# truth-table-generator
**truth-table-generator** is a tool that allows to generate a truth table.
It is a fork of *truths* by [tr3buchet](https://github.com/tr3buchet/truths).
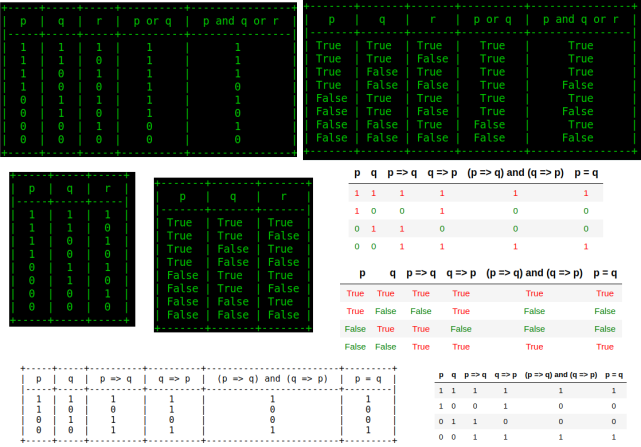
It merges some of the pull requests in the original and other external helpers.
The following are some of the changes and enhancements from the original:
- [tabulate](https://github.com/astanin/python-tabulate) instead of obsolete
[prettytable](https://code.google.com/archive/p/prettytable/) as main tool to
represent tabular data in ASCII tables (PrettyTable version is still available).
- so there are many table formats available as such LaTeX, Org Tables, HTML
and all others cited on [tabulate docs](https://github.com/astanin/python-tabulate)
- the table is now a Pandas DataFrame so you can make the output more visually
appealing with [Pandas Styling](https://pandas.pydata.org/pandas-docs/stable/user_guide/style.html).
See examples below.
- new function `valuation` that eval a proposition as a tautology, contradiction
or contingency.
- new command line interface (CLI) for printing a truth table from terminal.
## Installation
`pip install truth-table-generator`
## Usage
### Importing and syntax
First, let's import the package. `ttg` stands for *truth-table-generator*.
```python
import ttg
```
A truth table has one column for each input variable (for example, *p* and *q*),
and one final column showing all of the possible results of the logical
operation that the table represents. If the input has only one list of strings,
each string is considered an input variable:
```python
print(ttg.Truths(['p', 'q', 'r']))
```
```
+-----+-----+-----+
| p | q | r |
|-----+-----+-----|
| 1 | 1 | 1 |
| 1 | 1 | 0 |
| 1 | 0 | 1 |
| 1 | 0 | 0 |
| 0 | 1 | 1 |
| 0 | 1 | 0 |
| 0 | 0 | 1 |
| 0 | 0 | 0 |
+-----+-----+-----+
```
A second list of strings can be passed with propositional expressions created
with logical operators.
```python
print(ttg.Truths(['p', 'q', 'r'], ['p and q and r', 'p or q or r', '(p or (~q)) => r']))
```
```
+-----+-----+-----+-----------------+---------------+--------------------+
| p | q | r | p and q and r | p or q or r | (p or (~q)) => r |
|-----+-----+-----+-----------------+---------------+--------------------|
| 1 | 1 | 1 | 1 | 1 | 1 |
| 1 | 1 | 0 | 0 | 1 | 0 |
| 1 | 0 | 1 | 0 | 1 | 1 |
| 1 | 0 | 0 | 0 | 1 | 0 |
| 0 | 1 | 1 | 0 | 1 | 1 |
| 0 | 1 | 0 | 0 | 1 | 1 |
| 0 | 0 | 1 | 0 | 1 | 1 |
| 0 | 0 | 0 | 0 | 0 | 0 |
+-----+-----+-----+-----------------+---------------+--------------------+
```
### Operators and their representations:
- *negation*: `'not'`, `'-'`, `'~'`
- *logical disjunction*: `'or'`
- *logical nor*: `'nor'`
- *exclusive disjunction*: `'xor'`, `'!='`
- *logical conjunction*: `'and'`
- *logical NAND*: `'nand'`
- *material implication*: `'=>'`, `'implies'`
- *logical biconditional*: `'='`
**Note**: Use parentheses! Especially with the negation operator. Use tables
above and below as reference. Although precedence rules are used, sometimes
precedence between conjunction and disjunction is unspecified requiring to
provide it explicitly in given formula with parentheses.
### Showing words (True / False)
If you prefer the words True and False instead of numbers 0 and 1, there is a
third parameter, boolean type, `ints` that can be set to `False`:
```python
print(ttg.Truths(['p', 'q'], ['p and q', 'p or q', '(p or (~q)) => (~p)'], ints=False))
```
```
+-------+-------+-----------+----------+-----------------------+
| p | q | p and q | p or q | (p or (~q)) => (~p) |
|-------+-------+-----------+----------+-----------------------|
| True | True | True | True | False |
| True | False | False | True | False |
| False | True | False | True | True |
| False | False | False | False | True |
+-------+-------+-----------+----------+-----------------------+
```
### Reverse output option
If you prefer to have propositions in ascending order (0/False before 1/True),
there is the `ascending` parameter that can be set to `True`. Let's change
the above example to ascending order:
```python
print(ttg.Truths(['p', 'q'], ['p and q', 'p or q', '(p or (~q)) => (~p)'], ints=False, ascending=True))
```
```
+-------+-------+-----------+----------+-----------------------+
| p | q | p and q | p or q | (p or (~q)) => (~p) |
|-------+-------+-----------+----------+-----------------------|
| False | False | False | False | True |
| False | True | False | True | True |
| True | False | False | True | False |
| True | True | True | True | False |
+-------+-------+-----------+----------+-----------------------+
```
### Formatting options with PrettyTable and Tabulate
For more formatting options, let's create a truth table variable:
```python
table = ttg.Truths(['p', 'q'], ['p => q', 'p = q'])
```
The command `print(table)` renders the standard table as seen on above examples:
```
+-----+-----+----------+---------+
| p | q | p => q | p = q |
|-----+-----+----------+---------|
| 1 | 1 | 1 | 1 |
| 1 | 0 | 0 | 0 |
| 0 | 1 | 1 | 0 |
| 0 | 0 | 1 | 1 |
+-----+-----+----------+---------+
```
The command `print(table.as_prettytable())` renders the table with PrettyTable
package as on the original version of this package:
```
+---+---+--------+-------+
| p | q | p => q | p = q |
+---+---+--------+-------+
| 1 | 1 | 1 | 1 |
| 1 | 0 | 0 | 0 |
| 0 | 1 | 1 | 0 |
| 0 | 0 | 1 | 1 |
+---+---+--------+-------+
```
As can be seen, the PrettyTable output has less blank spaces. However, the
PrettyTable package has much less output options and it is deprecated. So I
decided to use the Tabulate package as standard.
The command `print(table.as_tabulate())` renders the table with Tabulate
package. The first column presents line numbers (that can be disabled with
the parameter `index=False`):
```
+----+-----+-----+----------+---------+
| | p | q | p => q | p = q |
|----+-----+-----+----------+---------|
| 1 | 1 | 1 | 1 | 1 |
| 2 | 1 | 0 | 0 | 0 |
| 3 | 0 | 1 | 1 | 0 |
| 4 | 0 | 0 | 1 | 1 |
+----+-----+-----+----------+---------+
```
Using Tabulate, we can use any of the formats available. Let's output a LaTeX
table without the line number column:
```python
print(table.as_tabulate(index=False, table_format='latex'))
```
```
\begin{tabular}{cccc}
\hline
p & q & p =\ensuremath{>} q & p = q \\
\hline
1 & 1 & 1 & 1 \\
1 & 0 & 0 & 0 \\
0 & 1 & 1 & 0 \\
0 & 0 & 1 & 1 \\
\hline
\end{tabular}
```
### Formatting options with Pandas
With an IPython terminal or a Jupyter Notebook, it is possible to render a Pandas
DataFrame with `table.as_pandas`:
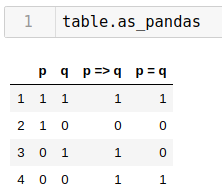
And this output can be modified with Pandas Styling.
More advanced modifications can be done with functions that apply styling changes.
See the [styles tutorial notebook](styling_tutorial.ipynb) for examples.
See the image below for a fancy example with two lines and two columns
highlighted with yellow background and different colors for True and False.
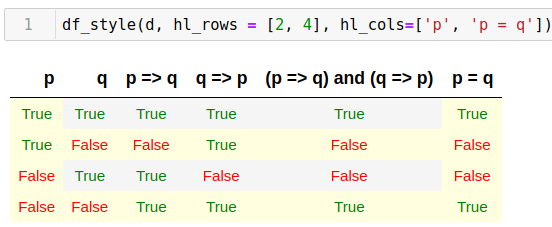
### The `valuation` function
Let's see the how to use the `valuation` function with a new truth table:
```python
table_val = ttg.Truths(['p', 'q'], ['p = q', 'p and (~p)', '(p and q) => p'])
print(table_val)
```
```
+-----+-----+---------+--------------+------------------+
| p | q | p = q | p and (~p) | (p and q) => p |
|-----+-----+---------+--------------+------------------|
| 1 | 1 | 1 | 0 | 1 |
| 1 | 0 | 0 | 0 | 1 |
| 0 | 1 | 0 | 0 | 1 |
| 0 | 0 | 1 | 0 | 1 |
+-----+-----+---------+--------------+------------------+
```
Without arguments, the `valuation` function classifies the *last column* as a
tautology, a contradiction or a contingency:
```python
table_val.valuation()
```
```
'Tautology'
```
If an integer is used as argument, the function classifies the correspondent
column:
```python
table_val.valuation(3)
```
```
'Contingency'
```
```python
table_val.valuation(4)
```
```
'Contradiction'
```
### CLI utility
For those who work in the terminal there is a simple command line interface
(CLI) for printing tables. The script name is `ttg_cly.py` and it accepts
the following syntax according to its `--help`:
```
usage: ttg_cli.py [-h] [-p PROPOSITIONS] [-i INTS] [-a ASCENDING] variables
positional arguments:
variables List of variables e. g. "['p', 'q']"
optional arguments:
-h, --help show this help message and exit
-p PROPOSITIONS, --propositions PROPOSITIONS
List of propositions e. g. "['p or q', 'p and q']"
-i INTS, --ints INTS True for 0 and 1; False for words
-a ASCENDING, --ascending ASCENDING
True for reverse output (False before True)
```
As seen, the list of variables is mandatory. Note that the lists must be between
`"`.
```bash
$ ttg_cli.py "['p', 'q', 'r']"
```
```
+-----+-----+-----+
| p | q | r |
|-----+-----+-----|
| 1 | 1 | 1 |
| 1 | 1 | 0 |
| 1 | 0 | 1 |
| 1 | 0 | 0 |
| 0 | 1 | 1 |
| 0 | 1 | 0 |
| 0 | 0 | 1 |
| 0 | 0 | 0 |
+-----+-----+-----+
```
The CLI utility also has an option, `-i`, to show words instead of numbers:
```bash
$ ttg_cli.py "['p', 'q', 'r']" -i False
```
```
+-------+-------+-------+
| p | q | r |
|-------+-------+-------|
| True | True | True |
| True | True | False |
| True | False | True |
| True | False | False |
| False | True | True |
| False | True | False |
| False | False | True |
| False | False | False |
+-------+-------+-------+
```
A `-p` parameter must be before the propositions list:
```bash
$ ttg_cli.py "['p', 'q', 'r']" -p "['p or q', 'p and q or r']"
```
```
+-----+-----+-----+----------+----------------+
| p | q | r | p or q | p and q or r |
|-----+-----+-----+----------+----------------|
| 1 | 1 | 1 | 1 | 1 |
| 1 | 1 | 0 | 1 | 1 |
| 1 | 0 | 1 | 1 | 1 |
| 1 | 0 | 0 | 1 | 0 |
| 0 | 1 | 1 | 1 | 1 |
| 0 | 1 | 0 | 1 | 0 |
| 0 | 0 | 1 | 0 | 1 |
| 0 | 0 | 0 | 0 | 0 |
+-----+-----+-----+----------+----------------+
```
With words instead of numbers:
```bash
$ ttg_cli.py "['p', 'q', 'r']" -p "['p or q', 'p and q or r']" -i False
```
```
+-------+-------+-------+----------+----------------+
| p | q | r | p or q | p and q or r |
|-------+-------+-------+----------+----------------|
| True | True | True | True | True |
| True | True | False | True | True |
| True | False | True | True | True |
| True | False | False | True | False |
| False | True | True | True | True |
| False | True | False | True | False |
| False | False | True | False | True |
| False | False | False | False | False |
+-------+-------+-------+----------+----------------+
```
In ascending order (0/False before 1/True):
```bash
$ ttg_cli.py "['p', 'q', 'r']" -p "['p or q', 'p and q or r']" -i False -a True
```
```
+-------+-------+-------+----------+----------------+
| p | q | r | p or q | p and q or r |
|-------+-------+-------+----------+----------------|
| False | False | False | False | False |
| False | False | True | False | True |
| False | True | False | True | False |
| False | True | True | True | True |
| True | False | False | True | False |
| True | False | True | True | True |
| True | True | False | True | True |
| True | True | True | True | True |
+-------+-------+-------+----------+----------------+
```
The real look of the table depends on your terminal appearance configuration.
The green on black background screenshots from the first picture of this README
are from my terminal.
## Contributing
All contributions are welcome.
**Issues**
Feel free to submit issues regarding:
- recommendations
- more examples for the tutorial
- enhancement requests and new useful features
- code bugs
**Pull requests**
- before starting to work on your pull request, please submit an issue first
- fork the repo
- clone the project to your own machine
- commit changes to your own branch
- push your work back up to your fork
- submit a pull request so that your changes can be reviewed
## License
Apache 2.0, see [LICENSE](LICENSE)
## Citing
If you use *truth-table-generator* in a scientific publication or in classes,
please consider citing as
F. L. S. Bustamante, *truth-table-generator* - generating truth tables., 2019 -
Available at: https://github.com/chicolucio/truth-table-generator
## Funding
If you enjoy this project and would like to see many more math and science
related programming projects, I would greatly appreciate any assistance. Send me
an e-mail to know how to assist. Many more projects are to come and your support
will be rewarded with more STEM coding projects :-)
Raw data
{
"_id": null,
"home_page": "https://github.com/chicolucio/truth-table-generator",
"name": "truth-table-generator",
"maintainer": "",
"docs_url": null,
"requires_python": ">=3.10",
"maintainer_email": "",
"keywords": "truth,table,truth table,truthtable,generator,logic,tautology,ttg",
"author": "Francisco Bustamante",
"author_email": "chicolucio@gmail.com",
"download_url": "https://files.pythonhosted.org/packages/c5/78/2caa93c3d97439c85c27196107618daae6ef5e0806cc1d499fc1a63db721/truth_table_generator-2.0.0.tar.gz",
"platform": null,
"description": "# truth-table-generator\n\n**truth-table-generator** is a tool that allows to generate a truth table.\nIt is a fork of *truths* by [tr3buchet](https://github.com/tr3buchet/truths).\n\n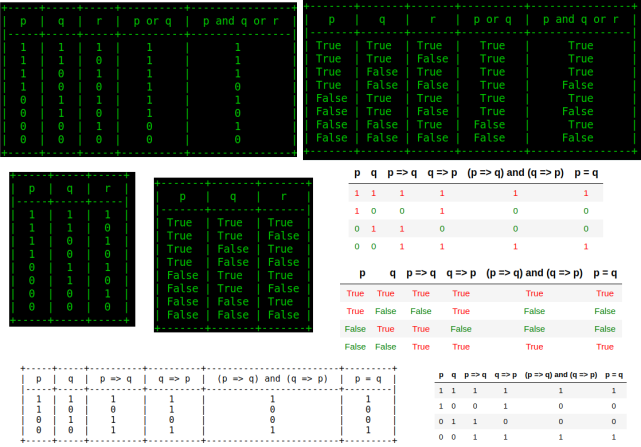\n\nIt merges some of the pull requests in the original and other external helpers.\nThe following are some of the changes and enhancements from the original:\n\n- [tabulate](https://github.com/astanin/python-tabulate) instead of obsolete\n[prettytable](https://code.google.com/archive/p/prettytable/) as main tool to\nrepresent tabular data in ASCII tables (PrettyTable version is still available).\n - so there are many table formats available as such LaTeX, Org Tables, HTML\n and all others cited on [tabulate docs](https://github.com/astanin/python-tabulate)\n- the table is now a Pandas DataFrame so you can make the output more visually\nappealing with [Pandas Styling](https://pandas.pydata.org/pandas-docs/stable/user_guide/style.html).\nSee examples below.\n- new function `valuation` that eval a proposition as a tautology, contradiction\nor contingency.\n- new command line interface (CLI) for printing a truth table from terminal.\n\n## Installation\n\n`pip install truth-table-generator`\n\n\n## Usage\n\n### Importing and syntax\n\nFirst, let's import the package. `ttg` stands for *truth-table-generator*.\n\n```python\nimport ttg\n```\n\nA truth table has one column for each input variable (for example, *p* and *q*),\nand one final column showing all of the possible results of the logical\noperation that the table represents. If the input has only one list of strings,\neach string is considered an input variable:\n\n```python\nprint(ttg.Truths(['p', 'q', 'r']))\n```\n```\n+-----+-----+-----+\n| p | q | r |\n|-----+-----+-----|\n| 1 | 1 | 1 |\n| 1 | 1 | 0 |\n| 1 | 0 | 1 |\n| 1 | 0 | 0 |\n| 0 | 1 | 1 |\n| 0 | 1 | 0 |\n| 0 | 0 | 1 |\n| 0 | 0 | 0 |\n+-----+-----+-----+\n```\n\nA second list of strings can be passed with propositional expressions created\nwith logical operators.\n\n```python\nprint(ttg.Truths(['p', 'q', 'r'], ['p and q and r', 'p or q or r', '(p or (~q)) => r']))\n```\n```\n+-----+-----+-----+-----------------+---------------+--------------------+\n| p | q | r | p and q and r | p or q or r | (p or (~q)) => r |\n|-----+-----+-----+-----------------+---------------+--------------------|\n| 1 | 1 | 1 | 1 | 1 | 1 |\n| 1 | 1 | 0 | 0 | 1 | 0 |\n| 1 | 0 | 1 | 0 | 1 | 1 |\n| 1 | 0 | 0 | 0 | 1 | 0 |\n| 0 | 1 | 1 | 0 | 1 | 1 |\n| 0 | 1 | 0 | 0 | 1 | 1 |\n| 0 | 0 | 1 | 0 | 1 | 1 |\n| 0 | 0 | 0 | 0 | 0 | 0 |\n+-----+-----+-----+-----------------+---------------+--------------------+\n```\n\n### Operators and their representations:\n\n- *negation*: `'not'`, `'-'`, `'~'`\n- *logical disjunction*: `'or'`\n- *logical nor*: `'nor'`\n- *exclusive disjunction*: `'xor'`, `'!='`\n- *logical conjunction*: `'and'`\n- *logical NAND*: `'nand'`\n- *material implication*: `'=>'`, `'implies'`\n- *logical biconditional*: `'='`\n\n**Note**: Use parentheses! Especially with the negation operator. Use tables\nabove and below as reference. Although precedence rules are used, sometimes\nprecedence between conjunction and disjunction is unspecified requiring to\n provide it explicitly in given formula with parentheses.\n\n### Showing words (True / False)\n\nIf you prefer the words True and False instead of numbers 0 and 1, there is a\nthird parameter, boolean type, `ints` that can be set to `False`:\n\n```python\nprint(ttg.Truths(['p', 'q'], ['p and q', 'p or q', '(p or (~q)) => (~p)'], ints=False))\n```\n```\n+-------+-------+-----------+----------+-----------------------+\n| p | q | p and q | p or q | (p or (~q)) => (~p) |\n|-------+-------+-----------+----------+-----------------------|\n| True | True | True | True | False |\n| True | False | False | True | False |\n| False | True | False | True | True |\n| False | False | False | False | True |\n+-------+-------+-----------+----------+-----------------------+\n```\n\n### Reverse output option\n\nIf you prefer to have propositions in ascending order (0/False before 1/True),\nthere is the `ascending` parameter that can be set to `True`. Let's change\nthe above example to ascending order:\n\n```python\nprint(ttg.Truths(['p', 'q'], ['p and q', 'p or q', '(p or (~q)) => (~p)'], ints=False, ascending=True))\n```\n```\n+-------+-------+-----------+----------+-----------------------+\n| p | q | p and q | p or q | (p or (~q)) => (~p) |\n|-------+-------+-----------+----------+-----------------------|\n| False | False | False | False | True |\n| False | True | False | True | True |\n| True | False | False | True | False |\n| True | True | True | True | False |\n+-------+-------+-----------+----------+-----------------------+\n```\n\n### Formatting options with PrettyTable and Tabulate\n\nFor more formatting options, let's create a truth table variable:\n```python\ntable = ttg.Truths(['p', 'q'], ['p => q', 'p = q'])\n```\nThe command `print(table)` renders the standard table as seen on above examples:\n```\n+-----+-----+----------+---------+\n| p | q | p => q | p = q |\n|-----+-----+----------+---------|\n| 1 | 1 | 1 | 1 |\n| 1 | 0 | 0 | 0 |\n| 0 | 1 | 1 | 0 |\n| 0 | 0 | 1 | 1 |\n+-----+-----+----------+---------+\n```\nThe command `print(table.as_prettytable())` renders the table with PrettyTable\npackage as on the original version of this package:\n```\n+---+---+--------+-------+\n| p | q | p => q | p = q |\n+---+---+--------+-------+\n| 1 | 1 | 1 | 1 |\n| 1 | 0 | 0 | 0 |\n| 0 | 1 | 1 | 0 |\n| 0 | 0 | 1 | 1 |\n+---+---+--------+-------+\n```\nAs can be seen, the PrettyTable output has less blank spaces. However, the\nPrettyTable package has much less output options and it is deprecated. So I\ndecided to use the Tabulate package as standard.\n\nThe command `print(table.as_tabulate())` renders the table with Tabulate\npackage. The first column presents line numbers (that can be disabled with\nthe parameter `index=False`):\n```\n+----+-----+-----+----------+---------+\n| | p | q | p => q | p = q |\n|----+-----+-----+----------+---------|\n| 1 | 1 | 1 | 1 | 1 |\n| 2 | 1 | 0 | 0 | 0 |\n| 3 | 0 | 1 | 1 | 0 |\n| 4 | 0 | 0 | 1 | 1 |\n+----+-----+-----+----------+---------+\n```\n\nUsing Tabulate, we can use any of the formats available. Let's output a LaTeX\ntable without the line number column:\n\n```python\nprint(table.as_tabulate(index=False, table_format='latex'))\n```\n```\n\\begin{tabular}{cccc}\n\\hline\n p & q & p =\\ensuremath{>} q & p = q \\\\\n\\hline\n 1 & 1 & 1 & 1 \\\\\n 1 & 0 & 0 & 0 \\\\\n 0 & 1 & 1 & 0 \\\\\n 0 & 0 & 1 & 1 \\\\\n\\hline\n\\end{tabular}\n```\n\n### Formatting options with Pandas\n\nWith an IPython terminal or a Jupyter Notebook, it is possible to render a Pandas\nDataFrame with `table.as_pandas`:\n\n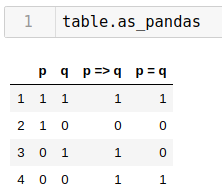\n\nAnd this output can be modified with Pandas Styling.\nMore advanced modifications can be done with functions that apply styling changes.\nSee the [styles tutorial notebook](styling_tutorial.ipynb) for examples.\nSee the image below for a fancy example with two lines and two columns\nhighlighted with yellow background and different colors for True and False.\n\n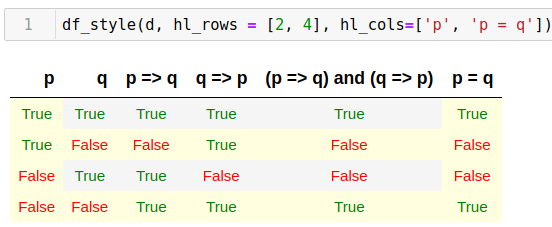\n\n### The `valuation` function\n\nLet's see the how to use the `valuation` function with a new truth table:\n```python\ntable_val = ttg.Truths(['p', 'q'], ['p = q', 'p and (~p)', '(p and q) => p'])\nprint(table_val)\n```\n```\n+-----+-----+---------+--------------+------------------+\n| p | q | p = q | p and (~p) | (p and q) => p |\n|-----+-----+---------+--------------+------------------|\n| 1 | 1 | 1 | 0 | 1 |\n| 1 | 0 | 0 | 0 | 1 |\n| 0 | 1 | 0 | 0 | 1 |\n| 0 | 0 | 1 | 0 | 1 |\n+-----+-----+---------+--------------+------------------+\n```\nWithout arguments, the `valuation` function classifies the *last column* as a\ntautology, a contradiction or a contingency:\n```python\ntable_val.valuation()\n```\n```\n'Tautology'\n```\nIf an integer is used as argument, the function classifies the correspondent\ncolumn:\n```python\ntable_val.valuation(3)\n```\n```\n'Contingency'\n```\n```python\ntable_val.valuation(4)\n```\n```\n'Contradiction'\n```\n\n### CLI utility\n\nFor those who work in the terminal there is a simple command line interface\n(CLI) for printing tables. The script name is `ttg_cly.py` and it accepts\nthe following syntax according to its `--help`:\n\n```\nusage: ttg_cli.py [-h] [-p PROPOSITIONS] [-i INTS] [-a ASCENDING] variables\n\npositional arguments:\n variables List of variables e. g. \"['p', 'q']\"\n\noptional arguments:\n -h, --help show this help message and exit\n -p PROPOSITIONS, --propositions PROPOSITIONS\n List of propositions e. g. \"['p or q', 'p and q']\"\n -i INTS, --ints INTS True for 0 and 1; False for words\n -a ASCENDING, --ascending ASCENDING\n True for reverse output (False before True)\n```\n\nAs seen, the list of variables is mandatory. Note that the lists must be between\n`\"`.\n\n```bash\n$ ttg_cli.py \"['p', 'q', 'r']\"\n```\n```\n+-----+-----+-----+\n| p | q | r |\n|-----+-----+-----|\n| 1 | 1 | 1 |\n| 1 | 1 | 0 |\n| 1 | 0 | 1 |\n| 1 | 0 | 0 |\n| 0 | 1 | 1 |\n| 0 | 1 | 0 |\n| 0 | 0 | 1 |\n| 0 | 0 | 0 |\n+-----+-----+-----+\n```\n\nThe CLI utility also has an option, `-i`, to show words instead of numbers:\n```bash\n$ ttg_cli.py \"['p', 'q', 'r']\" -i False\n```\n```\n+-------+-------+-------+\n| p | q | r |\n|-------+-------+-------|\n| True | True | True |\n| True | True | False |\n| True | False | True |\n| True | False | False |\n| False | True | True |\n| False | True | False |\n| False | False | True |\n| False | False | False |\n+-------+-------+-------+\n```\n\nA `-p` parameter must be before the propositions list:\n```bash\n$ ttg_cli.py \"['p', 'q', 'r']\" -p \"['p or q', 'p and q or r']\"\n```\n```\n+-----+-----+-----+----------+----------------+\n| p | q | r | p or q | p and q or r |\n|-----+-----+-----+----------+----------------|\n| 1 | 1 | 1 | 1 | 1 |\n| 1 | 1 | 0 | 1 | 1 |\n| 1 | 0 | 1 | 1 | 1 |\n| 1 | 0 | 0 | 1 | 0 |\n| 0 | 1 | 1 | 1 | 1 |\n| 0 | 1 | 0 | 1 | 0 |\n| 0 | 0 | 1 | 0 | 1 |\n| 0 | 0 | 0 | 0 | 0 |\n+-----+-----+-----+----------+----------------+\n```\n\nWith words instead of numbers:\n\n```bash\n$ ttg_cli.py \"['p', 'q', 'r']\" -p \"['p or q', 'p and q or r']\" -i False\n```\n```\n+-------+-------+-------+----------+----------------+\n| p | q | r | p or q | p and q or r |\n|-------+-------+-------+----------+----------------|\n| True | True | True | True | True |\n| True | True | False | True | True |\n| True | False | True | True | True |\n| True | False | False | True | False |\n| False | True | True | True | True |\n| False | True | False | True | False |\n| False | False | True | False | True |\n| False | False | False | False | False |\n+-------+-------+-------+----------+----------------+\n```\n\nIn ascending order (0/False before 1/True):\n\n```bash\n$ ttg_cli.py \"['p', 'q', 'r']\" -p \"['p or q', 'p and q or r']\" -i False -a True\n```\n```\n+-------+-------+-------+----------+----------------+\n| p | q | r | p or q | p and q or r |\n|-------+-------+-------+----------+----------------|\n| False | False | False | False | False |\n| False | False | True | False | True |\n| False | True | False | True | False |\n| False | True | True | True | True |\n| True | False | False | True | False |\n| True | False | True | True | True |\n| True | True | False | True | True |\n| True | True | True | True | True |\n+-------+-------+-------+----------+----------------+\n```\n\nThe real look of the table depends on your terminal appearance configuration.\nThe green on black background screenshots from the first picture of this README\nare from my terminal.\n\n## Contributing\n\nAll contributions are welcome.\n\n**Issues**\n\nFeel free to submit issues regarding:\n\n- recommendations\n- more examples for the tutorial\n- enhancement requests and new useful features\n- code bugs\n\n**Pull requests**\n\n- before starting to work on your pull request, please submit an issue first\n- fork the repo\n- clone the project to your own machine\n- commit changes to your own branch\n- push your work back up to your fork\n- submit a pull request so that your changes can be reviewed\n\n\n## License\n\nApache 2.0, see [LICENSE](LICENSE)\n\n## Citing\n\nIf you use *truth-table-generator* in a scientific publication or in classes,\nplease consider citing as\n\nF. L. S. Bustamante, *truth-table-generator* - generating truth tables., 2019 -\nAvailable at: https://github.com/chicolucio/truth-table-generator\n\n## Funding\n\nIf you enjoy this project and would like to see many more math and science\nrelated programming projects, I would greatly appreciate any assistance. Send me\nan e-mail to know how to assist. Many more projects are to come and your support\nwill be rewarded with more STEM coding projects :-)\n",
"bugtrack_url": null,
"license": "Apache Software License",
"summary": "Python API that auto generates truth tables",
"version": "2.0.0",
"project_urls": {
"Homepage": "https://github.com/chicolucio/truth-table-generator"
},
"split_keywords": [
"truth",
"table",
"truth table",
"truthtable",
"generator",
"logic",
"tautology",
"ttg"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "a83bb67f0b8aab0431711756535aa4ad6ec1955e61d2e641c459c93f23ef25c2",
"md5": "67b9ef07663505f43899d8279a781e6c",
"sha256": "83f4535bdcfdde208d7967bfd46e1ac9515822a17eeff8abfb77058d06d55036"
},
"downloads": -1,
"filename": "truth_table_generator-2.0.0-py3-none-any.whl",
"has_sig": false,
"md5_digest": "67b9ef07663505f43899d8279a781e6c",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.10",
"size": 19966,
"upload_time": "2023-11-08T22:50:52",
"upload_time_iso_8601": "2023-11-08T22:50:52.198436Z",
"url": "https://files.pythonhosted.org/packages/a8/3b/b67f0b8aab0431711756535aa4ad6ec1955e61d2e641c459c93f23ef25c2/truth_table_generator-2.0.0-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "c5782caa93c3d97439c85c27196107618daae6ef5e0806cc1d499fc1a63db721",
"md5": "0d34adef2983a27cf54495180d214876",
"sha256": "b4780e8424d1d06b1bedd39efb24836ff27cf51cc799406bfa833294e9220c85"
},
"downloads": -1,
"filename": "truth_table_generator-2.0.0.tar.gz",
"has_sig": false,
"md5_digest": "0d34adef2983a27cf54495180d214876",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.10",
"size": 18696,
"upload_time": "2023-11-08T22:50:54",
"upload_time_iso_8601": "2023-11-08T22:50:54.241302Z",
"url": "https://files.pythonhosted.org/packages/c5/78/2caa93c3d97439c85c27196107618daae6ef5e0806cc1d499fc1a63db721/truth_table_generator-2.0.0.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-11-08 22:50:54",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "chicolucio",
"github_project": "truth-table-generator",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"requirements": [],
"lcname": "truth-table-generator"
}