# Package [wizzi utils](https://github.com/2easy4wizzi/wizzi_utils_pypi/tree/main):
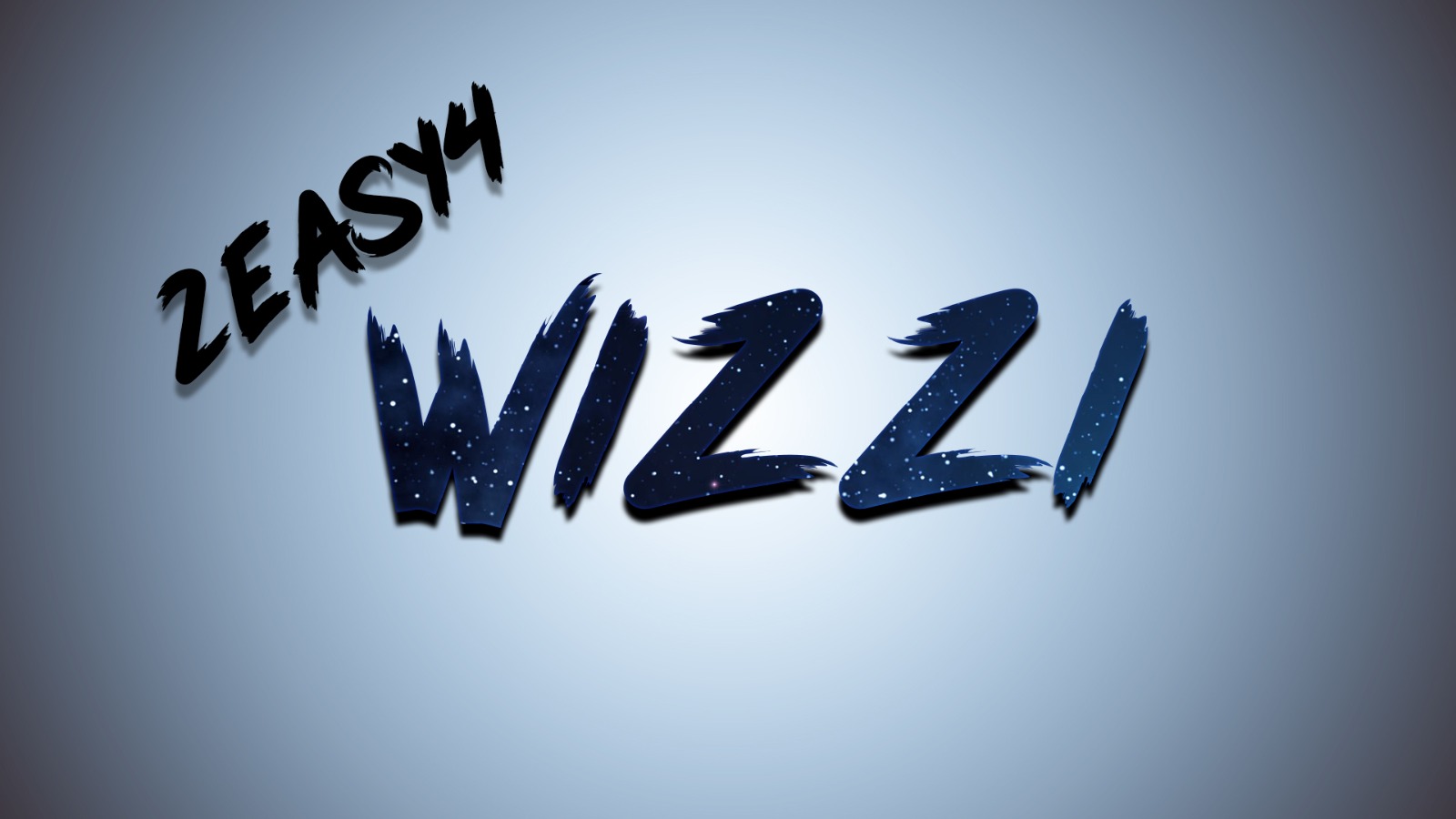
## Brief
* "wizzi_utils" main usage is for debugging and fast coding.
* The package is cross-platform (most of the functions tested on Windows and linux).
* The package is well documented and contain many easy access common functions.
* In addition, almost every function is used in a test(more an example) I've written for learning purposes.
* Package includes tools for json, open_cv, pyplot, socket, torch, text to speach and more.
* If by any chance, I used code and didn't give credit, please contact me, and I'll remove the code or specify the
source.
* Contact: giladeini@gmail.com
## Usage
```python
import wizzi_utils as wu # pip install wizzi_utils
# The above import, will give access to all wizzi utils main module and the extra modules
# only if the packages are installed
print(wu.to_str(var=2, title='my_int')) # this will 100% work
wu.test.to_str_test() # for a wide example of to_str function
print(wu.tt.to_str(var=3, title='my_int')) # tt for torch tools. will work if torch installed
wu.tt.test.to_str_test() # for a wide example of tt.to_str function
# If facing packages issues and want to know what packages I used, call the following
wu.download_wizzi_utils_env_snapshot() # updated on 10/10/2022
```
### list of all the packages
```python
import wizzi_utils as wu
wu.test.test_all() # main module - all function that dont require extra installations but wizzi_utils
wu.jt.test.test_all() # json tools
wu.cvt.test.test_all() # cv2 tools
wu.pyplt.test.test_all() # pyplot tools
wu.st.test.test_all() # socket tools
wu.tt.test.test_all() # torch tools
wu.tflt.test.test_all() # tensorflow lite tools
wu.tts.test.test_all() # text to speach tools
wu.models.test.test_all() # models - cv2 and tf lite models. tracking, object detection and pose estimation
wu.got.test.test_all() # google drive tools - work in progress
```
### Some examples
```python
import wizzi_utils as wu
"""
* wu.main_wrapper()
* Extremely useful if run experiments and want to have the meta data saved
* e.g. the interpreter, the time, the pc details ...
"""
def main():
msg = "Hello world in red "
msg += wu.get_emoji(wu.EMOJIS.SMILING_FACE_WITH_3_HEARTS)
msg = wu.add_color(string=msg, ops=['Red', 'bold', 'underlined'])
print(msg)
# wu.test.add_color_test()
# wu.test.get_emoji_test()
return
if __name__ == '__main__':
wu.main_wrapper(
main_function=main,
seed=42,
ipv4=True,
cuda_off=False,
nvid_gpu=True,
torch_v=True,
tf_v=True,
cv2_v=True,
with_pip_list=False,
with_profiler=False
)
```
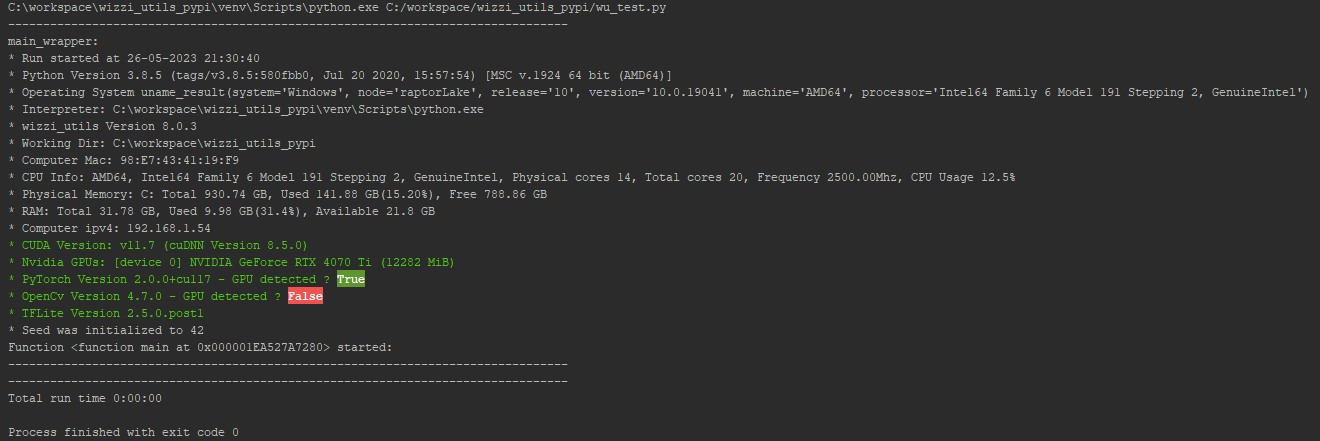
```python
import wizzi_utils as wu
# fps measurements:
fps = wu.FPS(last_k=3, cache_size=5, summary_title='classFPS_test')
for t in range(10):
ack = (t + 1) % 2 == 0 # display only on even rounds
fps.start(ack_progress=False)
# do_work of round t
wu.sleep(seconds=0.03)
if t == 0: # e.g. slow first iteration
wu.sleep(seconds=1)
fps.update(ack_progress=ack)
if t == 5:
print('\tget_fps() = {:.4f}'.format(fps.get_fps()))
fps.finalize()
```
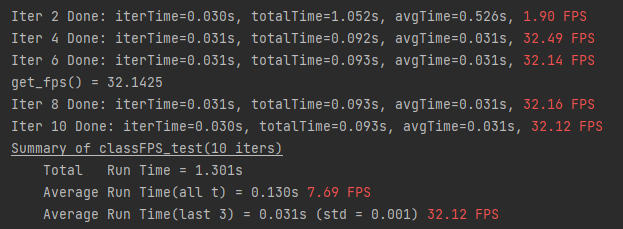
```python
import wizzi_utils as wu
"""
* cv2 show image with extra control:
* concatenation of several images(e.g. 2 cameras frames)
* display in a grid (2x1 1x2 3x3)
* resize (0.3 -> 30%, (200, 400)->total image to (200, 400), fs for full screen
* window location
* and more...
"""
img_utl = 'https://cdn.sstatic.net/Sites/stackoverflow/img/logo.png'
wu.st.download_file(url=img_utl, dst_path='./so_logo.png') # st for socket tools
bgr = wu.cvt.load_img(path='./so_logo.png', ack=True)
gray = wu.cvt.bgr_to_gray(bgr)
rgb = wu.cvt.bgr_to_rgb(bgr)
wu.cvt.add_header(bgr, header='bgr', loc='bl', text_color='lime')
wu.cvt.add_header(gray, header='gray', loc='bl')
wu.cvt.add_header(rgb, header='rgb', loc='bl', text_color='aqua')
wu.cvt.display_open_cv_images(
imgs=[bgr, gray, rgb],
ms=0,
title='display images',
loc='bc', # bottom center
resize=1.5, # 150%
grid=(3, 1), # 3 rows 1 col
header='cool, no?',
separator_c='aqua',
)
```
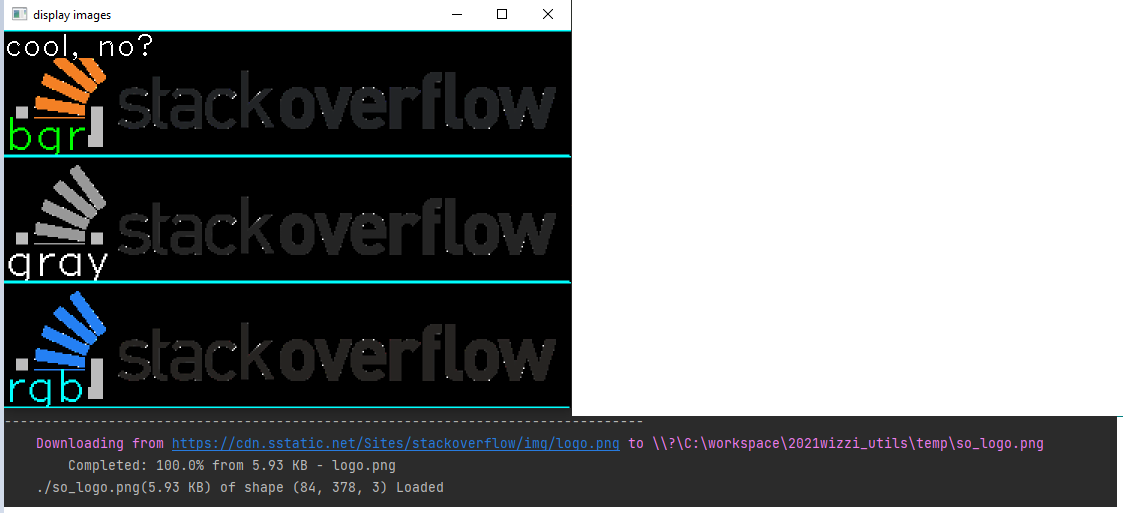
```python
import wizzi_utils as wu
"""
* object detection models
* pose detection models
* tracking
"""
wu.models.test.test_cv2_object_detection_models()
wu.models.test.test_cv2_pose_detection_models()
wu.models.test.test_cv2_tracking_models()
```
<!---  -->
<!---  -->
<!---  -->
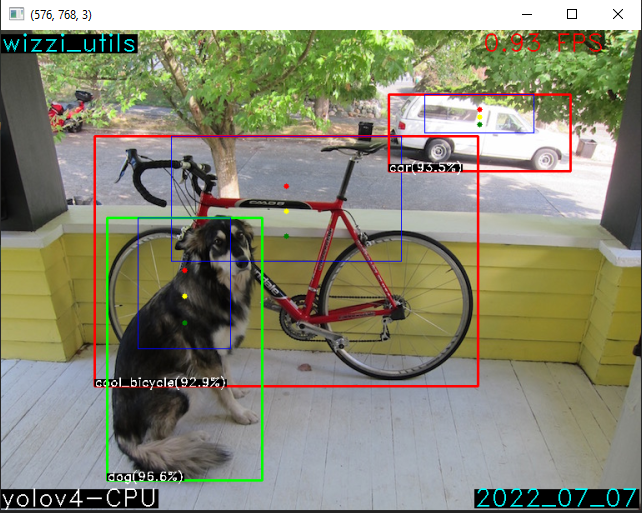
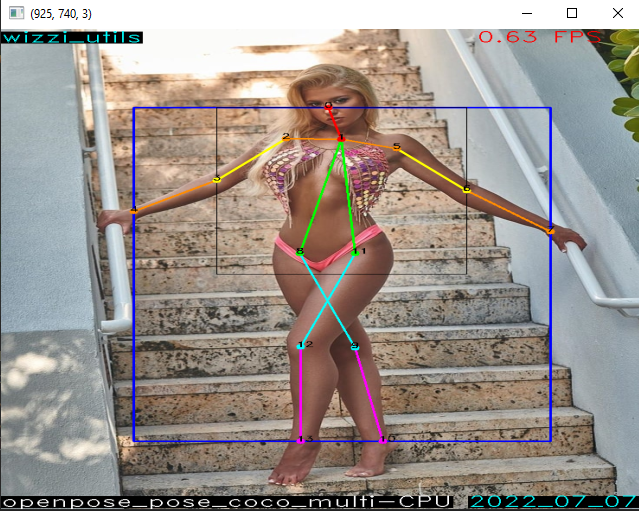
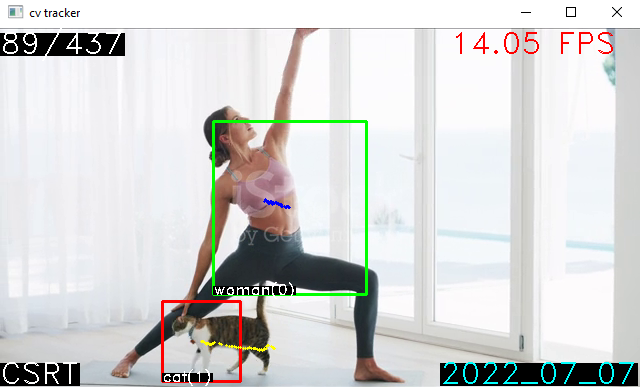
```python
import wizzi_utils as wu
# text to speak gui over pyttsx3 and pyQt5 packages
wu.tts.test.run_machine_buddy_gui_test()
```
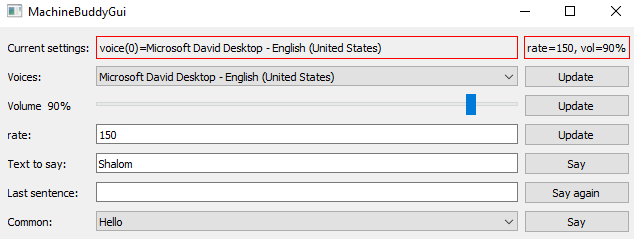
Raw data
{
"_id": null,
"home_page": "https://github.com/2easy4wizzi/wizzi_utils_pypi",
"name": "wizzi-utils",
"maintainer": "",
"docs_url": null,
"requires_python": "",
"maintainer_email": "",
"keywords": "debugging tools,json,open cv,pyplot,socket,torch,tf lite",
"author": "Gilad Eini",
"author_email": "giladEini@gmail.com",
"download_url": "https://files.pythonhosted.org/packages/ba/47/245715c89705365bbdae3a14d0a3d1a1a702cd4bc1a99320a022be032497/wizzi_utils-8.0.6.tar.gz",
"platform": "windows",
"description": "# Package [wizzi utils](https://github.com/2easy4wizzi/wizzi_utils_pypi/tree/main):\r\n\r\n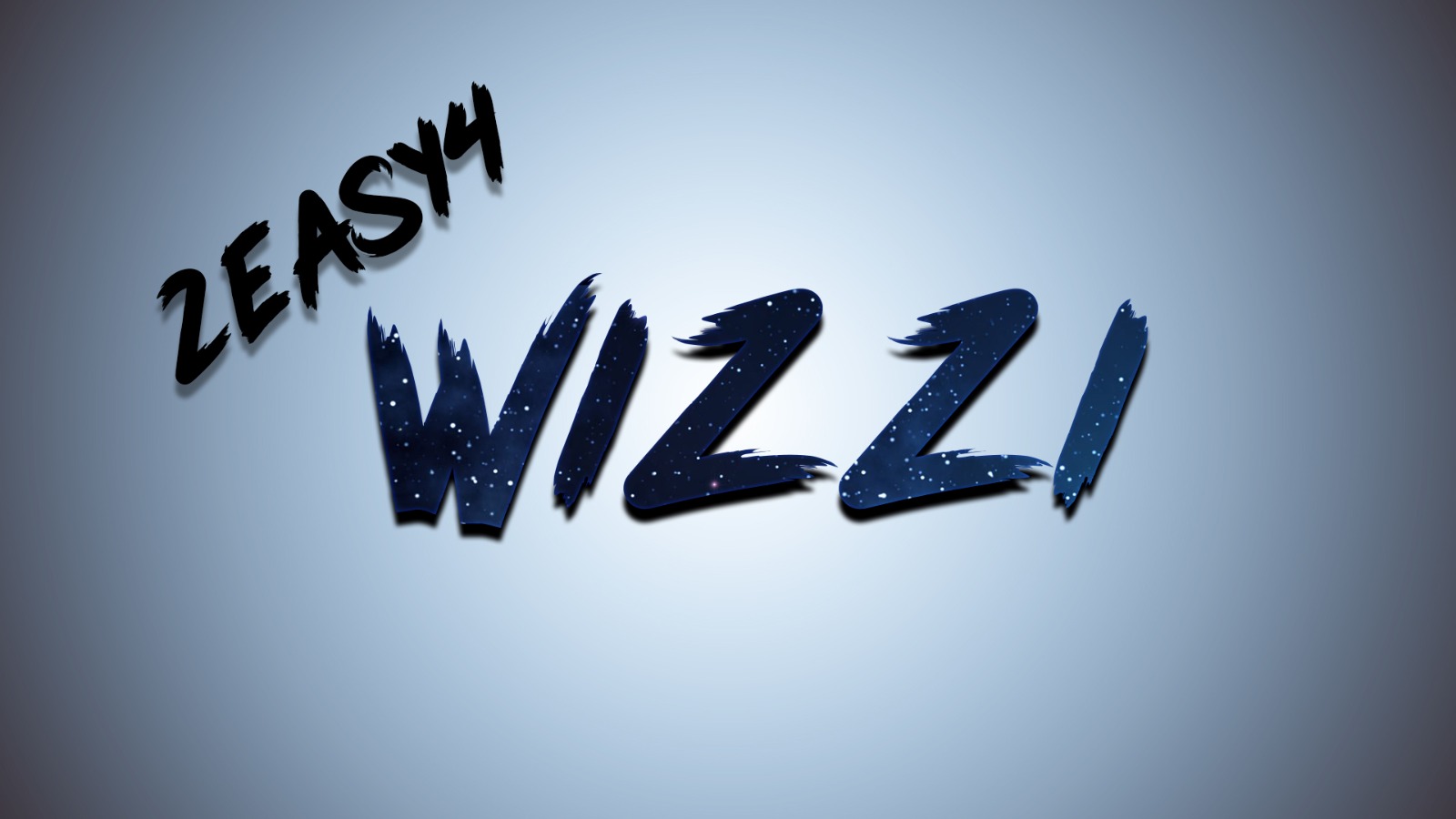\r\n\r\n## Brief\r\n\r\n* \"wizzi_utils\" main usage is for debugging and fast coding.\r\n* The package is cross-platform (most of the functions tested on Windows and linux).\r\n* The package is well documented and contain many easy access common functions.\r\n * In addition, almost every function is used in a test(more an example) I've written for learning purposes.\r\n* Package includes tools for json, open_cv, pyplot, socket, torch, text to speach and more.\r\n* If by any chance, I used code and didn't give credit, please contact me, and I'll remove the code or specify the\r\n source.\r\n* Contact: giladeini@gmail.com\r\n\r\n## Usage\r\n\r\n```python\r\nimport wizzi_utils as wu # pip install wizzi_utils\r\n\r\n# The above import, will give access to all wizzi utils main module and the extra modules \r\n# only if the packages are installed\r\nprint(wu.to_str(var=2, title='my_int')) # this will 100% work\r\nwu.test.to_str_test() # for a wide example of to_str function\r\nprint(wu.tt.to_str(var=3, title='my_int')) # tt for torch tools. will work if torch installed\r\nwu.tt.test.to_str_test() # for a wide example of tt.to_str function\r\n# If facing packages issues and want to know what packages I used, call the following\r\nwu.download_wizzi_utils_env_snapshot() # updated on 10/10/2022 \r\n```\r\n\r\n### list of all the packages\r\n\r\n```python\r\nimport wizzi_utils as wu\r\n\r\nwu.test.test_all() # main module - all function that dont require extra installations but wizzi_utils\r\nwu.jt.test.test_all() # json tools \r\nwu.cvt.test.test_all() # cv2 tools \r\nwu.pyplt.test.test_all() # pyplot tools \r\nwu.st.test.test_all() # socket tools\r\nwu.tt.test.test_all() # torch tools \r\nwu.tflt.test.test_all() # tensorflow lite tools\r\nwu.tts.test.test_all() # text to speach tools\r\nwu.models.test.test_all() # models - cv2 and tf lite models. tracking, object detection and pose estimation\r\nwu.got.test.test_all() # google drive tools - work in progress\r\n```\r\n\r\n### Some examples\r\n\r\n```python\r\nimport wizzi_utils as wu\r\n\r\n\"\"\"\r\n* wu.main_wrapper()\r\n* Extremely useful if run experiments and want to have the meta data saved\r\n* e.g. the interpreter, the time, the pc details ...\r\n\"\"\"\r\n\r\n\r\ndef main():\r\n msg = \"Hello world in red \"\r\n msg += wu.get_emoji(wu.EMOJIS.SMILING_FACE_WITH_3_HEARTS)\r\n msg = wu.add_color(string=msg, ops=['Red', 'bold', 'underlined'])\r\n print(msg)\r\n # wu.test.add_color_test()\r\n # wu.test.get_emoji_test()\r\n return\r\n\r\n\r\nif __name__ == '__main__':\r\n wu.main_wrapper(\r\n main_function=main,\r\n seed=42,\r\n ipv4=True,\r\n cuda_off=False,\r\n nvid_gpu=True,\r\n torch_v=True,\r\n tf_v=True,\r\n cv2_v=True,\r\n with_pip_list=False,\r\n with_profiler=False\r\n )\r\n```\r\n\r\n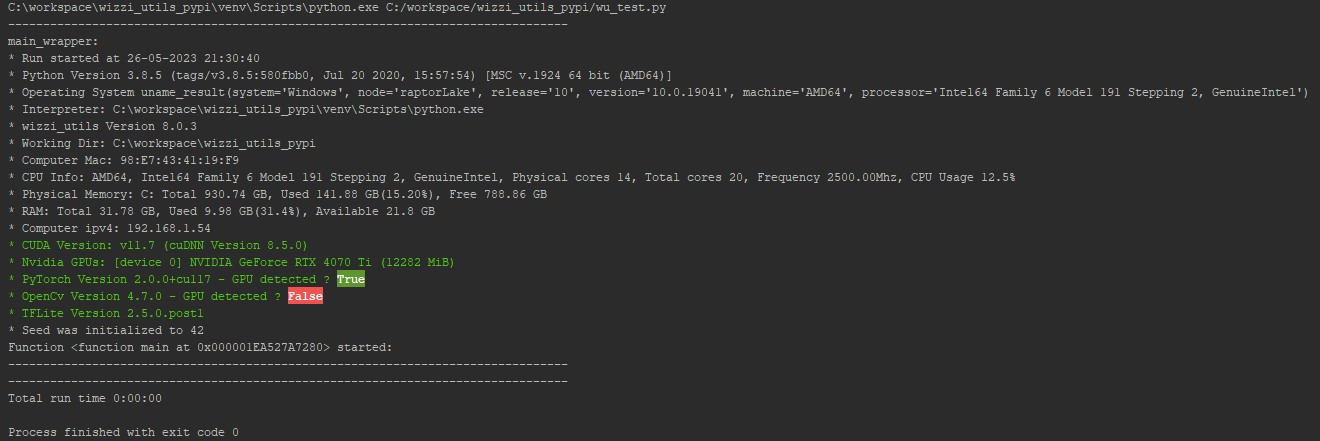\r\n\r\n```python\r\nimport wizzi_utils as wu\r\n\r\n# fps measurements:\r\nfps = wu.FPS(last_k=3, cache_size=5, summary_title='classFPS_test')\r\nfor t in range(10):\r\n ack = (t + 1) % 2 == 0 # display only on even rounds\r\n fps.start(ack_progress=False)\r\n # do_work of round t\r\n wu.sleep(seconds=0.03)\r\n if t == 0: # e.g. slow first iteration\r\n wu.sleep(seconds=1)\r\n fps.update(ack_progress=ack)\r\n if t == 5:\r\n print('\\tget_fps() = {:.4f}'.format(fps.get_fps()))\r\nfps.finalize()\r\n```\r\n\r\n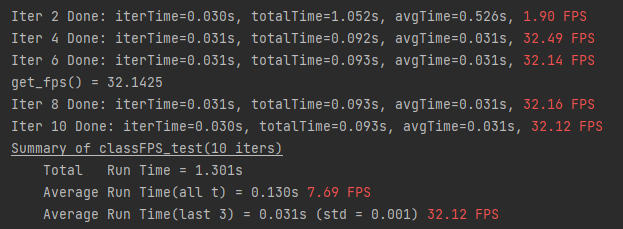\r\n\r\n```python\r\nimport wizzi_utils as wu\r\n\r\n\"\"\"\r\n* cv2 show image with extra control:\r\n* concatenation of several images(e.g. 2 cameras frames)\r\n* display in a grid (2x1 1x2 3x3)\r\n* resize (0.3 -> 30%, (200, 400)->total image to (200, 400), fs for full screen\r\n* window location\r\n* and more...\r\n\"\"\"\r\nimg_utl = 'https://cdn.sstatic.net/Sites/stackoverflow/img/logo.png'\r\nwu.st.download_file(url=img_utl, dst_path='./so_logo.png') # st for socket tools\r\nbgr = wu.cvt.load_img(path='./so_logo.png', ack=True)\r\ngray = wu.cvt.bgr_to_gray(bgr)\r\nrgb = wu.cvt.bgr_to_rgb(bgr)\r\nwu.cvt.add_header(bgr, header='bgr', loc='bl', text_color='lime')\r\nwu.cvt.add_header(gray, header='gray', loc='bl')\r\nwu.cvt.add_header(rgb, header='rgb', loc='bl', text_color='aqua')\r\nwu.cvt.display_open_cv_images(\r\n imgs=[bgr, gray, rgb],\r\n ms=0,\r\n title='display images',\r\n loc='bc', # bottom center\r\n resize=1.5, # 150%\r\n grid=(3, 1), # 3 rows 1 col\r\n header='cool, no?',\r\n separator_c='aqua',\r\n)\r\n```\r\n\r\n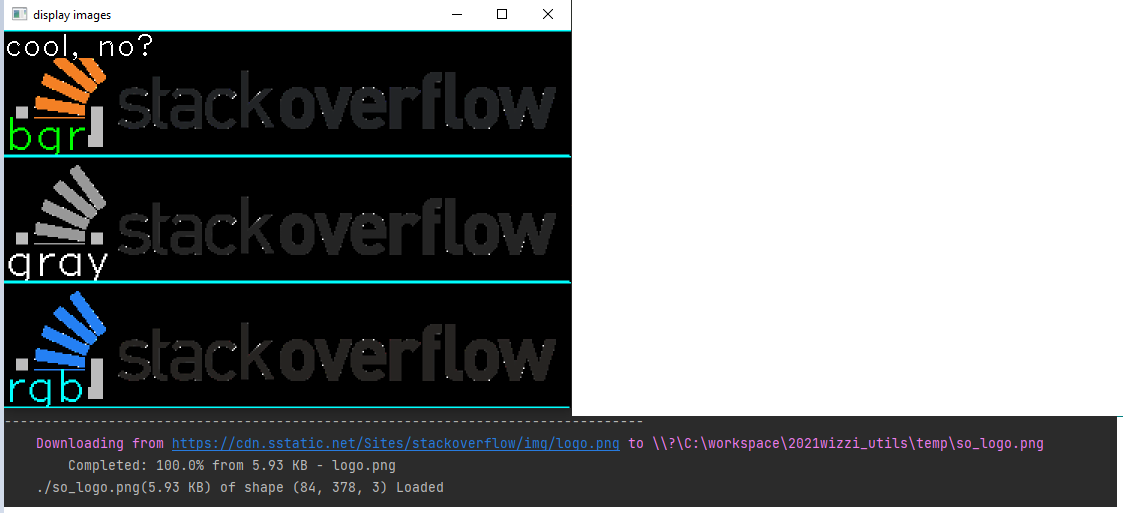\r\n\r\n```python\r\nimport wizzi_utils as wu\r\n\r\n\"\"\"\r\n* object detection models\r\n* pose detection models\r\n* tracking\r\n\"\"\"\r\nwu.models.test.test_cv2_object_detection_models()\r\nwu.models.test.test_cv2_pose_detection_models()\r\nwu.models.test.test_cv2_tracking_models()\r\n```\r\n\r\n<!---  -->\r\n<!---  -->\r\n<!---  -->\r\n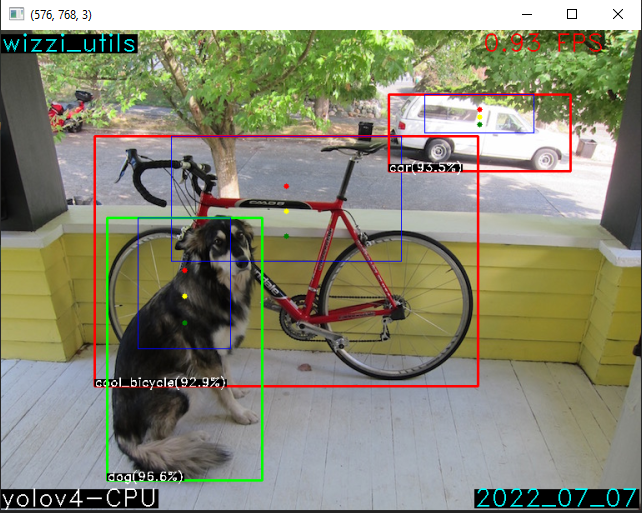\r\n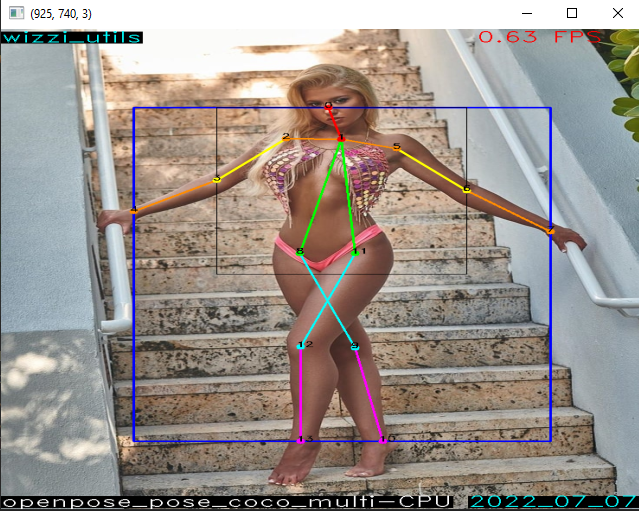\r\n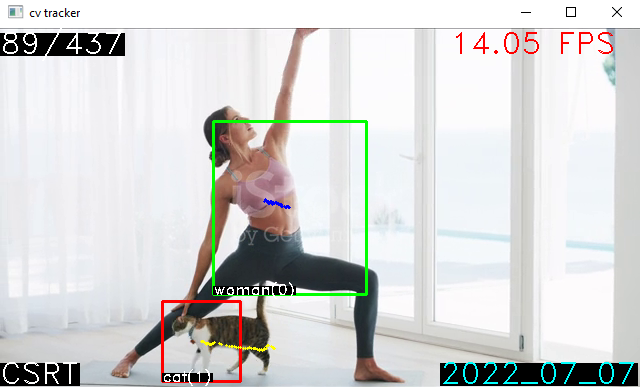\r\n\r\n```python\r\nimport wizzi_utils as wu\r\n\r\n# text to speak gui over pyttsx3 and pyQt5 packages\r\nwu.tts.test.run_machine_buddy_gui_test()\r\n```\r\n\r\n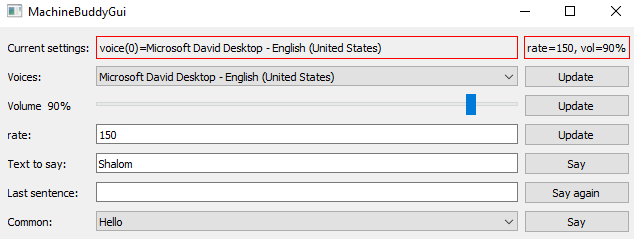\r\n\r\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "Debugging tools and fast coding",
"version": "8.0.6",
"project_urls": {
"Download": "https://github.com/2easy4wizzi/wizzi_utils_pypi/archive/refs/tags/v_8.0.6.tar.gz",
"Homepage": "https://github.com/2easy4wizzi/wizzi_utils_pypi"
},
"split_keywords": [
"debugging tools",
"json",
"open cv",
"pyplot",
"socket",
"torch",
"tf lite"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "ba47245715c89705365bbdae3a14d0a3d1a1a702cd4bc1a99320a022be032497",
"md5": "549f29f4c91fab144c2d05c4bd04af8f",
"sha256": "f699848160e1bd643ed6392b9c20e4bc91eebe7249ad1fc6155d2368b6654183"
},
"downloads": -1,
"filename": "wizzi_utils-8.0.6.tar.gz",
"has_sig": false,
"md5_digest": "549f29f4c91fab144c2d05c4bd04af8f",
"packagetype": "sdist",
"python_version": "source",
"requires_python": null,
"size": 161136,
"upload_time": "2023-07-01T10:00:48",
"upload_time_iso_8601": "2023-07-01T10:00:48.983991Z",
"url": "https://files.pythonhosted.org/packages/ba/47/245715c89705365bbdae3a14d0a3d1a1a702cd4bc1a99320a022be032497/wizzi_utils-8.0.6.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-07-01 10:00:48",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "2easy4wizzi",
"github_project": "wizzi_utils_pypi",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"lcname": "wizzi-utils"
}