# Wagtail Highlight.js
Wagtail Highlight.js is a syntax highlighter block for source code for the Wagtail CMS.
It features real-time highlighting in the Wagtail editor, the front end, and support for
[Highlight.js themes](https://highlightjs.org/demo).
It uses the [Highlight.js](https://highlightjs.org/) library both in Wagtail Admin and the website.
## Example Usage
First, add `wagtail_hljs` to your `INSTALLED_APPS` in Django's settings. Here's a bare bones example:
```python
from wagtail.blocks import TextBlock
from wagtail.fields import StreamField
from wagtail.models import Page
from wagtail.admin.panels import FieldPanel
from wagtail_hljs.blocks import CodeBlock
class HomePage(Page):
body = StreamField([
("heading", TextBlock()),
("code", CodeBlock(label='Code')),
])
content_panels = Page.content_panels + [
FieldPanel("body"),
]
```
You can also force it to use a single language or set a default language by providing a language code which must be included in your `WAGTAIL_HLJS_LANGUAGES` setting:
```python
bash_code = CodeBlock(label='Bash Code', language='bash')
any_code = CodeBlock(label='Any code', default_language='python')
```
## Screenshot of the CMS Editor Interface
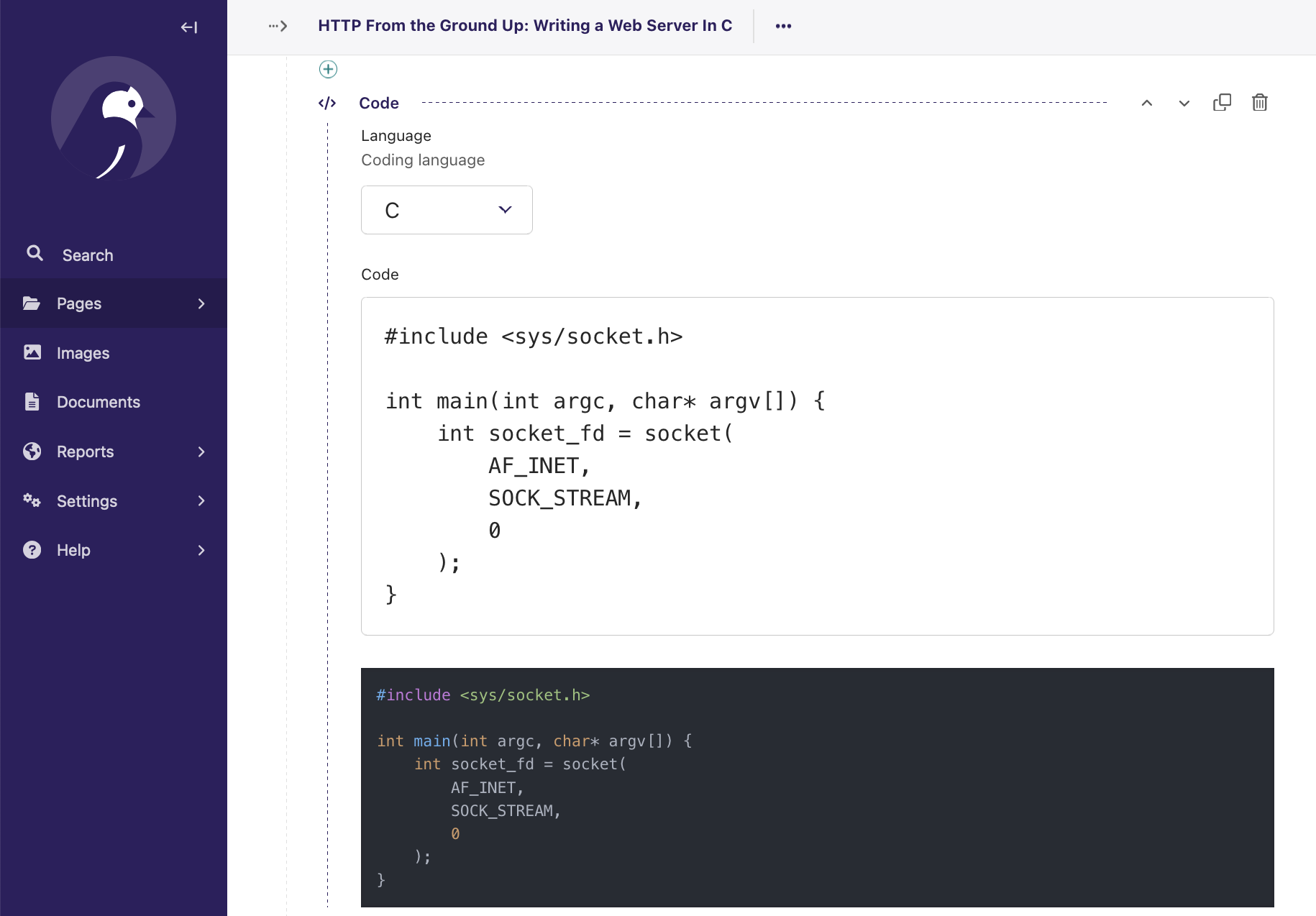
## Installation & Setup
To install Wagtail Highlight.js run:
```bash
# Wagtail 4.0 and greater
pip install wagtail-hljs
```
And add `wagtail_hljs` to your `INSTALLED_APPS` setting:
```python
INSTALLED_APPS = [
...
'wagtail_hljs',
...
]
```
## Django Settings
### Themes
Wagtail Highlight.js supports all themes that Highlight.js supports. Here are a few of them:
* **None**: <a href="https://highlightjs.org/demo#lang=&v=1&theme=base16-darcula&code=ZGVmIG1haW4oKToKCXByaW50KCdoZWxsbyB3b3JsZCcpCgppZiBfX25hbWVfXyA9PSAnX1%2FEMV9fJzoKCcY7" target="_blank">Default</a>
* **'atom-one-dark'**: <a href="https://highlightjs.org/demo#lang=&v=1&theme=atom-one-dark&code=ZGVmIG1haW4oKToKCXByaW50KCdoZWxsbyB3b3JsZCcpCgppZiBfX25hbWVfXyA9PSAnX1%2FEMV9fJzoKCcY7" target="_blank">Atom One Dark</a>
* **'base16/darcula'**: <a href="https://highlightjs.org/demo#lang=&v=1&theme=base16-darcula&code=ZGVmIG1haW4oKToKCXByaW50KCdoZWxsbyB3b3JsZCcpCgppZiBfX25hbWVfXyA9PSAnX1%2FEMV9fJzoKCcY7" target="_blank">Base 16 Darcula</a>
* **'nord'**: <a href="https://highlightjs.org/demo#lang=&v=1&theme=nord&code=ZGVmIG1haW4oKToKCXByaW50KCdoZWxsbyB3b3JsZCcpCgppZiBfX25hbWVfXyA9PSAnX1%2FEMV9fJzoKCcY7" target="_blank">Nord</a>
* **'srcery'**: <a href="https://highlightjs.org/demo#lang=&v=1&theme=srcery&code=ZGVmIG1haW4oKToKCXByaW50KCdoZWxsbyB3b3JsZCcpCgppZiBfX25hbWVfXyA9PSAnX1%2FEMV9fJzoKCcY7" target="_blank">Srcery</a>
* **'xt256'**: <a href="https://highlightjs.org/demo#lang=&v=1&theme=xt256&code=ZGVmIG1haW4oKToKCXByaW50KCdoZWxsbyB3b3JsZCcpCgppZiBfX25hbWVfXyA9PSAnX1%2FEMV9fJzoKCcY7" target="_blank">XT256</a>
* **'night-owl'**: <a href="https://highlightjs.org/demo#lang=&v=1&theme=night-owl&code=ZGVmIG1haW4oKToKCXByaW50KCdoZWxsbyB3b3JsZCcpCgppZiBfX25hbWVfXyA9PSAnX1%2FEMV9fJzoKCcY7" target="_blank">Night Owl</a>
For example, if you want to use the Night Owl theme: `WAGTAIL_HLJS_THEME = 'night-owl'`.
However, if you want the default theme then do this: `WAGTAIL_HLJS_THEME=None`.
### Languages Available
You can customize the languages available by configuring `WAGTAIL_HLJS_LANGUAGES` in your Django settings.
By default, it will be set with these languages, since most users are in the Python web development community:
```python
WAGTAIL_HLJS_LANGUAGES = (
('bash', 'Bash/Shell'),
('css', 'CSS'),
('diff', 'diff'),
('html', 'HTML'),
('javascript', 'Javascript'),
('json', 'JSON'),
('python', 'Python'),
('scss', 'SCSS'),
('yaml', 'YAML'),
)
```
Each language in this setting is a tuple of the Highlight.js code and a descriptive label.
If you want use all available languages, here is a list:
```python
WAGTAIL_CODE_BLOCK_LANGUAGES = (
('abap', 'ABAP'),
('abnf', 'Augmented Backus–Naur form'),
('actionscript', 'ActionScript'),
('ada', 'Ada'),
('antlr4', 'ANTLR4'),
('apacheconf', 'Apache Configuration'),
('apl', 'APL'),
('applescript', 'AppleScript'),
('aql', 'AQL'),
('arduino', 'Arduino'),
('arff', 'ARFF'),
('asciidoc', 'AsciiDoc'),
('asm6502', '6502 Assembly'),
('aspnet', 'ASP.NET (C#)'),
('autohotkey', 'AutoHotkey'),
('autoit', 'AutoIt'),
('bash', 'Bash + Shell'),
('basic', 'BASIC'),
('batch', 'Batch'),
('bison', 'Bison'),
('bnf', 'Backus–Naur form + Routing Backus–Naur form'),
('brainfuck', 'Brainfuck'),
('bro', 'Bro'),
('c', 'C'),
('clike', 'C-like'),
('cmake', 'CMake'),
('csharp', 'C#'),
('cpp', 'C++'),
('cil', 'CIL'),
('coffeescript', 'CoffeeScript'),
('clojure', 'Clojure'),
('crystal', 'Crystal'),
('csp', 'Content-Security-Policy'),
('css', 'CSS'),
('css-extras', 'CSS Extras'),
('d', 'D'),
('dart', 'Dart'),
('diff', 'Diff'),
('django', 'Django/Jinja2'),
('dns-zone-file', 'DNS Zone File'),
('docker', 'Docker'),
('ebnf', 'Extended Backus–Naur form'),
('eiffel', 'Eiffel'),
('ejs', 'EJS'),
('elixir', 'Elixir'),
('elm', 'Elm'),
('erb', 'ERB'),
('erlang', 'Erlang'),
('etlua', 'Embedded LUA Templating'),
('fsharp', 'F#'),
('flow', 'Flow'),
('fortran', 'Fortran'),
('ftl', 'Freemarker Template Language'),
('gcode', 'G-code'),
('gdscript', 'GDScript'),
('gedcom', 'GEDCOM'),
('gherkin', 'Gherkin'),
('git', 'Git'),
('glsl', 'GLSL'),
('gml', 'GameMaker Language'),
('go', 'Go'),
('graphql', 'GraphQL'),
('groovy', 'Groovy'),
('haml', 'Haml'),
('handlebars', 'Handlebars'),
('haskell', 'Haskell'),
('haxe', 'Haxe'),
('hcl', 'HCL'),
('http', 'HTTP'),
('hpkp', 'HTTP Public-Key-Pins'),
('hsts', 'HTTP Strict-Transport-Security'),
('ichigojam', 'IchigoJam'),
('icon', 'Icon'),
('inform7', 'Inform 7'),
('ini', 'Ini'),
('io', 'Io'),
('j', 'J'),
('java', 'Java'),
('javadoc', 'JavaDoc'),
('javadoclike', 'JavaDoc-like'),
('javascript', 'JavaScript'),
('javastacktrace', 'Java stack trace'),
('jolie', 'Jolie'),
('jq', 'JQ'),
('jsdoc', 'JSDoc'),
('js-extras', 'JS Extras'),
('js-templates', 'JS Templates'),
('json', 'JSON'),
('jsonp', 'JSONP'),
('json5', 'JSON5'),
('julia', 'Julia'),
('keyman', 'Keyman'),
('kotlin', 'Kotlin'),
('latex', 'LaTeX'),
('less', 'Less'),
('lilypond', 'Lilypond'),
('liquid', 'Liquid'),
('lisp', 'Lisp'),
('livescript', 'LiveScript'),
('lolcode', 'LOLCODE'),
('lua', 'Lua'),
('makefile', 'Makefile'),
('markdown', 'Markdown'),
('markup', 'Markup + HTML + XML + SVG + MathML'),
('markup-templating', 'Markup templating'),
('matlab', 'MATLAB'),
('mel', 'MEL'),
('mizar', 'Mizar'),
('monkey', 'Monkey'),
('n1ql', 'N1QL'),
('n4js', 'N4JS'),
('nand2tetris-hdl', 'Nand To Tetris HDL'),
('nasm', 'NASM'),
('nginx', 'nginx'),
('nim', 'Nim'),
('nix', 'Nix'),
('nsis', 'NSIS'),
('objectivec', 'Objective-C'),
('ocaml', 'OCaml'),
('opencl', 'OpenCL'),
('oz', 'Oz'),
('parigp', 'PARI/GP'),
('parser', 'Parser'),
('pascal', 'Pascal + Object Pascal'),
('pascaligo', 'Pascaligo'),
('pcaxis', 'PC Axis'),
('perl', 'Perl'),
('php', 'PHP'),
('phpdoc', 'PHPDoc'),
('php-extras', 'PHP Extras'),
('plsql', 'PL/SQL'),
('powershell', 'PowerShell'),
('processing', 'Processing'),
('prolog', 'Prolog'),
('properties', '.properties'),
('protobuf', 'Protocol Buffers'),
('pug', 'Pug'),
('puppet', 'Puppet'),
('pure', 'Pure'),
('python', 'Python'),
('q', 'Q (kdb+ database)'),
('qore', 'Qore'),
('r', 'R'),
('jsx', 'React JSX'),
('tsx', 'React TSX'),
('renpy', 'Ren\'py'),
('reason', 'Reason'),
('regex', 'Regex'),
('rest', 'reST (reStructuredText)'),
('rip', 'Rip'),
('roboconf', 'Roboconf'),
('robot-framework', 'Robot Framework'),
('ruby', 'Ruby'),
('rust', 'Rust'),
('sas', 'SAS'),
('sass', 'Sass (Sass)'),
('scss', 'Sass (Scss)'),
('scala', 'Scala'),
('scheme', 'Scheme'),
('shell-session', 'Shell Session'),
('smalltalk', 'Smalltalk'),
('smarty', 'Smarty'),
('solidity', 'Solidity (Ethereum)'),
('sparql', 'SPARQL'),
('splunk-spl', 'Splunk SPL'),
('sqf', 'SQF: Status Quo Function (Arma 3)'),
('sql', 'SQL'),
('soy', 'Soy (Closure Template)'),
('stylus', 'Stylus'),
('swift', 'Swift'),
('tap', 'TAP'),
('tcl', 'Tcl'),
('textile', 'Textile'),
('toml', 'TOML'),
('tt2', 'Template Toolkit 2'),
('twig', 'Twig'),
('typescript', 'TypeScript'),
('t4-cs', 'T4 Text Templates (C#)'),
('t4-vb', 'T4 Text Templates (VB)'),
('t4-templating', 'T4 templating'),
('vala', 'Vala'),
('vbnet', 'VB.Net'),
('velocity', 'Velocity'),
('verilog', 'Verilog'),
('vhdl', 'VHDL'),
('vim', 'vim'),
('visual-basic', 'Visual Basic'),
('wasm', 'WebAssembly'),
('wiki', 'Wiki markup'),
('xeora', 'Xeora + XeoraCube'),
('xojo', 'Xojo (REALbasic)'),
('xquery', 'XQuery'),
('yaml', 'YAML'),
('zig', 'Zig'),
)
```
# Running the Test Suite
Clone the repository, create a `venv`, `pip install -e .[dev]` and run `pytest`.
# Release Notes & Contributors
* This is fork of [wagtailcodeblock](https://github.com/FlipperPA/wagtailcodeblock/).
# Project Maintainers
* John Higgins (https://github.com/johnmatthiggins)
Raw data
{
"_id": null,
"home_page": null,
"name": "wagtail-hljs",
"maintainer": null,
"docs_url": null,
"requires_python": "<4.0,>=3.11",
"maintainer_email": null,
"keywords": "wagtail, cms, contact, syntax, code, highlighting, highlighter, highlight.js",
"author": "John Higgins",
"author_email": "johnmatthiggins@gmail.com",
"download_url": "https://files.pythonhosted.org/packages/38/21/33e71a0968b4b2403d4bd70e41d301d8d9674ad4351bd92b2062cbb8a7d0/wagtail_hljs-0.1.3.tar.gz",
"platform": null,
"description": "# Wagtail Highlight.js\n\nWagtail Highlight.js is a syntax highlighter block for source code for the Wagtail CMS.\nIt features real-time highlighting in the Wagtail editor, the front end, and support for\n[Highlight.js themes](https://highlightjs.org/demo).\n\nIt uses the [Highlight.js](https://highlightjs.org/) library both in Wagtail Admin and the website.\n\n## Example Usage\n\nFirst, add `wagtail_hljs` to your `INSTALLED_APPS` in Django's settings. Here's a bare bones example:\n\n```python\nfrom wagtail.blocks import TextBlock\nfrom wagtail.fields import StreamField\nfrom wagtail.models import Page\nfrom wagtail.admin.panels import FieldPanel\n\nfrom wagtail_hljs.blocks import CodeBlock\n\n\nclass HomePage(Page):\n body = StreamField([\n (\"heading\", TextBlock()),\n (\"code\", CodeBlock(label='Code')),\n ])\n\n content_panels = Page.content_panels + [\n FieldPanel(\"body\"),\n ]\n```\n\nYou can also force it to use a single language or set a default language by providing a language code which must be included in your `WAGTAIL_HLJS_LANGUAGES` setting:\n\n```python\nbash_code = CodeBlock(label='Bash Code', language='bash')\nany_code = CodeBlock(label='Any code', default_language='python')\n```\n\n## Screenshot of the CMS Editor Interface\n\n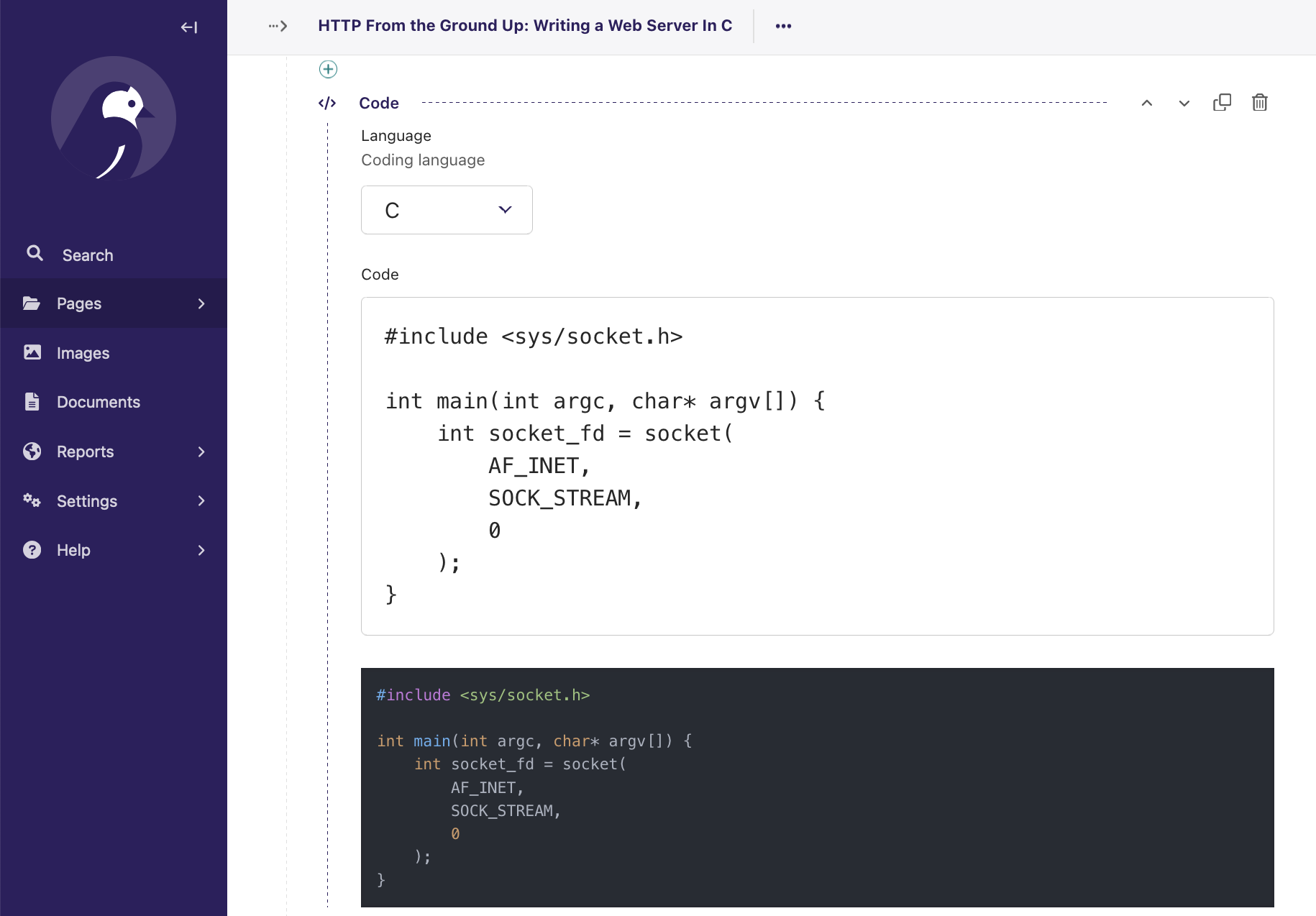\n\n## Installation & Setup\n\nTo install Wagtail Highlight.js run:\n\n```bash\n# Wagtail 4.0 and greater\npip install wagtail-hljs\n```\n\nAnd add `wagtail_hljs` to your `INSTALLED_APPS` setting:\n\n```python\nINSTALLED_APPS = [\n ...\n 'wagtail_hljs',\n ...\n]\n```\n\n## Django Settings\n\n### Themes\n\nWagtail Highlight.js supports all themes that Highlight.js supports. Here are a few of them:\n\n* **None**: <a href=\"https://highlightjs.org/demo#lang=&v=1&theme=base16-darcula&code=ZGVmIG1haW4oKToKCXByaW50KCdoZWxsbyB3b3JsZCcpCgppZiBfX25hbWVfXyA9PSAnX1%2FEMV9fJzoKCcY7\" target=\"_blank\">Default</a>\n* **'atom-one-dark'**: <a href=\"https://highlightjs.org/demo#lang=&v=1&theme=atom-one-dark&code=ZGVmIG1haW4oKToKCXByaW50KCdoZWxsbyB3b3JsZCcpCgppZiBfX25hbWVfXyA9PSAnX1%2FEMV9fJzoKCcY7\" target=\"_blank\">Atom One Dark</a>\n* **'base16/darcula'**: <a href=\"https://highlightjs.org/demo#lang=&v=1&theme=base16-darcula&code=ZGVmIG1haW4oKToKCXByaW50KCdoZWxsbyB3b3JsZCcpCgppZiBfX25hbWVfXyA9PSAnX1%2FEMV9fJzoKCcY7\" target=\"_blank\">Base 16 Darcula</a>\n* **'nord'**: <a href=\"https://highlightjs.org/demo#lang=&v=1&theme=nord&code=ZGVmIG1haW4oKToKCXByaW50KCdoZWxsbyB3b3JsZCcpCgppZiBfX25hbWVfXyA9PSAnX1%2FEMV9fJzoKCcY7\" target=\"_blank\">Nord</a>\n* **'srcery'**: <a href=\"https://highlightjs.org/demo#lang=&v=1&theme=srcery&code=ZGVmIG1haW4oKToKCXByaW50KCdoZWxsbyB3b3JsZCcpCgppZiBfX25hbWVfXyA9PSAnX1%2FEMV9fJzoKCcY7\" target=\"_blank\">Srcery</a>\n* **'xt256'**: <a href=\"https://highlightjs.org/demo#lang=&v=1&theme=xt256&code=ZGVmIG1haW4oKToKCXByaW50KCdoZWxsbyB3b3JsZCcpCgppZiBfX25hbWVfXyA9PSAnX1%2FEMV9fJzoKCcY7\" target=\"_blank\">XT256</a>\n* **'night-owl'**: <a href=\"https://highlightjs.org/demo#lang=&v=1&theme=night-owl&code=ZGVmIG1haW4oKToKCXByaW50KCdoZWxsbyB3b3JsZCcpCgppZiBfX25hbWVfXyA9PSAnX1%2FEMV9fJzoKCcY7\" target=\"_blank\">Night Owl</a>\n\nFor example, if you want to use the Night Owl theme: `WAGTAIL_HLJS_THEME = 'night-owl'`.\nHowever, if you want the default theme then do this: `WAGTAIL_HLJS_THEME=None`.\n\n### Languages Available\n\nYou can customize the languages available by configuring `WAGTAIL_HLJS_LANGUAGES` in your Django settings.\nBy default, it will be set with these languages, since most users are in the Python web development community:\n\n```python\nWAGTAIL_HLJS_LANGUAGES = (\n ('bash', 'Bash/Shell'),\n ('css', 'CSS'),\n ('diff', 'diff'),\n ('html', 'HTML'),\n ('javascript', 'Javascript'),\n ('json', 'JSON'),\n ('python', 'Python'),\n ('scss', 'SCSS'),\n ('yaml', 'YAML'),\n)\n```\n\nEach language in this setting is a tuple of the Highlight.js code and a descriptive label.\nIf you want use all available languages, here is a list:\n\n```python\nWAGTAIL_CODE_BLOCK_LANGUAGES = (\n ('abap', 'ABAP'),\n ('abnf', 'Augmented Backus\u2013Naur form'),\n ('actionscript', 'ActionScript'),\n ('ada', 'Ada'),\n ('antlr4', 'ANTLR4'),\n ('apacheconf', 'Apache Configuration'),\n ('apl', 'APL'),\n ('applescript', 'AppleScript'),\n ('aql', 'AQL'),\n ('arduino', 'Arduino'),\n ('arff', 'ARFF'),\n ('asciidoc', 'AsciiDoc'),\n ('asm6502', '6502 Assembly'),\n ('aspnet', 'ASP.NET (C#)'),\n ('autohotkey', 'AutoHotkey'),\n ('autoit', 'AutoIt'),\n ('bash', 'Bash + Shell'),\n ('basic', 'BASIC'),\n ('batch', 'Batch'),\n ('bison', 'Bison'),\n ('bnf', 'Backus\u2013Naur form + Routing Backus\u2013Naur form'),\n ('brainfuck', 'Brainfuck'),\n ('bro', 'Bro'),\n ('c', 'C'),\n ('clike', 'C-like'),\n ('cmake', 'CMake'),\n ('csharp', 'C#'),\n ('cpp', 'C++'),\n ('cil', 'CIL'),\n ('coffeescript', 'CoffeeScript'),\n ('clojure', 'Clojure'),\n ('crystal', 'Crystal'),\n ('csp', 'Content-Security-Policy'),\n ('css', 'CSS'),\n ('css-extras', 'CSS Extras'),\n ('d', 'D'),\n ('dart', 'Dart'),\n ('diff', 'Diff'),\n ('django', 'Django/Jinja2'),\n ('dns-zone-file', 'DNS Zone File'),\n ('docker', 'Docker'),\n ('ebnf', 'Extended Backus\u2013Naur form'),\n ('eiffel', 'Eiffel'),\n ('ejs', 'EJS'),\n ('elixir', 'Elixir'),\n ('elm', 'Elm'),\n ('erb', 'ERB'),\n ('erlang', 'Erlang'),\n ('etlua', 'Embedded LUA Templating'),\n ('fsharp', 'F#'),\n ('flow', 'Flow'),\n ('fortran', 'Fortran'),\n ('ftl', 'Freemarker Template Language'),\n ('gcode', 'G-code'),\n ('gdscript', 'GDScript'),\n ('gedcom', 'GEDCOM'),\n ('gherkin', 'Gherkin'),\n ('git', 'Git'),\n ('glsl', 'GLSL'),\n ('gml', 'GameMaker Language'),\n ('go', 'Go'),\n ('graphql', 'GraphQL'),\n ('groovy', 'Groovy'),\n ('haml', 'Haml'),\n ('handlebars', 'Handlebars'),\n ('haskell', 'Haskell'),\n ('haxe', 'Haxe'),\n ('hcl', 'HCL'),\n ('http', 'HTTP'),\n ('hpkp', 'HTTP Public-Key-Pins'),\n ('hsts', 'HTTP Strict-Transport-Security'),\n ('ichigojam', 'IchigoJam'),\n ('icon', 'Icon'),\n ('inform7', 'Inform 7'),\n ('ini', 'Ini'),\n ('io', 'Io'),\n ('j', 'J'),\n ('java', 'Java'),\n ('javadoc', 'JavaDoc'),\n ('javadoclike', 'JavaDoc-like'),\n ('javascript', 'JavaScript'),\n ('javastacktrace', 'Java stack trace'),\n ('jolie', 'Jolie'),\n ('jq', 'JQ'),\n ('jsdoc', 'JSDoc'),\n ('js-extras', 'JS Extras'),\n ('js-templates', 'JS Templates'),\n ('json', 'JSON'),\n ('jsonp', 'JSONP'),\n ('json5', 'JSON5'),\n ('julia', 'Julia'),\n ('keyman', 'Keyman'),\n ('kotlin', 'Kotlin'),\n ('latex', 'LaTeX'),\n ('less', 'Less'),\n ('lilypond', 'Lilypond'),\n ('liquid', 'Liquid'),\n ('lisp', 'Lisp'),\n ('livescript', 'LiveScript'),\n ('lolcode', 'LOLCODE'),\n ('lua', 'Lua'),\n ('makefile', 'Makefile'),\n ('markdown', 'Markdown'),\n ('markup', 'Markup + HTML + XML + SVG + MathML'),\n ('markup-templating', 'Markup templating'),\n ('matlab', 'MATLAB'),\n ('mel', 'MEL'),\n ('mizar', 'Mizar'),\n ('monkey', 'Monkey'),\n ('n1ql', 'N1QL'),\n ('n4js', 'N4JS'),\n ('nand2tetris-hdl', 'Nand To Tetris HDL'),\n ('nasm', 'NASM'),\n ('nginx', 'nginx'),\n ('nim', 'Nim'),\n ('nix', 'Nix'),\n ('nsis', 'NSIS'),\n ('objectivec', 'Objective-C'),\n ('ocaml', 'OCaml'),\n ('opencl', 'OpenCL'),\n ('oz', 'Oz'),\n ('parigp', 'PARI/GP'),\n ('parser', 'Parser'),\n ('pascal', 'Pascal + Object Pascal'),\n ('pascaligo', 'Pascaligo'),\n ('pcaxis', 'PC Axis'),\n ('perl', 'Perl'),\n ('php', 'PHP'),\n ('phpdoc', 'PHPDoc'),\n ('php-extras', 'PHP Extras'),\n ('plsql', 'PL/SQL'),\n ('powershell', 'PowerShell'),\n ('processing', 'Processing'),\n ('prolog', 'Prolog'),\n ('properties', '.properties'),\n ('protobuf', 'Protocol Buffers'),\n ('pug', 'Pug'),\n ('puppet', 'Puppet'),\n ('pure', 'Pure'),\n ('python', 'Python'),\n ('q', 'Q (kdb+ database)'),\n ('qore', 'Qore'),\n ('r', 'R'),\n ('jsx', 'React JSX'),\n ('tsx', 'React TSX'),\n ('renpy', 'Ren\\'py'),\n ('reason', 'Reason'),\n ('regex', 'Regex'),\n ('rest', 'reST (reStructuredText)'),\n ('rip', 'Rip'),\n ('roboconf', 'Roboconf'),\n ('robot-framework', 'Robot Framework'),\n ('ruby', 'Ruby'),\n ('rust', 'Rust'),\n ('sas', 'SAS'),\n ('sass', 'Sass (Sass)'),\n ('scss', 'Sass (Scss)'),\n ('scala', 'Scala'),\n ('scheme', 'Scheme'),\n ('shell-session', 'Shell Session'),\n ('smalltalk', 'Smalltalk'),\n ('smarty', 'Smarty'),\n ('solidity', 'Solidity (Ethereum)'),\n ('sparql', 'SPARQL'),\n ('splunk-spl', 'Splunk SPL'),\n ('sqf', 'SQF: Status Quo Function (Arma 3)'),\n ('sql', 'SQL'),\n ('soy', 'Soy (Closure Template)'),\n ('stylus', 'Stylus'),\n ('swift', 'Swift'),\n ('tap', 'TAP'),\n ('tcl', 'Tcl'),\n ('textile', 'Textile'),\n ('toml', 'TOML'),\n ('tt2', 'Template Toolkit 2'),\n ('twig', 'Twig'),\n ('typescript', 'TypeScript'),\n ('t4-cs', 'T4 Text Templates (C#)'),\n ('t4-vb', 'T4 Text Templates (VB)'),\n ('t4-templating', 'T4 templating'),\n ('vala', 'Vala'),\n ('vbnet', 'VB.Net'),\n ('velocity', 'Velocity'),\n ('verilog', 'Verilog'),\n ('vhdl', 'VHDL'),\n ('vim', 'vim'),\n ('visual-basic', 'Visual Basic'),\n ('wasm', 'WebAssembly'),\n ('wiki', 'Wiki markup'),\n ('xeora', 'Xeora + XeoraCube'),\n ('xojo', 'Xojo (REALbasic)'),\n ('xquery', 'XQuery'),\n ('yaml', 'YAML'),\n ('zig', 'Zig'),\n)\n```\n\n# Running the Test Suite\n\nClone the repository, create a `venv`, `pip install -e .[dev]` and run `pytest`.\n\n# Release Notes & Contributors\n\n* This is fork of [wagtailcodeblock](https://github.com/FlipperPA/wagtailcodeblock/).\n\n# Project Maintainers\n\n* John Higgins (https://github.com/johnmatthiggins)\n",
"bugtrack_url": null,
"license": "BSD-3-Clause",
"summary": "StreamField code blocks for the Wagtail CMS with real-time Highlight.js Syntax Highlighting.",
"version": "0.1.3",
"project_urls": null,
"split_keywords": [
"wagtail",
" cms",
" contact",
" syntax",
" code",
" highlighting",
" highlighter",
" highlight.js"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "badc7d07604bcd1f84976924e84303dee2fd8ee51081b4868ff3072e096dd4b0",
"md5": "f49e32df54a29547411b800534728f5e",
"sha256": "89fd6a7959ce5937539ccf44fb92179ebf77d9cb0e5a01a3d906624607b83399"
},
"downloads": -1,
"filename": "wagtail_hljs-0.1.3-py3-none-any.whl",
"has_sig": false,
"md5_digest": "f49e32df54a29547411b800534728f5e",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": "<4.0,>=3.11",
"size": 12818,
"upload_time": "2025-01-02T00:01:05",
"upload_time_iso_8601": "2025-01-02T00:01:05.854473Z",
"url": "https://files.pythonhosted.org/packages/ba/dc/7d07604bcd1f84976924e84303dee2fd8ee51081b4868ff3072e096dd4b0/wagtail_hljs-0.1.3-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "382133e71a0968b4b2403d4bd70e41d301d8d9674ad4351bd92b2062cbb8a7d0",
"md5": "0c0a1861fded9e20a92a915d112e4d70",
"sha256": "a1c9406198cfff3289bec3e8c59261cf853e6f95e98e7654a4d261a2c4faeed0"
},
"downloads": -1,
"filename": "wagtail_hljs-0.1.3.tar.gz",
"has_sig": false,
"md5_digest": "0c0a1861fded9e20a92a915d112e4d70",
"packagetype": "sdist",
"python_version": "source",
"requires_python": "<4.0,>=3.11",
"size": 13485,
"upload_time": "2025-01-02T00:01:08",
"upload_time_iso_8601": "2025-01-02T00:01:08.105352Z",
"url": "https://files.pythonhosted.org/packages/38/21/33e71a0968b4b2403d4bd70e41d301d8d9674ad4351bd92b2062cbb8a7d0/wagtail_hljs-0.1.3.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2025-01-02 00:01:08",
"github": false,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"lcname": "wagtail-hljs"
}