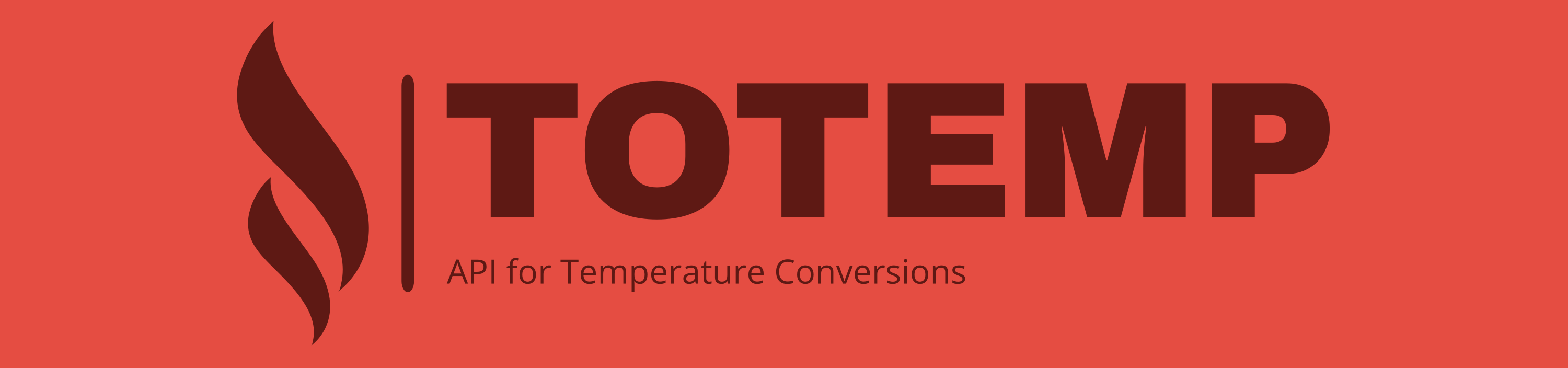
# ToTemp
<div style="display: inline-block">
<img src="https://shields.io/pypi/v/totemp" alt="package version"/>
<img src="https://img.shields.io/pypi/l/totemp.svg" alt="license"/>
<img src="https://results.pre-commit.ci/badge/github/eddyyxxyy/ToTemp/main.svg" alt="pre-commit.ci"/>
<img src='https://readthedocs.org/projects/totemp/badge/?version=latest' alt='Documentation Status'/>
</div>
**ToTemp** is a temperature conversion package with Celsius, Delisle, Fahrenheit, Kelvin, Rankine, Réaumur, Newton and Rømer scales.
For more information [read the docs](https://totemp.readthedocs.io/en/latest/).
> This package aims to bring the simple and straight to the point,
but precise, Object Oriented experience of working with temperature
scale data types.
---
## Usage
First of all, install the package:
````shell
pip install totemp
````
or, to have an example in poetry environments:
````shell
poetry add totemp
````
> For more information, read the docs: [ToTemp Docs]('insert link here')
### The instances:
````python
from totemp import Celsius, Fahrenheit
if __name__ == '__main__':
temps: list = [Celsius(12), Celsius(25), Celsius(50)]
print(temps[0]) # '12 ºC'
print(temps) # [Celsius(12), Celsius(25), Celsius(50)]
temps = list(map(Celsius.to_fahrenheit, temps))
print(temps[0]) # '53.6 ºF'
print(temps) # [Fahrenheit(53.6), Fahrenheit(77.0), Fahrenheit(122.0)]
````
### It's representations and properties:
> Property *`symbol`* is **read-only**.
````python
from totemp import Fahrenheit
if __name__ == '__main__':
temp0 = Fahrenheit(53.6)
print(temp0.__repr__()) # 'Fahrenheit(53.6)'
print(temp0.__str__()) # '53.6 ºF'
print(temp0.symbol) # 'ºF'
print(temp0.value) # 53.6
````
### Comparision operations ('==', '!=', '>', '>=', '<',...):
> The comparision/arithmetic implementation attempts to convert the value of `other` (if it is a temperature instance) and then evaluate the expression.
````python
import totemp as tp
if __name__ == '__main__':
temp0, temp1 = tp.Celsius(0), tp.Fahrenheit(32)
print(f'temp0: {repr(temp0)}') # Celsius(0)
print(f'temp1: {repr(temp1.to_celsius())}') # Celsius(0.0)
print(temp0 != temp1) # False
print(temp0 > temp1) # False
print(temp0 < temp1) # False
print(temp0 >= temp1) # True
print(temp0 <= temp1) # True
print(temp0 == temp1) # True
````
### Arithmetic operations ('+', '-', '*', '**', '/', '//', '%', ...):
````python
from totemp import Newton, Rankine
if __name__ == '__main__':
temp0 = Newton(33)
temp1 = Rankine(671.67)
temp2 = temp0 + temp1
print('temp2:', temp2) # temp2: 65.99999999999999 ºN
print('temp2:', repr(temp2)) # temp2: Newton(65.99999999999999)
print('temp2:', temp2.value, temp2.symbol) # temp2: 65.99999999999999 ºN
print((temp0 + temp1).rounded()) # 66 ºN
print(repr((temp0 + temp1).rounded())) # Newton(66)
print(temp2 + 12.55) # 78.54999999999998 ºN
print((12 + temp2.rounded())) # 78 ºN
````
### ToTemp classes can work with many built-in Python functions:
````python
from math import floor, ceil, trunc
from totemp import Reaumur
if __name__ == '__main__':
temp = Reaumur(100.4)
float(temp) # 100.4
int(temp) # 100
round(temp) # Reaumur(100)
abs(temp) # Reaumur(100)
floor(temp) # Reaumur(100)
ceil(temp) # Reaumur(101)
trunc(temp) # Reaumur(100)
divmod(temp, temp0 := Reaumur(25.1)) # (Reaumur(4.0), Reaumur(0.0))
````
### Temperature Instance Conversions:
````python
import totemp
if __name__ == '__main__':
temp = totemp.Fahrenheit(32)
print(temp.to_celsius()) # 0.0 ºC
print(temp.to_fahrenheit()) # 32 ºF
print(temp.to_delisle()) # 150.0 ºDe
print(temp.to_kelvin()) # 273.15 K
print(temp.to_newton()) # 0.0 ºN
print(temp.to_rankine()) # 491.67 ºR
print(temp.to_reaumur()) # 0.0 ºRé
print(temp.to_romer()) # 7.5 ºRø
````
## Changelog
---
- _0.1.0_:
- Yanked, not functional;
- _0.2.0_:
- Functional;
- Can convert Celsius to Delisle, Fahrenheit, Kelvin, Newton, Rankine, Réaumur and Rømer.
- _0.3.0_:
- Changed methods implementations and adds Fahrenheit conversions;
- <scale_value> parameter is now positional-only;
- Adds new parameter -> float_ret -> Float Return (True by default, keyword-only);
- Celsius class methods were updated and enhanced;
- Can now convert Fahrenheit to Celsius, Delisle, Kelvin, Newton, Rankine, Réaumur and Rømer.
- _0.4.0_:
- There are two new Classes, Kelvin and Delisle, functional and ready-to-use.
- **0.5.1**:
- The implementation has been **completely refactored**:
1 - ***All classes inhehits from `AbstractTemperature`** (our new abstract Base Class)*;
2 - ***All classes now available***:
- *`Celsius`;*
- *`Fahrenheit`;*
- *`Delisle`;*
- *`Kelvin`;*
- ***(\*New)** `Newton`;*
- ***(\*New)** `Rankine`;*
- ***(\*New)** `Réaumur`;*
- ***(\*New)** `Rømer`.*
3 - ***New features***:
- *The majority of Python's built-in functions works with the instances*;
- *More pythonic properties and methods implementations*;
- *Arithmetic operations;*
- *Comparision operations;*
- *`convert_to()` method;*
4 - ***Removals***:
- *`precise()` method;*
- *`float_ret()` param;*
- *differentiating int/float;*
5 - ***Known problemns***:
- *`pow()` doesn't work as intended;*
---
## License
For more information, check LICENSE file.
Raw data
{
"_id": null,
"home_page": "https://github.com/eddyyxxyy/ToTemp",
"name": "totemp",
"maintainer": "Edson Pimenta",
"docs_url": null,
"requires_python": ">=3.10,<4.0",
"maintainer_email": "edson.tibo@gmail.com",
"keywords": "converter,temperature,celsius,kelvin,fahrenheit,rankine,delisle,Newton,R\u00e9aumur,R\u00f8mer",
"author": "Edson Pimenta",
"author_email": "edson.tibo@gmail.com",
"download_url": "https://files.pythonhosted.org/packages/f2/28/5c9253caebbee515dc3ec0cc7392b354e9ee8b0fec59a244cee8922de310/totemp-0.5.1.tar.gz",
"platform": null,
"description": "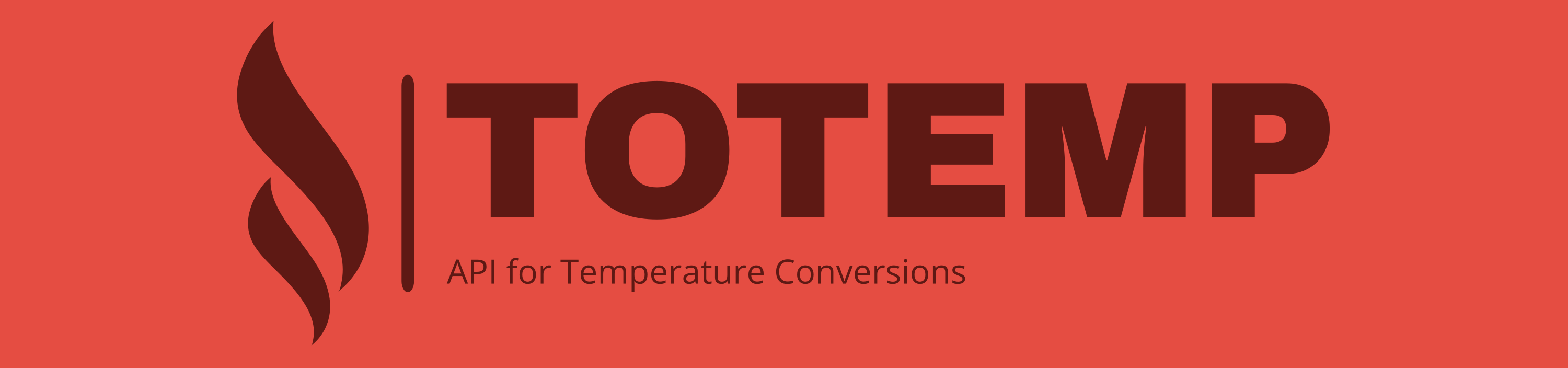\n\n# ToTemp\n<div style=\"display: inline-block\">\n <img src=\"https://shields.io/pypi/v/totemp\" alt=\"package version\"/>\n <img src=\"https://img.shields.io/pypi/l/totemp.svg\" alt=\"license\"/>\n <img src=\"https://results.pre-commit.ci/badge/github/eddyyxxyy/ToTemp/main.svg\" alt=\"pre-commit.ci\"/>\n <img src='https://readthedocs.org/projects/totemp/badge/?version=latest' alt='Documentation Status'/>\n</div>\n\n**ToTemp** is a temperature conversion package with Celsius, Delisle, Fahrenheit, Kelvin, Rankine, R\u00e9aumur, Newton and R\u00f8mer scales.\n\nFor more information [read the docs](https://totemp.readthedocs.io/en/latest/).\n\n> This package aims to bring the simple and straight to the point,\nbut precise, Object Oriented experience of working with temperature\nscale data types.\n\n---\n\n## Usage\n\nFirst of all, install the package:\n\n````shell\npip install totemp\n````\n\nor, to have an example in poetry environments:\n\n````shell\npoetry add totemp\n````\n\n> For more information, read the docs: [ToTemp Docs]('insert link here')\n\n### The instances:\n\n````python\nfrom totemp import Celsius, Fahrenheit\n\nif __name__ == '__main__':\n temps: list = [Celsius(12), Celsius(25), Celsius(50)]\n print(temps[0]) # '12 \u00baC'\n print(temps) # [Celsius(12), Celsius(25), Celsius(50)]\n\n temps = list(map(Celsius.to_fahrenheit, temps))\n print(temps[0]) # '53.6 \u00baF'\n print(temps) # [Fahrenheit(53.6), Fahrenheit(77.0), Fahrenheit(122.0)]\n````\n\n### It's representations and properties:\n\n> Property *`symbol`* is **read-only**.\n\n````python\nfrom totemp import Fahrenheit\n\nif __name__ == '__main__':\n temp0 = Fahrenheit(53.6)\n print(temp0.__repr__()) # 'Fahrenheit(53.6)'\n print(temp0.__str__()) # '53.6 \u00baF'\n print(temp0.symbol) # '\u00baF'\n print(temp0.value) # 53.6\n````\n\n### Comparision operations ('==', '!=', '>', '>=', '<',...):\n\n> The comparision/arithmetic implementation attempts to convert the value of `other` (if it is a temperature instance) and then evaluate the expression.\n\n````python\nimport totemp as tp\n\nif __name__ == '__main__':\n temp0, temp1 = tp.Celsius(0), tp.Fahrenheit(32)\n\n print(f'temp0: {repr(temp0)}') # Celsius(0)\n print(f'temp1: {repr(temp1.to_celsius())}') # Celsius(0.0)\n\n print(temp0 != temp1) # False\n\n print(temp0 > temp1) # False\n\n print(temp0 < temp1) # False\n\n print(temp0 >= temp1) # True\n\n print(temp0 <= temp1) # True\n\n print(temp0 == temp1) # True\n````\n\n### Arithmetic operations ('+', '-', '*', '**', '/', '//', '%', ...):\n\n````python\nfrom totemp import Newton, Rankine\n\nif __name__ == '__main__':\n temp0 = Newton(33)\n temp1 = Rankine(671.67)\n\n temp2 = temp0 + temp1\n\n print('temp2:', temp2) # temp2: 65.99999999999999 \u00baN\n print('temp2:', repr(temp2)) # temp2: Newton(65.99999999999999)\n print('temp2:', temp2.value, temp2.symbol) # temp2: 65.99999999999999 \u00baN\n\n print((temp0 + temp1).rounded()) # 66 \u00baN\n print(repr((temp0 + temp1).rounded())) # Newton(66)\n\n print(temp2 + 12.55) # 78.54999999999998 \u00baN\n print((12 + temp2.rounded())) # 78 \u00baN\n````\n\n### ToTemp classes can work with many built-in Python functions:\n\n````python\nfrom math import floor, ceil, trunc\n\nfrom totemp import Reaumur\n\nif __name__ == '__main__':\n temp = Reaumur(100.4)\n\n float(temp) # 100.4\n int(temp) # 100\n round(temp) # Reaumur(100)\n abs(temp) # Reaumur(100)\n floor(temp) # Reaumur(100)\n ceil(temp) # Reaumur(101)\n trunc(temp) # Reaumur(100)\n divmod(temp, temp0 := Reaumur(25.1)) # (Reaumur(4.0), Reaumur(0.0))\n\n````\n\n\n\n### Temperature Instance Conversions:\n\n````python\nimport totemp\n\nif __name__ == '__main__':\n temp = totemp.Fahrenheit(32)\n\n print(temp.to_celsius()) # 0.0 \u00baC\n print(temp.to_fahrenheit()) # 32 \u00baF\n print(temp.to_delisle()) # 150.0 \u00baDe\n print(temp.to_kelvin()) # 273.15 K\n print(temp.to_newton()) # 0.0 \u00baN\n print(temp.to_rankine()) # 491.67 \u00baR\n print(temp.to_reaumur()) # 0.0 \u00baR\u00e9\n print(temp.to_romer()) # 7.5 \u00baR\u00f8\n````\n\n## Changelog\n\n---\n\n- _0.1.0_:\n - Yanked, not functional;\n- _0.2.0_:\n - Functional;\n - Can convert Celsius to Delisle, Fahrenheit, Kelvin, Newton, Rankine, R\u00e9aumur and R\u00f8mer.\n- _0.3.0_:\n - Changed methods implementations and adds Fahrenheit conversions;\n - <scale_value> parameter is now positional-only;\n - Adds new parameter -> float_ret -> Float Return (True by default, keyword-only);\n - Celsius class methods were updated and enhanced;\n - Can now convert Fahrenheit to Celsius, Delisle, Kelvin, Newton, Rankine, R\u00e9aumur and R\u00f8mer.\n- _0.4.0_:\n - There are two new Classes, Kelvin and Delisle, functional and ready-to-use.\n\n- **0.5.1**:\n - The implementation has been **completely refactored**:\n\n 1 - ***All classes inhehits from `AbstractTemperature`** (our new abstract Base Class)*;\n\n 2 - ***All classes now available***:\n - *`Celsius`;*\n - *`Fahrenheit`;*\n - *`Delisle`;*\n - *`Kelvin`;*\n - ***(\\*New)** `Newton`;*\n - ***(\\*New)** `Rankine`;*\n - ***(\\*New)** `R\u00e9aumur`;*\n - ***(\\*New)** `R\u00f8mer`.*\n\n 3 - ***New features***:\n - *The majority of Python's built-in functions works with the instances*;\n - *More pythonic properties and methods implementations*;\n - *Arithmetic operations;*\n - *Comparision operations;*\n - *`convert_to()` method;*\n\n 4 - ***Removals***:\n - *`precise()` method;*\n - *`float_ret()` param;*\n - *differentiating int/float;*\n\n 5 - ***Known problemns***:\n - *`pow()` doesn't work as intended;*\n\n---\n\n## License\n\nFor more information, check LICENSE file.\n",
"bugtrack_url": null,
"license": "GPL-3.0-only",
"summary": "Temperature Converter",
"version": "0.5.1",
"split_keywords": [
"converter",
"temperature",
"celsius",
"kelvin",
"fahrenheit",
"rankine",
"delisle",
"newton",
"r\u00e9aumur",
"r\u00f8mer"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "39991a09c8f77ad2299951eefd10263f872035878fd894f91ddf9909d4b1812e",
"md5": "c95efddc14c7e69e30720ae03a890168",
"sha256": "809874867d555f5b8c65715405d4679e93563b4449a7599bf36fd08cd546a9ba"
},
"downloads": -1,
"filename": "totemp-0.5.1-py3-none-any.whl",
"has_sig": false,
"md5_digest": "c95efddc14c7e69e30720ae03a890168",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.10,<4.0",
"size": 20061,
"upload_time": "2023-02-06T21:38:43",
"upload_time_iso_8601": "2023-02-06T21:38:43.826295Z",
"url": "https://files.pythonhosted.org/packages/39/99/1a09c8f77ad2299951eefd10263f872035878fd894f91ddf9909d4b1812e/totemp-0.5.1-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "f2285c9253caebbee515dc3ec0cc7392b354e9ee8b0fec59a244cee8922de310",
"md5": "78c5369d57b349c4091a3d7184e87552",
"sha256": "94af38a6efcec925af37b3d3c9c13bae7207f4c9520715d079b3a055efd2c64f"
},
"downloads": -1,
"filename": "totemp-0.5.1.tar.gz",
"has_sig": false,
"md5_digest": "78c5369d57b349c4091a3d7184e87552",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.10,<4.0",
"size": 22642,
"upload_time": "2023-02-06T21:38:45",
"upload_time_iso_8601": "2023-02-06T21:38:45.525757Z",
"url": "https://files.pythonhosted.org/packages/f2/28/5c9253caebbee515dc3ec0cc7392b354e9ee8b0fec59a244cee8922de310/totemp-0.5.1.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-02-06 21:38:45",
"github": true,
"gitlab": false,
"bitbucket": false,
"github_user": "eddyyxxyy",
"github_project": "ToTemp",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "totemp"
}