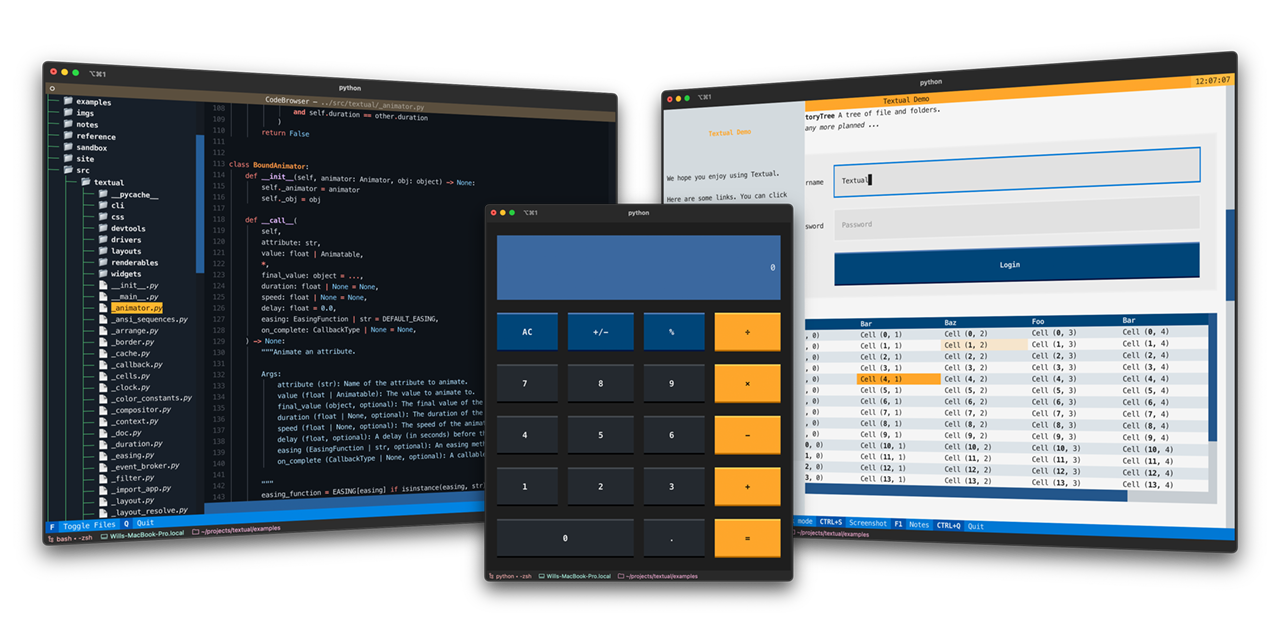
[](https://discord.gg/Enf6Z3qhVr)
# Textual
Textual is a *Rapid Application Development* framework for Python.
Build sophisticated user interfaces with a simple Python API. Run your apps in the terminal and a [web browser](https://github.com/Textualize/textual-web)!
<details>
<summary> 🎬 Demonstration </summary>
<hr>
A quick run through of some Textual features.
https://user-images.githubusercontent.com/554369/197355913-65d3c125-493d-4c05-a590-5311f16c40ff.mov
</details>
## About
Textual adds interactivity to [Rich](https://github.com/Textualize/rich) with an API inspired by modern web development.
On modern terminal software (installed by default on most systems), Textual apps can use **16.7 million** colors with mouse support and smooth flicker-free animation. A powerful layout engine and re-usable components makes it possible to build apps that rival the desktop and web experience.
## Compatibility
Textual runs on Linux, macOS, and Windows. Textual requires Python 3.8 or above.
## Installing
Install Textual via pip:
```
pip install textual
```
If you plan on developing Textual apps, you should also install the development tools with the following command:
```
pip install textual-dev
```
See the [docs](https://textual.textualize.io/getting_started/) if you need help getting started.
## Demo
Run the following command to see a little of what Textual can do:
```
python -m textual
```
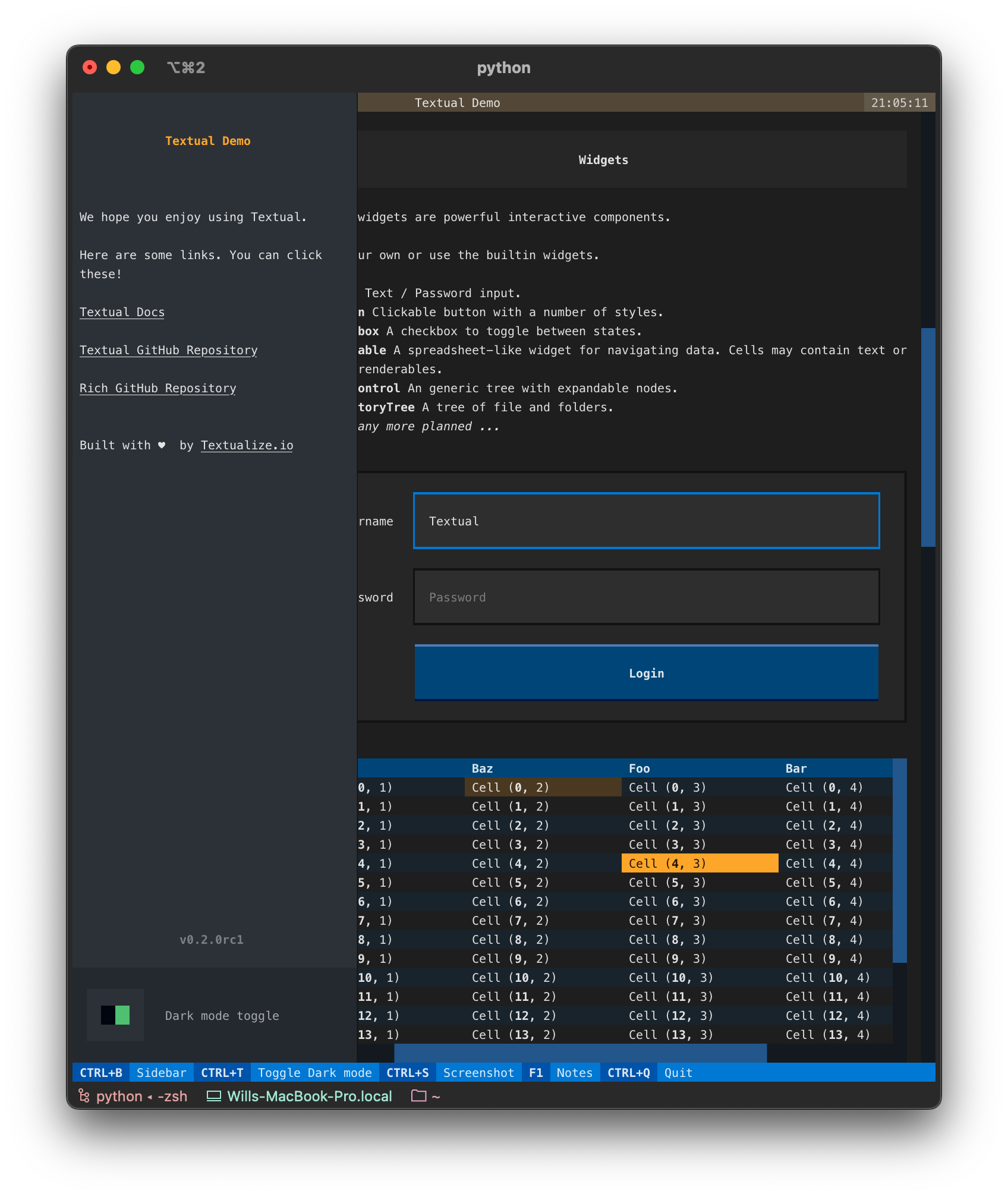
## Documentation
Head over to the [Textual documentation](http://textual.textualize.io/) to start building!
## Join us on Discord
Join the Textual developers and community on our [Discord Server](https://discord.gg/Enf6Z3qhVr).
## Examples
The Textual repository comes with a number of examples you can experiment with or use as a template for your own projects.
<details>
<summary> 🎬 Code browser </summary>
<hr>
This is the [code_browser.py](https://github.com/Textualize/textual/blob/main/examples/code_browser.py) example which clocks in at 61 lines (*including* docstrings and blank lines).
https://user-images.githubusercontent.com/554369/197188237-88d3f7e4-4e5f-40b5-b996-c47b19ee2f49.mov
</details>
<details>
<summary> 📷 Calculator </summary>
<hr>
This is [calculator.py](https://github.com/Textualize/textual/blob/main/examples/calculator.py) which demonstrates Textual grid layouts.
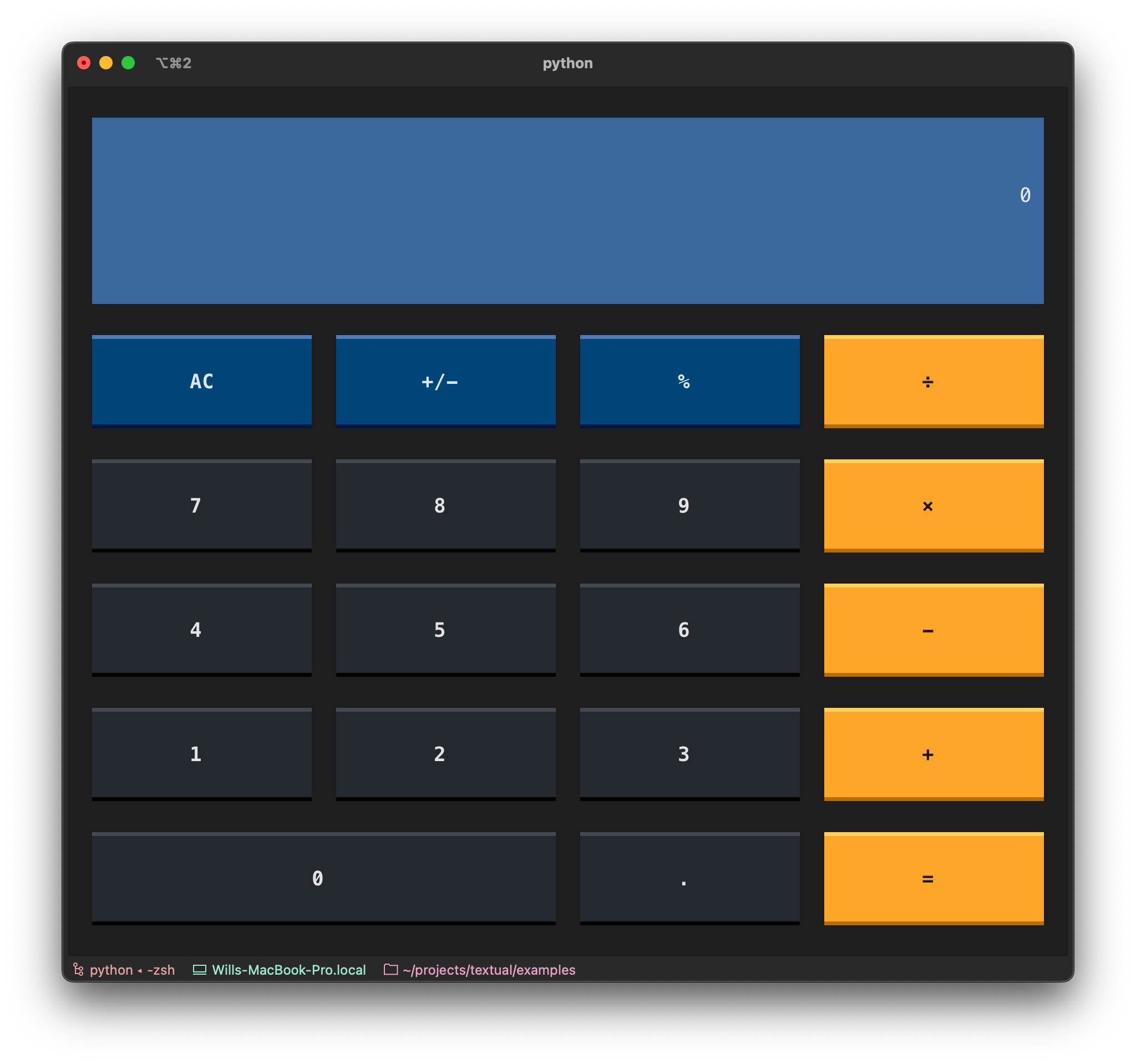
</details>
<details>
<summary> 🎬 Stopwatch </summary>
<hr>
This is the Stopwatch example from the [tutorial](https://textual.textualize.io/tutorial/).
https://user-images.githubusercontent.com/554369/197360718-0c834ef5-6285-4d37-85cf-23eed4aa56c5.mov
</details>
## Reference commands
The `textual` command has a few sub-commands to preview Textual styles.
<details>
<summary> 🎬 Easing reference </summary>
<hr>
This is the *easing* reference which demonstrates the easing parameter on animation, with both movement and opacity. You can run it with the following command:
```bash
textual easing
```
https://user-images.githubusercontent.com/554369/196157100-352852a6-2b09-4dc8-a888-55b53570aff9.mov
</details>
<details>
<summary> 🎬 Borders reference </summary>
<hr>
This is the borders reference which demonstrates some of the borders styles in Textual. You can run it with the following command:
```bash
textual borders
```
https://user-images.githubusercontent.com/554369/196158235-4b45fb78-053d-4fd5-b285-e09b4f1c67a8.mov
</details>
<details>
<summary> 🎬 Colors reference </summary>
<hr>
This is a reference for Textual's color design system.
```bash
textual colors
```
https://user-images.githubusercontent.com/554369/197357417-2d407aac-8969-44d3-8250-eea45df79d57.mov
</details>
Raw data
{
"_id": null,
"home_page": "https://github.com/Textualize/textual",
"name": "textual",
"maintainer": null,
"docs_url": null,
"requires_python": "<4.0.0,>=3.8.1",
"maintainer_email": null,
"keywords": null,
"author": "Will McGugan",
"author_email": "will@textualize.io",
"download_url": "https://files.pythonhosted.org/packages/56/c6/435723343db6a65ed92950d1e20ded920c4c22e6e785ea0e369abd4ac1c7/textual-0.86.1.tar.gz",
"platform": null,
"description": "\n\n\n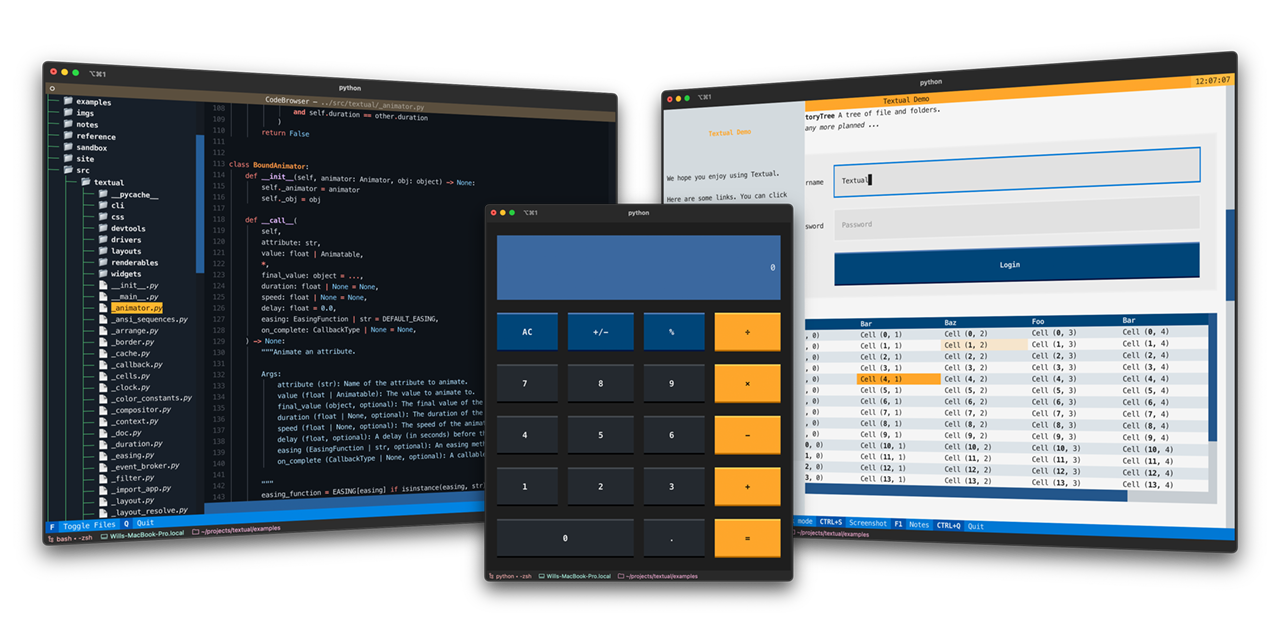\n\n[](https://discord.gg/Enf6Z3qhVr)\n\n\n# Textual\n\nTextual is a *Rapid Application Development* framework for Python.\n\nBuild sophisticated user interfaces with a simple Python API. Run your apps in the terminal and a [web browser](https://github.com/Textualize/textual-web)!\n\n\n<details>\n <summary> \ud83c\udfac Demonstration </summary>\n <hr>\n\nA quick run through of some Textual features.\n\n\n\nhttps://user-images.githubusercontent.com/554369/197355913-65d3c125-493d-4c05-a590-5311f16c40ff.mov\n\n\n\n </details>\n\n\n## About\n\nTextual adds interactivity to [Rich](https://github.com/Textualize/rich) with an API inspired by modern web development.\n\nOn modern terminal software (installed by default on most systems), Textual apps can use **16.7 million** colors with mouse support and smooth flicker-free animation. A powerful layout engine and re-usable components makes it possible to build apps that rival the desktop and web experience.\n\n## Compatibility\n\nTextual runs on Linux, macOS, and Windows. Textual requires Python 3.8 or above.\n\n## Installing\n\nInstall Textual via pip:\n\n```\npip install textual\n```\n\nIf you plan on developing Textual apps, you should also install the development tools with the following command:\n\n```\npip install textual-dev\n```\n\nSee the [docs](https://textual.textualize.io/getting_started/) if you need help getting started.\n\n## Demo\n\nRun the following command to see a little of what Textual can do:\n\n```\npython -m textual\n```\n\n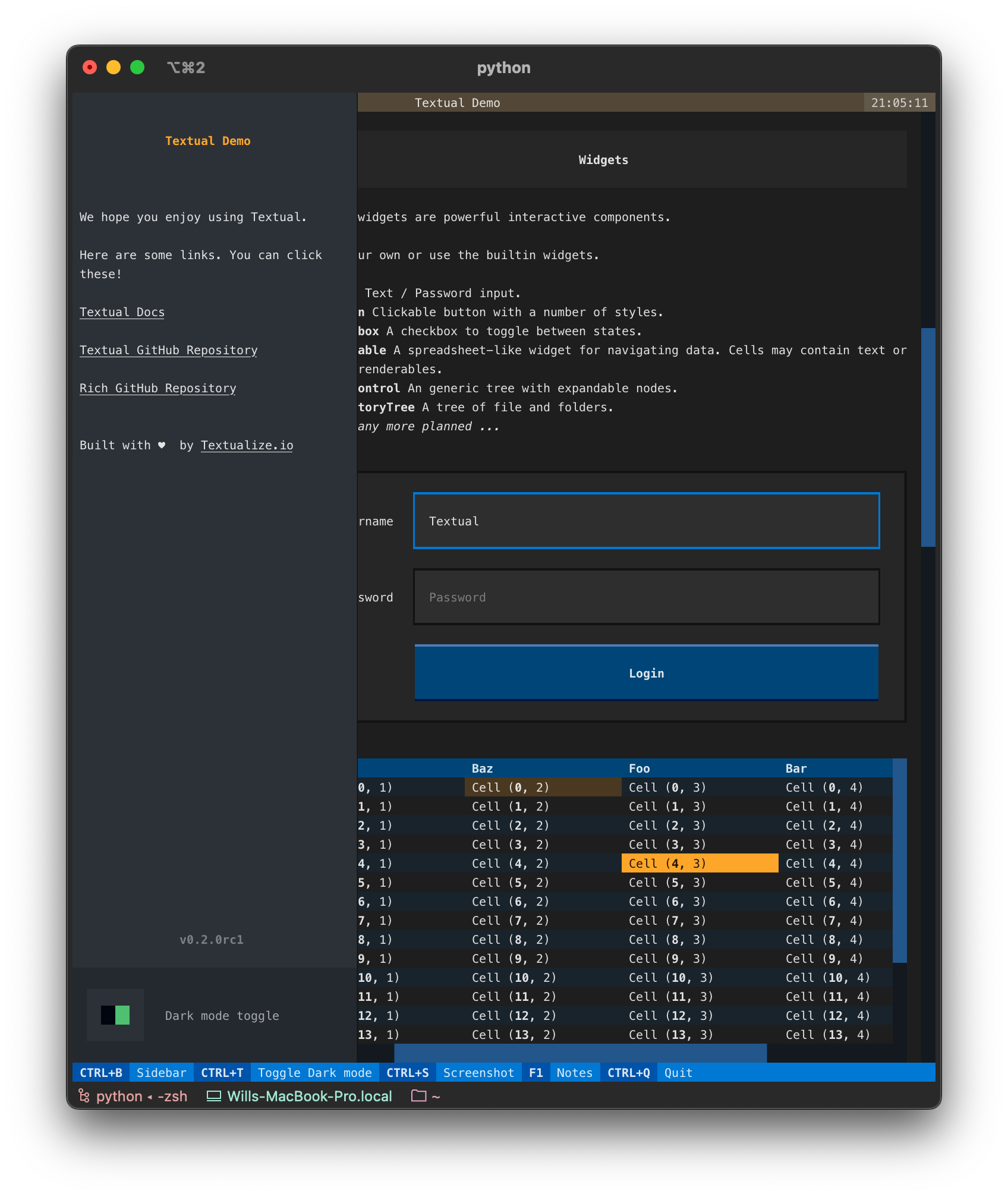\n\n## Documentation\n\nHead over to the [Textual documentation](http://textual.textualize.io/) to start building!\n\n## Join us on Discord\n\nJoin the Textual developers and community on our [Discord Server](https://discord.gg/Enf6Z3qhVr).\n\n## Examples\n\nThe Textual repository comes with a number of examples you can experiment with or use as a template for your own projects.\n\n\n<details>\n <summary> \ud83c\udfac Code browser </summary>\n <hr>\n\n This is the [code_browser.py](https://github.com/Textualize/textual/blob/main/examples/code_browser.py) example which clocks in at 61 lines (*including* docstrings and blank lines).\n\nhttps://user-images.githubusercontent.com/554369/197188237-88d3f7e4-4e5f-40b5-b996-c47b19ee2f49.mov\n\n </details>\n\n\n<details>\n <summary> \ud83d\udcf7 Calculator </summary>\n <hr>\n\nThis is [calculator.py](https://github.com/Textualize/textual/blob/main/examples/calculator.py) which demonstrates Textual grid layouts.\n\n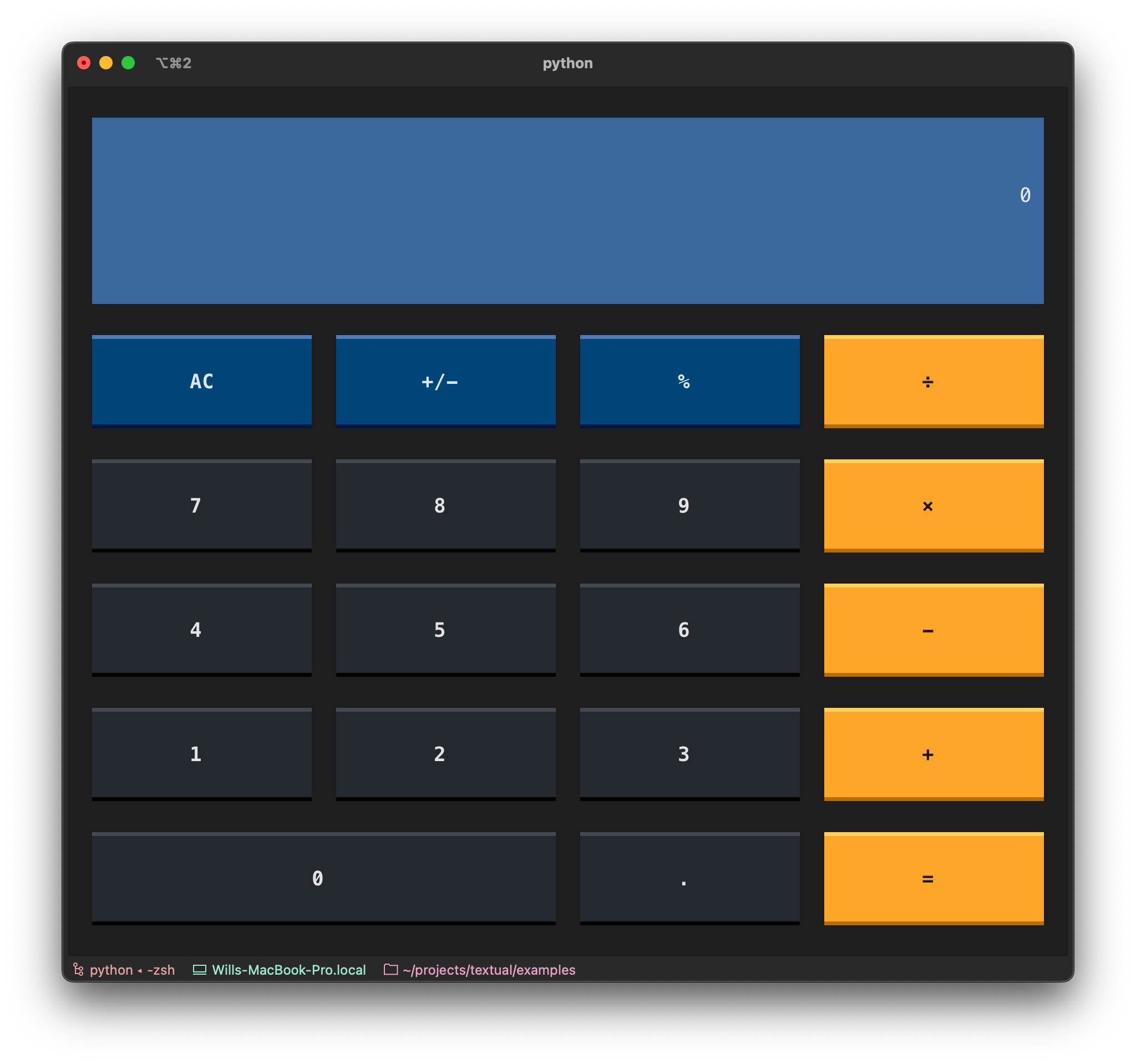\n</details>\n\n\n<details>\n <summary> \ud83c\udfac Stopwatch </summary>\n <hr>\n\n This is the Stopwatch example from the [tutorial](https://textual.textualize.io/tutorial/).\n\n\n\nhttps://user-images.githubusercontent.com/554369/197360718-0c834ef5-6285-4d37-85cf-23eed4aa56c5.mov\n\n\n\n</details>\n\n\n\n## Reference commands\n\nThe `textual` command has a few sub-commands to preview Textual styles.\n\n<details>\n <summary> \ud83c\udfac Easing reference </summary>\n <hr>\n\nThis is the *easing* reference which demonstrates the easing parameter on animation, with both movement and opacity. You can run it with the following command:\n\n```bash\ntextual easing\n```\n\n\nhttps://user-images.githubusercontent.com/554369/196157100-352852a6-2b09-4dc8-a888-55b53570aff9.mov\n\n\n </details>\n\n<details>\n <summary> \ud83c\udfac Borders reference </summary>\n <hr>\n\nThis is the borders reference which demonstrates some of the borders styles in Textual. You can run it with the following command:\n\n```bash\ntextual borders\n```\n\n\nhttps://user-images.githubusercontent.com/554369/196158235-4b45fb78-053d-4fd5-b285-e09b4f1c67a8.mov\n\n\n</details>\n\n\n<details>\n <summary> \ud83c\udfac Colors reference </summary>\n <hr>\n\nThis is a reference for Textual's color design system.\n\n```bash\ntextual colors\n```\n\n\n\nhttps://user-images.githubusercontent.com/554369/197357417-2d407aac-8969-44d3-8250-eea45df79d57.mov\n\n\n\n\n</details>\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "Modern Text User Interface framework",
"version": "0.86.1",
"project_urls": {
"Bug Tracker": "https://github.com/Textualize/textual/issues",
"Documentation": "https://textual.textualize.io/",
"Homepage": "https://github.com/Textualize/textual",
"Repository": "https://github.com/Textualize/textual"
},
"split_keywords": [],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "fea32b7bfd8d0665072a77459f51b600445939aee8a9d25bc3d546c9ac879a19",
"md5": "8adc4e2e3007c3ff7cdc4947333ca3c5",
"sha256": "ebc2bfd92c2f1a451c12dcbcfda002598a2fc9793d1684be83514b5fba75f915"
},
"downloads": -1,
"filename": "textual-0.86.1-py3-none-any.whl",
"has_sig": false,
"md5_digest": "8adc4e2e3007c3ff7cdc4947333ca3c5",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": "<4.0.0,>=3.8.1",
"size": 646857,
"upload_time": "2024-11-16T18:51:24",
"upload_time_iso_8601": "2024-11-16T18:51:24.956331Z",
"url": "https://files.pythonhosted.org/packages/fe/a3/2b7bfd8d0665072a77459f51b600445939aee8a9d25bc3d546c9ac879a19/textual-0.86.1-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "56c6435723343db6a65ed92950d1e20ded920c4c22e6e785ea0e369abd4ac1c7",
"md5": "548a5d54dd21fc369907fb19bf527af4",
"sha256": "a6e68de5383415f222f26b4049c2a92ed204071fdfebe3d729f8dd373ca5f519"
},
"downloads": -1,
"filename": "textual-0.86.1.tar.gz",
"has_sig": false,
"md5_digest": "548a5d54dd21fc369907fb19bf527af4",
"packagetype": "sdist",
"python_version": "source",
"requires_python": "<4.0.0,>=3.8.1",
"size": 1496752,
"upload_time": "2024-11-16T18:51:27",
"upload_time_iso_8601": "2024-11-16T18:51:27.335672Z",
"url": "https://files.pythonhosted.org/packages/56/c6/435723343db6a65ed92950d1e20ded920c4c22e6e785ea0e369abd4ac1c7/textual-0.86.1.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-11-16 18:51:27",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "Textualize",
"github_project": "textual",
"travis_ci": false,
"coveralls": true,
"github_actions": true,
"lcname": "textual"
}