Name | st-login JSON |
Version |
0.0.2
JSON |
| download |
home_page | https://github.com/lijiacaigit/st_login |
Summary | The st_login library is meant for streamlit application developers. It lets you connect your streamlit application to a pre-built and secure Login/ Sign-Up page |
upload_time | 2023-07-18 09:00:25 |
maintainer | |
docs_url | None |
author | Lijiacai |
requires_python | >=3.7.1 |
license | |
keywords |
|
VCS |
 |
bugtrack_url |
|
requirements |
No requirements were recorded.
|
Travis-CI |
No Travis.
|
coveralls test coverage |
No coveralls.
|
HOW TO INSTALL ALL LIBRARIES:
python3.10 -m venv venv
source venv/bin/activate
python3.10 -m pip install -r requirement.txt
# Streamlit Login/ Sign Up Library [](https://pepy.tech/project/streamlit-login-auth-ui)
The streamlit_login_auth_ui library is meant for streamlit application developers.
It lets you connect your streamlit application to a pre-built and secure Login/ Sign-Up page.
You can customize specific parts of the page without any hassle!
The library grants users an option to reset their password, users can click on ```Forgot Password?``` after which an Email is triggered containing a temporary, randomly generated password.
The library also sets encrypted cookies to remember and automatically authenticate the users without password. \
The users can logout using the ```Logout``` button.
## Authors
- [@lijiacai](https://github.com/lijiacaigit/)
## PyPi
https://pypi.org/project/streamlit-login-auth-ui/
## The UI:
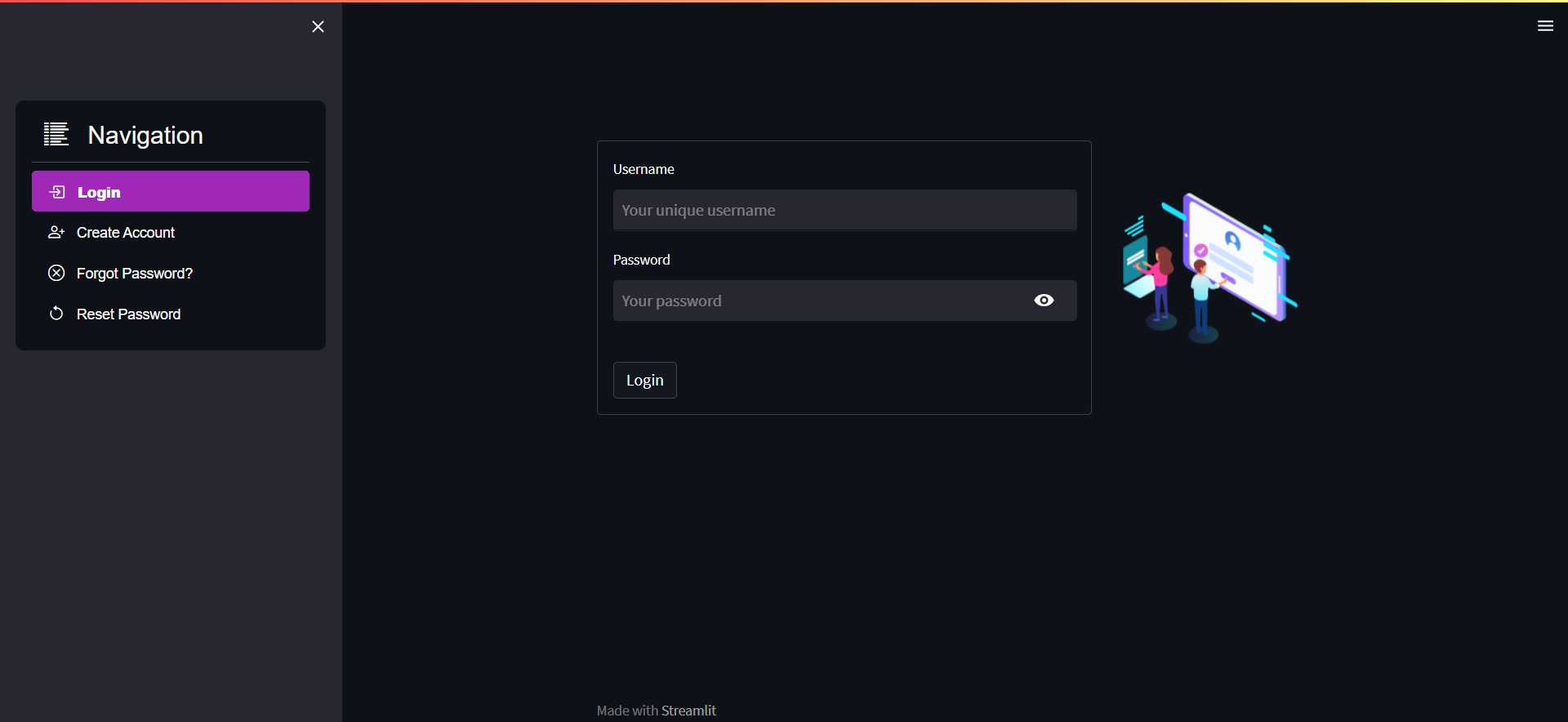
## Installation
```python
pip install st_login
```
## How to implement the library?
To import the library, just paste this at the starting of the code:
```python
from st_login import Login
```
All you need to do is create an object for the ```Login``` class and pass the following parameters:
1. auth_token : The unique authorization token received from - https://www.courier.com/email-api/
2. company_name : This is the name of the person/ organization which will send the password reset email.
3. width : Width of the animation on the login page.
4. height : Height of the animation on the login page.
5. logout_button_name : The logout button name.
6. hide_menu_bool : Pass True if the streamlit menu should be hidden.
7. hide_footer_bool : Pass True if the 'made with streamlit' footer should be hidden.
8. lottie_url : The lottie animation you would like to use on the login page. Explore animations at - https://lottiefiles.com/featured
#### Mandatory Arguments:
* ```auth_token```
* ```company_name```
* ```width```
* ```height```
#### Non Mandatory Arguments:
* ```logout_button_name``` [default = 'Logout']
* ```hide_menu_bool``` [default = False]
* ```hide_footer_bool``` [default = False]
* ```lottie_url``` [default = https://assets8.lottiefiles.com/packages/lf20_ktwnwv5m.json]
After doing that, just call the ```build_login_ui()``` function using the object you just created and store the return value in a variable.
# Example:
```python
import streamlit as st
from streamlit_option_menu import option_menu
from st_login import Login
class CustomLogin(Login):
def nav_sidebar(self):
"""
Creates the side navigaton bar
"""
main_page_sidebar = st.sidebar.empty()
with main_page_sidebar:
selected_option = option_menu(
menu_title='User Info',
menu_icon='list-columns-reverse',
icons=['box-arrow-in-right', 'person-plus', 'x-circle', 'arrow-counterclockwise'],
options=['Login', 'Reset Password'],
styles={
"container": {"padding": "5px"},
"nav-link": {"font-size": "14px", "text-align": "left", "margin": "0px"}})
return main_page_sidebar, selected_option
is_login = CustomLogin(auth_token="courier_auth_token",
company_name="Shims",
width=200, height=250,
logout_button_name='Logout', hide_menu_bool=True,
hide_footer_bool=False,
lottie_url='https://assets2.lottiefiles.com/packages/lf20_jcikwtux.json').build_login_ui()
if is_login is True:
st.markdown("Your Streamlit Application Begins here!")
```
That's it! The library handles the rest. \
Just make sure you call/ build your application indented under ```if st.session_state['LOGGED_IN'] == True:```, this guarantees that your application runs only after the user is securely logged in.
## Explanation
### Login page
The login page, authenticates the user.
### Create Account page
Stores the user info in a secure way in the ```_secret_auth_.json``` file. \
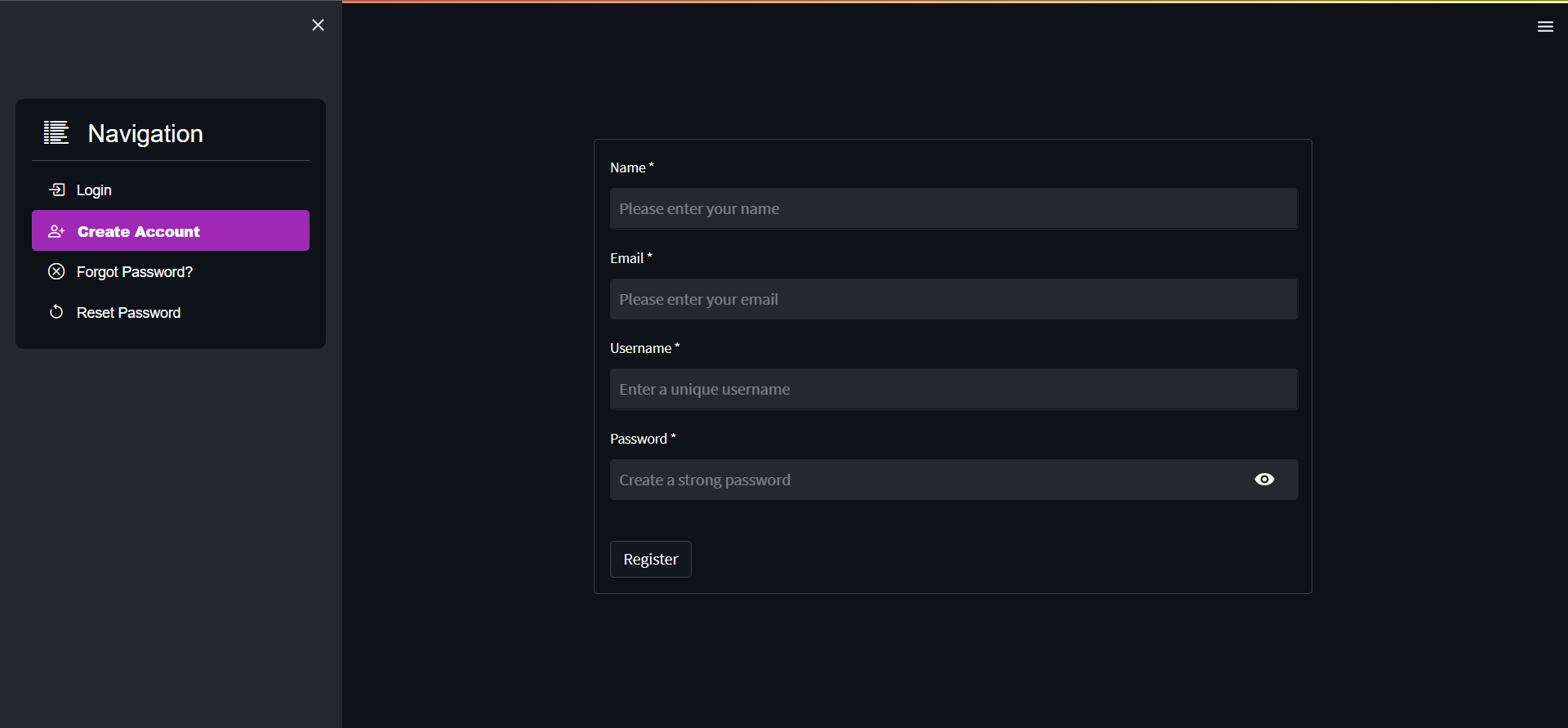
### Forgot Password page
After user authentication (email), triggers an email to the user containing a random password. \
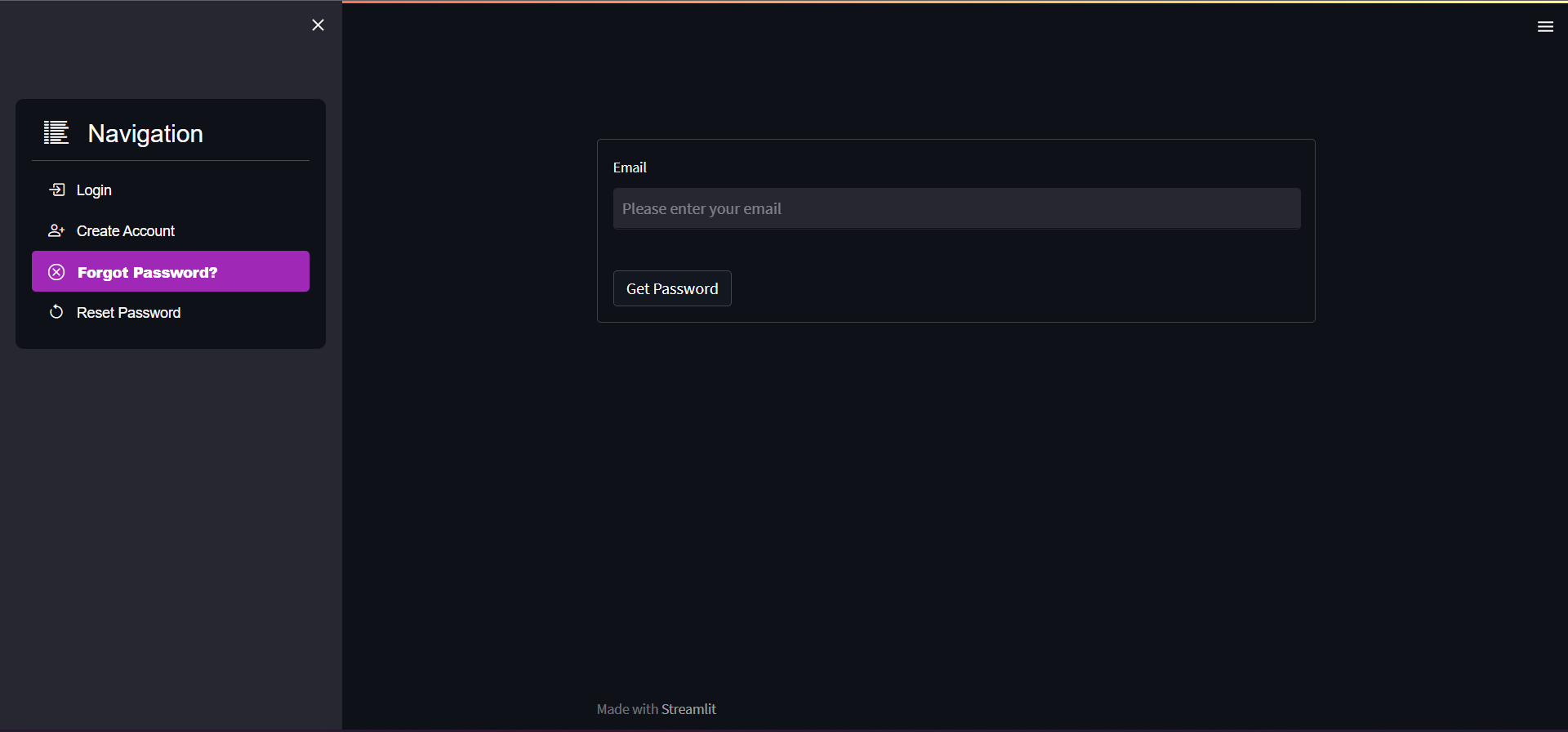
### Reset Password page
After user authentication (email and the password shared over email), resets the password and updates the same \
in the ```_secret_auth_.json``` file. \
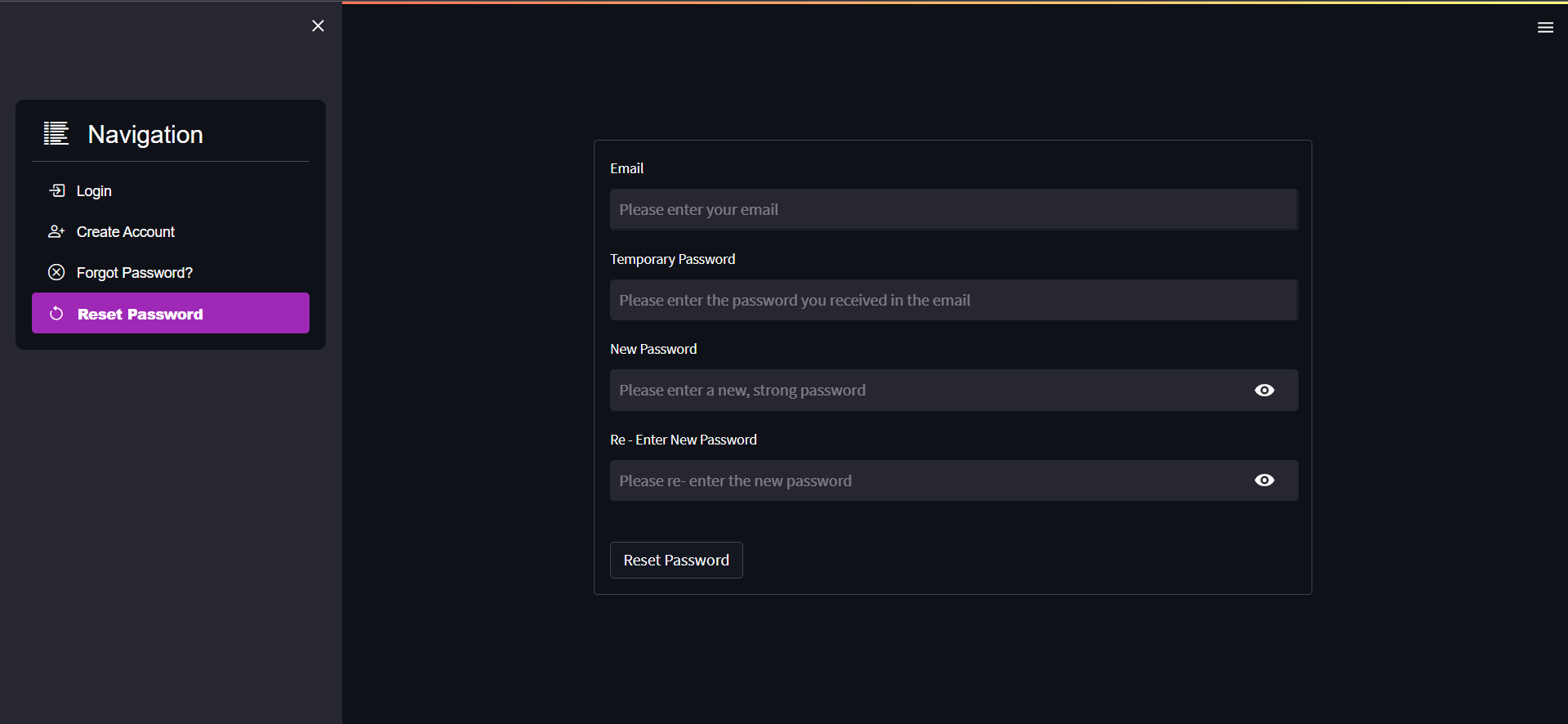
### Logout button
Generated in the sidebar only if the user is logged in, allows users to logout. \
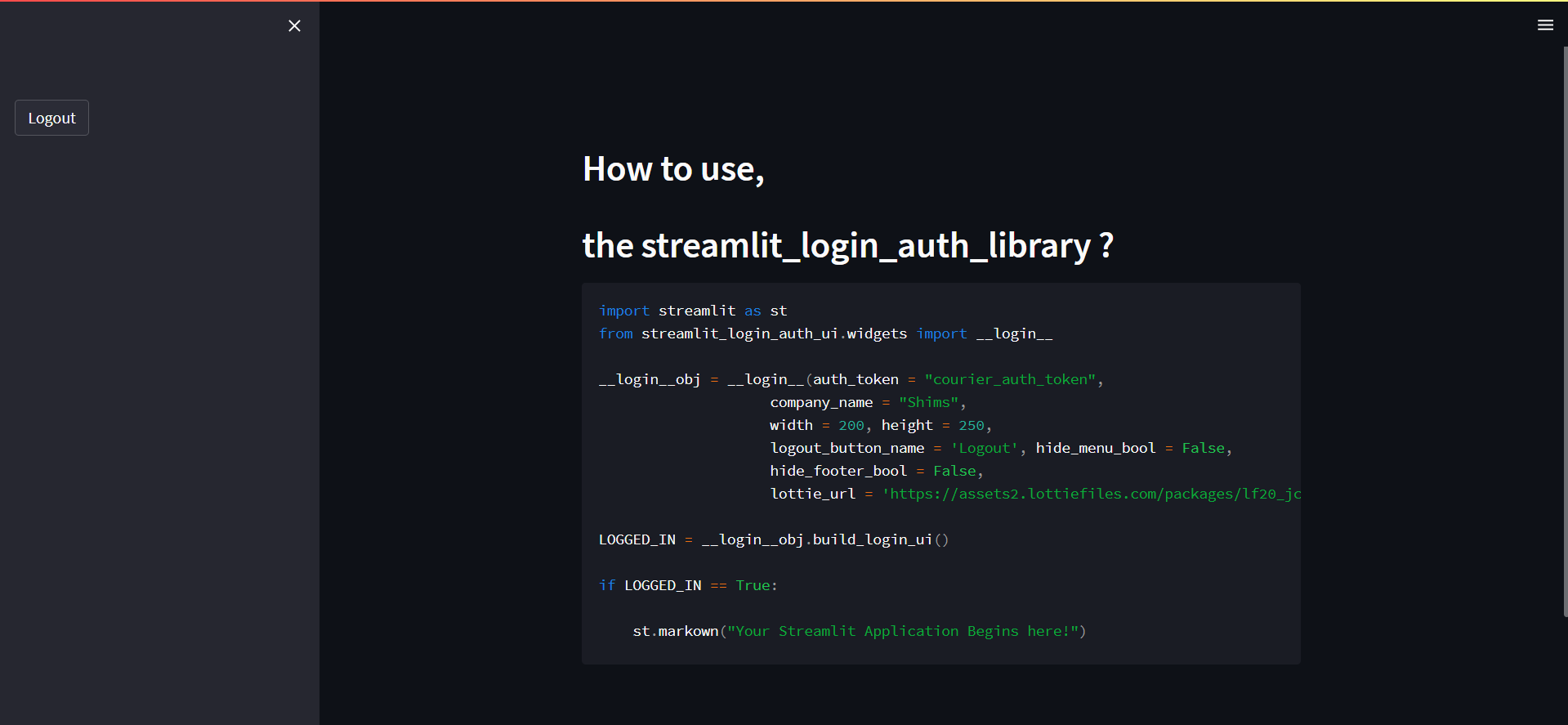
__Cookies are automatically created and destroyed depending on the user authentication status.__
## Version
v0.0.0
Raw data
{
"_id": null,
"home_page": "https://github.com/lijiacaigit/st_login",
"name": "st-login",
"maintainer": "",
"docs_url": null,
"requires_python": ">=3.7.1",
"maintainer_email": "",
"keywords": "",
"author": "Lijiacai",
"author_email": "1050518702@qq.com",
"download_url": "https://files.pythonhosted.org/packages/7c/27/f4c87cdcbd8b8741f4e8e0abc40235ce87cbe7901caddf6f333a0e0b96d3/st_login-0.0.2.tar.gz",
"platform": null,
"description": "\r\nHOW TO INSTALL ALL LIBRARIES:\r\npython3.10 -m venv venv\r\nsource venv/bin/activate\r\npython3.10 -m pip install -r requirement.txt\r\n\r\n# Streamlit Login/ Sign Up Library [](https://pepy.tech/project/streamlit-login-auth-ui)\r\n\r\nThe streamlit_login_auth_ui library is meant for streamlit application developers.\r\nIt lets you connect your streamlit application to a pre-built and secure Login/ Sign-Up page.\r\n\r\nYou can customize specific parts of the page without any hassle!\r\n\r\nThe library grants users an option to reset their password, users can click on ```Forgot Password?``` after which an Email is triggered containing a temporary, randomly generated password.\r\n\r\nThe library also sets encrypted cookies to remember and automatically authenticate the users without password. \\\r\nThe users can logout using the ```Logout``` button.\r\n\r\n\r\n## Authors\r\n- [@lijiacai](https://github.com/lijiacaigit/)\r\n\r\n## PyPi\r\nhttps://pypi.org/project/streamlit-login-auth-ui/\r\n\r\n## The UI:\r\n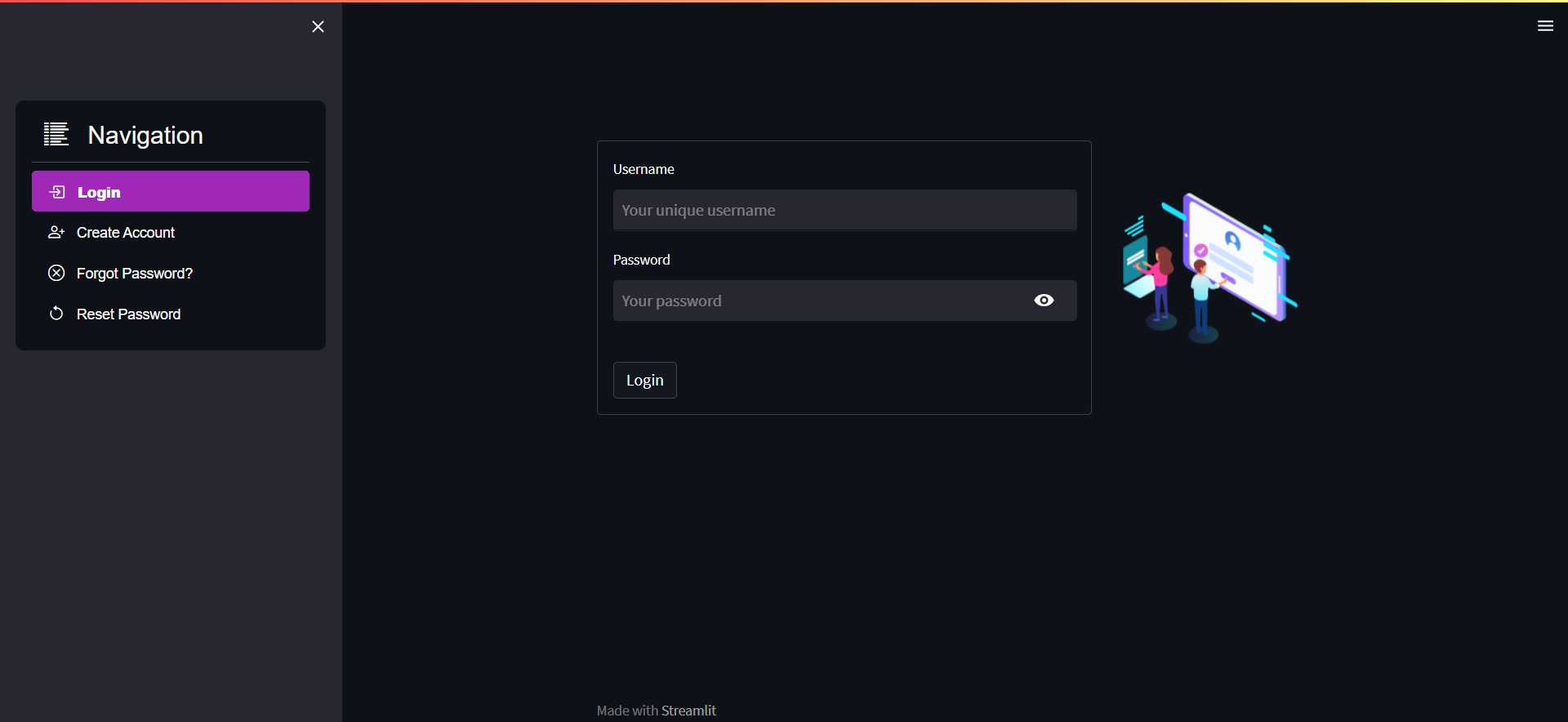\r\n \r\n## Installation\r\n\r\n```python\r\npip install st_login\r\n```\r\n\r\n## How to implement the library?\r\n\r\nTo import the library, just paste this at the starting of the code:\r\n```python\r\nfrom st_login import Login\r\n```\r\n\r\nAll you need to do is create an object for the ```Login``` class and pass the following parameters:\r\n1. auth_token : The unique authorization token received from - https://www.courier.com/email-api/\r\n2. company_name : This is the name of the person/ organization which will send the password reset email.\r\n3. width : Width of the animation on the login page.\r\n4. height : Height of the animation on the login page.\r\n5. logout_button_name : The logout button name.\r\n6. hide_menu_bool : Pass True if the streamlit menu should be hidden.\r\n7. hide_footer_bool : Pass True if the 'made with streamlit' footer should be hidden.\r\n8. lottie_url : The lottie animation you would like to use on the login page. Explore animations at - https://lottiefiles.com/featured\r\n\r\n#### Mandatory Arguments:\r\n* ```auth_token```\r\n* ```company_name```\r\n* ```width```\r\n* ```height```\r\n\r\n#### Non Mandatory Arguments:\r\n* ```logout_button_name``` [default = 'Logout']\r\n* ```hide_menu_bool``` [default = False]\r\n* ```hide_footer_bool``` [default = False]\r\n* ```lottie_url``` [default = https://assets8.lottiefiles.com/packages/lf20_ktwnwv5m.json]\r\n\r\nAfter doing that, just call the ```build_login_ui()``` function using the object you just created and store the return value in a variable.\r\n\r\n# Example:\r\n```python\r\nimport streamlit as st\r\nfrom streamlit_option_menu import option_menu\r\nfrom st_login import Login\r\n\r\n\r\nclass CustomLogin(Login):\r\n def nav_sidebar(self):\r\n \"\"\"\r\n Creates the side navigaton bar\r\n \"\"\"\r\n main_page_sidebar = st.sidebar.empty()\r\n with main_page_sidebar:\r\n selected_option = option_menu(\r\n menu_title='User Info',\r\n menu_icon='list-columns-reverse',\r\n icons=['box-arrow-in-right', 'person-plus', 'x-circle', 'arrow-counterclockwise'],\r\n options=['Login', 'Reset Password'],\r\n styles={\r\n \"container\": {\"padding\": \"5px\"},\r\n \"nav-link\": {\"font-size\": \"14px\", \"text-align\": \"left\", \"margin\": \"0px\"}})\r\n return main_page_sidebar, selected_option\r\n\r\n\r\nis_login = CustomLogin(auth_token=\"courier_auth_token\",\r\n company_name=\"Shims\",\r\n width=200, height=250,\r\n logout_button_name='Logout', hide_menu_bool=True,\r\n hide_footer_bool=False,\r\n lottie_url='https://assets2.lottiefiles.com/packages/lf20_jcikwtux.json').build_login_ui()\r\n\r\nif is_login is True:\r\n st.markdown(\"Your Streamlit Application Begins here!\")\r\n```\r\n\r\nThat's it! The library handles the rest. \\\r\nJust make sure you call/ build your application indented under ```if st.session_state['LOGGED_IN'] == True:```, this guarantees that your application runs only after the user is securely logged in. \r\n\r\n## Explanation\r\n### Login page\r\nThe login page, authenticates the user.\r\n\r\n### Create Account page\r\nStores the user info in a secure way in the ```_secret_auth_.json``` file. \\\r\n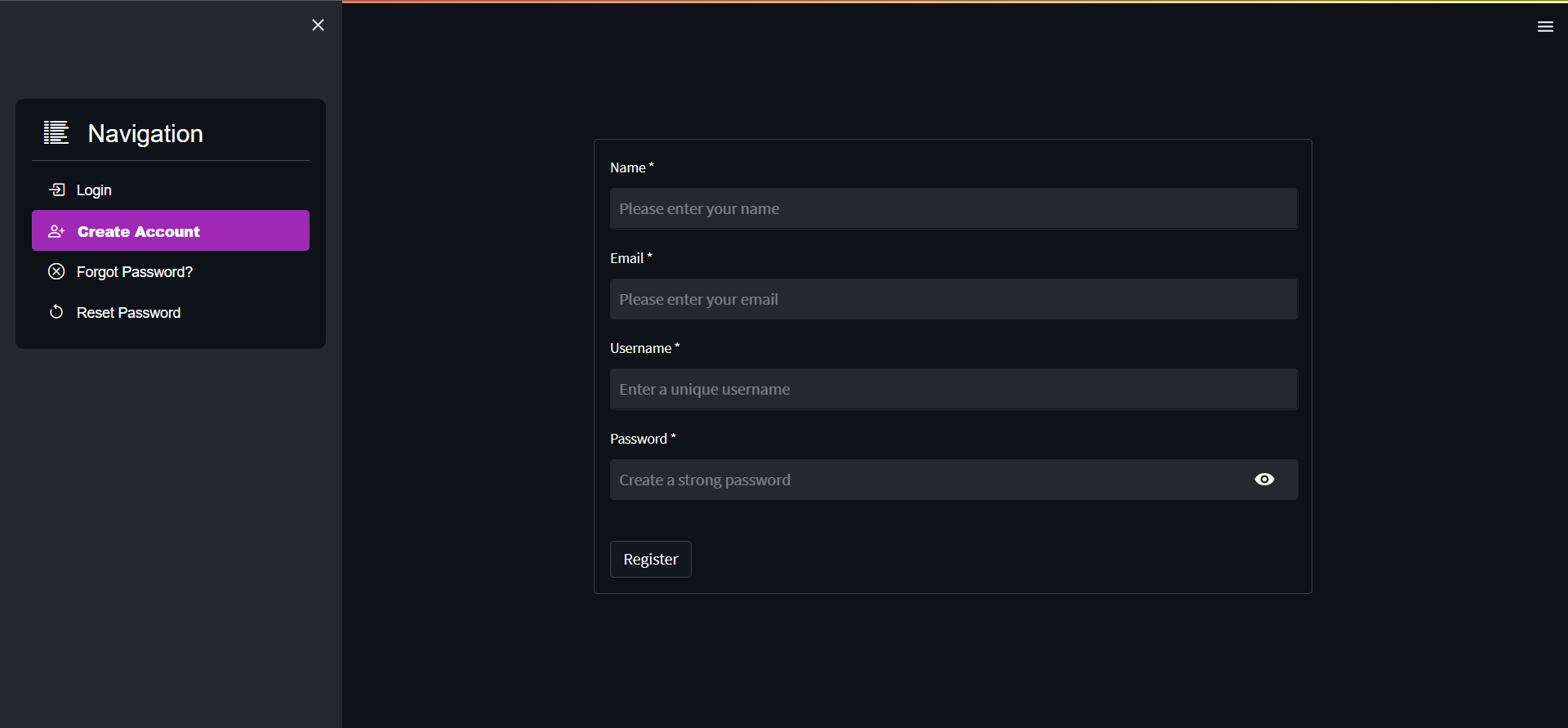\r\n\r\n### Forgot Password page\r\nAfter user authentication (email), triggers an email to the user containing a random password. \\\r\n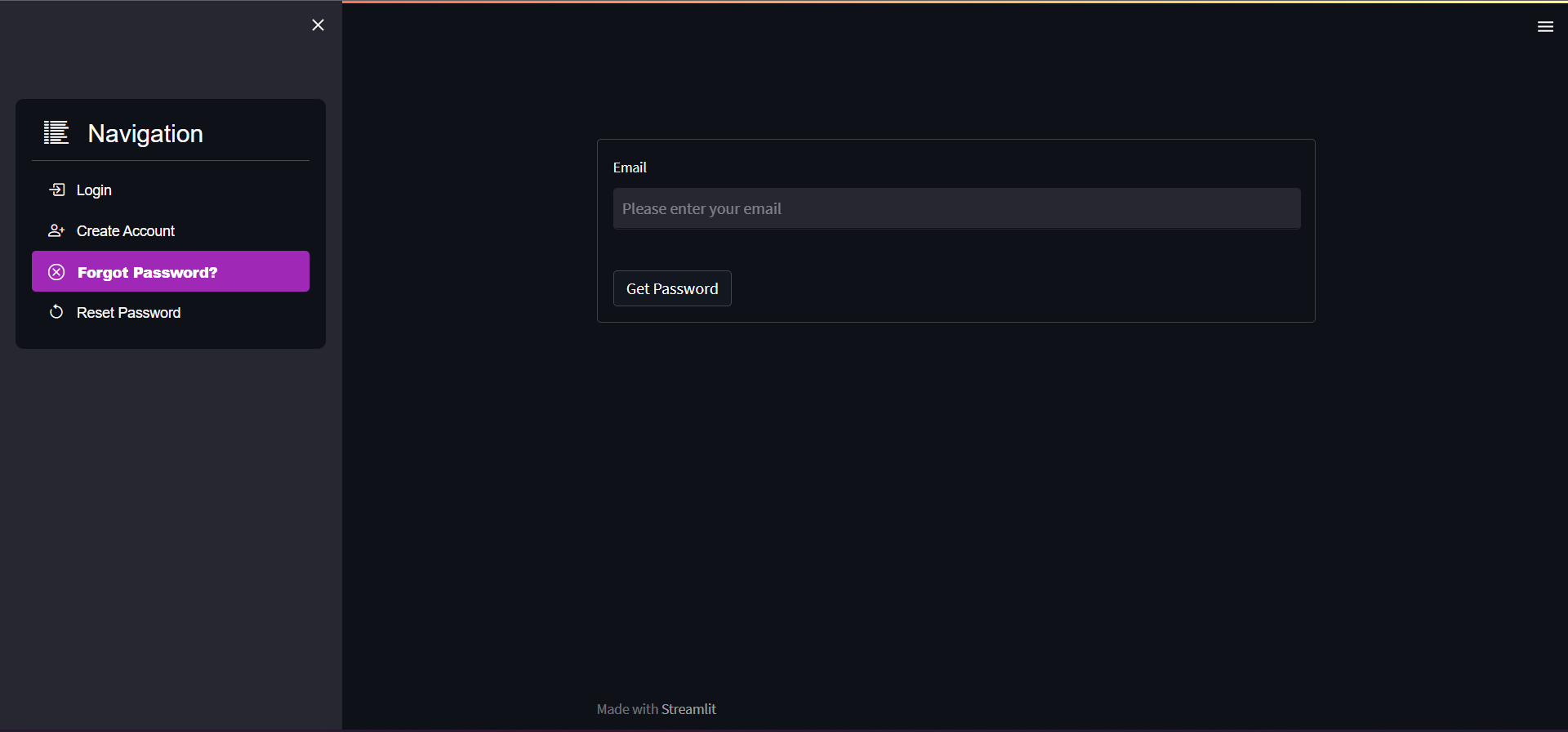\r\n\r\n### Reset Password page\r\nAfter user authentication (email and the password shared over email), resets the password and updates the same \\\r\nin the ```_secret_auth_.json``` file. \\\r\n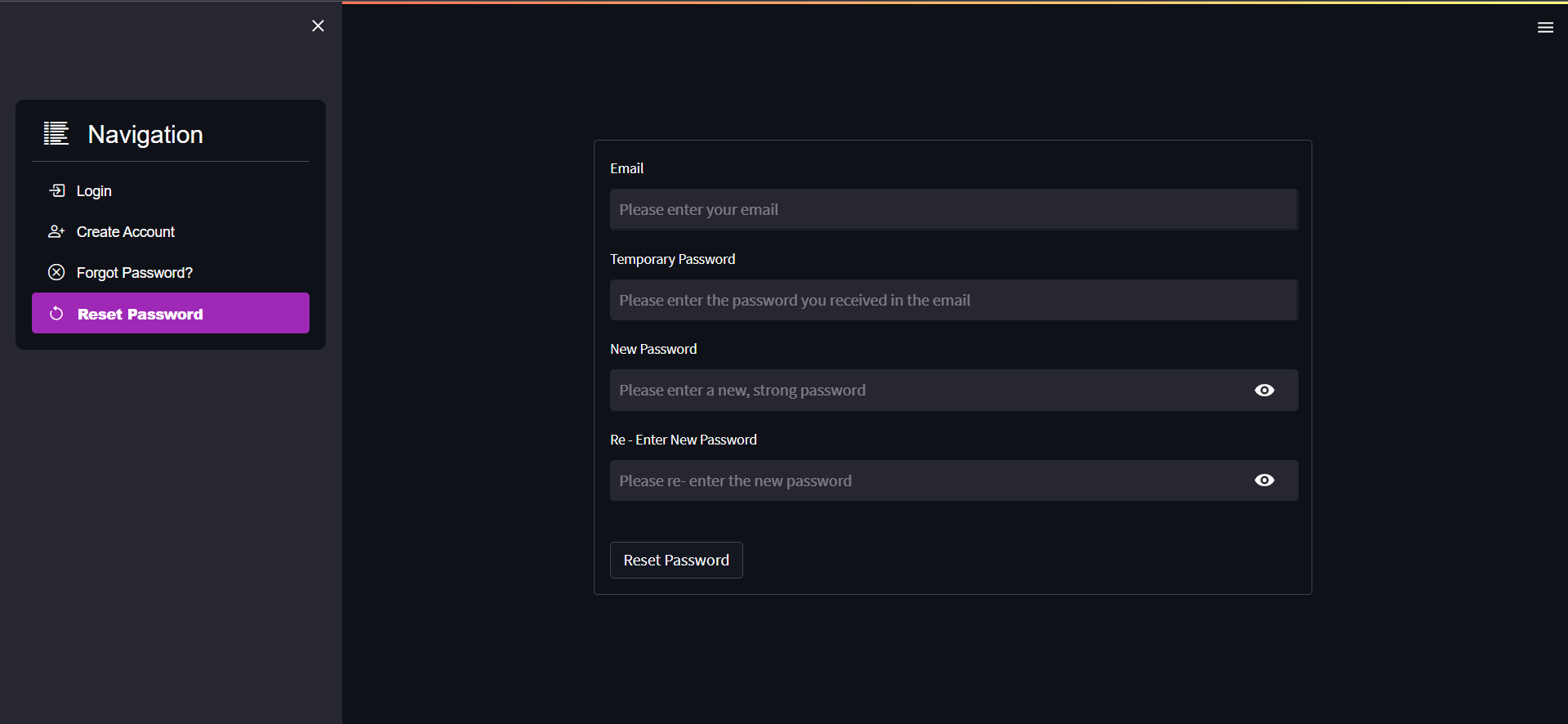\r\n\r\n### Logout button\r\nGenerated in the sidebar only if the user is logged in, allows users to logout. \\\r\n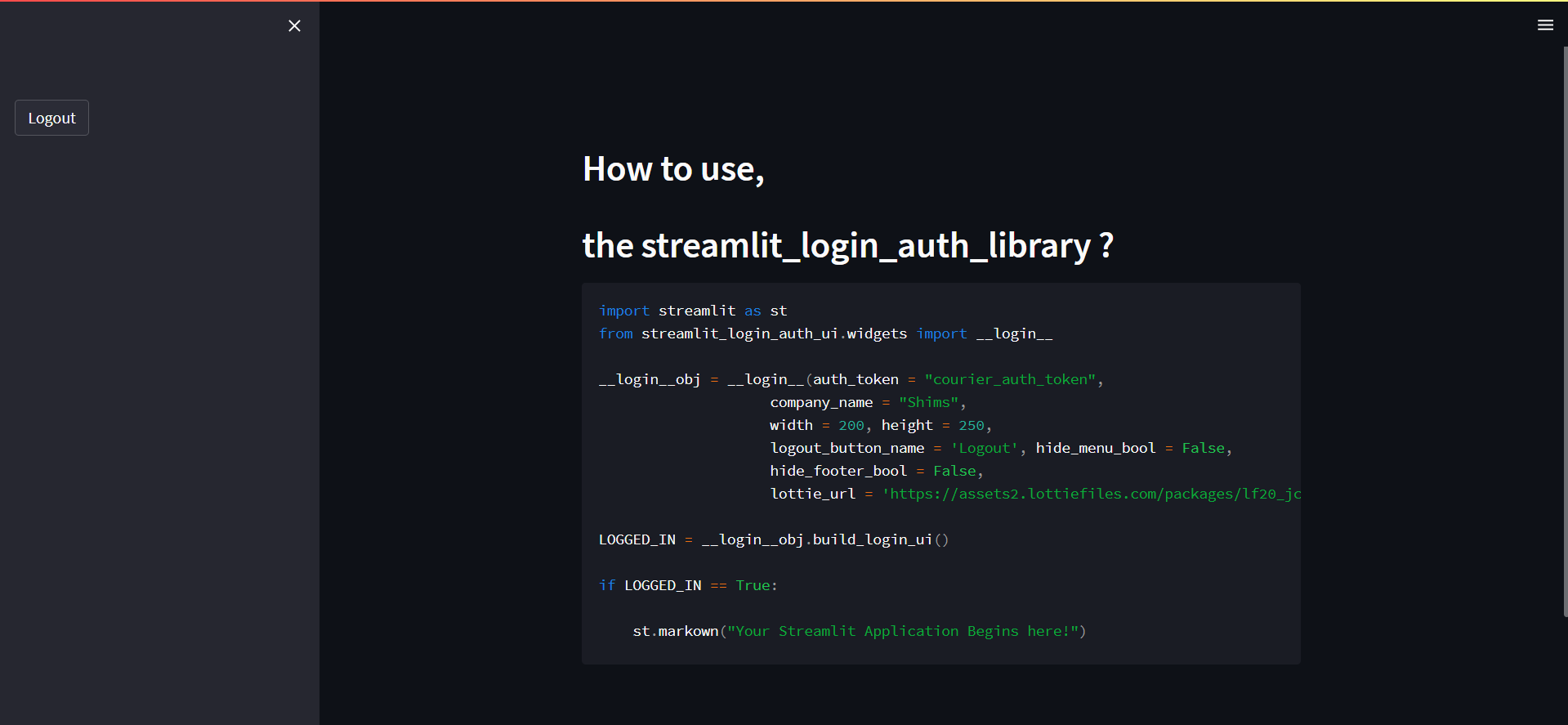\r\n\r\n__Cookies are automatically created and destroyed depending on the user authentication status.__\r\n\r\n## Version\r\nv0.0.0\r\n\r\n\r\n\r\n\r\n\r\n\r\n\r\n\r\n\r\n",
"bugtrack_url": null,
"license": "",
"summary": "The st_login library is meant for streamlit application developers. It lets you connect your streamlit application to a pre-built and secure Login/ Sign-Up page",
"version": "0.0.2",
"project_urls": {
"Homepage": "https://github.com/lijiacaigit/st_login"
},
"split_keywords": [],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "7c27f4c87cdcbd8b8741f4e8e0abc40235ce87cbe7901caddf6f333a0e0b96d3",
"md5": "e137d163b27da09be807d88cace021da",
"sha256": "b577a5be894cd07565b2917078508a205dc03652b84ce2c15c6c5377f9d68309"
},
"downloads": -1,
"filename": "st_login-0.0.2.tar.gz",
"has_sig": false,
"md5_digest": "e137d163b27da09be807d88cace021da",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.7.1",
"size": 10337,
"upload_time": "2023-07-18T09:00:25",
"upload_time_iso_8601": "2023-07-18T09:00:25.663574Z",
"url": "https://files.pythonhosted.org/packages/7c/27/f4c87cdcbd8b8741f4e8e0abc40235ce87cbe7901caddf6f333a0e0b96d3/st_login-0.0.2.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-07-18 09:00:25",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "lijiacaigit",
"github_project": "st_login",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"requirements": [],
"lcname": "st-login"
}