# Video Filter
Enhance your video with color filters. This Python project helps
you to apply a color filter to your Instagram reel or any other social platforms
like TikTok, YouTube, Facebook, etc. All formats are supported (reels, shorts,
4k, etc.). The output video is in mp4 format.
## Filter gallery selection
original | grayscale | warm |
:---:|:---:|:---:
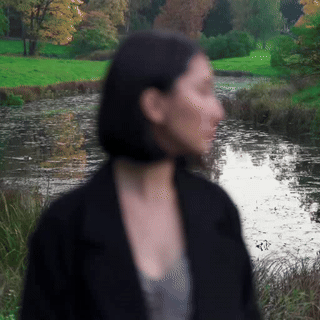 | 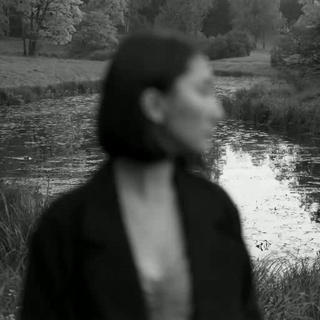 | 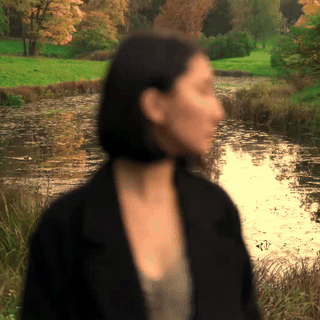
cool | red_only | invert_red_green
:---:|:---:|:---:
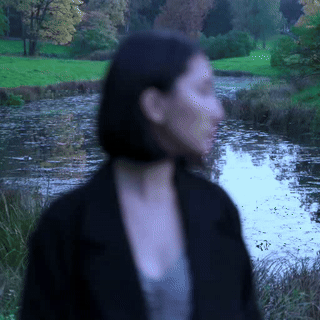 | 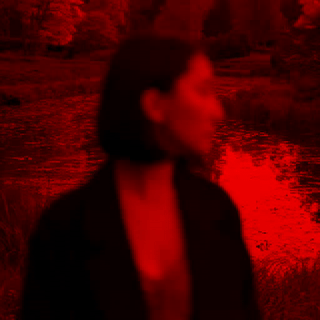 | 
## Installation
Run the following command in the terminal to install the package:
```
pip install video-filter
```
## Usage examples
### Example 1: Brightness and saturation
Brightness and saturation are both set to 0.0 by default, meaning the video
will be unchanged.
x | Brightness -1.0 | Brightness 0.0 | Brightness +1.0
:---:|:---:|:---:|:---:
**Saturation -1.0** | |  |
**Saturation 0.0** |  | 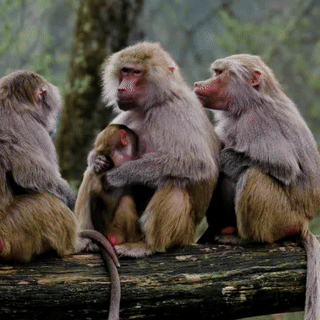 | 
**Saturation +1.0** | |  |
```
from video_filter import VideoFilter
vf = VideoFilter(brightness=1.0, saturation=0.0)
vf.process_video("examples/video_1.mp4", "output.mp4")
```
### Example 2: Color filters
Original | Sepia filter at 50% strength | Sepia filter at 100% strength
:---:|:---:|:---:
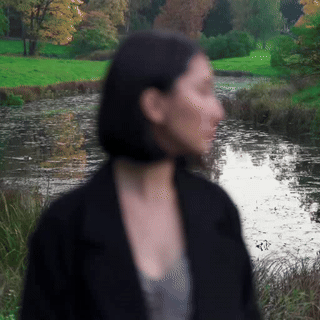 | 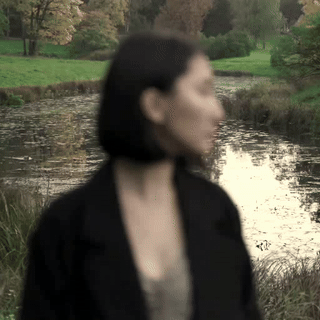 | 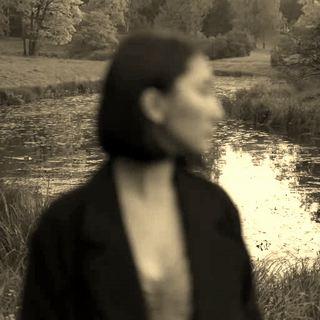
```
from video_filter import VideoFilter
vf = VideoFilter(filter_name="sepia", filter_strength=0.5)
vf.process_video("examples/video_2.mp4", "output.mp4")
```
### Example 3: Custom filter
Original | Custom filter
:-------------------------:|:-------------------------:
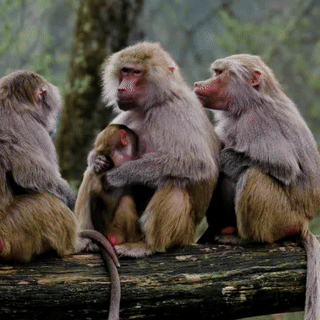 | 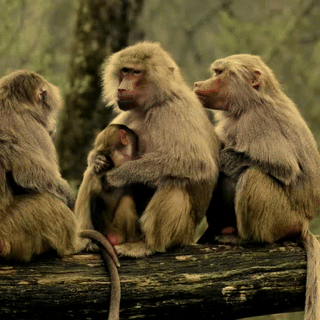
```
from video_filter import VideoFilter
import numpy as np
# Matrix (3x3) vector (3x1) [b, g, r]) multiplication
filter_matrix = np.array([
[0.7, 0.0, 0.1], # Blue
[0.2, 1.0, 0.3], # Green
[0.4, 0.2, 1.2] # Red
])
vf = VideoFilter(
custom_matrix=filter_matrix,
filter_strength=0.6,
brightness=0.8
)
vf.process_video("examples/video_1.mp4", "output.mp4")
```
Raw data
{
"_id": null,
"home_page": "https://github.com/merijnvanes/video-filter.git",
"name": "video-filter",
"maintainer": null,
"docs_url": null,
"requires_python": ">=3.7",
"maintainer_email": null,
"keywords": "video, filter, color, colour, color filter, colour filter, video filter, brightness, brightness filter, video brightness, mp4, video processing, video editor tools, short, shorts, reel, reels, social, social post, social posts, social media, social media post, social media posts, cool filter, warm filter, grayscale, grayscale filter",
"author": "Merijn van Es",
"author_email": "merijnvanes@gmail.com",
"download_url": "https://files.pythonhosted.org/packages/58/8a/3becf99e67f685e2a50d12ec4424f430bd5e964e47ac86413ac4b7d8de4d/video_filter-0.0.4.tar.gz",
"platform": null,
"description": "# Video Filter\n\nEnhance your video with color filters. This Python project helps\nyou to apply a color filter to your Instagram reel or any other social platforms\nlike TikTok, YouTube, Facebook, etc. All formats are supported (reels, shorts,\n4k, etc.). The output video is in mp4 format.\n\n## Filter gallery selection\n\noriginal | grayscale | warm |\n:---:|:---:|:---:\n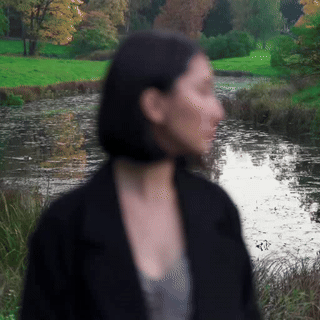 | 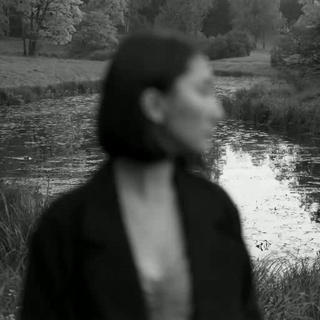 | 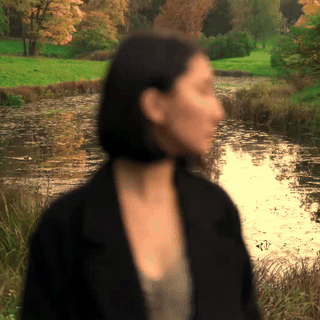\n\ncool | red_only | invert_red_green\n:---:|:---:|:---:\n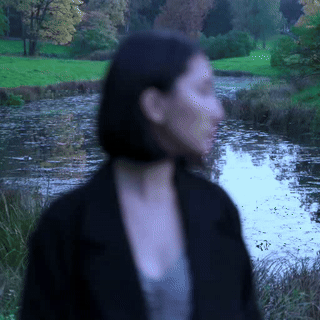 | 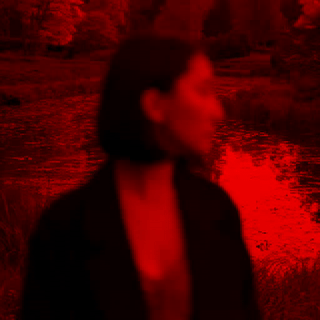 | \n\n## Installation\n\nRun the following command in the terminal to install the package:\n\n```\npip install video-filter\n```\n\n## Usage examples\n\n### Example 1: Brightness and saturation\n\nBrightness and saturation are both set to 0.0 by default, meaning the video \nwill be unchanged.\n\nx | Brightness -1.0 | Brightness 0.0 | Brightness +1.0\n:---:|:---:|:---:|:---:\n**Saturation -1.0** | |  | \n**Saturation 0.0** |  | 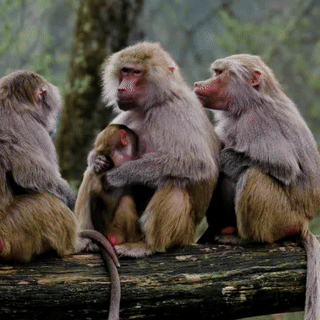 | \n**Saturation +1.0** | |  | \n\n```\nfrom video_filter import VideoFilter\n\nvf = VideoFilter(brightness=1.0, saturation=0.0)\nvf.process_video(\"examples/video_1.mp4\", \"output.mp4\")\n```\n\n### Example 2: Color filters\n\nOriginal | Sepia filter at 50% strength | Sepia filter at 100% strength\n:---:|:---:|:---:\n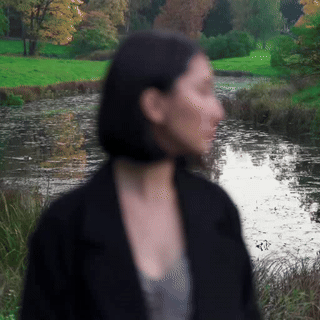 | 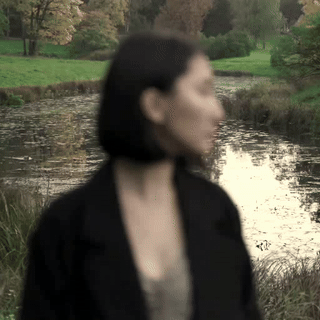 | 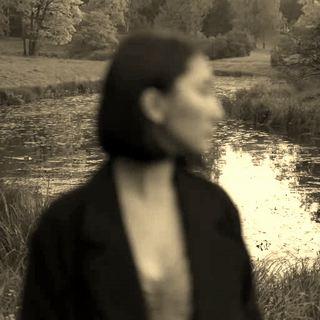\n\n```\nfrom video_filter import VideoFilter\n\nvf = VideoFilter(filter_name=\"sepia\", filter_strength=0.5)\nvf.process_video(\"examples/video_2.mp4\", \"output.mp4\")\n```\n\n### Example 3: Custom filter\n\nOriginal | Custom filter\n:-------------------------:|:-------------------------:\n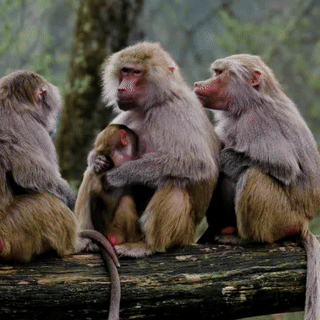 | 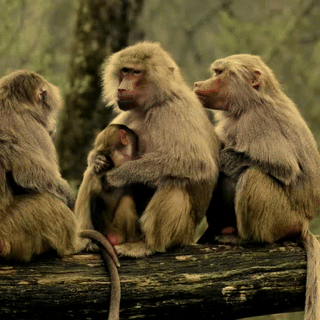\n\n```\nfrom video_filter import VideoFilter\nimport numpy as np\n\n# Matrix (3x3) vector (3x1) [b, g, r]) multiplication\nfilter_matrix = np.array([\n [0.7, 0.0, 0.1], # Blue\n [0.2, 1.0, 0.3], # Green\n [0.4, 0.2, 1.2] # Red\n])\n\nvf = VideoFilter(\n custom_matrix=filter_matrix,\n filter_strength=0.6,\n brightness=0.8\n)\nvf.process_video(\"examples/video_1.mp4\", \"output.mp4\")\n```\n",
"bugtrack_url": null,
"license": null,
"summary": "Enhance your video with filters.",
"version": "0.0.4",
"project_urls": {
"Homepage": "https://github.com/merijnvanes/video-filter.git"
},
"split_keywords": [
"video",
" filter",
" color",
" colour",
" color filter",
" colour filter",
" video filter",
" brightness",
" brightness filter",
" video brightness",
" mp4",
" video processing",
" video editor tools",
" short",
" shorts",
" reel",
" reels",
" social",
" social post",
" social posts",
" social media",
" social media post",
" social media posts",
" cool filter",
" warm filter",
" grayscale",
" grayscale filter"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "5eabd8c52866f52b3477261a5192af3af2b39f4c03771dbbe3c1a66c23510b85",
"md5": "2a6324aa1fd549637e5a8af38f70d682",
"sha256": "97cea301457bcfcdb85862717d177f47bc3e63d6186aa8f88f6a3fe05927938d"
},
"downloads": -1,
"filename": "video_filter-0.0.4-py3-none-any.whl",
"has_sig": false,
"md5_digest": "2a6324aa1fd549637e5a8af38f70d682",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.7",
"size": 5010,
"upload_time": "2024-07-29T09:26:00",
"upload_time_iso_8601": "2024-07-29T09:26:00.068495Z",
"url": "https://files.pythonhosted.org/packages/5e/ab/d8c52866f52b3477261a5192af3af2b39f4c03771dbbe3c1a66c23510b85/video_filter-0.0.4-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "588a3becf99e67f685e2a50d12ec4424f430bd5e964e47ac86413ac4b7d8de4d",
"md5": "4e7e3fcd769c0aec82cf62e82fb76839",
"sha256": "3b744b19462719fe3a000a1803be7524403c94362ad131e62a23fe883d4a330f"
},
"downloads": -1,
"filename": "video_filter-0.0.4.tar.gz",
"has_sig": false,
"md5_digest": "4e7e3fcd769c0aec82cf62e82fb76839",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.7",
"size": 4751,
"upload_time": "2024-07-29T09:26:01",
"upload_time_iso_8601": "2024-07-29T09:26:01.270822Z",
"url": "https://files.pythonhosted.org/packages/58/8a/3becf99e67f685e2a50d12ec4424f430bd5e964e47ac86413ac4b7d8de4d/video_filter-0.0.4.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-07-29 09:26:01",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "merijnvanes",
"github_project": "video-filter",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"requirements": [],
"lcname": "video-filter"
}