[](https://badge.fury.io/py/win11toast)
[](https://badge.fury.io/py/win11toast)
# win11toast
Toast notifications for Windows 10 and 11 based on [WinRT](https://docs.microsoft.com/en-us/windows/apps/design/shell/tiles-and-notifications/adaptive-interactive-toasts)
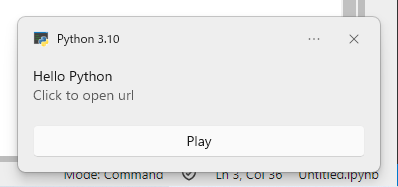
## Installation
```bash
pip install win11toast
```
## Usage
```python
from win11toast import toast
toast('Hello Python🐍')
```
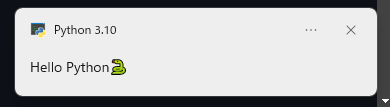
https://docs.microsoft.com/en-us/uwp/schemas/tiles/toastschema/element-text
### Body
```python
from win11toast import toast
toast('Hello Python', 'Click to open url', on_click='https://www.python.org')
```
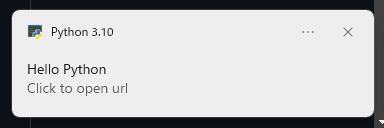
### Wrap text
```python
from win11toast import toast
toast('Hello', 'Lorem ipsum dolor sit amet, consectetur adipisicing elit. Earum accusantium porro numquam aspernatur voluptates cum, odio in, animi nihil cupiditate molestias laborum. Consequatur exercitationem modi vitae. In voluptates quia obcaecati!')
```
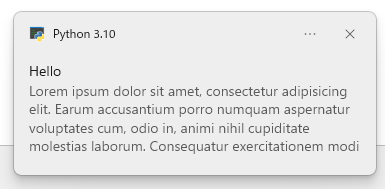
### Run Python script on Click
```python
from win11toast import toast
toast('Hello Pythonista', 'Click to run python script', on_click=r'C:\Users\Admin\Downloads\handler.pyw')
# {'arguments': 'C:\\Users\\Admin\\Downloads\\handler.pyw', 'user_input': {}}
```
Since the current directory when executing the script is `C:\Windows\system32`, use `os.chdir()` accordingly.
e.g. [handler.pyw](https://gist.github.com/GitHub30/dae1b257c93d8315ea38554c9554a2ad)
On Windows, you can run a Python script in the background using the pythonw.exe executable, which will run your program with no visible process or way to interact with it.
https://stackoverflow.com/questions/9705982/pythonw-exe-or-python-exe
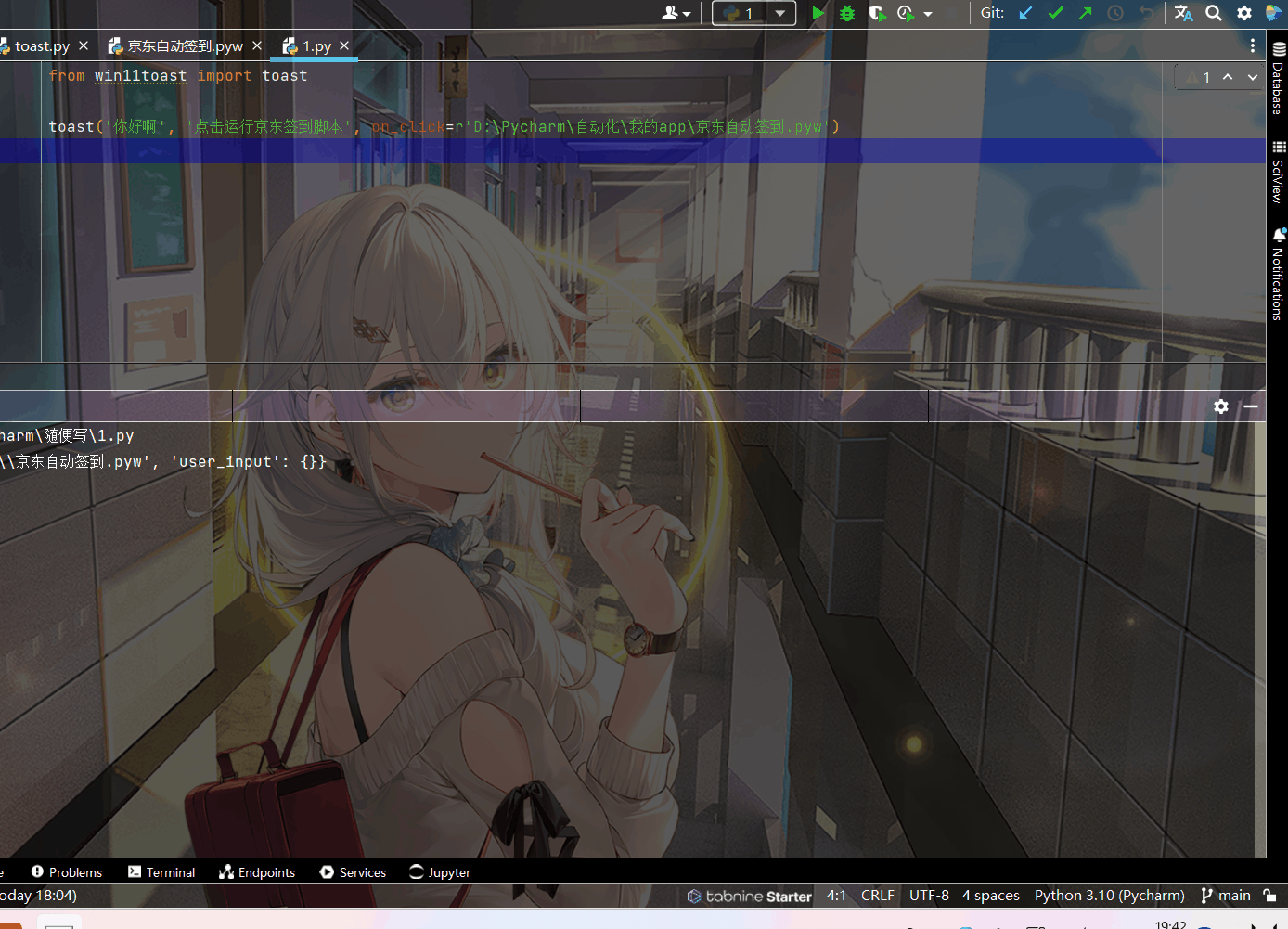
### Callback
```python
from win11toast import toast
toast('Hello Python', 'Click to open url', on_click=lambda args: print('clicked!', args))
# clicked! {'arguments': 'http:', 'user_input': {}}
```
### Icon
```python
from win11toast import toast
toast('Hello', 'Hello from Python', icon='https://unsplash.it/64?image=669')
```
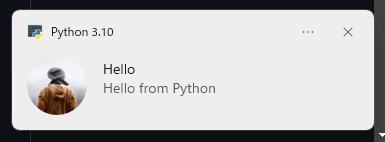
#### Square
```python
from win11toast import toast
icon = {
'src': 'https://unsplash.it/64?image=669',
'placement': 'appLogoOverride'
}
toast('Hello', 'Hello from Python', icon=icon)
```
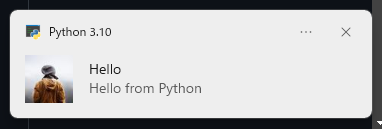
### Image
```python
from win11toast import toast
toast('Hello', 'Hello from Python', image='https://4.bp.blogspot.com/-u-uyq3FEqeY/UkJLl773BHI/AAAAAAAAYPQ/7bY05EeF1oI/s800/cooking_toaster.png')
```
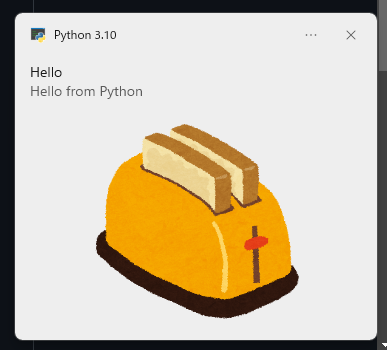
https://docs.microsoft.com/en-us/uwp/schemas/tiles/toastschema/element-image
#### Hero
```python
from win11toast import toast
image = {
'src': 'https://4.bp.blogspot.com/-u-uyq3FEqeY/UkJLl773BHI/AAAAAAAAYPQ/7bY05EeF1oI/s800/cooking_toaster.png',
'placement': 'hero'
}
toast('Hello', 'Hello from Python', image=image)
```
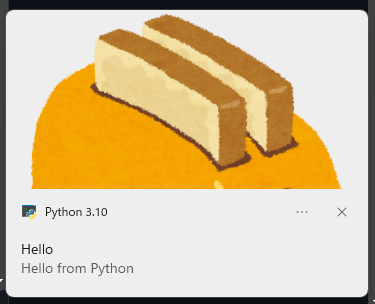
### Progress
```python
from time import sleep
from win11toast import notify, update_progress
notify(progress={
'title': 'YouTube',
'status': 'Downloading...',
'value': '0',
'valueStringOverride': '0/15 videos'
})
for i in range(1, 15+1):
sleep(1)
update_progress({'value': i/15, 'valueStringOverride': f'{i}/15 videos'})
update_progress({'status': 'Completed!'})
```
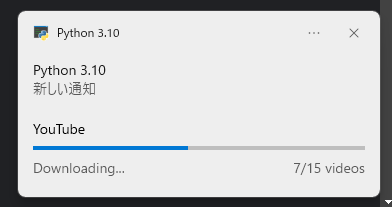
Attributes
https://docs.microsoft.com/en-ca/uwp/schemas/tiles/toastschema/element-progress
https://docs.microsoft.com/en-us/windows/apps/design/shell/tiles-and-notifications/toast-progress-bar
### Audio
```python
from win11toast import toast
toast('Hello', 'Hello from Python', audio='ms-winsoundevent:Notification.Looping.Alarm')
```
Available audio
https://docs.microsoft.com/en-us/uwp/schemas/tiles/toastschema/element-audio
##### From URL
```python
from win11toast import toast
toast('Hello', 'Hello from Python', audio='https://nyanpass.com/nyanpass.mp3')
```
##### From file
```python
from win11toast import toast
toast('Hello', 'Hello from Python', audio=r"C:\Users\Admin\Downloads\nyanpass.mp3")
```
I don't know how to add custom audio please help.
https://docs.microsoft.com/en-us/windows/apps/design/shell/tiles-and-notifications/custom-audio-on-toasts
#### Loop
```python
from win11toast import toast
toast('Hello', 'Hello from Python', audio={'loop': 'true'})
```
```python
from win11toast import toast
toast('Hello', 'Hello from Python', audio={'src': 'ms-winsoundevent:Notification.Looping.Alarm', 'loop': 'true'})
```
### Silent🤫
```python
from win11toast import toast
toast('Hello Python🐍', audio={'silent': 'true'})
```
### Speak🗣
```python
from win11toast import toast
toast('Hello Python🐍', dialogue='Hello world')
```
### OCR👀
```python
from win11toast import toast
toast(ocr='https://i.imgur.com/oYojrJW.png')
```
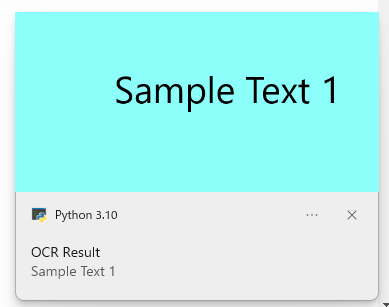
```python
from win11toast import toast
toast(ocr={'lang': 'ja', 'ocr': r'C:\Users\Admin\Downloads\hello.png'})
```
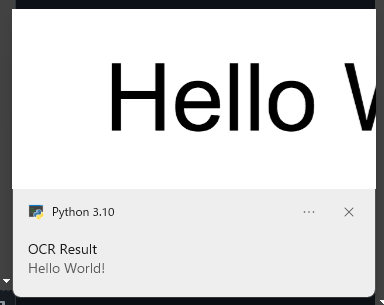
### Long duration
```python
from win11toast import toast
toast('Hello Python🐍', duration='long')
```
displayed for 25 seconds
https://docs.microsoft.com/en-us/uwp/schemas/tiles/toastschema/element-toast
### No timeout
```python
from win11toast import toast
toast('Hello Python🐍', scenario='incomingCall')
```
The scenario your toast is used for, like an alarm, reminder, incomingCall or urgent.
https://learn.microsoft.com/en-us/uwp/schemas/tiles/toastschema/element-toast#:~:text=None-,scenario,-The%20scenario%20your

### Button
```python
from win11toast import toast
toast('Hello', 'Hello from Python', button='Dismiss')
# {'arguments': 'http:Dismiss', 'user_input': {}}
```
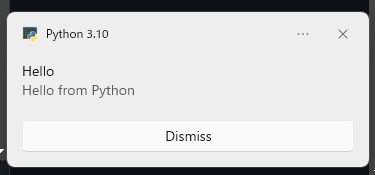
```python
from win11toast import toast
toast('Hello', 'Hello from Python', button={'activationType': 'protocol', 'arguments': 'https://google.com', 'content': 'Open Google'})
# {'arguments': 'https://google.com', 'user_input': {}}
```
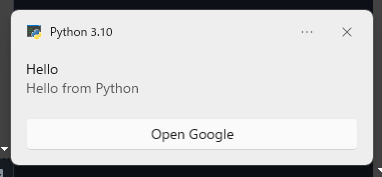
```python
from win11toast import toast
toast('Hello', 'Click a button', buttons=['Approve', 'Dismiss', 'Other'])
```
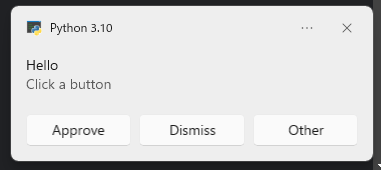
https://docs.microsoft.com/en-us/uwp/schemas/tiles/toastschema/element-action
#### Play music or Open Explorer
```python
from win11toast import toast
buttons = [
{'activationType': 'protocol', 'arguments': 'C:\Windows\Media\Alarm01.wav', 'content': 'Play'},
{'activationType': 'protocol', 'arguments': 'file:///C:/Windows/Media', 'content': 'Open Folder'}
]
toast('Music Player', 'Download Finished', buttons=buttons)
```
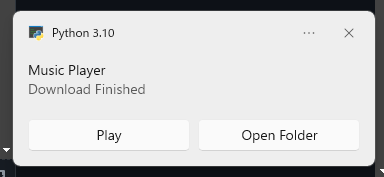
### Input
```python
from win11toast import toast
toast('Hello', 'Type anything', input='reply', button='Send')
# {'arguments': 'http:Send', 'user_input': {'reply': 'Hi there'}}
```
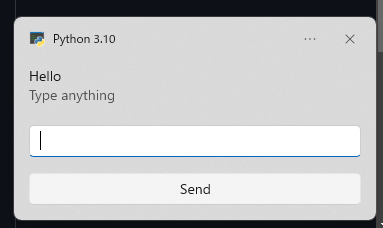
```python
from win11toast import toast
toast('Hello', 'Type anything', input='reply', button={'activationType': 'protocol', 'arguments': 'http:', 'content': 'Send', 'hint-inputId': 'reply'})
# {'arguments': 'http:', 'user_input': {'reply': 'Hi there'}}
```
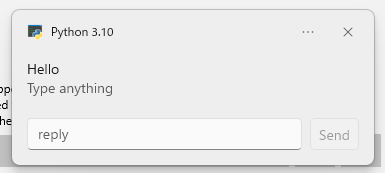
https://docs.microsoft.com/en-us/uwp/schemas/tiles/toastschema/element-input
### Selection
```python
from win11toast import toast
toast('Hello', 'Which do you like?', selection=['Apple', 'Banana', 'Grape'], button='Submit')
# {'arguments': 'dismiss', 'user_input': {'selection': 'Grape'}}
```
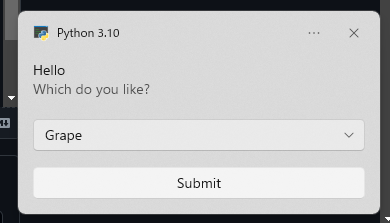
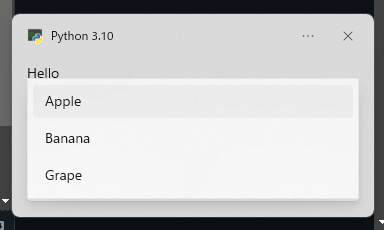
### No arguments
```python
from win11toast import toast
toast()
```
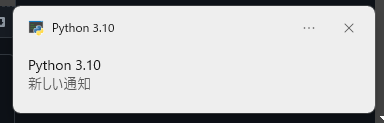
### Non blocking
```python
from win11toast import notify
notify('Hello Python', 'Click to open url', on_click='https://www.python.org')
```
### Async
```python
from win11toast import toast_async
async def main():
await toast_async('Hello Python', 'Click to open url', on_click='https://www.python.org')
```
### Jupyter
```python
from win11toast import notify
notify('Hello Python', 'Click to open url', on_click='https://www.python.org')
```

```python
from win11toast import toast_async
await toast_async('Hello Python', 'Click to open url', on_click='https://www.python.org')
```
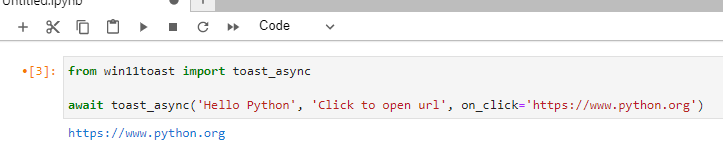
```python
import urllib.request
from pathlib import Path
src = str(Path(urllib.request.urlretrieve("https://i.imgur.com/p9dRdtP.jpg")[0]).absolute())
from win11toast import toast_async
await toast_async('にゃんぱすー', audio='https://nyanpass.com/nyanpass.mp3', image={'src': src, 'placement':'hero'})
```
```python
from win11toast import toast_async
await toast_async('Hello Python🐍', dialogue='にゃんぱすー')
```
## Debug
```python
from win11toast import toast
xml = """
<toast launch="action=openThread&threadId=92187">
<visual>
<binding template="ToastGeneric">
<text hint-maxLines="1">Jill Bender</text>
<text>Check out where we camped last weekend! It was incredible, wish you could have come on the backpacking trip!</text>
<image placement="appLogoOverride" hint-crop="circle" src="https://unsplash.it/64?image=1027"/>
<image placement="hero" src="https://unsplash.it/360/180?image=1043"/>
</binding>
</visual>
<actions>
<input id="textBox" type="text" placeHolderContent="reply"/>
<action
content="Send"
imageUri="Assets/Icons/send.png"
hint-inputId="textBox"
activationType="background"
arguments="action=reply&threadId=92187"/>
</actions>
</toast>"""
toast(xml=xml)
```
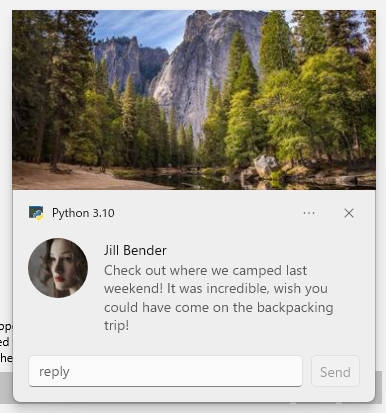
[Notifications Visualizer](https://www.microsoft.com/store/apps/notifications-visualizer/9nblggh5xsl1)
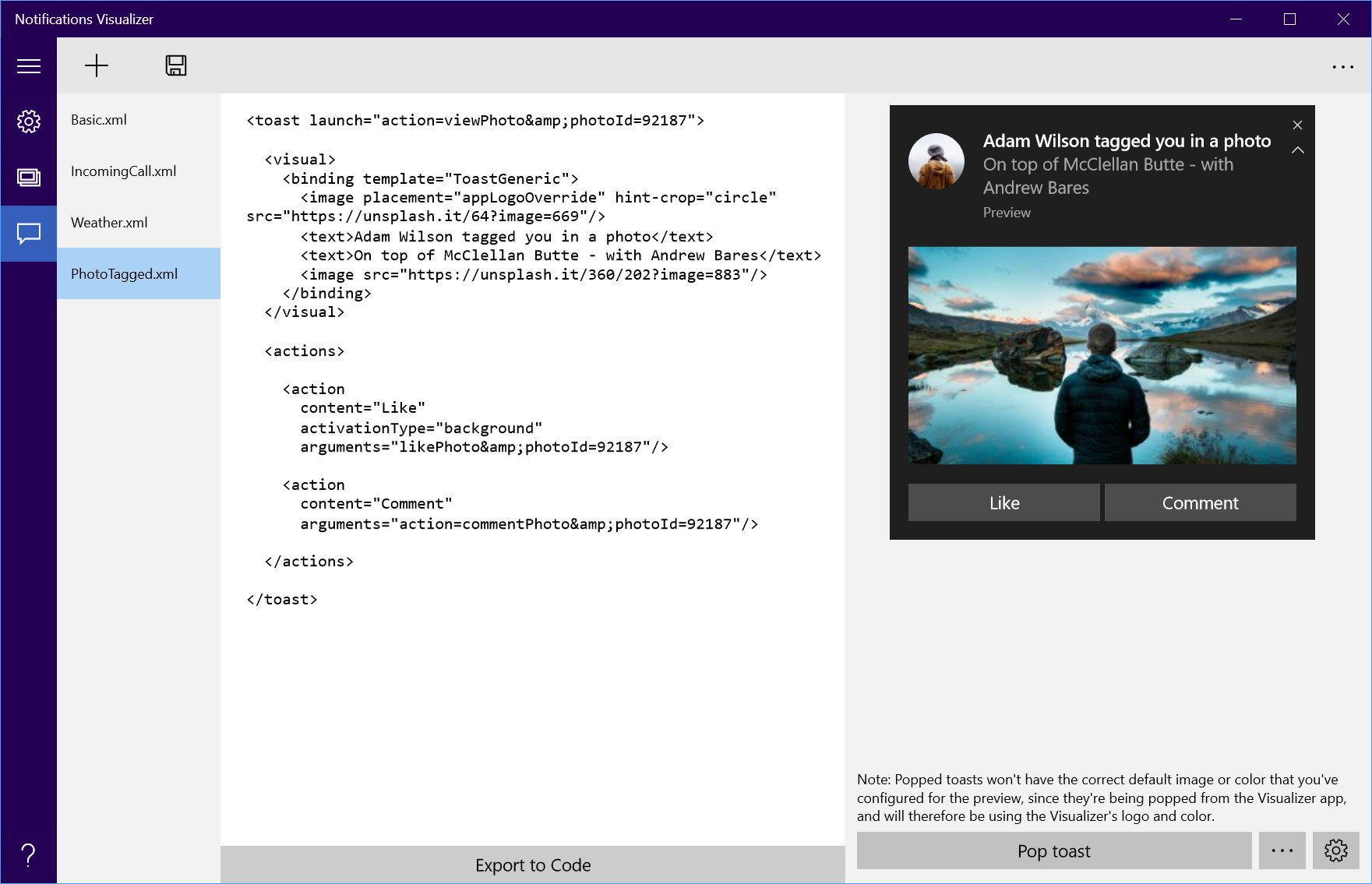
# Acknowledgements
- [winsdk_toast](https://github.com/Mo-Dabao/winsdk_toast)
- [Windows-Toasts](https://github.com/DatGuy1/Windows-Toasts)
- [MarcAlx/notification.py](https://gist.github.com/MarcAlx/443358d5e7167864679ffa1b7d51cd06)
Raw data
{
"_id": null,
"home_page": "https://github.com/GitHub30/win11toast",
"name": "win11toast",
"maintainer": "",
"docs_url": null,
"requires_python": "",
"maintainer_email": "",
"keywords": "",
"author": "Tomofumi Inoue",
"author_email": "funaox@gmail.com",
"download_url": "https://files.pythonhosted.org/packages/4c/24/b2b2e62a0ca54bf5635d77f923bfce313983b8120e719d78506947154b7e/win11toast-0.34.tar.gz",
"platform": null,
"description": "[](https://badge.fury.io/py/win11toast)\n[](https://badge.fury.io/py/win11toast)\n\n# win11toast\nToast notifications for Windows 10 and 11 based on [WinRT](https://docs.microsoft.com/en-us/windows/apps/design/shell/tiles-and-notifications/adaptive-interactive-toasts)\n\n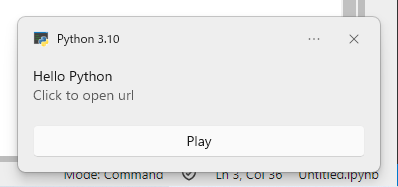\n\n## Installation\n\n```bash\npip install win11toast\n```\n\n## Usage\n\n```python\nfrom win11toast import toast\n\ntoast('Hello Python\ud83d\udc0d')\n```\n\n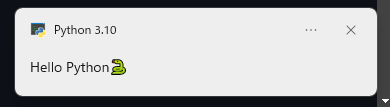\n\nhttps://docs.microsoft.com/en-us/uwp/schemas/tiles/toastschema/element-text\n\n### Body\n\n```python\nfrom win11toast import toast\n\ntoast('Hello Python', 'Click to open url', on_click='https://www.python.org')\n```\n\n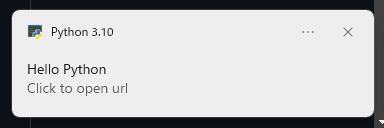\n\n### Wrap text\n\n```python\nfrom win11toast import toast\n\ntoast('Hello', 'Lorem ipsum dolor sit amet, consectetur adipisicing elit. Earum accusantium porro numquam aspernatur voluptates cum, odio in, animi nihil cupiditate molestias laborum. Consequatur exercitationem modi vitae. In voluptates quia obcaecati!')\n```\n\n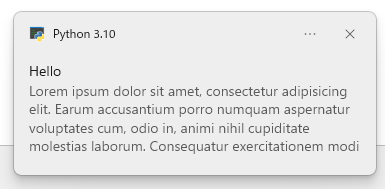\n\n### Run Python script on Click\n```python\nfrom win11toast import toast\n\ntoast('Hello Pythonista', 'Click to run python script', on_click=r'C:\\Users\\Admin\\Downloads\\handler.pyw')\n# {'arguments': 'C:\\\\Users\\\\Admin\\\\Downloads\\\\handler.pyw', 'user_input': {}}\n```\n\nSince the current directory when executing the script is `C:\\Windows\\system32`, use `os.chdir()` accordingly.\n\ne.g. [handler.pyw](https://gist.github.com/GitHub30/dae1b257c93d8315ea38554c9554a2ad)\n\nOn Windows, you can run a Python script in the background using the pythonw.exe executable, which will run your program with no visible process or way to interact with it.\n\nhttps://stackoverflow.com/questions/9705982/pythonw-exe-or-python-exe\n\n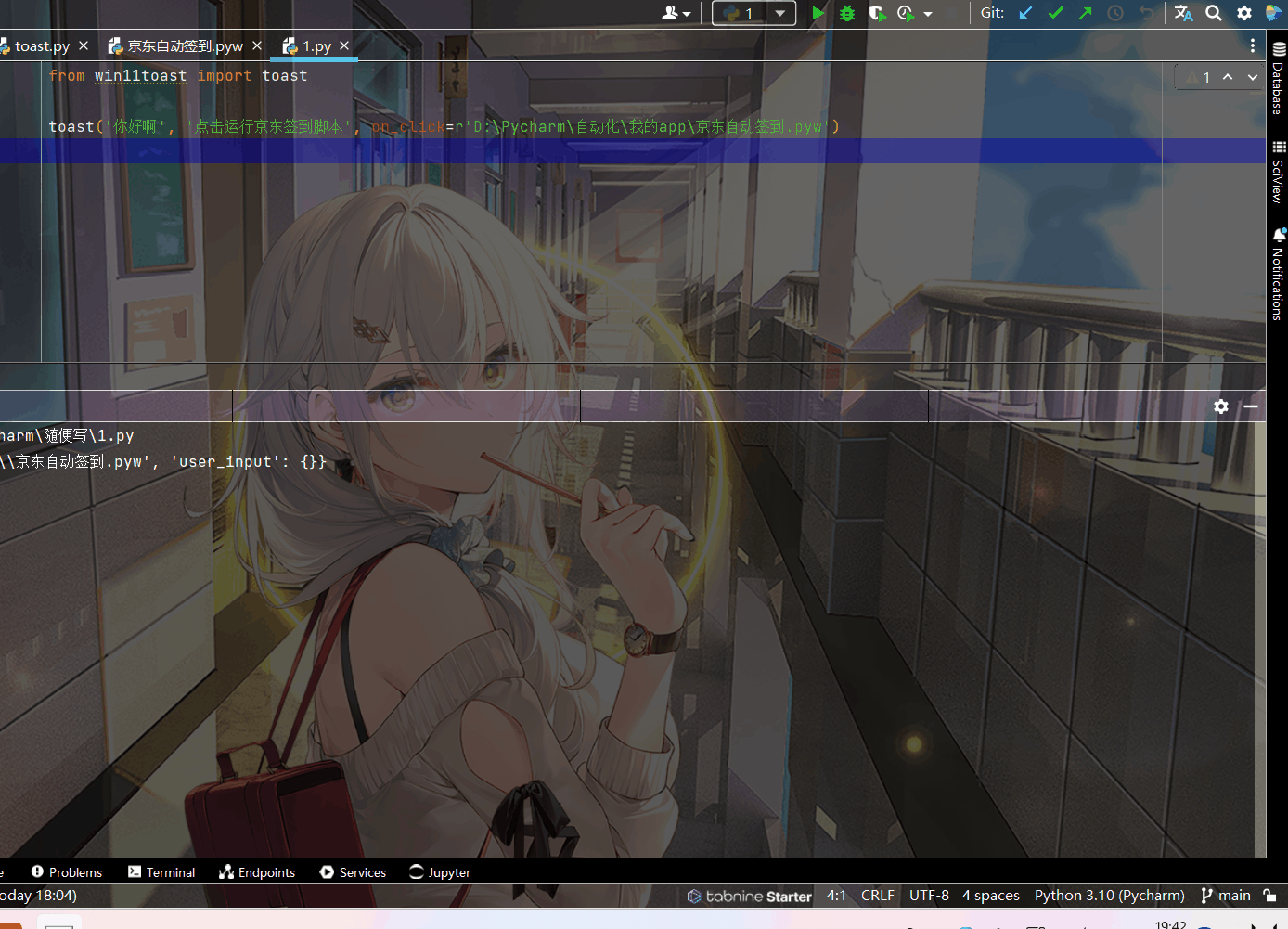\n\n### Callback\n\n```python\nfrom win11toast import toast\n\ntoast('Hello Python', 'Click to open url', on_click=lambda args: print('clicked!', args))\n# clicked! {'arguments': 'http:', 'user_input': {}}\n```\n\n### Icon\n\n```python\nfrom win11toast import toast\n\ntoast('Hello', 'Hello from Python', icon='https://unsplash.it/64?image=669')\n```\n\n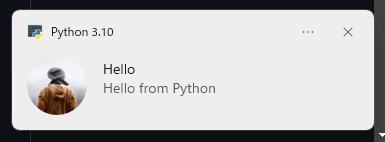\n\n#### Square\n\n```python\nfrom win11toast import toast\n\nicon = {\n 'src': 'https://unsplash.it/64?image=669',\n 'placement': 'appLogoOverride'\n}\n\ntoast('Hello', 'Hello from Python', icon=icon)\n```\n\n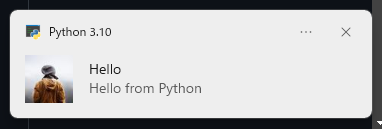\n\n### Image\n\n```python\nfrom win11toast import toast\n\ntoast('Hello', 'Hello from Python', image='https://4.bp.blogspot.com/-u-uyq3FEqeY/UkJLl773BHI/AAAAAAAAYPQ/7bY05EeF1oI/s800/cooking_toaster.png')\n```\n\n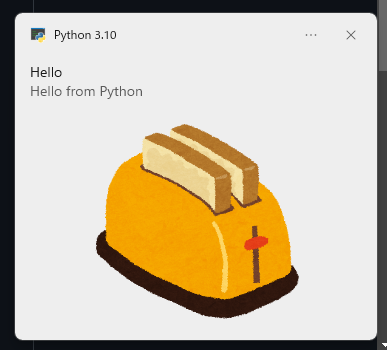\n\nhttps://docs.microsoft.com/en-us/uwp/schemas/tiles/toastschema/element-image\n\n#### Hero\n\n```python\nfrom win11toast import toast\n\nimage = {\n 'src': 'https://4.bp.blogspot.com/-u-uyq3FEqeY/UkJLl773BHI/AAAAAAAAYPQ/7bY05EeF1oI/s800/cooking_toaster.png',\n 'placement': 'hero'\n}\n\ntoast('Hello', 'Hello from Python', image=image)\n```\n\n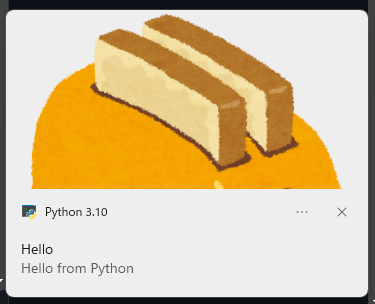\n\n### Progress\n\n```python\nfrom time import sleep\nfrom win11toast import notify, update_progress\n\nnotify(progress={\n 'title': 'YouTube',\n 'status': 'Downloading...',\n 'value': '0',\n 'valueStringOverride': '0/15 videos'\n})\n\nfor i in range(1, 15+1):\n sleep(1)\n update_progress({'value': i/15, 'valueStringOverride': f'{i}/15 videos'})\n\nupdate_progress({'status': 'Completed!'})\n```\n\n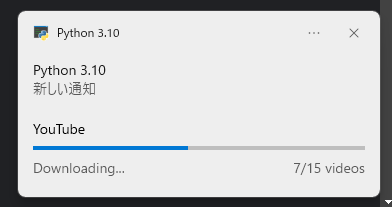\n\nAttributes\nhttps://docs.microsoft.com/en-ca/uwp/schemas/tiles/toastschema/element-progress\n\nhttps://docs.microsoft.com/en-us/windows/apps/design/shell/tiles-and-notifications/toast-progress-bar\n\n### Audio\n\n```python\nfrom win11toast import toast\n\ntoast('Hello', 'Hello from Python', audio='ms-winsoundevent:Notification.Looping.Alarm')\n```\n\nAvailable audio\nhttps://docs.microsoft.com/en-us/uwp/schemas/tiles/toastschema/element-audio\n\n##### From URL\n\n```python\nfrom win11toast import toast\n\ntoast('Hello', 'Hello from Python', audio='https://nyanpass.com/nyanpass.mp3')\n```\n\n##### From file\n\n```python\nfrom win11toast import toast\n\ntoast('Hello', 'Hello from Python', audio=r\"C:\\Users\\Admin\\Downloads\\nyanpass.mp3\")\n```\n\nI don't know how to add custom audio please help.\n\nhttps://docs.microsoft.com/en-us/windows/apps/design/shell/tiles-and-notifications/custom-audio-on-toasts\n\n#### Loop\n\n```python\nfrom win11toast import toast\n\ntoast('Hello', 'Hello from Python', audio={'loop': 'true'})\n```\n\n```python\nfrom win11toast import toast\n\ntoast('Hello', 'Hello from Python', audio={'src': 'ms-winsoundevent:Notification.Looping.Alarm', 'loop': 'true'})\n```\n\n### Silent\ud83e\udd2b\n\n```python\nfrom win11toast import toast\n\ntoast('Hello Python\ud83d\udc0d', audio={'silent': 'true'})\n```\n\n### Speak\ud83d\udde3\n\n```python\nfrom win11toast import toast\n\ntoast('Hello Python\ud83d\udc0d', dialogue='Hello world')\n```\n\n### OCR\ud83d\udc40\n\n```python\nfrom win11toast import toast\n\ntoast(ocr='https://i.imgur.com/oYojrJW.png')\n```\n\n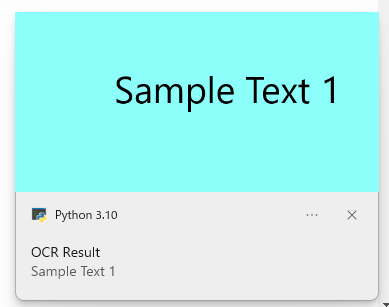\n\n```python\nfrom win11toast import toast\n\ntoast(ocr={'lang': 'ja', 'ocr': r'C:\\Users\\Admin\\Downloads\\hello.png'})\n```\n\n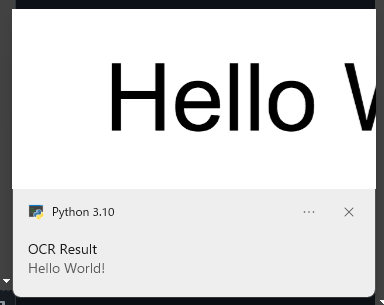\n\n### Long duration\n\n```python\nfrom win11toast import toast\n\ntoast('Hello Python\ud83d\udc0d', duration='long')\n```\n\ndisplayed for 25 seconds\nhttps://docs.microsoft.com/en-us/uwp/schemas/tiles/toastschema/element-toast\n\n### No timeout\n\n```python\nfrom win11toast import toast\n\ntoast('Hello Python\ud83d\udc0d', scenario='incomingCall')\n```\n\nThe scenario your toast is used for, like an alarm, reminder, incomingCall or urgent.\n\nhttps://learn.microsoft.com/en-us/uwp/schemas/tiles/toastschema/element-toast#:~:text=None-,scenario,-The%20scenario%20your\n\n\n\n\n### Button\n\n```python\nfrom win11toast import toast\n\ntoast('Hello', 'Hello from Python', button='Dismiss')\n# {'arguments': 'http:Dismiss', 'user_input': {}}\n```\n\n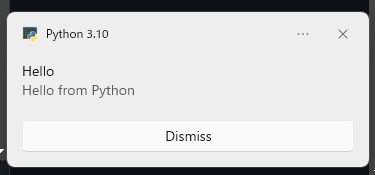\n\n```python\nfrom win11toast import toast\n\ntoast('Hello', 'Hello from Python', button={'activationType': 'protocol', 'arguments': 'https://google.com', 'content': 'Open Google'})\n# {'arguments': 'https://google.com', 'user_input': {}}\n```\n\n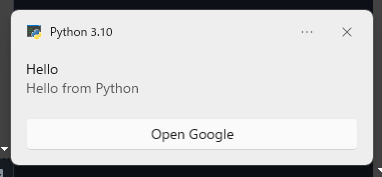\n\n```python\nfrom win11toast import toast\n\ntoast('Hello', 'Click a button', buttons=['Approve', 'Dismiss', 'Other'])\n```\n\n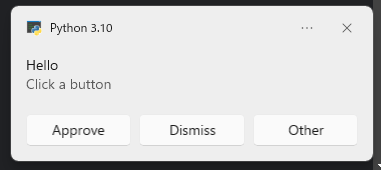\n\nhttps://docs.microsoft.com/en-us/uwp/schemas/tiles/toastschema/element-action\n\n#### Play music or Open Explorer\n\n```python\nfrom win11toast import toast\n\nbuttons = [\n {'activationType': 'protocol', 'arguments': 'C:\\Windows\\Media\\Alarm01.wav', 'content': 'Play'},\n {'activationType': 'protocol', 'arguments': 'file:///C:/Windows/Media', 'content': 'Open Folder'}\n]\n\ntoast('Music Player', 'Download Finished', buttons=buttons)\n```\n\n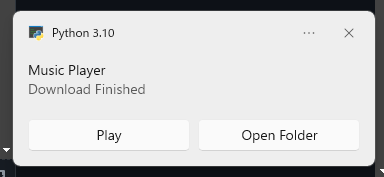\n\n### Input\n\n```python\nfrom win11toast import toast\n\ntoast('Hello', 'Type anything', input='reply', button='Send')\n# {'arguments': 'http:Send', 'user_input': {'reply': 'Hi there'}}\n```\n\n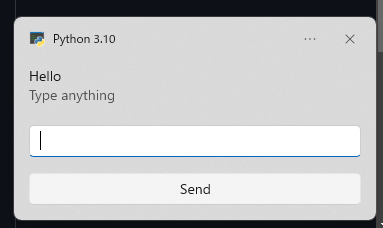\n\n```python\nfrom win11toast import toast\n\ntoast('Hello', 'Type anything', input='reply', button={'activationType': 'protocol', 'arguments': 'http:', 'content': 'Send', 'hint-inputId': 'reply'})\n# {'arguments': 'http:', 'user_input': {'reply': 'Hi there'}}\n```\n\n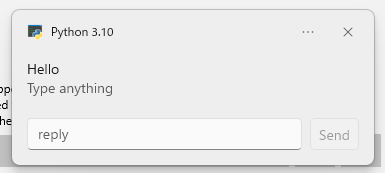\n\nhttps://docs.microsoft.com/en-us/uwp/schemas/tiles/toastschema/element-input\n\n### Selection\n\n```python\nfrom win11toast import toast\n\ntoast('Hello', 'Which do you like?', selection=['Apple', 'Banana', 'Grape'], button='Submit')\n# {'arguments': 'dismiss', 'user_input': {'selection': 'Grape'}}\n```\n\n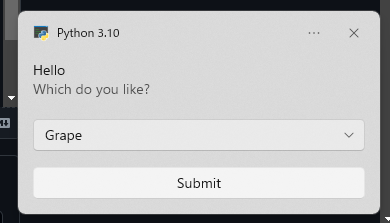\n\n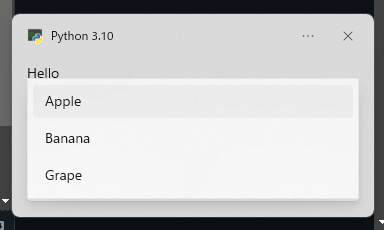\n\n### No arguments\n\n```python\nfrom win11toast import toast\n\ntoast()\n```\n\n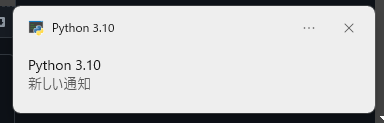\n\n### Non blocking\n\n```python\nfrom win11toast import notify\n\nnotify('Hello Python', 'Click to open url', on_click='https://www.python.org')\n```\n\n### Async\n\n```python\nfrom win11toast import toast_async\n\nasync def main():\n await toast_async('Hello Python', 'Click to open url', on_click='https://www.python.org')\n```\n\n### Jupyter\n\n```python\nfrom win11toast import notify\n\nnotify('Hello Python', 'Click to open url', on_click='https://www.python.org')\n```\n\n\n\n```python\nfrom win11toast import toast_async\n\nawait toast_async('Hello Python', 'Click to open url', on_click='https://www.python.org')\n```\n\n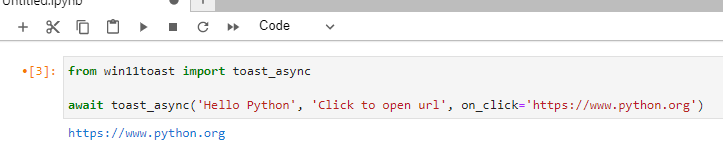\n\n```python\nimport urllib.request\nfrom pathlib import Path\nsrc = str(Path(urllib.request.urlretrieve(\"https://i.imgur.com/p9dRdtP.jpg\")[0]).absolute())\n\nfrom win11toast import toast_async\nawait toast_async('\u306b\u3083\u3093\u3071\u3059\u30fc', audio='https://nyanpass.com/nyanpass.mp3', image={'src': src, 'placement':'hero'})\n```\n\n```python\nfrom win11toast import toast_async\n\nawait toast_async('Hello Python\ud83d\udc0d', dialogue='\u306b\u3083\u3093\u3071\u3059\u30fc')\n```\n\n## Debug\n\n```python\nfrom win11toast import toast\n\nxml = \"\"\"\n<toast launch=\"action=openThread&threadId=92187\">\n\n <visual>\n <binding template=\"ToastGeneric\">\n <text hint-maxLines=\"1\">Jill Bender</text>\n <text>Check out where we camped last weekend! It was incredible, wish you could have come on the backpacking trip!</text>\n <image placement=\"appLogoOverride\" hint-crop=\"circle\" src=\"https://unsplash.it/64?image=1027\"/>\n <image placement=\"hero\" src=\"https://unsplash.it/360/180?image=1043\"/>\n </binding>\n </visual>\n\n <actions>\n\n <input id=\"textBox\" type=\"text\" placeHolderContent=\"reply\"/>\n\n <action\n content=\"Send\"\n imageUri=\"Assets/Icons/send.png\"\n hint-inputId=\"textBox\"\n activationType=\"background\"\n arguments=\"action=reply&threadId=92187\"/>\n\n </actions>\n\n</toast>\"\"\"\n\ntoast(xml=xml)\n```\n\n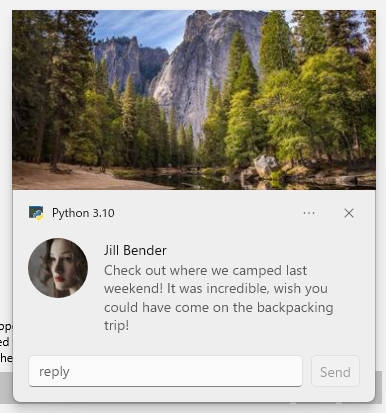\n\n\n[Notifications Visualizer](https://www.microsoft.com/store/apps/notifications-visualizer/9nblggh5xsl1)\n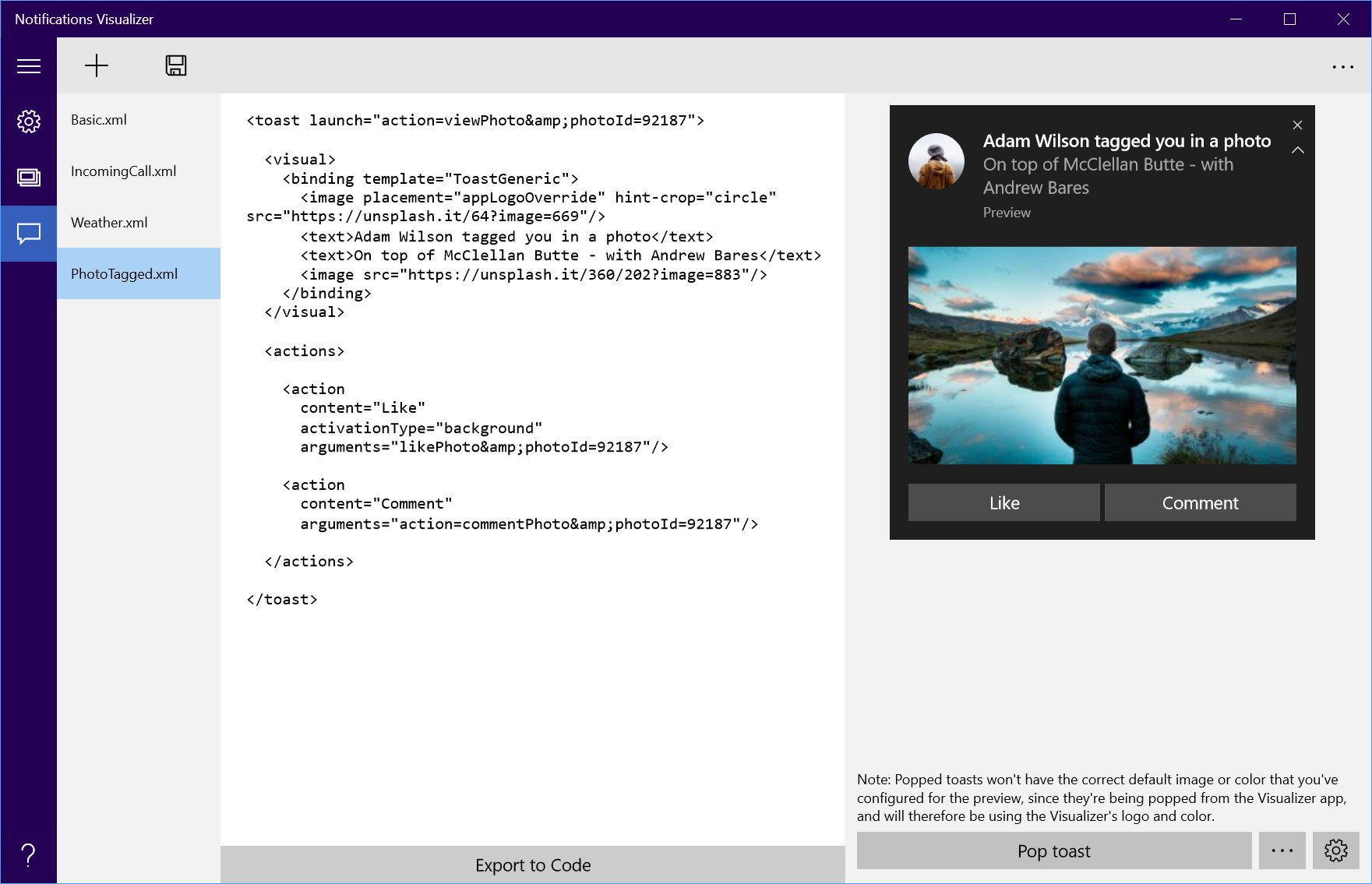\n\n\n# Acknowledgements\n\n- [winsdk_toast](https://github.com/Mo-Dabao/winsdk_toast)\n- [Windows-Toasts](https://github.com/DatGuy1/Windows-Toasts)\n- [MarcAlx/notification.py](https://gist.github.com/MarcAlx/443358d5e7167864679ffa1b7d51cd06)\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "Toast notifications for Windows 10 and 11",
"version": "0.34",
"project_urls": {
"Bug Tracker": "https://github.com/GitHub30/win11toast/issues",
"Homepage": "https://github.com/GitHub30/win11toast"
},
"split_keywords": [],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "56ca98b20cb99d50188f534effccec57700b4ccbcc26571ddbfc2d932d8f20af",
"md5": "c87f262e77236597811b033bcffc9563",
"sha256": "5c1df6301135703330fba3cbb41b0d9d044ba25c1840305b424dc08e98c9a7b6"
},
"downloads": -1,
"filename": "win11toast-0.34-py3-none-any.whl",
"has_sig": false,
"md5_digest": "c87f262e77236597811b033bcffc9563",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": null,
"size": 9408,
"upload_time": "2023-10-25T15:40:10",
"upload_time_iso_8601": "2023-10-25T15:40:10.743208Z",
"url": "https://files.pythonhosted.org/packages/56/ca/98b20cb99d50188f534effccec57700b4ccbcc26571ddbfc2d932d8f20af/win11toast-0.34-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "4c24b2b2e62a0ca54bf5635d77f923bfce313983b8120e719d78506947154b7e",
"md5": "f0dca079d2f1e7a09fa408aa64d59721",
"sha256": "516e259bd38e124bbed39214143f1c71c0f535751bfed9454489efd2bc0cd70c"
},
"downloads": -1,
"filename": "win11toast-0.34.tar.gz",
"has_sig": false,
"md5_digest": "f0dca079d2f1e7a09fa408aa64d59721",
"packagetype": "sdist",
"python_version": "source",
"requires_python": null,
"size": 9506,
"upload_time": "2023-10-25T15:40:12",
"upload_time_iso_8601": "2023-10-25T15:40:12.617279Z",
"url": "https://files.pythonhosted.org/packages/4c/24/b2b2e62a0ca54bf5635d77f923bfce313983b8120e719d78506947154b7e/win11toast-0.34.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-10-25 15:40:12",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "GitHub30",
"github_project": "win11toast",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "win11toast"
}