<div align="center">
[](https://github.com/slickml/slick-ml/actions/workflows/ci.yml)
[](https://github.com/slickml/slick-ml/actions/workflows/cd.yml)
[](https://codecov.io/gh/slickml/slick-ml)
[](https://pepy.tech/project/slickml)
[](https://github.com/slickml/slick-ml/blob/master/LICENSE/)


[](https://www.slickml.com/slack-invite)

</div>
<p align="center">
<a href="https://www.docs.slickml.com/">
<img src="https://raw.githubusercontent.com/slickml/slick-ml/master/assets/designs/logo_clear.png" width="250"></img>
</a>
</p>
<div align="center">
<h1 align="center">SlickMLπ§: Slick Machine Learning in Python</h1>
<p align="center">
<a href="https://github.com/slickml/slick-ml/releases"> Explore Releases</a>
π£
<a href="https://github.com/slickml/slick-ml/blob/master/CONTRIBUTING.md"> Become a Contributor</a>
π£
<a href="https://www.docs.slickml.com"> Explore API Docs</a>
π£
<a href="https://www.slickml.com/slack-invite"> Join our Slack</a>
π£
<a href="https://twitter.com/slickml"> Tweet Us</a>
</p>
</div>
## π§ SlickMLπ§ Philosophy
**SlickML** is an open-source machine learning library written in Python aimed at accelerating the
experimentation time for ML applications with tabular data while maximizing the amount of information
can be inferred. Data Scientists' tasks can often be repetitive such as feature selection, model
tuning, or evaluating metrics for classification and regression problems. We strongly believe that a
good portion of the tasks based on tabular data can be addressed via gradient boosting and generalized
linear models<sup>[1](https://arxiv.org/pdf/2207.08815.pdf)</sup>. SlickML provides Data Scientists
with a toolbox to quickly prototype solutions for a given problem with minimal code while maximizing
the amount of information that can be inferred. Additionally, the prototype solutions can be easily
promoted and served in production with our recommended recipes via various model serving frameworks
including [ZenML](https://github.com/zenml-io/zenml), [BentoML](https://github.com/bentoml/BentoML),
and [Prefect](https://github.com/PrefectHQ/prefect). More details coming soon π€ ...
## π Documentation
β¨ The API documentation is available at [docs.slickml.com](https://www.docs.slickml.com).
## π Installation
To begin with, install [Python version >=3.8,<3.12](https://www.python.org) and to install the library
from [PyPI](https://pypi.org/project/slickml/) simply run πββοΈ :
```
pip install slickml
```
or if you are a [python poetry](https://python-poetry.org/) user, simply run πββοΈ :
```
poetry add slickml
```
π£ Please note that a working [Fortran Compiler](https://gcc.gnu.org/install/) (`gfortran`) is also required to build the package. If you do not have `gcc` installed, the following commands depending on your operating system will take care of this requirement.
```
# Mac Users
brew install gcc
# Linux Users
sudo apt install build-essential gfortran
```
The SlickML CLI tool behaves similarly to many other CLIs for basic features. In order to find out
which version of SlickML you are running, simply run πββοΈ :
```
slickml --version
```
### π Python Virtual Environments
In order to avoid any potential conflicts with other installed Python packages, it is
recommended to use a virtual environment, e.g. [python poetry](https://python-poetry.org/), [python virtualenv](https://docs.python.org/3/library/venv.html), [pyenv virtualenv](https://github.com/pyenv/pyenv-virtualenv), or [conda environment](https://docs.conda.io/projects/conda/en/latest/user-guide/tasks/manage-environments.html). Our recommendation is to use `python-poetry` π₯° for everything π.
## π Quick Start
β
An example to quickly run a `Feature Selection` pipeline with embedded `Cross-Validation` and `Feature-Importance` visualization:
```python
from slickml.feautre_selection import XGBoostFeatureSelector
xfs = XGBoostFeatureSelector()
xfs.fit(X, y)
```
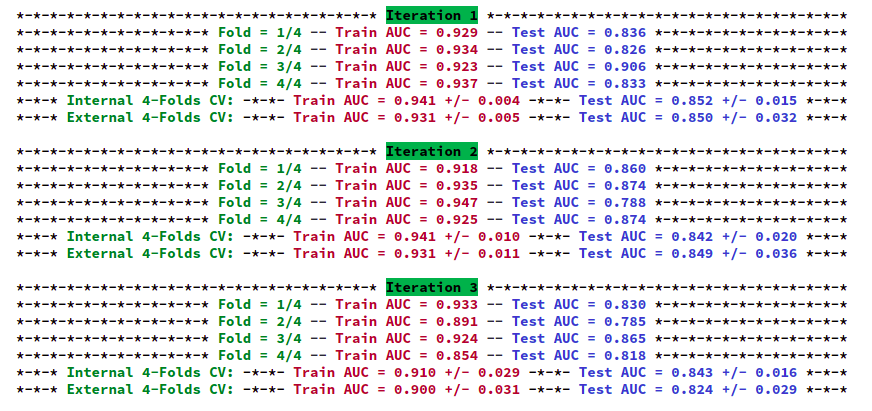
```python
xfs.plot_cv_results()
```

```python
xfs.plot_frequency()
```
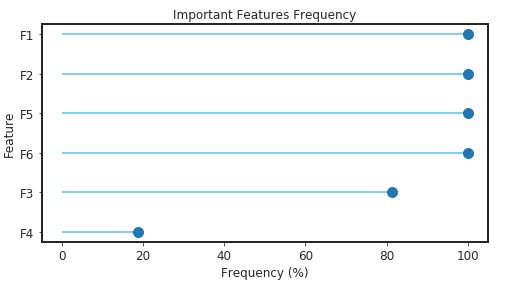
β
An example to quickly find the `tuned hyper-parameter` with `Bayesian Optimization`:
```python
from slickml.optimization import XGBoostBayesianOptimizer
xbo = XGBoostBayesianOptimizer()
xbo.fit(X_train, y_train)
```
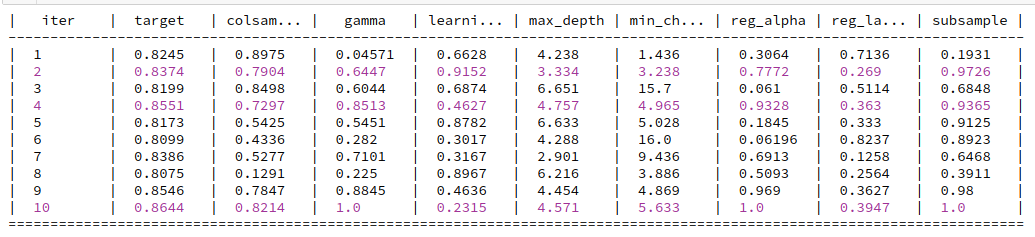
```python
best_params = xbo.get_best_params()
best_params
{"colsample_bytree": 0.8213916662259918,
"gamma": 1.0,
"learning_rate": 0.23148232373451072,
"max_depth": 4,
"min_child_weight": 5.632602921054691,
"reg_alpha": 1.0,
"reg_lambda": 0.39468801734425263,
"subsample": 1.0
}
```
β
An example to quickly train/validate a `XGBoostCV Classifier` with `Cross-Validation`, `Feature-Importance`, and `Shap` visualizations:
```python
from slickml.classification import XGBoostCVClassifier
clf = XGBoostCVClassifier(params=best_params)
clf.fit(X_train, y_train)
y_pred_proba = clf.predict_proba(X_test)
clf.plot_cv_results()
```
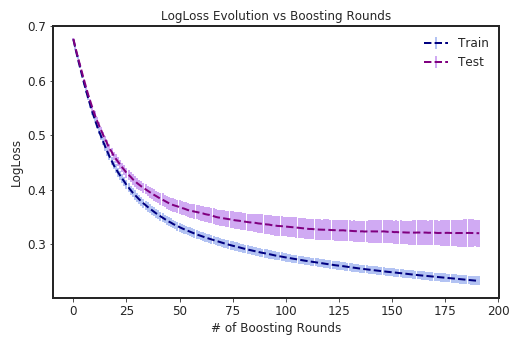
```python
clf.plot_feature_importance()
```
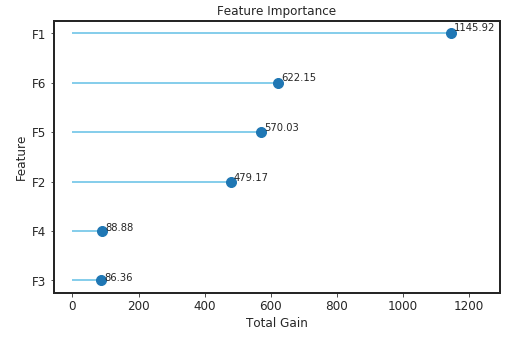
```python
clf.plot_shap_summary(plot_type="violin")
```
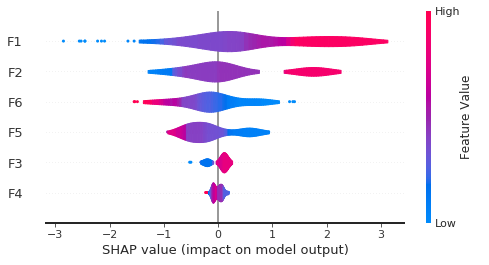
```python
clf.plot_shap_summary(plot_type="layered_violin", layered_violin_max_num_bins=5)
```
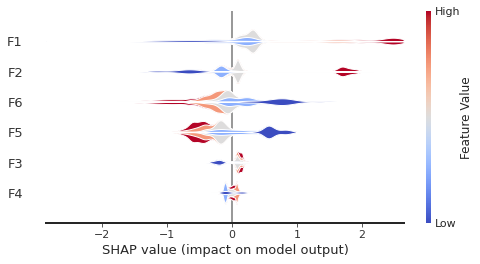
```python
clf.plot_shap_waterfall()
```

β
An example to train/validate a `GLMNetCV Classifier` with `Cross-Validation` and `Coefficients` visualizations:
```python
from slickml.classification import GLMNetCVClassifier
clf = GLMNetCVClassifier(alpha=0.3, n_splits=4, metric="auc")
clf.fit(X_train, y_train)
y_pred_proba = clf.predict_proba(X_test)
clf.plot_cv_results()
```
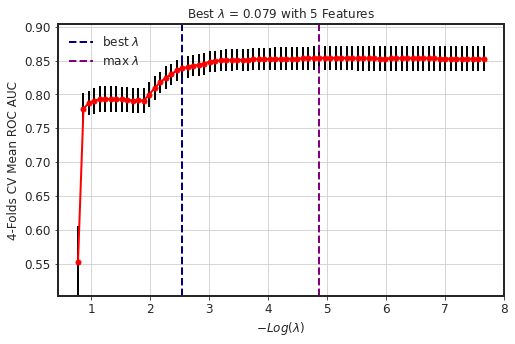
```python
clf.plot_coeff_path()
```
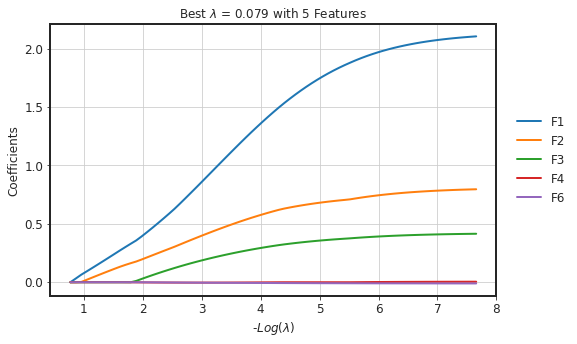
β
An example to quickly visualize the `binary classification metrics` based on multiple `thresholds`:
```python
from slickml.metrics import BinaryClassificationMetrics
clf_metrics = BinaryClassificationMetrics(y_test, y_pred_proba)
clf_metrics.plot()
```
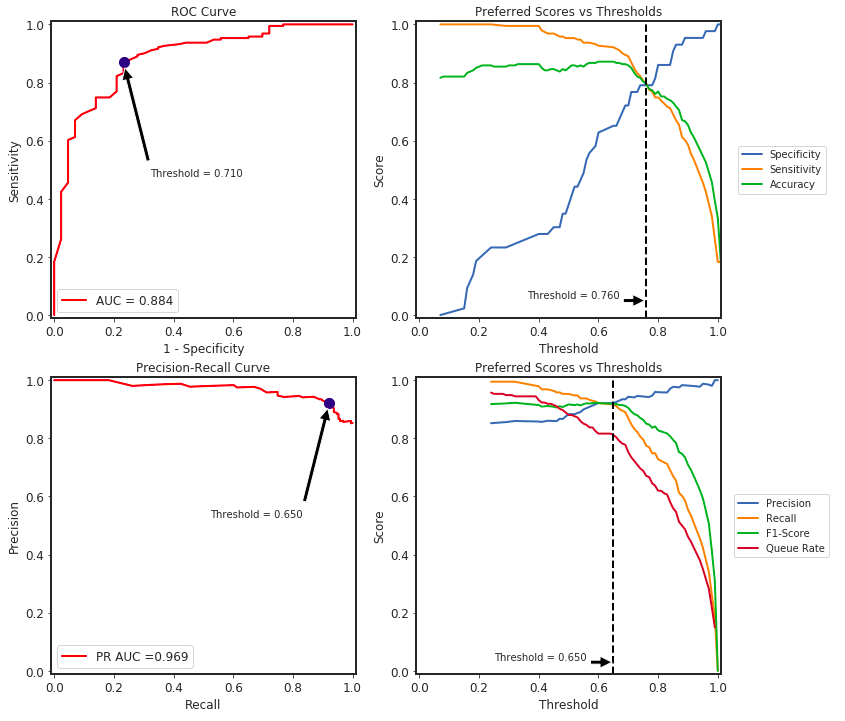
β
An example to quickly visualize some `regression metrics`:
```python
from slickml.metrics import RegressionMetrics
reg_metrics = RegressionMetrics(y_test, y_pred)
reg_metrics.plot()
```
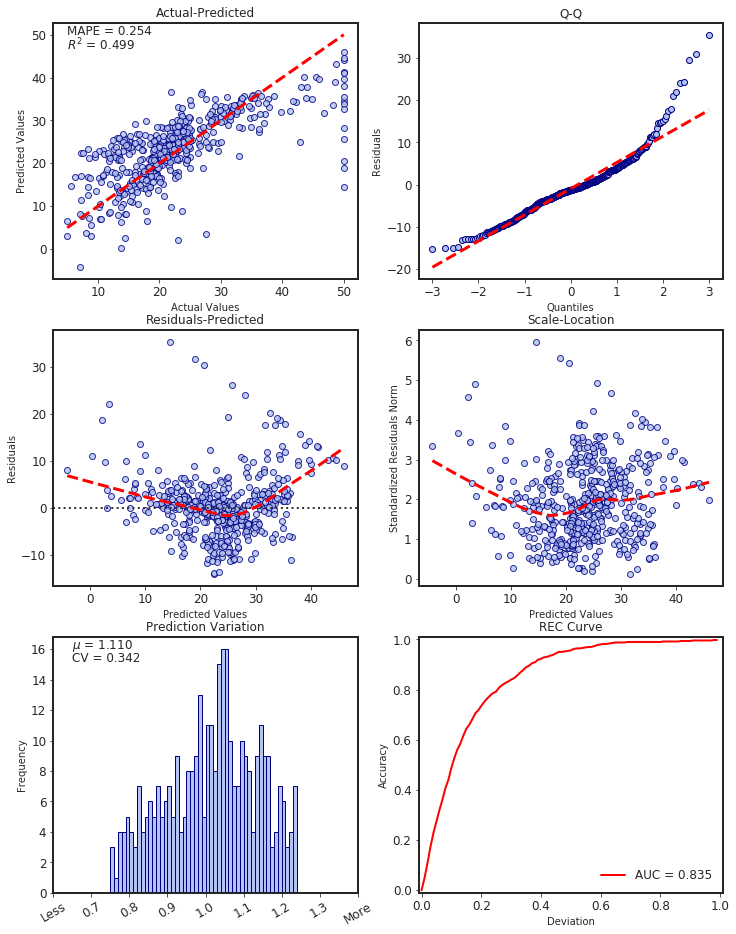
## π§βπ»π€ Contributing to SlickMLπ§
You can find the details of the development process in our [Contributing](CONTRIBUTING.md) guidelines. We strongly believe that reading and following these guidelines will help us make the contribution process easy and effective for everyone involved ππ .
Special thanks to all of our amazing contributors π
<a href="https://github.com/slickml/slick-ml/graphs/contributors">
<img src="https://contrib.rocks/image?repo=slickml/slick-ml" />
</a>

## β π π² Need Help?
Please join our [Slack Channel](https://www.slickml.com/slack-invite) to interact directly with the core team and our small community. This is a good place to discuss your questions and ideas or in general ask for help π¨βπ©βπ§ π« π¨βπ©βπ¦ .
## π Citing SlickMLπ§
If you use SlickML in an academic work π π§ͺ 𧬠, please consider citing it π .
### Bibtex Entry:
```bib
@software{slickml2020,
title={SlickML: Slick Machine Learning in Python},
author={Tahmassebi, Amirhessam and Smith, Trace},
url={https://github.com/slickml/slick-ml},
version={0.2.0},
year={2021},
}
@article{tahmassebi2021slickml,
title={Slickml: Slick machine learning in python},
author={Tahmassebi, Amirhessam and Smith, Trace},
journal={URL available at: https://github. com/slickml/slick-ml},
year={2021}
}
```
Raw data
{
"_id": null,
"home_page": "https://www.slickml.com",
"name": "slickml",
"maintainer": "Amirhessam Tahmassebi",
"docs_url": null,
"requires_python": "<3.13,>=3.9",
"maintainer_email": "admin@slickml.com",
"keywords": "python, data-science, machine-learning",
"author": "Amirhessam Tahmassebi",
"author_email": "admin@slickml.com",
"download_url": "https://files.pythonhosted.org/packages/86/3c/aa3c03d41debcd6606daca4fa087fa6411b1f20433e32f229dbc3e7019f6/slickml-0.3.1.tar.gz",
"platform": null,
"description": "<div align=\"center\">\n\n[](https://github.com/slickml/slick-ml/actions/workflows/ci.yml)\n[](https://github.com/slickml/slick-ml/actions/workflows/cd.yml)\n[](https://codecov.io/gh/slickml/slick-ml)\n[](https://pepy.tech/project/slickml)\n[](https://github.com/slickml/slick-ml/blob/master/LICENSE/)\n\n\n[](https://www.slickml.com/slack-invite)\n\n\n</div>\n\n<p align=\"center\">\n <a href=\"https://www.docs.slickml.com/\">\n <img src=\"https://raw.githubusercontent.com/slickml/slick-ml/master/assets/designs/logo_clear.png\" width=\"250\"></img>\n </a>\n</p>\n\n<div align=\"center\">\n<h1 align=\"center\">SlickML\ud83e\uddde: Slick Machine Learning in Python</h1>\n <p align=\"center\">\n <a href=\"https://github.com/slickml/slick-ml/releases\"> Explore Releases</a>\n \ud83d\udfe3 \n <a href=\"https://github.com/slickml/slick-ml/blob/master/CONTRIBUTING.md\"> Become a Contributor</a>\n \ud83d\udfe3 \n <a href=\"https://www.docs.slickml.com\"> Explore API Docs</a>\n \ud83d\udfe3 \n <a href=\"https://www.slickml.com/slack-invite\"> Join our Slack</a>\n \ud83d\udfe3 \n <a href=\"https://twitter.com/slickml\"> Tweet Us</a> \n </p>\n</div>\n\n## \ud83e\udde0 SlickML\ud83e\uddde Philosophy\n**SlickML** is an open-source machine learning library written in Python aimed at accelerating the\nexperimentation time for ML applications with tabular data while maximizing the amount of information\ncan be inferred. Data Scientists' tasks can often be repetitive such as feature selection, model\ntuning, or evaluating metrics for classification and regression problems. We strongly believe that a\ngood portion of the tasks based on tabular data can be addressed via gradient boosting and generalized\nlinear models<sup>[1](https://arxiv.org/pdf/2207.08815.pdf)</sup>. SlickML provides Data Scientists\nwith a toolbox to quickly prototype solutions for a given problem with minimal code while maximizing\nthe amount of information that can be inferred. Additionally, the prototype solutions can be easily\npromoted and served in production with our recommended recipes via various model serving frameworks\nincluding [ZenML](https://github.com/zenml-io/zenml), [BentoML](https://github.com/bentoml/BentoML),\nand [Prefect](https://github.com/PrefectHQ/prefect). More details coming soon \ud83e\udd1e ...\n\n\n## \ud83d\udcd6 Documentation\n\u2728 The API documentation is available at [docs.slickml.com](https://www.docs.slickml.com).\n\n## \ud83d\udee0 Installation\nTo begin with, install [Python version >=3.8,<3.12](https://www.python.org) and to install the library\nfrom [PyPI](https://pypi.org/project/slickml/) simply run \ud83c\udfc3\u200d\u2640\ufe0f :\n```\npip install slickml\n```\nor if you are a [python poetry](https://python-poetry.org/) user, simply run \ud83c\udfc3\u200d\u2640\ufe0f :\n```\npoetry add slickml\n```\n\n\ud83d\udce3 Please note that a working [Fortran Compiler](https://gcc.gnu.org/install/) (`gfortran`) is also required to build the package. If you do not have `gcc` installed, the following commands depending on your operating system will take care of this requirement.\n```\n# Mac Users\nbrew install gcc\n\n# Linux Users\nsudo apt install build-essential gfortran\n```\n\nThe SlickML CLI tool behaves similarly to many other CLIs for basic features. In order to find out\nwhich version of SlickML you are running, simply run \ud83c\udfc3\u200d\u2640\ufe0f :\n```\nslickml --version\n```\n\n### \ud83d\udc0d Python Virtual Environments\nIn order to avoid any potential conflicts with other installed Python packages, it is\nrecommended to use a virtual environment, e.g. [python poetry](https://python-poetry.org/), [python virtualenv](https://docs.python.org/3/library/venv.html), [pyenv virtualenv](https://github.com/pyenv/pyenv-virtualenv), or [conda environment](https://docs.conda.io/projects/conda/en/latest/user-guide/tasks/manage-environments.html). Our recommendation is to use `python-poetry` \ud83e\udd70 for everything \ud83d\ude01.\n\n\n## \ud83d\udccc Quick Start\n\u2705 An example to quickly run a `Feature Selection` pipeline with embedded `Cross-Validation` and `Feature-Importance` visualization: \n```python\nfrom slickml.feautre_selection import XGBoostFeatureSelector\nxfs = XGBoostFeatureSelector()\nxfs.fit(X, y)\n```\n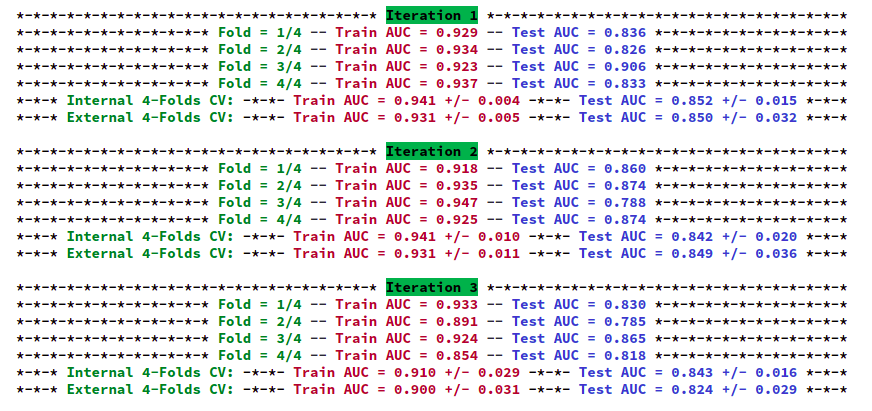\n\n```python\nxfs.plot_cv_results()\n```\n\n\n```python\nxfs.plot_frequency()\n```\n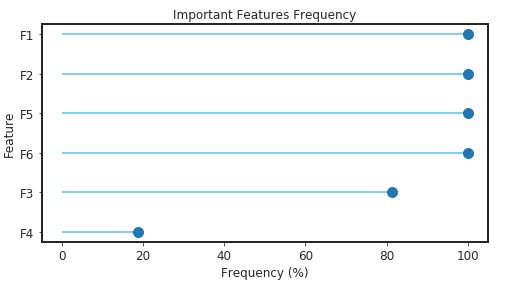\n\n\u2705 An example to quickly find the `tuned hyper-parameter` with `Bayesian Optimization`:\n```python\nfrom slickml.optimization import XGBoostBayesianOptimizer\nxbo = XGBoostBayesianOptimizer()\nxbo.fit(X_train, y_train)\n```\n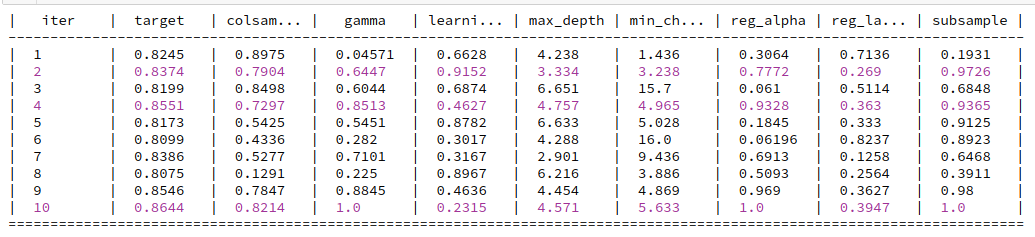\n\n```python\nbest_params = xbo.get_best_params()\nbest_params\n\n{\"colsample_bytree\": 0.8213916662259918,\n \"gamma\": 1.0,\n \"learning_rate\": 0.23148232373451072,\n \"max_depth\": 4,\n \"min_child_weight\": 5.632602921054691,\n \"reg_alpha\": 1.0,\n \"reg_lambda\": 0.39468801734425263,\n \"subsample\": 1.0\n }\n```\n\n\u2705 An example to quickly train/validate a `XGBoostCV Classifier` with `Cross-Validation`, `Feature-Importance`, and `Shap` visualizations:\n```python\nfrom slickml.classification import XGBoostCVClassifier\nclf = XGBoostCVClassifier(params=best_params)\nclf.fit(X_train, y_train)\ny_pred_proba = clf.predict_proba(X_test)\n\nclf.plot_cv_results()\n```\n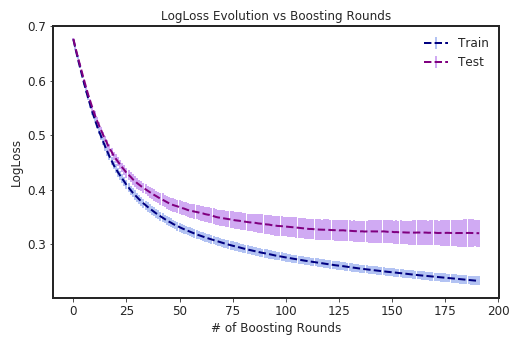\n\n```python\nclf.plot_feature_importance()\n```\n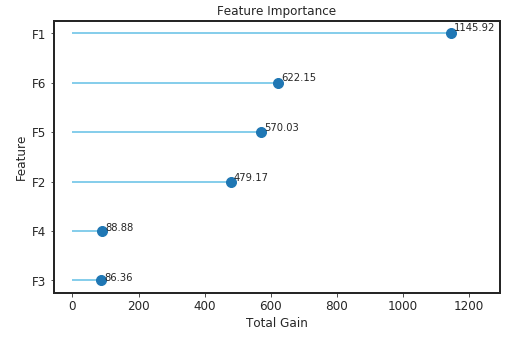\n\n```python\nclf.plot_shap_summary(plot_type=\"violin\")\n```\n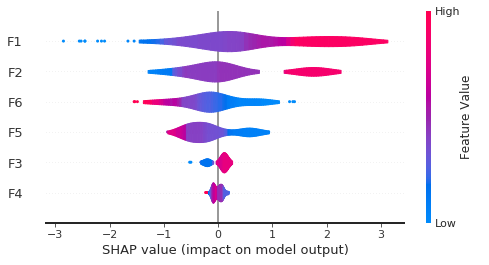\n\n```python\nclf.plot_shap_summary(plot_type=\"layered_violin\", layered_violin_max_num_bins=5)\n```\n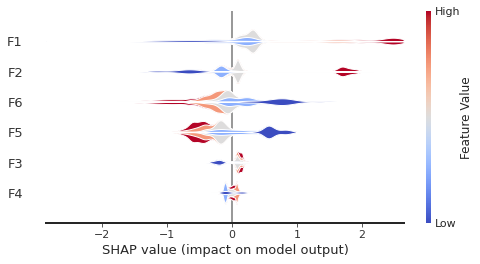\n\n```python\nclf.plot_shap_waterfall()\n```\n\n\n\n\u2705 An example to train/validate a `GLMNetCV Classifier` with `Cross-Validation` and `Coefficients` visualizations:\n```python\nfrom slickml.classification import GLMNetCVClassifier\nclf = GLMNetCVClassifier(alpha=0.3, n_splits=4, metric=\"auc\")\nclf.fit(X_train, y_train)\ny_pred_proba = clf.predict_proba(X_test)\n\nclf.plot_cv_results()\n```\n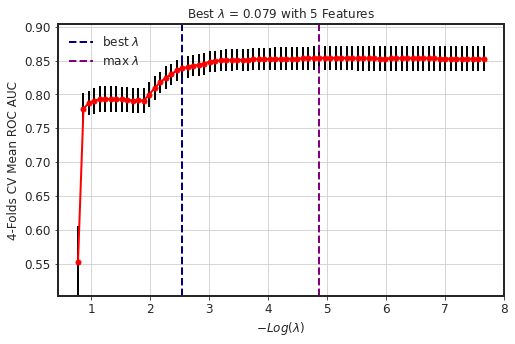\n\n```python\nclf.plot_coeff_path()\n```\n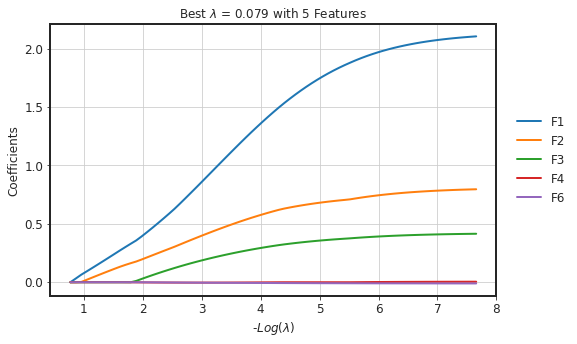\n\n\n\u2705 An example to quickly visualize the `binary classification metrics` based on multiple `thresholds`:\n```python\nfrom slickml.metrics import BinaryClassificationMetrics\nclf_metrics = BinaryClassificationMetrics(y_test, y_pred_proba)\nclf_metrics.plot()\n```\n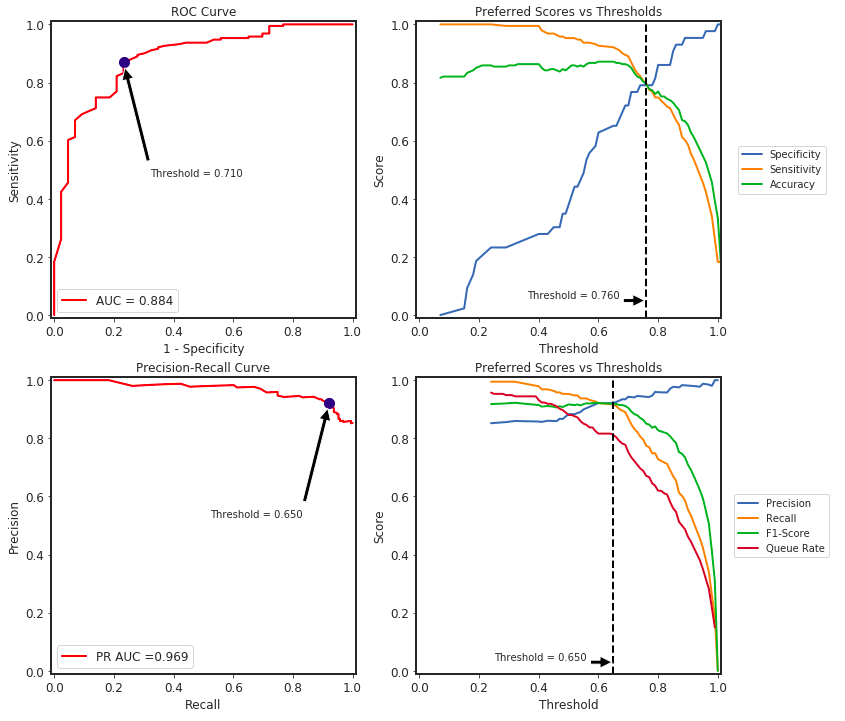\n\n\n\u2705 An example to quickly visualize some `regression metrics`:\n```python\nfrom slickml.metrics import RegressionMetrics\nreg_metrics = RegressionMetrics(y_test, y_pred)\nreg_metrics.plot()\n```\n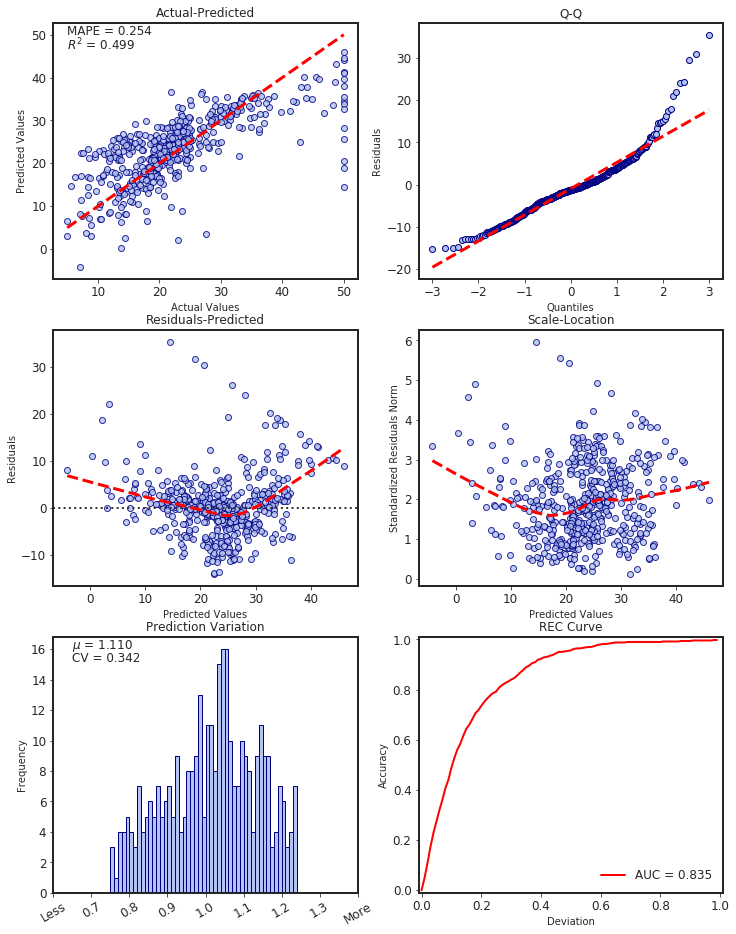\n\n\n## \ud83e\uddd1\u200d\ud83d\udcbb\ud83e\udd1d Contributing to SlickML\ud83e\uddde\nYou can find the details of the development process in our [Contributing](CONTRIBUTING.md) guidelines. We strongly believe that reading and following these guidelines will help us make the contribution process easy and effective for everyone involved \ud83d\ude80\ud83c\udf19 .\nSpecial thanks to all of our amazing contributors \ud83d\udc47\n\n<a href=\"https://github.com/slickml/slick-ml/graphs/contributors\">\n <img src=\"https://contrib.rocks/image?repo=slickml/slick-ml\" />\n</a>\n\n\n\n\n\n## \u2753 \ud83c\udd98 \ud83d\udcf2 Need Help?\nPlease join our [Slack Channel](https://www.slickml.com/slack-invite) to interact directly with the core team and our small community. This is a good place to discuss your questions and ideas or in general ask for help \ud83d\udc68\u200d\ud83d\udc69\u200d\ud83d\udc67 \ud83d\udc6b \ud83d\udc68\u200d\ud83d\udc69\u200d\ud83d\udc66 .\n\n\n## \ud83d\udcda Citing SlickML\ud83e\uddde\nIf you use SlickML in an academic work \ud83d\udcc3 \ud83e\uddea \ud83e\uddec , please consider citing it \ud83d\ude4f .\n### Bibtex Entry:\n```bib\n@software{slickml2020,\n title={SlickML: Slick Machine Learning in Python},\n author={Tahmassebi, Amirhessam and Smith, Trace},\n url={https://github.com/slickml/slick-ml},\n version={0.2.0},\n year={2021},\n}\n\n@article{tahmassebi2021slickml,\n title={Slickml: Slick machine learning in python},\n author={Tahmassebi, Amirhessam and Smith, Trace},\n journal={URL available at: https://github. com/slickml/slick-ml},\n year={2021}\n}\n```\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "SlickML: Slick Machine Learning in Python",
"version": "0.3.1",
"project_urls": {
"Documentation": "https://www.docs.slickml.com",
"Homepage": "https://www.slickml.com",
"Repository": "https://github.com/slickml/slick-ml"
},
"split_keywords": [
"python",
" data-science",
" machine-learning"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "27d48ec24a5a488d9e579f256bf8d2a5d72fd50db8ebbb35a494f403e208d202",
"md5": "a8e39ab6ddecc87b40ee34995278233b",
"sha256": "27f238f6824739608b375ca5224629699ff6689f3407cb0c04cfa62441921247"
},
"downloads": -1,
"filename": "slickml-0.3.1-py3-none-any.whl",
"has_sig": false,
"md5_digest": "a8e39ab6ddecc87b40ee34995278233b",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": "<3.13,>=3.9",
"size": 116565,
"upload_time": "2024-07-26T00:53:31",
"upload_time_iso_8601": "2024-07-26T00:53:31.521493Z",
"url": "https://files.pythonhosted.org/packages/27/d4/8ec24a5a488d9e579f256bf8d2a5d72fd50db8ebbb35a494f403e208d202/slickml-0.3.1-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "863caa3c03d41debcd6606daca4fa087fa6411b1f20433e32f229dbc3e7019f6",
"md5": "2b9c2bec8ca9e725bb9f8fcd76558f66",
"sha256": "dff89e8fb86df902dae572d833f93ccef1224dde376037a36f2fe3eac21792dd"
},
"downloads": -1,
"filename": "slickml-0.3.1.tar.gz",
"has_sig": false,
"md5_digest": "2b9c2bec8ca9e725bb9f8fcd76558f66",
"packagetype": "sdist",
"python_version": "source",
"requires_python": "<3.13,>=3.9",
"size": 86711,
"upload_time": "2024-07-26T00:53:33",
"upload_time_iso_8601": "2024-07-26T00:53:33.194628Z",
"url": "https://files.pythonhosted.org/packages/86/3c/aa3c03d41debcd6606daca4fa087fa6411b1f20433e32f229dbc3e7019f6/slickml-0.3.1.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-07-26 00:53:33",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "slickml",
"github_project": "slick-ml",
"travis_ci": false,
"coveralls": true,
"github_actions": true,
"tox": true,
"lcname": "slickml"
}