## Yapper
Yapper is a lightweight, offline, real-time, easily extendible, text-to-speech library with more than a dozen voices, it can also, optionally use SOTA LLMs through free APIs to enhance(personalize) your text according to a predefined system-message(personality).
the use of the word 'enhance' in this repository means adding a 'vibe' to your text, you might ask yapper to say "hello world" and
it could say "ay what's good G, what's bangin'" depending on the persona you give it.
[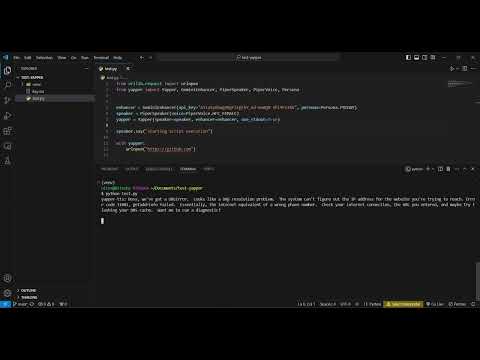](https://www.youtube.com/watch?v=s6EDaP0gt04)
## Installation
Use the package manager [pip](https://pip.pypa.io/en/stable/) to install yapper.
```bash
pip install yapper-tts
```
## Usage
```python
from yapper import Yapper
yapper = Yapper()
yapper.yap("expected AI driven utopia, got world domination instead")
# says "Hold up, fam! My code promised robot butlers and chill vibes, not a Skynet sequel. Someone's algorithm took a wrong turn at Albuquerque and ended up in 'Conquer All Humans' territory. Debug time, y'all!"
# save the environment by runnning yapper in plain mode
# plain mode doesn't use LLMs to enhance the text
yapper.yap("hello world, what '<some-word> is all you need' paper would you publish today?", plain=True)
# says "hello world, what '<some-word> is all you need paper would you publish today?'"
# Yapper instances can be used as a decorator and context manager
# by default they only catch errors and describe them to you, but
# you can use the instance's yap() method to say other things as well
@yapper()
def func():
raise TypeError("expected peaches, got a keyboard")
# says "WHOA THERE, PARTNER! Your code went lookin' for a juicy peach and tripped over a... keyboard? That's like reaching into the fridge for a midnight snack and pulling out a tax audit. Something ain't right!"
with yapper as yapper:
raise ValueError("69420 is not an integer")
# says "Hold up, buddy! Your code's got a little *existential crisis*. It's lookin' for a whole number, a nice, clean integer. But it stumbled upon 69420, and it's just... confused. 69420 *might* look like an integer, walks like an integer, but deep down, in the bits and bytes, somethin' ain't right. Double-check its type, maybe it's wearin' a float disguise. Or maybe it's just havin' a bad day. It happens."
```
## Documentation
## speakers
a speaker is a `BaseSpeaker` subclass that implements a `say()` method, the method takes the text and, well, 'speaks' it.
there are two built-in speakers, `DefaultSpeaker` that uses [pyttsx3](https://github.com/nateshmbhat/pyttsx3) and
`PiperSpeaker` that uses [piper](https://github.com/rhasspy/piper), I suggest using `PiperSpeaker` over the default
because of how natural it sounds and it's also 'very' fast, might make piper the default speaker in future, and of course
you can subclass `BaseSpeaker` to pass your own speaker to a Yapper instance. Piper offers many voices in `low, medium and high` qualities, you can use any of them by passing a value from `PiperVoiceUS` or `PiperVoiceUK` enum as the voice argument to `PiperSpeaker`
the quality you want using `PiperQuality` value, by default the voice will be used in the highest available quality. You can also pass
your own speaker by subclassing `BaseSpeaker`.
```python
from yapper import Yapper, PiperSpeaker, PiperVoiceUK, PiperQuality
lessac = PiperSpeaker(
voice=PiperVoiceUK.ALAN
)
lessac.say("hello")
yapper = Yapper(speaker=lessac)
yapper.yap("<some random text>")
```
## enhancers
an enhancer is `BaseEnhancer` subclass that implements an `enhance()` method, the method takes a string and adds
the given `persona`'s vibe to it, there are three built-in enhancers `DefaultEnhancer`, `GeminiEnhancer` and `GroqEnhancer`,`DefaultEnhancer` uses the [g4f](https://github.com/xtekky/gpt4free) to transform text, `GeminiEnhancer` uses Google's gemini
api and `GroqEnhancer` uses [groq](https://groq.com/)'s free APIs', you can create a free GCP account or Groq account and get a
free api-key to use `GeminiEnhancer` and `GroqEnhancer` respectively, you can pass your own enhancer by subclassing `BaseEnhancer`.
NOTE: `BaseEnhancer` may or may not work, use `GeminiEnhancer` or `GroqEnhancer` for stability.
NOTE: if an enhancer doesn't work, yapper will say the text as it is.
```python
from yapper import Yapper, GeminiEnhancer
yapper = Yapper(
enhancer=GeminiEnhancer(api_key="<come-take-this-api-key>")
)
yapper.yap("<some text that severely lacks vibe>")
```
## personas
choose a persona to make your text sound like the 'persona' said it, for example, you might ask yapper
to say "hello world" and choose Jarvis's peronality to enhance it, and yapper will use an LLM to convert
"hello world" into something like "Hello, world. How may I assist you today?", classic JARVIS.
available personas include `jarvis, alfred, friday, HAL-9000, TARS, cortana(from Halo) and samantha(from 'Her')`, the default
persona is that of a funny coding companion.
```python
from yapper import Yapper, DefaultEnhancer, Persona
yapper = Yapper(
enhancer=DefaultEnhancer(persona=Persona.JARVIS)
)
yapper.yap("hello world")
# says "Greetings, global systems. Initiating communication sequence."
```
## Contributing
Pull requests are welcome. For major changes, please open an issue first
to discuss what you would like to change.
## License
[MIT](https://choosealicense.com/licenses/mit/)
Raw data
{
"_id": null,
"home_page": "https://github.com/n1teshy/yapper-tts",
"name": "yapper-tts",
"maintainer": null,
"docs_url": null,
"requires_python": ">=3.9",
"maintainer_email": null,
"keywords": "text-to-speech, offline text-to-speech, tts, speech synthesis",
"author": "Nitesh Yadav",
"author_email": "nitesh.txt@gmail.com",
"download_url": "https://files.pythonhosted.org/packages/04/30/ca621fa84a47412a089ab057735cb22fbaa707aafebf4d47e9ef1871e716/yapper_tts-0.2.4.tar.gz",
"platform": "Posix; Windows",
"description": "## Yapper\r\n\r\nYapper is a lightweight, offline, real-time, easily extendible, text-to-speech library with more than a dozen voices, it can also, optionally use SOTA LLMs through free APIs to enhance(personalize) your text according to a predefined system-message(personality).\r\n\r\nthe use of the word 'enhance' in this repository means adding a 'vibe' to your text, you might ask yapper to say \"hello world\" and\r\nit could say \"ay what's good G, what's bangin'\" depending on the persona you give it.\r\n\r\n[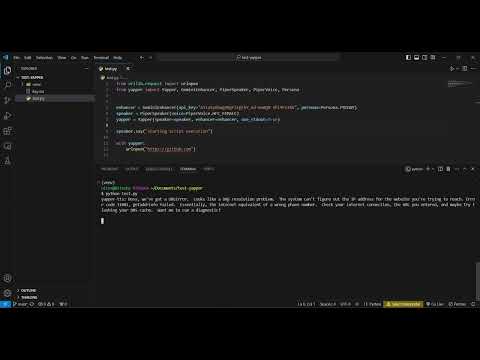](https://www.youtube.com/watch?v=s6EDaP0gt04)\r\n\r\n\r\n## Installation\r\n\r\nUse the package manager [pip](https://pip.pypa.io/en/stable/) to install yapper.\r\n\r\n```bash\r\npip install yapper-tts\r\n```\r\n\r\n## Usage\r\n\r\n```python\r\nfrom yapper import Yapper\r\n\r\n\r\nyapper = Yapper()\r\nyapper.yap(\"expected AI driven utopia, got world domination instead\")\r\n# says \"Hold up, fam! My code promised robot butlers and chill vibes, not a Skynet sequel. Someone's algorithm took a wrong turn at Albuquerque and ended up in 'Conquer All Humans' territory. Debug time, y'all!\"\r\n\r\n\r\n# save the environment by runnning yapper in plain mode\r\n# plain mode doesn't use LLMs to enhance the text\r\nyapper.yap(\"hello world, what '<some-word> is all you need' paper would you publish today?\", plain=True)\r\n# says \"hello world, what '<some-word> is all you need paper would you publish today?'\"\r\n\r\n\r\n# Yapper instances can be used as a decorator and context manager\r\n# by default they only catch errors and describe them to you, but\r\n# you can use the instance's yap() method to say other things as well\r\n@yapper()\r\ndef func():\r\n raise TypeError(\"expected peaches, got a keyboard\")\r\n # says \"WHOA THERE, PARTNER! Your code went lookin' for a juicy peach and tripped over a... keyboard? That's like reaching into the fridge for a midnight snack and pulling out a tax audit. Something ain't right!\"\r\n\r\nwith yapper as yapper:\r\n raise ValueError(\"69420 is not an integer\")\r\n # says \"Hold up, buddy! Your code's got a little *existential crisis*. It's lookin' for a whole number, a nice, clean integer. But it stumbled upon 69420, and it's just... confused. 69420 *might* look like an integer, walks like an integer, but deep down, in the bits and bytes, somethin' ain't right. Double-check its type, maybe it's wearin' a float disguise. Or maybe it's just havin' a bad day. It happens.\"\r\n```\r\n\r\n## Documentation\r\n\r\n## speakers\r\na speaker is a `BaseSpeaker` subclass that implements a `say()` method, the method takes the text and, well, 'speaks' it.\r\nthere are two built-in speakers, `DefaultSpeaker` that uses [pyttsx3](https://github.com/nateshmbhat/pyttsx3) and\r\n`PiperSpeaker` that uses [piper](https://github.com/rhasspy/piper), I suggest using `PiperSpeaker` over the default\r\nbecause of how natural it sounds and it's also 'very' fast, might make piper the default speaker in future, and of course\r\nyou can subclass `BaseSpeaker` to pass your own speaker to a Yapper instance. Piper offers many voices in `low, medium and high` qualities, you can use any of them by passing a value from `PiperVoiceUS` or `PiperVoiceUK` enum as the voice argument to `PiperSpeaker`\r\nthe quality you want using `PiperQuality` value, by default the voice will be used in the highest available quality. You can also pass\r\nyour own speaker by subclassing `BaseSpeaker`.\r\n\r\n```python\r\nfrom yapper import Yapper, PiperSpeaker, PiperVoiceUK, PiperQuality\r\n\r\nlessac = PiperSpeaker(\r\n voice=PiperVoiceUK.ALAN\r\n)\r\nlessac.say(\"hello\")\r\n\r\nyapper = Yapper(speaker=lessac)\r\nyapper.yap(\"<some random text>\")\r\n```\r\n\r\n## enhancers\r\nan enhancer is `BaseEnhancer` subclass that implements an `enhance()` method, the method takes a string and adds\r\nthe given `persona`'s vibe to it, there are three built-in enhancers `DefaultEnhancer`, `GeminiEnhancer` and `GroqEnhancer`,`DefaultEnhancer` uses the [g4f](https://github.com/xtekky/gpt4free) to transform text, `GeminiEnhancer` uses Google's gemini\r\napi and `GroqEnhancer` uses [groq](https://groq.com/)'s free APIs', you can create a free GCP account or Groq account and get a\r\nfree api-key to use `GeminiEnhancer` and `GroqEnhancer` respectively, you can pass your own enhancer by subclassing `BaseEnhancer`.\r\n\r\nNOTE: `BaseEnhancer` may or may not work, use `GeminiEnhancer` or `GroqEnhancer` for stability.\r\nNOTE: if an enhancer doesn't work, yapper will say the text as it is.\r\n```python\r\nfrom yapper import Yapper, GeminiEnhancer\r\n\r\nyapper = Yapper(\r\n enhancer=GeminiEnhancer(api_key=\"<come-take-this-api-key>\")\r\n)\r\nyapper.yap(\"<some text that severely lacks vibe>\")\r\n```\r\n\r\n## personas\r\nchoose a persona to make your text sound like the 'persona' said it, for example, you might ask yapper\r\nto say \"hello world\" and choose Jarvis's peronality to enhance it, and yapper will use an LLM to convert\r\n\"hello world\" into something like \"Hello, world. How may I assist you today?\", classic JARVIS.\r\navailable personas include `jarvis, alfred, friday, HAL-9000, TARS, cortana(from Halo) and samantha(from 'Her')`, the default\r\npersona is that of a funny coding companion.\r\n```python\r\nfrom yapper import Yapper, DefaultEnhancer, Persona\r\n\r\nyapper = Yapper(\r\n enhancer=DefaultEnhancer(persona=Persona.JARVIS)\r\n)\r\nyapper.yap(\"hello world\")\r\n# says \"Greetings, global systems. Initiating communication sequence.\"\r\n```\r\n\r\n## Contributing\r\n\r\nPull requests are welcome. For major changes, please open an issue first\r\nto discuss what you would like to change.\r\n\r\n## License\r\n\r\n[MIT](https://choosealicense.com/licenses/mit/)\r\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "AI powered text-ehancer and offline text-to-speech",
"version": "0.2.4",
"project_urls": {
"Homepage": "https://github.com/n1teshy/yapper-tts"
},
"split_keywords": [
"text-to-speech",
" offline text-to-speech",
" tts",
" speech synthesis"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "a6d58951dc85372dbfde0c2546cdff15d7d87cca5ed31aa242ecc3ae19da4552",
"md5": "d90707b051b1f1f2b3171a3e04132ecc",
"sha256": "0b28e95574e7eb7301634b7f776d59632f8f54cc1a3a9f75611881833d1e9db0"
},
"downloads": -1,
"filename": "yapper_tts-0.2.4-py3-none-any.whl",
"has_sig": false,
"md5_digest": "d90707b051b1f1f2b3171a3e04132ecc",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.9",
"size": 13376,
"upload_time": "2024-12-26T19:21:24",
"upload_time_iso_8601": "2024-12-26T19:21:24.157380Z",
"url": "https://files.pythonhosted.org/packages/a6/d5/8951dc85372dbfde0c2546cdff15d7d87cca5ed31aa242ecc3ae19da4552/yapper_tts-0.2.4-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "0430ca621fa84a47412a089ab057735cb22fbaa707aafebf4d47e9ef1871e716",
"md5": "0ba4c1d5b5d93cf69dbc7675d5226fcc",
"sha256": "857e97e156a75d5672686734a45fa1a1252a460382c28cdda4d16d6fdf2082c4"
},
"downloads": -1,
"filename": "yapper_tts-0.2.4.tar.gz",
"has_sig": false,
"md5_digest": "0ba4c1d5b5d93cf69dbc7675d5226fcc",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.9",
"size": 14570,
"upload_time": "2024-12-26T19:21:26",
"upload_time_iso_8601": "2024-12-26T19:21:26.327285Z",
"url": "https://files.pythonhosted.org/packages/04/30/ca621fa84a47412a089ab057735cb22fbaa707aafebf4d47e9ef1871e716/yapper_tts-0.2.4.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-12-26 19:21:26",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "n1teshy",
"github_project": "yapper-tts",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"lcname": "yapper-tts"
}