# Change Theme (on the Client Side!)
## Code example
```python
import streamlit as st
from streamlit_plugins.components.theme_changer import st_theme_changer
st.title("Theme Changer Component")
st.caption("Just push the button to change the theme! On the client side, of course.")
# specify the primary menu definition
st_theme_changer()
st_theme_changer(render_mode="pills")
```
## Custom Config
The theme name can’t be "Dark" or "Light" since those are reserved for the default Streamlit themes.
### Editable custom theme app
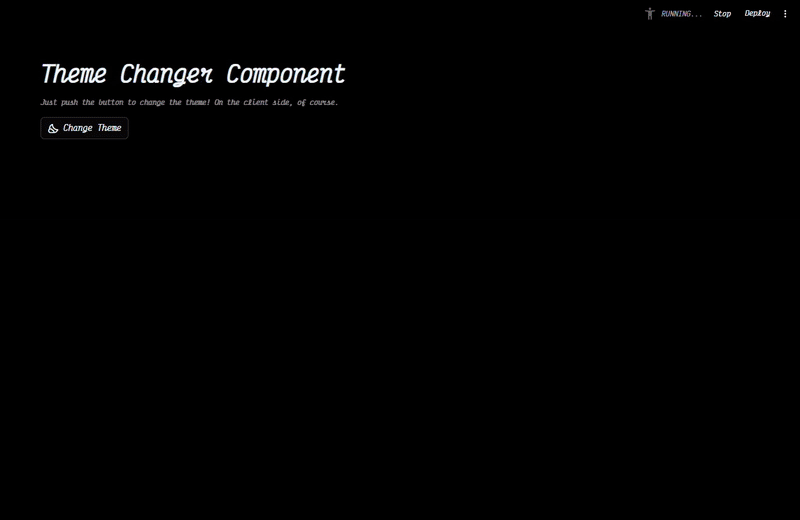
```python
import streamlit as st
from streamlit_plugins.components.theme_changer import get_active_theme_key, st_theme_changer
from streamlit_plugins.components.theme_changer.entity import ThemeInfo, ThemeInput, ThemeBaseLight, ThemeBaseDark
# make it look nice from the start
st.set_page_config(layout='wide', initial_sidebar_state='collapsed')
st.title("Theme Changer Component")
st.caption("Just push the button to change the theme! On the client side, of course.")
init_theme_data = dict(
soft_light=ThemeInput(
name="Soft Sunrise",
icon=":material/sunny_snowing:",
order=0,
themeInfo=ThemeInfo(
base=ThemeBaseLight.base,
primaryColor="#6100FF",
backgroundColor="#EFF4FF",
secondaryBackgroundColor="#E7EEF5",
textColor="#000000",
widgetBackgroundColor="#F3F5F7",
widgetBorderColor="#9000FF",
skeletonBackgroundColor="#E0E0E0",
bodyFont=ThemeBaseLight.bodyFont,
codeFont=ThemeBaseLight.codeFont,
fontFaces=ThemeBaseLight.fontFaces,
)
),
soft_dark=ThemeInput(
name="Dark Midnight",
icon=":material/nights_stay:",
order=1,
themeInfo=ThemeInfo(
base=ThemeBaseDark.base,
primaryColor="#7AF8FF",
backgroundColor="#000000",
secondaryBackgroundColor="#045367",
textColor="#f0f8ff",
widgetBackgroundColor="#092927",
widgetBorderColor="#75D9FF",
skeletonBackgroundColor="#365252",
bodyFont=ThemeBaseDark.bodyFont,
codeFont=ThemeBaseDark.codeFont,
fontFaces=ThemeBaseDark.fontFaces,
)
),
sepia=ThemeInput(
name="Sepia",
icon=":material/gradient:",
order=2,
themeInfo=ThemeInfo(
base=ThemeBaseLight.base,
primaryColor="#FF0004",
backgroundColor="#F9F9E0",
secondaryBackgroundColor="#EFEFB4",
textColor="#000000",
widgetBackgroundColor="#F5F5D7",
widgetBorderColor="#E2DEAD",
skeletonBackgroundColor="#F5F5DC",
bodyFont=ThemeBaseLight.bodyFont,
codeFont=ThemeBaseLight.codeFont,
fontFaces=ThemeBaseLight.fontFaces,
)
)
)
if st.session_state.get("theme_data") is None:
st.session_state["theme_data"] = init_theme_data
theme_data = st.session_state["theme_data"]
st_theme_changer(themes_data=theme_data, render_mode="init", default_init_theme_name="soft_dark")
st_theme_changer(themes_data=theme_data, rerun_whole_st=True)
with st.expander("Theme Editor", expanded=False):
with st.container(border=False):
theme_keys = list(theme_data.keys())
tabs = st.tabs(theme_keys)
for i, tab in enumerate(tabs):
theme_key = theme_keys[i]
with tab:
with st.form(key=f"{theme_key}_form", border=False):
col1, col2, col3 = st.columns(3)
name = col1.text_input("Theme Label", value=theme_data[theme_key].name, key=f"{theme_key}_text_input")
icon = col2.text_input("Theme Icon", value=theme_data[theme_key].icon, key=f"{theme_key}_icon_input")
order = col3.number_input("Order", value=theme_data[theme_key].order, key=f"{theme_key}_order_input")
col1, col2, col3 = st.columns(3)
primaryColor = col1.color_picker("Primary Color", value=theme_data[theme_key].themeInfo.primaryColor, key=f"{theme_key}_primary_color_input")
textColor = col2.color_picker("Text Color", value=theme_data[theme_key].themeInfo.textColor, key=f"{theme_key}_text_color_input")
col1, col2, col3 = st.columns(3)
backgroundColor = col1.color_picker("Background Color", value=theme_data[theme_key].themeInfo.backgroundColor, key=f"{theme_key}_background_color_input")
secondaryBackgroundColor = col2.color_picker("Secondary Background Color", value=theme_data[theme_key].themeInfo.secondaryBackgroundColor, key=f"{theme_key}_secondary_background_color_input")
skeletonBackgroundColor = col3.color_picker("Skeleton Background Color", value=theme_data[theme_key].themeInfo.skeletonBackgroundColor, key=f"{theme_key}_skeleton_background_color_input")
col1, col2, col3 = st.columns(3)
widgetBackgroundColor = col1.color_picker("Widget Background Color", value=theme_data[theme_key].themeInfo.widgetBackgroundColor, key=f"{theme_key}_widget_background_color_input")
widgetBorderColor = col2.color_picker("Widget Border Color", value=theme_data[theme_key].themeInfo.widgetBorderColor, key=f"{theme_key}_widget_border_color_input")
baseWidgetRadius = col3.number_input("Base Widget Radius", value=theme_data[theme_key].themeInfo.radii["baseWidgetRadius"], key=f"{theme_key}_base_widget_radius_input")
col1, col2, col3 = st.columns(3)
checkbox_radius = col1.number_input("Checkbox Radius", value=theme_data[theme_key].themeInfo.radii["checkboxRadius"], key=f"{theme_key}_checkbox_radius_input")
col2.checkbox("Example Checkbox", key=f"{theme_key}_example_checkbox")
col1, col2, col3 = st.columns(3)
tiny_font_size = col1.number_input("Tiny Font Size", value=theme_data[theme_key].themeInfo.fontSizes["tinyFontSize"], key=f"{theme_key}_tiny_font_size_input")
small_font_size = col2.number_input("Small Font Size", value=theme_data[theme_key].themeInfo.fontSizes["smallFontSize"], key=f"{theme_key}_small_font_size_input")
base_font_size = col3.number_input("Base Font Size", value=theme_data[theme_key].themeInfo.fontSizes["baseFontSize"], key=f"{theme_key}_base_font_size_input")
col1, col2 = st.columns(2)
bodyFont = col1.text_input("Body Font", value=theme_data[theme_key].themeInfo.bodyFont, key=f"{theme_key}_body_font_input")
codeFont = col2.text_input("Code Font", value=theme_data[theme_key].themeInfo.codeFont, key=f"{theme_key}_code_font_input")
# fontFaces = col3.text_area("Font Faces", value=str(theme_data[theme_key].themeInfo.fontFaces), key=f"{theme_key}_font_faces_input")
theme_data[theme_key].name = name
theme_data[theme_key].icon = icon
theme_data[theme_key].order = order
theme_data[theme_key].themeInfo.primaryColor = primaryColor
theme_data[theme_key].themeInfo.backgroundColor = backgroundColor
theme_data[theme_key].themeInfo.secondaryBackgroundColor = secondaryBackgroundColor
theme_data[theme_key].themeInfo.textColor = textColor
theme_data[theme_key].themeInfo.widgetBackgroundColor = widgetBackgroundColor
theme_data[theme_key].themeInfo.widgetBorderColor = widgetBorderColor
theme_data[theme_key].themeInfo.skeletonBackgroundColor = skeletonBackgroundColor
theme_data[theme_key].themeInfo.bodyFont = bodyFont
theme_data[theme_key].themeInfo.codeFont = codeFont
theme_data[theme_key].themeInfo.radii["baseWidgetRadius"] = baseWidgetRadius
theme_data[theme_key].themeInfo.fontSizes["tinyFontSize"] = tiny_font_size
theme_data[theme_key].themeInfo.fontSizes["smallFontSize"] = small_font_size
theme_data[theme_key].themeInfo.fontSizes["baseFontSize"] = base_font_size
update_theme = st.form_submit_button("Save")
if update_theme:
st.session_state["theme_data"] = theme_data
if get_active_theme_key() == theme_key:
st_theme_changer(themes_data=theme_data, render_mode="change", default_init_theme_name=theme_key)
st.rerun()
with st.sidebar:
st_theme_changer(
themes_data=theme_data, render_mode="pills",
rerun_whole_st=True, key="first_pills"
)
```
## All Theme Configurations (CSS Properties)
### Theme Configuration Table
Here’s an overview of the theme configuration options and what they do:
| **Key** | **Value** | **Description** |
|-----------------------------|--------------------------------------------------------------------------------|---------------------------------------------------------------------------------------------------|
| `primaryColor` | `"#1A6CE7"` | The main accent color used throughout your app. For example, widgets like `st.checkbox`, `st.slider`, and `st.text_input` (when focused) use this color. |
| `backgroundColor` | `"#FFFFFF"` | The main background color of your app. |
| `secondaryBackgroundColor` | `"#F5F5F5"` | Used as a secondary background color, like for the sidebar or interactive widgets. |
| `textColor` | `"#1A1D21"` | Controls the text color for most elements in your app. |
| `font` | `0` `1` `2` [`"sans serif" ` `"serif"` `"monospace"`] | Sets the font used in your app. Options are `"sans serif"`, `"serif"`, or `"monospace"`. Defaults to `"sans serif"` if unset. |
| `base` | `0` `1` [`light` `dark`] | Lets you create custom themes by slightly modifying one of the preset Streamlit themes. |
| *`widgetBackgroundColor` | `"#FFFFFF"` | Background color for widgets like inputs or sliders. |
| *`widgetBorderColor` | `"#D3DAE8"` | Border color for widgets and interactive elements. |
| *`skeletonBackgroundColor` | `"#CCDDEE"` | Background color for loading placeholders (skeletons). |
| *`bodyFont` | `"Inter", "Source Sans Pro", sans-serif` | Default font for body text. |
| *`codeFont` | `"Apercu Mono", "Source Code Pro", monospace` | Font used for code or monospaced text. |
| *`fontFaces` | [Array of font families with URLs and weights](#font-faces-details-fontfaces-) | Custom fonts, including font-family, URL, and weight (e.g., Inter, Apercu Mono). |
| *`radii.checkboxRadius` | `3` | Border radius for checkboxes. |
| *`radii.baseWidgetRadius` | `6` | Border radius for widgets like buttons or inputs. |
| *`fontSizes.tinyFontSize` | `10` | Font size for tiny text (e.g., labels). |
| *`fontSizes.smallFontSize` | `12` | Font size for small text, like secondary descriptions. |
| *`fontSizes.baseFontSize` | `14` | Base font size for regular text. |
> The parameters marked with a * are new in Streamlit’s configuration. You can tweak them to adjust fonts and font sizes.
> Properties with a `.` in their names are part of nested objects. Details about these nested objects are provided below.
### Font Faces Details `"fontFaces": [...]`
| **`"family"`** | **`"url"`** | **`"weight"`** | **Description** |
|------------------|------------------------------------------------------------------------------------------------|------------|-----------------------------------------|
| `Inter` | `https://rsms.me/inter/font-files/Inter-Regular.woff2?v=3.19` | 400 | Regular weight of the Inter font. |
| `Inter` | `https://rsms.me/inter/font-files/Inter-SemiBold.woff2?v=3.19` | 600 | Semi-bold weight of the Inter font. |
| `Inter` | `https://rsms.me/inter/font-files/Inter-Bold.woff2?v=3.19` | 700 | Bold weight of the Inter font. |
| `Apercu Mono` | `https://app.snowflake.com/static/2c4863733dec5a69523e.woff2` | 400 | Regular weight of the Apercu Mono font. |
| `Apercu Mono` | `https://app.snowflake.com/static/e903ae189d31a97e231e.woff2` | 500 | Medium weight of the Apercu Mono font. |
| `Apercu Mono` | `https://app.snowflake.com/static/32447307374154c88bc0.woff2` | 700 | Bold weight of the Apercu Mono font. |
### Theme Configuration: Properties and Data Types
### Enum Descriptions
#### Font Family (`font`)
- `0` (SANS_SERIF): Standard sans-serif font family.
- `1` (SERIF): Standard serif font family.
- `2` (MONOSPACE): Monospaced font family, often for code.
#### Base Theme (`base`)
- `0` (LIGHT): Light base theme.
- `1` (DARK): Dark base theme.
### Nested Object Details
#### `radii`
| **Sub-Property** | **Type** | **Description** |
|-----------------------|----------|------------------------------------------------------|
| `checkboxRadius` | `number` | Radius for checkboxes. |
| `baseWidgetRadius` | `number` | Radius for widgets like buttons or inputs. |
#### `fontSizes`
| **Sub-Property** | **Type** | **Description** |
|-----------------------|----------|------------------------------------------------------|
| `tinyFontSize` | `number` | Font size for tiny text. |
| `smallFontSize` | `number` | Font size for small text. |
| `baseFontSize` | `number` | Default font size for regular text. |
---
### Workaround for Switching Themes Without Custom Components
You can use query parameters in the URL to switch between light and dark themes. However, this requires a page reload and won’t allow custom themes. Examples:
- `?embed_options=light_theme`
- `?embed_options=dark_theme`
---
### Override Base "Dark" or "Light" Themes (Maybe in future 🤞0)
Currently, you can’t override the default "Dark" or "Light" themes directly in Streamlit. Until the Streamlit team releases support for the `SET_CUSTOM_THEME_CONFIG` event, you’re limited to modifying the available properties listed above. But for your understanding, here's the all properties that streamlit team can configurate the frontend styles for Dark and Light themes.
```json
{
"name": "Dark",
"emotion": {
"inSidebar": false,
"breakpoints": {
"hideWidgetDetails": 180,
"hideNumberInputControls": 120,
"sm": "576px",
"columns": "640px",
"md": "768px"
},
"colors": {
"transparent": "transparent",
"black": "#000000",
"white": "#ffffff",
"gray10": "#fafafa",
"gray20": "#f0f2f6",
"gray30": "#e6eaf1",
"gray40": "#d5dae5",
"gray50": "#bfc5d3",
"gray60": "#a3a8b8",
"gray70": "#808495",
"gray80": "#555867",
"gray85": "#31333F",
"gray90": "#262730",
"gray100": "#0e1117",
"red10": "#fff0f0",
"red20": "#ffdede",
"red30": "#ffc7c7",
"red40": "#ffabab",
"red50": "#ff8c8c",
"red60": "#ff6c6c",
"red70": "#ff4b4b",
"red80": "#ff2b2b",
"red90": "#bd4043",
"red100": "#7d353b",
"orange10": "#fffae8",
"orange20": "#fff6d0",
"orange30": "#ffecb0",
"orange40": "#ffe08e",
"orange50": "#ffd16a",
"orange60": "#ffbd45",
"orange70": "#ffa421",
"orange80": "#ff8700",
"orange90": "#ed6f13",
"orange100": "#d95a00",
"yellow10": "#ffffe1",
"yellow20": "#ffffc2",
"yellow30": "#ffffa0",
"yellow40": "#ffff7d",
"yellow50": "#ffff59",
"yellow60": "#fff835",
"yellow70": "#ffe312",
"yellow80": "#faca2b",
"yellow90": "#edbb16",
"yellow100": "#dea816",
"yellow110": "#916e10",
"green10": "#dffde9",
"green20": "#c0fcd3",
"green30": "#9ef6bb",
"green40": "#7defa1",
"green50": "#5ce488",
"green60": "#3dd56d",
"green70": "#21c354",
"green80": "#09ab3b",
"green90": "#158237",
"green100": "#177233",
"blueGreen10": "#dcfffb",
"blueGreen20": "#bafff7",
"blueGreen30": "#93ffee",
"blueGreen40": "#6bfde3",
"blueGreen50": "#45f4d5",
"blueGreen60": "#20e7c5",
"blueGreen70": "#00d4b1",
"blueGreen80": "#29b09d",
"blueGreen90": "#2c867c",
"blueGreen100": "#246e69",
"lightBlue10": "#e0feff",
"lightBlue20": "#bffdff",
"lightBlue30": "#9af8ff",
"lightBlue40": "#73efff",
"lightBlue50": "#4be4ff",
"lightBlue60": "#24d4ff",
"lightBlue70": "#00c0f2",
"lightBlue80": "#00a4d4",
"lightBlue90": "#0d8cb5",
"lightBlue100": "#15799e",
"blue10": "#e4f5ff",
"blue20": "#c7ebff",
"blue30": "#a6dcff",
"blue40": "#83c9ff",
"blue50": "#60b4ff",
"blue60": "#3d9df3",
"blue70": "#1c83e1",
"blue80": "#0068c9",
"blue90": "#0054a3",
"blue100": "#004280",
"purple10": "#f5ebff",
"purple20": "#ebd6ff",
"purple30": "#dbbbff",
"purple40": "#c89dff",
"purple50": "#b27eff",
"purple60": "#9a5dff",
"purple70": "#803df5",
"purple80": "#6d3fc0",
"purple90": "#583f84",
"purple100": "#3f3163",
"bgColor": "#0e1117",
"secondaryBg": "#262730",
"bodyText": "#fafafa",
"warning": "#ffffc2",
"warningBg": "rgba(255, 227, 18, 0.2)",
"success": "#dffde9",
"successBg": "rgba(61, 213, 109, 0.2)",
"infoBg": "rgba(61, 157, 243, 0.2)",
"info": "#c7ebff",
"danger": "#ffdede",
"dangerBg": "rgba(255, 108, 108, 0.2)",
"primary": "#ff4b4b",
"disabled": "#808495",
"lightestGray": "#f0f2f6",
"lightGray": "#e6eaf1",
"gray": "#a3a8b8",
"darkGray": "#808495",
"red": "#ff2b2b",
"blue": "#0068c9",
"green": "#09ab3b",
"yellow": "#faca2b",
"linkText": "hsla(209, 100%, 59%, 1)",
"fadedText05": "rgba(250, 250, 250, 0.1)",
"fadedText10": "rgba(250, 250, 250, 0.2)",
"fadedText20": "rgba(250, 250, 250, 0.3)",
"fadedText40": "rgba(250, 250, 250, 0.4)",
"fadedText60": "rgba(250, 250, 250, 0.6)",
"bgMix": "rgba(26, 28, 36, 1)",
"darkenedBgMix100": "hsla(228, 16%, 72%, 1)",
"darkenedBgMix25": "rgba(172, 177, 195, 0.25)",
"darkenedBgMix15": "rgba(172, 177, 195, 0.15)",
"lightenedBg05": "hsla(220, 24%, 10%, 1)",
"borderColor": "rgba(250, 250, 250, 0.2)",
"borderColorLight": "rgba(250, 250, 250, 0.1)",
"codeTextColor": "#09ab3b",
"codeHighlightColor": "rgba(26, 28, 36, 1)",
"metricPositiveDeltaColor": "#09ab3b",
"metricNegativeDeltaColor": "#ff2b2b",
"metricNeutralDeltaColor": "rgba(250, 250, 250, 0.6)",
"docStringModuleText": "#fafafa",
"docStringTypeText": "#21c354",
"docStringContainerBackground": "rgba(38, 39, 48, 0.4)",
"headingColor": "#fafafa",
"navTextColor": "#bfc5d3",
"navActiveTextColor": "#fafafa",
"navIconColor": "#808495",
"sidebarControlColor": "#fafafa"
},
"fonts": {
"sansSerif": "\"Source Sans Pro\", sans-serif",
"monospace": "\"Source Code Pro\", monospace",
"serif": "\"Source Serif Pro\", serif",
"materialIcons": "Material Symbols Rounded"
},
"fontSizes": {
"twoSm": "12px",
"sm": "14px",
"md": "1rem",
"mdLg": "1.125rem",
"lg": "1.25rem",
"xl": "1.5rem",
"twoXL": "1.75rem",
"threeXL": "2.25rem",
"fourXL": "2.75rem",
"twoSmPx": 12,
"smPx": 14,
"mdPx": 16
},
"fontWeights": {
"normal": 400,
"bold": 600,
"extrabold": 700
},
"genericFonts": {
"bodyFont": "\"Source Sans Pro\", sans-serif",
"codeFont": "\"Source Code Pro\", monospace",
"headingFont": "\"Source Sans Pro\", sans-serif",
"iconFont": "Material Symbols Rounded"
},
"iconSizes": {
"xs": "0.5rem",
"sm": "0.75rem",
"md": "0.9rem",
"base": "1rem",
"lg": "1.25rem",
"xl": "1.5rem",
"twoXL": "1.8rem",
"threeXL": "2.3rem"
},
"lineHeights": {
"none": 1,
"headings": 1.2,
"tight": 1.25,
"inputWidget": 1.4,
"small": 1.5,
"base": 1.6,
"menuItem": 2
},
"radii": {
"md": "0.25rem",
"default": "0.5rem",
"xl": "0.75rem",
"xxl": "1rem",
"full": "9999px"
},
"sizes": {
"full": "100%",
"headerHeight": "3.75rem",
"fullScreenHeaderHeight": "2.875rem",
"sidebarTopSpace": "6rem",
"toastWidth": "21rem",
"contentMaxWidth": "46rem",
"maxChartTooltipWidth": "30rem",
"checkbox": "1rem",
"borderWidth": "1px",
"smallElementHeight": "1.5rem",
"minElementHeight": "2.5rem",
"largestElementHeight": "4.25rem",
"smallLogoHeight": "1.25rem",
"defaultLogoHeight": "1.5rem",
"largeLogoHeight": "2rem",
"sliderThumb": "0.75rem",
"wideSidePadding": "5rem",
"headerDecorationHeight": "0.125rem",
"appRunningMen": "1.6rem",
"appStatusMaxWidth": "20rem",
"spinnerSize": "1.375rem",
"spinnerThickness": "0.2rem",
"tabHeight": "2.5rem",
"minPopupWidth": "20rem",
"maxTooltipHeight": "18.75rem",
"chatAvatarSize": "2rem",
"clearIconSize": "1.5em",
"numberInputControlsWidth": "2rem",
"emptyDropdownHeight": "5.625rem",
"dropdownItemHeight": "2.5rem",
"maxDropdownHeight": "18.75rem",
"appDefaultBottomPadding": "3.5rem"
},
"spacing": {
"px": "1px",
"none": "0",
"threeXS": "0.125rem",
"twoXS": "0.25rem",
"xs": "0.375rem",
"sm": "0.5rem",
"md": "0.75rem",
"lg": "1rem",
"xl": "1.25rem",
"twoXL": "1.5rem",
"threeXL": "2rem",
"fourXL": "4rem"
},
"zIndices": {
"hide": -1,
"auto": "auto",
"base": 0,
"priority": 1,
"sidebar": 100,
"menuButton": 110,
"balloons": 1000000,
"header": 999990,
"sidebarMobile": 999995,
"popupMenu": 1000040,
"fullscreenWrapper": 1000050,
"tablePortal": 1000110,
"bottom": 99,
"cacheSpinner": 101,
"toast": 100,
"vegaTooltips": 1000060
}
},
"basewebTheme": {
"animation": {
"timing100": "100ms",
"timing200": "200ms",
"timing300": "300ms",
"timing400": "400ms",
"timing500": "500ms",
"timing600": "600ms",
"timing700": "700ms",
"timing800": "800ms",
"timing900": "900ms",
"timing1000": "1000ms",
"easeInCurve": "cubic-bezier(.8, .2, .6, 1)",
"easeOutCurve": "cubic-bezier(.2, .8, .4, 1)",
"easeInOutCurve": "cubic-bezier(0.4, 0, 0.2, 1)",
"easeInQuinticCurve": "cubic-bezier(0.755, 0.05, 0.855, 0.06)",
"easeOutQuinticCurve": "cubic-bezier(0.23, 1, 0.32, 1)",
"easeInOutQuinticCurve": "cubic-bezier(0.86, 0, 0.07, 1)",
"linearCurve": "cubic-bezier(0, 0, 1, 1)"
},
"borders": {
"border100": {
"borderColor": "hsla(0, 0%, 0%, 0.04)",
"borderStyle": "solid",
"borderWidth": "1px"
},
"border200": {
"borderColor": "hsla(0, 0%, 0%, 0.08)",
"borderStyle": "solid",
"borderWidth": "1px"
},
"border300": {
"borderColor": "hsla(0, 0%, 0%, 0.12)",
"borderStyle": "solid",
"borderWidth": "1px"
},
"border400": {
"borderColor": "hsla(0, 0%, 0%, 0.16)",
"borderStyle": "solid",
"borderWidth": "1px"
},
"border500": {
"borderColor": "hsla(0, 0%, 0%, 0.2)",
"borderStyle": "solid",
"borderWidth": "1px"
},
"border600": {
"borderColor": "hsla(0, 0%, 0%, 0.24)",
"borderStyle": "solid",
"borderWidth": "1px"
},
"radius100": "0.5rem",
"radius200": "0.5rem",
"radius300": "0.5rem",
"radius400": "0.5rem",
"radius500": "0.5rem",
"useRoundedCorners": true,
"buttonBorderRadiusMini": "0.25rem",
"buttonBorderRadius": "0.5rem",
"checkboxBorderRadius": "0.25rem",
"inputBorderRadiusMini": "0.25rem",
"inputBorderRadius": "0.5rem",
"popoverBorderRadius": "0.5rem",
"surfaceBorderRadius": "0.5rem",
"tagBorderRadius": "0.25rem"
},
"breakpoints": {
"small": 320,
"medium": 600,
"large": 1136
},
"colors": {
"primaryA": "#ff4b4b",
"primaryB": "#141414",
"primary": "#ff4b4b",
"primary50": "#F6F6F6",
"primary100": "#ff4b4b",
"primary200": "#ff4b4b",
"primary300": "#ff4b4b",
"primary400": "#ff4b4b",
"primary500": "#ff4b4b",
"primary600": "#ff4b4b",
"primary700": "#ff4b4b",
"accent": "rgba(255, 75, 75, 0.5)",
"accent50": "#EFF3FE",
"accent100": "#D4E2FC",
"accent200": "#A0BFF8",
"accent300": "#5B91F5",
"accent400": "#276EF1",
"accent500": "#1E54B7",
"accent600": "#174291",
"accent700": "#102C60",
"negative": "#AB1300",
"negative50": "#FFEFED",
"negative100": "#FED7D2",
"negative200": "#F1998E",
"negative300": "#E85C4A",
"negative400": "#E11900",
"negative500": "#AB1300",
"negative600": "#870F00",
"negative700": "#5A0A00",
"warning": "#BC8B2C",
"warning50": "#FFFAF0",
"warning100": "#FFF2D9",
"warning200": "#FFE3AC",
"warning300": "#FFCF70",
"warning400": "#FFC043",
"warning500": "#BC8B2C",
"warning600": "#996F00",
"warning700": "#674D1B",
"positive": "#048848",
"positive50": "#E6F2ED",
"positive100": "#ADDEC9",
"positive200": "#66D19E",
"positive300": "#06C167",
"positive400": "#048848",
"positive500": "#03703C",
"positive600": "#03582F",
"positive700": "#10462D",
"white": "#ffffff",
"black": "#000000",
"mono100": "#0e1117",
"mono200": "#262730",
"mono300": "#e6eaf1",
"mono400": "#e6eaf1",
"mono500": "#a3a8b8",
"mono600": "rgba(250, 250, 250, 0.4)",
"mono700": "#a3a8b8",
"mono800": "#fafafa",
"mono900": "#fafafa",
"mono1000": "#000000",
"ratingInactiveFill": "#6B6B6B",
"ratingStroke": "#333333",
"bannerActionLowInfo": "#D4E2FC",
"bannerActionLowNegative": "#FED7D2",
"bannerActionLowPositive": "#ADDEC9",
"bannerActionLowWarning": "#FFE3AC",
"bannerActionHighInfo": "#1E54B7",
"bannerActionHighNegative": "#AB1300",
"bannerActionHighPositive": "#03703C",
"bannerActionHighWarning": "#FFE3AC",
"buttonPrimaryFill": "#FFFFFF",
"buttonPrimaryText": "#FFFFFF",
"buttonPrimaryHover": "#ff4b4b",
"buttonPrimaryActive": "#ff4b4b",
"buttonPrimarySelectedFill": "#ff4b4b",
"buttonPrimarySelectedText": "#FFFFFF",
"buttonPrimarySpinnerForeground": "#276EF1",
"buttonPrimarySpinnerBackground": "#141414",
"buttonSecondaryFill": "#ff4b4b",
"buttonSecondaryText": "#FFFFFF",
"buttonSecondaryHover": "#ff4b4b",
"buttonSecondaryActive": "#ff4b4b",
"buttonSecondarySelectedFill": "#ff4b4b",
"buttonSecondarySelectedText": "#FFFFFF",
"buttonSecondarySpinnerForeground": "#276EF1",
"buttonSecondarySpinnerBackground": "#141414",
"buttonTertiaryFill": "transparent",
"buttonTertiaryText": "#FFFFFF",
"buttonTertiaryHover": "#F6F6F6",
"buttonTertiaryActive": "#ff4b4b",
"buttonTertiarySelectedFill": "#ff4b4b",
"buttonTertiarySelectedText": "#FFFFFF",
"buttonTertiaryDisabledActiveFill": "#F6F6F6",
"buttonTertiaryDisabledActiveText": "rgba(250, 250, 250, 0.4)",
"buttonTertiarySpinnerForeground": "#276EF1",
"buttonTertiarySpinnerBackground": "#ff4b4b",
"buttonDisabledFill": "hsla(220, 24%, 10%, 1)",
"buttonDisabledText": "rgba(250, 250, 250, 0.4)",
"buttonDisabledActiveFill": "#a3a8b8",
"buttonDisabledActiveText": "#0e1117",
"buttonDisabledSpinnerForeground": "rgba(250, 250, 250, 0.4)",
"buttonDisabledSpinnerBackground": "#d5dae5",
"breadcrumbsText": "#000000",
"breadcrumbsSeparatorFill": "#a3a8b8",
"calendarBackground": "#0e1117",
"calendarForeground": "#fafafa",
"calendarForegroundDisabled": "#a3a8b8",
"calendarHeaderBackground": "#262730",
"calendarHeaderForeground": "#fafafa",
"calendarHeaderBackgroundActive": "#262730",
"calendarHeaderForegroundDisabled": "#d5dae5",
"calendarDayForegroundPseudoSelected": "#fafafa",
"calendarDayBackgroundPseudoSelectedHighlighted": "#ff4b4b",
"calendarDayForegroundPseudoSelectedHighlighted": "#fafafa",
"calendarDayBackgroundSelected": "#ff4b4b",
"calendarDayForegroundSelected": "#ffffff",
"calendarDayBackgroundSelectedHighlighted": "#ff4b4b",
"calendarDayForegroundSelectedHighlighted": "#ffffff",
"comboboxListItemFocus": "#262730",
"comboboxListItemHover": "#e6eaf1",
"fileUploaderBackgroundColor": "#262730",
"fileUploaderBackgroundColorActive": "#F6F6F6",
"fileUploaderBorderColorActive": "#FFFFFF",
"fileUploaderBorderColorDefault": "#a3a8b8",
"fileUploaderMessageColor": "#fafafa",
"linkText": "#FFFFFF",
"linkVisited": "#ff4b4b",
"linkHover": "#ff4b4b",
"linkActive": "#ff4b4b",
"listHeaderFill": "#FFFFFF",
"listBodyFill": "#FFFFFF",
"progressStepsCompletedText": "#FFFFFF",
"progressStepsCompletedFill": "#FFFFFF",
"progressStepsActiveText": "#FFFFFF",
"progressStepsActiveFill": "#FFFFFF",
"toggleFill": "#FFFFFF",
"toggleFillChecked": "#FFFFFF",
"toggleFillDisabled": "rgba(250, 250, 250, 0.4)",
"toggleTrackFill": "#d5dae5",
"toggleTrackFillDisabled": "#262730",
"tickFill": "hsla(220, 24%, 10%, 1)",
"tickFillHover": "#262730",
"tickFillActive": "#262730",
"tickFillSelected": "#ff4b4b",
"tickFillSelectedHover": "#ff4b4b",
"tickFillSelectedHoverActive": "#ff4b4b",
"tickFillError": "#FFEFED",
"tickFillErrorHover": "#FED7D2",
"tickFillErrorHoverActive": "#F1998E",
"tickFillErrorSelected": "#E11900",
"tickFillErrorSelectedHover": "#AB1300",
"tickFillErrorSelectedHoverActive": "#870F00",
"tickFillDisabled": "rgba(250, 250, 250, 0.4)",
"tickBorder": "#a3a8b8",
"tickBorderError": "#E11900",
"tickMarkFill": "#f0f2f6",
"tickMarkFillError": "#FFFFFF",
"tickMarkFillDisabled": "hsla(220, 24%, 10%, 1)",
"sliderTrackFill": "#d5dae5",
"sliderHandleFill": "#E2E2E2",
"sliderHandleFillDisabled": "#ff4b4b",
"sliderHandleInnerFill": "#d5dae5",
"sliderTrackFillHover": "#a3a8b8",
"sliderTrackFillActive": "rgba(250, 250, 250, 0.4)",
"sliderTrackFillDisabled": "#262730",
"sliderHandleInnerFillDisabled": "#d5dae5",
"sliderHandleInnerFillSelectedHover": "#FFFFFF",
"sliderHandleInnerFillSelectedActive": "#ff4b4b",
"inputBorder": "#262730",
"inputFill": "#262730",
"inputFillError": "#FFEFED",
"inputFillDisabled": "#262730",
"inputFillActive": "#262730",
"inputFillPositive": "#E6F2ED",
"inputTextDisabled": "rgba(250, 250, 250, 0.4)",
"inputBorderError": "#F1998E",
"inputBorderPositive": "#66D19E",
"inputEnhancerFill": "#e6eaf1",
"inputEnhancerFillDisabled": "#262730",
"inputEnhancerTextDisabled": "rgba(250, 250, 250, 0.4)",
"inputPlaceholder": "rgba(250, 250, 250, 0.6)",
"inputPlaceholderDisabled": "rgba(250, 250, 250, 0.4)",
"menuFill": "#0e1117",
"menuFillHover": "#262730",
"menuFontDefault": "#fafafa",
"menuFontDisabled": "#a3a8b8",
"menuFontHighlighted": "#fafafa",
"menuFontSelected": "#fafafa",
"modalCloseColor": "#fafafa",
"modalCloseColorHover": "#fafafa",
"modalCloseColorFocus": "#fafafa",
"tabBarFill": "#262730",
"tabColor": "#fafafa",
"notificationInfoBackground": "rgba(61, 157, 243, 0.2)",
"notificationInfoText": "#c7ebff",
"notificationPositiveBackground": "rgba(61, 213, 109, 0.2)",
"notificationPositiveText": "#dffde9",
"notificationWarningBackground": "rgba(255, 227, 18, 0.2)",
"notificationWarningText": "#ffffc2",
"notificationNegativeBackground": "rgba(255, 108, 108, 0.2)",
"notificationNegativeText": "#ffdede",
"tagFontDisabledRampUnit": "100",
"tagSolidFontRampUnit": "0",
"tagSolidRampUnit": "400",
"tagOutlinedFontRampUnit": "400",
"tagOutlinedRampUnit": "200",
"tagSolidHoverRampUnit": "50",
"tagSolidActiveRampUnit": "100",
"tagSolidDisabledRampUnit": "50",
"tagSolidFontHoverRampUnit": "500",
"tagLightRampUnit": "50",
"tagLightHoverRampUnit": "100",
"tagLightActiveRampUnit": "100",
"tagLightFontRampUnit": "500",
"tagLightFontHoverRampUnit": "500",
"tagOutlinedHoverRampUnit": "50",
"tagOutlinedActiveRampUnit": "0",
"tagOutlinedFontHoverRampUnit": "400",
"tagNeutralFontDisabled": "rgba(250, 250, 250, 0.4)",
"tagNeutralOutlinedDisabled": "#e6eaf1",
"tagNeutralSolidFont": "#FFFFFF",
"tagNeutralSolidBackground": "#000000",
"tagNeutralOutlinedBackground": "rgba(250, 250, 250, 0.4)",
"tagNeutralOutlinedFont": "#000000",
"tagNeutralSolidHover": "#e6eaf1",
"tagNeutralSolidActive": "#e6eaf1",
"tagNeutralSolidDisabled": "#262730",
"tagNeutralSolidFontHover": "#fafafa",
"tagNeutralLightBackground": "#e6eaf1",
"tagNeutralLightHover": "#e6eaf1",
"tagNeutralLightActive": "#e6eaf1",
"tagNeutralLightDisabled": "#262730",
"tagNeutralLightFont": "#fafafa",
"tagNeutralLightFontHover": "#fafafa",
"tagNeutralOutlinedActive": "#fafafa",
"tagNeutralOutlinedFontHover": "#fafafa",
"tagNeutralOutlinedHover": "rgba(0, 0, 0, 0.08)",
"tagPrimaryFontDisabled": "rgba(250, 250, 250, 0.4)",
"tagPrimaryOutlinedDisabled": "transparent",
"tagPrimarySolidFont": "#FFFFFF",
"tagPrimarySolidBackground": "#ff4b4b",
"tagPrimaryOutlinedFontHover": "#FFFFFF",
"tagPrimaryOutlinedFont": "#FFFFFF",
"tagPrimarySolidHover": "#ff4b4b",
"tagPrimarySolidActive": "#ff4b4b",
"tagPrimarySolidDisabled": "#F6F6F6",
"tagPrimarySolidFontHover": "#ff4b4b",
"tagPrimaryLightBackground": "#F6F6F6",
"tagPrimaryLightHover": "#ff4b4b",
"tagPrimaryLightActive": "#ff4b4b",
"tagPrimaryLightDisabled": "#F6F6F6",
"tagPrimaryLightFont": "#ff4b4b",
"tagPrimaryLightFontHover": "#ff4b4b",
"tagPrimaryOutlinedActive": "#ff4b4b",
"tagPrimaryOutlinedHover": "rgba(0, 0, 0, 0.08)",
"tagPrimaryOutlinedBackground": "#ff4b4b",
"tagAccentFontDisabled": "#A0BFF8",
"tagAccentOutlinedDisabled": "#A0BFF8",
"tagAccentSolidFont": "#FFFFFF",
"tagAccentSolidBackground": "#276EF1",
"tagAccentOutlinedBackground": "#A0BFF8",
"tagAccentOutlinedFont": "#276EF1",
"tagAccentSolidHover": "#EFF3FE",
"tagAccentSolidActive": "#D4E2FC",
"tagAccentSolidDisabled": "#EFF3FE",
"tagAccentSolidFontHover": "#1E54B7",
"tagAccentLightBackground": "#EFF3FE",
"tagAccentLightHover": "#D4E2FC",
"tagAccentLightActive": "#D4E2FC",
"tagAccentLightDisabled": "#EFF3FE",
"tagAccentLightFont": "#1E54B7",
"tagAccentLightFontHover": "#1E54B7",
"tagAccentOutlinedActive": "#174291",
"tagAccentOutlinedFontHover": "#276EF1",
"tagAccentOutlinedHover": "rgba(0, 0, 0, 0.08)",
"tagPositiveFontDisabled": "#66D19E",
"tagPositiveOutlinedDisabled": "#66D19E",
"tagPositiveSolidFont": "#FFFFFF",
"tagPositiveSolidBackground": "#048848",
"tagPositiveOutlinedBackground": "#66D19E",
"tagPositiveOutlinedFont": "#048848",
"tagPositiveSolidHover": "#E6F2ED",
"tagPositiveSolidActive": "#ADDEC9",
"tagPositiveSolidDisabled": "#E6F2ED",
"tagPositiveSolidFontHover": "#03703C",
"tagPositiveLightBackground": "#E6F2ED",
"tagPositiveLightHover": "#ADDEC9",
"tagPositiveLightActive": "#ADDEC9",
"tagPositiveLightDisabled": "#E6F2ED",
"tagPositiveLightFont": "#03703C",
"tagPositiveLightFontHover": "#03703C",
"tagPositiveOutlinedActive": "#03582F",
"tagPositiveOutlinedFontHover": "#048848",
"tagPositiveOutlinedHover": "rgba(0, 0, 0, 0.08)",
"tagWarningFontDisabled": "#FFCF70",
"tagWarningOutlinedDisabled": "#FFCF70",
"tagWarningSolidFont": "#674D1B",
"tagWarningSolidBackground": "#FFC043",
"tagWarningOutlinedBackground": "#FFCF70",
"tagWarningOutlinedFont": "#996F00",
"tagWarningSolidHover": "#FFFAF0",
"tagWarningSolidActive": "#FFF2D9",
"tagWarningSolidDisabled": "#FFFAF0",
"tagWarningSolidFontHover": "#BC8B2C",
"tagWarningLightBackground": "#FFFAF0",
"tagWarningLightHover": "#FFF2D9",
"tagWarningLightActive": "#FFF2D9",
"tagWarningLightDisabled": "#FFFAF0",
"tagWarningLightFont": "#BC8B2C",
"tagWarningLightFontHover": "#BC8B2C",
"tagWarningOutlinedActive": "#996F00",
"tagWarningOutlinedFontHover": "#996F00",
"tagWarningOutlinedHover": "rgba(0, 0, 0, 0.08)",
"tagNegativeFontDisabled": "#F1998E",
"tagNegativeOutlinedDisabled": "#F1998E",
"tagNegativeSolidFont": "#FFFFFF",
"tagNegativeSolidBackground": "#E11900",
"tagNegativeOutlinedBackground": "#F1998E",
"tagNegativeOutlinedFont": "#E11900",
"tagNegativeSolidHover": "#FFEFED",
"tagNegativeSolidActive": "#FED7D2",
"tagNegativeSolidDisabled": "#FFEFED",
"tagNegativeSolidFontHover": "#AB1300",
"tagNegativeLightBackground": "#FFEFED",
"tagNegativeLightHover": "#FED7D2",
"tagNegativeLightActive": "#FED7D2",
"tagNegativeLightDisabled": "#FFEFED",
"tagNegativeLightFont": "#AB1300",
"tagNegativeLightFontHover": "#AB1300",
"tagNegativeOutlinedActive": "#870F00",
"tagNegativeOutlinedFontHover": "#E11900",
"tagNegativeOutlinedHover": "rgba(0, 0, 0, 0.08)",
"tableHeadBackgroundColor": "#0e1117",
"tableBackground": "#0e1117",
"tableStripedBackground": "#262730",
"tableFilter": "rgba(250, 250, 250, 0.4)",
"tableFilterHeading": "#a3a8b8",
"tableFilterBackground": "#0e1117",
"tableFilterFooterBackground": "#262730",
"toastText": "#FFFFFF",
"toastPrimaryText": "#FFFFFF",
"toastInfoBackground": "#276EF1",
"toastInfoText": "#FFFFFF",
"toastPositiveBackground": "#048848",
"toastPositiveText": "#FFFFFF",
"toastWarningBackground": "#FFC043",
"toastWarningText": "#000000",
"toastNegativeBackground": "#E11900",
"toastNegativeText": "#FFFFFF",
"spinnerTrackFill": "#fafafa",
"progressbarTrackFill": "#262730",
"tooltipBackground": "#fafafa",
"tooltipText": "#0e1117",
"backgroundPrimary": "#0e1117",
"backgroundSecondary": "#262730",
"backgroundTertiary": "#0e1117",
"backgroundInversePrimary": "#E2E2E2",
"backgroundInverseSecondary": "#1F1F1F",
"contentPrimary": "#fafafa",
"contentSecondary": "#545454",
"contentTertiary": "#6B6B6B",
"contentInversePrimary": "#141414",
"contentInverseSecondary": "#CBCBCB",
"contentInverseTertiary": "#AFAFAF",
"borderOpaque": "rgba(172, 177, 195, 0.25)",
"borderTransparent": "rgba(226, 226, 226, 0.08)",
"borderSelected": "#ff4b4b",
"borderInverseOpaque": "#333333",
"borderInverseTransparent": "rgba(20, 20, 20, 0.2)",
"borderInverseSelected": "#141414",
"backgroundStateDisabled": "#F6F6F6",
"backgroundOverlayDark": "rgba(0, 0, 0, 0.3)",
"backgroundOverlayLight": "rgba(0, 0, 0, 0.08)",
"backgroundOverlayArt": "rgba(0, 0, 0, 0.00)",
"backgroundAccent": "#276EF1",
"backgroundNegative": "#AB1300",
"backgroundWarning": "#BC8B2C",
"backgroundPositive": "#048848",
"backgroundLightAccent": "#EFF3FE",
"backgroundLightNegative": "#FFEFED",
"backgroundLightWarning": "#FFFAF0",
"backgroundLightPositive": "#E6F2ED",
"backgroundAlwaysDark": "#000000",
"backgroundAlwaysLight": "#FFFFFF",
"contentStateDisabled": "#AFAFAF",
"contentAccent": "#276EF1",
"contentOnColor": "#FFFFFF",
"contentOnColorInverse": "#000000",
"contentNegative": "#AB1300",
"contentWarning": "#996F00",
"contentPositive": "#048848",
"borderStateDisabled": "#F6F6F6",
"borderAccent": "#276EF1",
"borderAccentLight": "#A0BFF8",
"borderNegative": "#F1998E",
"borderWarning": "#FFE3AC",
"borderPositive": "#66D19E",
"safety": "#276EF1",
"eatsGreen400": "#048848",
"freightBlue400": "#0E1FC1",
"jumpRed400": "#E11900",
"rewardsTier1": "#276EF1",
"rewardsTier2": "#FFC043",
"rewardsTier3": "#8EA3AD",
"rewardsTier4": "#000000",
"membership": "#996F00",
"datepickerBackground": "#0e1117",
"inputEnhanceFill": "#262730"
},
"direction": "auto",
"grid": {
"columns": [
4,
8,
12
],
"gutters": [
16,
36,
36
],
"margins": [
16,
36,
64
],
"gaps": 0,
"unit": "px",
"maxWidth": 1280
},
"lighting": {
"shadow400": "0 1px 4px hsla(0, 0%, 0%, 0.16)",
"shadow500": "0 2px 8px hsla(0, 0%, 0%, 0.16)",
"shadow600": "0 4px 16px hsla(0, 0%, 0%, 0.16)",
"shadow700": "0 8px 24px hsla(0, 0%, 0%, 0.16)",
"overlay0": "inset 0 0 0 1000px hsla(0, 0%, 0%, 0)",
"overlay100": "inset 0 0 0 1000px hsla(0, 0%, 0%, 0.04)",
"overlay200": "inset 0 0 0 1000px hsla(0, 0%, 0%, 0.08)",
"overlay300": "inset 0 0 0 1000px hsla(0, 0%, 0%, 0.12)",
"overlay400": "inset 0 0 0 1000px hsla(0, 0%, 0%, 0.16)",
"overlay500": "inset 0 0 0 1000px hsla(0, 0%, 0%, 0.2)",
"overlay600": "inset 0 0 0 1000px hsla(0, 0%, 0%, 0.24)",
"shallowAbove": "0px -4px 16px rgba(0, 0, 0, 0.12)",
"shallowBelow": "0px 4px 16px rgba(0, 0, 0, 0.12)",
"deepAbove": "0px -16px 48px rgba(0, 0, 0, 0.22)",
"deepBelow": "0px 16px 48px rgba(0, 0, 0, 0.22)"
},
"mediaQuery": {
"small": "@media screen and (min-width: 320px)",
"medium": "@media screen and (min-width: 600px)",
"large": "@media screen and (min-width: 1136px)"
},
"sizing": {
"scale0": "2px",
"scale100": "4px",
"scale200": "6px",
"scale300": "8px",
"scale400": "10px",
"scale500": "12px",
"scale550": "14px",
"scale600": "16px",
"scale650": "18px",
"scale700": "20px",
"scale750": "22px",
"scale800": "24px",
"scale850": "28px",
"scale900": "32px",
"scale950": "36px",
"scale1000": "40px",
"scale1200": "48px",
"scale1400": "56px",
"scale1600": "64px",
"scale2400": "96px",
"scale3200": "128px",
"scale4800": "192px"
},
"typography": {
"font100": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "12px",
"fontWeight": "normal",
"lineHeight": "20px"
},
"font150": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "1rem",
"fontWeight": "normal",
"lineHeight": 1.6,
"fontSizeSm": "14px",
"lineHeightTight": 1.25
},
"font200": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "1rem",
"fontWeight": "normal",
"lineHeight": 1.6,
"fontSizeSm": "14px",
"lineHeightTight": 1.25
},
"font250": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "1rem",
"fontWeight": "normal",
"lineHeight": 1.6,
"fontSizeSm": "14px",
"lineHeightTight": 1.25
},
"font300": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "1rem",
"fontWeight": "normal",
"lineHeight": 1.6,
"fontSizeSm": "14px",
"lineHeightTight": 1.25
},
"font350": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "1rem",
"fontWeight": "normal",
"lineHeight": 1.6,
"fontSizeSm": "14px",
"lineHeightTight": 1.25
},
"font400": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "1rem",
"fontWeight": "normal",
"lineHeight": 1.6,
"fontSizeSm": "14px",
"lineHeightTight": 1.25
},
"font450": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "1rem",
"fontWeight": "normal",
"lineHeight": 1.6,
"fontSizeSm": "14px",
"lineHeightTight": 1.25
},
"font550": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "20px",
"fontWeight": 700,
"lineHeight": "28px"
},
"font650": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "24px",
"fontWeight": 700,
"lineHeight": "32px"
},
"font750": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "28px",
"fontWeight": 700,
"lineHeight": "36px"
},
"font850": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "32px",
"fontWeight": 700,
"lineHeight": "40px"
},
"font950": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "36px",
"fontWeight": 700,
"lineHeight": "44px"
},
"font1050": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "40px",
"fontWeight": 700,
"lineHeight": "52px"
},
"font1150": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "36px",
"fontWeight": 700,
"lineHeight": "44px"
},
"font1250": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "44px",
"fontWeight": 700,
"lineHeight": "52px"
},
"font1350": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "52px",
"fontWeight": 700,
"lineHeight": "64px"
},
"font1450": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "96px",
"fontWeight": 700,
"lineHeight": "112px"
},
"ParagraphXSmall": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "12px",
"fontWeight": "normal",
"lineHeight": "20px"
},
"ParagraphSmall": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "1rem",
"fontWeight": "normal",
"lineHeight": 1.6,
"fontSizeSm": "14px",
"lineHeightTight": 1.25
},
"ParagraphMedium": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "1rem",
"fontWeight": "normal",
"lineHeight": 1.6,
"fontSizeSm": "14px",
"lineHeightTight": 1.25
},
"ParagraphLarge": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "1rem",
"fontWeight": "normal",
"lineHeight": 1.6,
"fontSizeSm": "14px",
"lineHeightTight": 1.25
},
"LabelXSmall": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "1rem",
"fontWeight": "normal",
"lineHeight": 1.6,
"fontSizeSm": "14px",
"lineHeightTight": 1.25
},
"LabelSmall": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "1rem",
"fontWeight": "normal",
"lineHeight": 1.6,
"fontSizeSm": "14px",
"lineHeightTight": 1.25
},
"LabelMedium": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "1rem",
"fontWeight": "normal",
"lineHeight": 1.6,
"fontSizeSm": "14px",
"lineHeightTight": 1.25
},
"LabelLarge": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "1rem",
"fontWeight": "normal",
"lineHeight": 1.6,
"fontSizeSm": "14px",
"lineHeightTight": 1.25
},
"HeadingXSmall": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "20px",
"fontWeight": 700,
"lineHeight": "28px"
},
"HeadingSmall": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "24px",
"fontWeight": 700,
"lineHeight": "32px"
},
"HeadingMedium": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "28px",
"fontWeight": 700,
"lineHeight": "36px"
},
"HeadingLarge": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "32px",
"fontWeight": 700,
"lineHeight": "40px"
},
"HeadingXLarge": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "36px",
"fontWeight": 700,
"lineHeight": "44px"
},
"HeadingXXLarge": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "40px",
"fontWeight": 700,
"lineHeight": "52px"
},
"DisplayXSmall": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "36px",
"fontWeight": 700,
"lineHeight": "44px"
},
"DisplaySmall": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "44px",
"fontWeight": 700,
"lineHeight": "52px"
},
"DisplayMedium": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "52px",
"fontWeight": 700,
"lineHeight": "64px"
},
"DisplayLarge": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "96px",
"fontWeight": 700,
"lineHeight": "112px"
},
"MonoParagraphXSmall": {
"fontFamily": "\"Lucida Console\", Monaco, monospace",
"fontSize": "12px",
"fontWeight": "normal",
"lineHeight": "20px"
},
"MonoParagraphSmall": {
"fontFamily": "\"Lucida Console\", Monaco, monospace",
"fontSize": "14px",
"fontWeight": "normal",
"lineHeight": "20px"
},
"MonoParagraphMedium": {
"fontFamily": "\"Lucida Console\", Monaco, monospace",
"fontSize": "16px",
"fontWeight": "normal",
"lineHeight": "24px"
},
"MonoParagraphLarge": {
"fontFamily": "\"Lucida Console\", Monaco, monospace",
"fontSize": "18px",
"fontWeight": "normal",
"lineHeight": "28px"
},
"MonoLabelXSmall": {
"fontFamily": "\"Lucida Console\", Monaco, monospace",
"fontSize": "12px",
"fontWeight": 500,
"lineHeight": "16px"
},
"MonoLabelSmall": {
"fontFamily": "\"Lucida Console\", Monaco, monospace",
"fontSize": "14px",
"fontWeight": 500,
"lineHeight": "16px"
},
"MonoLabelMedium": {
"fontFamily": "\"Lucida Console\", Monaco, monospace",
"fontSize": "16px",
"fontWeight": 500,
"lineHeight": "20px"
},
"MonoLabelLarge": {
"fontFamily": "\"Lucida Console\", Monaco, monospace",
"fontSize": "18px",
"fontWeight": 500,
"lineHeight": "24px"
},
"MonoHeadingXSmall": {
"fontFamily": "\"Lucida Console\", Monaco, monospace",
"fontSize": "20px",
"fontWeight": 700,
"lineHeight": "28px"
},
"MonoHeadingSmall": {
"fontFamily": "\"Lucida Console\", Monaco, monospace",
"fontSize": "24px",
"fontWeight": 700,
"lineHeight": "32px"
},
"MonoHeadingMedium": {
"fontFamily": "\"Lucida Console\", Monaco, monospace",
"fontSize": "28px",
"fontWeight": 700,
"lineHeight": "36px"
},
"MonoHeadingLarge": {
"fontFamily": "\"Lucida Console\", Monaco, monospace",
"fontSize": "32px",
"fontWeight": 700,
"lineHeight": "40px"
},
"MonoHeadingXLarge": {
"fontFamily": "\"Lucida Console\", Monaco, monospace",
"fontSize": "36px",
"fontWeight": 700,
"lineHeight": "44px"
},
"MonoHeadingXXLarge": {
"fontFamily": "\"Lucida Console\", Monaco, monospace",
"fontSize": "40px",
"fontWeight": 700,
"lineHeight": "52px"
},
"MonoDisplayXSmall": {
"fontFamily": "\"Lucida Console\", Monaco, monospace",
"fontSize": "36px",
"fontWeight": 700,
"lineHeight": "44px"
},
"MonoDisplaySmall": {
"fontFamily": "\"Lucida Console\", Monaco, monospace",
"fontSize": "44px",
"fontWeight": 700,
"lineHeight": "52px"
},
"MonoDisplayMedium": {
"fontFamily": "\"Lucida Console\", Monaco, monospace",
"fontSize": "52px",
"fontWeight": 700,
"lineHeight": "64px"
},
"MonoDisplayLarge": {
"fontFamily": "\"Lucida Console\", Monaco, monospace",
"fontSize": "96px",
"fontWeight": 700,
"lineHeight": "112px"
},
"font460": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "1rem",
"fontSizeSm": "14px",
"fontWeight": "normal",
"lineHeight": 1.6,
"lineHeightTight": 1.25
},
"font470": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "1rem",
"fontSizeSm": "14px",
"fontWeight": "normal",
"lineHeight": 1.6,
"lineHeightTight": 1.25
},
"font500": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "1rem",
"fontSizeSm": "14px",
"fontWeight": "normal",
"lineHeight": 1.6,
"lineHeightTight": 1.25
},
"font600": {}
},
"zIndex": {
"modal": 2000
}
},
"primitives": {
"primaryA": "#E2E2E2",
"primaryB": "#141414",
"primary": "#FFFFFF",
"primary50": "#F6F6F6",
"primary100": "#EEEEEE",
"primary200": "#E2E2E2",
"primary300": "#CBCBCB",
"primary400": "#AFAFAF",
"primary500": "#6B6B6B",
"primary600": "#545454",
"primary700": "#333333",
"accent": "#276EF1",
"accent50": "#EFF3FE",
"accent100": "#D4E2FC",
"accent200": "#A0BFF8",
"accent300": "#5B91F5",
"accent400": "#276EF1",
"accent500": "#1E54B7",
"accent600": "#174291",
"accent700": "#102C60",
"negative": "#AB1300",
"negative50": "#FFEFED",
"negative100": "#FED7D2",
"negative200": "#F1998E",
"negative300": "#E85C4A",
"negative400": "#E11900",
"negative500": "#AB1300",
"negative600": "#870F00",
"negative700": "#5A0A00",
"warning": "#BC8B2C",
"warning50": "#FFFAF0",
"warning100": "#FFF2D9",
"warning200": "#FFE3AC",
"warning300": "#FFCF70",
"warning400": "#FFC043",
"warning500": "#BC8B2C",
"warning600": "#996F00",
"warning700": "#674D1B",
"positive": "#048848",
"positive50": "#E6F2ED",
"positive100": "#ADDEC9",
"positive200": "#66D19E",
"positive300": "#06C167",
"positive400": "#048848",
"positive500": "#03703C",
"positive600": "#03582F",
"positive700": "#10462D",
"white": "#FFFFFF",
"black": "#000000",
"mono100": "#CBCBCB",
"mono200": "#AFAFAF",
"mono300": "#6B6B6B",
"mono400": "#545454",
"mono500": "#333333",
"mono600": "#292929",
"mono700": "#1F1F1F",
"mono800": "#141414",
"mono900": "#111111",
"mono1000": "#000000",
"ratingInactiveFill": "#6B6B6B",
"ratingStroke": "#333333",
"primaryFontFamily": "system-ui, \"Helvetica Neue\", Helvetica, Arial, sans-serif"
}
}
```
### JSON Base Configuration for Streamlit Light Theme
```json
{
"name": "Light",
"emotion": {
"inSidebar": false,
"breakpoints": {
"hideWidgetDetails": 180,
"hideNumberInputControls": 120,
"sm": "576px",
"columns": "640px",
"md": "768px"
},
"colors": {
"transparent": "transparent",
"black": "#000000",
"white": "#ffffff",
"gray10": "#fafafa",
"gray20": "#f0f2f6",
"gray30": "#e6eaf1",
"gray40": "#d5dae5",
"gray50": "#bfc5d3",
"gray60": "#a3a8b8",
"gray70": "#808495",
"gray80": "#555867",
"gray85": "#31333F",
"gray90": "#262730",
"gray100": "#0e1117",
"red10": "#fff0f0",
"red20": "#ffdede",
"red30": "#ffc7c7",
"red40": "#ffabab",
"red50": "#ff8c8c",
"red60": "#ff6c6c",
"red70": "#ff4b4b",
"red80": "#ff2b2b",
"red90": "#bd4043",
"red100": "#7d353b",
"orange10": "#fffae8",
"orange20": "#fff6d0",
"orange30": "#ffecb0",
"orange40": "#ffe08e",
"orange50": "#ffd16a",
"orange60": "#ffbd45",
"orange70": "#ffa421",
"orange80": "#ff8700",
"orange90": "#ed6f13",
"orange100": "#d95a00",
"yellow10": "#ffffe1",
"yellow20": "#ffffc2",
"yellow30": "#ffffa0",
"yellow40": "#ffff7d",
"yellow50": "#ffff59",
"yellow60": "#fff835",
"yellow70": "#ffe312",
"yellow80": "#faca2b",
"yellow90": "#edbb16",
"yellow100": "#dea816",
"yellow110": "#916e10",
"green10": "#dffde9",
"green20": "#c0fcd3",
"green30": "#9ef6bb",
"green40": "#7defa1",
"green50": "#5ce488",
"green60": "#3dd56d",
"green70": "#21c354",
"green80": "#09ab3b",
"green90": "#158237",
"green100": "#177233",
"blueGreen10": "#dcfffb",
"blueGreen20": "#bafff7",
"blueGreen30": "#93ffee",
"blueGreen40": "#6bfde3",
"blueGreen50": "#45f4d5",
"blueGreen60": "#20e7c5",
"blueGreen70": "#00d4b1",
"blueGreen80": "#29b09d",
"blueGreen90": "#2c867c",
"blueGreen100": "#246e69",
"lightBlue10": "#e0feff",
"lightBlue20": "#bffdff",
"lightBlue30": "#9af8ff",
"lightBlue40": "#73efff",
"lightBlue50": "#4be4ff",
"lightBlue60": "#24d4ff",
"lightBlue70": "#00c0f2",
"lightBlue80": "#00a4d4",
"lightBlue90": "#0d8cb5",
"lightBlue100": "#15799e",
"blue10": "#e4f5ff",
"blue20": "#c7ebff",
"blue30": "#a6dcff",
"blue40": "#83c9ff",
"blue50": "#60b4ff",
"blue60": "#3d9df3",
"blue70": "#1c83e1",
"blue80": "#0068c9",
"blue90": "#0054a3",
"blue100": "#004280",
"purple10": "#f5ebff",
"purple20": "#ebd6ff",
"purple30": "#dbbbff",
"purple40": "#c89dff",
"purple50": "#b27eff",
"purple60": "#9a5dff",
"purple70": "#803df5",
"purple80": "#6d3fc0",
"purple90": "#583f84",
"purple100": "#3f3163",
"bgColor": "#ffffff",
"secondaryBg": "#f0f2f6",
"bodyText": "#31333F",
"warning": "#926C05",
"warningBg": "rgba(255, 227, 18, 0.1)",
"success": "#177233",
"successBg": "rgba(33, 195, 84, 0.1)",
"infoBg": "rgba(28, 131, 225, 0.1)",
"info": "#004280",
"danger": "#7d353b",
"dangerBg": "rgba(255, 43, 43, 0.09)",
"primary": "#ff4b4b",
"disabled": "#d5dae5",
"lightestGray": "#f0f2f6",
"lightGray": "#e6eaf1",
"gray": "#a3a8b8",
"darkGray": "#808495",
"red": "#ff2b2b",
"blue": "#0068c9",
"green": "#09ab3b",
"yellow": "#faca2b",
"linkText": "#0068c9",
"fadedText05": "rgba(49, 51, 63, 0.1)",
"fadedText10": "rgba(49, 51, 63, 0.2)",
"fadedText20": "rgba(49, 51, 63, 0.3)",
"fadedText40": "rgba(49, 51, 63, 0.4)",
"fadedText60": "rgba(49, 51, 63, 0.6)",
"bgMix": "rgba(248, 249, 251, 1)",
"darkenedBgMix100": "hsla(220, 27%, 68%, 1)",
"darkenedBgMix25": "rgba(151, 166, 195, 0.25)",
"darkenedBgMix15": "rgba(151, 166, 195, 0.15)",
"lightenedBg05": "hsla(0, 0%, 100%, 1)",
"borderColor": "rgba(49, 51, 63, 0.2)",
"borderColorLight": "rgba(49, 51, 63, 0.1)",
"codeTextColor": "#09ab3b",
"codeHighlightColor": "rgba(248, 249, 251, 1)",
"metricPositiveDeltaColor": "#09ab3b",
"metricNegativeDeltaColor": "#ff2b2b",
"metricNeutralDeltaColor": "rgba(49, 51, 63, 0.6)",
"docStringModuleText": "#31333F",
"docStringTypeText": "#21c354",
"docStringContainerBackground": "rgba(240, 242, 246, 0.4)",
"headingColor": "#31333F",
"navTextColor": "#555867",
"navActiveTextColor": "#262730",
"navIconColor": "#a3a8b8",
"sidebarControlColor": "#808495"
},
"fonts": {
"sansSerif": "\"Source Sans Pro\", sans-serif",
"monospace": "\"Source Code Pro\", monospace",
"serif": "\"Source Serif Pro\", serif",
"materialIcons": "Material Symbols Rounded"
},
"fontSizes": {
"twoSm": "12px",
"sm": "14px",
"md": "1rem",
"mdLg": "1.125rem",
"lg": "1.25rem",
"xl": "1.5rem",
"twoXL": "1.75rem",
"threeXL": "2.25rem",
"fourXL": "2.75rem",
"twoSmPx": 12,
"smPx": 14,
"mdPx": 16
},
"fontWeights": {
"normal": 400,
"bold": 600,
"extrabold": 700
},
"genericFonts": {
"bodyFont": "\"Source Sans Pro\", sans-serif",
"codeFont": "\"Source Code Pro\", monospace",
"headingFont": "\"Source Sans Pro\", sans-serif",
"iconFont": "Material Symbols Rounded"
},
"iconSizes": {
"xs": "0.5rem",
"sm": "0.75rem",
"md": "0.9rem",
"base": "1rem",
"lg": "1.25rem",
"xl": "1.5rem",
"twoXL": "1.8rem",
"threeXL": "2.3rem"
},
"lineHeights": {
"none": 1,
"headings": 1.2,
"tight": 1.25,
"inputWidget": 1.4,
"small": 1.5,
"base": 1.6,
"menuItem": 2
},
"radii": {
"md": "0.25rem",
"default": "0.5rem",
"xl": "0.75rem",
"xxl": "1rem",
"full": "9999px"
},
"sizes": {
"full": "100%",
"headerHeight": "3.75rem",
"fullScreenHeaderHeight": "2.875rem",
"sidebarTopSpace": "6rem",
"toastWidth": "21rem",
"contentMaxWidth": "46rem",
"maxChartTooltipWidth": "30rem",
"checkbox": "1rem",
"borderWidth": "1px",
"smallElementHeight": "1.5rem",
"minElementHeight": "2.5rem",
"largestElementHeight": "4.25rem",
"smallLogoHeight": "1.25rem",
"defaultLogoHeight": "1.5rem",
"largeLogoHeight": "2rem",
"sliderThumb": "0.75rem",
"wideSidePadding": "5rem",
"headerDecorationHeight": "0.125rem",
"appRunningMen": "1.6rem",
"appStatusMaxWidth": "20rem",
"spinnerSize": "1.375rem",
"spinnerThickness": "0.2rem",
"tabHeight": "2.5rem",
"minPopupWidth": "20rem",
"maxTooltipHeight": "18.75rem",
"chatAvatarSize": "2rem",
"clearIconSize": "1.5em",
"numberInputControlsWidth": "2rem",
"emptyDropdownHeight": "5.625rem",
"dropdownItemHeight": "2.5rem",
"maxDropdownHeight": "18.75rem",
"appDefaultBottomPadding": "3.5rem"
},
"spacing": {
"px": "1px",
"none": "0",
"threeXS": "0.125rem",
"twoXS": "0.25rem",
"xs": "0.375rem",
"sm": "0.5rem",
"md": "0.75rem",
"lg": "1rem",
"xl": "1.25rem",
"twoXL": "1.5rem",
"threeXL": "2rem",
"fourXL": "4rem"
},
"zIndices": {
"hide": -1,
"auto": "auto",
"base": 0,
"priority": 1,
"sidebar": 100,
"menuButton": 110,
"balloons": 1000000,
"header": 999990,
"sidebarMobile": 999995,
"popupMenu": 1000040,
"fullscreenWrapper": 1000050,
"tablePortal": 1000110,
"bottom": 99,
"cacheSpinner": 101,
"toast": 100,
"vegaTooltips": 1000060
}
},
"basewebTheme": {
"animation": {
"timing100": "100ms",
"timing200": "200ms",
"timing300": "300ms",
"timing400": "400ms",
"timing500": "500ms",
"timing600": "600ms",
"timing700": "700ms",
"timing800": "800ms",
"timing900": "900ms",
"timing1000": "1000ms",
"easeInCurve": "cubic-bezier(.8, .2, .6, 1)",
"easeOutCurve": "cubic-bezier(.2, .8, .4, 1)",
"easeInOutCurve": "cubic-bezier(0.4, 0, 0.2, 1)",
"easeInQuinticCurve": "cubic-bezier(0.755, 0.05, 0.855, 0.06)",
"easeOutQuinticCurve": "cubic-bezier(0.23, 1, 0.32, 1)",
"easeInOutQuinticCurve": "cubic-bezier(0.86, 0, 0.07, 1)",
"linearCurve": "cubic-bezier(0, 0, 1, 1)"
},
"borders": {
"border100": {
"borderColor": "hsla(0, 0%, 0%, 0.04)",
"borderStyle": "solid",
"borderWidth": "1px"
},
"border200": {
"borderColor": "hsla(0, 0%, 0%, 0.08)",
"borderStyle": "solid",
"borderWidth": "1px"
},
"border300": {
"borderColor": "hsla(0, 0%, 0%, 0.12)",
"borderStyle": "solid",
"borderWidth": "1px"
},
"border400": {
"borderColor": "hsla(0, 0%, 0%, 0.16)",
"borderStyle": "solid",
"borderWidth": "1px"
},
"border500": {
"borderColor": "hsla(0, 0%, 0%, 0.2)",
"borderStyle": "solid",
"borderWidth": "1px"
},
"border600": {
"borderColor": "hsla(0, 0%, 0%, 0.24)",
"borderStyle": "solid",
"borderWidth": "1px"
},
"radius100": "0.5rem",
"radius200": "0.5rem",
"radius300": "0.5rem",
"radius400": "0.5rem",
"radius500": "0.5rem",
"useRoundedCorners": true,
"buttonBorderRadiusMini": "0.25rem",
"buttonBorderRadius": "0.5rem",
"checkboxBorderRadius": "0.25rem",
"inputBorderRadiusMini": "0.25rem",
"inputBorderRadius": "0.5rem",
"popoverBorderRadius": "0.5rem",
"surfaceBorderRadius": "0.5rem",
"tagBorderRadius": "0.25rem"
},
"breakpoints": {
"small": 320,
"medium": 600,
"large": 1136
},
"colors": {
"primaryA": "#ff4b4b",
"primaryB": "#FFFFFF",
"primary": "#ff4b4b",
"primary50": "#F6F6F6",
"primary100": "#ff4b4b",
"primary200": "#ff4b4b",
"primary300": "#ff4b4b",
"primary400": "#ff4b4b",
"primary500": "#ff4b4b",
"primary600": "#ff4b4b",
"primary700": "#ff4b4b",
"accent": "rgba(255, 75, 75, 0.5)",
"accent50": "#EFF3FE",
"accent100": "#D4E2FC",
"accent200": "#A0BFF8",
"accent300": "#5B91F5",
"accent400": "#276EF1",
"accent500": "#1E54B7",
"accent600": "#174291",
"accent700": "#102C60",
"negative": "#E11900",
"negative50": "#FFEFED",
"negative100": "#FED7D2",
"negative200": "#F1998E",
"negative300": "#E85C4A",
"negative400": "#E11900",
"negative500": "#AB1300",
"negative600": "#870F00",
"negative700": "#5A0A00",
"warning": "#FFC043",
"warning50": "#FFFAF0",
"warning100": "#FFF2D9",
"warning200": "#FFE3AC",
"warning300": "#FFCF70",
"warning400": "#FFC043",
"warning500": "#BC8B2C",
"warning600": "#996F00",
"warning700": "#674D1B",
"positive": "#03703C",
"positive50": "#E6F2ED",
"positive100": "#ADDEC9",
"positive200": "#66D19E",
"positive300": "#06C167",
"positive400": "#048848",
"positive500": "#03703C",
"positive600": "#03582F",
"positive700": "#10462D",
"white": "#ffffff",
"black": "#000000",
"mono100": "#ffffff",
"mono200": "#f0f2f6",
"mono300": "#e6eaf1",
"mono400": "#e6eaf1",
"mono500": "#a3a8b8",
"mono600": "rgba(49, 51, 63, 0.4)",
"mono700": "#a3a8b8",
"mono800": "#31333F",
"mono900": "#31333F",
"mono1000": "#000000",
"ratingInactiveFill": "#EEEEEE",
"ratingStroke": "#CBCBCB",
"bannerActionLowInfo": "#D4E2FC",
"bannerActionLowNegative": "#FED7D2",
"bannerActionLowPositive": "#ADDEC9",
"bannerActionLowWarning": "#FFE3AC",
"bannerActionHighInfo": "#1E54B7",
"bannerActionHighNegative": "#AB1300",
"bannerActionHighPositive": "#03703C",
"bannerActionHighWarning": "#FFE3AC",
"buttonPrimaryFill": "#000000",
"buttonPrimaryText": "#FFFFFF",
"buttonPrimaryHover": "#ff4b4b",
"buttonPrimaryActive": "#ff4b4b",
"buttonPrimarySelectedFill": "#ff4b4b",
"buttonPrimarySelectedText": "#FFFFFF",
"buttonPrimarySpinnerForeground": "#276EF1",
"buttonPrimarySpinnerBackground": "#FFFFFF",
"buttonSecondaryFill": "#ff4b4b",
"buttonSecondaryText": "#000000",
"buttonSecondaryHover": "#ff4b4b",
"buttonSecondaryActive": "#ff4b4b",
"buttonSecondarySelectedFill": "#ff4b4b",
"buttonSecondarySelectedText": "#000000",
"buttonSecondarySpinnerForeground": "#276EF1",
"buttonSecondarySpinnerBackground": "#FFFFFF",
"buttonTertiaryFill": "transparent",
"buttonTertiaryText": "#000000",
"buttonTertiaryHover": "#F6F6F6",
"buttonTertiaryActive": "#ff4b4b",
"buttonTertiarySelectedFill": "#ff4b4b",
"buttonTertiarySelectedText": "#000000",
"buttonTertiaryDisabledActiveFill": "#F6F6F6",
"buttonTertiaryDisabledActiveText": "rgba(49, 51, 63, 0.4)",
"buttonTertiarySpinnerForeground": "#276EF1",
"buttonTertiarySpinnerBackground": "#ff4b4b",
"buttonDisabledFill": "hsla(0, 0%, 100%, 1)",
"buttonDisabledText": "rgba(49, 51, 63, 0.4)",
"buttonDisabledActiveFill": "#a3a8b8",
"buttonDisabledActiveText": "#ffffff",
"buttonDisabledSpinnerForeground": "rgba(49, 51, 63, 0.4)",
"buttonDisabledSpinnerBackground": "#d5dae5",
"breadcrumbsText": "#000000",
"breadcrumbsSeparatorFill": "#a3a8b8",
"calendarBackground": "#ffffff",
"calendarForeground": "#31333F",
"calendarForegroundDisabled": "#a3a8b8",
"calendarHeaderBackground": "#f0f2f6",
"calendarHeaderForeground": "#31333F",
"calendarHeaderBackgroundActive": "#f0f2f6",
"calendarHeaderForegroundDisabled": "#d5dae5",
"calendarDayForegroundPseudoSelected": "#31333F",
"calendarDayBackgroundPseudoSelectedHighlighted": "#ff4b4b",
"calendarDayForegroundPseudoSelectedHighlighted": "#31333F",
"calendarDayBackgroundSelected": "#ff4b4b",
"calendarDayForegroundSelected": "#ffffff",
"calendarDayBackgroundSelectedHighlighted": "#ff4b4b",
"calendarDayForegroundSelectedHighlighted": "#ffffff",
"comboboxListItemFocus": "#f0f2f6",
"comboboxListItemHover": "#e6eaf1",
"fileUploaderBackgroundColor": "#f0f2f6",
"fileUploaderBackgroundColorActive": "#F6F6F6",
"fileUploaderBorderColorActive": "#000000",
"fileUploaderBorderColorDefault": "#a3a8b8",
"fileUploaderMessageColor": "#31333F",
"linkText": "#000000",
"linkVisited": "#ff4b4b",
"linkHover": "#ff4b4b",
"linkActive": "#ff4b4b",
"listHeaderFill": "#FFFFFF",
"listBodyFill": "#FFFFFF",
"progressStepsCompletedText": "#FFFFFF",
"progressStepsCompletedFill": "#000000",
"progressStepsActiveText": "#FFFFFF",
"progressStepsActiveFill": "#000000",
"toggleFill": "#FFFFFF",
"toggleFillChecked": "#000000",
"toggleFillDisabled": "rgba(49, 51, 63, 0.4)",
"toggleTrackFill": "#d5dae5",
"toggleTrackFillDisabled": "#f0f2f6",
"tickFill": "hsla(0, 0%, 100%, 1)",
"tickFillHover": "#f0f2f6",
"tickFillActive": "#f0f2f6",
"tickFillSelected": "#ff4b4b",
"tickFillSelectedHover": "#ff4b4b",
"tickFillSelectedHoverActive": "#ff4b4b",
"tickFillError": "#FFEFED",
"tickFillErrorHover": "#FED7D2",
"tickFillErrorHoverActive": "#F1998E",
"tickFillErrorSelected": "#E11900",
"tickFillErrorSelectedHover": "#AB1300",
"tickFillErrorSelectedHoverActive": "#870F00",
"tickFillDisabled": "rgba(49, 51, 63, 0.4)",
"tickBorder": "#a3a8b8",
"tickBorderError": "#E11900",
"tickMarkFill": "#f0f2f6",
"tickMarkFillError": "#FFFFFF",
"tickMarkFillDisabled": "hsla(0, 0%, 100%, 1)",
"sliderTrackFill": "#d5dae5",
"sliderHandleFill": "#000000",
"sliderHandleFillDisabled": "#ff4b4b",
"sliderHandleInnerFill": "#d5dae5",
"sliderTrackFillHover": "#a3a8b8",
"sliderTrackFillActive": "rgba(49, 51, 63, 0.4)",
"sliderTrackFillDisabled": "#f0f2f6",
"sliderHandleInnerFillDisabled": "#d5dae5",
"sliderHandleInnerFillSelectedHover": "#000000",
"sliderHandleInnerFillSelectedActive": "#ff4b4b",
"inputBorder": "#f0f2f6",
"inputFill": "#f0f2f6",
"inputFillError": "#FFEFED",
"inputFillDisabled": "#f0f2f6",
"inputFillActive": "#f0f2f6",
"inputFillPositive": "#E6F2ED",
"inputTextDisabled": "rgba(49, 51, 63, 0.4)",
"inputBorderError": "#F1998E",
"inputBorderPositive": "#66D19E",
"inputEnhancerFill": "#e6eaf1",
"inputEnhancerFillDisabled": "#f0f2f6",
"inputEnhancerTextDisabled": "rgba(49, 51, 63, 0.4)",
"inputPlaceholder": "rgba(49, 51, 63, 0.6)",
"inputPlaceholderDisabled": "rgba(49, 51, 63, 0.4)",
"menuFill": "#ffffff",
"menuFillHover": "#f0f2f6",
"menuFontDefault": "#31333F",
"menuFontDisabled": "#a3a8b8",
"menuFontHighlighted": "#31333F",
"menuFontSelected": "#31333F",
"modalCloseColor": "#31333F",
"modalCloseColorHover": "#31333F",
"modalCloseColorFocus": "#31333F",
"tabBarFill": "#f0f2f6",
"tabColor": "#31333F",
"notificationInfoBackground": "rgba(28, 131, 225, 0.1)",
"notificationInfoText": "#004280",
"notificationPositiveBackground": "rgba(33, 195, 84, 0.1)",
"notificationPositiveText": "#177233",
"notificationWarningBackground": "rgba(255, 227, 18, 0.1)",
"notificationWarningText": "#926C05",
"notificationNegativeBackground": "rgba(255, 43, 43, 0.09)",
"notificationNegativeText": "#7d353b",
"tagFontDisabledRampUnit": "100",
"tagSolidFontRampUnit": "0",
"tagSolidRampUnit": "400",
"tagOutlinedFontRampUnit": "400",
"tagOutlinedRampUnit": "200",
"tagSolidHoverRampUnit": "50",
"tagSolidActiveRampUnit": "100",
"tagSolidDisabledRampUnit": "50",
"tagSolidFontHoverRampUnit": "500",
"tagLightRampUnit": "50",
"tagLightHoverRampUnit": "100",
"tagLightActiveRampUnit": "100",
"tagLightFontRampUnit": "500",
"tagLightFontHoverRampUnit": "500",
"tagOutlinedHoverRampUnit": "50",
"tagOutlinedActiveRampUnit": "0",
"tagOutlinedFontHoverRampUnit": "400",
"tagNeutralFontDisabled": "rgba(49, 51, 63, 0.4)",
"tagNeutralOutlinedDisabled": "#e6eaf1",
"tagNeutralSolidFont": "#FFFFFF",
"tagNeutralSolidBackground": "#000000",
"tagNeutralOutlinedBackground": "rgba(49, 51, 63, 0.4)",
"tagNeutralOutlinedFont": "#000000",
"tagNeutralSolidHover": "#e6eaf1",
"tagNeutralSolidActive": "#e6eaf1",
"tagNeutralSolidDisabled": "#f0f2f6",
"tagNeutralSolidFontHover": "#31333F",
"tagNeutralLightBackground": "#e6eaf1",
"tagNeutralLightHover": "#e6eaf1",
"tagNeutralLightActive": "#e6eaf1",
"tagNeutralLightDisabled": "#f0f2f6",
"tagNeutralLightFont": "#31333F",
"tagNeutralLightFontHover": "#31333F",
"tagNeutralOutlinedActive": "#31333F",
"tagNeutralOutlinedFontHover": "#31333F",
"tagNeutralOutlinedHover": "rgba(0, 0, 0, 0.08)",
"tagPrimaryFontDisabled": "rgba(49, 51, 63, 0.4)",
"tagPrimaryOutlinedDisabled": "transparent",
"tagPrimarySolidFont": "#FFFFFF",
"tagPrimarySolidBackground": "#ff4b4b",
"tagPrimaryOutlinedFontHover": "#000000",
"tagPrimaryOutlinedFont": "#000000",
"tagPrimarySolidHover": "#ff4b4b",
"tagPrimarySolidActive": "#ff4b4b",
"tagPrimarySolidDisabled": "#F6F6F6",
"tagPrimarySolidFontHover": "#ff4b4b",
"tagPrimaryLightBackground": "#F6F6F6",
"tagPrimaryLightHover": "#ff4b4b",
"tagPrimaryLightActive": "#ff4b4b",
"tagPrimaryLightDisabled": "#F6F6F6",
"tagPrimaryLightFont": "#ff4b4b",
"tagPrimaryLightFontHover": "#ff4b4b",
"tagPrimaryOutlinedActive": "#ff4b4b",
"tagPrimaryOutlinedHover": "rgba(0, 0, 0, 0.08)",
"tagPrimaryOutlinedBackground": "#ff4b4b",
"tagAccentFontDisabled": "#A0BFF8",
"tagAccentOutlinedDisabled": "#A0BFF8",
"tagAccentSolidFont": "#FFFFFF",
"tagAccentSolidBackground": "#276EF1",
"tagAccentOutlinedBackground": "#A0BFF8",
"tagAccentOutlinedFont": "#276EF1",
"tagAccentSolidHover": "#EFF3FE",
"tagAccentSolidActive": "#D4E2FC",
"tagAccentSolidDisabled": "#EFF3FE",
"tagAccentSolidFontHover": "#1E54B7",
"tagAccentLightBackground": "#EFF3FE",
"tagAccentLightHover": "#D4E2FC",
"tagAccentLightActive": "#D4E2FC",
"tagAccentLightDisabled": "#EFF3FE",
"tagAccentLightFont": "#1E54B7",
"tagAccentLightFontHover": "#1E54B7",
"tagAccentOutlinedActive": "#174291",
"tagAccentOutlinedFontHover": "#276EF1",
"tagAccentOutlinedHover": "rgba(0, 0, 0, 0.08)",
"tagPositiveFontDisabled": "#66D19E",
"tagPositiveOutlinedDisabled": "#66D19E",
"tagPositiveSolidFont": "#FFFFFF",
"tagPositiveSolidBackground": "#048848",
"tagPositiveOutlinedBackground": "#66D19E",
"tagPositiveOutlinedFont": "#048848",
"tagPositiveSolidHover": "#E6F2ED",
"tagPositiveSolidActive": "#ADDEC9",
"tagPositiveSolidDisabled": "#E6F2ED",
"tagPositiveSolidFontHover": "#03703C",
"tagPositiveLightBackground": "#E6F2ED",
"tagPositiveLightHover": "#ADDEC9",
"tagPositiveLightActive": "#ADDEC9",
"tagPositiveLightDisabled": "#E6F2ED",
"tagPositiveLightFont": "#03703C",
"tagPositiveLightFontHover": "#03703C",
"tagPositiveOutlinedActive": "#03582F",
"tagPositiveOutlinedFontHover": "#048848",
"tagPositiveOutlinedHover": "rgba(0, 0, 0, 0.08)",
"tagWarningFontDisabled": "#FFCF70",
"tagWarningOutlinedDisabled": "#FFCF70",
"tagWarningSolidFont": "#674D1B",
"tagWarningSolidBackground": "#FFC043",
"tagWarningOutlinedBackground": "#FFCF70",
"tagWarningOutlinedFont": "#996F00",
"tagWarningSolidHover": "#FFFAF0",
"tagWarningSolidActive": "#FFF2D9",
"tagWarningSolidDisabled": "#FFFAF0",
"tagWarningSolidFontHover": "#BC8B2C",
"tagWarningLightBackground": "#FFFAF0",
"tagWarningLightHover": "#FFF2D9",
"tagWarningLightActive": "#FFF2D9",
"tagWarningLightDisabled": "#FFFAF0",
"tagWarningLightFont": "#BC8B2C",
"tagWarningLightFontHover": "#BC8B2C",
"tagWarningOutlinedActive": "#996F00",
"tagWarningOutlinedFontHover": "#996F00",
"tagWarningOutlinedHover": "rgba(0, 0, 0, 0.08)",
"tagNegativeFontDisabled": "#F1998E",
"tagNegativeOutlinedDisabled": "#F1998E",
"tagNegativeSolidFont": "#FFFFFF",
"tagNegativeSolidBackground": "#E11900",
"tagNegativeOutlinedBackground": "#F1998E",
"tagNegativeOutlinedFont": "#E11900",
"tagNegativeSolidHover": "#FFEFED",
"tagNegativeSolidActive": "#FED7D2",
"tagNegativeSolidDisabled": "#FFEFED",
"tagNegativeSolidFontHover": "#AB1300",
"tagNegativeLightBackground": "#FFEFED",
"tagNegativeLightHover": "#FED7D2",
"tagNegativeLightActive": "#FED7D2",
"tagNegativeLightDisabled": "#FFEFED",
"tagNegativeLightFont": "#AB1300",
"tagNegativeLightFontHover": "#AB1300",
"tagNegativeOutlinedActive": "#870F00",
"tagNegativeOutlinedFontHover": "#E11900",
"tagNegativeOutlinedHover": "rgba(0, 0, 0, 0.08)",
"tableHeadBackgroundColor": "#ffffff",
"tableBackground": "#ffffff",
"tableStripedBackground": "#f0f2f6",
"tableFilter": "rgba(49, 51, 63, 0.4)",
"tableFilterHeading": "#a3a8b8",
"tableFilterBackground": "#ffffff",
"tableFilterFooterBackground": "#f0f2f6",
"toastText": "#FFFFFF",
"toastPrimaryText": "#FFFFFF",
"toastInfoBackground": "#276EF1",
"toastInfoText": "#FFFFFF",
"toastPositiveBackground": "#048848",
"toastPositiveText": "#FFFFFF",
"toastWarningBackground": "#FFC043",
"toastWarningText": "#000000",
"toastNegativeBackground": "#E11900",
"toastNegativeText": "#FFFFFF",
"spinnerTrackFill": "#31333F",
"progressbarTrackFill": "#f0f2f6",
"tooltipBackground": "#31333F",
"tooltipText": "#ffffff",
"backgroundPrimary": "#ffffff",
"backgroundSecondary": "#f0f2f6",
"backgroundTertiary": "#ffffff",
"backgroundInversePrimary": "#000000",
"backgroundInverseSecondary": "#1F1F1F",
"contentPrimary": "#31333F",
"contentSecondary": "#545454",
"contentTertiary": "#6B6B6B",
"contentInversePrimary": "#FFFFFF",
"contentInverseSecondary": "#CBCBCB",
"contentInverseTertiary": "#AFAFAF",
"borderOpaque": "rgba(151, 166, 195, 0.25)",
"borderTransparent": "rgba(0, 0, 0, 0.08)",
"borderSelected": "#ff4b4b",
"borderInverseOpaque": "#333333",
"borderInverseTransparent": "rgba(255, 255, 255, 0.2)",
"borderInverseSelected": "#FFFFFF",
"backgroundStateDisabled": "#F6F6F6",
"backgroundOverlayDark": "rgba(0, 0, 0, 0.3)",
"backgroundOverlayLight": "rgba(0, 0, 0, 0.08)",
"backgroundOverlayArt": "rgba(0, 0, 0, 0.00)",
"backgroundAccent": "#276EF1",
"backgroundNegative": "#E11900",
"backgroundWarning": "#FFC043",
"backgroundPositive": "#048848",
"backgroundLightAccent": "#EFF3FE",
"backgroundLightNegative": "#FFEFED",
"backgroundLightWarning": "#FFFAF0",
"backgroundLightPositive": "#E6F2ED",
"backgroundAlwaysDark": "#000000",
"backgroundAlwaysLight": "#FFFFFF",
"contentStateDisabled": "#AFAFAF",
"contentAccent": "#276EF1",
"contentOnColor": "#FFFFFF",
"contentOnColorInverse": "#000000",
"contentNegative": "#E11900",
"contentWarning": "#996F00",
"contentPositive": "#048848",
"borderStateDisabled": "#F6F6F6",
"borderAccent": "#276EF1",
"borderAccentLight": "#A0BFF8",
"borderNegative": "#F1998E",
"borderWarning": "#FFE3AC",
"borderPositive": "#66D19E",
"safety": "#276EF1",
"eatsGreen400": "#048848",
"freightBlue400": "#0E1FC1",
"jumpRed400": "#E11900",
"rewardsTier1": "#276EF1",
"rewardsTier2": "#FFC043",
"rewardsTier3": "#8EA3AD",
"rewardsTier4": "#000000",
"membership": "#996F00",
"datepickerBackground": "#ffffff",
"inputEnhanceFill": "#f0f2f6"
},
"direction": "auto",
"grid": {
"columns": [
4,
8,
12
],
"gutters": [
16,
36,
36
],
"margins": [
16,
36,
64
],
"gaps": 0,
"unit": "px",
"maxWidth": 1280
},
"lighting": {
"shadow400": "0 1px 4px hsla(0, 0%, 0%, 0.16)",
"shadow500": "0 2px 8px hsla(0, 0%, 0%, 0.16)",
"shadow600": "0 4px 16px hsla(0, 0%, 0%, 0.16)",
"shadow700": "0 8px 24px hsla(0, 0%, 0%, 0.16)",
"overlay0": "inset 0 0 0 1000px hsla(0, 0%, 0%, 0)",
"overlay100": "inset 0 0 0 1000px hsla(0, 0%, 0%, 0.04)",
"overlay200": "inset 0 0 0 1000px hsla(0, 0%, 0%, 0.08)",
"overlay300": "inset 0 0 0 1000px hsla(0, 0%, 0%, 0.12)",
"overlay400": "inset 0 0 0 1000px hsla(0, 0%, 0%, 0.16)",
"overlay500": "inset 0 0 0 1000px hsla(0, 0%, 0%, 0.2)",
"overlay600": "inset 0 0 0 1000px hsla(0, 0%, 0%, 0.24)",
"shallowAbove": "0px -4px 16px rgba(0, 0, 0, 0.12)",
"shallowBelow": "0px 4px 16px rgba(0, 0, 0, 0.12)",
"deepAbove": "0px -16px 48px rgba(0, 0, 0, 0.22)",
"deepBelow": "0px 16px 48px rgba(0, 0, 0, 0.22)"
},
"mediaQuery": {
"small": "@media screen and (min-width: 320px)",
"medium": "@media screen and (min-width: 600px)",
"large": "@media screen and (min-width: 1136px)"
},
"sizing": {
"scale0": "2px",
"scale100": "4px",
"scale200": "6px",
"scale300": "8px",
"scale400": "10px",
"scale500": "12px",
"scale550": "14px",
"scale600": "16px",
"scale650": "18px",
"scale700": "20px",
"scale750": "22px",
"scale800": "24px",
"scale850": "28px",
"scale900": "32px",
"scale950": "36px",
"scale1000": "40px",
"scale1200": "48px",
"scale1400": "56px",
"scale1600": "64px",
"scale2400": "96px",
"scale3200": "128px",
"scale4800": "192px"
},
"typography": {
"font100": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "12px",
"fontWeight": "normal",
"lineHeight": "20px"
},
"font150": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "1rem",
"fontWeight": "normal",
"lineHeight": 1.6,
"fontSizeSm": "14px",
"lineHeightTight": 1.25
},
"font200": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "1rem",
"fontWeight": "normal",
"lineHeight": 1.6,
"fontSizeSm": "14px",
"lineHeightTight": 1.25
},
"font250": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "1rem",
"fontWeight": "normal",
"lineHeight": 1.6,
"fontSizeSm": "14px",
"lineHeightTight": 1.25
},
"font300": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "1rem",
"fontWeight": "normal",
"lineHeight": 1.6,
"fontSizeSm": "14px",
"lineHeightTight": 1.25
},
"font350": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "1rem",
"fontWeight": "normal",
"lineHeight": 1.6,
"fontSizeSm": "14px",
"lineHeightTight": 1.25
},
"font400": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "1rem",
"fontWeight": "normal",
"lineHeight": 1.6,
"fontSizeSm": "14px",
"lineHeightTight": 1.25
},
"font450": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "1rem",
"fontWeight": "normal",
"lineHeight": 1.6,
"fontSizeSm": "14px",
"lineHeightTight": 1.25
},
"font550": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "20px",
"fontWeight": 700,
"lineHeight": "28px"
},
"font650": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "24px",
"fontWeight": 700,
"lineHeight": "32px"
},
"font750": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "28px",
"fontWeight": 700,
"lineHeight": "36px"
},
"font850": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "32px",
"fontWeight": 700,
"lineHeight": "40px"
},
"font950": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "36px",
"fontWeight": 700,
"lineHeight": "44px"
},
"font1050": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "40px",
"fontWeight": 700,
"lineHeight": "52px"
},
"font1150": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "36px",
"fontWeight": 700,
"lineHeight": "44px"
},
"font1250": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "44px",
"fontWeight": 700,
"lineHeight": "52px"
},
"font1350": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "52px",
"fontWeight": 700,
"lineHeight": "64px"
},
"font1450": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "96px",
"fontWeight": 700,
"lineHeight": "112px"
},
"ParagraphXSmall": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "12px",
"fontWeight": "normal",
"lineHeight": "20px"
},
"ParagraphSmall": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "1rem",
"fontWeight": "normal",
"lineHeight": 1.6,
"fontSizeSm": "14px",
"lineHeightTight": 1.25
},
"ParagraphMedium": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "1rem",
"fontWeight": "normal",
"lineHeight": 1.6,
"fontSizeSm": "14px",
"lineHeightTight": 1.25
},
"ParagraphLarge": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "1rem",
"fontWeight": "normal",
"lineHeight": 1.6,
"fontSizeSm": "14px",
"lineHeightTight": 1.25
},
"LabelXSmall": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "1rem",
"fontWeight": "normal",
"lineHeight": 1.6,
"fontSizeSm": "14px",
"lineHeightTight": 1.25
},
"LabelSmall": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "1rem",
"fontWeight": "normal",
"lineHeight": 1.6,
"fontSizeSm": "14px",
"lineHeightTight": 1.25
},
"LabelMedium": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "1rem",
"fontWeight": "normal",
"lineHeight": 1.6,
"fontSizeSm": "14px",
"lineHeightTight": 1.25
},
"LabelLarge": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "1rem",
"fontWeight": "normal",
"lineHeight": 1.6,
"fontSizeSm": "14px",
"lineHeightTight": 1.25
},
"HeadingXSmall": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "20px",
"fontWeight": 700,
"lineHeight": "28px"
},
"HeadingSmall": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "24px",
"fontWeight": 700,
"lineHeight": "32px"
},
"HeadingMedium": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "28px",
"fontWeight": 700,
"lineHeight": "36px"
},
"HeadingLarge": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "32px",
"fontWeight": 700,
"lineHeight": "40px"
},
"HeadingXLarge": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "36px",
"fontWeight": 700,
"lineHeight": "44px"
},
"HeadingXXLarge": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "40px",
"fontWeight": 700,
"lineHeight": "52px"
},
"DisplayXSmall": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "36px",
"fontWeight": 700,
"lineHeight": "44px"
},
"DisplaySmall": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "44px",
"fontWeight": 700,
"lineHeight": "52px"
},
"DisplayMedium": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "52px",
"fontWeight": 700,
"lineHeight": "64px"
},
"DisplayLarge": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "96px",
"fontWeight": 700,
"lineHeight": "112px"
},
"MonoParagraphXSmall": {
"fontFamily": "\"Lucida Console\", Monaco, monospace",
"fontSize": "12px",
"fontWeight": "normal",
"lineHeight": "20px"
},
"MonoParagraphSmall": {
"fontFamily": "\"Lucida Console\", Monaco, monospace",
"fontSize": "14px",
"fontWeight": "normal",
"lineHeight": "20px"
},
"MonoParagraphMedium": {
"fontFamily": "\"Lucida Console\", Monaco, monospace",
"fontSize": "16px",
"fontWeight": "normal",
"lineHeight": "24px"
},
"MonoParagraphLarge": {
"fontFamily": "\"Lucida Console\", Monaco, monospace",
"fontSize": "18px",
"fontWeight": "normal",
"lineHeight": "28px"
},
"MonoLabelXSmall": {
"fontFamily": "\"Lucida Console\", Monaco, monospace",
"fontSize": "12px",
"fontWeight": 500,
"lineHeight": "16px"
},
"MonoLabelSmall": {
"fontFamily": "\"Lucida Console\", Monaco, monospace",
"fontSize": "14px",
"fontWeight": 500,
"lineHeight": "16px"
},
"MonoLabelMedium": {
"fontFamily": "\"Lucida Console\", Monaco, monospace",
"fontSize": "16px",
"fontWeight": 500,
"lineHeight": "20px"
},
"MonoLabelLarge": {
"fontFamily": "\"Lucida Console\", Monaco, monospace",
"fontSize": "18px",
"fontWeight": 500,
"lineHeight": "24px"
},
"MonoHeadingXSmall": {
"fontFamily": "\"Lucida Console\", Monaco, monospace",
"fontSize": "20px",
"fontWeight": 700,
"lineHeight": "28px"
},
"MonoHeadingSmall": {
"fontFamily": "\"Lucida Console\", Monaco, monospace",
"fontSize": "24px",
"fontWeight": 700,
"lineHeight": "32px"
},
"MonoHeadingMedium": {
"fontFamily": "\"Lucida Console\", Monaco, monospace",
"fontSize": "28px",
"fontWeight": 700,
"lineHeight": "36px"
},
"MonoHeadingLarge": {
"fontFamily": "\"Lucida Console\", Monaco, monospace",
"fontSize": "32px",
"fontWeight": 700,
"lineHeight": "40px"
},
"MonoHeadingXLarge": {
"fontFamily": "\"Lucida Console\", Monaco, monospace",
"fontSize": "36px",
"fontWeight": 700,
"lineHeight": "44px"
},
"MonoHeadingXXLarge": {
"fontFamily": "\"Lucida Console\", Monaco, monospace",
"fontSize": "40px",
"fontWeight": 700,
"lineHeight": "52px"
},
"MonoDisplayXSmall": {
"fontFamily": "\"Lucida Console\", Monaco, monospace",
"fontSize": "36px",
"fontWeight": 700,
"lineHeight": "44px"
},
"MonoDisplaySmall": {
"fontFamily": "\"Lucida Console\", Monaco, monospace",
"fontSize": "44px",
"fontWeight": 700,
"lineHeight": "52px"
},
"MonoDisplayMedium": {
"fontFamily": "\"Lucida Console\", Monaco, monospace",
"fontSize": "52px",
"fontWeight": 700,
"lineHeight": "64px"
},
"MonoDisplayLarge": {
"fontFamily": "\"Lucida Console\", Monaco, monospace",
"fontSize": "96px",
"fontWeight": 700,
"lineHeight": "112px"
},
"font460": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "1rem",
"fontSizeSm": "14px",
"fontWeight": "normal",
"lineHeight": 1.6,
"lineHeightTight": 1.25
},
"font470": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "1rem",
"fontSizeSm": "14px",
"fontWeight": "normal",
"lineHeight": 1.6,
"lineHeightTight": 1.25
},
"font500": {
"fontFamily": "\"Source Sans Pro\", sans-serif",
"fontSize": "1rem",
"fontSizeSm": "14px",
"fontWeight": "normal",
"lineHeight": 1.6,
"lineHeightTight": 1.25
},
"font600": {}
},
"zIndex": {
"modal": 2000
}
},
"primitives": {
"primaryA": "#000000",
"primaryB": "#FFFFFF",
"primary": "#000000",
"primary50": "#F6F6F6",
"primary100": "#EEEEEE",
"primary200": "#E2E2E2",
"primary300": "#CBCBCB",
"primary400": "#AFAFAF",
"primary500": "#6B6B6B",
"primary600": "#545454",
"primary700": "#333333",
"accent": "#276EF1",
"accent50": "#EFF3FE",
"accent100": "#D4E2FC",
"accent200": "#A0BFF8",
"accent300": "#5B91F5",
"accent400": "#276EF1",
"accent500": "#1E54B7",
"accent600": "#174291",
"accent700": "#102C60",
"negative": "#E11900",
"negative50": "#FFEFED",
"negative100": "#FED7D2",
"negative200": "#F1998E",
"negative300": "#E85C4A",
"negative400": "#E11900",
"negative500": "#AB1300",
"negative600": "#870F00",
"negative700": "#5A0A00",
"warning": "#FFC043",
"warning50": "#FFFAF0",
"warning100": "#FFF2D9",
"warning200": "#FFE3AC",
"warning300": "#FFCF70",
"warning400": "#FFC043",
"warning500": "#BC8B2C",
"warning600": "#996F00",
"warning700": "#674D1B",
"positive": "#03703C",
"positive50": "#E6F2ED",
"positive100": "#ADDEC9",
"positive200": "#66D19E",
"positive300": "#06C167",
"positive400": "#048848",
"positive500": "#03703C",
"positive600": "#03582F",
"positive700": "#10462D",
"white": "#FFFFFF",
"black": "#000000",
"mono100": "#FFFFFF",
"mono200": "#F6F6F6",
"mono300": "#EEEEEE",
"mono400": "#E2E2E2",
"mono500": "#CBCBCB",
"mono600": "#AFAFAF",
"mono700": "#6B6B6B",
"mono800": "#545454",
"mono900": "#333333",
"mono1000": "#000000",
"ratingInactiveFill": "#EEEEEE",
"ratingStroke": "#CBCBCB",
"primaryFontFamily": "system-ui, \"Helvetica Neue\", Helvetica, Arial, sans-serif"
}
}
```
Raw data
{
"_id": null,
"home_page": "https://github.com/quiradev/streamlit-plugins",
"name": "streamlit-component-theme-changer",
"maintainer": null,
"docs_url": null,
"requires_python": ">=3.10",
"maintainer_email": null,
"keywords": "streamlit, plugins, components, theme-changer",
"author": "Victor Quilon Ranera",
"author_email": "v.quilonr@gmail.com",
"download_url": "https://files.pythonhosted.org/packages/bc/b3/d1c45ab92af5ea19b9f28f3425fcfca978ed2b175d1c2dc7212c309816a6/streamlit_component_theme_changer-0.5.0.tar.gz",
"platform": null,
"description": "# Change Theme (on the Client Side!)\n\n## Code example\n```python\nimport streamlit as st\n\nfrom streamlit_plugins.components.theme_changer import st_theme_changer\n\nst.title(\"Theme Changer Component\")\nst.caption(\"Just push the button to change the theme! On the client side, of course.\")\n# specify the primary menu definition\nst_theme_changer()\n\nst_theme_changer(render_mode=\"pills\")\n```\n\n## Custom Config\n\nThe theme name can\u2019t be \"Dark\" or \"Light\" since those are reserved for the default Streamlit themes.\n\n### Editable custom theme app\n\n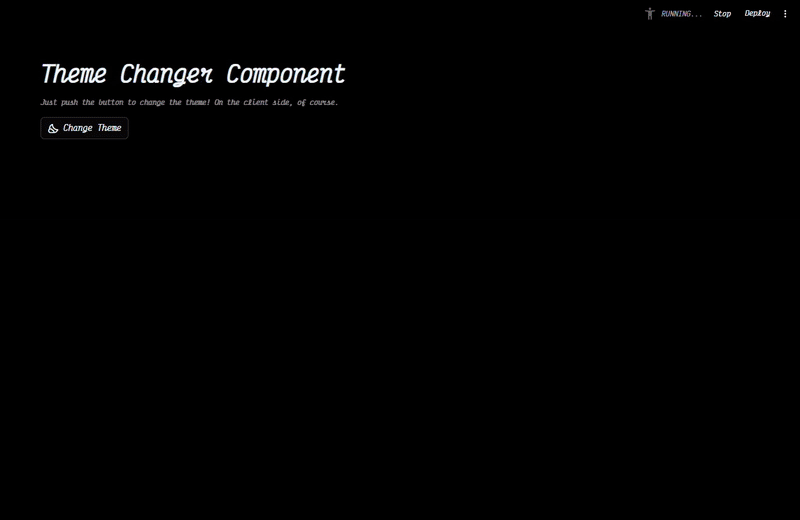\n\n```python\nimport streamlit as st\n\nfrom streamlit_plugins.components.theme_changer import get_active_theme_key, st_theme_changer\nfrom streamlit_plugins.components.theme_changer.entity import ThemeInfo, ThemeInput, ThemeBaseLight, ThemeBaseDark\n\n# make it look nice from the start\nst.set_page_config(layout='wide', initial_sidebar_state='collapsed')\n\nst.title(\"Theme Changer Component\")\nst.caption(\"Just push the button to change the theme! On the client side, of course.\")\n\n\n\ninit_theme_data = dict(\n soft_light=ThemeInput(\n name=\"Soft Sunrise\",\n icon=\":material/sunny_snowing:\",\n order=0,\n themeInfo=ThemeInfo(\n base=ThemeBaseLight.base,\n primaryColor=\"#6100FF\",\n backgroundColor=\"#EFF4FF\",\n secondaryBackgroundColor=\"#E7EEF5\",\n textColor=\"#000000\",\n widgetBackgroundColor=\"#F3F5F7\",\n widgetBorderColor=\"#9000FF\",\n skeletonBackgroundColor=\"#E0E0E0\",\n bodyFont=ThemeBaseLight.bodyFont,\n codeFont=ThemeBaseLight.codeFont,\n fontFaces=ThemeBaseLight.fontFaces,\n )\n ),\n soft_dark=ThemeInput(\n name=\"Dark Midnight\",\n icon=\":material/nights_stay:\",\n order=1,\n themeInfo=ThemeInfo(\n base=ThemeBaseDark.base,\n primaryColor=\"#7AF8FF\",\n backgroundColor=\"#000000\",\n secondaryBackgroundColor=\"#045367\",\n textColor=\"#f0f8ff\",\n widgetBackgroundColor=\"#092927\",\n widgetBorderColor=\"#75D9FF\",\n skeletonBackgroundColor=\"#365252\",\n bodyFont=ThemeBaseDark.bodyFont,\n codeFont=ThemeBaseDark.codeFont,\n fontFaces=ThemeBaseDark.fontFaces,\n )\n ),\n sepia=ThemeInput(\n name=\"Sepia\",\n icon=\":material/gradient:\",\n order=2,\n themeInfo=ThemeInfo(\n base=ThemeBaseLight.base,\n primaryColor=\"#FF0004\",\n backgroundColor=\"#F9F9E0\",\n secondaryBackgroundColor=\"#EFEFB4\",\n textColor=\"#000000\",\n widgetBackgroundColor=\"#F5F5D7\",\n widgetBorderColor=\"#E2DEAD\",\n skeletonBackgroundColor=\"#F5F5DC\",\n bodyFont=ThemeBaseLight.bodyFont,\n codeFont=ThemeBaseLight.codeFont,\n fontFaces=ThemeBaseLight.fontFaces,\n )\n )\n)\n\nif st.session_state.get(\"theme_data\") is None:\n st.session_state[\"theme_data\"] = init_theme_data\n\ntheme_data = st.session_state[\"theme_data\"]\n\nst_theme_changer(themes_data=theme_data, render_mode=\"init\", default_init_theme_name=\"soft_dark\")\nst_theme_changer(themes_data=theme_data, rerun_whole_st=True)\n\nwith st.expander(\"Theme Editor\", expanded=False):\n with st.container(border=False):\n theme_keys = list(theme_data.keys())\n tabs = st.tabs(theme_keys)\n for i, tab in enumerate(tabs):\n theme_key = theme_keys[i]\n with tab:\n with st.form(key=f\"{theme_key}_form\", border=False):\n col1, col2, col3 = st.columns(3)\n name = col1.text_input(\"Theme Label\", value=theme_data[theme_key].name, key=f\"{theme_key}_text_input\")\n icon = col2.text_input(\"Theme Icon\", value=theme_data[theme_key].icon, key=f\"{theme_key}_icon_input\")\n order = col3.number_input(\"Order\", value=theme_data[theme_key].order, key=f\"{theme_key}_order_input\")\n \n col1, col2, col3 = st.columns(3)\n primaryColor = col1.color_picker(\"Primary Color\", value=theme_data[theme_key].themeInfo.primaryColor, key=f\"{theme_key}_primary_color_input\")\n textColor = col2.color_picker(\"Text Color\", value=theme_data[theme_key].themeInfo.textColor, key=f\"{theme_key}_text_color_input\")\n \n col1, col2, col3 = st.columns(3)\n backgroundColor = col1.color_picker(\"Background Color\", value=theme_data[theme_key].themeInfo.backgroundColor, key=f\"{theme_key}_background_color_input\")\n secondaryBackgroundColor = col2.color_picker(\"Secondary Background Color\", value=theme_data[theme_key].themeInfo.secondaryBackgroundColor, key=f\"{theme_key}_secondary_background_color_input\")\n skeletonBackgroundColor = col3.color_picker(\"Skeleton Background Color\", value=theme_data[theme_key].themeInfo.skeletonBackgroundColor, key=f\"{theme_key}_skeleton_background_color_input\")\n \n col1, col2, col3 = st.columns(3)\n widgetBackgroundColor = col1.color_picker(\"Widget Background Color\", value=theme_data[theme_key].themeInfo.widgetBackgroundColor, key=f\"{theme_key}_widget_background_color_input\")\n widgetBorderColor = col2.color_picker(\"Widget Border Color\", value=theme_data[theme_key].themeInfo.widgetBorderColor, key=f\"{theme_key}_widget_border_color_input\")\n baseWidgetRadius = col3.number_input(\"Base Widget Radius\", value=theme_data[theme_key].themeInfo.radii[\"baseWidgetRadius\"], key=f\"{theme_key}_base_widget_radius_input\")\n\n col1, col2, col3 = st.columns(3)\n checkbox_radius = col1.number_input(\"Checkbox Radius\", value=theme_data[theme_key].themeInfo.radii[\"checkboxRadius\"], key=f\"{theme_key}_checkbox_radius_input\")\n col2.checkbox(\"Example Checkbox\", key=f\"{theme_key}_example_checkbox\")\n \n col1, col2, col3 = st.columns(3)\n tiny_font_size = col1.number_input(\"Tiny Font Size\", value=theme_data[theme_key].themeInfo.fontSizes[\"tinyFontSize\"], key=f\"{theme_key}_tiny_font_size_input\")\n small_font_size = col2.number_input(\"Small Font Size\", value=theme_data[theme_key].themeInfo.fontSizes[\"smallFontSize\"], key=f\"{theme_key}_small_font_size_input\")\n base_font_size = col3.number_input(\"Base Font Size\", value=theme_data[theme_key].themeInfo.fontSizes[\"baseFontSize\"], key=f\"{theme_key}_base_font_size_input\")\n\n col1, col2 = st.columns(2)\n bodyFont = col1.text_input(\"Body Font\", value=theme_data[theme_key].themeInfo.bodyFont, key=f\"{theme_key}_body_font_input\")\n codeFont = col2.text_input(\"Code Font\", value=theme_data[theme_key].themeInfo.codeFont, key=f\"{theme_key}_code_font_input\")\n # fontFaces = col3.text_area(\"Font Faces\", value=str(theme_data[theme_key].themeInfo.fontFaces), key=f\"{theme_key}_font_faces_input\")\n\n\n theme_data[theme_key].name = name\n theme_data[theme_key].icon = icon\n theme_data[theme_key].order = order\n theme_data[theme_key].themeInfo.primaryColor = primaryColor\n theme_data[theme_key].themeInfo.backgroundColor = backgroundColor\n theme_data[theme_key].themeInfo.secondaryBackgroundColor = secondaryBackgroundColor\n theme_data[theme_key].themeInfo.textColor = textColor\n theme_data[theme_key].themeInfo.widgetBackgroundColor = widgetBackgroundColor\n theme_data[theme_key].themeInfo.widgetBorderColor = widgetBorderColor\n theme_data[theme_key].themeInfo.skeletonBackgroundColor = skeletonBackgroundColor\n theme_data[theme_key].themeInfo.bodyFont = bodyFont\n theme_data[theme_key].themeInfo.codeFont = codeFont\n theme_data[theme_key].themeInfo.radii[\"baseWidgetRadius\"] = baseWidgetRadius\n theme_data[theme_key].themeInfo.fontSizes[\"tinyFontSize\"] = tiny_font_size\n theme_data[theme_key].themeInfo.fontSizes[\"smallFontSize\"] = small_font_size\n theme_data[theme_key].themeInfo.fontSizes[\"baseFontSize\"] = base_font_size\n \n update_theme = st.form_submit_button(\"Save\")\n if update_theme:\n st.session_state[\"theme_data\"] = theme_data\n if get_active_theme_key() == theme_key:\n st_theme_changer(themes_data=theme_data, render_mode=\"change\", default_init_theme_name=theme_key)\n st.rerun()\n\nwith st.sidebar:\n st_theme_changer(\n themes_data=theme_data, render_mode=\"pills\",\n rerun_whole_st=True, key=\"first_pills\"\n )\n\n```\n\n## All Theme Configurations (CSS Properties)\n\n### Theme Configuration Table\n\nHere\u2019s an overview of the theme configuration options and what they do:\n\n| **Key** | **Value** | **Description** |\n|-----------------------------|--------------------------------------------------------------------------------|---------------------------------------------------------------------------------------------------|\n| `primaryColor` | `\"#1A6CE7\"` | The main accent color used throughout your app. For example, widgets like `st.checkbox`, `st.slider`, and `st.text_input` (when focused) use this color. |\n| `backgroundColor` | `\"#FFFFFF\"` | The main background color of your app. |\n| `secondaryBackgroundColor` | `\"#F5F5F5\"` | Used as a secondary background color, like for the sidebar or interactive widgets. |\n| `textColor` | `\"#1A1D21\"` | Controls the text color for most elements in your app. |\n| `font` | `0` `1` `2` [`\"sans serif\" ` `\"serif\"` `\"monospace\"`] | Sets the font used in your app. Options are `\"sans serif\"`, `\"serif\"`, or `\"monospace\"`. Defaults to `\"sans serif\"` if unset. |\n| `base` | `0` `1` [`light` `dark`] | Lets you create custom themes by slightly modifying one of the preset Streamlit themes. |\n| *`widgetBackgroundColor` | `\"#FFFFFF\"` | Background color for widgets like inputs or sliders. |\n| *`widgetBorderColor` | `\"#D3DAE8\"` | Border color for widgets and interactive elements. |\n| *`skeletonBackgroundColor` | `\"#CCDDEE\"` | Background color for loading placeholders (skeletons). |\n| *`bodyFont` | `\"Inter\", \"Source Sans Pro\", sans-serif` | Default font for body text. |\n| *`codeFont` | `\"Apercu Mono\", \"Source Code Pro\", monospace` | Font used for code or monospaced text. |\n| *`fontFaces` | [Array of font families with URLs and weights](#font-faces-details-fontfaces-) | Custom fonts, including font-family, URL, and weight (e.g., Inter, Apercu Mono). |\n| *`radii.checkboxRadius` | `3` | Border radius for checkboxes. |\n| *`radii.baseWidgetRadius` | `6` | Border radius for widgets like buttons or inputs. |\n| *`fontSizes.tinyFontSize` | `10` | Font size for tiny text (e.g., labels). |\n| *`fontSizes.smallFontSize` | `12` | Font size for small text, like secondary descriptions. |\n| *`fontSizes.baseFontSize` | `14` | Base font size for regular text. |\n\n> The parameters marked with a * are new in Streamlit\u2019s configuration. You can tweak them to adjust fonts and font sizes. \n> Properties with a `.` in their names are part of nested objects. Details about these nested objects are provided below.\n\n### Font Faces Details `\"fontFaces\": [...]`\n\n| **`\"family\"`** | **`\"url\"`** | **`\"weight\"`** | **Description** |\n|------------------|------------------------------------------------------------------------------------------------|------------|-----------------------------------------|\n| `Inter` | `https://rsms.me/inter/font-files/Inter-Regular.woff2?v=3.19` | 400 | Regular weight of the Inter font. |\n| `Inter` | `https://rsms.me/inter/font-files/Inter-SemiBold.woff2?v=3.19` | 600 | Semi-bold weight of the Inter font. |\n| `Inter` | `https://rsms.me/inter/font-files/Inter-Bold.woff2?v=3.19` | 700 | Bold weight of the Inter font. |\n| `Apercu Mono` | `https://app.snowflake.com/static/2c4863733dec5a69523e.woff2` | 400 | Regular weight of the Apercu Mono font. |\n| `Apercu Mono` | `https://app.snowflake.com/static/e903ae189d31a97e231e.woff2` | 500 | Medium weight of the Apercu Mono font. |\n| `Apercu Mono` | `https://app.snowflake.com/static/32447307374154c88bc0.woff2` | 700 | Bold weight of the Apercu Mono font. |\n\n### Theme Configuration: Properties and Data Types\n\n### Enum Descriptions\n\n#### Font Family (`font`)\n- `0` (SANS_SERIF): Standard sans-serif font family. \n- `1` (SERIF): Standard serif font family. \n- `2` (MONOSPACE): Monospaced font family, often for code. \n\n#### Base Theme (`base`)\n- `0` (LIGHT): Light base theme. \n- `1` (DARK): Dark base theme. \n\n### Nested Object Details\n\n#### `radii`\n| **Sub-Property** | **Type** | **Description** |\n|-----------------------|----------|------------------------------------------------------|\n| `checkboxRadius` | `number` | Radius for checkboxes. |\n| `baseWidgetRadius` | `number` | Radius for widgets like buttons or inputs. |\n\n#### `fontSizes`\n| **Sub-Property** | **Type** | **Description** |\n|-----------------------|----------|------------------------------------------------------|\n| `tinyFontSize` | `number` | Font size for tiny text. |\n| `smallFontSize` | `number` | Font size for small text. |\n| `baseFontSize` | `number` | Default font size for regular text. |\n\n---\n\n### Workaround for Switching Themes Without Custom Components\n\nYou can use query parameters in the URL to switch between light and dark themes. However, this requires a page reload and won\u2019t allow custom themes. Examples:\n\n- `?embed_options=light_theme` \n- `?embed_options=dark_theme` \n\n---\n\n### Override Base \"Dark\" or \"Light\" Themes (Maybe in future \ud83e\udd1e0)\n\nCurrently, you can\u2019t override the default \"Dark\" or \"Light\" themes directly in Streamlit. Until the Streamlit team releases support for the `SET_CUSTOM_THEME_CONFIG` event, you\u2019re limited to modifying the available properties listed above. But for your understanding, here's the all properties that streamlit team can configurate the frontend styles for Dark and Light themes.\n\n```json\n{\n \"name\": \"Dark\",\n \"emotion\": {\n \"inSidebar\": false,\n \"breakpoints\": {\n \"hideWidgetDetails\": 180,\n \"hideNumberInputControls\": 120,\n \"sm\": \"576px\",\n \"columns\": \"640px\",\n \"md\": \"768px\"\n },\n \"colors\": {\n \"transparent\": \"transparent\",\n \"black\": \"#000000\",\n \"white\": \"#ffffff\",\n \"gray10\": \"#fafafa\",\n \"gray20\": \"#f0f2f6\",\n \"gray30\": \"#e6eaf1\",\n \"gray40\": \"#d5dae5\",\n \"gray50\": \"#bfc5d3\",\n \"gray60\": \"#a3a8b8\",\n \"gray70\": \"#808495\",\n \"gray80\": \"#555867\",\n \"gray85\": \"#31333F\",\n \"gray90\": \"#262730\",\n \"gray100\": \"#0e1117\",\n \"red10\": \"#fff0f0\",\n \"red20\": \"#ffdede\",\n \"red30\": \"#ffc7c7\",\n \"red40\": \"#ffabab\",\n \"red50\": \"#ff8c8c\",\n \"red60\": \"#ff6c6c\",\n \"red70\": \"#ff4b4b\",\n \"red80\": \"#ff2b2b\",\n \"red90\": \"#bd4043\",\n \"red100\": \"#7d353b\",\n \"orange10\": \"#fffae8\",\n \"orange20\": \"#fff6d0\",\n \"orange30\": \"#ffecb0\",\n \"orange40\": \"#ffe08e\",\n \"orange50\": \"#ffd16a\",\n \"orange60\": \"#ffbd45\",\n \"orange70\": \"#ffa421\",\n \"orange80\": \"#ff8700\",\n \"orange90\": \"#ed6f13\",\n \"orange100\": \"#d95a00\",\n \"yellow10\": \"#ffffe1\",\n \"yellow20\": \"#ffffc2\",\n \"yellow30\": \"#ffffa0\",\n \"yellow40\": \"#ffff7d\",\n \"yellow50\": \"#ffff59\",\n \"yellow60\": \"#fff835\",\n \"yellow70\": \"#ffe312\",\n \"yellow80\": \"#faca2b\",\n \"yellow90\": \"#edbb16\",\n \"yellow100\": \"#dea816\",\n \"yellow110\": \"#916e10\",\n \"green10\": \"#dffde9\",\n \"green20\": \"#c0fcd3\",\n \"green30\": \"#9ef6bb\",\n \"green40\": \"#7defa1\",\n \"green50\": \"#5ce488\",\n \"green60\": \"#3dd56d\",\n \"green70\": \"#21c354\",\n \"green80\": \"#09ab3b\",\n \"green90\": \"#158237\",\n \"green100\": \"#177233\",\n \"blueGreen10\": \"#dcfffb\",\n \"blueGreen20\": \"#bafff7\",\n \"blueGreen30\": \"#93ffee\",\n \"blueGreen40\": \"#6bfde3\",\n \"blueGreen50\": \"#45f4d5\",\n \"blueGreen60\": \"#20e7c5\",\n \"blueGreen70\": \"#00d4b1\",\n \"blueGreen80\": \"#29b09d\",\n \"blueGreen90\": \"#2c867c\",\n \"blueGreen100\": \"#246e69\",\n \"lightBlue10\": \"#e0feff\",\n \"lightBlue20\": \"#bffdff\",\n \"lightBlue30\": \"#9af8ff\",\n \"lightBlue40\": \"#73efff\",\n \"lightBlue50\": \"#4be4ff\",\n \"lightBlue60\": \"#24d4ff\",\n \"lightBlue70\": \"#00c0f2\",\n \"lightBlue80\": \"#00a4d4\",\n \"lightBlue90\": \"#0d8cb5\",\n \"lightBlue100\": \"#15799e\",\n \"blue10\": \"#e4f5ff\",\n \"blue20\": \"#c7ebff\",\n \"blue30\": \"#a6dcff\",\n \"blue40\": \"#83c9ff\",\n \"blue50\": \"#60b4ff\",\n \"blue60\": \"#3d9df3\",\n \"blue70\": \"#1c83e1\",\n \"blue80\": \"#0068c9\",\n \"blue90\": \"#0054a3\",\n \"blue100\": \"#004280\",\n \"purple10\": \"#f5ebff\",\n \"purple20\": \"#ebd6ff\",\n \"purple30\": \"#dbbbff\",\n \"purple40\": \"#c89dff\",\n \"purple50\": \"#b27eff\",\n \"purple60\": \"#9a5dff\",\n \"purple70\": \"#803df5\",\n \"purple80\": \"#6d3fc0\",\n \"purple90\": \"#583f84\",\n \"purple100\": \"#3f3163\",\n \"bgColor\": \"#0e1117\",\n \"secondaryBg\": \"#262730\",\n \"bodyText\": \"#fafafa\",\n \"warning\": \"#ffffc2\",\n \"warningBg\": \"rgba(255, 227, 18, 0.2)\",\n \"success\": \"#dffde9\",\n \"successBg\": \"rgba(61, 213, 109, 0.2)\",\n \"infoBg\": \"rgba(61, 157, 243, 0.2)\",\n \"info\": \"#c7ebff\",\n \"danger\": \"#ffdede\",\n \"dangerBg\": \"rgba(255, 108, 108, 0.2)\",\n \"primary\": \"#ff4b4b\",\n \"disabled\": \"#808495\",\n \"lightestGray\": \"#f0f2f6\",\n \"lightGray\": \"#e6eaf1\",\n \"gray\": \"#a3a8b8\",\n \"darkGray\": \"#808495\",\n \"red\": \"#ff2b2b\",\n \"blue\": \"#0068c9\",\n \"green\": \"#09ab3b\",\n \"yellow\": \"#faca2b\",\n \"linkText\": \"hsla(209, 100%, 59%, 1)\",\n \"fadedText05\": \"rgba(250, 250, 250, 0.1)\",\n \"fadedText10\": \"rgba(250, 250, 250, 0.2)\",\n \"fadedText20\": \"rgba(250, 250, 250, 0.3)\",\n \"fadedText40\": \"rgba(250, 250, 250, 0.4)\",\n \"fadedText60\": \"rgba(250, 250, 250, 0.6)\",\n \"bgMix\": \"rgba(26, 28, 36, 1)\",\n \"darkenedBgMix100\": \"hsla(228, 16%, 72%, 1)\",\n \"darkenedBgMix25\": \"rgba(172, 177, 195, 0.25)\",\n \"darkenedBgMix15\": \"rgba(172, 177, 195, 0.15)\",\n \"lightenedBg05\": \"hsla(220, 24%, 10%, 1)\",\n \"borderColor\": \"rgba(250, 250, 250, 0.2)\",\n \"borderColorLight\": \"rgba(250, 250, 250, 0.1)\",\n \"codeTextColor\": \"#09ab3b\",\n \"codeHighlightColor\": \"rgba(26, 28, 36, 1)\",\n \"metricPositiveDeltaColor\": \"#09ab3b\",\n \"metricNegativeDeltaColor\": \"#ff2b2b\",\n \"metricNeutralDeltaColor\": \"rgba(250, 250, 250, 0.6)\",\n \"docStringModuleText\": \"#fafafa\",\n \"docStringTypeText\": \"#21c354\",\n \"docStringContainerBackground\": \"rgba(38, 39, 48, 0.4)\",\n \"headingColor\": \"#fafafa\",\n \"navTextColor\": \"#bfc5d3\",\n \"navActiveTextColor\": \"#fafafa\",\n \"navIconColor\": \"#808495\",\n \"sidebarControlColor\": \"#fafafa\"\n },\n \"fonts\": {\n \"sansSerif\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"monospace\": \"\\\"Source Code Pro\\\", monospace\",\n \"serif\": \"\\\"Source Serif Pro\\\", serif\",\n \"materialIcons\": \"Material Symbols Rounded\"\n },\n \"fontSizes\": {\n \"twoSm\": \"12px\",\n \"sm\": \"14px\",\n \"md\": \"1rem\",\n \"mdLg\": \"1.125rem\",\n \"lg\": \"1.25rem\",\n \"xl\": \"1.5rem\",\n \"twoXL\": \"1.75rem\",\n \"threeXL\": \"2.25rem\",\n \"fourXL\": \"2.75rem\",\n \"twoSmPx\": 12,\n \"smPx\": 14,\n \"mdPx\": 16\n },\n \"fontWeights\": {\n \"normal\": 400,\n \"bold\": 600,\n \"extrabold\": 700\n },\n \"genericFonts\": {\n \"bodyFont\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"codeFont\": \"\\\"Source Code Pro\\\", monospace\",\n \"headingFont\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"iconFont\": \"Material Symbols Rounded\"\n },\n \"iconSizes\": {\n \"xs\": \"0.5rem\",\n \"sm\": \"0.75rem\",\n \"md\": \"0.9rem\",\n \"base\": \"1rem\",\n \"lg\": \"1.25rem\",\n \"xl\": \"1.5rem\",\n \"twoXL\": \"1.8rem\",\n \"threeXL\": \"2.3rem\"\n },\n \"lineHeights\": {\n \"none\": 1,\n \"headings\": 1.2,\n \"tight\": 1.25,\n \"inputWidget\": 1.4,\n \"small\": 1.5,\n \"base\": 1.6,\n \"menuItem\": 2\n },\n \"radii\": {\n \"md\": \"0.25rem\",\n \"default\": \"0.5rem\",\n \"xl\": \"0.75rem\",\n \"xxl\": \"1rem\",\n \"full\": \"9999px\"\n },\n \"sizes\": {\n \"full\": \"100%\",\n \"headerHeight\": \"3.75rem\",\n \"fullScreenHeaderHeight\": \"2.875rem\",\n \"sidebarTopSpace\": \"6rem\",\n \"toastWidth\": \"21rem\",\n \"contentMaxWidth\": \"46rem\",\n \"maxChartTooltipWidth\": \"30rem\",\n \"checkbox\": \"1rem\",\n \"borderWidth\": \"1px\",\n \"smallElementHeight\": \"1.5rem\",\n \"minElementHeight\": \"2.5rem\",\n \"largestElementHeight\": \"4.25rem\",\n \"smallLogoHeight\": \"1.25rem\",\n \"defaultLogoHeight\": \"1.5rem\",\n \"largeLogoHeight\": \"2rem\",\n \"sliderThumb\": \"0.75rem\",\n \"wideSidePadding\": \"5rem\",\n \"headerDecorationHeight\": \"0.125rem\",\n \"appRunningMen\": \"1.6rem\",\n \"appStatusMaxWidth\": \"20rem\",\n \"spinnerSize\": \"1.375rem\",\n \"spinnerThickness\": \"0.2rem\",\n \"tabHeight\": \"2.5rem\",\n \"minPopupWidth\": \"20rem\",\n \"maxTooltipHeight\": \"18.75rem\",\n \"chatAvatarSize\": \"2rem\",\n \"clearIconSize\": \"1.5em\",\n \"numberInputControlsWidth\": \"2rem\",\n \"emptyDropdownHeight\": \"5.625rem\",\n \"dropdownItemHeight\": \"2.5rem\",\n \"maxDropdownHeight\": \"18.75rem\",\n \"appDefaultBottomPadding\": \"3.5rem\"\n },\n \"spacing\": {\n \"px\": \"1px\",\n \"none\": \"0\",\n \"threeXS\": \"0.125rem\",\n \"twoXS\": \"0.25rem\",\n \"xs\": \"0.375rem\",\n \"sm\": \"0.5rem\",\n \"md\": \"0.75rem\",\n \"lg\": \"1rem\",\n \"xl\": \"1.25rem\",\n \"twoXL\": \"1.5rem\",\n \"threeXL\": \"2rem\",\n \"fourXL\": \"4rem\"\n },\n \"zIndices\": {\n \"hide\": -1,\n \"auto\": \"auto\",\n \"base\": 0,\n \"priority\": 1,\n \"sidebar\": 100,\n \"menuButton\": 110,\n \"balloons\": 1000000,\n \"header\": 999990,\n \"sidebarMobile\": 999995,\n \"popupMenu\": 1000040,\n \"fullscreenWrapper\": 1000050,\n \"tablePortal\": 1000110,\n \"bottom\": 99,\n \"cacheSpinner\": 101,\n \"toast\": 100,\n \"vegaTooltips\": 1000060\n }\n },\n \"basewebTheme\": {\n \"animation\": {\n \"timing100\": \"100ms\",\n \"timing200\": \"200ms\",\n \"timing300\": \"300ms\",\n \"timing400\": \"400ms\",\n \"timing500\": \"500ms\",\n \"timing600\": \"600ms\",\n \"timing700\": \"700ms\",\n \"timing800\": \"800ms\",\n \"timing900\": \"900ms\",\n \"timing1000\": \"1000ms\",\n \"easeInCurve\": \"cubic-bezier(.8, .2, .6, 1)\",\n \"easeOutCurve\": \"cubic-bezier(.2, .8, .4, 1)\",\n \"easeInOutCurve\": \"cubic-bezier(0.4, 0, 0.2, 1)\",\n \"easeInQuinticCurve\": \"cubic-bezier(0.755, 0.05, 0.855, 0.06)\",\n \"easeOutQuinticCurve\": \"cubic-bezier(0.23, 1, 0.32, 1)\",\n \"easeInOutQuinticCurve\": \"cubic-bezier(0.86, 0, 0.07, 1)\",\n \"linearCurve\": \"cubic-bezier(0, 0, 1, 1)\"\n },\n \"borders\": {\n \"border100\": {\n \"borderColor\": \"hsla(0, 0%, 0%, 0.04)\",\n \"borderStyle\": \"solid\",\n \"borderWidth\": \"1px\"\n },\n \"border200\": {\n \"borderColor\": \"hsla(0, 0%, 0%, 0.08)\",\n \"borderStyle\": \"solid\",\n \"borderWidth\": \"1px\"\n },\n \"border300\": {\n \"borderColor\": \"hsla(0, 0%, 0%, 0.12)\",\n \"borderStyle\": \"solid\",\n \"borderWidth\": \"1px\"\n },\n \"border400\": {\n \"borderColor\": \"hsla(0, 0%, 0%, 0.16)\",\n \"borderStyle\": \"solid\",\n \"borderWidth\": \"1px\"\n },\n \"border500\": {\n \"borderColor\": \"hsla(0, 0%, 0%, 0.2)\",\n \"borderStyle\": \"solid\",\n \"borderWidth\": \"1px\"\n },\n \"border600\": {\n \"borderColor\": \"hsla(0, 0%, 0%, 0.24)\",\n \"borderStyle\": \"solid\",\n \"borderWidth\": \"1px\"\n },\n \"radius100\": \"0.5rem\",\n \"radius200\": \"0.5rem\",\n \"radius300\": \"0.5rem\",\n \"radius400\": \"0.5rem\",\n \"radius500\": \"0.5rem\",\n \"useRoundedCorners\": true,\n \"buttonBorderRadiusMini\": \"0.25rem\",\n \"buttonBorderRadius\": \"0.5rem\",\n \"checkboxBorderRadius\": \"0.25rem\",\n \"inputBorderRadiusMini\": \"0.25rem\",\n \"inputBorderRadius\": \"0.5rem\",\n \"popoverBorderRadius\": \"0.5rem\",\n \"surfaceBorderRadius\": \"0.5rem\",\n \"tagBorderRadius\": \"0.25rem\"\n },\n \"breakpoints\": {\n \"small\": 320,\n \"medium\": 600,\n \"large\": 1136\n },\n \"colors\": {\n \"primaryA\": \"#ff4b4b\",\n \"primaryB\": \"#141414\",\n \"primary\": \"#ff4b4b\",\n \"primary50\": \"#F6F6F6\",\n \"primary100\": \"#ff4b4b\",\n \"primary200\": \"#ff4b4b\",\n \"primary300\": \"#ff4b4b\",\n \"primary400\": \"#ff4b4b\",\n \"primary500\": \"#ff4b4b\",\n \"primary600\": \"#ff4b4b\",\n \"primary700\": \"#ff4b4b\",\n \"accent\": \"rgba(255, 75, 75, 0.5)\",\n \"accent50\": \"#EFF3FE\",\n \"accent100\": \"#D4E2FC\",\n \"accent200\": \"#A0BFF8\",\n \"accent300\": \"#5B91F5\",\n \"accent400\": \"#276EF1\",\n \"accent500\": \"#1E54B7\",\n \"accent600\": \"#174291\",\n \"accent700\": \"#102C60\",\n \"negative\": \"#AB1300\",\n \"negative50\": \"#FFEFED\",\n \"negative100\": \"#FED7D2\",\n \"negative200\": \"#F1998E\",\n \"negative300\": \"#E85C4A\",\n \"negative400\": \"#E11900\",\n \"negative500\": \"#AB1300\",\n \"negative600\": \"#870F00\",\n \"negative700\": \"#5A0A00\",\n \"warning\": \"#BC8B2C\",\n \"warning50\": \"#FFFAF0\",\n \"warning100\": \"#FFF2D9\",\n \"warning200\": \"#FFE3AC\",\n \"warning300\": \"#FFCF70\",\n \"warning400\": \"#FFC043\",\n \"warning500\": \"#BC8B2C\",\n \"warning600\": \"#996F00\",\n \"warning700\": \"#674D1B\",\n \"positive\": \"#048848\",\n \"positive50\": \"#E6F2ED\",\n \"positive100\": \"#ADDEC9\",\n \"positive200\": \"#66D19E\",\n \"positive300\": \"#06C167\",\n \"positive400\": \"#048848\",\n \"positive500\": \"#03703C\",\n \"positive600\": \"#03582F\",\n \"positive700\": \"#10462D\",\n \"white\": \"#ffffff\",\n \"black\": \"#000000\",\n \"mono100\": \"#0e1117\",\n \"mono200\": \"#262730\",\n \"mono300\": \"#e6eaf1\",\n \"mono400\": \"#e6eaf1\",\n \"mono500\": \"#a3a8b8\",\n \"mono600\": \"rgba(250, 250, 250, 0.4)\",\n \"mono700\": \"#a3a8b8\",\n \"mono800\": \"#fafafa\",\n \"mono900\": \"#fafafa\",\n \"mono1000\": \"#000000\",\n \"ratingInactiveFill\": \"#6B6B6B\",\n \"ratingStroke\": \"#333333\",\n \"bannerActionLowInfo\": \"#D4E2FC\",\n \"bannerActionLowNegative\": \"#FED7D2\",\n \"bannerActionLowPositive\": \"#ADDEC9\",\n \"bannerActionLowWarning\": \"#FFE3AC\",\n \"bannerActionHighInfo\": \"#1E54B7\",\n \"bannerActionHighNegative\": \"#AB1300\",\n \"bannerActionHighPositive\": \"#03703C\",\n \"bannerActionHighWarning\": \"#FFE3AC\",\n \"buttonPrimaryFill\": \"#FFFFFF\",\n \"buttonPrimaryText\": \"#FFFFFF\",\n \"buttonPrimaryHover\": \"#ff4b4b\",\n \"buttonPrimaryActive\": \"#ff4b4b\",\n \"buttonPrimarySelectedFill\": \"#ff4b4b\",\n \"buttonPrimarySelectedText\": \"#FFFFFF\",\n \"buttonPrimarySpinnerForeground\": \"#276EF1\",\n \"buttonPrimarySpinnerBackground\": \"#141414\",\n \"buttonSecondaryFill\": \"#ff4b4b\",\n \"buttonSecondaryText\": \"#FFFFFF\",\n \"buttonSecondaryHover\": \"#ff4b4b\",\n \"buttonSecondaryActive\": \"#ff4b4b\",\n \"buttonSecondarySelectedFill\": \"#ff4b4b\",\n \"buttonSecondarySelectedText\": \"#FFFFFF\",\n \"buttonSecondarySpinnerForeground\": \"#276EF1\",\n \"buttonSecondarySpinnerBackground\": \"#141414\",\n \"buttonTertiaryFill\": \"transparent\",\n \"buttonTertiaryText\": \"#FFFFFF\",\n \"buttonTertiaryHover\": \"#F6F6F6\",\n \"buttonTertiaryActive\": \"#ff4b4b\",\n \"buttonTertiarySelectedFill\": \"#ff4b4b\",\n \"buttonTertiarySelectedText\": \"#FFFFFF\",\n \"buttonTertiaryDisabledActiveFill\": \"#F6F6F6\",\n \"buttonTertiaryDisabledActiveText\": \"rgba(250, 250, 250, 0.4)\",\n \"buttonTertiarySpinnerForeground\": \"#276EF1\",\n \"buttonTertiarySpinnerBackground\": \"#ff4b4b\",\n \"buttonDisabledFill\": \"hsla(220, 24%, 10%, 1)\",\n \"buttonDisabledText\": \"rgba(250, 250, 250, 0.4)\",\n \"buttonDisabledActiveFill\": \"#a3a8b8\",\n \"buttonDisabledActiveText\": \"#0e1117\",\n \"buttonDisabledSpinnerForeground\": \"rgba(250, 250, 250, 0.4)\",\n \"buttonDisabledSpinnerBackground\": \"#d5dae5\",\n \"breadcrumbsText\": \"#000000\",\n \"breadcrumbsSeparatorFill\": \"#a3a8b8\",\n \"calendarBackground\": \"#0e1117\",\n \"calendarForeground\": \"#fafafa\",\n \"calendarForegroundDisabled\": \"#a3a8b8\",\n \"calendarHeaderBackground\": \"#262730\",\n \"calendarHeaderForeground\": \"#fafafa\",\n \"calendarHeaderBackgroundActive\": \"#262730\",\n \"calendarHeaderForegroundDisabled\": \"#d5dae5\",\n \"calendarDayForegroundPseudoSelected\": \"#fafafa\",\n \"calendarDayBackgroundPseudoSelectedHighlighted\": \"#ff4b4b\",\n \"calendarDayForegroundPseudoSelectedHighlighted\": \"#fafafa\",\n \"calendarDayBackgroundSelected\": \"#ff4b4b\",\n \"calendarDayForegroundSelected\": \"#ffffff\",\n \"calendarDayBackgroundSelectedHighlighted\": \"#ff4b4b\",\n \"calendarDayForegroundSelectedHighlighted\": \"#ffffff\",\n \"comboboxListItemFocus\": \"#262730\",\n \"comboboxListItemHover\": \"#e6eaf1\",\n \"fileUploaderBackgroundColor\": \"#262730\",\n \"fileUploaderBackgroundColorActive\": \"#F6F6F6\",\n \"fileUploaderBorderColorActive\": \"#FFFFFF\",\n \"fileUploaderBorderColorDefault\": \"#a3a8b8\",\n \"fileUploaderMessageColor\": \"#fafafa\",\n \"linkText\": \"#FFFFFF\",\n \"linkVisited\": \"#ff4b4b\",\n \"linkHover\": \"#ff4b4b\",\n \"linkActive\": \"#ff4b4b\",\n \"listHeaderFill\": \"#FFFFFF\",\n \"listBodyFill\": \"#FFFFFF\",\n \"progressStepsCompletedText\": \"#FFFFFF\",\n \"progressStepsCompletedFill\": \"#FFFFFF\",\n \"progressStepsActiveText\": \"#FFFFFF\",\n \"progressStepsActiveFill\": \"#FFFFFF\",\n \"toggleFill\": \"#FFFFFF\",\n \"toggleFillChecked\": \"#FFFFFF\",\n \"toggleFillDisabled\": \"rgba(250, 250, 250, 0.4)\",\n \"toggleTrackFill\": \"#d5dae5\",\n \"toggleTrackFillDisabled\": \"#262730\",\n \"tickFill\": \"hsla(220, 24%, 10%, 1)\",\n \"tickFillHover\": \"#262730\",\n \"tickFillActive\": \"#262730\",\n \"tickFillSelected\": \"#ff4b4b\",\n \"tickFillSelectedHover\": \"#ff4b4b\",\n \"tickFillSelectedHoverActive\": \"#ff4b4b\",\n \"tickFillError\": \"#FFEFED\",\n \"tickFillErrorHover\": \"#FED7D2\",\n \"tickFillErrorHoverActive\": \"#F1998E\",\n \"tickFillErrorSelected\": \"#E11900\",\n \"tickFillErrorSelectedHover\": \"#AB1300\",\n \"tickFillErrorSelectedHoverActive\": \"#870F00\",\n \"tickFillDisabled\": \"rgba(250, 250, 250, 0.4)\",\n \"tickBorder\": \"#a3a8b8\",\n \"tickBorderError\": \"#E11900\",\n \"tickMarkFill\": \"#f0f2f6\",\n \"tickMarkFillError\": \"#FFFFFF\",\n \"tickMarkFillDisabled\": \"hsla(220, 24%, 10%, 1)\",\n \"sliderTrackFill\": \"#d5dae5\",\n \"sliderHandleFill\": \"#E2E2E2\",\n \"sliderHandleFillDisabled\": \"#ff4b4b\",\n \"sliderHandleInnerFill\": \"#d5dae5\",\n \"sliderTrackFillHover\": \"#a3a8b8\",\n \"sliderTrackFillActive\": \"rgba(250, 250, 250, 0.4)\",\n \"sliderTrackFillDisabled\": \"#262730\",\n \"sliderHandleInnerFillDisabled\": \"#d5dae5\",\n \"sliderHandleInnerFillSelectedHover\": \"#FFFFFF\",\n \"sliderHandleInnerFillSelectedActive\": \"#ff4b4b\",\n \"inputBorder\": \"#262730\",\n \"inputFill\": \"#262730\",\n \"inputFillError\": \"#FFEFED\",\n \"inputFillDisabled\": \"#262730\",\n \"inputFillActive\": \"#262730\",\n \"inputFillPositive\": \"#E6F2ED\",\n \"inputTextDisabled\": \"rgba(250, 250, 250, 0.4)\",\n \"inputBorderError\": \"#F1998E\",\n \"inputBorderPositive\": \"#66D19E\",\n \"inputEnhancerFill\": \"#e6eaf1\",\n \"inputEnhancerFillDisabled\": \"#262730\",\n \"inputEnhancerTextDisabled\": \"rgba(250, 250, 250, 0.4)\",\n \"inputPlaceholder\": \"rgba(250, 250, 250, 0.6)\",\n \"inputPlaceholderDisabled\": \"rgba(250, 250, 250, 0.4)\",\n \"menuFill\": \"#0e1117\",\n \"menuFillHover\": \"#262730\",\n \"menuFontDefault\": \"#fafafa\",\n \"menuFontDisabled\": \"#a3a8b8\",\n \"menuFontHighlighted\": \"#fafafa\",\n \"menuFontSelected\": \"#fafafa\",\n \"modalCloseColor\": \"#fafafa\",\n \"modalCloseColorHover\": \"#fafafa\",\n \"modalCloseColorFocus\": \"#fafafa\",\n \"tabBarFill\": \"#262730\",\n \"tabColor\": \"#fafafa\",\n \"notificationInfoBackground\": \"rgba(61, 157, 243, 0.2)\",\n \"notificationInfoText\": \"#c7ebff\",\n \"notificationPositiveBackground\": \"rgba(61, 213, 109, 0.2)\",\n \"notificationPositiveText\": \"#dffde9\",\n \"notificationWarningBackground\": \"rgba(255, 227, 18, 0.2)\",\n \"notificationWarningText\": \"#ffffc2\",\n \"notificationNegativeBackground\": \"rgba(255, 108, 108, 0.2)\",\n \"notificationNegativeText\": \"#ffdede\",\n \"tagFontDisabledRampUnit\": \"100\",\n \"tagSolidFontRampUnit\": \"0\",\n \"tagSolidRampUnit\": \"400\",\n \"tagOutlinedFontRampUnit\": \"400\",\n \"tagOutlinedRampUnit\": \"200\",\n \"tagSolidHoverRampUnit\": \"50\",\n \"tagSolidActiveRampUnit\": \"100\",\n \"tagSolidDisabledRampUnit\": \"50\",\n \"tagSolidFontHoverRampUnit\": \"500\",\n \"tagLightRampUnit\": \"50\",\n \"tagLightHoverRampUnit\": \"100\",\n \"tagLightActiveRampUnit\": \"100\",\n \"tagLightFontRampUnit\": \"500\",\n \"tagLightFontHoverRampUnit\": \"500\",\n \"tagOutlinedHoverRampUnit\": \"50\",\n \"tagOutlinedActiveRampUnit\": \"0\",\n \"tagOutlinedFontHoverRampUnit\": \"400\",\n \"tagNeutralFontDisabled\": \"rgba(250, 250, 250, 0.4)\",\n \"tagNeutralOutlinedDisabled\": \"#e6eaf1\",\n \"tagNeutralSolidFont\": \"#FFFFFF\",\n \"tagNeutralSolidBackground\": \"#000000\",\n \"tagNeutralOutlinedBackground\": \"rgba(250, 250, 250, 0.4)\",\n \"tagNeutralOutlinedFont\": \"#000000\",\n \"tagNeutralSolidHover\": \"#e6eaf1\",\n \"tagNeutralSolidActive\": \"#e6eaf1\",\n \"tagNeutralSolidDisabled\": \"#262730\",\n \"tagNeutralSolidFontHover\": \"#fafafa\",\n \"tagNeutralLightBackground\": \"#e6eaf1\",\n \"tagNeutralLightHover\": \"#e6eaf1\",\n \"tagNeutralLightActive\": \"#e6eaf1\",\n \"tagNeutralLightDisabled\": \"#262730\",\n \"tagNeutralLightFont\": \"#fafafa\",\n \"tagNeutralLightFontHover\": \"#fafafa\",\n \"tagNeutralOutlinedActive\": \"#fafafa\",\n \"tagNeutralOutlinedFontHover\": \"#fafafa\",\n \"tagNeutralOutlinedHover\": \"rgba(0, 0, 0, 0.08)\",\n \"tagPrimaryFontDisabled\": \"rgba(250, 250, 250, 0.4)\",\n \"tagPrimaryOutlinedDisabled\": \"transparent\",\n \"tagPrimarySolidFont\": \"#FFFFFF\",\n \"tagPrimarySolidBackground\": \"#ff4b4b\",\n \"tagPrimaryOutlinedFontHover\": \"#FFFFFF\",\n \"tagPrimaryOutlinedFont\": \"#FFFFFF\",\n \"tagPrimarySolidHover\": \"#ff4b4b\",\n \"tagPrimarySolidActive\": \"#ff4b4b\",\n \"tagPrimarySolidDisabled\": \"#F6F6F6\",\n \"tagPrimarySolidFontHover\": \"#ff4b4b\",\n \"tagPrimaryLightBackground\": \"#F6F6F6\",\n \"tagPrimaryLightHover\": \"#ff4b4b\",\n \"tagPrimaryLightActive\": \"#ff4b4b\",\n \"tagPrimaryLightDisabled\": \"#F6F6F6\",\n \"tagPrimaryLightFont\": \"#ff4b4b\",\n \"tagPrimaryLightFontHover\": \"#ff4b4b\",\n \"tagPrimaryOutlinedActive\": \"#ff4b4b\",\n \"tagPrimaryOutlinedHover\": \"rgba(0, 0, 0, 0.08)\",\n \"tagPrimaryOutlinedBackground\": \"#ff4b4b\",\n \"tagAccentFontDisabled\": \"#A0BFF8\",\n \"tagAccentOutlinedDisabled\": \"#A0BFF8\",\n \"tagAccentSolidFont\": \"#FFFFFF\",\n \"tagAccentSolidBackground\": \"#276EF1\",\n \"tagAccentOutlinedBackground\": \"#A0BFF8\",\n \"tagAccentOutlinedFont\": \"#276EF1\",\n \"tagAccentSolidHover\": \"#EFF3FE\",\n \"tagAccentSolidActive\": \"#D4E2FC\",\n \"tagAccentSolidDisabled\": \"#EFF3FE\",\n \"tagAccentSolidFontHover\": \"#1E54B7\",\n \"tagAccentLightBackground\": \"#EFF3FE\",\n \"tagAccentLightHover\": \"#D4E2FC\",\n \"tagAccentLightActive\": \"#D4E2FC\",\n \"tagAccentLightDisabled\": \"#EFF3FE\",\n \"tagAccentLightFont\": \"#1E54B7\",\n \"tagAccentLightFontHover\": \"#1E54B7\",\n \"tagAccentOutlinedActive\": \"#174291\",\n \"tagAccentOutlinedFontHover\": \"#276EF1\",\n \"tagAccentOutlinedHover\": \"rgba(0, 0, 0, 0.08)\",\n \"tagPositiveFontDisabled\": \"#66D19E\",\n \"tagPositiveOutlinedDisabled\": \"#66D19E\",\n \"tagPositiveSolidFont\": \"#FFFFFF\",\n \"tagPositiveSolidBackground\": \"#048848\",\n \"tagPositiveOutlinedBackground\": \"#66D19E\",\n \"tagPositiveOutlinedFont\": \"#048848\",\n \"tagPositiveSolidHover\": \"#E6F2ED\",\n \"tagPositiveSolidActive\": \"#ADDEC9\",\n \"tagPositiveSolidDisabled\": \"#E6F2ED\",\n \"tagPositiveSolidFontHover\": \"#03703C\",\n \"tagPositiveLightBackground\": \"#E6F2ED\",\n \"tagPositiveLightHover\": \"#ADDEC9\",\n \"tagPositiveLightActive\": \"#ADDEC9\",\n \"tagPositiveLightDisabled\": \"#E6F2ED\",\n \"tagPositiveLightFont\": \"#03703C\",\n \"tagPositiveLightFontHover\": \"#03703C\",\n \"tagPositiveOutlinedActive\": \"#03582F\",\n \"tagPositiveOutlinedFontHover\": \"#048848\",\n \"tagPositiveOutlinedHover\": \"rgba(0, 0, 0, 0.08)\",\n \"tagWarningFontDisabled\": \"#FFCF70\",\n \"tagWarningOutlinedDisabled\": \"#FFCF70\",\n \"tagWarningSolidFont\": \"#674D1B\",\n \"tagWarningSolidBackground\": \"#FFC043\",\n \"tagWarningOutlinedBackground\": \"#FFCF70\",\n \"tagWarningOutlinedFont\": \"#996F00\",\n \"tagWarningSolidHover\": \"#FFFAF0\",\n \"tagWarningSolidActive\": \"#FFF2D9\",\n \"tagWarningSolidDisabled\": \"#FFFAF0\",\n \"tagWarningSolidFontHover\": \"#BC8B2C\",\n \"tagWarningLightBackground\": \"#FFFAF0\",\n \"tagWarningLightHover\": \"#FFF2D9\",\n \"tagWarningLightActive\": \"#FFF2D9\",\n \"tagWarningLightDisabled\": \"#FFFAF0\",\n \"tagWarningLightFont\": \"#BC8B2C\",\n \"tagWarningLightFontHover\": \"#BC8B2C\",\n \"tagWarningOutlinedActive\": \"#996F00\",\n \"tagWarningOutlinedFontHover\": \"#996F00\",\n \"tagWarningOutlinedHover\": \"rgba(0, 0, 0, 0.08)\",\n \"tagNegativeFontDisabled\": \"#F1998E\",\n \"tagNegativeOutlinedDisabled\": \"#F1998E\",\n \"tagNegativeSolidFont\": \"#FFFFFF\",\n \"tagNegativeSolidBackground\": \"#E11900\",\n \"tagNegativeOutlinedBackground\": \"#F1998E\",\n \"tagNegativeOutlinedFont\": \"#E11900\",\n \"tagNegativeSolidHover\": \"#FFEFED\",\n \"tagNegativeSolidActive\": \"#FED7D2\",\n \"tagNegativeSolidDisabled\": \"#FFEFED\",\n \"tagNegativeSolidFontHover\": \"#AB1300\",\n \"tagNegativeLightBackground\": \"#FFEFED\",\n \"tagNegativeLightHover\": \"#FED7D2\",\n \"tagNegativeLightActive\": \"#FED7D2\",\n \"tagNegativeLightDisabled\": \"#FFEFED\",\n \"tagNegativeLightFont\": \"#AB1300\",\n \"tagNegativeLightFontHover\": \"#AB1300\",\n \"tagNegativeOutlinedActive\": \"#870F00\",\n \"tagNegativeOutlinedFontHover\": \"#E11900\",\n \"tagNegativeOutlinedHover\": \"rgba(0, 0, 0, 0.08)\",\n \"tableHeadBackgroundColor\": \"#0e1117\",\n \"tableBackground\": \"#0e1117\",\n \"tableStripedBackground\": \"#262730\",\n \"tableFilter\": \"rgba(250, 250, 250, 0.4)\",\n \"tableFilterHeading\": \"#a3a8b8\",\n \"tableFilterBackground\": \"#0e1117\",\n \"tableFilterFooterBackground\": \"#262730\",\n \"toastText\": \"#FFFFFF\",\n \"toastPrimaryText\": \"#FFFFFF\",\n \"toastInfoBackground\": \"#276EF1\",\n \"toastInfoText\": \"#FFFFFF\",\n \"toastPositiveBackground\": \"#048848\",\n \"toastPositiveText\": \"#FFFFFF\",\n \"toastWarningBackground\": \"#FFC043\",\n \"toastWarningText\": \"#000000\",\n \"toastNegativeBackground\": \"#E11900\",\n \"toastNegativeText\": \"#FFFFFF\",\n \"spinnerTrackFill\": \"#fafafa\",\n \"progressbarTrackFill\": \"#262730\",\n \"tooltipBackground\": \"#fafafa\",\n \"tooltipText\": \"#0e1117\",\n \"backgroundPrimary\": \"#0e1117\",\n \"backgroundSecondary\": \"#262730\",\n \"backgroundTertiary\": \"#0e1117\",\n \"backgroundInversePrimary\": \"#E2E2E2\",\n \"backgroundInverseSecondary\": \"#1F1F1F\",\n \"contentPrimary\": \"#fafafa\",\n \"contentSecondary\": \"#545454\",\n \"contentTertiary\": \"#6B6B6B\",\n \"contentInversePrimary\": \"#141414\",\n \"contentInverseSecondary\": \"#CBCBCB\",\n \"contentInverseTertiary\": \"#AFAFAF\",\n \"borderOpaque\": \"rgba(172, 177, 195, 0.25)\",\n \"borderTransparent\": \"rgba(226, 226, 226, 0.08)\",\n \"borderSelected\": \"#ff4b4b\",\n \"borderInverseOpaque\": \"#333333\",\n \"borderInverseTransparent\": \"rgba(20, 20, 20, 0.2)\",\n \"borderInverseSelected\": \"#141414\",\n \"backgroundStateDisabled\": \"#F6F6F6\",\n \"backgroundOverlayDark\": \"rgba(0, 0, 0, 0.3)\",\n \"backgroundOverlayLight\": \"rgba(0, 0, 0, 0.08)\",\n \"backgroundOverlayArt\": \"rgba(0, 0, 0, 0.00)\",\n \"backgroundAccent\": \"#276EF1\",\n \"backgroundNegative\": \"#AB1300\",\n \"backgroundWarning\": \"#BC8B2C\",\n \"backgroundPositive\": \"#048848\",\n \"backgroundLightAccent\": \"#EFF3FE\",\n \"backgroundLightNegative\": \"#FFEFED\",\n \"backgroundLightWarning\": \"#FFFAF0\",\n \"backgroundLightPositive\": \"#E6F2ED\",\n \"backgroundAlwaysDark\": \"#000000\",\n \"backgroundAlwaysLight\": \"#FFFFFF\",\n \"contentStateDisabled\": \"#AFAFAF\",\n \"contentAccent\": \"#276EF1\",\n \"contentOnColor\": \"#FFFFFF\",\n \"contentOnColorInverse\": \"#000000\",\n \"contentNegative\": \"#AB1300\",\n \"contentWarning\": \"#996F00\",\n \"contentPositive\": \"#048848\",\n \"borderStateDisabled\": \"#F6F6F6\",\n \"borderAccent\": \"#276EF1\",\n \"borderAccentLight\": \"#A0BFF8\",\n \"borderNegative\": \"#F1998E\",\n \"borderWarning\": \"#FFE3AC\",\n \"borderPositive\": \"#66D19E\",\n \"safety\": \"#276EF1\",\n \"eatsGreen400\": \"#048848\",\n \"freightBlue400\": \"#0E1FC1\",\n \"jumpRed400\": \"#E11900\",\n \"rewardsTier1\": \"#276EF1\",\n \"rewardsTier2\": \"#FFC043\",\n \"rewardsTier3\": \"#8EA3AD\",\n \"rewardsTier4\": \"#000000\",\n \"membership\": \"#996F00\",\n \"datepickerBackground\": \"#0e1117\",\n \"inputEnhanceFill\": \"#262730\"\n },\n \"direction\": \"auto\",\n \"grid\": {\n \"columns\": [\n 4,\n 8,\n 12\n ],\n \"gutters\": [\n 16,\n 36,\n 36\n ],\n \"margins\": [\n 16,\n 36,\n 64\n ],\n \"gaps\": 0,\n \"unit\": \"px\",\n \"maxWidth\": 1280\n },\n \"lighting\": {\n \"shadow400\": \"0 1px 4px hsla(0, 0%, 0%, 0.16)\",\n \"shadow500\": \"0 2px 8px hsla(0, 0%, 0%, 0.16)\",\n \"shadow600\": \"0 4px 16px hsla(0, 0%, 0%, 0.16)\",\n \"shadow700\": \"0 8px 24px hsla(0, 0%, 0%, 0.16)\",\n \"overlay0\": \"inset 0 0 0 1000px hsla(0, 0%, 0%, 0)\",\n \"overlay100\": \"inset 0 0 0 1000px hsla(0, 0%, 0%, 0.04)\",\n \"overlay200\": \"inset 0 0 0 1000px hsla(0, 0%, 0%, 0.08)\",\n \"overlay300\": \"inset 0 0 0 1000px hsla(0, 0%, 0%, 0.12)\",\n \"overlay400\": \"inset 0 0 0 1000px hsla(0, 0%, 0%, 0.16)\",\n \"overlay500\": \"inset 0 0 0 1000px hsla(0, 0%, 0%, 0.2)\",\n \"overlay600\": \"inset 0 0 0 1000px hsla(0, 0%, 0%, 0.24)\",\n \"shallowAbove\": \"0px -4px 16px rgba(0, 0, 0, 0.12)\",\n \"shallowBelow\": \"0px 4px 16px rgba(0, 0, 0, 0.12)\",\n \"deepAbove\": \"0px -16px 48px rgba(0, 0, 0, 0.22)\",\n \"deepBelow\": \"0px 16px 48px rgba(0, 0, 0, 0.22)\"\n },\n \"mediaQuery\": {\n \"small\": \"@media screen and (min-width: 320px)\",\n \"medium\": \"@media screen and (min-width: 600px)\",\n \"large\": \"@media screen and (min-width: 1136px)\"\n },\n \"sizing\": {\n \"scale0\": \"2px\",\n \"scale100\": \"4px\",\n \"scale200\": \"6px\",\n \"scale300\": \"8px\",\n \"scale400\": \"10px\",\n \"scale500\": \"12px\",\n \"scale550\": \"14px\",\n \"scale600\": \"16px\",\n \"scale650\": \"18px\",\n \"scale700\": \"20px\",\n \"scale750\": \"22px\",\n \"scale800\": \"24px\",\n \"scale850\": \"28px\",\n \"scale900\": \"32px\",\n \"scale950\": \"36px\",\n \"scale1000\": \"40px\",\n \"scale1200\": \"48px\",\n \"scale1400\": \"56px\",\n \"scale1600\": \"64px\",\n \"scale2400\": \"96px\",\n \"scale3200\": \"128px\",\n \"scale4800\": \"192px\"\n },\n \"typography\": {\n \"font100\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"12px\",\n \"fontWeight\": \"normal\",\n \"lineHeight\": \"20px\"\n },\n \"font150\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"1rem\",\n \"fontWeight\": \"normal\",\n \"lineHeight\": 1.6,\n \"fontSizeSm\": \"14px\",\n \"lineHeightTight\": 1.25\n },\n \"font200\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"1rem\",\n \"fontWeight\": \"normal\",\n \"lineHeight\": 1.6,\n \"fontSizeSm\": \"14px\",\n \"lineHeightTight\": 1.25\n },\n \"font250\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"1rem\",\n \"fontWeight\": \"normal\",\n \"lineHeight\": 1.6,\n \"fontSizeSm\": \"14px\",\n \"lineHeightTight\": 1.25\n },\n \"font300\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"1rem\",\n \"fontWeight\": \"normal\",\n \"lineHeight\": 1.6,\n \"fontSizeSm\": \"14px\",\n \"lineHeightTight\": 1.25\n },\n \"font350\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"1rem\",\n \"fontWeight\": \"normal\",\n \"lineHeight\": 1.6,\n \"fontSizeSm\": \"14px\",\n \"lineHeightTight\": 1.25\n },\n \"font400\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"1rem\",\n \"fontWeight\": \"normal\",\n \"lineHeight\": 1.6,\n \"fontSizeSm\": \"14px\",\n \"lineHeightTight\": 1.25\n },\n \"font450\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"1rem\",\n \"fontWeight\": \"normal\",\n \"lineHeight\": 1.6,\n \"fontSizeSm\": \"14px\",\n \"lineHeightTight\": 1.25\n },\n \"font550\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"20px\",\n \"fontWeight\": 700,\n \"lineHeight\": \"28px\"\n },\n \"font650\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"24px\",\n \"fontWeight\": 700,\n \"lineHeight\": \"32px\"\n },\n \"font750\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"28px\",\n \"fontWeight\": 700,\n \"lineHeight\": \"36px\"\n },\n \"font850\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"32px\",\n \"fontWeight\": 700,\n \"lineHeight\": \"40px\"\n },\n \"font950\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"36px\",\n \"fontWeight\": 700,\n \"lineHeight\": \"44px\"\n },\n \"font1050\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"40px\",\n \"fontWeight\": 700,\n \"lineHeight\": \"52px\"\n },\n \"font1150\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"36px\",\n \"fontWeight\": 700,\n \"lineHeight\": \"44px\"\n },\n \"font1250\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"44px\",\n \"fontWeight\": 700,\n \"lineHeight\": \"52px\"\n },\n \"font1350\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"52px\",\n \"fontWeight\": 700,\n \"lineHeight\": \"64px\"\n },\n \"font1450\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"96px\",\n \"fontWeight\": 700,\n \"lineHeight\": \"112px\"\n },\n \"ParagraphXSmall\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"12px\",\n \"fontWeight\": \"normal\",\n \"lineHeight\": \"20px\"\n },\n \"ParagraphSmall\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"1rem\",\n \"fontWeight\": \"normal\",\n \"lineHeight\": 1.6,\n \"fontSizeSm\": \"14px\",\n \"lineHeightTight\": 1.25\n },\n \"ParagraphMedium\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"1rem\",\n \"fontWeight\": \"normal\",\n \"lineHeight\": 1.6,\n \"fontSizeSm\": \"14px\",\n \"lineHeightTight\": 1.25\n },\n \"ParagraphLarge\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"1rem\",\n \"fontWeight\": \"normal\",\n \"lineHeight\": 1.6,\n \"fontSizeSm\": \"14px\",\n \"lineHeightTight\": 1.25\n },\n \"LabelXSmall\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"1rem\",\n \"fontWeight\": \"normal\",\n \"lineHeight\": 1.6,\n \"fontSizeSm\": \"14px\",\n \"lineHeightTight\": 1.25\n },\n \"LabelSmall\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"1rem\",\n \"fontWeight\": \"normal\",\n \"lineHeight\": 1.6,\n \"fontSizeSm\": \"14px\",\n \"lineHeightTight\": 1.25\n },\n \"LabelMedium\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"1rem\",\n \"fontWeight\": \"normal\",\n \"lineHeight\": 1.6,\n \"fontSizeSm\": \"14px\",\n \"lineHeightTight\": 1.25\n },\n \"LabelLarge\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"1rem\",\n \"fontWeight\": \"normal\",\n \"lineHeight\": 1.6,\n \"fontSizeSm\": \"14px\",\n \"lineHeightTight\": 1.25\n },\n \"HeadingXSmall\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"20px\",\n \"fontWeight\": 700,\n \"lineHeight\": \"28px\"\n },\n \"HeadingSmall\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"24px\",\n \"fontWeight\": 700,\n \"lineHeight\": \"32px\"\n },\n \"HeadingMedium\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"28px\",\n \"fontWeight\": 700,\n \"lineHeight\": \"36px\"\n },\n \"HeadingLarge\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"32px\",\n \"fontWeight\": 700,\n \"lineHeight\": \"40px\"\n },\n \"HeadingXLarge\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"36px\",\n \"fontWeight\": 700,\n \"lineHeight\": \"44px\"\n },\n \"HeadingXXLarge\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"40px\",\n \"fontWeight\": 700,\n \"lineHeight\": \"52px\"\n },\n \"DisplayXSmall\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"36px\",\n \"fontWeight\": 700,\n \"lineHeight\": \"44px\"\n },\n \"DisplaySmall\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"44px\",\n \"fontWeight\": 700,\n \"lineHeight\": \"52px\"\n },\n \"DisplayMedium\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"52px\",\n \"fontWeight\": 700,\n \"lineHeight\": \"64px\"\n },\n \"DisplayLarge\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"96px\",\n \"fontWeight\": 700,\n \"lineHeight\": \"112px\"\n },\n \"MonoParagraphXSmall\": {\n \"fontFamily\": \"\\\"Lucida Console\\\", Monaco, monospace\",\n \"fontSize\": \"12px\",\n \"fontWeight\": \"normal\",\n \"lineHeight\": \"20px\"\n },\n \"MonoParagraphSmall\": {\n \"fontFamily\": \"\\\"Lucida Console\\\", Monaco, monospace\",\n \"fontSize\": \"14px\",\n \"fontWeight\": \"normal\",\n \"lineHeight\": \"20px\"\n },\n \"MonoParagraphMedium\": {\n \"fontFamily\": \"\\\"Lucida Console\\\", Monaco, monospace\",\n \"fontSize\": \"16px\",\n \"fontWeight\": \"normal\",\n \"lineHeight\": \"24px\"\n },\n \"MonoParagraphLarge\": {\n \"fontFamily\": \"\\\"Lucida Console\\\", Monaco, monospace\",\n \"fontSize\": \"18px\",\n \"fontWeight\": \"normal\",\n \"lineHeight\": \"28px\"\n },\n \"MonoLabelXSmall\": {\n \"fontFamily\": \"\\\"Lucida Console\\\", Monaco, monospace\",\n \"fontSize\": \"12px\",\n \"fontWeight\": 500,\n \"lineHeight\": \"16px\"\n },\n \"MonoLabelSmall\": {\n \"fontFamily\": \"\\\"Lucida Console\\\", Monaco, monospace\",\n \"fontSize\": \"14px\",\n \"fontWeight\": 500,\n \"lineHeight\": \"16px\"\n },\n \"MonoLabelMedium\": {\n \"fontFamily\": \"\\\"Lucida Console\\\", Monaco, monospace\",\n \"fontSize\": \"16px\",\n \"fontWeight\": 500,\n \"lineHeight\": \"20px\"\n },\n \"MonoLabelLarge\": {\n \"fontFamily\": \"\\\"Lucida Console\\\", Monaco, monospace\",\n \"fontSize\": \"18px\",\n \"fontWeight\": 500,\n \"lineHeight\": \"24px\"\n },\n \"MonoHeadingXSmall\": {\n \"fontFamily\": \"\\\"Lucida Console\\\", Monaco, monospace\",\n \"fontSize\": \"20px\",\n \"fontWeight\": 700,\n \"lineHeight\": \"28px\"\n },\n \"MonoHeadingSmall\": {\n \"fontFamily\": \"\\\"Lucida Console\\\", Monaco, monospace\",\n \"fontSize\": \"24px\",\n \"fontWeight\": 700,\n \"lineHeight\": \"32px\"\n },\n \"MonoHeadingMedium\": {\n \"fontFamily\": \"\\\"Lucida Console\\\", Monaco, monospace\",\n \"fontSize\": \"28px\",\n \"fontWeight\": 700,\n \"lineHeight\": \"36px\"\n },\n \"MonoHeadingLarge\": {\n \"fontFamily\": \"\\\"Lucida Console\\\", Monaco, monospace\",\n \"fontSize\": \"32px\",\n \"fontWeight\": 700,\n \"lineHeight\": \"40px\"\n },\n \"MonoHeadingXLarge\": {\n \"fontFamily\": \"\\\"Lucida Console\\\", Monaco, monospace\",\n \"fontSize\": \"36px\",\n \"fontWeight\": 700,\n \"lineHeight\": \"44px\"\n },\n \"MonoHeadingXXLarge\": {\n \"fontFamily\": \"\\\"Lucida Console\\\", Monaco, monospace\",\n \"fontSize\": \"40px\",\n \"fontWeight\": 700,\n \"lineHeight\": \"52px\"\n },\n \"MonoDisplayXSmall\": {\n \"fontFamily\": \"\\\"Lucida Console\\\", Monaco, monospace\",\n \"fontSize\": \"36px\",\n \"fontWeight\": 700,\n \"lineHeight\": \"44px\"\n },\n \"MonoDisplaySmall\": {\n \"fontFamily\": \"\\\"Lucida Console\\\", Monaco, monospace\",\n \"fontSize\": \"44px\",\n \"fontWeight\": 700,\n \"lineHeight\": \"52px\"\n },\n \"MonoDisplayMedium\": {\n \"fontFamily\": \"\\\"Lucida Console\\\", Monaco, monospace\",\n \"fontSize\": \"52px\",\n \"fontWeight\": 700,\n \"lineHeight\": \"64px\"\n },\n \"MonoDisplayLarge\": {\n \"fontFamily\": \"\\\"Lucida Console\\\", Monaco, monospace\",\n \"fontSize\": \"96px\",\n \"fontWeight\": 700,\n \"lineHeight\": \"112px\"\n },\n \"font460\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"1rem\",\n \"fontSizeSm\": \"14px\",\n \"fontWeight\": \"normal\",\n \"lineHeight\": 1.6,\n \"lineHeightTight\": 1.25\n },\n \"font470\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"1rem\",\n \"fontSizeSm\": \"14px\",\n \"fontWeight\": \"normal\",\n \"lineHeight\": 1.6,\n \"lineHeightTight\": 1.25\n },\n \"font500\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"1rem\",\n \"fontSizeSm\": \"14px\",\n \"fontWeight\": \"normal\",\n \"lineHeight\": 1.6,\n \"lineHeightTight\": 1.25\n },\n \"font600\": {}\n },\n \"zIndex\": {\n \"modal\": 2000\n }\n },\n \"primitives\": {\n \"primaryA\": \"#E2E2E2\",\n \"primaryB\": \"#141414\",\n \"primary\": \"#FFFFFF\",\n \"primary50\": \"#F6F6F6\",\n \"primary100\": \"#EEEEEE\",\n \"primary200\": \"#E2E2E2\",\n \"primary300\": \"#CBCBCB\",\n \"primary400\": \"#AFAFAF\",\n \"primary500\": \"#6B6B6B\",\n \"primary600\": \"#545454\",\n \"primary700\": \"#333333\",\n \"accent\": \"#276EF1\",\n \"accent50\": \"#EFF3FE\",\n \"accent100\": \"#D4E2FC\",\n \"accent200\": \"#A0BFF8\",\n \"accent300\": \"#5B91F5\",\n \"accent400\": \"#276EF1\",\n \"accent500\": \"#1E54B7\",\n \"accent600\": \"#174291\",\n \"accent700\": \"#102C60\",\n \"negative\": \"#AB1300\",\n \"negative50\": \"#FFEFED\",\n \"negative100\": \"#FED7D2\",\n \"negative200\": \"#F1998E\",\n \"negative300\": \"#E85C4A\",\n \"negative400\": \"#E11900\",\n \"negative500\": \"#AB1300\",\n \"negative600\": \"#870F00\",\n \"negative700\": \"#5A0A00\",\n \"warning\": \"#BC8B2C\",\n \"warning50\": \"#FFFAF0\",\n \"warning100\": \"#FFF2D9\",\n \"warning200\": \"#FFE3AC\",\n \"warning300\": \"#FFCF70\",\n \"warning400\": \"#FFC043\",\n \"warning500\": \"#BC8B2C\",\n \"warning600\": \"#996F00\",\n \"warning700\": \"#674D1B\",\n \"positive\": \"#048848\",\n \"positive50\": \"#E6F2ED\",\n \"positive100\": \"#ADDEC9\",\n \"positive200\": \"#66D19E\",\n \"positive300\": \"#06C167\",\n \"positive400\": \"#048848\",\n \"positive500\": \"#03703C\",\n \"positive600\": \"#03582F\",\n \"positive700\": \"#10462D\",\n \"white\": \"#FFFFFF\",\n \"black\": \"#000000\",\n \"mono100\": \"#CBCBCB\",\n \"mono200\": \"#AFAFAF\",\n \"mono300\": \"#6B6B6B\",\n \"mono400\": \"#545454\",\n \"mono500\": \"#333333\",\n \"mono600\": \"#292929\",\n \"mono700\": \"#1F1F1F\",\n \"mono800\": \"#141414\",\n \"mono900\": \"#111111\",\n \"mono1000\": \"#000000\",\n \"ratingInactiveFill\": \"#6B6B6B\",\n \"ratingStroke\": \"#333333\",\n \"primaryFontFamily\": \"system-ui, \\\"Helvetica Neue\\\", Helvetica, Arial, sans-serif\"\n }\n}\n```\n\n### JSON Base Configuration for Streamlit Light Theme\n\n```json\n{\n \"name\": \"Light\",\n \"emotion\": {\n \"inSidebar\": false,\n \"breakpoints\": {\n \"hideWidgetDetails\": 180,\n \"hideNumberInputControls\": 120,\n \"sm\": \"576px\",\n \"columns\": \"640px\",\n \"md\": \"768px\"\n },\n \"colors\": {\n \"transparent\": \"transparent\",\n \"black\": \"#000000\",\n \"white\": \"#ffffff\",\n \"gray10\": \"#fafafa\",\n \"gray20\": \"#f0f2f6\",\n \"gray30\": \"#e6eaf1\",\n \"gray40\": \"#d5dae5\",\n \"gray50\": \"#bfc5d3\",\n \"gray60\": \"#a3a8b8\",\n \"gray70\": \"#808495\",\n \"gray80\": \"#555867\",\n \"gray85\": \"#31333F\",\n \"gray90\": \"#262730\",\n \"gray100\": \"#0e1117\",\n \"red10\": \"#fff0f0\",\n \"red20\": \"#ffdede\",\n \"red30\": \"#ffc7c7\",\n \"red40\": \"#ffabab\",\n \"red50\": \"#ff8c8c\",\n \"red60\": \"#ff6c6c\",\n \"red70\": \"#ff4b4b\",\n \"red80\": \"#ff2b2b\",\n \"red90\": \"#bd4043\",\n \"red100\": \"#7d353b\",\n \"orange10\": \"#fffae8\",\n \"orange20\": \"#fff6d0\",\n \"orange30\": \"#ffecb0\",\n \"orange40\": \"#ffe08e\",\n \"orange50\": \"#ffd16a\",\n \"orange60\": \"#ffbd45\",\n \"orange70\": \"#ffa421\",\n \"orange80\": \"#ff8700\",\n \"orange90\": \"#ed6f13\",\n \"orange100\": \"#d95a00\",\n \"yellow10\": \"#ffffe1\",\n \"yellow20\": \"#ffffc2\",\n \"yellow30\": \"#ffffa0\",\n \"yellow40\": \"#ffff7d\",\n \"yellow50\": \"#ffff59\",\n \"yellow60\": \"#fff835\",\n \"yellow70\": \"#ffe312\",\n \"yellow80\": \"#faca2b\",\n \"yellow90\": \"#edbb16\",\n \"yellow100\": \"#dea816\",\n \"yellow110\": \"#916e10\",\n \"green10\": \"#dffde9\",\n \"green20\": \"#c0fcd3\",\n \"green30\": \"#9ef6bb\",\n \"green40\": \"#7defa1\",\n \"green50\": \"#5ce488\",\n \"green60\": \"#3dd56d\",\n \"green70\": \"#21c354\",\n \"green80\": \"#09ab3b\",\n \"green90\": \"#158237\",\n \"green100\": \"#177233\",\n \"blueGreen10\": \"#dcfffb\",\n \"blueGreen20\": \"#bafff7\",\n \"blueGreen30\": \"#93ffee\",\n \"blueGreen40\": \"#6bfde3\",\n \"blueGreen50\": \"#45f4d5\",\n \"blueGreen60\": \"#20e7c5\",\n \"blueGreen70\": \"#00d4b1\",\n \"blueGreen80\": \"#29b09d\",\n \"blueGreen90\": \"#2c867c\",\n \"blueGreen100\": \"#246e69\",\n \"lightBlue10\": \"#e0feff\",\n \"lightBlue20\": \"#bffdff\",\n \"lightBlue30\": \"#9af8ff\",\n \"lightBlue40\": \"#73efff\",\n \"lightBlue50\": \"#4be4ff\",\n \"lightBlue60\": \"#24d4ff\",\n \"lightBlue70\": \"#00c0f2\",\n \"lightBlue80\": \"#00a4d4\",\n \"lightBlue90\": \"#0d8cb5\",\n \"lightBlue100\": \"#15799e\",\n \"blue10\": \"#e4f5ff\",\n \"blue20\": \"#c7ebff\",\n \"blue30\": \"#a6dcff\",\n \"blue40\": \"#83c9ff\",\n \"blue50\": \"#60b4ff\",\n \"blue60\": \"#3d9df3\",\n \"blue70\": \"#1c83e1\",\n \"blue80\": \"#0068c9\",\n \"blue90\": \"#0054a3\",\n \"blue100\": \"#004280\",\n \"purple10\": \"#f5ebff\",\n \"purple20\": \"#ebd6ff\",\n \"purple30\": \"#dbbbff\",\n \"purple40\": \"#c89dff\",\n \"purple50\": \"#b27eff\",\n \"purple60\": \"#9a5dff\",\n \"purple70\": \"#803df5\",\n \"purple80\": \"#6d3fc0\",\n \"purple90\": \"#583f84\",\n \"purple100\": \"#3f3163\",\n \"bgColor\": \"#ffffff\",\n \"secondaryBg\": \"#f0f2f6\",\n \"bodyText\": \"#31333F\",\n \"warning\": \"#926C05\",\n \"warningBg\": \"rgba(255, 227, 18, 0.1)\",\n \"success\": \"#177233\",\n \"successBg\": \"rgba(33, 195, 84, 0.1)\",\n \"infoBg\": \"rgba(28, 131, 225, 0.1)\",\n \"info\": \"#004280\",\n \"danger\": \"#7d353b\",\n \"dangerBg\": \"rgba(255, 43, 43, 0.09)\",\n \"primary\": \"#ff4b4b\",\n \"disabled\": \"#d5dae5\",\n \"lightestGray\": \"#f0f2f6\",\n \"lightGray\": \"#e6eaf1\",\n \"gray\": \"#a3a8b8\",\n \"darkGray\": \"#808495\",\n \"red\": \"#ff2b2b\",\n \"blue\": \"#0068c9\",\n \"green\": \"#09ab3b\",\n \"yellow\": \"#faca2b\",\n \"linkText\": \"#0068c9\",\n \"fadedText05\": \"rgba(49, 51, 63, 0.1)\",\n \"fadedText10\": \"rgba(49, 51, 63, 0.2)\",\n \"fadedText20\": \"rgba(49, 51, 63, 0.3)\",\n \"fadedText40\": \"rgba(49, 51, 63, 0.4)\",\n \"fadedText60\": \"rgba(49, 51, 63, 0.6)\",\n \"bgMix\": \"rgba(248, 249, 251, 1)\",\n \"darkenedBgMix100\": \"hsla(220, 27%, 68%, 1)\",\n \"darkenedBgMix25\": \"rgba(151, 166, 195, 0.25)\",\n \"darkenedBgMix15\": \"rgba(151, 166, 195, 0.15)\",\n \"lightenedBg05\": \"hsla(0, 0%, 100%, 1)\",\n \"borderColor\": \"rgba(49, 51, 63, 0.2)\",\n \"borderColorLight\": \"rgba(49, 51, 63, 0.1)\",\n \"codeTextColor\": \"#09ab3b\",\n \"codeHighlightColor\": \"rgba(248, 249, 251, 1)\",\n \"metricPositiveDeltaColor\": \"#09ab3b\",\n \"metricNegativeDeltaColor\": \"#ff2b2b\",\n \"metricNeutralDeltaColor\": \"rgba(49, 51, 63, 0.6)\",\n \"docStringModuleText\": \"#31333F\",\n \"docStringTypeText\": \"#21c354\",\n \"docStringContainerBackground\": \"rgba(240, 242, 246, 0.4)\",\n \"headingColor\": \"#31333F\",\n \"navTextColor\": \"#555867\",\n \"navActiveTextColor\": \"#262730\",\n \"navIconColor\": \"#a3a8b8\",\n \"sidebarControlColor\": \"#808495\"\n },\n \"fonts\": {\n \"sansSerif\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"monospace\": \"\\\"Source Code Pro\\\", monospace\",\n \"serif\": \"\\\"Source Serif Pro\\\", serif\",\n \"materialIcons\": \"Material Symbols Rounded\"\n },\n \"fontSizes\": {\n \"twoSm\": \"12px\",\n \"sm\": \"14px\",\n \"md\": \"1rem\",\n \"mdLg\": \"1.125rem\",\n \"lg\": \"1.25rem\",\n \"xl\": \"1.5rem\",\n \"twoXL\": \"1.75rem\",\n \"threeXL\": \"2.25rem\",\n \"fourXL\": \"2.75rem\",\n \"twoSmPx\": 12,\n \"smPx\": 14,\n \"mdPx\": 16\n },\n \"fontWeights\": {\n \"normal\": 400,\n \"bold\": 600,\n \"extrabold\": 700\n },\n \"genericFonts\": {\n \"bodyFont\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"codeFont\": \"\\\"Source Code Pro\\\", monospace\",\n \"headingFont\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"iconFont\": \"Material Symbols Rounded\"\n },\n \"iconSizes\": {\n \"xs\": \"0.5rem\",\n \"sm\": \"0.75rem\",\n \"md\": \"0.9rem\",\n \"base\": \"1rem\",\n \"lg\": \"1.25rem\",\n \"xl\": \"1.5rem\",\n \"twoXL\": \"1.8rem\",\n \"threeXL\": \"2.3rem\"\n },\n \"lineHeights\": {\n \"none\": 1,\n \"headings\": 1.2,\n \"tight\": 1.25,\n \"inputWidget\": 1.4,\n \"small\": 1.5,\n \"base\": 1.6,\n \"menuItem\": 2\n },\n \"radii\": {\n \"md\": \"0.25rem\",\n \"default\": \"0.5rem\",\n \"xl\": \"0.75rem\",\n \"xxl\": \"1rem\",\n \"full\": \"9999px\"\n },\n \"sizes\": {\n \"full\": \"100%\",\n \"headerHeight\": \"3.75rem\",\n \"fullScreenHeaderHeight\": \"2.875rem\",\n \"sidebarTopSpace\": \"6rem\",\n \"toastWidth\": \"21rem\",\n \"contentMaxWidth\": \"46rem\",\n \"maxChartTooltipWidth\": \"30rem\",\n \"checkbox\": \"1rem\",\n \"borderWidth\": \"1px\",\n \"smallElementHeight\": \"1.5rem\",\n \"minElementHeight\": \"2.5rem\",\n \"largestElementHeight\": \"4.25rem\",\n \"smallLogoHeight\": \"1.25rem\",\n \"defaultLogoHeight\": \"1.5rem\",\n \"largeLogoHeight\": \"2rem\",\n \"sliderThumb\": \"0.75rem\",\n \"wideSidePadding\": \"5rem\",\n \"headerDecorationHeight\": \"0.125rem\",\n \"appRunningMen\": \"1.6rem\",\n \"appStatusMaxWidth\": \"20rem\",\n \"spinnerSize\": \"1.375rem\",\n \"spinnerThickness\": \"0.2rem\",\n \"tabHeight\": \"2.5rem\",\n \"minPopupWidth\": \"20rem\",\n \"maxTooltipHeight\": \"18.75rem\",\n \"chatAvatarSize\": \"2rem\",\n \"clearIconSize\": \"1.5em\",\n \"numberInputControlsWidth\": \"2rem\",\n \"emptyDropdownHeight\": \"5.625rem\",\n \"dropdownItemHeight\": \"2.5rem\",\n \"maxDropdownHeight\": \"18.75rem\",\n \"appDefaultBottomPadding\": \"3.5rem\"\n },\n \"spacing\": {\n \"px\": \"1px\",\n \"none\": \"0\",\n \"threeXS\": \"0.125rem\",\n \"twoXS\": \"0.25rem\",\n \"xs\": \"0.375rem\",\n \"sm\": \"0.5rem\",\n \"md\": \"0.75rem\",\n \"lg\": \"1rem\",\n \"xl\": \"1.25rem\",\n \"twoXL\": \"1.5rem\",\n \"threeXL\": \"2rem\",\n \"fourXL\": \"4rem\"\n },\n \"zIndices\": {\n \"hide\": -1,\n \"auto\": \"auto\",\n \"base\": 0,\n \"priority\": 1,\n \"sidebar\": 100,\n \"menuButton\": 110,\n \"balloons\": 1000000,\n \"header\": 999990,\n \"sidebarMobile\": 999995,\n \"popupMenu\": 1000040,\n \"fullscreenWrapper\": 1000050,\n \"tablePortal\": 1000110,\n \"bottom\": 99,\n \"cacheSpinner\": 101,\n \"toast\": 100,\n \"vegaTooltips\": 1000060\n }\n },\n \"basewebTheme\": {\n \"animation\": {\n \"timing100\": \"100ms\",\n \"timing200\": \"200ms\",\n \"timing300\": \"300ms\",\n \"timing400\": \"400ms\",\n \"timing500\": \"500ms\",\n \"timing600\": \"600ms\",\n \"timing700\": \"700ms\",\n \"timing800\": \"800ms\",\n \"timing900\": \"900ms\",\n \"timing1000\": \"1000ms\",\n \"easeInCurve\": \"cubic-bezier(.8, .2, .6, 1)\",\n \"easeOutCurve\": \"cubic-bezier(.2, .8, .4, 1)\",\n \"easeInOutCurve\": \"cubic-bezier(0.4, 0, 0.2, 1)\",\n \"easeInQuinticCurve\": \"cubic-bezier(0.755, 0.05, 0.855, 0.06)\",\n \"easeOutQuinticCurve\": \"cubic-bezier(0.23, 1, 0.32, 1)\",\n \"easeInOutQuinticCurve\": \"cubic-bezier(0.86, 0, 0.07, 1)\",\n \"linearCurve\": \"cubic-bezier(0, 0, 1, 1)\"\n },\n \"borders\": {\n \"border100\": {\n \"borderColor\": \"hsla(0, 0%, 0%, 0.04)\",\n \"borderStyle\": \"solid\",\n \"borderWidth\": \"1px\"\n },\n \"border200\": {\n \"borderColor\": \"hsla(0, 0%, 0%, 0.08)\",\n \"borderStyle\": \"solid\",\n \"borderWidth\": \"1px\"\n },\n \"border300\": {\n \"borderColor\": \"hsla(0, 0%, 0%, 0.12)\",\n \"borderStyle\": \"solid\",\n \"borderWidth\": \"1px\"\n },\n \"border400\": {\n \"borderColor\": \"hsla(0, 0%, 0%, 0.16)\",\n \"borderStyle\": \"solid\",\n \"borderWidth\": \"1px\"\n },\n \"border500\": {\n \"borderColor\": \"hsla(0, 0%, 0%, 0.2)\",\n \"borderStyle\": \"solid\",\n \"borderWidth\": \"1px\"\n },\n \"border600\": {\n \"borderColor\": \"hsla(0, 0%, 0%, 0.24)\",\n \"borderStyle\": \"solid\",\n \"borderWidth\": \"1px\"\n },\n \"radius100\": \"0.5rem\",\n \"radius200\": \"0.5rem\",\n \"radius300\": \"0.5rem\",\n \"radius400\": \"0.5rem\",\n \"radius500\": \"0.5rem\",\n \"useRoundedCorners\": true,\n \"buttonBorderRadiusMini\": \"0.25rem\",\n \"buttonBorderRadius\": \"0.5rem\",\n \"checkboxBorderRadius\": \"0.25rem\",\n \"inputBorderRadiusMini\": \"0.25rem\",\n \"inputBorderRadius\": \"0.5rem\",\n \"popoverBorderRadius\": \"0.5rem\",\n \"surfaceBorderRadius\": \"0.5rem\",\n \"tagBorderRadius\": \"0.25rem\"\n },\n \"breakpoints\": {\n \"small\": 320,\n \"medium\": 600,\n \"large\": 1136\n },\n \"colors\": {\n \"primaryA\": \"#ff4b4b\",\n \"primaryB\": \"#FFFFFF\",\n \"primary\": \"#ff4b4b\",\n \"primary50\": \"#F6F6F6\",\n \"primary100\": \"#ff4b4b\",\n \"primary200\": \"#ff4b4b\",\n \"primary300\": \"#ff4b4b\",\n \"primary400\": \"#ff4b4b\",\n \"primary500\": \"#ff4b4b\",\n \"primary600\": \"#ff4b4b\",\n \"primary700\": \"#ff4b4b\",\n \"accent\": \"rgba(255, 75, 75, 0.5)\",\n \"accent50\": \"#EFF3FE\",\n \"accent100\": \"#D4E2FC\",\n \"accent200\": \"#A0BFF8\",\n \"accent300\": \"#5B91F5\",\n \"accent400\": \"#276EF1\",\n \"accent500\": \"#1E54B7\",\n \"accent600\": \"#174291\",\n \"accent700\": \"#102C60\",\n \"negative\": \"#E11900\",\n \"negative50\": \"#FFEFED\",\n \"negative100\": \"#FED7D2\",\n \"negative200\": \"#F1998E\",\n \"negative300\": \"#E85C4A\",\n \"negative400\": \"#E11900\",\n \"negative500\": \"#AB1300\",\n \"negative600\": \"#870F00\",\n \"negative700\": \"#5A0A00\",\n \"warning\": \"#FFC043\",\n \"warning50\": \"#FFFAF0\",\n \"warning100\": \"#FFF2D9\",\n \"warning200\": \"#FFE3AC\",\n \"warning300\": \"#FFCF70\",\n \"warning400\": \"#FFC043\",\n \"warning500\": \"#BC8B2C\",\n \"warning600\": \"#996F00\",\n \"warning700\": \"#674D1B\",\n \"positive\": \"#03703C\",\n \"positive50\": \"#E6F2ED\",\n \"positive100\": \"#ADDEC9\",\n \"positive200\": \"#66D19E\",\n \"positive300\": \"#06C167\",\n \"positive400\": \"#048848\",\n \"positive500\": \"#03703C\",\n \"positive600\": \"#03582F\",\n \"positive700\": \"#10462D\",\n \"white\": \"#ffffff\",\n \"black\": \"#000000\",\n \"mono100\": \"#ffffff\",\n \"mono200\": \"#f0f2f6\",\n \"mono300\": \"#e6eaf1\",\n \"mono400\": \"#e6eaf1\",\n \"mono500\": \"#a3a8b8\",\n \"mono600\": \"rgba(49, 51, 63, 0.4)\",\n \"mono700\": \"#a3a8b8\",\n \"mono800\": \"#31333F\",\n \"mono900\": \"#31333F\",\n \"mono1000\": \"#000000\",\n \"ratingInactiveFill\": \"#EEEEEE\",\n \"ratingStroke\": \"#CBCBCB\",\n \"bannerActionLowInfo\": \"#D4E2FC\",\n \"bannerActionLowNegative\": \"#FED7D2\",\n \"bannerActionLowPositive\": \"#ADDEC9\",\n \"bannerActionLowWarning\": \"#FFE3AC\",\n \"bannerActionHighInfo\": \"#1E54B7\",\n \"bannerActionHighNegative\": \"#AB1300\",\n \"bannerActionHighPositive\": \"#03703C\",\n \"bannerActionHighWarning\": \"#FFE3AC\",\n \"buttonPrimaryFill\": \"#000000\",\n \"buttonPrimaryText\": \"#FFFFFF\",\n \"buttonPrimaryHover\": \"#ff4b4b\",\n \"buttonPrimaryActive\": \"#ff4b4b\",\n \"buttonPrimarySelectedFill\": \"#ff4b4b\",\n \"buttonPrimarySelectedText\": \"#FFFFFF\",\n \"buttonPrimarySpinnerForeground\": \"#276EF1\",\n \"buttonPrimarySpinnerBackground\": \"#FFFFFF\",\n \"buttonSecondaryFill\": \"#ff4b4b\",\n \"buttonSecondaryText\": \"#000000\",\n \"buttonSecondaryHover\": \"#ff4b4b\",\n \"buttonSecondaryActive\": \"#ff4b4b\",\n \"buttonSecondarySelectedFill\": \"#ff4b4b\",\n \"buttonSecondarySelectedText\": \"#000000\",\n \"buttonSecondarySpinnerForeground\": \"#276EF1\",\n \"buttonSecondarySpinnerBackground\": \"#FFFFFF\",\n \"buttonTertiaryFill\": \"transparent\",\n \"buttonTertiaryText\": \"#000000\",\n \"buttonTertiaryHover\": \"#F6F6F6\",\n \"buttonTertiaryActive\": \"#ff4b4b\",\n \"buttonTertiarySelectedFill\": \"#ff4b4b\",\n \"buttonTertiarySelectedText\": \"#000000\",\n \"buttonTertiaryDisabledActiveFill\": \"#F6F6F6\",\n \"buttonTertiaryDisabledActiveText\": \"rgba(49, 51, 63, 0.4)\",\n \"buttonTertiarySpinnerForeground\": \"#276EF1\",\n \"buttonTertiarySpinnerBackground\": \"#ff4b4b\",\n \"buttonDisabledFill\": \"hsla(0, 0%, 100%, 1)\",\n \"buttonDisabledText\": \"rgba(49, 51, 63, 0.4)\",\n \"buttonDisabledActiveFill\": \"#a3a8b8\",\n \"buttonDisabledActiveText\": \"#ffffff\",\n \"buttonDisabledSpinnerForeground\": \"rgba(49, 51, 63, 0.4)\",\n \"buttonDisabledSpinnerBackground\": \"#d5dae5\",\n \"breadcrumbsText\": \"#000000\",\n \"breadcrumbsSeparatorFill\": \"#a3a8b8\",\n \"calendarBackground\": \"#ffffff\",\n \"calendarForeground\": \"#31333F\",\n \"calendarForegroundDisabled\": \"#a3a8b8\",\n \"calendarHeaderBackground\": \"#f0f2f6\",\n \"calendarHeaderForeground\": \"#31333F\",\n \"calendarHeaderBackgroundActive\": \"#f0f2f6\",\n \"calendarHeaderForegroundDisabled\": \"#d5dae5\",\n \"calendarDayForegroundPseudoSelected\": \"#31333F\",\n \"calendarDayBackgroundPseudoSelectedHighlighted\": \"#ff4b4b\",\n \"calendarDayForegroundPseudoSelectedHighlighted\": \"#31333F\",\n \"calendarDayBackgroundSelected\": \"#ff4b4b\",\n \"calendarDayForegroundSelected\": \"#ffffff\",\n \"calendarDayBackgroundSelectedHighlighted\": \"#ff4b4b\",\n \"calendarDayForegroundSelectedHighlighted\": \"#ffffff\",\n \"comboboxListItemFocus\": \"#f0f2f6\",\n \"comboboxListItemHover\": \"#e6eaf1\",\n \"fileUploaderBackgroundColor\": \"#f0f2f6\",\n \"fileUploaderBackgroundColorActive\": \"#F6F6F6\",\n \"fileUploaderBorderColorActive\": \"#000000\",\n \"fileUploaderBorderColorDefault\": \"#a3a8b8\",\n \"fileUploaderMessageColor\": \"#31333F\",\n \"linkText\": \"#000000\",\n \"linkVisited\": \"#ff4b4b\",\n \"linkHover\": \"#ff4b4b\",\n \"linkActive\": \"#ff4b4b\",\n \"listHeaderFill\": \"#FFFFFF\",\n \"listBodyFill\": \"#FFFFFF\",\n \"progressStepsCompletedText\": \"#FFFFFF\",\n \"progressStepsCompletedFill\": \"#000000\",\n \"progressStepsActiveText\": \"#FFFFFF\",\n \"progressStepsActiveFill\": \"#000000\",\n \"toggleFill\": \"#FFFFFF\",\n \"toggleFillChecked\": \"#000000\",\n \"toggleFillDisabled\": \"rgba(49, 51, 63, 0.4)\",\n \"toggleTrackFill\": \"#d5dae5\",\n \"toggleTrackFillDisabled\": \"#f0f2f6\",\n \"tickFill\": \"hsla(0, 0%, 100%, 1)\",\n \"tickFillHover\": \"#f0f2f6\",\n \"tickFillActive\": \"#f0f2f6\",\n \"tickFillSelected\": \"#ff4b4b\",\n \"tickFillSelectedHover\": \"#ff4b4b\",\n \"tickFillSelectedHoverActive\": \"#ff4b4b\",\n \"tickFillError\": \"#FFEFED\",\n \"tickFillErrorHover\": \"#FED7D2\",\n \"tickFillErrorHoverActive\": \"#F1998E\",\n \"tickFillErrorSelected\": \"#E11900\",\n \"tickFillErrorSelectedHover\": \"#AB1300\",\n \"tickFillErrorSelectedHoverActive\": \"#870F00\",\n \"tickFillDisabled\": \"rgba(49, 51, 63, 0.4)\",\n \"tickBorder\": \"#a3a8b8\",\n \"tickBorderError\": \"#E11900\",\n \"tickMarkFill\": \"#f0f2f6\",\n \"tickMarkFillError\": \"#FFFFFF\",\n \"tickMarkFillDisabled\": \"hsla(0, 0%, 100%, 1)\",\n \"sliderTrackFill\": \"#d5dae5\",\n \"sliderHandleFill\": \"#000000\",\n \"sliderHandleFillDisabled\": \"#ff4b4b\",\n \"sliderHandleInnerFill\": \"#d5dae5\",\n \"sliderTrackFillHover\": \"#a3a8b8\",\n \"sliderTrackFillActive\": \"rgba(49, 51, 63, 0.4)\",\n \"sliderTrackFillDisabled\": \"#f0f2f6\",\n \"sliderHandleInnerFillDisabled\": \"#d5dae5\",\n \"sliderHandleInnerFillSelectedHover\": \"#000000\",\n \"sliderHandleInnerFillSelectedActive\": \"#ff4b4b\",\n \"inputBorder\": \"#f0f2f6\",\n \"inputFill\": \"#f0f2f6\",\n \"inputFillError\": \"#FFEFED\",\n \"inputFillDisabled\": \"#f0f2f6\",\n \"inputFillActive\": \"#f0f2f6\",\n \"inputFillPositive\": \"#E6F2ED\",\n \"inputTextDisabled\": \"rgba(49, 51, 63, 0.4)\",\n \"inputBorderError\": \"#F1998E\",\n \"inputBorderPositive\": \"#66D19E\",\n \"inputEnhancerFill\": \"#e6eaf1\",\n \"inputEnhancerFillDisabled\": \"#f0f2f6\",\n \"inputEnhancerTextDisabled\": \"rgba(49, 51, 63, 0.4)\",\n \"inputPlaceholder\": \"rgba(49, 51, 63, 0.6)\",\n \"inputPlaceholderDisabled\": \"rgba(49, 51, 63, 0.4)\",\n \"menuFill\": \"#ffffff\",\n \"menuFillHover\": \"#f0f2f6\",\n \"menuFontDefault\": \"#31333F\",\n \"menuFontDisabled\": \"#a3a8b8\",\n \"menuFontHighlighted\": \"#31333F\",\n \"menuFontSelected\": \"#31333F\",\n \"modalCloseColor\": \"#31333F\",\n \"modalCloseColorHover\": \"#31333F\",\n \"modalCloseColorFocus\": \"#31333F\",\n \"tabBarFill\": \"#f0f2f6\",\n \"tabColor\": \"#31333F\",\n \"notificationInfoBackground\": \"rgba(28, 131, 225, 0.1)\",\n \"notificationInfoText\": \"#004280\",\n \"notificationPositiveBackground\": \"rgba(33, 195, 84, 0.1)\",\n \"notificationPositiveText\": \"#177233\",\n \"notificationWarningBackground\": \"rgba(255, 227, 18, 0.1)\",\n \"notificationWarningText\": \"#926C05\",\n \"notificationNegativeBackground\": \"rgba(255, 43, 43, 0.09)\",\n \"notificationNegativeText\": \"#7d353b\",\n \"tagFontDisabledRampUnit\": \"100\",\n \"tagSolidFontRampUnit\": \"0\",\n \"tagSolidRampUnit\": \"400\",\n \"tagOutlinedFontRampUnit\": \"400\",\n \"tagOutlinedRampUnit\": \"200\",\n \"tagSolidHoverRampUnit\": \"50\",\n \"tagSolidActiveRampUnit\": \"100\",\n \"tagSolidDisabledRampUnit\": \"50\",\n \"tagSolidFontHoverRampUnit\": \"500\",\n \"tagLightRampUnit\": \"50\",\n \"tagLightHoverRampUnit\": \"100\",\n \"tagLightActiveRampUnit\": \"100\",\n \"tagLightFontRampUnit\": \"500\",\n \"tagLightFontHoverRampUnit\": \"500\",\n \"tagOutlinedHoverRampUnit\": \"50\",\n \"tagOutlinedActiveRampUnit\": \"0\",\n \"tagOutlinedFontHoverRampUnit\": \"400\",\n \"tagNeutralFontDisabled\": \"rgba(49, 51, 63, 0.4)\",\n \"tagNeutralOutlinedDisabled\": \"#e6eaf1\",\n \"tagNeutralSolidFont\": \"#FFFFFF\",\n \"tagNeutralSolidBackground\": \"#000000\",\n \"tagNeutralOutlinedBackground\": \"rgba(49, 51, 63, 0.4)\",\n \"tagNeutralOutlinedFont\": \"#000000\",\n \"tagNeutralSolidHover\": \"#e6eaf1\",\n \"tagNeutralSolidActive\": \"#e6eaf1\",\n \"tagNeutralSolidDisabled\": \"#f0f2f6\",\n \"tagNeutralSolidFontHover\": \"#31333F\",\n \"tagNeutralLightBackground\": \"#e6eaf1\",\n \"tagNeutralLightHover\": \"#e6eaf1\",\n \"tagNeutralLightActive\": \"#e6eaf1\",\n \"tagNeutralLightDisabled\": \"#f0f2f6\",\n \"tagNeutralLightFont\": \"#31333F\",\n \"tagNeutralLightFontHover\": \"#31333F\",\n \"tagNeutralOutlinedActive\": \"#31333F\",\n \"tagNeutralOutlinedFontHover\": \"#31333F\",\n \"tagNeutralOutlinedHover\": \"rgba(0, 0, 0, 0.08)\",\n \"tagPrimaryFontDisabled\": \"rgba(49, 51, 63, 0.4)\",\n \"tagPrimaryOutlinedDisabled\": \"transparent\",\n \"tagPrimarySolidFont\": \"#FFFFFF\",\n \"tagPrimarySolidBackground\": \"#ff4b4b\",\n \"tagPrimaryOutlinedFontHover\": \"#000000\",\n \"tagPrimaryOutlinedFont\": \"#000000\",\n \"tagPrimarySolidHover\": \"#ff4b4b\",\n \"tagPrimarySolidActive\": \"#ff4b4b\",\n \"tagPrimarySolidDisabled\": \"#F6F6F6\",\n \"tagPrimarySolidFontHover\": \"#ff4b4b\",\n \"tagPrimaryLightBackground\": \"#F6F6F6\",\n \"tagPrimaryLightHover\": \"#ff4b4b\",\n \"tagPrimaryLightActive\": \"#ff4b4b\",\n \"tagPrimaryLightDisabled\": \"#F6F6F6\",\n \"tagPrimaryLightFont\": \"#ff4b4b\",\n \"tagPrimaryLightFontHover\": \"#ff4b4b\",\n \"tagPrimaryOutlinedActive\": \"#ff4b4b\",\n \"tagPrimaryOutlinedHover\": \"rgba(0, 0, 0, 0.08)\",\n \"tagPrimaryOutlinedBackground\": \"#ff4b4b\",\n \"tagAccentFontDisabled\": \"#A0BFF8\",\n \"tagAccentOutlinedDisabled\": \"#A0BFF8\",\n \"tagAccentSolidFont\": \"#FFFFFF\",\n \"tagAccentSolidBackground\": \"#276EF1\",\n \"tagAccentOutlinedBackground\": \"#A0BFF8\",\n \"tagAccentOutlinedFont\": \"#276EF1\",\n \"tagAccentSolidHover\": \"#EFF3FE\",\n \"tagAccentSolidActive\": \"#D4E2FC\",\n \"tagAccentSolidDisabled\": \"#EFF3FE\",\n \"tagAccentSolidFontHover\": \"#1E54B7\",\n \"tagAccentLightBackground\": \"#EFF3FE\",\n \"tagAccentLightHover\": \"#D4E2FC\",\n \"tagAccentLightActive\": \"#D4E2FC\",\n \"tagAccentLightDisabled\": \"#EFF3FE\",\n \"tagAccentLightFont\": \"#1E54B7\",\n \"tagAccentLightFontHover\": \"#1E54B7\",\n \"tagAccentOutlinedActive\": \"#174291\",\n \"tagAccentOutlinedFontHover\": \"#276EF1\",\n \"tagAccentOutlinedHover\": \"rgba(0, 0, 0, 0.08)\",\n \"tagPositiveFontDisabled\": \"#66D19E\",\n \"tagPositiveOutlinedDisabled\": \"#66D19E\",\n \"tagPositiveSolidFont\": \"#FFFFFF\",\n \"tagPositiveSolidBackground\": \"#048848\",\n \"tagPositiveOutlinedBackground\": \"#66D19E\",\n \"tagPositiveOutlinedFont\": \"#048848\",\n \"tagPositiveSolidHover\": \"#E6F2ED\",\n \"tagPositiveSolidActive\": \"#ADDEC9\",\n \"tagPositiveSolidDisabled\": \"#E6F2ED\",\n \"tagPositiveSolidFontHover\": \"#03703C\",\n \"tagPositiveLightBackground\": \"#E6F2ED\",\n \"tagPositiveLightHover\": \"#ADDEC9\",\n \"tagPositiveLightActive\": \"#ADDEC9\",\n \"tagPositiveLightDisabled\": \"#E6F2ED\",\n \"tagPositiveLightFont\": \"#03703C\",\n \"tagPositiveLightFontHover\": \"#03703C\",\n \"tagPositiveOutlinedActive\": \"#03582F\",\n \"tagPositiveOutlinedFontHover\": \"#048848\",\n \"tagPositiveOutlinedHover\": \"rgba(0, 0, 0, 0.08)\",\n \"tagWarningFontDisabled\": \"#FFCF70\",\n \"tagWarningOutlinedDisabled\": \"#FFCF70\",\n \"tagWarningSolidFont\": \"#674D1B\",\n \"tagWarningSolidBackground\": \"#FFC043\",\n \"tagWarningOutlinedBackground\": \"#FFCF70\",\n \"tagWarningOutlinedFont\": \"#996F00\",\n \"tagWarningSolidHover\": \"#FFFAF0\",\n \"tagWarningSolidActive\": \"#FFF2D9\",\n \"tagWarningSolidDisabled\": \"#FFFAF0\",\n \"tagWarningSolidFontHover\": \"#BC8B2C\",\n \"tagWarningLightBackground\": \"#FFFAF0\",\n \"tagWarningLightHover\": \"#FFF2D9\",\n \"tagWarningLightActive\": \"#FFF2D9\",\n \"tagWarningLightDisabled\": \"#FFFAF0\",\n \"tagWarningLightFont\": \"#BC8B2C\",\n \"tagWarningLightFontHover\": \"#BC8B2C\",\n \"tagWarningOutlinedActive\": \"#996F00\",\n \"tagWarningOutlinedFontHover\": \"#996F00\",\n \"tagWarningOutlinedHover\": \"rgba(0, 0, 0, 0.08)\",\n \"tagNegativeFontDisabled\": \"#F1998E\",\n \"tagNegativeOutlinedDisabled\": \"#F1998E\",\n \"tagNegativeSolidFont\": \"#FFFFFF\",\n \"tagNegativeSolidBackground\": \"#E11900\",\n \"tagNegativeOutlinedBackground\": \"#F1998E\",\n \"tagNegativeOutlinedFont\": \"#E11900\",\n \"tagNegativeSolidHover\": \"#FFEFED\",\n \"tagNegativeSolidActive\": \"#FED7D2\",\n \"tagNegativeSolidDisabled\": \"#FFEFED\",\n \"tagNegativeSolidFontHover\": \"#AB1300\",\n \"tagNegativeLightBackground\": \"#FFEFED\",\n \"tagNegativeLightHover\": \"#FED7D2\",\n \"tagNegativeLightActive\": \"#FED7D2\",\n \"tagNegativeLightDisabled\": \"#FFEFED\",\n \"tagNegativeLightFont\": \"#AB1300\",\n \"tagNegativeLightFontHover\": \"#AB1300\",\n \"tagNegativeOutlinedActive\": \"#870F00\",\n \"tagNegativeOutlinedFontHover\": \"#E11900\",\n \"tagNegativeOutlinedHover\": \"rgba(0, 0, 0, 0.08)\",\n \"tableHeadBackgroundColor\": \"#ffffff\",\n \"tableBackground\": \"#ffffff\",\n \"tableStripedBackground\": \"#f0f2f6\",\n \"tableFilter\": \"rgba(49, 51, 63, 0.4)\",\n \"tableFilterHeading\": \"#a3a8b8\",\n \"tableFilterBackground\": \"#ffffff\",\n \"tableFilterFooterBackground\": \"#f0f2f6\",\n \"toastText\": \"#FFFFFF\",\n \"toastPrimaryText\": \"#FFFFFF\",\n \"toastInfoBackground\": \"#276EF1\",\n \"toastInfoText\": \"#FFFFFF\",\n \"toastPositiveBackground\": \"#048848\",\n \"toastPositiveText\": \"#FFFFFF\",\n \"toastWarningBackground\": \"#FFC043\",\n \"toastWarningText\": \"#000000\",\n \"toastNegativeBackground\": \"#E11900\",\n \"toastNegativeText\": \"#FFFFFF\",\n \"spinnerTrackFill\": \"#31333F\",\n \"progressbarTrackFill\": \"#f0f2f6\",\n \"tooltipBackground\": \"#31333F\",\n \"tooltipText\": \"#ffffff\",\n \"backgroundPrimary\": \"#ffffff\",\n \"backgroundSecondary\": \"#f0f2f6\",\n \"backgroundTertiary\": \"#ffffff\",\n \"backgroundInversePrimary\": \"#000000\",\n \"backgroundInverseSecondary\": \"#1F1F1F\",\n \"contentPrimary\": \"#31333F\",\n \"contentSecondary\": \"#545454\",\n \"contentTertiary\": \"#6B6B6B\",\n \"contentInversePrimary\": \"#FFFFFF\",\n \"contentInverseSecondary\": \"#CBCBCB\",\n \"contentInverseTertiary\": \"#AFAFAF\",\n \"borderOpaque\": \"rgba(151, 166, 195, 0.25)\",\n \"borderTransparent\": \"rgba(0, 0, 0, 0.08)\",\n \"borderSelected\": \"#ff4b4b\",\n \"borderInverseOpaque\": \"#333333\",\n \"borderInverseTransparent\": \"rgba(255, 255, 255, 0.2)\",\n \"borderInverseSelected\": \"#FFFFFF\",\n \"backgroundStateDisabled\": \"#F6F6F6\",\n \"backgroundOverlayDark\": \"rgba(0, 0, 0, 0.3)\",\n \"backgroundOverlayLight\": \"rgba(0, 0, 0, 0.08)\",\n \"backgroundOverlayArt\": \"rgba(0, 0, 0, 0.00)\",\n \"backgroundAccent\": \"#276EF1\",\n \"backgroundNegative\": \"#E11900\",\n \"backgroundWarning\": \"#FFC043\",\n \"backgroundPositive\": \"#048848\",\n \"backgroundLightAccent\": \"#EFF3FE\",\n \"backgroundLightNegative\": \"#FFEFED\",\n \"backgroundLightWarning\": \"#FFFAF0\",\n \"backgroundLightPositive\": \"#E6F2ED\",\n \"backgroundAlwaysDark\": \"#000000\",\n \"backgroundAlwaysLight\": \"#FFFFFF\",\n \"contentStateDisabled\": \"#AFAFAF\",\n \"contentAccent\": \"#276EF1\",\n \"contentOnColor\": \"#FFFFFF\",\n \"contentOnColorInverse\": \"#000000\",\n \"contentNegative\": \"#E11900\",\n \"contentWarning\": \"#996F00\",\n \"contentPositive\": \"#048848\",\n \"borderStateDisabled\": \"#F6F6F6\",\n \"borderAccent\": \"#276EF1\",\n \"borderAccentLight\": \"#A0BFF8\",\n \"borderNegative\": \"#F1998E\",\n \"borderWarning\": \"#FFE3AC\",\n \"borderPositive\": \"#66D19E\",\n \"safety\": \"#276EF1\",\n \"eatsGreen400\": \"#048848\",\n \"freightBlue400\": \"#0E1FC1\",\n \"jumpRed400\": \"#E11900\",\n \"rewardsTier1\": \"#276EF1\",\n \"rewardsTier2\": \"#FFC043\",\n \"rewardsTier3\": \"#8EA3AD\",\n \"rewardsTier4\": \"#000000\",\n \"membership\": \"#996F00\",\n \"datepickerBackground\": \"#ffffff\",\n \"inputEnhanceFill\": \"#f0f2f6\"\n },\n \"direction\": \"auto\",\n \"grid\": {\n \"columns\": [\n 4,\n 8,\n 12\n ],\n \"gutters\": [\n 16,\n 36,\n 36\n ],\n \"margins\": [\n 16,\n 36,\n 64\n ],\n \"gaps\": 0,\n \"unit\": \"px\",\n \"maxWidth\": 1280\n },\n \"lighting\": {\n \"shadow400\": \"0 1px 4px hsla(0, 0%, 0%, 0.16)\",\n \"shadow500\": \"0 2px 8px hsla(0, 0%, 0%, 0.16)\",\n \"shadow600\": \"0 4px 16px hsla(0, 0%, 0%, 0.16)\",\n \"shadow700\": \"0 8px 24px hsla(0, 0%, 0%, 0.16)\",\n \"overlay0\": \"inset 0 0 0 1000px hsla(0, 0%, 0%, 0)\",\n \"overlay100\": \"inset 0 0 0 1000px hsla(0, 0%, 0%, 0.04)\",\n \"overlay200\": \"inset 0 0 0 1000px hsla(0, 0%, 0%, 0.08)\",\n \"overlay300\": \"inset 0 0 0 1000px hsla(0, 0%, 0%, 0.12)\",\n \"overlay400\": \"inset 0 0 0 1000px hsla(0, 0%, 0%, 0.16)\",\n \"overlay500\": \"inset 0 0 0 1000px hsla(0, 0%, 0%, 0.2)\",\n \"overlay600\": \"inset 0 0 0 1000px hsla(0, 0%, 0%, 0.24)\",\n \"shallowAbove\": \"0px -4px 16px rgba(0, 0, 0, 0.12)\",\n \"shallowBelow\": \"0px 4px 16px rgba(0, 0, 0, 0.12)\",\n \"deepAbove\": \"0px -16px 48px rgba(0, 0, 0, 0.22)\",\n \"deepBelow\": \"0px 16px 48px rgba(0, 0, 0, 0.22)\"\n },\n \"mediaQuery\": {\n \"small\": \"@media screen and (min-width: 320px)\",\n \"medium\": \"@media screen and (min-width: 600px)\",\n \"large\": \"@media screen and (min-width: 1136px)\"\n },\n \"sizing\": {\n \"scale0\": \"2px\",\n \"scale100\": \"4px\",\n \"scale200\": \"6px\",\n \"scale300\": \"8px\",\n \"scale400\": \"10px\",\n \"scale500\": \"12px\",\n \"scale550\": \"14px\",\n \"scale600\": \"16px\",\n \"scale650\": \"18px\",\n \"scale700\": \"20px\",\n \"scale750\": \"22px\",\n \"scale800\": \"24px\",\n \"scale850\": \"28px\",\n \"scale900\": \"32px\",\n \"scale950\": \"36px\",\n \"scale1000\": \"40px\",\n \"scale1200\": \"48px\",\n \"scale1400\": \"56px\",\n \"scale1600\": \"64px\",\n \"scale2400\": \"96px\",\n \"scale3200\": \"128px\",\n \"scale4800\": \"192px\"\n },\n \"typography\": {\n \"font100\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"12px\",\n \"fontWeight\": \"normal\",\n \"lineHeight\": \"20px\"\n },\n \"font150\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"1rem\",\n \"fontWeight\": \"normal\",\n \"lineHeight\": 1.6,\n \"fontSizeSm\": \"14px\",\n \"lineHeightTight\": 1.25\n },\n \"font200\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"1rem\",\n \"fontWeight\": \"normal\",\n \"lineHeight\": 1.6,\n \"fontSizeSm\": \"14px\",\n \"lineHeightTight\": 1.25\n },\n \"font250\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"1rem\",\n \"fontWeight\": \"normal\",\n \"lineHeight\": 1.6,\n \"fontSizeSm\": \"14px\",\n \"lineHeightTight\": 1.25\n },\n \"font300\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"1rem\",\n \"fontWeight\": \"normal\",\n \"lineHeight\": 1.6,\n \"fontSizeSm\": \"14px\",\n \"lineHeightTight\": 1.25\n },\n \"font350\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"1rem\",\n \"fontWeight\": \"normal\",\n \"lineHeight\": 1.6,\n \"fontSizeSm\": \"14px\",\n \"lineHeightTight\": 1.25\n },\n \"font400\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"1rem\",\n \"fontWeight\": \"normal\",\n \"lineHeight\": 1.6,\n \"fontSizeSm\": \"14px\",\n \"lineHeightTight\": 1.25\n },\n \"font450\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"1rem\",\n \"fontWeight\": \"normal\",\n \"lineHeight\": 1.6,\n \"fontSizeSm\": \"14px\",\n \"lineHeightTight\": 1.25\n },\n \"font550\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"20px\",\n \"fontWeight\": 700,\n \"lineHeight\": \"28px\"\n },\n \"font650\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"24px\",\n \"fontWeight\": 700,\n \"lineHeight\": \"32px\"\n },\n \"font750\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"28px\",\n \"fontWeight\": 700,\n \"lineHeight\": \"36px\"\n },\n \"font850\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"32px\",\n \"fontWeight\": 700,\n \"lineHeight\": \"40px\"\n },\n \"font950\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"36px\",\n \"fontWeight\": 700,\n \"lineHeight\": \"44px\"\n },\n \"font1050\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"40px\",\n \"fontWeight\": 700,\n \"lineHeight\": \"52px\"\n },\n \"font1150\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"36px\",\n \"fontWeight\": 700,\n \"lineHeight\": \"44px\"\n },\n \"font1250\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"44px\",\n \"fontWeight\": 700,\n \"lineHeight\": \"52px\"\n },\n \"font1350\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"52px\",\n \"fontWeight\": 700,\n \"lineHeight\": \"64px\"\n },\n \"font1450\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"96px\",\n \"fontWeight\": 700,\n \"lineHeight\": \"112px\"\n },\n \"ParagraphXSmall\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"12px\",\n \"fontWeight\": \"normal\",\n \"lineHeight\": \"20px\"\n },\n \"ParagraphSmall\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"1rem\",\n \"fontWeight\": \"normal\",\n \"lineHeight\": 1.6,\n \"fontSizeSm\": \"14px\",\n \"lineHeightTight\": 1.25\n },\n \"ParagraphMedium\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"1rem\",\n \"fontWeight\": \"normal\",\n \"lineHeight\": 1.6,\n \"fontSizeSm\": \"14px\",\n \"lineHeightTight\": 1.25\n },\n \"ParagraphLarge\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"1rem\",\n \"fontWeight\": \"normal\",\n \"lineHeight\": 1.6,\n \"fontSizeSm\": \"14px\",\n \"lineHeightTight\": 1.25\n },\n \"LabelXSmall\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"1rem\",\n \"fontWeight\": \"normal\",\n \"lineHeight\": 1.6,\n \"fontSizeSm\": \"14px\",\n \"lineHeightTight\": 1.25\n },\n \"LabelSmall\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"1rem\",\n \"fontWeight\": \"normal\",\n \"lineHeight\": 1.6,\n \"fontSizeSm\": \"14px\",\n \"lineHeightTight\": 1.25\n },\n \"LabelMedium\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"1rem\",\n \"fontWeight\": \"normal\",\n \"lineHeight\": 1.6,\n \"fontSizeSm\": \"14px\",\n \"lineHeightTight\": 1.25\n },\n \"LabelLarge\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"1rem\",\n \"fontWeight\": \"normal\",\n \"lineHeight\": 1.6,\n \"fontSizeSm\": \"14px\",\n \"lineHeightTight\": 1.25\n },\n \"HeadingXSmall\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"20px\",\n \"fontWeight\": 700,\n \"lineHeight\": \"28px\"\n },\n \"HeadingSmall\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"24px\",\n \"fontWeight\": 700,\n \"lineHeight\": \"32px\"\n },\n \"HeadingMedium\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"28px\",\n \"fontWeight\": 700,\n \"lineHeight\": \"36px\"\n },\n \"HeadingLarge\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"32px\",\n \"fontWeight\": 700,\n \"lineHeight\": \"40px\"\n },\n \"HeadingXLarge\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"36px\",\n \"fontWeight\": 700,\n \"lineHeight\": \"44px\"\n },\n \"HeadingXXLarge\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"40px\",\n \"fontWeight\": 700,\n \"lineHeight\": \"52px\"\n },\n \"DisplayXSmall\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"36px\",\n \"fontWeight\": 700,\n \"lineHeight\": \"44px\"\n },\n \"DisplaySmall\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"44px\",\n \"fontWeight\": 700,\n \"lineHeight\": \"52px\"\n },\n \"DisplayMedium\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"52px\",\n \"fontWeight\": 700,\n \"lineHeight\": \"64px\"\n },\n \"DisplayLarge\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"96px\",\n \"fontWeight\": 700,\n \"lineHeight\": \"112px\"\n },\n \"MonoParagraphXSmall\": {\n \"fontFamily\": \"\\\"Lucida Console\\\", Monaco, monospace\",\n \"fontSize\": \"12px\",\n \"fontWeight\": \"normal\",\n \"lineHeight\": \"20px\"\n },\n \"MonoParagraphSmall\": {\n \"fontFamily\": \"\\\"Lucida Console\\\", Monaco, monospace\",\n \"fontSize\": \"14px\",\n \"fontWeight\": \"normal\",\n \"lineHeight\": \"20px\"\n },\n \"MonoParagraphMedium\": {\n \"fontFamily\": \"\\\"Lucida Console\\\", Monaco, monospace\",\n \"fontSize\": \"16px\",\n \"fontWeight\": \"normal\",\n \"lineHeight\": \"24px\"\n },\n \"MonoParagraphLarge\": {\n \"fontFamily\": \"\\\"Lucida Console\\\", Monaco, monospace\",\n \"fontSize\": \"18px\",\n \"fontWeight\": \"normal\",\n \"lineHeight\": \"28px\"\n },\n \"MonoLabelXSmall\": {\n \"fontFamily\": \"\\\"Lucida Console\\\", Monaco, monospace\",\n \"fontSize\": \"12px\",\n \"fontWeight\": 500,\n \"lineHeight\": \"16px\"\n },\n \"MonoLabelSmall\": {\n \"fontFamily\": \"\\\"Lucida Console\\\", Monaco, monospace\",\n \"fontSize\": \"14px\",\n \"fontWeight\": 500,\n \"lineHeight\": \"16px\"\n },\n \"MonoLabelMedium\": {\n \"fontFamily\": \"\\\"Lucida Console\\\", Monaco, monospace\",\n \"fontSize\": \"16px\",\n \"fontWeight\": 500,\n \"lineHeight\": \"20px\"\n },\n \"MonoLabelLarge\": {\n \"fontFamily\": \"\\\"Lucida Console\\\", Monaco, monospace\",\n \"fontSize\": \"18px\",\n \"fontWeight\": 500,\n \"lineHeight\": \"24px\"\n },\n \"MonoHeadingXSmall\": {\n \"fontFamily\": \"\\\"Lucida Console\\\", Monaco, monospace\",\n \"fontSize\": \"20px\",\n \"fontWeight\": 700,\n \"lineHeight\": \"28px\"\n },\n \"MonoHeadingSmall\": {\n \"fontFamily\": \"\\\"Lucida Console\\\", Monaco, monospace\",\n \"fontSize\": \"24px\",\n \"fontWeight\": 700,\n \"lineHeight\": \"32px\"\n },\n \"MonoHeadingMedium\": {\n \"fontFamily\": \"\\\"Lucida Console\\\", Monaco, monospace\",\n \"fontSize\": \"28px\",\n \"fontWeight\": 700,\n \"lineHeight\": \"36px\"\n },\n \"MonoHeadingLarge\": {\n \"fontFamily\": \"\\\"Lucida Console\\\", Monaco, monospace\",\n \"fontSize\": \"32px\",\n \"fontWeight\": 700,\n \"lineHeight\": \"40px\"\n },\n \"MonoHeadingXLarge\": {\n \"fontFamily\": \"\\\"Lucida Console\\\", Monaco, monospace\",\n \"fontSize\": \"36px\",\n \"fontWeight\": 700,\n \"lineHeight\": \"44px\"\n },\n \"MonoHeadingXXLarge\": {\n \"fontFamily\": \"\\\"Lucida Console\\\", Monaco, monospace\",\n \"fontSize\": \"40px\",\n \"fontWeight\": 700,\n \"lineHeight\": \"52px\"\n },\n \"MonoDisplayXSmall\": {\n \"fontFamily\": \"\\\"Lucida Console\\\", Monaco, monospace\",\n \"fontSize\": \"36px\",\n \"fontWeight\": 700,\n \"lineHeight\": \"44px\"\n },\n \"MonoDisplaySmall\": {\n \"fontFamily\": \"\\\"Lucida Console\\\", Monaco, monospace\",\n \"fontSize\": \"44px\",\n \"fontWeight\": 700,\n \"lineHeight\": \"52px\"\n },\n \"MonoDisplayMedium\": {\n \"fontFamily\": \"\\\"Lucida Console\\\", Monaco, monospace\",\n \"fontSize\": \"52px\",\n \"fontWeight\": 700,\n \"lineHeight\": \"64px\"\n },\n \"MonoDisplayLarge\": {\n \"fontFamily\": \"\\\"Lucida Console\\\", Monaco, monospace\",\n \"fontSize\": \"96px\",\n \"fontWeight\": 700,\n \"lineHeight\": \"112px\"\n },\n \"font460\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"1rem\",\n \"fontSizeSm\": \"14px\",\n \"fontWeight\": \"normal\",\n \"lineHeight\": 1.6,\n \"lineHeightTight\": 1.25\n },\n \"font470\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"1rem\",\n \"fontSizeSm\": \"14px\",\n \"fontWeight\": \"normal\",\n \"lineHeight\": 1.6,\n \"lineHeightTight\": 1.25\n },\n \"font500\": {\n \"fontFamily\": \"\\\"Source Sans Pro\\\", sans-serif\",\n \"fontSize\": \"1rem\",\n \"fontSizeSm\": \"14px\",\n \"fontWeight\": \"normal\",\n \"lineHeight\": 1.6,\n \"lineHeightTight\": 1.25\n },\n \"font600\": {}\n },\n \"zIndex\": {\n \"modal\": 2000\n }\n },\n \"primitives\": {\n \"primaryA\": \"#000000\",\n \"primaryB\": \"#FFFFFF\",\n \"primary\": \"#000000\",\n \"primary50\": \"#F6F6F6\",\n \"primary100\": \"#EEEEEE\",\n \"primary200\": \"#E2E2E2\",\n \"primary300\": \"#CBCBCB\",\n \"primary400\": \"#AFAFAF\",\n \"primary500\": \"#6B6B6B\",\n \"primary600\": \"#545454\",\n \"primary700\": \"#333333\",\n \"accent\": \"#276EF1\",\n \"accent50\": \"#EFF3FE\",\n \"accent100\": \"#D4E2FC\",\n \"accent200\": \"#A0BFF8\",\n \"accent300\": \"#5B91F5\",\n \"accent400\": \"#276EF1\",\n \"accent500\": \"#1E54B7\",\n \"accent600\": \"#174291\",\n \"accent700\": \"#102C60\",\n \"negative\": \"#E11900\",\n \"negative50\": \"#FFEFED\",\n \"negative100\": \"#FED7D2\",\n \"negative200\": \"#F1998E\",\n \"negative300\": \"#E85C4A\",\n \"negative400\": \"#E11900\",\n \"negative500\": \"#AB1300\",\n \"negative600\": \"#870F00\",\n \"negative700\": \"#5A0A00\",\n \"warning\": \"#FFC043\",\n \"warning50\": \"#FFFAF0\",\n \"warning100\": \"#FFF2D9\",\n \"warning200\": \"#FFE3AC\",\n \"warning300\": \"#FFCF70\",\n \"warning400\": \"#FFC043\",\n \"warning500\": \"#BC8B2C\",\n \"warning600\": \"#996F00\",\n \"warning700\": \"#674D1B\",\n \"positive\": \"#03703C\",\n \"positive50\": \"#E6F2ED\",\n \"positive100\": \"#ADDEC9\",\n \"positive200\": \"#66D19E\",\n \"positive300\": \"#06C167\",\n \"positive400\": \"#048848\",\n \"positive500\": \"#03703C\",\n \"positive600\": \"#03582F\",\n \"positive700\": \"#10462D\",\n \"white\": \"#FFFFFF\",\n \"black\": \"#000000\",\n \"mono100\": \"#FFFFFF\",\n \"mono200\": \"#F6F6F6\",\n \"mono300\": \"#EEEEEE\",\n \"mono400\": \"#E2E2E2\",\n \"mono500\": \"#CBCBCB\",\n \"mono600\": \"#AFAFAF\",\n \"mono700\": \"#6B6B6B\",\n \"mono800\": \"#545454\",\n \"mono900\": \"#333333\",\n \"mono1000\": \"#000000\",\n \"ratingInactiveFill\": \"#EEEEEE\",\n \"ratingStroke\": \"#CBCBCB\",\n \"primaryFontFamily\": \"system-ui, \\\"Helvetica Neue\\\", Helvetica, Arial, sans-serif\"\n }\n}\n```\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "Components and Frameworks to give new features to streamlit",
"version": "0.5.0",
"project_urls": {
"Homepage": "https://github.com/quiradev/streamlit-plugins"
},
"split_keywords": [
"streamlit",
" plugins",
" components",
" theme-changer"
],
"urls": [
{
"comment_text": null,
"digests": {
"blake2b_256": "6fe50244a32a6d337e04812698aafb59c3f6851f403d547518aa2294ae45a9af",
"md5": "441f60ce73c50eda052096c4c6cfa7bc",
"sha256": "5d546a831980c281b4c5707902580a548c015ca384a64cedd55f8a8841aed0f5"
},
"downloads": -1,
"filename": "streamlit_component_theme_changer-0.5.0-py3-none-any.whl",
"has_sig": false,
"md5_digest": "441f60ce73c50eda052096c4c6cfa7bc",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.10",
"size": 27497,
"upload_time": "2025-02-13T22:13:20",
"upload_time_iso_8601": "2025-02-13T22:13:20.757158Z",
"url": "https://files.pythonhosted.org/packages/6f/e5/0244a32a6d337e04812698aafb59c3f6851f403d547518aa2294ae45a9af/streamlit_component_theme_changer-0.5.0-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "bcb3d1c45ab92af5ea19b9f28f3425fcfca978ed2b175d1c2dc7212c309816a6",
"md5": "5230faca092f2b18f650e72fa5895f4d",
"sha256": "ff035fcf74ceb90dfd325c120b7ad6b8bc41f2f3329b1697abfb90d6dc955bb0"
},
"downloads": -1,
"filename": "streamlit_component_theme_changer-0.5.0.tar.gz",
"has_sig": false,
"md5_digest": "5230faca092f2b18f650e72fa5895f4d",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.10",
"size": 45676,
"upload_time": "2025-02-13T22:13:23",
"upload_time_iso_8601": "2025-02-13T22:13:23.618300Z",
"url": "https://files.pythonhosted.org/packages/bc/b3/d1c45ab92af5ea19b9f28f3425fcfca978ed2b175d1c2dc7212c309816a6/streamlit_component_theme_changer-0.5.0.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2025-02-13 22:13:23",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "quiradev",
"github_project": "streamlit-plugins",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"requirements": [
{
"name": "streamlit",
"specs": [
[
">=",
"1.41.0"
]
]
},
{
"name": "compress-pickle",
"specs": [
[
">=",
"2.1.0"
]
]
},
{
"name": "bokeh",
"specs": [
[
">=",
"3.1.1"
]
]
},
{
"name": "validators",
"specs": [
[
">=",
"0.22.0"
]
]
},
{
"name": "pandas",
"specs": [
[
">=",
"2.2.0"
]
]
},
{
"name": "pydantic",
"specs": []
}
],
"lcname": "streamlit-component-theme-changer"
}