# vcolorpicker
Simple visual Color Picker with a modern UI created with Qt to easily get color input from the user.
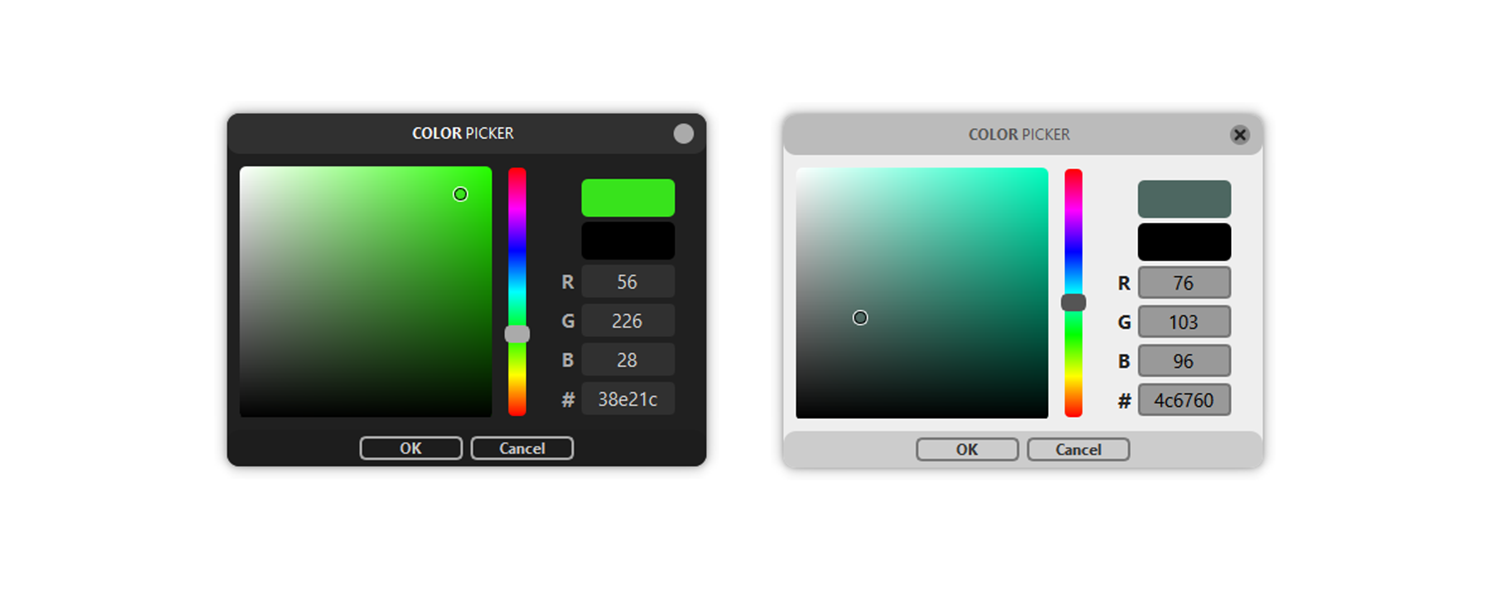
## Installation
1. Install using pip:
```
pip install vcolorpicker
```
or clone the repository yourself and run:
```
pip install .
```
## Usage
2. To ask for a color, import the `getColor` function and run it:
```python
from vcolorpicker import getColor
color = getColor()
```
## Customization
* **Showing custom last color:**
```python
old_color = (255,255,255)
picked_color = getColor(old_color)
```
* **Changing the UI Theme**
```python
from vcolorpicker import useLightTheme
useLightTheme(True)
```
* **Adding Alpha selection**
```python
from vcolorpicker import useAlpha
useAlpha(True)
```
When the ColorPicker uses Alpha, you have to pass a RGBA tuple\
as the last color, otherwise there wil be an error.
```python
old_color = (255,255,255,100)
picked_color = getColor(old_color) # => (r,g,b,a)
```
## Color Formats and Conversion
* The default format `getColor` will give you is RGB(A),\
but you can use vcolorpickers color conversion functions\
if you have a different format like HSV or HEX.
`hsv2rgb` **HSV(A)** to **RGB(A)**\
`rgb2hsv` **RGB(A)** to **HSV(A)**\
`rgb2hex` **RGB(A)** to **HEX**\
`hex2rgb` **HEX** to **RGB**\
`hex2hsv` **HEX** to **HSV**\
`hsv2hex` **HSV(A)** to **HEX**
* Example:
```python
from vcolorpicker import getColor, hsv2rgb, rgb2hsv
old_color = hsv2rgb((50,50,100,100)) # => (127,255,255,100)
picked_color = rgb2hsv(getColor(old_color))
```
* **Color Formats:**
**RGB** values range from **0** to **255**\
**HSV** values range from **0** to **100**\
**HEX** values should be in format: `"XXXXXX"` or `"xxxxxx"`\
**Alpha** values range from **0** to **100**
## Compatibility
This package is compatible with **Python 3.7+** and above.
It uses [qtpy](https://github.com/spyder-ide/qtpy) under the hood, so it should work with all Qt bindings (PyQt5, PySide2, PySide6, PyQt6).
## Previous versions
In previous versions you had to create a ColorPicker object first and then\
call it's `getColor` method. This is still supported, you just have to\
import the `ColorPicker` class.
The color conversion functions are not methods anymore, you can import them\
directly with `from vcolorpicker import hsv2rgb, rgb2hsv`.
You also had to create a `QApplication` object before being able to run the\
ColorPicker, now it automatically creates one by itself if there isn't one yet.\
If you need to get the auto-created application, you can use this:
```python
from PyQt5.QtWidgets import QApplication
app = QApplication.instance()
```
## Bugs and Improvement ideas
If you find a bug, you can open an issue or write me an email (nlfmt@gmx.de)\
and I will try to get to it as fast as possible, or you can implement it\
yourself and create a pull request.
## License
This software is licensed under the **MIT License**.\
More information is provided in the dedicated LICENSE file.
Raw data
{
"_id": null,
"home_page": "https://github.com/nlfmt/pyqt-colorpicker",
"name": "vcolorpicker",
"maintainer": "",
"docs_url": null,
"requires_python": ">=3.7",
"maintainer_email": "",
"keywords": "python color gui colorpicker visual qt",
"author": "nlfmt",
"author_email": "nlfmt@gmx.de",
"download_url": "https://files.pythonhosted.org/packages/4b/dc/490bf7e3a4d99ce59ab0ed68743510c1756e5faa594cca8eb82d29a4a2a1/vcolorpicker-1.4.3.tar.gz",
"platform": null,
"description": "# vcolorpicker\nSimple visual Color Picker with a modern UI created with Qt to easily get color input from the user.\n\n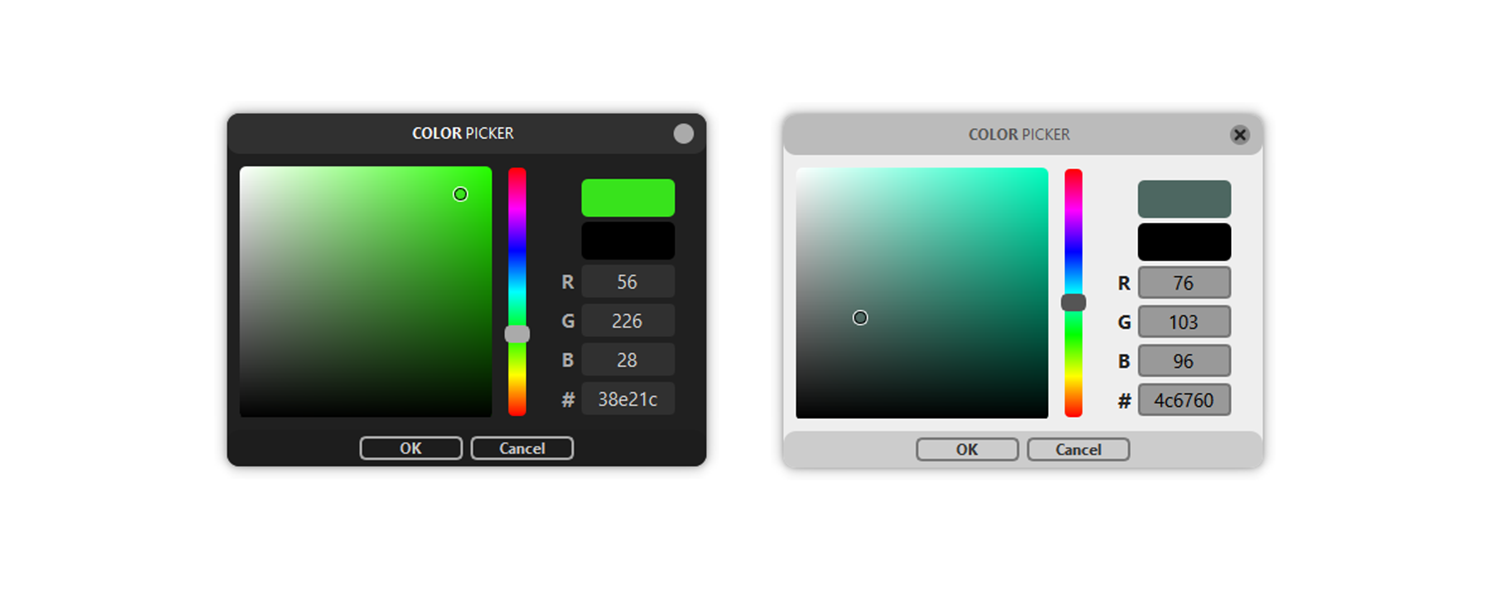\n\n\n## Installation\n\n1. Install using pip:\n\n ```\n pip install vcolorpicker\n ```\n\n or clone the repository yourself and run:\n\n ```\n pip install .\n ```\n\n## Usage\n\n2. To ask for a color, import the `getColor` function and run it:\n\n ```python\n from vcolorpicker import getColor\n \n color = getColor()\n ```\n\n## Customization\n\n* **Showing custom last color:**\n\n ```python\n old_color = (255,255,255)\n picked_color = getColor(old_color)\n ```\n\n* **Changing the UI Theme**\n\n ```python\n from vcolorpicker import useLightTheme\n \n useLightTheme(True)\n ```\n\n* **Adding Alpha selection**\n\n ```python\n from vcolorpicker import useAlpha\n \n useAlpha(True)\n ```\n\n When the ColorPicker uses Alpha, you have to pass a RGBA tuple\\\n as the last color, otherwise there wil be an error.\n\n ```python\n old_color = (255,255,255,100)\n picked_color = getColor(old_color) # => (r,g,b,a)\n ```\n\n## Color Formats and Conversion\n\n* The default format `getColor` will give you is RGB(A),\\\n but you can use vcolorpickers color conversion functions\\\n if you have a different format like HSV or HEX.\n\n `hsv2rgb` **HSV(A)** to **RGB(A)**\\\n `rgb2hsv` **RGB(A)** to **HSV(A)**\\\n `rgb2hex` **RGB(A)** to **HEX**\\\n `hex2rgb` **HEX** to **RGB**\\\n `hex2hsv` **HEX** to **HSV**\\\n `hsv2hex` **HSV(A)** to **HEX**\n\n* Example:\n ```python\n from vcolorpicker import getColor, hsv2rgb, rgb2hsv \n \n old_color = hsv2rgb((50,50,100,100)) # => (127,255,255,100)\n\n picked_color = rgb2hsv(getColor(old_color))\n ```\n\n* **Color Formats:**\n\n **RGB** values range from **0** to **255**\\\n **HSV** values range from **0** to **100**\\\n **HEX** values should be in format: `\"XXXXXX\"` or `\"xxxxxx\"`\\\n **Alpha** values range from **0** to **100**\n\n\n## Compatibility\n This package is compatible with **Python 3.7+** and above.\n It uses [qtpy](https://github.com/spyder-ide/qtpy) under the hood, so it should work with all Qt bindings (PyQt5, PySide2, PySide6, PyQt6).\n\n## Previous versions\n In previous versions you had to create a ColorPicker object first and then\\\n call it's `getColor` method. This is still supported, you just have to\\\n import the `ColorPicker` class.\n\n The color conversion functions are not methods anymore, you can import them\\\n directly with `from vcolorpicker import hsv2rgb, rgb2hsv`.\n\n You also had to create a `QApplication` object before being able to run the\\\n ColorPicker, now it automatically creates one by itself if there isn't one yet.\\\n If you need to get the auto-created application, you can use this:\n\n ```python\n from PyQt5.QtWidgets import QApplication\n app = QApplication.instance()\n ```\n\n## Bugs and Improvement ideas\n If you find a bug, you can open an issue or write me an email (nlfmt@gmx.de)\\\n and I will try to get to it as fast as possible, or you can implement it\\\n yourself and create a pull request.\n\n\n## License\n This software is licensed under the **MIT License**.\\\n More information is provided in the dedicated LICENSE file.\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "Open a visual vcolorpicker from any project.",
"version": "1.4.3",
"project_urls": {
"Bug Tracker": "https://github.com/nlfmt/pyqt-colorpicker/issues",
"GitHub": "https://github.com/nlfmt/pyqt-colorpicker",
"Homepage": "https://github.com/nlfmt/pyqt-colorpicker"
},
"split_keywords": [
"python",
"color",
"gui",
"colorpicker",
"visual",
"qt"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "2ea4d6c2bea5b1b8883f8fa7277cd48a59baa6b14bab624537b2f8247df6cff3",
"md5": "79550173bbf55dded8b093bec55f38f4",
"sha256": "d509ec52823a2529ca5d7391cbe1216eec665e7d25d45a76b17708037e0b9854"
},
"downloads": -1,
"filename": "vcolorpicker-1.4.3-py3-none-any.whl",
"has_sig": false,
"md5_digest": "79550173bbf55dded8b093bec55f38f4",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.7",
"size": 22973,
"upload_time": "2023-08-16T23:41:53",
"upload_time_iso_8601": "2023-08-16T23:41:53.496617Z",
"url": "https://files.pythonhosted.org/packages/2e/a4/d6c2bea5b1b8883f8fa7277cd48a59baa6b14bab624537b2f8247df6cff3/vcolorpicker-1.4.3-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "4bdc490bf7e3a4d99ce59ab0ed68743510c1756e5faa594cca8eb82d29a4a2a1",
"md5": "2255b20f33fd2e4adb9763d4a14b31f7",
"sha256": "7aa221c3b517ca32300c9317f4e488a3d0772196c2e76648bac39943aae00551"
},
"downloads": -1,
"filename": "vcolorpicker-1.4.3.tar.gz",
"has_sig": false,
"md5_digest": "2255b20f33fd2e4adb9763d4a14b31f7",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.7",
"size": 15882,
"upload_time": "2023-08-16T23:41:55",
"upload_time_iso_8601": "2023-08-16T23:41:55.152415Z",
"url": "https://files.pythonhosted.org/packages/4b/dc/490bf7e3a4d99ce59ab0ed68743510c1756e5faa594cca8eb82d29a4a2a1/vcolorpicker-1.4.3.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-08-16 23:41:55",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "nlfmt",
"github_project": "pyqt-colorpicker",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "vcolorpicker"
}