_connection = HubConnectionBuilder()\\\r\n .with_url(server_url)\\\r\n .configure_logging(logging.DEBUG)\\\r\n .with_automatic_reconnect({\r\n \"type\": \"raw\",\r\n \"keep_alive_interval\": 10,\r\n \"reconnect_interval\": 5,\r\n \"max_attempts\": 5\r\n }).build()\r\n```\r\n\r\n## Configure messagepack\r\n\r\n```python\r\nfrom signalrcore.protocol.messagepack_protocol import MessagePackHubProtocol\r\n\r\nHubConnectionBuilder()\\\r\n .with_url(self.server_url, options={\"verify_ssl\":False})\\\r\n ... \r\n .with_hub_protocol(MessagePackHubProtocol())\\\r\n ...\r\n .build()\r\n```\r\n## Events\r\n\r\n### On Connect / On Disconnect\r\non_open - fires when connection is opened and ready to send messages\r\non_close - fires when connection is closed\r\n```python\r\nhub_connection.on_open(lambda: print(\"connection opened and handshake received ready to send messages\"))\r\nhub_connection.on_close(lambda: print(\"connection closed\"))\r\n\r\n```\r\n### On Hub Error (Hub Exceptions ...)\r\n```\r\nhub_connection.on_error(lambda data: print(f\"An exception was thrown closed{data.error}\"))\r\n```\r\n### Register an operation \r\nReceiveMessage - signalr method\r\nprint - function that has as parameters args of signalr method\r\n```python\r\nhub_connection.on(\"ReceiveMessage\", print)\r\n```\r\n## Sending messages\r\nSendMessage - signalr method\r\nusername, message - parameters of signalrmethod\r\n```python\r\n hub_connection.send(\"SendMessage\", [username, message])\r\n```\r\n\r\n## Sending messages with callback\r\nSendMessage - signalr method\r\nusername, message - parameters of signalrmethod\r\n```python\r\n send_callback_received = threading.Lock()\r\n send_callback_received.acquire()\r\n self.connection.send(\r\n \"SendMessage\", # Method\r\n [self.username, self.message], # Params\r\n lambda m: send_callback_received.release()) # Callback\r\n if not send_callback_received.acquire(timeout=1):\r\n raise ValueError(\"CALLBACK NOT RECEIVED\")\r\n```\r\n\r\n## Requesting streaming (Server to client)\r\n```python\r\nhub_connection.stream(\r\n \"Counter\",\r\n [len(self.items), 500]).subscribe({\r\n \"next\": self.on_next,\r\n \"complete\": self.on_complete,\r\n \"error\": self.on_error\r\n })\r\n```\r\n## Client side Streaming\r\n```python\r\nfrom signalrcore.subject import Subject\r\n\r\nsubject = Subject()\r\n\r\n# Start Streaming\r\nhub_connection.send(\"UploadStream\", subject)\r\n\r\n# Each iteration\r\nsubject.next(str(iteration))\r\n\r\n# End streaming\r\nsubject.complete()\r\n```\r\n\r\n# Full Examples\r\n\r\nExamples will be avaiable [here](https://github.com/mandrewcito/signalrcore/tree/master/test/examples)\r\nIt were developed using package from [aspnet core - SignalRChat](https://codeload.github.com/aspnet/Docs/zip/master) \r\n\r\n## Chat example\r\nA mini example could be something like this:\r\n\r\n```python\r\nimport logging\r\nimport sys\r\nfrom signalrcore.hub_connection_builder import HubConnectionBuilder\r\n\r\n\r\ndef input_with_default(input_text, default_value):\r\n value = input(input_text.format(default_value))\r\n return default_value if value is None or value.strip() == \"\" else value\r\n\r\n\r\nserver_url = input_with_default('Enter your server url(default: {0}): ', \"wss://localhost:44376/chatHub\")\r\nusername = input_with_default('Enter your username (default: {0}): ', \"mandrewcito\")\r\nhandler = logging.StreamHandler()\r\nhandler.setLevel(logging.DEBUG)\r\nhub_connection = HubConnectionBuilder()\\\r\n .with_url(server_url, options={\"verify_ssl\": False}) \\\r\n .configure_logging(logging.DEBUG, socket_trace=True, handler=handler) \\\r\n .with_automatic_reconnect({\r\n \"type\": \"interval\",\r\n \"keep_alive_interval\": 10,\r\n \"intervals\": [1, 3, 5, 6, 7, 87, 3]\r\n }).build()\r\n\r\nhub_connection.on_open(lambda: print(\"connection opened and handshake received ready to send messages\"))\r\nhub_connection.on_close(lambda: print(\"connection closed\"))\r\n\r\nhub_connection.on(\"ReceiveMessage\", print)\r\nhub_connection.start()\r\nmessage = None\r\n\r\n# Do login\r\n\r\nwhile message != \"exit()\":\r\n message = input(\">> \")\r\n if message is not None and message != \"\" and message != \"exit()\":\r\n hub_connection.send(\"SendMessage\", [username, message])\r\n\r\nhub_connection.stop()\r\n\r\nsys.exit(0)\r\n\r\n```\r\n",
"bugtrack_url": null,
"license": "",
"summary": "A Python SignalR Core client(json and messagepack), with invocation auth and two way streaming. Compatible with azure / serverless functions. Also with automatic reconnect and manually reconnect.",
"version": "0.10.3",
"split_keywords": [
"signalr",
"core",
"client",
"3.1"
],
"urls": [
{
"comment_text": "",
"digests": {
"md5": "74c71146c5e04350323825fca194fc41",
"sha256": "87c69c629cadc30c63d55fb7d15bbcf601ce240a763d816d64f3b4bb2dea4ae9"
},
"downloads": -1,
"filename": "signalrcoreplus-0.10.3-py3-none-any.whl",
"has_sig": false,
"md5_digest": "74c71146c5e04350323825fca194fc41",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": null,
"size": 35912,
"upload_time": "2022-12-29T19:15:41",
"upload_time_iso_8601": "2022-12-29T19:15:41.194684Z",
"url": "https://files.pythonhosted.org/packages/80/b9/18a33fe78904501987e00699838c5269f67bf5f6ebadbdb1b863682779ea/signalrcoreplus-0.10.3-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"md5": "6e2abd2171d84bfc7341925f5e62ee7d",
"sha256": "3bdc1697d240d01150e0dfd58d7f10158620842e55b6a552decef78b6eb38d5d"
},
"downloads": -1,
"filename": "signalrcoreplus-0.10.3.tar.gz",
"has_sig": false,
"md5_digest": "6e2abd2171d84bfc7341925f5e62ee7d",
"packagetype": "sdist",
"python_version": "source",
"requires_python": null,
"size": 24619,
"upload_time": "2022-12-29T19:15:45",
"upload_time_iso_8601": "2022-12-29T19:15:45.562414Z",
"url": "https://files.pythonhosted.org/packages/64/ea/5498299c0832a581b068c7e7cb86dc4ef580b61f414dbef010acf84f9cfd/signalrcoreplus-0.10.3.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2022-12-29 19:15:45",
"github": true,
"gitlab": false,
"bitbucket": false,
"github_user": "dcmeglio",
"github_project": "signalrcore",
"travis_ci": true,
"coveralls": false,
"github_actions": true,
"requirements": [],
"lcname": "signalrcoreplus"
}