# tripplite
Python USB interface and command-line tool for TrippLite UPS battery backups.
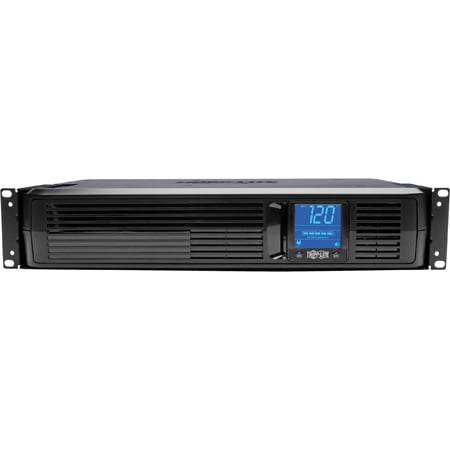
## Background
TrippLite offers [UI software](https://www.tripplite.com/products/power-alert)
for monitoring its batteries. However, most of its batteries don't have
network access, and the existing TrippLite software requires a local install.
I wanted to monitor the UPS from a remote headless Linux server, so I wrote
this tool.
## Supported Hardware
This has been exclusively tested on the TrippLite SMART1500LCD UPS. It will
likely work on similar firmware but there is a known communication issue with
some other TrippLite models (see [numat/tripplite#3](https://github.com/numat/tripplite/issues/3)).
Use `lsusb` to check. `09ae:2012` should work, while `09ae:3016` may not.
## Installation
```console
apt install gcc libusb-1.0-0-dev libudev-dev
pip install tripplite
```
Connect a USB cable from the UPS to your headless server, and you should be
ready to run. If you don't want to run as root, see *Note on Permissions*
below.
# Command Line
```
$ tripplite
{
"config": {
"frequency": 60, # Hz
"power": 1500, # VA
"voltage": 120 # V
},
"health": 100, # %
"input": {
"frequency": 59.7, # Hz
"voltage": 117.2 # V
},
"output": {
"power": 324, # W
"voltage": 117.2 # V
},
"status": {
"ac present": true,
"below remaining capacity": true,
"charging": false,
"discharging": false,
"fully charged": true,
"fully discharged": false,
"needs replacement": false,
"shutdown imminent": false
},
"time to empty": 1004 # s
}
```
To use in shell scripts, parse the json output with something like
[jq](https://stedolan.github.io/jq/). For example,
`tripplite | jq '.status."ac present"'` will return whether or not the unit
detects AC power.
## Python
If you'd like to link this to more complex behavior (e.g. data logging,
text alerts), consider using a Python script.
```python
from tripplite import Battery
with Battery() as battery:
print(battery.get())
```
The `state` variable will contain an object with the same format as above. Use
`state['status']['ac present']` and `state['status']['shutdown imminent']` for
alerts, and consider logging voltage, frequency, and power.
If you are logging multiple batteries, you will need to handle each connection
separately.
```python
from tripplite import Battery, battery_paths
for path in battery_paths:
with Battery(path) as battery:
print(battery.get())
```
These paths are unfortunately non-deterministic and will change on each
iteration.
For long polling, you can improve stability by keeping connections open as long
as possible and reconnecting on error. For example:
```python
state = None
def read_batteries(check_period=5):
"""Read battery and reopen in error. Use for long polling."""
battery = Battery()
battery.open()
while True:
time.sleep(check_period)
try:
state = battery.get()
except OSError:
logging.exception(f"Could not read battery {battery}.")
battery.close()
battery.open()
```
An example for multiple batteries can be found in [numat/tripplite#6](https://github.com/numat/tripplite/pull/6#issuecomment-700152340).
## Note on Permissions
To read the TrippLite, you need access to the USB port. You have options:
* Run everything as root
* Add your user to the `dialout` group to access *all* serial ports
* Create a group restricted to accessing TrippLite USB devices through `udev`
For the last option, the rule looks like:
```console
echo 'SUBSYSTEM=="usb", ATTRS{idVendor}=="09ae", GROUP="tripplite"' > /etc/udev/rules.d/tripplite.rules
udevadm control --reload-rules
```
## Prometheus Exporter
This package offers an extra install to include a [Prometheus Exporter](https://prometheus.io/docs/instrumenting/exporters/)
which allows for data collection into a prometheus time series database. Esentially it's a small `HTTP` server that allows
Prometheus to *scrape* grabbing metrics at a configurable period.
### Install
```console
pip install tripplite[exporter]
```
* This adds the [prometheus_client](https://pypi.org/project/prometheus-client/) dependency.
You can then manually run the `triplite-exporter` cli or use the
[tripplite_exporter.service](https://github.com/numat/tripplite/blob/master/tripplite_exporter.service)
systemd unit file to have systemd run and supervise the process.
### Failure Mode
The script will try to close and reopen the USB serial connection to the device on an `OSError`. If an open fails,
the script will exit with the return code of 2.
Raw data
{
"_id": null,
"home_page": "https://github.com/numat/tripplite/",
"name": "tripplite",
"maintainer": "",
"docs_url": null,
"requires_python": "",
"maintainer_email": "",
"keywords": "",
"author": "Patrick Fuller",
"author_email": "pat@numat-tech.com",
"download_url": "https://files.pythonhosted.org/packages/24/74/02dcc11f51e22a2307821369e10952af6404cff38c34f897be70fcc75f7e/tripplite-0.4.0.tar.gz",
"platform": null,
"description": "# tripplite\n\nPython USB interface and command-line tool for TrippLite UPS battery backups.\n\n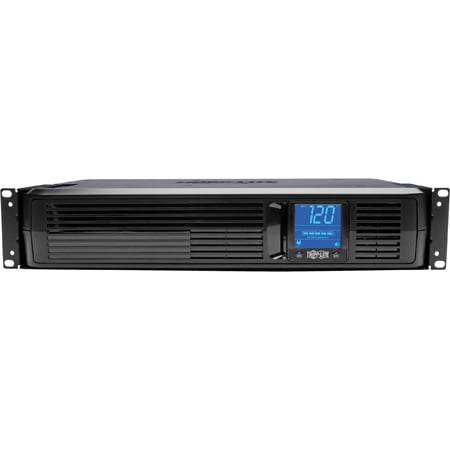\n\n## Background\n\nTrippLite offers [UI software](https://www.tripplite.com/products/power-alert)\nfor monitoring its batteries. However, most of its batteries don't have\nnetwork access, and the existing TrippLite software requires a local install.\n\nI wanted to monitor the UPS from a remote headless Linux server, so I wrote\nthis tool.\n\n## Supported Hardware\n\nThis has been exclusively tested on the TrippLite SMART1500LCD UPS. It will\nlikely work on similar firmware but there is a known communication issue with\nsome other TrippLite models (see [numat/tripplite#3](https://github.com/numat/tripplite/issues/3)).\n\nUse `lsusb` to check. `09ae:2012` should work, while `09ae:3016` may not.\n\n## Installation\n\n```console\napt install gcc libusb-1.0-0-dev libudev-dev\npip install tripplite\n```\n\nConnect a USB cable from the UPS to your headless server, and you should be\nready to run. If you don't want to run as root, see *Note on Permissions*\nbelow.\n\n# Command Line\n\n```\n$ tripplite\n{\n \"config\": {\n \"frequency\": 60, # Hz\n \"power\": 1500, # VA\n \"voltage\": 120 # V\n },\n \"health\": 100, # %\n \"input\": {\n \"frequency\": 59.7, # Hz\n \"voltage\": 117.2 # V\n },\n \"output\": {\n \"power\": 324, # W\n \"voltage\": 117.2 # V\n },\n \"status\": {\n \"ac present\": true,\n \"below remaining capacity\": true,\n \"charging\": false,\n \"discharging\": false,\n \"fully charged\": true,\n \"fully discharged\": false,\n \"needs replacement\": false,\n \"shutdown imminent\": false\n },\n \"time to empty\": 1004 # s\n}\n```\n\nTo use in shell scripts, parse the json output with something like\n[jq](https://stedolan.github.io/jq/). For example,\n`tripplite | jq '.status.\"ac present\"'` will return whether or not the unit\ndetects AC power.\n\n## Python\n\nIf you'd like to link this to more complex behavior (e.g. data logging,\ntext alerts), consider using a Python script.\n\n```python\nfrom tripplite import Battery\nwith Battery() as battery:\n print(battery.get())\n```\n\nThe `state` variable will contain an object with the same format as above. Use\n`state['status']['ac present']` and `state['status']['shutdown imminent']` for\nalerts, and consider logging voltage, frequency, and power.\n\nIf you are logging multiple batteries, you will need to handle each connection\nseparately.\n\n```python\nfrom tripplite import Battery, battery_paths\nfor path in battery_paths:\n with Battery(path) as battery:\n print(battery.get())\n```\n\nThese paths are unfortunately non-deterministic and will change on each\niteration.\n\nFor long polling, you can improve stability by keeping connections open as long\nas possible and reconnecting on error. For example:\n\n```python\nstate = None\n\ndef read_batteries(check_period=5):\n \"\"\"Read battery and reopen in error. Use for long polling.\"\"\"\n battery = Battery()\n battery.open()\n while True:\n time.sleep(check_period)\n try:\n state = battery.get()\n except OSError:\n logging.exception(f\"Could not read battery {battery}.\")\n battery.close()\n battery.open()\n```\n\nAn example for multiple batteries can be found in [numat/tripplite#6](https://github.com/numat/tripplite/pull/6#issuecomment-700152340).\n\n## Note on Permissions\n\nTo read the TrippLite, you need access to the USB port. You have options:\n\n* Run everything as root\n* Add your user to the `dialout` group to access *all* serial ports\n* Create a group restricted to accessing TrippLite USB devices through `udev`\n\nFor the last option, the rule looks like:\n\n```console\necho 'SUBSYSTEM==\"usb\", ATTRS{idVendor}==\"09ae\", GROUP=\"tripplite\"' > /etc/udev/rules.d/tripplite.rules\nudevadm control --reload-rules\n```\n\n## Prometheus Exporter\n\nThis package offers an extra install to include a [Prometheus Exporter](https://prometheus.io/docs/instrumenting/exporters/)\nwhich allows for data collection into a prometheus time series database. Esentially it's a small `HTTP` server that allows\nPrometheus to *scrape* grabbing metrics at a configurable period.\n\n### Install\n\n```console\npip install tripplite[exporter]\n```\n\n* This adds the [prometheus_client](https://pypi.org/project/prometheus-client/) dependency.\n\nYou can then manually run the `triplite-exporter` cli or use the\n[tripplite_exporter.service](https://github.com/numat/tripplite/blob/master/tripplite_exporter.service)\nsystemd unit file to have systemd run and supervise the process.\n\n### Failure Mode\n\nThe script will try to close and reopen the USB serial connection to the device on an `OSError`. If an open fails,\nthe script will exit with the return code of 2.\n",
"bugtrack_url": null,
"license": "GPLv2",
"summary": "Python driver for TrippLite UPS battery backups.",
"version": "0.4.0",
"project_urls": {
"Homepage": "https://github.com/numat/tripplite/"
},
"split_keywords": [],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "eed75a866ff735be26ed446bd2cd271cd05f33d8b7e6c8e7cf56df64df3948db",
"md5": "b3a6365334dfa0f66187a66962f9baaa",
"sha256": "6ec65375a3457d04d55b85f9e093d76ae51a99c9139b68d2ad88040f69b0457f"
},
"downloads": -1,
"filename": "tripplite-0.4.0-py3-none-any.whl",
"has_sig": false,
"md5_digest": "b3a6365334dfa0f66187a66962f9baaa",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": null,
"size": 15177,
"upload_time": "2023-07-09T01:08:00",
"upload_time_iso_8601": "2023-07-09T01:08:00.895478Z",
"url": "https://files.pythonhosted.org/packages/ee/d7/5a866ff735be26ed446bd2cd271cd05f33d8b7e6c8e7cf56df64df3948db/tripplite-0.4.0-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "247402dcc11f51e22a2307821369e10952af6404cff38c34f897be70fcc75f7e",
"md5": "49145842495101e65b35b99cd9113aa7",
"sha256": "556ed1a1572e3cbab7f763324d4947cebaff30824b8c4feda8797647f95f4524"
},
"downloads": -1,
"filename": "tripplite-0.4.0.tar.gz",
"has_sig": false,
"md5_digest": "49145842495101e65b35b99cd9113aa7",
"packagetype": "sdist",
"python_version": "source",
"requires_python": null,
"size": 15637,
"upload_time": "2023-07-09T01:08:02",
"upload_time_iso_8601": "2023-07-09T01:08:02.926998Z",
"url": "https://files.pythonhosted.org/packages/24/74/02dcc11f51e22a2307821369e10952af6404cff38c34f897be70fcc75f7e/tripplite-0.4.0.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-07-09 01:08:02",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "numat",
"github_project": "tripplite",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"lcname": "tripplite"
}