# Python Document Scanner for TWAIN, WIA, SANE, ICA, and eSCL
The package provides methods for calling [Dynamsoft Service REST APIs](https://www.dynamsoft.com/blog/announcement/dynamsoft-service-restful-api/). This allows developers to build Python applications for digitizing documents from **TWAIN (32-bit/64-bit)**, **WIA**, **SANE**, **ICA** and **eSCL** scanners.
## Prerequisites
1. Install Dynamsoft Service.
- Windows: [Dynamsoft-Service-Setup.msi](https://demo.dynamsoft.com/DWT/DWTResources/dist/DynamsoftServiceSetup.msi)
- macOS: [Dynamsoft-Service-Setup.pkg](https://demo.dynamsoft.com/DWT/DWTResources/dist/DynamsoftServiceSetup.pkg)
- Linux:
- [Dynamsoft-Service-Setup.deb](https://demo.dynamsoft.com/DWT/DWTResources/dist/DynamsoftServiceSetup.deb)
- [Dynamsoft-Service-Setup-arm64.deb](https://demo.dynamsoft.com/DWT/DWTResources/dist/DynamsoftServiceSetup-arm64.deb)
- [Dynamsoft-Service-Setup-mips64el.deb](https://demo.dynamsoft.com/DWT/DWTResources/dist/DynamsoftServiceSetup-mips64el.deb)
- [Dynamsoft-Service-Setup.rpm](https://demo.dynamsoft.com/DWT/DWTResources/dist/DynamsoftServiceSetup.rpm)
2. Request a [free trial license](https://www.dynamsoft.com/customer/license/trialLicense?product=dwt).
## Dynamsoft Service REST API
By default, the REST API's host address is set to `http://127.0.0.1:18622`.
| Method | Endpoint | Description | Parameters | Response |
|--------|-----------------|-------------------------------|------------------------------------|-------------------------------|
| GET | `/DWTAPI/Scanners` | Get a list of scanners | None | `200 OK` with scanner list |
| POST | `/DWTAPI/ScanJobs` | Creates a scan job | `license`, `device`, `config` | `201 Created` with job ID |
| GET | `/DWTAPI/ScanJobs/:id/NextDocument`| Retrieves a document image | `id`: Job ID | `200 OK` with image stream |
| DELETE | `/DWTAPI/ScanJobs/:id`| Deletes a scan job | `id`: Job ID | `200 OK` |
You can navigate to `http://127.0.0.1:18625/` to access the service. To make it accessible from desktop, mobile, and web applications on the same network, you can change the host address to a LAN IP address. For example, you might use `http://192.168.8.72`.
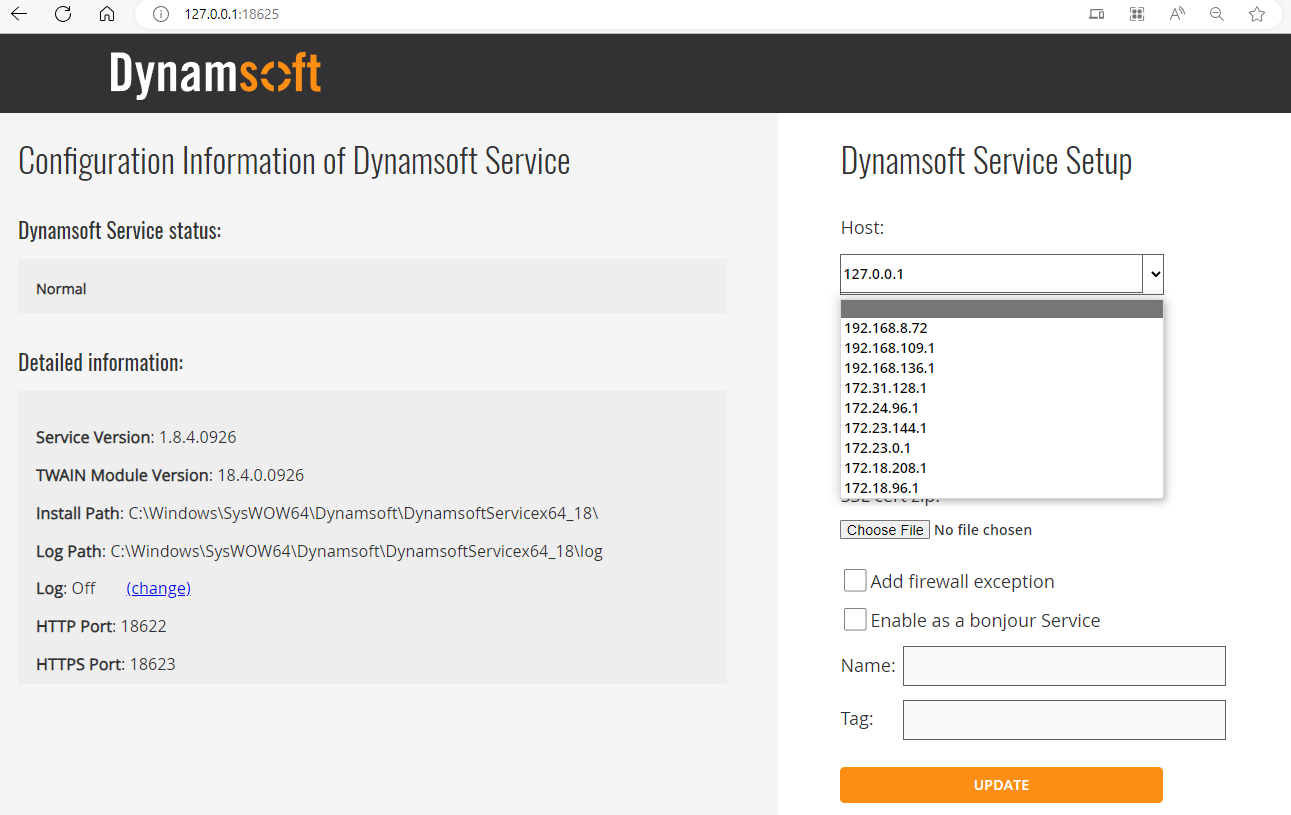
The scanner parameter configuration is based on [Dynamsoft Web TWAIN documentation](https://www.dynamsoft.com/web-twain/docs/info/api/Interfaces.html#DeviceConfiguration).
## Quick Start
Replace the license key in the code below with a valid one and run the code.
```python
from dynamsoftservice import ScannerController, ScannerType
scannerController = ScannerController()
devices = []
host = "http://127.0.0.1:18622"
license_key = "LICENSE-KEY"
questions = """
Please select an operation:
1. Get scanners
2. Acquire documents by scanner index
3. Quit
"""
def ask_question():
while True:
print(".............................................")
answer = input(questions)
if answer == '3':
break
elif answer == '1':
scanners = scannerController.getDevices(
host, ScannerType.TWAINSCANNER | ScannerType.TWAINX64SCANNER)
devices.clear()
for i, scanner in enumerate(scanners):
devices.append(scanner)
print(f"\nIndex: {i}, Name: {scanner['name']}")
elif answer == '2':
if len(devices) == 0:
print("Please get scanners first!\n")
continue
index = input(f"\nSelect an index (<= {len(devices) - 1}): ")
index = int(index)
if index < 0 or index >= len(devices):
print("It is out of range.")
continue
parameters = {
"license": license_key,
"device": devices[index]["device"],
}
parameters["config"] = {
"IfShowUI": False,
"PixelType": 2,
"Resolution": 200,
"IfFeederEnabled": False,
"IfDuplexEnabled": False,
}
job_id = scannerController.scanDocument(host, parameters)
if job_id != "":
images = scannerController.getImageFiles(host, job_id, "./")
for i, image in enumerate(images):
print(f"Image {i}: {image}")
scannerController.deleteJob(host, job_id)
else:
continue
if __name__ == "__main__":
ask_question()
```
## Example
- [Flet App](https://github.com/yushulx/twain-wia-sane-scanner/tree/main/example)

## DynamsoftService API
The `DynamsoftService` class provides methods to interact with the Dynamsoft service.
- `getDevices(self, host: str, scannerType: int = None) -> List[Any]`: Get a list of available devices.
- `scanDocument(self, host: str, parameters: Dict[str, Any]) -> str`: Scan a document.
- `deleteJob(self, host: str, jobId: str) -> None`: Delete a job.
- `getImageFile(self, host, job_id, directory)`: Get an image file.
- `getImageFiles(self, host: str, jobId: str, directory: str) -> List[str]`: Get a list of image files.
- `getImageStreams(self, host: str, jobId: str) -> List[bytes]`: Get a list of image streams.
## How to Build the Package
- Source distribution:
```bash
python setup.py sdist
```
- Wheel:
```bash
pip wheel . --verbose
# Or
python setup.py bdist_wheel
```
Raw data
{
"_id": null,
"home_page": "https://github.com/yushulx/twain-wia-sane-scanner",
"name": "twain-wia-sane-scanner",
"maintainer": "",
"docs_url": null,
"requires_python": "",
"maintainer_email": "",
"keywords": "",
"author": "yushulx",
"author_email": "",
"download_url": "https://files.pythonhosted.org/packages/b6/86/d445d6e350e0204ca3b8956084ae53b2e67b02ac8a0b2bb7255221604d9e/twain-wia-sane-scanner-1.0.0.tar.gz",
"platform": null,
"description": "# Python Document Scanner for TWAIN, WIA, SANE, ICA, and eSCL\nThe package provides methods for calling [Dynamsoft Service REST APIs](https://www.dynamsoft.com/blog/announcement/dynamsoft-service-restful-api/). This allows developers to build Python applications for digitizing documents from **TWAIN (32-bit/64-bit)**, **WIA**, **SANE**, **ICA** and **eSCL** scanners.\n\n## Prerequisites\n1. Install Dynamsoft Service.\n - Windows: [Dynamsoft-Service-Setup.msi](https://demo.dynamsoft.com/DWT/DWTResources/dist/DynamsoftServiceSetup.msi)\n - macOS: [Dynamsoft-Service-Setup.pkg](https://demo.dynamsoft.com/DWT/DWTResources/dist/DynamsoftServiceSetup.pkg)\n - Linux: \n - [Dynamsoft-Service-Setup.deb](https://demo.dynamsoft.com/DWT/DWTResources/dist/DynamsoftServiceSetup.deb)\n - [Dynamsoft-Service-Setup-arm64.deb](https://demo.dynamsoft.com/DWT/DWTResources/dist/DynamsoftServiceSetup-arm64.deb)\n - [Dynamsoft-Service-Setup-mips64el.deb](https://demo.dynamsoft.com/DWT/DWTResources/dist/DynamsoftServiceSetup-mips64el.deb)\n - [Dynamsoft-Service-Setup.rpm](https://demo.dynamsoft.com/DWT/DWTResources/dist/DynamsoftServiceSetup.rpm)\n \n2. Request a [free trial license](https://www.dynamsoft.com/customer/license/trialLicense?product=dwt).\n\n## Dynamsoft Service REST API\nBy default, the REST API's host address is set to `http://127.0.0.1:18622`. \n\n| Method | Endpoint | Description | Parameters | Response |\n|--------|-----------------|-------------------------------|------------------------------------|-------------------------------|\n| GET | `/DWTAPI/Scanners` | Get a list of scanners | None | `200 OK` with scanner list |\n| POST | `/DWTAPI/ScanJobs` | Creates a scan job | `license`, `device`, `config` | `201 Created` with job ID |\n| GET | `/DWTAPI/ScanJobs/:id/NextDocument`| Retrieves a document image | `id`: Job ID | `200 OK` with image stream |\n| DELETE | `/DWTAPI/ScanJobs/:id`| Deletes a scan job | `id`: Job ID | `200 OK` |\n\nYou can navigate to `http://127.0.0.1:18625/` to access the service. To make it accessible from desktop, mobile, and web applications on the same network, you can change the host address to a LAN IP address. For example, you might use `http://192.168.8.72`.\n\n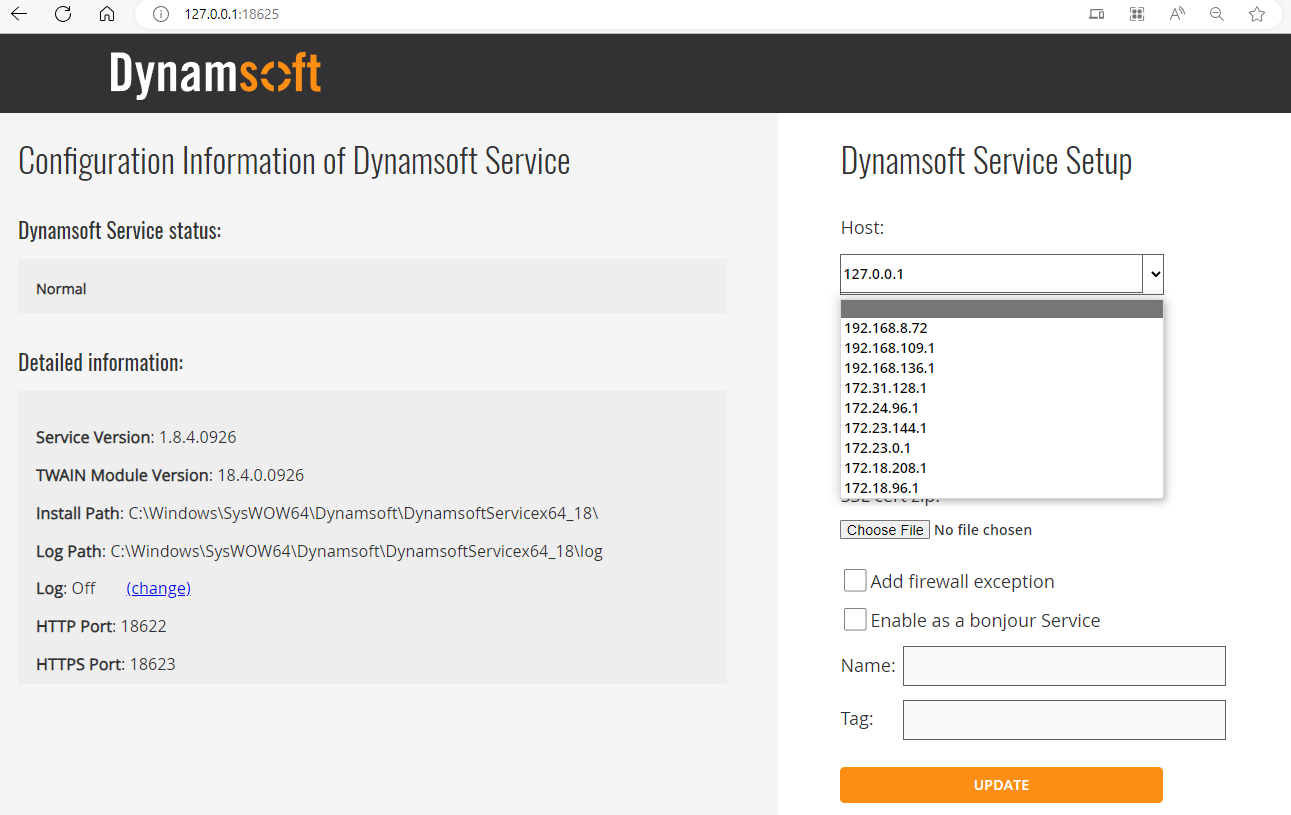\n\nThe scanner parameter configuration is based on [Dynamsoft Web TWAIN documentation](https://www.dynamsoft.com/web-twain/docs/info/api/Interfaces.html#DeviceConfiguration). \n\n## Quick Start\nReplace the license key in the code below with a valid one and run the code.\n\n```python\nfrom dynamsoftservice import ScannerController, ScannerType\n\nscannerController = ScannerController()\ndevices = []\nhost = \"http://127.0.0.1:18622\"\nlicense_key = \"LICENSE-KEY\"\n\nquestions = \"\"\"\nPlease select an operation:\n1. Get scanners\n2. Acquire documents by scanner index\n3. Quit\n\"\"\"\n\n\ndef ask_question():\n while True:\n print(\".............................................\")\n answer = input(questions)\n\n if answer == '3':\n break\n elif answer == '1':\n scanners = scannerController.getDevices(\n host, ScannerType.TWAINSCANNER | ScannerType.TWAINX64SCANNER)\n devices.clear()\n for i, scanner in enumerate(scanners):\n devices.append(scanner)\n print(f\"\\nIndex: {i}, Name: {scanner['name']}\")\n elif answer == '2':\n if len(devices) == 0:\n print(\"Please get scanners first!\\n\")\n continue\n\n index = input(f\"\\nSelect an index (<= {len(devices) - 1}): \")\n index = int(index) \n\n if index < 0 or index >= len(devices):\n print(\"It is out of range.\")\n continue\n\n parameters = {\n \"license\": license_key,\n \"device\": devices[index][\"device\"],\n }\n\n parameters[\"config\"] = {\n \"IfShowUI\": False,\n \"PixelType\": 2,\n \"Resolution\": 200,\n \"IfFeederEnabled\": False,\n \"IfDuplexEnabled\": False,\n }\n\n job_id = scannerController.scanDocument(host, parameters)\n\n if job_id != \"\":\n images = scannerController.getImageFiles(host, job_id, \"./\")\n for i, image in enumerate(images):\n print(f\"Image {i}: {image}\")\n\n scannerController.deleteJob(host, job_id)\n else:\n continue\n\n\nif __name__ == \"__main__\":\n ask_question()\n```\n\n## Example\n- [Flet App](https://github.com/yushulx/twain-wia-sane-scanner/tree/main/example)\n\n \n\n## DynamsoftService API\nThe `DynamsoftService` class provides methods to interact with the Dynamsoft service.\n\n- `getDevices(self, host: str, scannerType: int = None) -> List[Any]`: Get a list of available devices.\n- `scanDocument(self, host: str, parameters: Dict[str, Any]) -> str`: Scan a document.\n- `deleteJob(self, host: str, jobId: str) -> None`: Delete a job.\n- `getImageFile(self, host, job_id, directory)`: Get an image file.\n- `getImageFiles(self, host: str, jobId: str, directory: str) -> List[str]`: Get a list of image files.\n- `getImageStreams(self, host: str, jobId: str) -> List[bytes]`: Get a list of image streams.\n\n## How to Build the Package\n- Source distribution:\n \n ```bash\n python setup.py sdist\n ```\n\n- Wheel:\n \n ```bash\n pip wheel . --verbose\n # Or\n python setup.py bdist_wheel\n ```\n\n\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "A Python package for digitizing documents from TWAIN, WIA, SANE, ICA and eSCL compatible scanners.",
"version": "1.0.0",
"project_urls": {
"Homepage": "https://github.com/yushulx/twain-wia-sane-scanner"
},
"split_keywords": [],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "6fd9b3d6ca4fca2c2e29e5c621749ee918d42a3a3ba561d69b656ed81d421c70",
"md5": "14ab2192db0bd2d3af8ab0c569f03a8c",
"sha256": "e42d40d45709c289af132ea2c4ddd86d637c463c6e8e5649f8c4e951375c2afc"
},
"downloads": -1,
"filename": "twain_wia_sane_scanner-1.0.0-py3-none-any.whl",
"has_sig": false,
"md5_digest": "14ab2192db0bd2d3af8ab0c569f03a8c",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": null,
"size": 5893,
"upload_time": "2023-09-28T02:09:54",
"upload_time_iso_8601": "2023-09-28T02:09:54.003756Z",
"url": "https://files.pythonhosted.org/packages/6f/d9/b3d6ca4fca2c2e29e5c621749ee918d42a3a3ba561d69b656ed81d421c70/twain_wia_sane_scanner-1.0.0-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "b686d445d6e350e0204ca3b8956084ae53b2e67b02ac8a0b2bb7255221604d9e",
"md5": "0ea17f54c04b1b5cd10fa3fe59750a32",
"sha256": "64248859d776b228f2fec5e6d58c5cac82fc7712a61c6f56d5cf5e95f8bed3e7"
},
"downloads": -1,
"filename": "twain-wia-sane-scanner-1.0.0.tar.gz",
"has_sig": false,
"md5_digest": "0ea17f54c04b1b5cd10fa3fe59750a32",
"packagetype": "sdist",
"python_version": "source",
"requires_python": null,
"size": 5903,
"upload_time": "2023-09-28T02:09:55",
"upload_time_iso_8601": "2023-09-28T02:09:55.917488Z",
"url": "https://files.pythonhosted.org/packages/b6/86/d445d6e350e0204ca3b8956084ae53b2e67b02ac8a0b2bb7255221604d9e/twain-wia-sane-scanner-1.0.0.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-09-28 02:09:55",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "yushulx",
"github_project": "twain-wia-sane-scanner",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "twain-wia-sane-scanner"
}