# :page_facing_up: unitreport  
UnitReport is a small unittest-based tool for generating single page html reports in Python.
The reports can include matplotlib figures and html tables.
It is designed to be minimal and fit into the unittesting framework, allowing users to make assertions about their data (e.g. data quality) before generating figures.
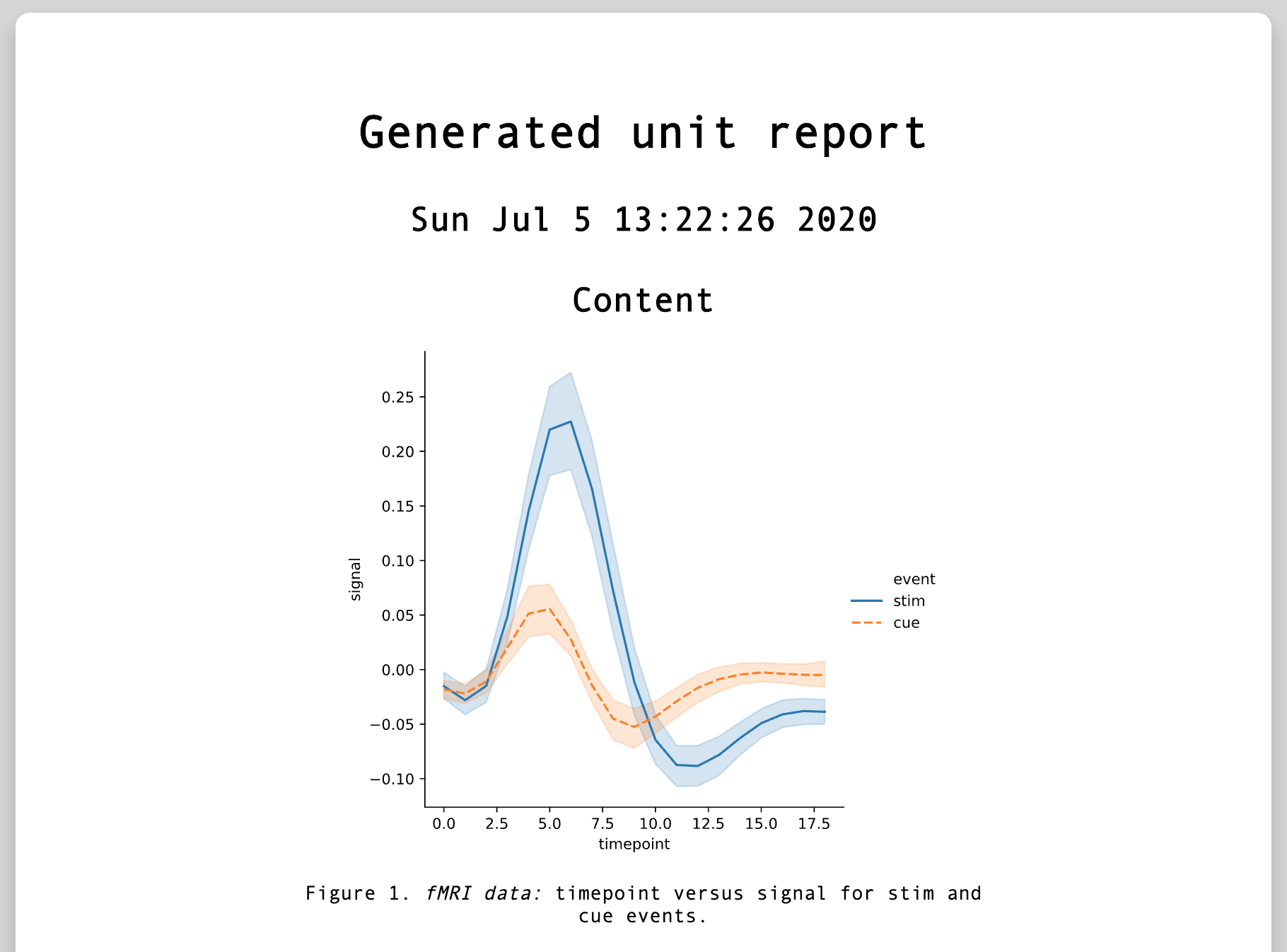
## Getting Started
You can install the library using,
`pip3 install unitreport`
There are usage examples in `test_plots.py` and `test_tables.py`.
You need to create test cases using the unittest Python library, and utilise unitreport's decorators:
```python
import unittest
import unitreport
import seaborn as sns
import pandas as pd
class TestExample(unittest.TestCase):
"""Example test suite producing plots and tables in a report using unitreport."""
dataset: pd.DataFrame = None
@classmethod
def setUpClass(cls):
"""Load dataset on setup."""
cls.dataset = sns.load_dataset("fmri")
@unitreport.plotting
def test_timepoint_vs_signal_by_event(self):
"""*fMRI data:* timepoint versus signal for stim and cue events."""
# you can still run assertions to check data quality before plotting
self.assertEqual(self.dataset.shape, (1064, 5))
# plotting decorator will call plt.savefig() to generate the plot
sns.relplot(
x="timepoint",
y="signal",
hue="event",
style="event",
kind="line",
data=self.dataset,
)
# could also return a figure & .savefig() will be called on returned object
@unitreport.tabling
def test_region_counts(self):
"""*fMRI data:* table summary description of timepoints and signals."""
# you can still run assertions to check data quality before making table
self.assertEqual(self.dataset.shape, (1064, 5))
return self.dataset.describe().to_html()
```
You can run the tests using,
`python3 -m unitreport`
This will discover and run the tests (which could be across multiple test files), generate the report and save it to the current directory.
For extra parameters you can run the following,
```
python3 -m unitreport -h
usage: __main__.py [-h] [--tests_dir TESTS_DIR] [--pattern PATTERN]
[--templates_dir TEMPLATES_DIR] [--output_file OUTPUT_FILE]
optional arguments:
-h, --help show this help message and exit
--tests_dir TESTS_DIR
Path to test files. (default: .)
--pattern PATTERN File patterns to discover test cases in. (default:
test*.py)
--templates_dir TEMPLATES_DIR
Path to jinja2 templates directory including
index.html and main.css. (default: (unitreport) templates)
--output_file OUTPUT_FILE
Output path including name. (default: report.html)
```
There are template `index.html` and `main.css` files which will be used by default to generate the style of the report.
You can also specify a path to your own templates using `--templates_dir`, where the html Jinja2 template can expect to receive `date` (today's date), and `figures`, a dictionary with test function names as keys mapped to values of `type` (table or plot), `content` (svg or html table) and `description` (test function's docstring).
You can also invoke unitreport from a Python script using the library's `main()` (runs tests and generates report), `discover_and_run()` (only run tests) and `generate_report()` (only generate report) functions.
These utilise the default values for the above parameters if not specified by the user, and `generate_report()` uses the global FIGURES dictionary generated from the tests if not passed. For more details on what they do, you can check the source code.
```python
import unitreport
# result is a unittest.TestResult which you can access things such as result.errors
result, figs = unitreport.discover_and_run()
print(result) # <unittest.runner.TextTestResult run=3 errors=0 failures=0>
print(figs) # Dict with test function names as keys mapped to 'type', 'content', and 'description'
# html_report is a string containing the generated report
html_report = unitreport.generate_report()
# same as above, raises assertion error if there are errors in tests or no tests found
result, figs, html_report = unitreport.main()
```
## Built With
- [unittest](https://docs.python.org/3/library/unittest.html) - underlying testing framework
- [Jinja2](https://jinja.palletsprojects.com/en/2.11.x/) - rendering and generating the report
- [matplotlib](https://matplotlib.org/) - plotting library
- [Markdown](https://python-markdown.github.io/) - Python markdown library for markdown captions
Raw data
{
"_id": null,
"home_page": "https://github.com/annahadji/unitreport",
"name": "unitreport",
"maintainer": "",
"docs_url": null,
"requires_python": ">=3.6",
"maintainer_email": "",
"keywords": "static unittest report generator Markdown plots tables",
"author": "annahadji",
"author_email": "annahadji@users.noreply.github.com",
"download_url": "https://files.pythonhosted.org/packages/c2/47/aabb7395e82da94754b6152202491fa9a3f61fba49241d8380775ae7580b/unitreport-0.1.1.tar.gz",
"platform": null,
"description": "# :page_facing_up: unitreport  \n\nUnitReport is a small unittest-based tool for generating single page html reports in Python.\nThe reports can include matplotlib figures and html tables.\nIt is designed to be minimal and fit into the unittesting framework, allowing users to make assertions about their data (e.g. data quality) before generating figures.\n\n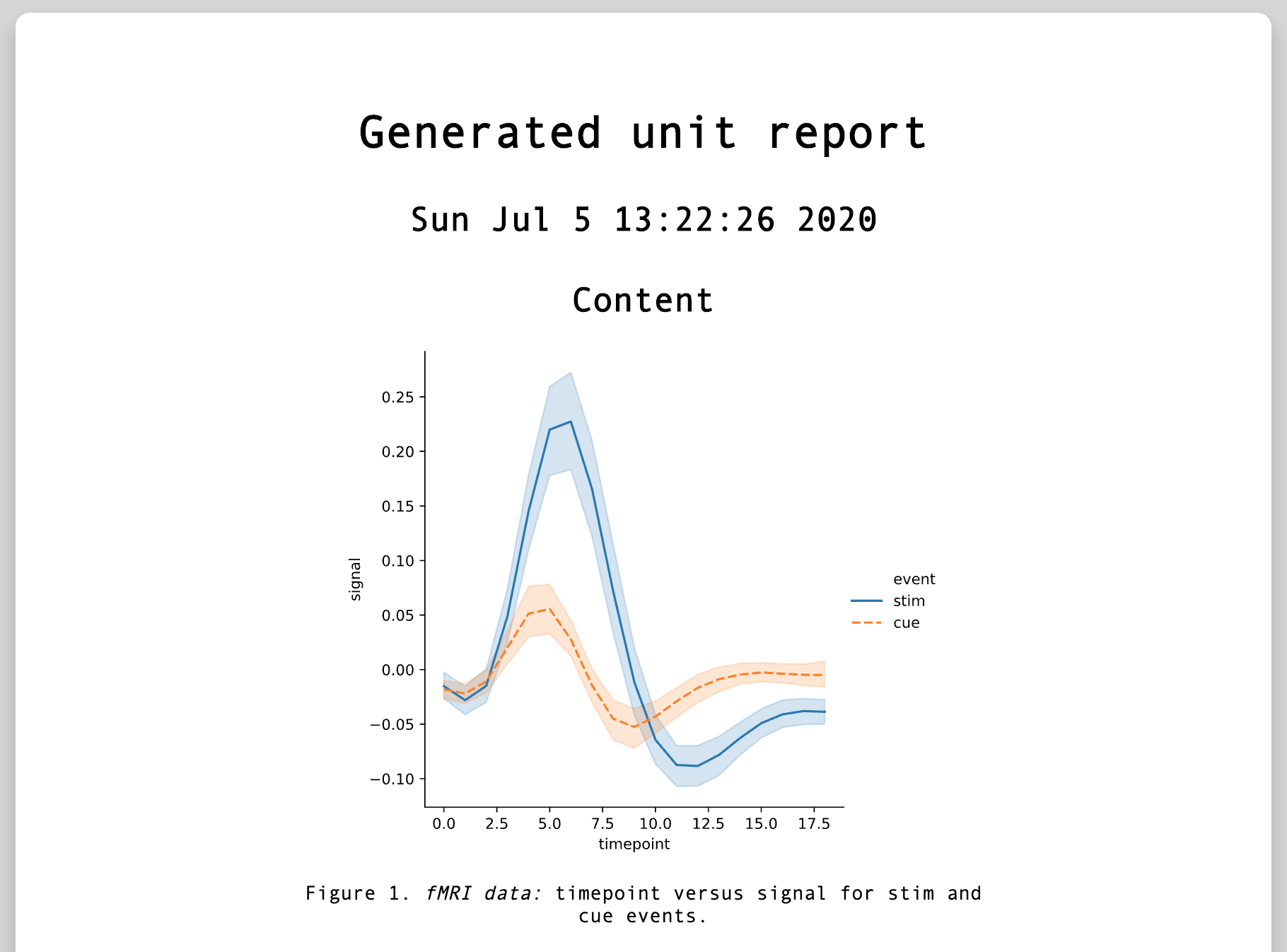\n\n## Getting Started\n\nYou can install the library using,\n\n`pip3 install unitreport`\n\nThere are usage examples in `test_plots.py` and `test_tables.py`.\nYou need to create test cases using the unittest Python library, and utilise unitreport's decorators:\n\n```python\nimport unittest\nimport unitreport\n\nimport seaborn as sns\nimport pandas as pd\n\nclass TestExample(unittest.TestCase):\n \"\"\"Example test suite producing plots and tables in a report using unitreport.\"\"\"\n\n dataset: pd.DataFrame = None\n\n @classmethod\n def setUpClass(cls):\n \"\"\"Load dataset on setup.\"\"\"\n cls.dataset = sns.load_dataset(\"fmri\")\n\n @unitreport.plotting\n def test_timepoint_vs_signal_by_event(self):\n \"\"\"*fMRI data:* timepoint versus signal for stim and cue events.\"\"\"\n # you can still run assertions to check data quality before plotting\n self.assertEqual(self.dataset.shape, (1064, 5))\n\n # plotting decorator will call plt.savefig() to generate the plot\n sns.relplot(\n x=\"timepoint\",\n y=\"signal\",\n hue=\"event\",\n style=\"event\",\n kind=\"line\",\n data=self.dataset,\n )\n # could also return a figure & .savefig() will be called on returned object\n\n @unitreport.tabling\n def test_region_counts(self):\n \"\"\"*fMRI data:* table summary description of timepoints and signals.\"\"\"\n # you can still run assertions to check data quality before making table\n self.assertEqual(self.dataset.shape, (1064, 5))\n\n return self.dataset.describe().to_html()\n```\n\nYou can run the tests using,\n\n`python3 -m unitreport`\n\nThis will discover and run the tests (which could be across multiple test files), generate the report and save it to the current directory.\n\nFor extra parameters you can run the following,\n\n```\npython3 -m unitreport -h\n\nusage: __main__.py [-h] [--tests_dir TESTS_DIR] [--pattern PATTERN]\n [--templates_dir TEMPLATES_DIR] [--output_file OUTPUT_FILE]\n\noptional arguments:\n -h, --help show this help message and exit\n --tests_dir TESTS_DIR\n Path to test files. (default: .)\n --pattern PATTERN File patterns to discover test cases in. (default:\n test*.py)\n --templates_dir TEMPLATES_DIR\n Path to jinja2 templates directory including\n index.html and main.css. (default: (unitreport) templates)\n --output_file OUTPUT_FILE\n Output path including name. (default: report.html)\n```\n\nThere are template `index.html` and `main.css` files which will be used by default to generate the style of the report.\nYou can also specify a path to your own templates using `--templates_dir`, where the html Jinja2 template can expect to receive `date` (today's date), and `figures`, a dictionary with test function names as keys mapped to values of `type` (table or plot), `content` (svg or html table) and `description` (test function's docstring).\n\nYou can also invoke unitreport from a Python script using the library's `main()` (runs tests and generates report), `discover_and_run()` (only run tests) and `generate_report()` (only generate report) functions.\nThese utilise the default values for the above parameters if not specified by the user, and `generate_report()` uses the global FIGURES dictionary generated from the tests if not passed. For more details on what they do, you can check the source code.\n\n```python\nimport unitreport\n\n# result is a unittest.TestResult which you can access things such as result.errors\nresult, figs = unitreport.discover_and_run()\nprint(result) # <unittest.runner.TextTestResult run=3 errors=0 failures=0>\nprint(figs) # Dict with test function names as keys mapped to 'type', 'content', and 'description'\n# html_report is a string containing the generated report\nhtml_report = unitreport.generate_report()\n\n# same as above, raises assertion error if there are errors in tests or no tests found\nresult, figs, html_report = unitreport.main()\n```\n\n## Built With\n\n- [unittest](https://docs.python.org/3/library/unittest.html) - underlying testing framework\n- [Jinja2](https://jinja.palletsprojects.com/en/2.11.x/) - rendering and generating the report\n- [matplotlib](https://matplotlib.org/) - plotting library\n- [Markdown](https://python-markdown.github.io/) - Python markdown library for markdown captions\n\n\n",
"bugtrack_url": null,
"license": "",
"summary": "A small unittest-based tool for generating single page html reports in Python.",
"version": "0.1.1",
"project_urls": {
"Homepage": "https://github.com/annahadji/unitreport"
},
"split_keywords": [
"static",
"unittest",
"report",
"generator",
"markdown",
"plots",
"tables"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "1c28643735e43141bcca9c6030fef867b23e1c333a7a46ac6a45dfe9e6aa4b4e",
"md5": "26d572ac84722233807a5dfa8a3be0b5",
"sha256": "4d5ec32393fb26603791962b10a2a301ad852798f1a992e882ed6f10af519455"
},
"downloads": -1,
"filename": "unitreport-0.1.1-py3-none-any.whl",
"has_sig": false,
"md5_digest": "26d572ac84722233807a5dfa8a3be0b5",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.6",
"size": 8901,
"upload_time": "2023-06-23T19:41:34",
"upload_time_iso_8601": "2023-06-23T19:41:34.288133Z",
"url": "https://files.pythonhosted.org/packages/1c/28/643735e43141bcca9c6030fef867b23e1c333a7a46ac6a45dfe9e6aa4b4e/unitreport-0.1.1-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "c247aabb7395e82da94754b6152202491fa9a3f61fba49241d8380775ae7580b",
"md5": "144ae26eadcb00925326288fb2ef1b7a",
"sha256": "b19826e09c04527467ecedbb5d3053bf52d7c24debb1f4ded603138f92c5d99a"
},
"downloads": -1,
"filename": "unitreport-0.1.1.tar.gz",
"has_sig": false,
"md5_digest": "144ae26eadcb00925326288fb2ef1b7a",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.6",
"size": 7294,
"upload_time": "2023-06-23T19:41:35",
"upload_time_iso_8601": "2023-06-23T19:41:35.675409Z",
"url": "https://files.pythonhosted.org/packages/c2/47/aabb7395e82da94754b6152202491fa9a3f61fba49241d8380775ae7580b/unitreport-0.1.1.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-06-23 19:41:35",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "annahadji",
"github_project": "unitreport",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "unitreport"
}