# utilityai
This package brings language model capabilities into the coding environment, providing a variety of functionalities such as:
- Message and ask anything
- Chat and have a conversation
- Chat about a function
- Chat about a numpy array
- Chat about a pandas dataframe
- Chat about a pytorch tensor
- Generate a function interactively
- Generate a function by setting data within the code
- Generate a function and provide a comment on the result for guided generation
Function generation feature iteratively builds and refines functions by evaluating them against predefined test cases.
## install
```
pip install utilityai
```
## quick start
Download the model once after installation
```
from utilityai.model import download
download()
```
Message and ask anything
```
from utilityai.chat import message
message("How do you transpose a PyTorch tensor?");
```
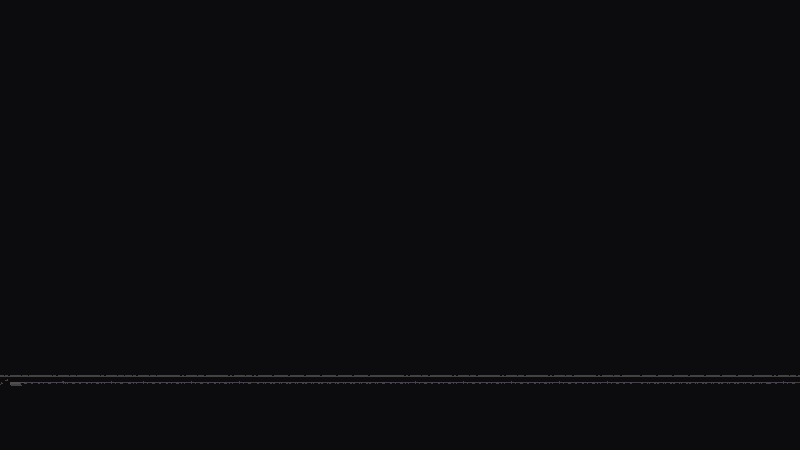
Chat and have a conversation
```
from utilityai.chat import message
r1, c1 = message("What do mutable and immutable mean?")
message("Give more examples.", c1);
```
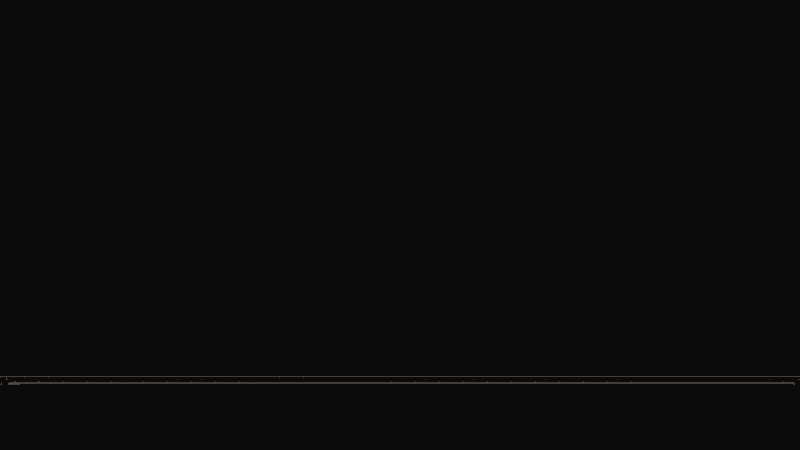
Chat about a function
```
from utilityai.chat import message
def list_sum(numbers):
return sum(numbers)
r1, c1 = message("What does this do?", attachment=list_sum)
message("Return the minimum and maximum values of the numbers instead.", c1);
```
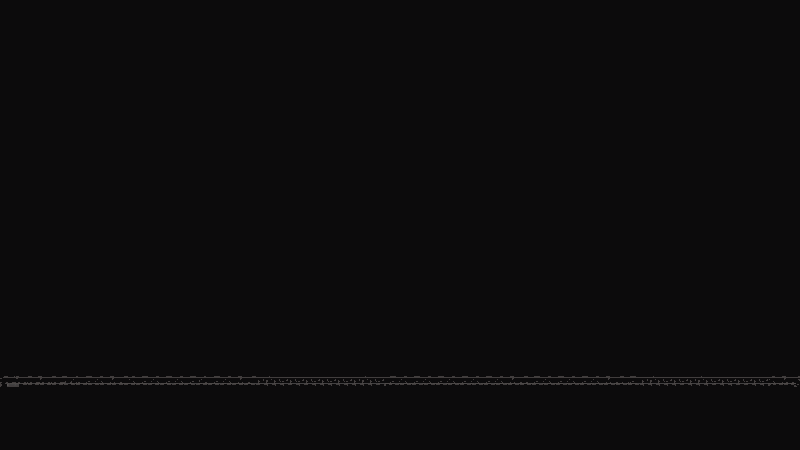
Chat about a numpy array
```
from utilityai.chat import message
import numpy as np
array = np.array([[1, 2, 3, 4],
[5, 6, 7, 8],
[9, 10, 11, 12]])
r1, c1 = message("Each row represents the salary of a person. How do I calculate the average salary of each person in another array?", attachment=array)
message("How do I calculate the average salary of these people for each year in an array?", c1);
```
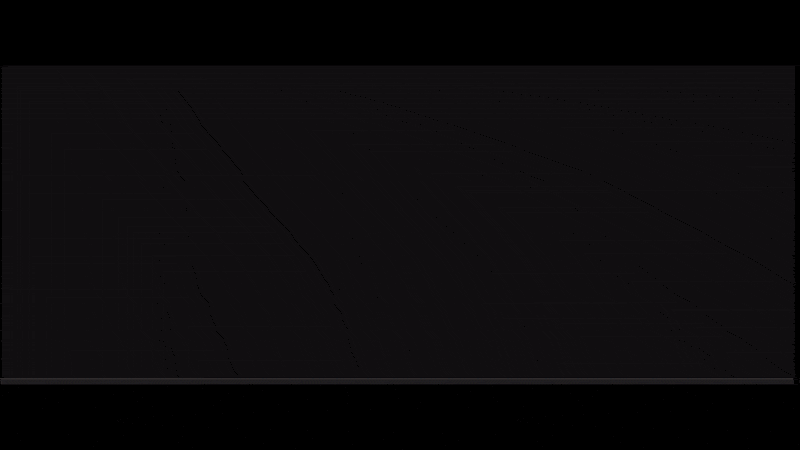
Chat about a pandas dataframe
```
from utilityai.chat import message
import pandas as pd
data = {
'Name': ['Alice', 'Bob', 'Charlie', 'David', 'Eve'],
'Age': [24, 27, 22, 32, 29],
'Salary': [50000, 54000, 49000, 62000, 58000],
'Department': ['HR', 'Engineering', 'Marketing', 'Finance', 'Engineering'],
'Joining Date': pd.to_datetime(['2020-01-15', '2019-06-23', '2021-03-01', '2018-11-15', '2020-08-30'])
}
df = pd.DataFrame(data)
r1, c1 = message("How to calculate the average salary?", attachment=df)
message("How to calculate the average salary for each department?", c1);
```
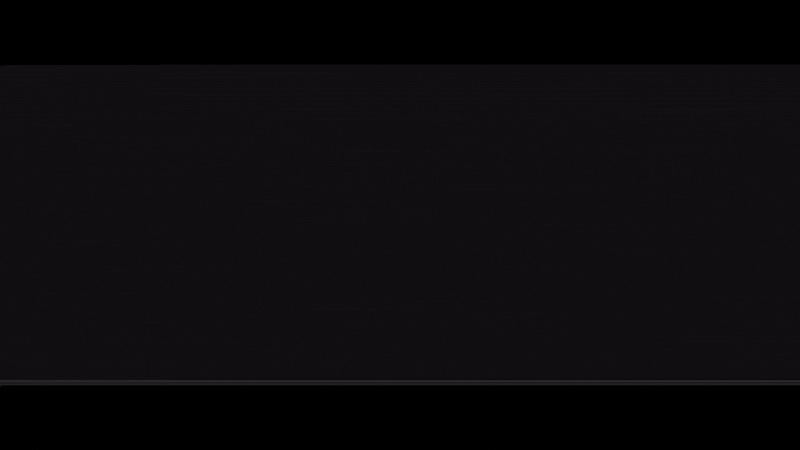
Chat about a pytorch tensor
```
from utilityai.chat import message
import torch
tensor = torch.tensor([[1, 2, 3], [4, 5, 6]])
r1, c1 = message("How to transpose this tensor?", attachment=tensor)
message("How to determine the size of the resulting tensor?", c1);
```
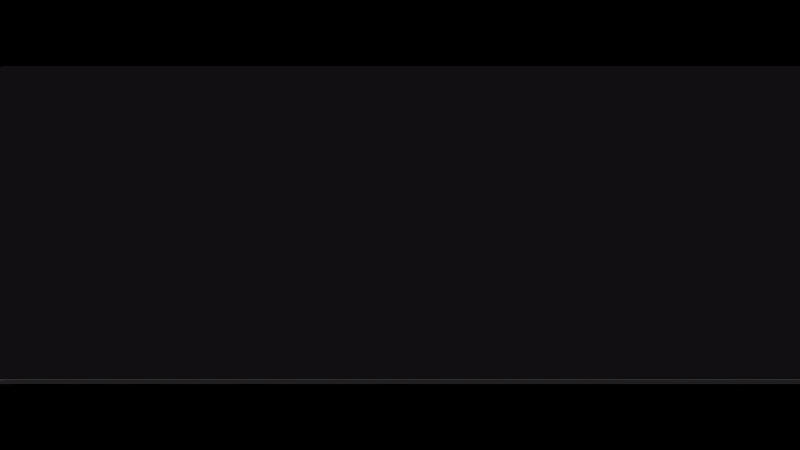
Generate a function interactively by calling data() first, then provide function information
```
from utilityai.code import InputData, function
data = InputData()
data()
function(data);
```
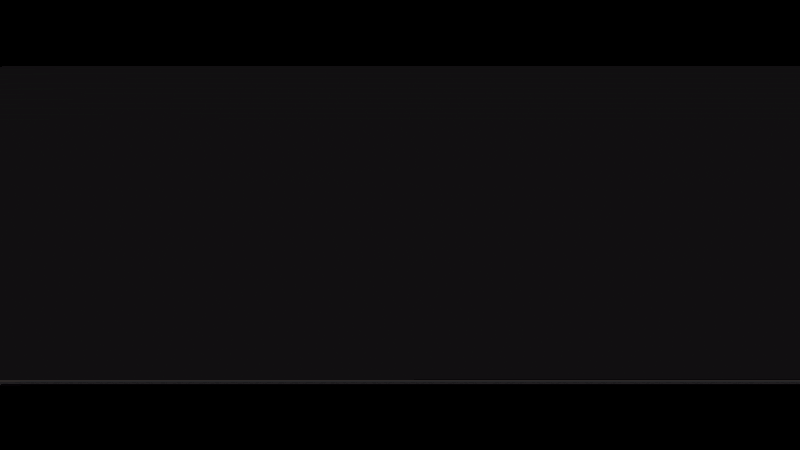
Generate a function by setting data within the code
```
from utilityai.code import InputData, function
data = InputData()
data_dict = {
'function_name': 'prime_number_checker',
'input_names': ['num'],
'input_types': ['int'],
'output_names': ['is_prime'],
'output_types': ['bool'],
'description': "A function to check if a given number is prime.",
'test_cases': [
{'inputs': [5], 'outputs': [True]},
{'inputs': [10], 'outputs': [False]},
{'inputs': [17], 'outputs': [True]}
]
}
data.set_data(data_dict['function_name'], data_dict['input_names'], data_dict['output_names'], data_dict['input_types'], data_dict['output_types'], data_dict['description'], data_dict['test_cases'])
function(data);
```
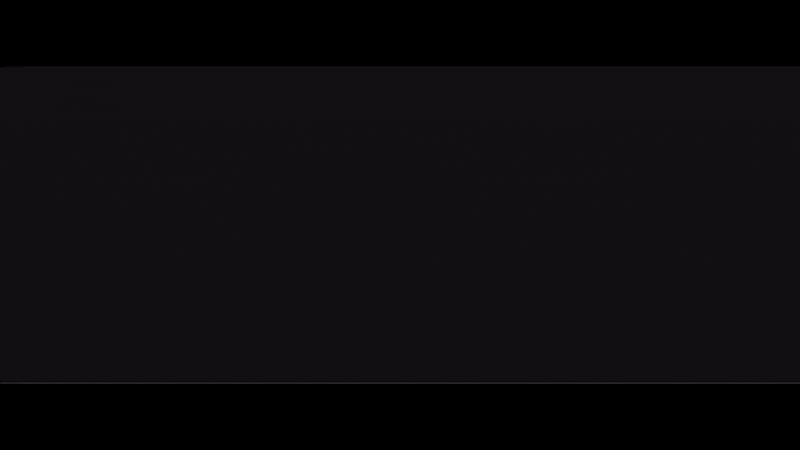
Generate a function and provide a comment on the result for guided generation
```
from utilityai.code import InputData, function
data = InputData()
data_dict = {
'function_name': 'vague_function',
'input_names': ['a', 'b'],
'input_types': ['int', 'int'],
'output_names': ['subtract'],
'output_types': ['int'],
'description': "A function to subtract two numbers.",
'test_cases': [
{'inputs': [1,2], 'outputs': [1]}
]
}
data.set_data(data_dict['function_name'], data_dict['input_names'], data_dict['output_names'], data_dict['input_types'], data_dict['output_types'], data_dict['description'], data_dict['test_cases'])
res = function(data, max_tries=1)
res.comment = "A function that subtracts the smaller number from the larger one."
function(data, res);
```
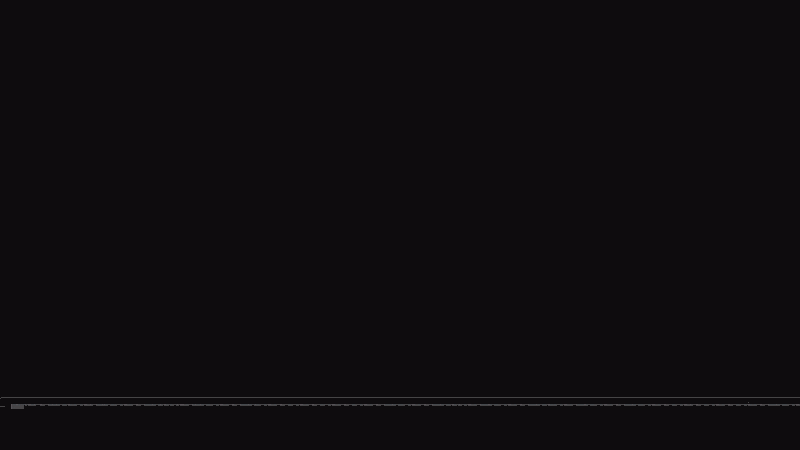
Raw data
{
"_id": null,
"home_page": "https://github.com/navid-matinmo/utilityai",
"name": "utilityai",
"maintainer": null,
"docs_url": null,
"requires_python": ">=3.0",
"maintainer_email": null,
"keywords": "LLM, AI",
"author": "Navid Matin Moghaddam",
"author_email": "navid.matinmo@gmail.com",
"download_url": "https://files.pythonhosted.org/packages/e6/03/9f1a096c0d7381973660420ea017a5cf3bc0eab2d1a007eae1a49f779cc7/utilityai-1.0.1.tar.gz",
"platform": null,
"description": "# utilityai\n\nThis package brings language model capabilities into the coding environment, providing a variety of functionalities such as:\n\n- Message and ask anything\n- Chat and have a conversation\n- Chat about a function\n- Chat about a numpy array\n- Chat about a pandas dataframe\n- Chat about a pytorch tensor\n- Generate a function interactively\n- Generate a function by setting data within the code\n- Generate a function and provide a comment on the result for guided generation\n\nFunction generation feature iteratively builds and refines functions by evaluating them against predefined test cases.\n\n## install\n\n```\npip install utilityai\n```\n\n## quick start\n\nDownload the model once after installation\n```\nfrom utilityai.model import download\ndownload()\n```\n\nMessage and ask anything\n```\nfrom utilityai.chat import message\nmessage(\"How do you transpose a PyTorch tensor?\");\n```\n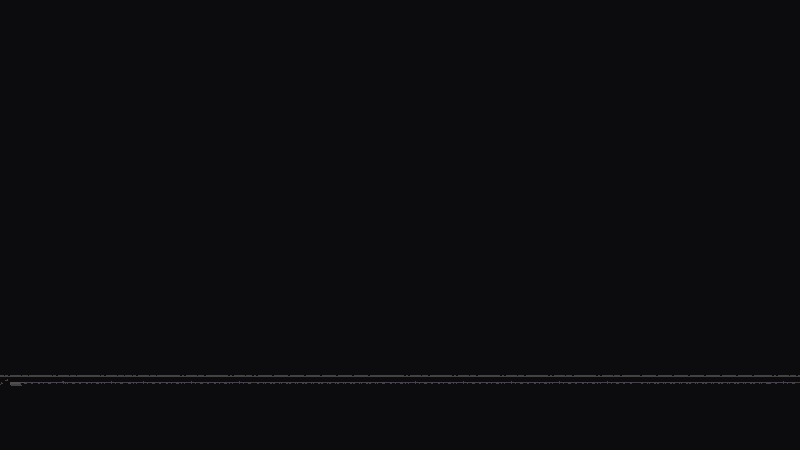\n\nChat and have a conversation\n```\nfrom utilityai.chat import message\nr1, c1 = message(\"What do mutable and immutable mean?\")\nmessage(\"Give more examples.\", c1);\n```\n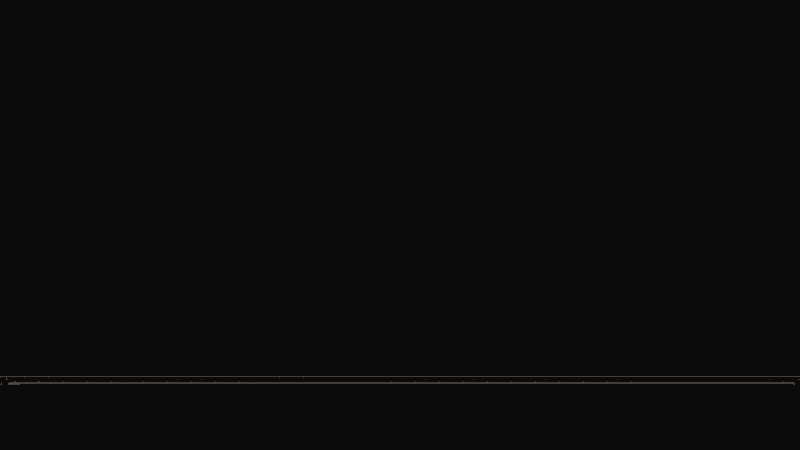\n\nChat about a function\n```\nfrom utilityai.chat import message\ndef list_sum(numbers):\n return sum(numbers)\nr1, c1 = message(\"What does this do?\", attachment=list_sum)\nmessage(\"Return the minimum and maximum values of the numbers instead.\", c1);\n```\n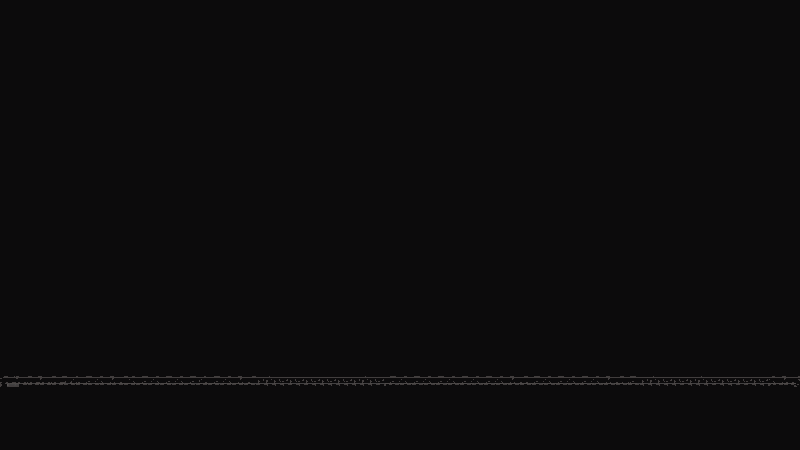\n\n\nChat about a numpy array\n```\nfrom utilityai.chat import message\nimport numpy as np\narray = np.array([[1, 2, 3, 4], \n [5, 6, 7, 8], \n [9, 10, 11, 12]])\nr1, c1 = message(\"Each row represents the salary of a person. How do I calculate the average salary of each person in another array?\", attachment=array)\nmessage(\"How do I calculate the average salary of these people for each year in an array?\", c1);\n```\n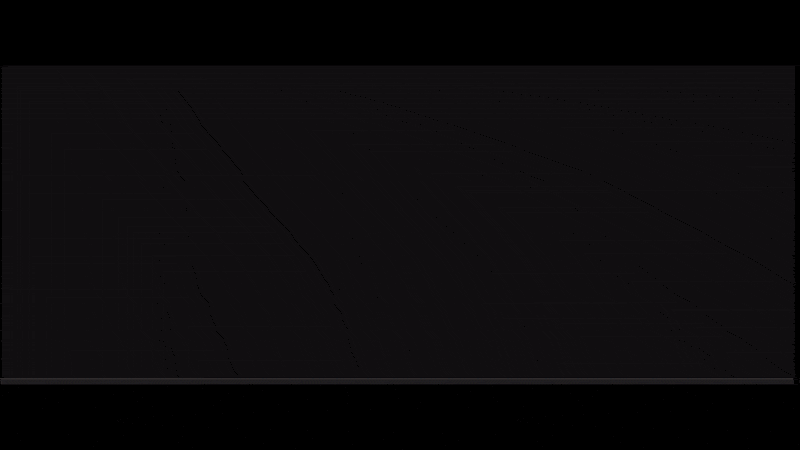\n\nChat about a pandas dataframe\n```\nfrom utilityai.chat import message\nimport pandas as pd\ndata = {\n 'Name': ['Alice', 'Bob', 'Charlie', 'David', 'Eve'],\n 'Age': [24, 27, 22, 32, 29],\n 'Salary': [50000, 54000, 49000, 62000, 58000],\n 'Department': ['HR', 'Engineering', 'Marketing', 'Finance', 'Engineering'],\n 'Joining Date': pd.to_datetime(['2020-01-15', '2019-06-23', '2021-03-01', '2018-11-15', '2020-08-30'])\n}\ndf = pd.DataFrame(data)\nr1, c1 = message(\"How to calculate the average salary?\", attachment=df)\nmessage(\"How to calculate the average salary for each department?\", c1);\n```\n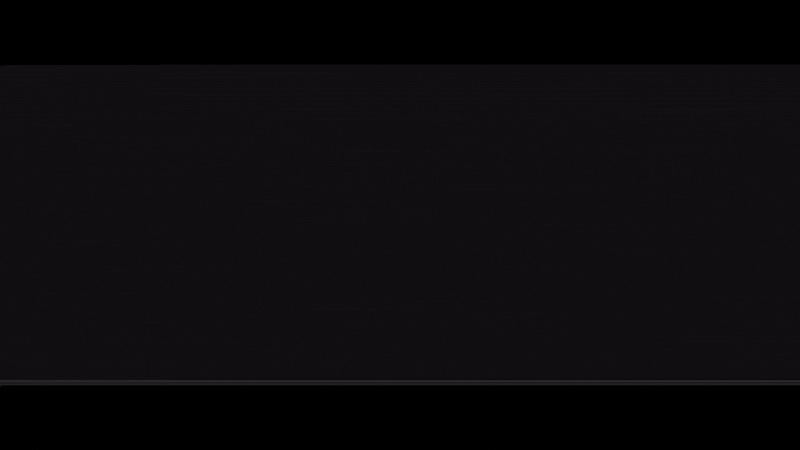\n\nChat about a pytorch tensor\n```\nfrom utilityai.chat import message\nimport torch\ntensor = torch.tensor([[1, 2, 3], [4, 5, 6]])\nr1, c1 = message(\"How to transpose this tensor?\", attachment=tensor)\nmessage(\"How to determine the size of the resulting tensor?\", c1);\n```\n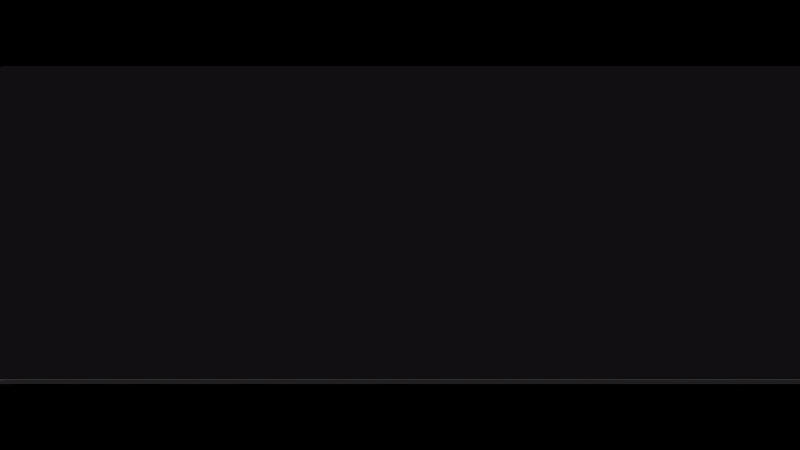\n\nGenerate a function interactively by calling data() first, then provide function information\n```\nfrom utilityai.code import InputData, function\ndata = InputData()\ndata()\nfunction(data);\n```\n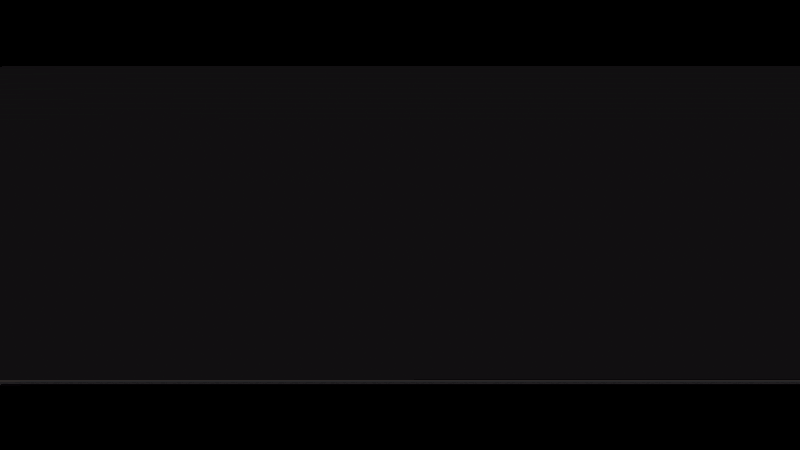\n\nGenerate a function by setting data within the code\n```\nfrom utilityai.code import InputData, function\ndata = InputData()\ndata_dict = {\n 'function_name': 'prime_number_checker',\n 'input_names': ['num'],\n 'input_types': ['int'],\n 'output_names': ['is_prime'],\n 'output_types': ['bool'],\n 'description': \"A function to check if a given number is prime.\",\n 'test_cases': [\n {'inputs': [5], 'outputs': [True]},\n {'inputs': [10], 'outputs': [False]},\n {'inputs': [17], 'outputs': [True]}\n ]\n}\ndata.set_data(data_dict['function_name'], data_dict['input_names'], data_dict['output_names'], data_dict['input_types'], data_dict['output_types'], data_dict['description'], data_dict['test_cases'])\nfunction(data);\n```\n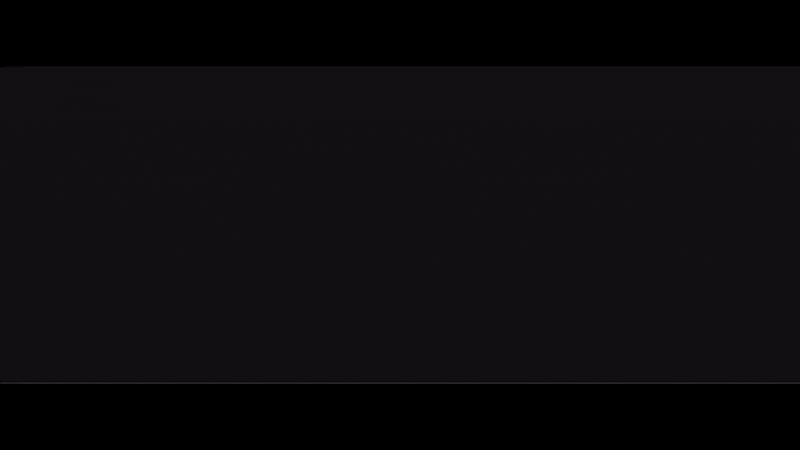\n\nGenerate a function and provide a comment on the result for guided generation\n```\nfrom utilityai.code import InputData, function\ndata = InputData()\ndata_dict = {\n 'function_name': 'vague_function',\n 'input_names': ['a', 'b'],\n 'input_types': ['int', 'int'],\n 'output_names': ['subtract'],\n 'output_types': ['int'],\n 'description': \"A function to subtract two numbers.\",\n 'test_cases': [\n {'inputs': [1,2], 'outputs': [1]}\n ]\n}\ndata.set_data(data_dict['function_name'], data_dict['input_names'], data_dict['output_names'], data_dict['input_types'], data_dict['output_types'], data_dict['description'], data_dict['test_cases'])\nres = function(data, max_tries=1)\nres.comment = \"A function that subtracts the smaller number from the larger one.\"\nfunction(data, res);\n```\n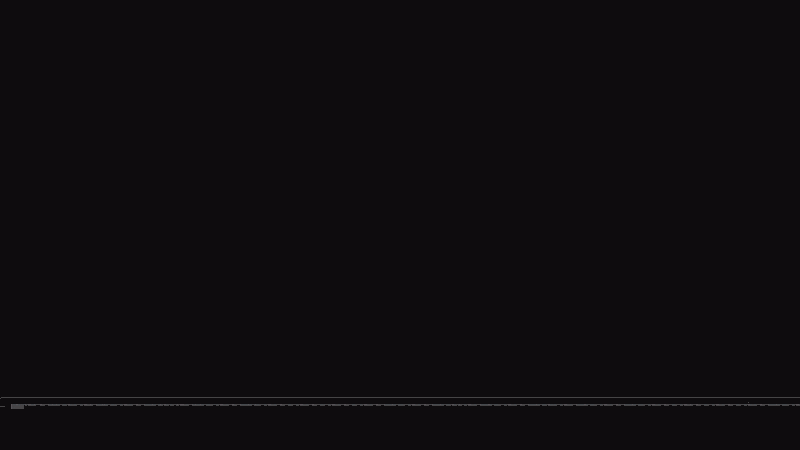",
"bugtrack_url": null,
"license": "MIT",
"summary": "This package brings language model capabilities into the coding environment, providing a variety of functionalities.",
"version": "1.0.1",
"project_urls": {
"Documentation": "https://github.com/navid-matinmo/utilityai",
"Homepage": "https://github.com/navid-matinmo/utilityai",
"Repository": "https://github.com/navid-matinmo/utilityai",
"issues": "https://github.com/navid-matinmo/utilityai/issues"
},
"split_keywords": [
"llm",
" ai"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "95b8e475cbcf5a5f17ff5650f943f59350a6f1c6a0f5484c25c32b692a843d4f",
"md5": "4b0f15d22a971f343d2bf412f2985b97",
"sha256": "7cb15bd4334f1ec664b2ea0981b499d820cf7cac6e5c1846695a5f2eb7fe056b"
},
"downloads": -1,
"filename": "utilityai-1.0.1-py3-none-any.whl",
"has_sig": false,
"md5_digest": "4b0f15d22a971f343d2bf412f2985b97",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.0",
"size": 11485,
"upload_time": "2024-06-05T14:53:48",
"upload_time_iso_8601": "2024-06-05T14:53:48.136981Z",
"url": "https://files.pythonhosted.org/packages/95/b8/e475cbcf5a5f17ff5650f943f59350a6f1c6a0f5484c25c32b692a843d4f/utilityai-1.0.1-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "e6039f1a096c0d7381973660420ea017a5cf3bc0eab2d1a007eae1a49f779cc7",
"md5": "666321ae358418986320369c7524bf23",
"sha256": "1b909a370ea7b7fd12dd382369226510390f2e1eb047b4925a74cced56586ee1"
},
"downloads": -1,
"filename": "utilityai-1.0.1.tar.gz",
"has_sig": false,
"md5_digest": "666321ae358418986320369c7524bf23",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.0",
"size": 10654,
"upload_time": "2024-06-05T14:53:49",
"upload_time_iso_8601": "2024-06-05T14:53:49.654325Z",
"url": "https://files.pythonhosted.org/packages/e6/03/9f1a096c0d7381973660420ea017a5cf3bc0eab2d1a007eae1a49f779cc7/utilityai-1.0.1.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-06-05 14:53:49",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "navid-matinmo",
"github_project": "utilityai",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"lcname": "utilityai"
}