Name | umami-analytics JSON |
Version |
0.2.15
JSON |
| download |
home_page | None |
Summary | Umami Analytics Client for Python |
upload_time | 2024-03-01 05:37:29 |
maintainer | None |
docs_url | None |
author | None |
requires_python | >=3.8 |
license | None |
keywords |
analytics
umami
website
|
VCS |
 |
bugtrack_url |
|
requirements |
No requirements were recorded.
|
Travis-CI |
No Travis.
|
coveralls test coverage |
No coveralls.
|
# Umami Analytics Client for Python
Client for privacy-preserving, open source [Umami analytics platform](https://umami.is) based on
`httpx` and `pydantic`.
`umami-analytics` is intended for adding custom data to your Umami instance (self-hosted or SaaS). Many umami events can supplied directly from HTML via their `data-*` attributes. However, some cannot. For example, if you have an event that is triggered in your app but doesn't have a clear HTML action you can add custom events. These will appear at the bottom of your Umami analtytics page for a website.
One example is a **purchase-course** event that happens deep inside the Python code rather than in HTML at [Talk Python Training](https://training.talkpython.fm). This is what our events section looks like for a typical weekend day (US Pacific Time):
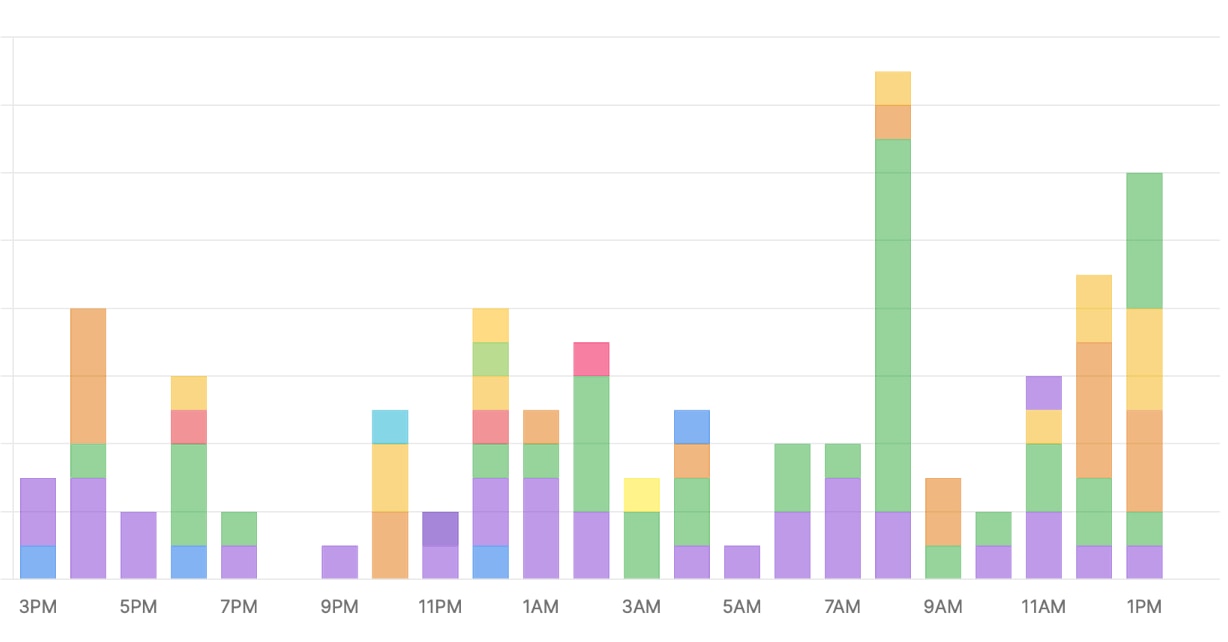
## Focused on what you need, not what is offered
The [Umami API is extensive](https://umami.is/docs/api) and much of that is intended for their frontend code to be able to function. You probably don't want or need that. `umami-analytics` only covers the subset that most developers will need for common SaaS actions such as adding [custom events](https://umami.is/docs/event-data). That said, PRs are weclome.
## Core Features
* ➕ **Add a custom event** to your Umami analytics dashboard.
* 🌐 List all websites with details that you have registered at Umami.
* 🔀 Both **sync** and **async** programming models.
* ⚒️ **Structured data with Pydantic** models for API responses.
* 👩💻 **Login / authenticate** for either a self-hosted or SaaS hosted instance of Umami.
* 🥇Set a **default website** for a **simplified API** going forward.
See the usage example below for the Python API around these features.
## Async or sync API? You choose
🔀 **Async is supported but not required** for your Python code. For functions that access the network, there is a `func()` and `func_async()` variant that works with Python's `async` and `await`.
## Installation
Just `pip install umami-analytics`
## Usage
```python
import umami
umami.set_url_base("https://umami.hostedbyyouorthem.com")
# Auth is NOT required to send events, but is for other features.
login = umami.login(username, password)
# Skip the need to pass the target website in subsequent calls.
umami.set_website_id('cc726914-8e68-4d1a-4be0-af4ca8933456')
umami.set_hostname('somedomain.com')
# List your websites
websites = umami.websites()
# Create a new event in the events section of the dashboards.
event_resp = umami.new_event(
website_id='a7cd-5d1a-2b33', # Only send if overriding default above
event_name='Umami-Test',
title='Umami-Test', # Defaults to event_name if omitted.
hostname='somedomain.com', # Only send if overriding default above.
url='/users/actions',
custom_data={'client': 'umami-tester-v1'},
referrer='https://some_url')
# Create a new page view in the pages section of the dashboards.
page_view_resp = umami.new_page_view(
website_id='a7cd-5d1a-2b33', # Only send if overriding default above
page_title='Umami-Test', # Defaults to event_name if omitted.
hostname='somedomain.com', # Only send if overriding default above.
url='/users/actions',
referrer='https://some_url')
# Call after logging in to make sure the auth token is still valid.
umami.verify_token()
```
This code listing is very-very high fidelity psuedo code. If you want an actually executable example, see the [example client](./umami/example_client) in the repo.
## Want to contribute?
See the [API documentation](https://umami.is/docs/api) for the remaining endpoints to be added. PRs are welcome. But please open an issue first to see if the proposed feature fits with the direction of this library.
Enjoy.
Raw data
{
"_id": null,
"home_page": null,
"name": "umami-analytics",
"maintainer": null,
"docs_url": null,
"requires_python": ">=3.8",
"maintainer_email": null,
"keywords": "analytics,umami,website",
"author": null,
"author_email": "Michael Kennedy <michael@talkpython.fm>",
"download_url": "https://files.pythonhosted.org/packages/40/0e/89bdfab417287e260521b41238c85df9634dcf6bb2e1c3104de1c7e8a3d0/umami_analytics-0.2.15.tar.gz",
"platform": null,
"description": "# Umami Analytics Client for Python\n\nClient for privacy-preserving, open source [Umami analytics platform](https://umami.is) based on \n`httpx` and `pydantic`. \n\n`umami-analytics` is intended for adding custom data to your Umami instance (self-hosted or SaaS). Many umami events can supplied directly from HTML via their `data-*` attributes. However, some cannot. For example, if you have an event that is triggered in your app but doesn't have a clear HTML action you can add custom events. These will appear at the bottom of your Umami analtytics page for a website.\n\nOne example is a **purchase-course** event that happens deep inside the Python code rather than in HTML at [Talk Python Training](https://training.talkpython.fm). This is what our events section looks like for a typical weekend day (US Pacific Time):\n\n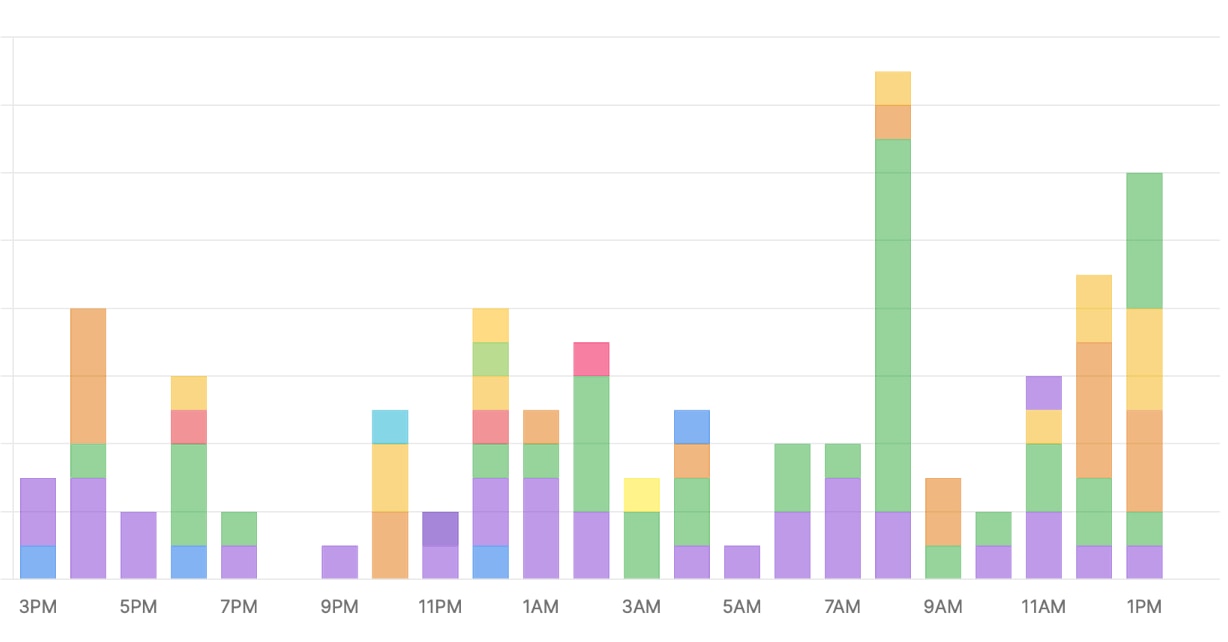\n\n## Focused on what you need, not what is offered\n\nThe [Umami API is extensive](https://umami.is/docs/api) and much of that is intended for their frontend code to be able to function. You probably don't want or need that. `umami-analytics` only covers the subset that most developers will need for common SaaS actions such as adding [custom events](https://umami.is/docs/event-data). That said, PRs are weclome.\n\n## Core Features\n\n* \u2795 **Add a custom event** to your Umami analytics dashboard.\n* \ud83c\udf10 List all websites with details that you have registered at Umami.\n* \ud83d\udd00 Both **sync** and **async** programming models.\n* \u2692\ufe0f **Structured data with Pydantic** models for API responses.\n* \ud83d\udc69\u200d\ud83d\udcbb **Login / authenticate** for either a self-hosted or SaaS hosted instance of Umami.\n* \ud83e\udd47Set a **default website** for a **simplified API** going forward.\n\nSee the usage example below for the Python API around these features.\n\n## Async or sync API? You choose\n\n\ud83d\udd00 **Async is supported but not required** for your Python code. For functions that access the network, there is a `func()` and `func_async()` variant that works with Python's `async` and `await`.\n\n## Installation\n\nJust `pip install umami-analytics`\n\n## Usage\n\n```python\n\nimport umami\n\numami.set_url_base(\"https://umami.hostedbyyouorthem.com\")\n\n# Auth is NOT required to send events, but is for other features.\nlogin = umami.login(username, password)\n\n# Skip the need to pass the target website in subsequent calls.\numami.set_website_id('cc726914-8e68-4d1a-4be0-af4ca8933456')\numami.set_hostname('somedomain.com')\n\n# List your websites\nwebsites = umami.websites()\n\n# Create a new event in the events section of the dashboards.\nevent_resp = umami.new_event(\n website_id='a7cd-5d1a-2b33', # Only send if overriding default above\n event_name='Umami-Test',\n title='Umami-Test', # Defaults to event_name if omitted.\n hostname='somedomain.com', # Only send if overriding default above.\n url='/users/actions',\n custom_data={'client': 'umami-tester-v1'},\n referrer='https://some_url')\n\n# Create a new page view in the pages section of the dashboards.\npage_view_resp = umami.new_page_view(\n website_id='a7cd-5d1a-2b33', # Only send if overriding default above\n page_title='Umami-Test', # Defaults to event_name if omitted.\n hostname='somedomain.com', # Only send if overriding default above.\n url='/users/actions',\n referrer='https://some_url')\n\n# Call after logging in to make sure the auth token is still valid.\numami.verify_token()\n```\n\nThis code listing is very-very high fidelity psuedo code. If you want an actually executable example, see the [example client](./umami/example_client) in the repo.\n\n## Want to contribute?\n\nSee the [API documentation](https://umami.is/docs/api) for the remaining endpoints to be added. PRs are welcome. But please open an issue first to see if the proposed feature fits with the direction of this library.\n\nEnjoy.\n",
"bugtrack_url": null,
"license": null,
"summary": "Umami Analytics Client for Python",
"version": "0.2.15",
"project_urls": {
"Homepage": "https://github.com/mikeckennedy/umami-python",
"Source": "https://github.com/mikeckennedy/umami-python",
"Tracker": "https://github.com/mikeckennedy/umami-python/issues"
},
"split_keywords": [
"analytics",
"umami",
"website"
],
"urls": [
{
"comment_text": null,
"digests": {
"blake2b_256": "b9d109bab36cb65de7e4d4eee732b35d3f92934ca912165d6941a38b3122ed14",
"md5": "8cac872410e6d739875077ee6fc0d36b",
"sha256": "2c2808a1d75aaa35e116e349a8f090790cebb6c8b8be3b7f2576cfbbf3bbe947"
},
"downloads": -1,
"filename": "umami_analytics-0.2.15-py3-none-any.whl",
"has_sig": false,
"md5_digest": "8cac872410e6d739875077ee6fc0d36b",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.8",
"size": 8869,
"upload_time": "2024-03-01T05:37:31",
"upload_time_iso_8601": "2024-03-01T05:37:31.281958Z",
"url": "https://files.pythonhosted.org/packages/b9/d1/09bab36cb65de7e4d4eee732b35d3f92934ca912165d6941a38b3122ed14/umami_analytics-0.2.15-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "400e89bdfab417287e260521b41238c85df9634dcf6bb2e1c3104de1c7e8a3d0",
"md5": "eb71f46324e7f045d7e9cfaeba218d0d",
"sha256": "523f357b46634b18c14061edc69f7116d01ae1f5c978521486480333b6e7a35c"
},
"downloads": -1,
"filename": "umami_analytics-0.2.15.tar.gz",
"has_sig": false,
"md5_digest": "eb71f46324e7f045d7e9cfaeba218d0d",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.8",
"size": 7608,
"upload_time": "2024-03-01T05:37:29",
"upload_time_iso_8601": "2024-03-01T05:37:29.508242Z",
"url": "https://files.pythonhosted.org/packages/40/0e/89bdfab417287e260521b41238c85df9634dcf6bb2e1c3104de1c7e8a3d0/umami_analytics-0.2.15.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-03-01 05:37:29",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "mikeckennedy",
"github_project": "umami-python",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"requirements": [],
"lcname": "umami-analytics"
}