Name | textX JSON |
Version |
4.1.0
JSON |
| download |
home_page | None |
Summary | Meta-language for DSL implementation inspired by Xtext |
upload_time | 2024-10-26 13:11:35 |
maintainer | None |
docs_url | None |
author | None |
requires_python | >=3.8 |
license | None |
keywords |
parser
meta-language
meta-model
language
dsl
|
VCS |
 |
bugtrack_url |
|
requirements |
No requirements were recorded.
|
Travis-CI |
No Travis.
|
coveralls test coverage |
No coveralls.
|

[](https://pypi.python.org/pypi/textX)

[](https://github.com/textx/textx/actions)
[](https://coveralls.io/github/textX/textX?branch=master)
[](https://textx.github.io/textX/)
textX is a meta-language for building Domain-Specific Languages (DSLs) in
Python. It is inspired by [Xtext].
In a nutshell, textX will help you build your textual language in an easy way.
You can invent your own language or build a support for already existing textual
language or file format.
From a single language description (grammar), textX will build a parser and a
meta-model (a.k.a. abstract syntax) for the language. See the docs for the
details.
textX follows the syntax and semantics of Xtext but [differs in some
places](http://textx.github.io/textX/about/comparison.html) and is
implemented 100% in Python using [Arpeggio] PEG parser - no grammar ambiguities,
unlimited lookahead, interpreter style of work.
## Quick intro
Here is a complete example that shows the definition of a simple DSL for
drawing. We also show how to define a custom class, interpret models and search
for instances of a particular type.
```python
from textx import metamodel_from_str, get_children_of_type
grammar = """
Model: commands*=DrawCommand;
DrawCommand: MoveCommand | ShapeCommand;
ShapeCommand: LineTo | Circle;
MoveCommand: MoveTo | MoveBy;
MoveTo: 'move' 'to' position=Point;
MoveBy: 'move' 'by' vector=Point;
Circle: 'circle' radius=INT;
LineTo: 'line' 'to' point=Point;
Point: x=INT ',' y=INT;
"""
# We will provide our class for Point.
# Classes for other rules will be dynamically generated.
class Point:
def __init__(self, parent, x, y):
self.parent = parent
self.x = x
self.y = y
def __str__(self):
return "{},{}".format(self.x, self.y)
def __add__(self, other):
return Point(self.parent, self.x + other.x, self.y + other.y)
# Create meta-model from the grammar. Provide `Point` class to be used for
# the rule `Point` from the grammar.
mm = metamodel_from_str(grammar, classes=[Point])
model_str = """
move to 5, 10
line to 10, 10
line to 20, 20
move by 5, -7
circle 10
line to 10, 10
"""
# Meta-model knows how to parse and instantiate models.
model = mm.model_from_str(model_str)
# At this point model is a plain Python object graph with instances of
# dynamically created classes and attributes following the grammar.
def cname(o):
return o.__class__.__name__
# Let's interpret the model
position = Point(None, 0, 0)
for command in model.commands:
if cname(command) == 'MoveTo':
print('Moving to position', command.position)
position = command.position
elif cname(command) == 'MoveBy':
position = position + command.vector
print('Moving by', command.vector, 'to a new position', position)
elif cname(command) == 'Circle':
print('Drawing circle at', position, 'with radius', command.radius)
else:
print('Drawing line from', position, 'to', command.point)
position = command.point
print('End position is', position)
# Output:
# Moving to position 5,10
# Drawing line from 5,10 to 10,10
# Drawing line from 10,10 to 20,20
# Moving by 5,-7 to a new position 25,13
# Drawing circle at 25,13 with radius 10
# Drawing line from 25,13 to 10,10
# Collect all points starting from the root of the model
points = get_children_of_type("Point", model)
for point in points:
print('Point: {}'.format(point))
# Output:
# Point: 5,10
# Point: 10,10
# Point: 20,20
# Point: 5,-7
# Point: 10,10
```
## Video tutorials
### Introduction to textX
[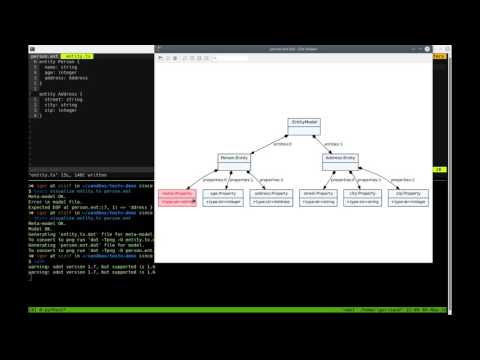](https://www.youtube.com/watch?v=CN2IVtInapo)
### Implementing Martin Fowler's State Machine DSL in textX
[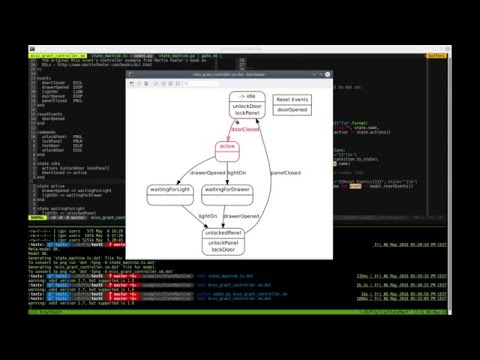](https://www.youtube.com/watch?v=HI14jk0JIR0)
## Docs and tutorials
The full documentation with tutorials is available at
http://textx.github.io/textX/stable/
You can also try textX in [our
playground](https://textx.github.io/textx-playground/). There is a dropdown with
several examples to get you started.
# Support in IDE/editors
Projects that are currently in progress are:
- [textX-LS](https://github.com/textX/textX-LS) - support for Language Server
Protocol and VS Code for any textX based language. This project is about to
supersede the following projects:
- [textX-languageserver](https://github.com/textX/textX-languageserver) -
Language Server Protocol support for textX languages
- [textX-extensions](https://github.com/textX/textX-extensions) - syntax
highlighting, code outline
- [viewX](https://github.com/danielkupco/viewX-vscode) - creating visualizers
for textX languages
If you are a vim editor user check
out [support for vim](https://github.com/textX/textx.vim/).
For emacs there is [textx-mode](https://github.com/textX/textx-mode) which is
also available in [MELPA](https://melpa.org/#/textx-mode).
You can also check
out [textX-ninja project](https://github.com/textX/textX-ninja). It is
currently unmaintained.
## Discussion and help
For general questions, suggestions, and feature requests please use [GitHub
Discussions](https://github.com/textX/textX/discussions).
For issues please use [GitHub issue
tracker](https://github.com/textX/textX/issues).
## Citing textX
If you are using textX in your research project we would be very grateful if you
cite our paper:
Dejanović I., Vaderna R., Milosavljević G., Vuković Ž. (2017). [TextX: A Python
tool for Domain-Specific Languages
implementation](https://www.doi.org/10.1016/j.knosys.2016.10.023).
Knowledge-Based Systems, 115, 1-4.
## License
MIT
## Python versions
Tested for 3.8+
[Arpeggio]: https://github.com/textX/Arpeggio
[Xtext]: http://www.eclipse.org/Xtext/
Raw data
{
"_id": null,
"home_page": null,
"name": "textX",
"maintainer": null,
"docs_url": null,
"requires_python": ">=3.8",
"maintainer_email": "\"Igor R. Dejanovi\u0107\" <igor.dejanovic@gmail.com>, Pierre Bayerl <noemail@nowhere.com>",
"keywords": "parser, meta-language, meta-model, language, DSL",
"author": null,
"author_email": "\"Igor R. Dejanovi\u0107\" <igor.dejanovic@gmail.com>, Pierre Bayerl <noemail@nowhere.com>",
"download_url": "https://files.pythonhosted.org/packages/50/34/8577e4d4ccea98b0bcc7b05a80e02bb8521e1267bc6792864abe85a46546/textx-4.1.0.tar.gz",
"platform": null,
"description": "\n\n[](https://pypi.python.org/pypi/textX)\n\n[](https://github.com/textx/textx/actions)\n[](https://coveralls.io/github/textX/textX?branch=master)\n[](https://textx.github.io/textX/)\n\n\ntextX is a meta-language for building Domain-Specific Languages (DSLs) in\nPython. It is inspired by [Xtext].\n\nIn a nutshell, textX will help you build your textual language in an easy way.\nYou can invent your own language or build a support for already existing textual\nlanguage or file format.\n\nFrom a single language description (grammar), textX will build a parser and a\nmeta-model (a.k.a. abstract syntax) for the language. See the docs for the\ndetails.\n\ntextX follows the syntax and semantics of Xtext but [differs in some\nplaces](http://textx.github.io/textX/about/comparison.html) and is\nimplemented 100% in Python using [Arpeggio] PEG parser - no grammar ambiguities,\nunlimited lookahead, interpreter style of work.\n\n\n## Quick intro\n\nHere is a complete example that shows the definition of a simple DSL for\ndrawing. We also show how to define a custom class, interpret models and search\nfor instances of a particular type.\n\n```python\nfrom textx import metamodel_from_str, get_children_of_type\n\ngrammar = \"\"\"\nModel: commands*=DrawCommand;\nDrawCommand: MoveCommand | ShapeCommand;\nShapeCommand: LineTo | Circle;\nMoveCommand: MoveTo | MoveBy;\nMoveTo: 'move' 'to' position=Point;\nMoveBy: 'move' 'by' vector=Point;\nCircle: 'circle' radius=INT;\nLineTo: 'line' 'to' point=Point;\nPoint: x=INT ',' y=INT;\n\"\"\"\n\n# We will provide our class for Point.\n# Classes for other rules will be dynamically generated.\nclass Point:\n def __init__(self, parent, x, y):\n self.parent = parent\n self.x = x\n self.y = y\n\n def __str__(self):\n return \"{},{}\".format(self.x, self.y)\n\n def __add__(self, other):\n return Point(self.parent, self.x + other.x, self.y + other.y)\n\n# Create meta-model from the grammar. Provide `Point` class to be used for\n# the rule `Point` from the grammar.\nmm = metamodel_from_str(grammar, classes=[Point])\n\nmodel_str = \"\"\"\n move to 5, 10\n line to 10, 10\n line to 20, 20\n move by 5, -7\n circle 10\n line to 10, 10\n\"\"\"\n\n# Meta-model knows how to parse and instantiate models.\nmodel = mm.model_from_str(model_str)\n\n# At this point model is a plain Python object graph with instances of\n# dynamically created classes and attributes following the grammar.\n\ndef cname(o):\n return o.__class__.__name__\n\n# Let's interpret the model\nposition = Point(None, 0, 0)\nfor command in model.commands:\n if cname(command) == 'MoveTo':\n print('Moving to position', command.position)\n position = command.position\n elif cname(command) == 'MoveBy':\n position = position + command.vector\n print('Moving by', command.vector, 'to a new position', position)\n elif cname(command) == 'Circle':\n print('Drawing circle at', position, 'with radius', command.radius)\n else:\n print('Drawing line from', position, 'to', command.point)\n position = command.point\nprint('End position is', position)\n\n# Output:\n# Moving to position 5,10\n# Drawing line from 5,10 to 10,10\n# Drawing line from 10,10 to 20,20\n# Moving by 5,-7 to a new position 25,13\n# Drawing circle at 25,13 with radius 10\n# Drawing line from 25,13 to 10,10\n\n# Collect all points starting from the root of the model\npoints = get_children_of_type(\"Point\", model)\nfor point in points:\n print('Point: {}'.format(point))\n\n# Output:\n# Point: 5,10\n# Point: 10,10\n# Point: 20,20\n# Point: 5,-7\n# Point: 10,10\n```\n\n\n## Video tutorials\n\n\n### Introduction to textX\n\n\n[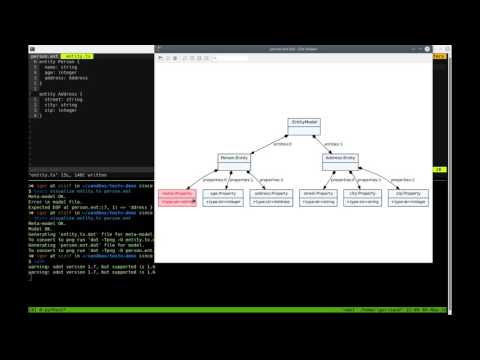](https://www.youtube.com/watch?v=CN2IVtInapo)\n\n\n### Implementing Martin Fowler's State Machine DSL in textX\n\n[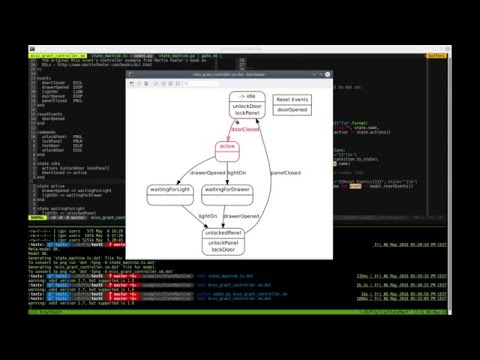](https://www.youtube.com/watch?v=HI14jk0JIR0)\n\n\n## Docs and tutorials\n\nThe full documentation with tutorials is available at\nhttp://textx.github.io/textX/stable/\n\nYou can also try textX in [our\nplayground](https://textx.github.io/textx-playground/). There is a dropdown with\nseveral examples to get you started.\n\n\n# Support in IDE/editors\n\nProjects that are currently in progress are:\n\n- [textX-LS](https://github.com/textX/textX-LS) - support for Language Server\n Protocol and VS Code for any textX based language. This project is about to\n supersede the following projects:\n - [textX-languageserver](https://github.com/textX/textX-languageserver) -\n Language Server Protocol support for textX languages\n - [textX-extensions](https://github.com/textX/textX-extensions) - syntax\n highlighting, code outline\n- [viewX](https://github.com/danielkupco/viewX-vscode) - creating visualizers\n for textX languages\n \nIf you are a vim editor user check\nout [support for vim](https://github.com/textX/textx.vim/).\n\nFor emacs there is [textx-mode](https://github.com/textX/textx-mode) which is\nalso available in [MELPA](https://melpa.org/#/textx-mode).\n\nYou can also check\nout [textX-ninja project](https://github.com/textX/textX-ninja). It is\ncurrently unmaintained.\n\n\n## Discussion and help\n\nFor general questions, suggestions, and feature requests please use [GitHub\nDiscussions](https://github.com/textX/textX/discussions).\n\n\nFor issues please use [GitHub issue\ntracker](https://github.com/textX/textX/issues).\n\n\n## Citing textX\n\nIf you are using textX in your research project we would be very grateful if you\ncite our paper:\n\nDejanovi\u0107 I., Vaderna R., Milosavljevi\u0107 G., Vukovi\u0107 \u017d. (2017). [TextX: A Python\ntool for Domain-Specific Languages\nimplementation](https://www.doi.org/10.1016/j.knosys.2016.10.023).\nKnowledge-Based Systems, 115, 1-4.\n\n\n## License\n\nMIT\n\n## Python versions\n\nTested for 3.8+\n\n\n[Arpeggio]: https://github.com/textX/Arpeggio\n[Xtext]: http://www.eclipse.org/Xtext/\n",
"bugtrack_url": null,
"license": null,
"summary": "Meta-language for DSL implementation inspired by Xtext",
"version": "4.1.0",
"project_urls": {
"Changelog": "https://github.com/textX/textX/blob/master/CHANGELOG.md",
"Homepage": "https://textx.github.io/textX/",
"Repository": "https://github.com/textX/textX/"
},
"split_keywords": [
"parser",
" meta-language",
" meta-model",
" language",
" dsl"
],
"urls": [
{
"comment_text": null,
"digests": {
"blake2b_256": "dfdf1e6006b005fcffbfee9d065d80d0a628512bc22f1814904e23243f457f7e",
"md5": "c29f7aeef148d022024488e8cbafd795",
"sha256": "297784421e81a27b3701c968cf820353b79969e0d443f4ca6ac9352a827bf871"
},
"downloads": -1,
"filename": "textx-4.1.0-py3-none-any.whl",
"has_sig": false,
"md5_digest": "c29f7aeef148d022024488e8cbafd795",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.8",
"size": 67845,
"upload_time": "2024-10-26T13:11:30",
"upload_time_iso_8601": "2024-10-26T13:11:30.277670Z",
"url": "https://files.pythonhosted.org/packages/df/df/1e6006b005fcffbfee9d065d80d0a628512bc22f1814904e23243f457f7e/textx-4.1.0-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "50348577e4d4ccea98b0bcc7b05a80e02bb8521e1267bc6792864abe85a46546",
"md5": "db9f2cab8d4e9a599b11f815c3fc4c9c",
"sha256": "37b4f0c455452e27cc0f13d40777b5d20549eaa871311b26e2ed83c019456692"
},
"downloads": -1,
"filename": "textx-4.1.0.tar.gz",
"has_sig": false,
"md5_digest": "db9f2cab8d4e9a599b11f815c3fc4c9c",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.8",
"size": 2136296,
"upload_time": "2024-10-26T13:11:35",
"upload_time_iso_8601": "2024-10-26T13:11:35.956637Z",
"url": "https://files.pythonhosted.org/packages/50/34/8577e4d4ccea98b0bcc7b05a80e02bb8521e1267bc6792864abe85a46546/textx-4.1.0.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-10-26 13:11:35",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "textX",
"github_project": "textX",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "textx"
}