# TkDial
**This is a library containing some circular rotatory dial-knob widgets for Tkinter. It can be used in place of normal sliders and scale.**
[](https://pypi.org/project/tkdial)

[](https://pepy.tech/project/tkdial)
## Installation
```
pip install tkdial
```
# Dial Widget
## Usage
**Simple Example:**
```python
import tkinter as tk
from tkdial import Dial
app = tk.Tk()
dial = Dial(app)
dial.grid(padx=10, pady=10)
app.mainloop()
```
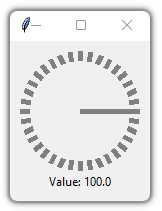
### **Example 2**
```python
import tkinter as tk
from tkdial import Dial
app = tk.Tk()
# some color combinations
color_combinations = [("yellow", "red"), ("white", "cyan"), ("red", "pink"), ("black", "green"),
("white", "black"), ("blue", "blue"), ("green", "green"), ("white", "pink"),
("red", "black"), ("green", "cyan"), ("cyan","black"), ("pink", "blue")]
for i in range (12):
dial = Dial(master=app, color_gradient=color_combinations[i],
unit_length=10, radius=40, needle_color=color_combinations[i][1])
if i<6:
dial.grid(row=1, padx=10, pady=10, column=i)
else:
dial.grid(row=2, padx=10, pady=10, column=11-i)
app.mainloop()
```
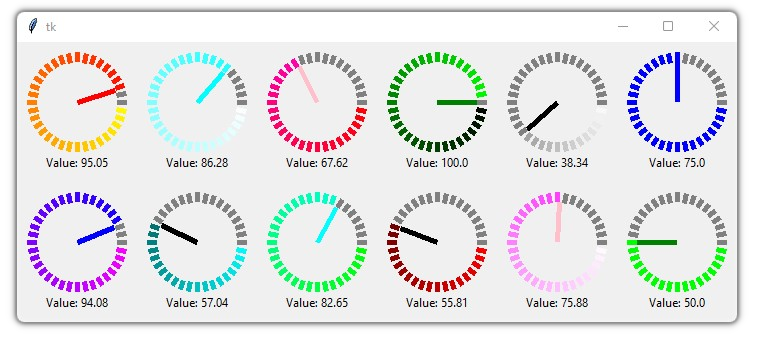
### **Example 3**
**Implemented with [CustomTkinter](https://github.com/TomSchimansky/CustomTkinter)**
```python
import customtkinter
from tkdial import Dial
customtkinter.set_appearance_mode("Dark")
app = customtkinter.CTk()
app.geometry("350x400")
app.grid_columnconfigure((0,1), weight=1)
app.grid_rowconfigure((0,1), weight=1)
frame_1 = customtkinter.CTkFrame(master=app)
frame_1.grid(padx=20, pady=20, sticky="nswe")
dial1 = Dial(master=frame_1, color_gradient=("green", "cyan"),
text_color="white", text="Current: ", unit_length=10, radius=50)
dial1.grid(padx=20, pady=20)
dial2 = Dial(master=frame_1, color_gradient=("yellow", "white"),
text_color="white", text="Position: ", unit_length=10, radius=50)
dial2.grid(padx=20, pady=20)
dial3 = Dial(master=frame_1, color_gradient=("white", "pink"),
text_color="white", text=" ", unit_length=10, radius=50)
dial3.grid(row=0, column=1, padx=20, pady=20)
dial4 = Dial(master=frame_1, color_gradient=("green", "green"),
text_color="white", text="", unit_width=15, radius=50)
dial4.grid(row=1, column=1, padx=20, pady=20)
app.mainloop()
```
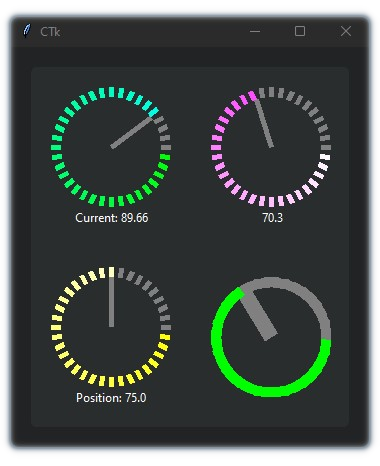
## Arguments:
| Parameters | Description |
| -------- | ----------- |
| _master_ | The master parameter is the parent widget |
| _bg_ | The default background color of the dial widget |
| _width_ | Define width of the widget manually (optional) |
| _height_ | Define height of the widget manually (optional) |
| _x_ | Determines the horizontal position of the dial in the canvas |
| _y_ | Determines the vertical position of the dial in the canvas |
| _start_ | The start point of the range from where the needle will rotate |
| _end_ | The end point of the range |
| _radius_ | Determines the distance of the unit lines between the center and the edge and also the length of the needle line |
| _unit_length_ | Specify the length of the lines |
| _unit_width_ | Specify the width of the lines |
| _unit_color_ | Specify the color of the unit lines |
| _needle_color_ | Specify the color of the needle line |
| _color_gradient_ | Specify which color gradient will be used for the units |
| _text_ | A string that will be displayed under the dial object with value |
| _text_color_ | Specify the color of the text that will be displayed under the dial object |
| _text_font_ | Specify the font of the text that will be displayed under the dial object |
| _integer_ | A boolean (True/False), displays only the integer value in text if True (default=False) |
| _scroll_ | A boolean (True/False), enables mouse scroll in dial (default=True) |
| _scroll_steps_ | Number of steps per scroll |
| _state_ | Specify the state of the needle |
| _command_ | Call a function whenever the needle is rotated |
### Methods:
| Methods | Description |
|-----------|-------------|
| _.get()_ | get the current value of the dial |
| _.set()_ | set the value of the dial |
| _.configure()_ | configure parameters of the dial |
# Scroll Knob
## Usage
**Simple Example**
```python
import tkinter
from tkdial import ScrollKnob
app = tkinter.Tk()
knob = ScrollKnob(app, start=0, end=100, steps=10)
knob.grid()
app.mainloop()
```
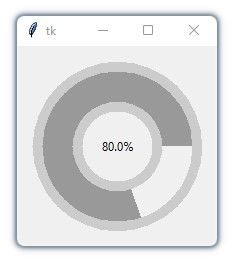
### **Different Knob styles:**
```python
import customtkinter
from tkdial import ScrollKnob
app = customtkinter.CTk()
app.geometry("500x500")
knob1 = ScrollKnob(app, radius=250, progress_color="#87ceeb", steps=10,
border_width=40, start_angle=90, inner_width=1, outer_width=1,
text_font="calibri 20", text_color="#87ceeb", bar_color="black")
knob1.grid(row=0, column=0)
knob2 = ScrollKnob(app, radius=200, progress_color="#7eff00",
border_width=40, start_angle=90, inner_width=1, outer_width=0,
text_font="calibri 20", text_color="#7eff00", integer=True,
fg="#212325")
knob2.grid(row=1, column=0)
knob3 = ScrollKnob(app, text=" ", radius=250, progress_color="white",
bar_color="#2937a6", border_width=30, start_angle=0, inner_width=5,
outer_width=0, text_font="calibri 20", steps=1, text_color="white", fg="#303ba1")
knob3.grid(row=0, column=1)
knob4 = ScrollKnob(app, text=" ", steps=10, radius=200, bar_color="#242424",
progress_color="yellow", outer_color="yellow", outer_length=10,
border_width=30, start_angle=270, inner_width=0, outer_width=5, text_font="calibri 20",
text_color="white", fg="#212325")
knob4.grid(row=1, column=1)
app.mainloop()
```
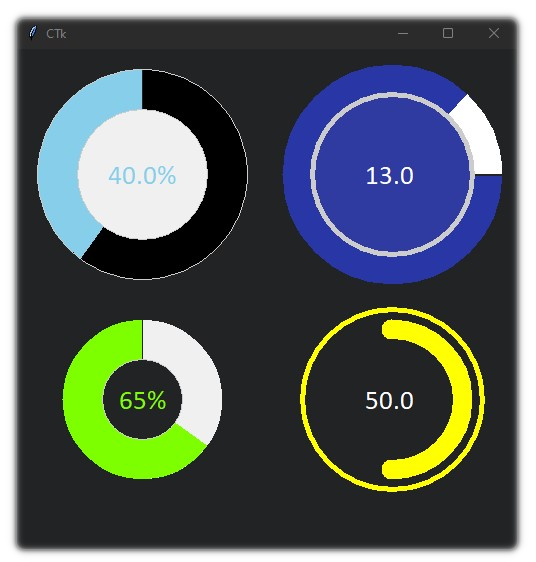
## Arguments:
| Parameters | Description |
| -------- | ----------- |
| _master_ | The master parameter is the parent widget |
| _bg_ | The default background color of the knob widget |
| _width_ | Define width of the widget manually (optional) |
| _height_ | Define height of the widget manually (optional) |
| _start_ | The start point of the range from where the bar will rotate |
| _end_ | The end point of the range |
| _radius_ | Define the radius of the knob |
| _border_width_ | Define the width of progress bar with respect to the outer and inner ring |
| _start_angle_ | Determines the angle from where to rotate |
| _text_ | A string that will be displayed on the knob with value |
| _text_color_ | Specify the color of the text that will be displayed on the knob |
| _text_font_ | Specify the font of the text that will be displayed on the knob |
| _integer_ | A boolean (True/False), displays only the integer value in text if True (default=False) |
| _fg_ | Specify the color of the inner circle |
| _progress_color_ | Define the color of the progress bar |
| _bar_color_ | Define the color of the progress bar's background |
| _inner_width_ | Define the width of the inner ring |
| _inner_color_ | Specify the color of the inner ring |
| _outer_width_ | Define the width of the outer ring |
| _outer_length_ | Define the distance between progress bar and outer ring |
| _inner_color_ | Specify the color of the outer ring |
| _steps_ | Number of steps per scroll |
| _state_ | Specify the state of the needle |
| _command_ | Call a function whenever the bar is moved |
### Methods:
| Methods | Description |
|-----------|-------------|
| _.get()_ | get the current value of the knob |
| _.set()_ | set the value of the knob |
| _.configure()_ | configure parameters of the knob |
# Meter
## Usage
**Simple Example**
```python
import tkinter
from tkdial import Meter
root = tkinter.Tk()
dial = Meter(root)
dial.pack(padx=10, pady=10)
root.mainloop()
```
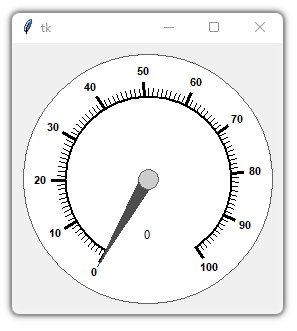
### **Different Meter Styles:**
```python
import customtkinter
from tkdial import Meter
app = customtkinter.CTk()
app.geometry("950x350")
meter1 = Meter(app, radius=300, start=0, end=160, border_width=0,
fg="black", text_color="white", start_angle=270, end_angle=-270,
text_font="DS-Digital 30", scale_color="white", needle_color="red")
meter1.set_mark(140, 160) # set red marking from 140 to 160
meter1.grid(row=0, column=1, padx=20, pady=30)
meter2 = Meter(app, radius=260, start=0, end=200, border_width=5,
fg="black", text_color="white", start_angle=270, end_angle=-360,
text_font="DS-Digital 30", scale_color="black", axis_color="white",
needle_color="white")
meter2.set_mark(1, 100, "#92d050")
meter2.set_mark(105, 150, "yellow")
meter2.set_mark(155, 196, "red")
meter2.set(80) # set value
meter2.grid(row=0, column=0, padx=20, pady=30)
meter3 = Meter(app, fg="#242424", radius=300, start=0, end=50,
major_divisions=10, border_width=0, text_color="white",
start_angle=0, end_angle=-360, scale_color="white", axis_color="cyan",
needle_color="white", scroll_steps=0.2)
meter3.set(15)
meter3.grid(row=0, column=2, pady=30)
app.mainloop()
```
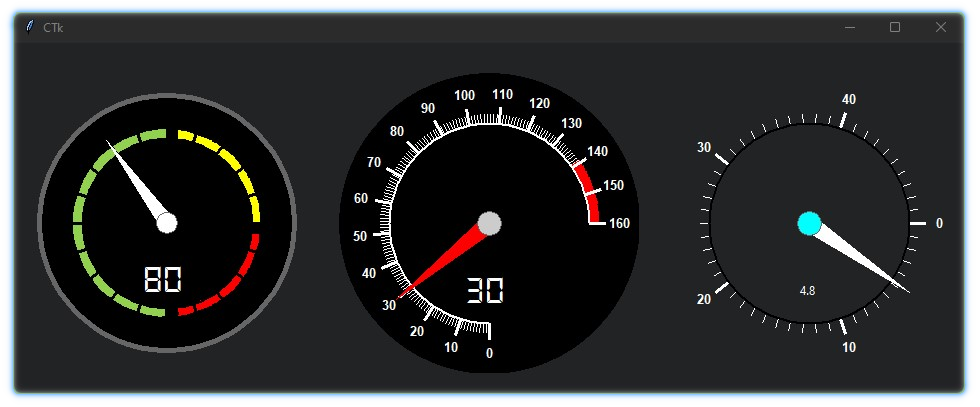
## Arguments:
| Parameters | Description |
| -------- | ----------- |
| _master_ | The master parameter is the parent widget |
| _bg_ | The default background color of the meter widget |
| _fg_ | Specify the color of the meter face |
| _width_ | Define width of the widget manually (optional) |
| _height_ | Define height of the widget manually (optional) |
| _start_ | The start point of the range from where the needle will rotate |
| _end_ | The end point of the range |
| _start_angle_ | Determines the starting angle of the arc |
| _end_angle_ | Determines the final angle of the arc |
| _radius_ | Determines the radius for the widget |
| _major_divisions_ | Determines the number of major lines in the scale |
| _minor_divisions_ | Determines the number of minor lines in the scale |
| _scale_color_ | Specify the color of the meter scale |
| _border_width_ | Define the width of the border case (default=1) |
| _border_color_ | Specify the color of the border case |
| _needle_color_ | Specify the color of the needle line |
| _axis_color_ | Specify which color of the axis wheel |
| _text_ | A string that will be displayed under the meter with value |
| _text_color_ | Specify the color of the text that will be displayed under the meter |
| _text_font_ | Specify the font of the text that will be displayed under the meter |
| _integer_ | A boolean (True/False), displays only the integer value in text if True (default=False) |
| _scroll_ | A boolean (True/False), enables mouse scroll in meter (default=True) |
| _scroll_steps_ | Number of steps per scroll |
| _state_ | Unbind/Bind the mouse movement with the needle |
| _command_ | Call a function whenever the needle is rotated |
### Methods:
| Methods | Description |
|-----------|-------------|
| _.get()_ | get the current value of the meter |
| _.set()_ | set the value of the meter |
| _.configure()_ | configure parameters of the meter|
| _.set_mark()_ | set markings for the scale. Eg: **meter.set_mark(from, to, color)** |
# JogWheel
## Usage
```python
import tkinter
from tkdial import Jogwheel
app = tkinter.Tk()
knob = Jogwheel(app)
knob.grid()
app.mainloop()
```
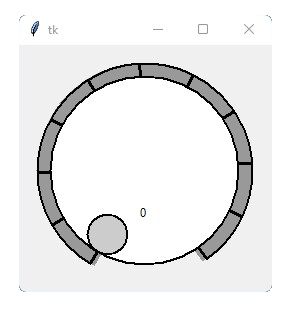
### Styles:
```python
import customtkinter
from tkdial import Jogwheel
app = customtkinter.CTk()
wheel_1 = Jogwheel(app, radius=200, fg="#045252", scale_color="white",
text=None, button_radius=10)
wheel_1.set_mark(0,100, "green")
wheel_1.grid()
wheel_2 = Jogwheel(app, radius=200, fg="#045252", scale_color="white", start_angle=0,
end_angle=360, start=0, end=200, text="Volume: ", button_radius=10)
wheel_2.set_mark(0,50, "blue")
wheel_2.set_mark(50, 90, "green")
wheel_2.set_mark(90, 150, "orange")
wheel_2.set_mark(150, 200, "red")
wheel_2.grid()
app.mainloop()
```
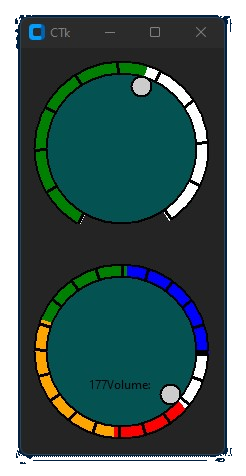
## Arguments:
| Parameters | Description |
| -------- | ----------- |
| _master_ | The master parameter is the parent widget |
| _bg_ | The default background color of the widget |
| _fg_ | Specify the color of the wheel face |
| _width_ | Define width of the widget manually (optional) |
| _height_ | Define height of the widget manually (optional) |
| _start_ | The start point of the range from where the knob will rotate |
| _end_ | The end point of the range |
| _start_angle_ | Determines the starting angle of the arc |
| _end_angle_ | Determines the final angle of the arc |
| _radius_ | Determines the radius for the widget |
| _divisions_ | Determines the number of scale lines in the scale |
| _division_height_ | Determines the height of scale lines |
| _scale_color_ | Specify the color of the knob scale |
| _border_width_ | Define the width of the border case (default=1) |
| _border_color_ | Specify the color of the border case |
| _button_color_ | Specify the color of the knob |
| _button_radius_ | Specify the radius the knob |
| _text_ | A string that will be displayed with value |
| _text_color_ | Specify the color of the text that will be displayed |
| _text_font_ | Specify the font of the text that will be displayed |
| _integer_ | A boolean (True/False), displays only the integer value in text if True (default=False) |
| _scroll_ | A boolean (True/False), enables mouse scroll (default=True) |
| _scroll_steps_ | Number of steps per scroll |
| _state_ | Unbind/Bind the mouse movement with the widget |
| _command_ | Call a function whenever the needle is rotated |
### Methods:
| Methods | Description |
|-----------|-------------|
| _.get()_ | get the current value of the knob |
| _.set()_ | set the value of the knob |
| _.configure()_ | configure parameters of the knob |
| _.set_mark()_ | set markings for the scale. Eg: **meter.set_mark(from, to, color)** |
## ImageKnob
### Usage
```python
import tkinter
from tkdial import ImageKnob
app = tkinter.Tk()
customknob = ImageKnob(app, image="knob.png")
customknob.grid()
app.mainloop()
```
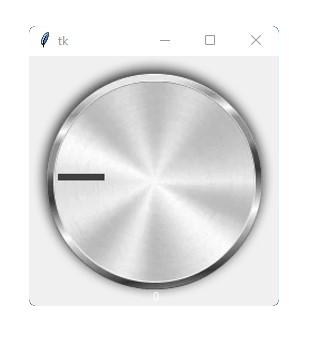
### Styles:
```python
# Note: images are not provided, only for reference
import customtkinter
from tkdial import ImageKnob
app = customtkinter.CTk()
customknob = ImageKnob(app, image="knob.png", text_color="white", text="Volume ")
customknob.grid(row=0, column=0)
customknob2 = ImageKnob(app, image="knob2.png", scale_image="scale1.png",text="", scale_width=120)
customknob2.grid(row=0, column=1, padx=20)
customknob3 = ImageKnob(app, image="knob3.png", scale_image="scale2.png",text="",
scale_width=50, start_angle=20, end_angle=-240,
progress_color="cyan", progress=True)
customknob3.grid(row=0, column=2)
app.mainloop()
```
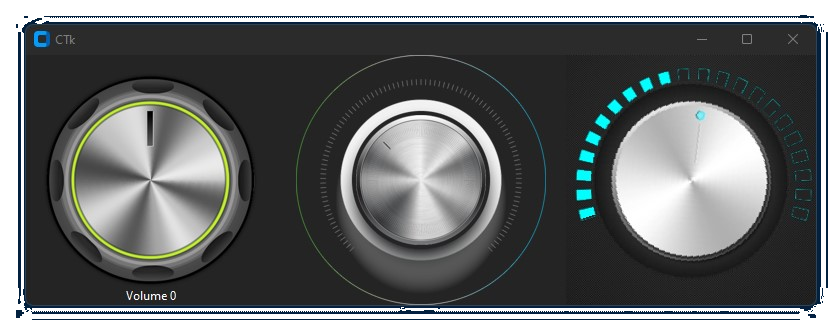
## Arguments:
| Parameters | Description |
| -------- | ----------- |
| _master_ | The master parameter is the parent widget |
| _bg_ | The default background color of the widget |
| _width_ | Define width of the widget manually (optional) |
| _height_ | Define height of the widget manually (optional) |
| _start_ | The start point of the range from where the knob will rotate |
| _end_ | The end point of the range |
| _image_ | pass the knob image |
| _scale_image_ | add a scale image (optional) |
| _scale_width_ | specify relative distance between scale and knob image |
| _start_angle_ | Determines the starting angle of the knob |
| _end_angle_ | Determines the final angle of the knob |
| _radius_ | Determines the radius for the widget |
| _text_ | A string that will be displayed with value |
| _text_color_ | Specify the color of the text that will be displayed |
| _text_font_ | Specify the font of the text that will be displayed |
| _integer_ | A boolean (True/False), displays only the integer value in text if True (default=False) |
| _scroll_ | A boolean (True/False), enables mouse scroll (default=True) |
| _scroll_steps_ | Number of steps per scroll |
| _state_ | Unbind/Bind the mouse movement with the widget |
| _command_ | Call a function whenever the needle is rotated |
### Methods:
| Methods | Description |
|-----------|-------------|
| _.get()_ | get the current value of the widget |
| _.set()_ | set the value of the widget |
| _.configure()_ | configure parameters of the widget |
Note: Images should be cropped in fixed ratio (1:1) and saved with transparency(png).
## Conclusion
This library is focused to create some circular widgets that can be used with **Tkinter/Customtkinter** easily.
I hope it will be helpful in UI development with python.
[<img src="https://img.shields.io/badge/LICENSE-CC0_v0.1-informational?&color=blue&style=for-the-badge" width="200">](https://github.com/Akascape/TkDial/blob/main/LICENSE)
Raw data
{
"_id": null,
"home_page": "https://github.com/Akascape/TkDial",
"name": "tkdial",
"maintainer": "",
"docs_url": null,
"requires_python": ">=3.6",
"maintainer_email": "",
"keywords": "tkinter,tkinter-dial,tkinter-widget,tkinter-knob,tkinter-meter,tkinter-dial-knob,dial-knob-widget,tkinter-gui",
"author": "Akash Bora",
"author_email": "",
"download_url": "https://files.pythonhosted.org/packages/31/28/914bb0e866143602fc6d7c150c2c070f01e8ac20cacdced95c31e4d83e59/tkdial-0.0.7.tar.gz",
"platform": null,
"description": "# TkDial\r\n**This is a library containing some circular rotatory dial-knob widgets for Tkinter. It can be used in place of normal sliders and scale.**\r\n\r\n[](https://pypi.org/project/tkdial)\r\n\r\n[](https://pepy.tech/project/tkdial)\r\n\r\n## Installation\r\n```\r\npip install tkdial\r\n```\r\n\r\n# Dial Widget\r\n\r\n## Usage\r\n\r\n**Simple Example:**\r\n```python\r\nimport tkinter as tk\r\nfrom tkdial import Dial\r\n\r\napp = tk.Tk()\r\n\r\ndial = Dial(app)\r\ndial.grid(padx=10, pady=10)\r\n\r\napp.mainloop()\r\n```\r\n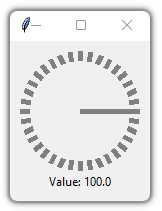\r\n\r\n### **Example 2**\r\n```python\r\nimport tkinter as tk\r\nfrom tkdial import Dial\r\n\r\napp = tk.Tk()\r\n\r\n# some color combinations\r\ncolor_combinations = [(\"yellow\", \"red\"), (\"white\", \"cyan\"), (\"red\", \"pink\"), (\"black\", \"green\"),\r\n (\"white\", \"black\"), (\"blue\", \"blue\"), (\"green\", \"green\"), (\"white\", \"pink\"),\r\n (\"red\", \"black\"), (\"green\", \"cyan\"), (\"cyan\",\"black\"), (\"pink\", \"blue\")]\r\n\r\nfor i in range (12):\r\n dial = Dial(master=app, color_gradient=color_combinations[i],\r\n unit_length=10, radius=40, needle_color=color_combinations[i][1])\r\n if i<6:\r\n dial.grid(row=1, padx=10, pady=10, column=i)\r\n else:\r\n dial.grid(row=2, padx=10, pady=10, column=11-i)\r\n\r\napp.mainloop()\r\n```\r\n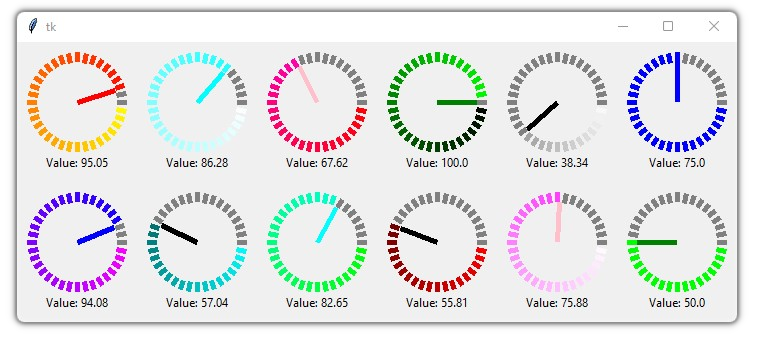\r\n\r\n### **Example 3**\r\n\r\n**Implemented with [CustomTkinter](https://github.com/TomSchimansky/CustomTkinter)**\r\n\r\n```python\r\nimport customtkinter\r\nfrom tkdial import Dial\r\n\r\ncustomtkinter.set_appearance_mode(\"Dark\") \r\n \r\napp = customtkinter.CTk()\r\napp.geometry(\"350x400\")\r\n \r\napp.grid_columnconfigure((0,1), weight=1)\r\napp.grid_rowconfigure((0,1), weight=1)\r\n\r\nframe_1 = customtkinter.CTkFrame(master=app)\r\nframe_1.grid(padx=20, pady=20, sticky=\"nswe\")\r\n\r\ndial1 = Dial(master=frame_1, color_gradient=(\"green\", \"cyan\"),\r\n text_color=\"white\", text=\"Current: \", unit_length=10, radius=50)\r\ndial1.grid(padx=20, pady=20)\r\n\r\ndial2 = Dial(master=frame_1, color_gradient=(\"yellow\", \"white\"),\r\n text_color=\"white\", text=\"Position: \", unit_length=10, radius=50)\r\ndial2.grid(padx=20, pady=20)\r\n\r\ndial3 = Dial(master=frame_1, color_gradient=(\"white\", \"pink\"),\r\n text_color=\"white\", text=\" \", unit_length=10, radius=50)\r\ndial3.grid(row=0, column=1, padx=20, pady=20)\r\n\r\ndial4 = Dial(master=frame_1, color_gradient=(\"green\", \"green\"),\r\n text_color=\"white\", text=\"\", unit_width=15, radius=50)\r\ndial4.grid(row=1, column=1, padx=20, pady=20)\r\n\r\napp.mainloop() \r\n```\r\n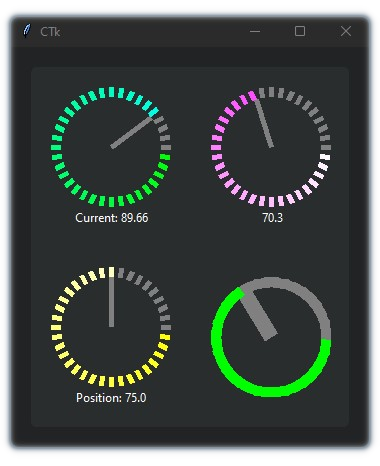\r\n\r\n## Arguments:\r\n | Parameters | Description |\r\n | -------- | ----------- |\r\n | _master_ | The master parameter is the parent widget |\r\n | _bg_ | The default background color of the dial widget |\r\n | _width_ | Define width of the widget manually (optional) |\r\n | _height_ | Define height of the widget manually (optional) |\r\n | _x_ | Determines the horizontal position of the dial in the canvas |\r\n | _y_ | Determines the vertical position of the dial in the canvas |\r\n | _start_ | The start point of the range from where the needle will rotate |\r\n | _end_ | The end point of the range |\r\n | _radius_ | Determines the distance of the unit lines between the center and the edge and also the length of the needle line |\r\n | _unit_length_ | Specify the length of the lines |\r\n | _unit_width_ | Specify the width of the lines |\r\n | _unit_color_ | Specify the color of the unit lines |\r\n | _needle_color_ | Specify the color of the needle line |\r\n | _color_gradient_ | Specify which color gradient will be used for the units |\r\n | _text_ | A string that will be displayed under the dial object with value |\r\n | _text_color_ | Specify the color of the text that will be displayed under the dial object |\r\n | _text_font_ | Specify the font of the text that will be displayed under the dial object |\r\n | _integer_ | A boolean (True/False), displays only the integer value in text if True (default=False) |\r\n | _scroll_ | A boolean (True/False), enables mouse scroll in dial (default=True) |\r\n | _scroll_steps_ | Number of steps per scroll |\r\n | _state_ | Specify the state of the needle |\r\n | _command_ | Call a function whenever the needle is rotated |\r\n \r\n### Methods:\r\n\r\n | Methods | Description |\r\n |-----------|-------------|\r\n | _.get()_ | get the current value of the dial |\r\n | _.set()_ | set the value of the dial |\r\n | _.configure()_ | configure parameters of the dial |\r\n \r\n# Scroll Knob\r\n\r\n## Usage\r\n**Simple Example**\r\n```python\r\nimport tkinter\r\nfrom tkdial import ScrollKnob\r\n \r\napp = tkinter.Tk()\r\n\r\nknob = ScrollKnob(app, start=0, end=100, steps=10)\r\nknob.grid()\r\n \r\napp.mainloop() \r\n```\r\n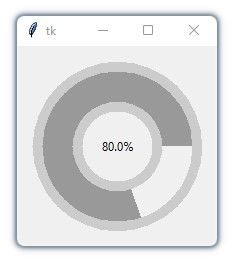\r\n\r\n### **Different Knob styles:**\r\n```python\r\nimport customtkinter\r\nfrom tkdial import ScrollKnob\r\n\r\napp = customtkinter.CTk()\r\napp.geometry(\"500x500\")\r\n\r\nknob1 = ScrollKnob(app, radius=250, progress_color=\"#87ceeb\", steps=10,\r\n border_width=40, start_angle=90, inner_width=1, outer_width=1,\r\n text_font=\"calibri 20\", text_color=\"#87ceeb\", bar_color=\"black\")\r\nknob1.grid(row=0, column=0)\r\n\r\nknob2 = ScrollKnob(app, radius=200, progress_color=\"#7eff00\",\r\n border_width=40, start_angle=90, inner_width=1, outer_width=0,\r\n text_font=\"calibri 20\", text_color=\"#7eff00\", integer=True,\r\n fg=\"#212325\")\r\nknob2.grid(row=1, column=0)\r\n\r\nknob3 = ScrollKnob(app, text=\" \", radius=250, progress_color=\"white\",\r\n bar_color=\"#2937a6\", border_width=30, start_angle=0, inner_width=5,\r\n outer_width=0, text_font=\"calibri 20\", steps=1, text_color=\"white\", fg=\"#303ba1\")\r\nknob3.grid(row=0, column=1)\r\n\r\nknob4 = ScrollKnob(app, text=\" \", steps=10, radius=200, bar_color=\"#242424\", \r\n progress_color=\"yellow\", outer_color=\"yellow\", outer_length=10, \r\n border_width=30, start_angle=270, inner_width=0, outer_width=5, text_font=\"calibri 20\", \r\n text_color=\"white\", fg=\"#212325\")\r\nknob4.grid(row=1, column=1)\r\n \r\napp.mainloop() \r\n```\r\n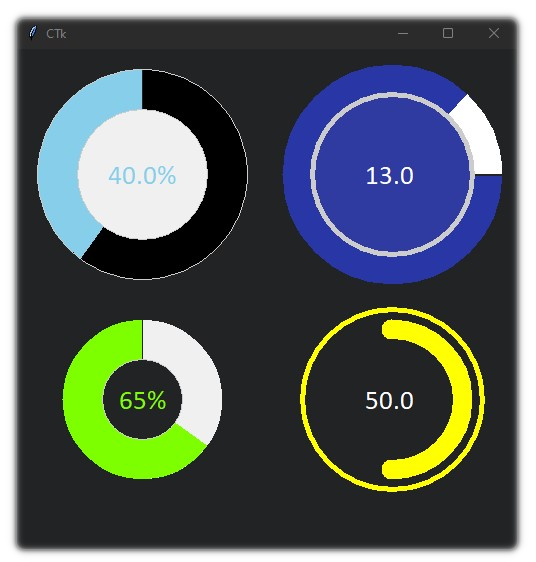\r\n\r\n## Arguments:\r\n | Parameters | Description |\r\n | -------- | ----------- |\r\n | _master_ | The master parameter is the parent widget |\r\n | _bg_ | The default background color of the knob widget |\r\n | _width_ | Define width of the widget manually (optional) |\r\n | _height_ | Define height of the widget manually (optional) |\r\n | _start_ | The start point of the range from where the bar will rotate |\r\n | _end_ | The end point of the range |\r\n | _radius_ | Define the radius of the knob |\r\n | _border_width_ | Define the width of progress bar with respect to the outer and inner ring |\r\n | _start_angle_ | Determines the angle from where to rotate |\r\n | _text_ | A string that will be displayed on the knob with value |\r\n | _text_color_ | Specify the color of the text that will be displayed on the knob |\r\n | _text_font_ | Specify the font of the text that will be displayed on the knob |\r\n | _integer_ | A boolean (True/False), displays only the integer value in text if True (default=False) |\r\n | _fg_ | Specify the color of the inner circle |\r\n | _progress_color_ | Define the color of the progress bar |\r\n | _bar_color_ | Define the color of the progress bar's background |\r\n | _inner_width_ | Define the width of the inner ring |\r\n | _inner_color_ | Specify the color of the inner ring |\r\n | _outer_width_ | Define the width of the outer ring |\r\n | _outer_length_ | Define the distance between progress bar and outer ring |\r\n | _inner_color_ | Specify the color of the outer ring |\r\n | _steps_ | Number of steps per scroll |\r\n | _state_ | Specify the state of the needle |\r\n | _command_ | Call a function whenever the bar is moved |\r\n \r\n### Methods:\r\n | Methods | Description |\r\n |-----------|-------------|\r\n | _.get()_ | get the current value of the knob |\r\n | _.set()_ | set the value of the knob |\r\n | _.configure()_ | configure parameters of the knob | \r\n \r\n# Meter\r\n\r\n## Usage\r\n**Simple Example**\r\n```python\r\nimport tkinter\r\nfrom tkdial import Meter\r\n\r\nroot = tkinter.Tk()\r\ndial = Meter(root)\r\ndial.pack(padx=10, pady=10)\r\n\r\nroot.mainloop()\r\n```\r\n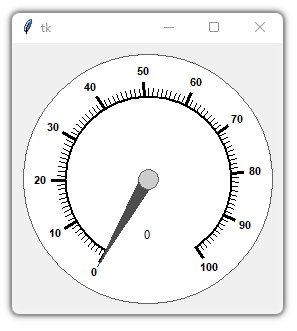\r\n\r\n### **Different Meter Styles:**\r\n```python\r\nimport customtkinter\r\nfrom tkdial import Meter\r\n\r\napp = customtkinter.CTk()\r\napp.geometry(\"950x350\")\r\n\r\nmeter1 = Meter(app, radius=300, start=0, end=160, border_width=0,\r\n fg=\"black\", text_color=\"white\", start_angle=270, end_angle=-270,\r\n text_font=\"DS-Digital 30\", scale_color=\"white\", needle_color=\"red\")\r\nmeter1.set_mark(140, 160) # set red marking from 140 to 160\r\nmeter1.grid(row=0, column=1, padx=20, pady=30)\r\n\r\nmeter2 = Meter(app, radius=260, start=0, end=200, border_width=5,\r\n fg=\"black\", text_color=\"white\", start_angle=270, end_angle=-360,\r\n text_font=\"DS-Digital 30\", scale_color=\"black\", axis_color=\"white\",\r\n needle_color=\"white\")\r\nmeter2.set_mark(1, 100, \"#92d050\")\r\nmeter2.set_mark(105, 150, \"yellow\")\r\nmeter2.set_mark(155, 196, \"red\")\r\nmeter2.set(80) # set value\r\nmeter2.grid(row=0, column=0, padx=20, pady=30)\r\n\r\nmeter3 = Meter(app, fg=\"#242424\", radius=300, start=0, end=50,\r\n major_divisions=10, border_width=0, text_color=\"white\",\r\n start_angle=0, end_angle=-360, scale_color=\"white\", axis_color=\"cyan\",\r\n needle_color=\"white\", scroll_steps=0.2)\r\nmeter3.set(15)\r\nmeter3.grid(row=0, column=2, pady=30)\r\n\r\napp.mainloop()\r\n```\r\n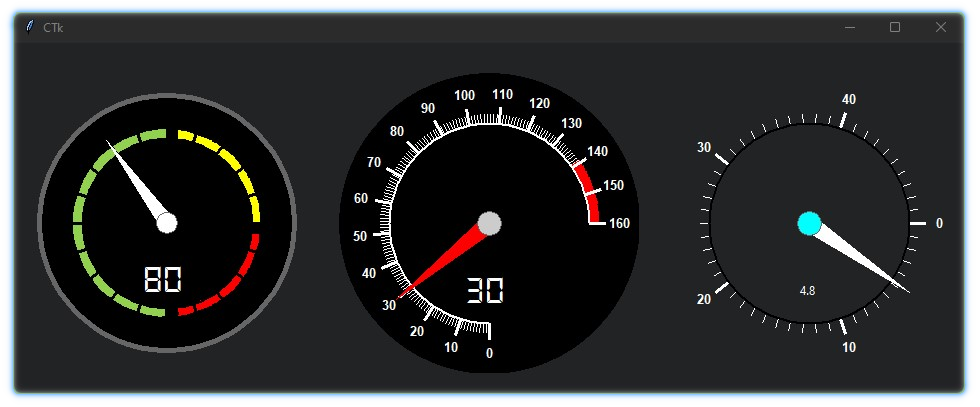\r\n\r\n## Arguments:\r\n | Parameters | Description |\r\n | -------- | ----------- |\r\n | _master_ | The master parameter is the parent widget |\r\n | _bg_ | The default background color of the meter widget |\r\n | _fg_ | Specify the color of the meter face |\r\n | _width_ | Define width of the widget manually (optional) |\r\n | _height_ | Define height of the widget manually (optional) |\r\n | _start_ | The start point of the range from where the needle will rotate |\r\n | _end_ | The end point of the range |\r\n | _start_angle_ | Determines the starting angle of the arc |\r\n | _end_angle_ | Determines the final angle of the arc |\r\n | _radius_ | Determines the radius for the widget |\r\n | _major_divisions_ | Determines the number of major lines in the scale |\r\n | _minor_divisions_ | Determines the number of minor lines in the scale |\r\n | _scale_color_ | Specify the color of the meter scale |\r\n | _border_width_ | Define the width of the border case (default=1) |\r\n | _border_color_ | Specify the color of the border case |\r\n | _needle_color_ | Specify the color of the needle line |\r\n | _axis_color_ | Specify which color of the axis wheel |\r\n | _text_ | A string that will be displayed under the meter with value |\r\n | _text_color_ | Specify the color of the text that will be displayed under the meter |\r\n | _text_font_ | Specify the font of the text that will be displayed under the meter |\r\n | _integer_ | A boolean (True/False), displays only the integer value in text if True (default=False) |\r\n | _scroll_ | A boolean (True/False), enables mouse scroll in meter (default=True) |\r\n | _scroll_steps_ | Number of steps per scroll |\r\n | _state_ | Unbind/Bind the mouse movement with the needle |\r\n | _command_ | Call a function whenever the needle is rotated |\r\n \r\n### Methods:\r\n | Methods | Description |\r\n |-----------|-------------|\r\n | _.get()_ | get the current value of the meter |\r\n | _.set()_ | set the value of the meter |\r\n | _.configure()_ | configure parameters of the meter| \r\n | _.set_mark()_ | set markings for the scale. Eg: **meter.set_mark(from, to, color)** | \r\n \r\n# JogWheel\r\n\r\n## Usage\r\n\r\n```python\r\nimport tkinter\r\nfrom tkdial import Jogwheel\r\n\r\napp = tkinter.Tk()\r\n\r\nknob = Jogwheel(app)\r\nknob.grid()\r\n\r\napp.mainloop()\r\n```\r\n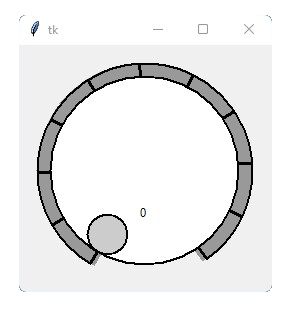\r\n\r\n### Styles: \r\n```python\r\nimport customtkinter\r\nfrom tkdial import Jogwheel\r\n\r\napp = customtkinter.CTk()\r\n\r\nwheel_1 = Jogwheel(app, radius=200, fg=\"#045252\", scale_color=\"white\",\r\n text=None, button_radius=10)\r\nwheel_1.set_mark(0,100, \"green\")\r\n\r\nwheel_1.grid()\r\n\r\nwheel_2 = Jogwheel(app, radius=200, fg=\"#045252\", scale_color=\"white\", start_angle=0,\r\n end_angle=360, start=0, end=200, text=\"Volume: \", button_radius=10)\r\nwheel_2.set_mark(0,50, \"blue\")\r\nwheel_2.set_mark(50, 90, \"green\")\r\nwheel_2.set_mark(90, 150, \"orange\")\r\nwheel_2.set_mark(150, 200, \"red\")\r\nwheel_2.grid()\r\n\r\napp.mainloop()\r\n```\r\n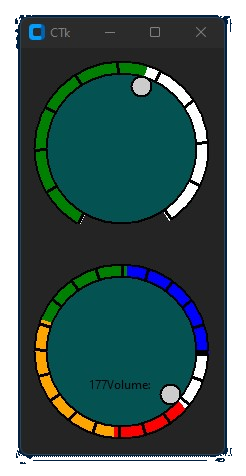\r\n\r\n\r\n## Arguments:\r\n | Parameters | Description |\r\n | -------- | ----------- |\r\n | _master_ | The master parameter is the parent widget |\r\n | _bg_ | The default background color of the widget |\r\n | _fg_ | Specify the color of the wheel face |\r\n | _width_ | Define width of the widget manually (optional) |\r\n | _height_ | Define height of the widget manually (optional) |\r\n | _start_ | The start point of the range from where the knob will rotate |\r\n | _end_ | The end point of the range |\r\n | _start_angle_ | Determines the starting angle of the arc |\r\n | _end_angle_ | Determines the final angle of the arc |\r\n | _radius_ | Determines the radius for the widget |\r\n | _divisions_ | Determines the number of scale lines in the scale |\r\n | _division_height_ | Determines the height of scale lines |\r\n | _scale_color_ | Specify the color of the knob scale |\r\n | _border_width_ | Define the width of the border case (default=1) |\r\n | _border_color_ | Specify the color of the border case |\r\n | _button_color_ | Specify the color of the knob |\r\n | _button_radius_ | Specify the radius the knob |\r\n | _text_ | A string that will be displayed with value |\r\n | _text_color_ | Specify the color of the text that will be displayed |\r\n | _text_font_ | Specify the font of the text that will be displayed |\r\n | _integer_ | A boolean (True/False), displays only the integer value in text if True (default=False) |\r\n | _scroll_ | A boolean (True/False), enables mouse scroll (default=True) |\r\n | _scroll_steps_ | Number of steps per scroll |\r\n | _state_ | Unbind/Bind the mouse movement with the widget |\r\n | _command_ | Call a function whenever the needle is rotated |\r\n \r\n### Methods:\r\n | Methods | Description |\r\n |-----------|-------------|\r\n | _.get()_ | get the current value of the knob |\r\n | _.set()_ | set the value of the knob |\r\n | _.configure()_ | configure parameters of the knob | \r\n | _.set_mark()_ | set markings for the scale. Eg: **meter.set_mark(from, to, color)** | \r\n \r\n## ImageKnob\r\n### Usage\r\n```python\r\nimport tkinter\r\nfrom tkdial import ImageKnob\r\n\r\napp = tkinter.Tk()\r\n\r\ncustomknob = ImageKnob(app, image=\"knob.png\")\r\ncustomknob.grid()\r\n\r\napp.mainloop()\r\n```\r\n\r\n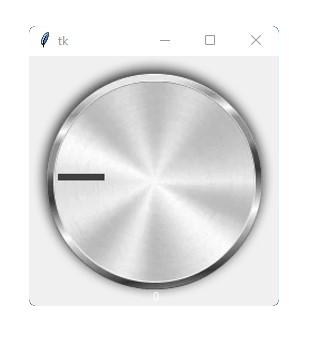\r\n\r\n### Styles:\r\n```python\r\n# Note: images are not provided, only for reference\r\nimport customtkinter\r\nfrom tkdial import ImageKnob\r\n\r\napp = customtkinter.CTk()\r\n\r\ncustomknob = ImageKnob(app, image=\"knob.png\", text_color=\"white\", text=\"Volume \")\r\ncustomknob.grid(row=0, column=0)\r\n\r\ncustomknob2 = ImageKnob(app, image=\"knob2.png\", scale_image=\"scale1.png\",text=\"\", scale_width=120)\r\ncustomknob2.grid(row=0, column=1, padx=20)\r\n\r\ncustomknob3 = ImageKnob(app, image=\"knob3.png\", scale_image=\"scale2.png\",text=\"\",\r\n scale_width=50, start_angle=20, end_angle=-240,\r\n progress_color=\"cyan\", progress=True)\r\ncustomknob3.grid(row=0, column=2)\r\n\r\napp.mainloop()\r\n```\r\n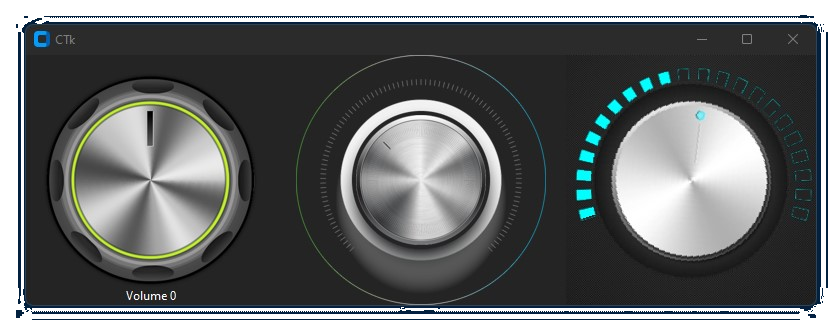\r\n\r\n\r\n## Arguments:\r\n | Parameters | Description |\r\n | -------- | ----------- |\r\n | _master_ | The master parameter is the parent widget |\r\n | _bg_ | The default background color of the widget |\r\n | _width_ | Define width of the widget manually (optional) |\r\n | _height_ | Define height of the widget manually (optional) |\r\n | _start_ | The start point of the range from where the knob will rotate |\r\n | _end_ | The end point of the range |\r\n | _image_ | pass the knob image |\r\n | _scale_image_ | add a scale image (optional) |\r\n | _scale_width_ | specify relative distance between scale and knob image |\r\n | _start_angle_ | Determines the starting angle of the knob |\r\n | _end_angle_ | Determines the final angle of the knob |\r\n | _radius_ | Determines the radius for the widget |\r\n | _text_ | A string that will be displayed with value |\r\n | _text_color_ | Specify the color of the text that will be displayed |\r\n | _text_font_ | Specify the font of the text that will be displayed |\r\n | _integer_ | A boolean (True/False), displays only the integer value in text if True (default=False) |\r\n | _scroll_ | A boolean (True/False), enables mouse scroll (default=True) |\r\n | _scroll_steps_ | Number of steps per scroll |\r\n | _state_ | Unbind/Bind the mouse movement with the widget |\r\n | _command_ | Call a function whenever the needle is rotated |\r\n \r\n### Methods:\r\n | Methods | Description |\r\n |-----------|-------------|\r\n | _.get()_ | get the current value of the widget |\r\n | _.set()_ | set the value of the widget |\r\n | _.configure()_ | configure parameters of the widget | \r\n\r\nNote: Images should be cropped in fixed ratio (1:1) and saved with transparency(png).\r\n\r\n## Conclusion\r\nThis library is focused to create some circular widgets that can be used with **Tkinter/Customtkinter** easily.\r\nI hope it will be helpful in UI development with python.\r\n\r\n[<img src=\"https://img.shields.io/badge/LICENSE-CC0_v0.1-informational?&color=blue&style=for-the-badge\" width=\"200\">](https://github.com/Akascape/TkDial/blob/main/LICENSE)\r\n",
"bugtrack_url": null,
"license": "Creative Commons Zero v1.0 Universal",
"summary": "Rotatory dial-knob widgets for Tkinter.",
"version": "0.0.7",
"project_urls": {
"Homepage": "https://github.com/Akascape/TkDial"
},
"split_keywords": [
"tkinter",
"tkinter-dial",
"tkinter-widget",
"tkinter-knob",
"tkinter-meter",
"tkinter-dial-knob",
"dial-knob-widget",
"tkinter-gui"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "9bf06769ca194d4c23505dd34fa3f78db583fe45320603212a06fe7689bffa6f",
"md5": "bb7f518187aa962b9eb81c6cd08f815f",
"sha256": "ebf7322c5607c8772054f35daa042731a1c2c2c158e77d2bf715646fa8458c82"
},
"downloads": -1,
"filename": "tkdial-0.0.7-py3-none-any.whl",
"has_sig": false,
"md5_digest": "bb7f518187aa962b9eb81c6cd08f815f",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.6",
"size": 23325,
"upload_time": "2023-05-21T12:32:08",
"upload_time_iso_8601": "2023-05-21T12:32:08.763494Z",
"url": "https://files.pythonhosted.org/packages/9b/f0/6769ca194d4c23505dd34fa3f78db583fe45320603212a06fe7689bffa6f/tkdial-0.0.7-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "3128914bb0e866143602fc6d7c150c2c070f01e8ac20cacdced95c31e4d83e59",
"md5": "da7d662f351c791f4dae19e786df9367",
"sha256": "8101831e4961b683a94dc12526e96fa79c582a62d02596023fae76b585612189"
},
"downloads": -1,
"filename": "tkdial-0.0.7.tar.gz",
"has_sig": false,
"md5_digest": "da7d662f351c791f4dae19e786df9367",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.6",
"size": 21523,
"upload_time": "2023-05-21T12:32:11",
"upload_time_iso_8601": "2023-05-21T12:32:11.667481Z",
"url": "https://files.pythonhosted.org/packages/31/28/914bb0e866143602fc6d7c150c2c070f01e8ac20cacdced95c31e4d83e59/tkdial-0.0.7.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-05-21 12:32:11",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "Akascape",
"github_project": "TkDial",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"lcname": "tkdial"
}