# Simple workS with neural networks #
## What is this? ##
The module allows you to work with simple neural networks (At the moment, the simplest convolutional neural network model is used with the method of backpropagation of error and sigmoidal activation function).
## Quick Guide ##
The module is based on the following structure:
from simple_neural_works import Neuro
a = [25,30,30,20,10]
ne = Neuro(a,0.1)
ne.fill(False)
in = [0.5,1,1,0,0.001] # some values to input
a = ne.get_result(in,False)
ou = [1,1,1,1,0,1,0,0,1] # some values to train
ne.backpropagation(ou,False)
ne.save_m("filename.res",False)
Which Python provides by standard.
----------
### Using ###
Using the library is as simple and convenient as possible:
First, import main module using "from simple_neural_works import Neuro"
The second, you need to load or create an array with data using the load() or fill() function.
Examples of all operations:
Creating an instance of a neural network with which you will then work.
ne = Neuro(array_width_of_slices_neaural_network,speed of backpropagation)
Filling the weights with initial random values is used to create a neural network from scratch:
ne.fill(mute)
To load previously saved values from a file:
ne.load("filenamehere.txt",mute)
Function used to obtain the result of a neural network calculation:
ne.get_result([some float or int values to input in array],mute)
To train a neural network, use the following function. The input is an array, which should be the output of the neural network, the learning rate is controlled by the internal variable `ne.spd`, set manually and during network initialization. Only used after the `get_result()` or `image_get_result()` function.
ne.backpropagation([some int or float values to train in array],mute)
To quickly save an array of weights:
ne.save("filenamehere.txt",mute)
For compact (up to two times smaller) but slower saving:
ne.zip_save("filename.txt",mute)
Blank for recognizing monochrome numbers. To read data from a PNG image (the image is inverted in color, that is, you need to draw it black, although this is not so important). To avoid specifying the entire path to the file, start the file name with "./".
ne.image_get_result("filename.png",mute)
----------
### Structure ###
Here are the main variables used in the library; for details, please refer directly to the library code, everything is described there in great detail.
An array storing weight values:
ne.w
An array storing the output values of the activation function:
ne.ou
Speed of backpropagation (between 0 and 1). If you don’t want to bother, then set it to 0.1. In more detail, at first you can use 1, towards the end of training 0.1:
ne.speed
Please do not change the width array while working, this will cause the operation to malfunction.
----------
----------
## Developer ##
My GitHub: [link](https://github.com/TwentyOneError)
My Email: ourmail20210422@gmail.com
----------
I would be glad if someone knowledgeable about the topic gives advice or points out errors.
----------
Русский гайд будет позже, я так знатно подзаколебался писать гайд на английском на никому ненужный кусок говна написанный на коленке.
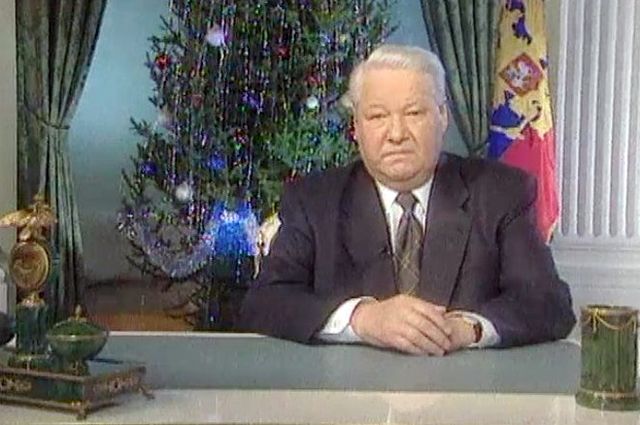
### Я устал. Я сделал все что мог. ###
Raw data
{
"_id": null,
"home_page": "https://github.com/TwentyOneError/simple_neural_works",
"name": "simple-neural-works",
"maintainer": null,
"docs_url": null,
"requires_python": ">=3.8",
"maintainer_email": null,
"keywords": "neural networks simple backpropagation",
"author": "TwentyOneError",
"author_email": "ourmail20210422@gmail.com",
"download_url": "https://files.pythonhosted.org/packages/16/ef/7e337c4a3d83e45f83aed8f2ffe8ee1f1487faa40fe0211e08a418893bdb/simple_neural_works-1.0.0.tar.gz",
"platform": null,
"description": "# Simple workS with neural networks #\r\n\r\n## What is this? ##\r\nThe module allows you to work with simple neural networks (At the moment, the simplest convolutional neural network model is used with the method of backpropagation of error and sigmoidal activation function).\r\n\r\n## Quick Guide ##\r\nThe module is based on the following structure:\r\n\r\n \r\n from simple_neural_works import Neuro\r\n a = [25,30,30,20,10]\r\n ne = Neuro(a,0.1)\r\n ne.fill(False)\r\n in = [0.5,1,1,0,0.001] # some values to input\r\n a = ne.get_result(in,False)\r\n ou = [1,1,1,1,0,1,0,0,1] # some values to train\r\n ne.backpropagation(ou,False)\r\n ne.save_m(\"filename.res\",False)\r\n \r\n \r\n\r\nWhich Python provides by standard.\r\n\r\n\r\n----------\r\n\r\n\r\n### Using ###\r\n\r\n\r\nUsing the library is as simple and convenient as possible:\r\n\r\nFirst, import main module using \"from simple_neural_works import Neuro\"\r\n\r\nThe second, you need to load or create an array with data using the load() or fill() function.\r\n\r\nExamples of all operations:\r\n\r\nCreating an instance of a neural network with which you will then work.\r\n\r\n ne = Neuro(array_width_of_slices_neaural_network,speed of backpropagation)\r\n\r\n\r\nFilling the weights with initial random values is used to create a neural network from scratch:\r\n\r\n ne.fill(mute)\r\n\r\n\r\nTo load previously saved values from a file:\r\n\r\n ne.load(\"filenamehere.txt\",mute)\r\n \r\n\r\nFunction used to obtain the result of a neural network calculation:\r\n\r\n ne.get_result([some float or int values to input in array],mute)\r\n\r\n\r\nTo train a neural network, use the following function. The input is an array, which should be the output of the neural network, the learning rate is controlled by the internal variable `ne.spd`, set manually and during network initialization. Only used after the `get_result()` or `image_get_result()` function.\r\n\r\n ne.backpropagation([some int or float values to train in array],mute)\r\n\r\n\r\nTo quickly save an array of weights:\r\n\r\n ne.save(\"filenamehere.txt\",mute)\r\n\r\n\r\nFor compact (up to two times smaller) but slower saving:\r\n\r\n ne.zip_save(\"filename.txt\",mute)\r\n\r\n\r\nBlank for recognizing monochrome numbers. To read data from a PNG image (the image is inverted in color, that is, you need to draw it black, although this is not so important). To avoid specifying the entire path to the file, start the file name with \"./\".\r\n\r\n ne.image_get_result(\"filename.png\",mute)\r\n\r\n\r\n----------\r\n\r\n\r\n### Structure ###\r\n\r\nHere are the main variables used in the library; for details, please refer directly to the library code, everything is described there in great detail.\r\n\r\nAn array storing weight values:\r\n \r\n ne.w\r\n\r\nAn array storing the output values of the activation function:\r\n\r\n ne.ou\r\n\r\n\r\nSpeed of backpropagation (between 0 and 1). If you don\u0432\u0402\u2122t want to bother, then set it to 0.1. In more detail, at first you can use 1, towards the end of training 0.1:\r\n\r\n ne.speed\r\n\r\nPlease do not change the width array while working, this will cause the operation to malfunction.\r\n----------\r\n\r\n----------\r\n\r\n## Developer ##\r\n\r\nMy GitHub: [link](https://github.com/TwentyOneError)\r\n\r\nMy Email: ourmail20210422@gmail.com\r\n\r\n----------\r\n\r\nI would be glad if someone knowledgeable about the topic gives advice or points out errors.\r\n\r\n----------\r\n\r\n\u0420\u00a0\u0421\u0453\u0421\u0403\u0421\u0403\u0420\u0454\u0420\u0451\u0420\u2116 \u0420\u0456\u0420\u00b0\u0420\u2116\u0420\u0491 \u0420\u00b1\u0421\u0453\u0420\u0491\u0420\u00b5\u0421\u201a \u0420\u0457\u0420\u0455\u0420\u00b7\u0420\u00b6\u0420\u00b5, \u0421\u040f \u0421\u201a\u0420\u00b0\u0420\u0454 \u0420\u00b7\u0420\u0405\u0420\u00b0\u0421\u201a\u0420\u0405\u0420\u0455 \u0420\u0457\u0420\u0455\u0420\u0491\u0420\u00b7\u0420\u00b0\u0420\u0454\u0420\u0455\u0420\u00bb\u0420\u00b5\u0420\u00b1\u0420\u00b0\u0420\u00bb\u0421\u0403\u0421\u040f \u0420\u0457\u0420\u0451\u0421\u0403\u0420\u00b0\u0421\u201a\u0421\u040a \u0420\u0456\u0420\u00b0\u0420\u2116\u0420\u0491 \u0420\u0405\u0420\u00b0 \u0420\u00b0\u0420\u0405\u0420\u0456\u0420\u00bb\u0420\u0451\u0420\u2116\u0421\u0403\u0420\u0454\u0420\u0455\u0420\u0458 \u0420\u0405\u0420\u00b0 \u0420\u0405\u0420\u0451\u0420\u0454\u0420\u0455\u0420\u0458\u0421\u0453 \u0420\u0405\u0420\u00b5\u0420\u0405\u0421\u0453\u0420\u00b6\u0420\u0405\u0421\u2039\u0420\u2116 \u0420\u0454\u0421\u0453\u0421\u0403\u0420\u0455\u0420\u0454 \u0420\u0456\u0420\u0455\u0420\u0406\u0420\u0405\u0420\u00b0 \u0420\u0405\u0420\u00b0\u0420\u0457\u0420\u0451\u0421\u0403\u0420\u00b0\u0420\u0405\u0420\u0405\u0421\u2039\u0420\u2116 \u0420\u0405\u0420\u00b0 \u0420\u0454\u0420\u0455\u0420\u00bb\u0420\u00b5\u0420\u0405\u0420\u0454\u0420\u00b5.\r\n\r\n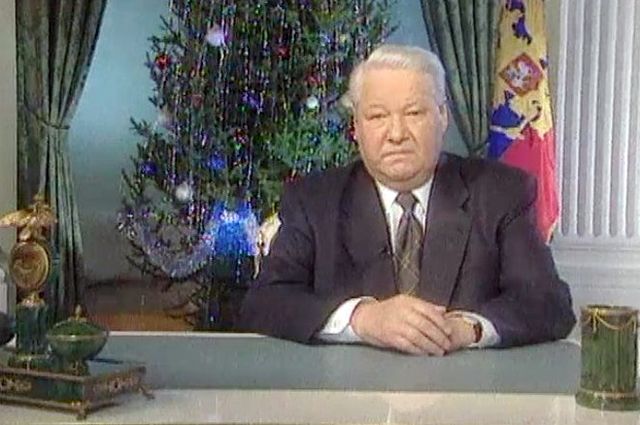\r\n\r\n### \u0420\u0407 \u0421\u0453\u0421\u0403\u0421\u201a\u0420\u00b0\u0420\u00bb. \u0420\u0407 \u0421\u0403\u0420\u0491\u0420\u00b5\u0420\u00bb\u0420\u00b0\u0420\u00bb \u0420\u0406\u0421\u0403\u0420\u00b5 \u0421\u2021\u0421\u201a\u0420\u0455 \u0420\u0458\u0420\u0455\u0420\u0456. ###\r\n\r\n",
"bugtrack_url": null,
"license": null,
"summary": "This is the simplest module for quick work with neural networks.",
"version": "1.0.0",
"project_urls": {
"GitHub": "https://github.com/TwentyOneError",
"Homepage": "https://github.com/TwentyOneError/simple_neural_works"
},
"split_keywords": [
"neural",
"networks",
"simple",
"backpropagation"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "570382667243de06ef1fd3f23fe417a7e410699f5cad22599cdc066af04a0ae9",
"md5": "cc5d90a19e9f9f3fc7c1eed31158bf07",
"sha256": "4f777d3d8c38a2a2e20c84779e8bfe973395f8f7ebeb3b1deb72cc12427c571e"
},
"downloads": -1,
"filename": "simple_neural_works-1.0.0-py3-none-any.whl",
"has_sig": false,
"md5_digest": "cc5d90a19e9f9f3fc7c1eed31158bf07",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.8",
"size": 14709,
"upload_time": "2024-04-20T18:10:44",
"upload_time_iso_8601": "2024-04-20T18:10:44.991712Z",
"url": "https://files.pythonhosted.org/packages/57/03/82667243de06ef1fd3f23fe417a7e410699f5cad22599cdc066af04a0ae9/simple_neural_works-1.0.0-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "16ef7e337c4a3d83e45f83aed8f2ffe8ee1f1487faa40fe0211e08a418893bdb",
"md5": "9087e3ccc36feff59d1f1c04e9aa327b",
"sha256": "60b1f53f4881a51dc48444b83e4d2e0c0ea921b047f7ea0a71f0df32810f0bec"
},
"downloads": -1,
"filename": "simple_neural_works-1.0.0.tar.gz",
"has_sig": false,
"md5_digest": "9087e3ccc36feff59d1f1c04e9aa327b",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.8",
"size": 9162,
"upload_time": "2024-04-20T18:10:46",
"upload_time_iso_8601": "2024-04-20T18:10:46.269462Z",
"url": "https://files.pythonhosted.org/packages/16/ef/7e337c4a3d83e45f83aed8f2ffe8ee1f1487faa40fe0211e08a418893bdb/simple_neural_works-1.0.0.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-04-20 18:10:46",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "TwentyOneError",
"github_project": "simple_neural_works",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"lcname": "simple-neural-works"
}