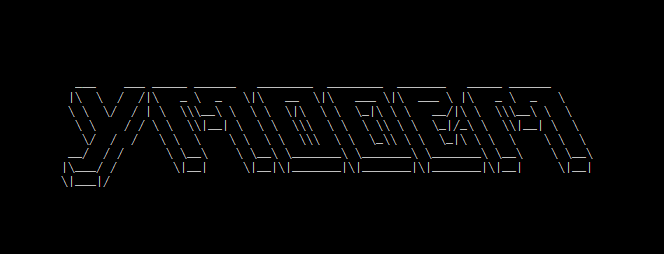
The YMODEM project is based on XMODEM implementation written by tehmaze. It is also compatible with XMODEM mode.
[](https://opensource.org/licenses/MIT)
README: [ENGLISH](https://github.com/alexwoo1900/ymodem/blob/master/README.md) | [简体中文](https://github.com/alexwoo1900/ymodem/blob/master/README_CN.md)
- [**Installation**](#installation)
- [**Usage**](#usage)
- [CLI TOOL](#cli-tool)
- [Sending a batch of files](#sending-a-batch-of-files)
- [Receive a file](#receive-a-file)
- [Source Code](#source-code)
- [API](#api)
- [Create MODEM Object](#create-modem-object)
- [Send files](#send-files)
- [Receive files](#receive-files)
- [Debug](#debug)
- [Changelog](#changelog)
- [License](#license)
## Demo
### Test the sending and receiving functions
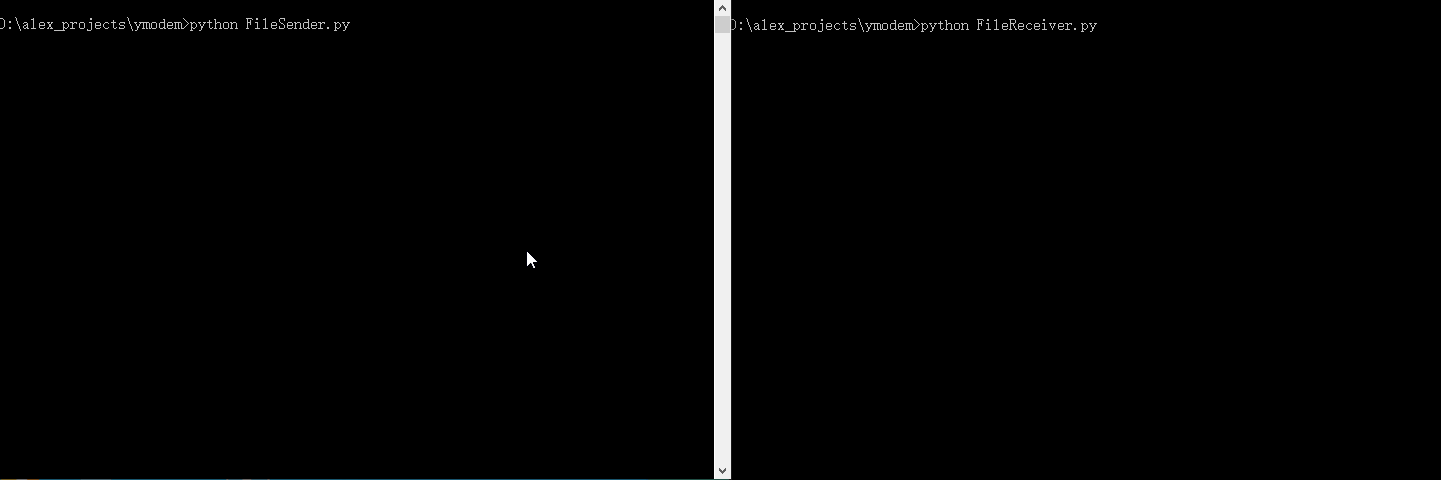
### Interact with SecureCRT
Interact with SecureCRT as sender
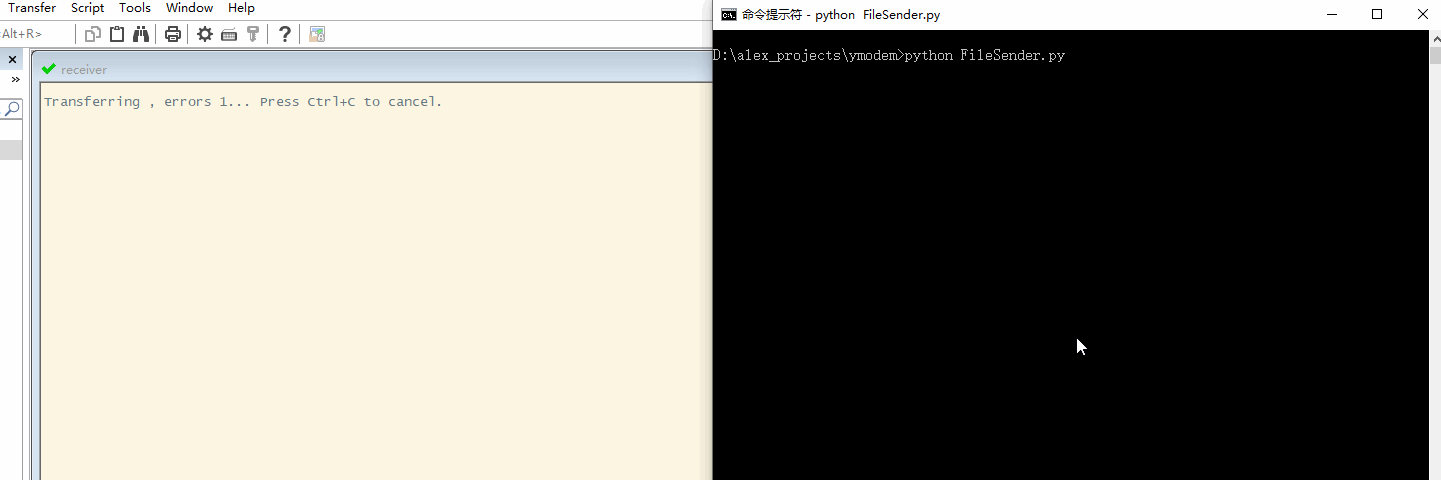
Interact with SecureCRT as Finder
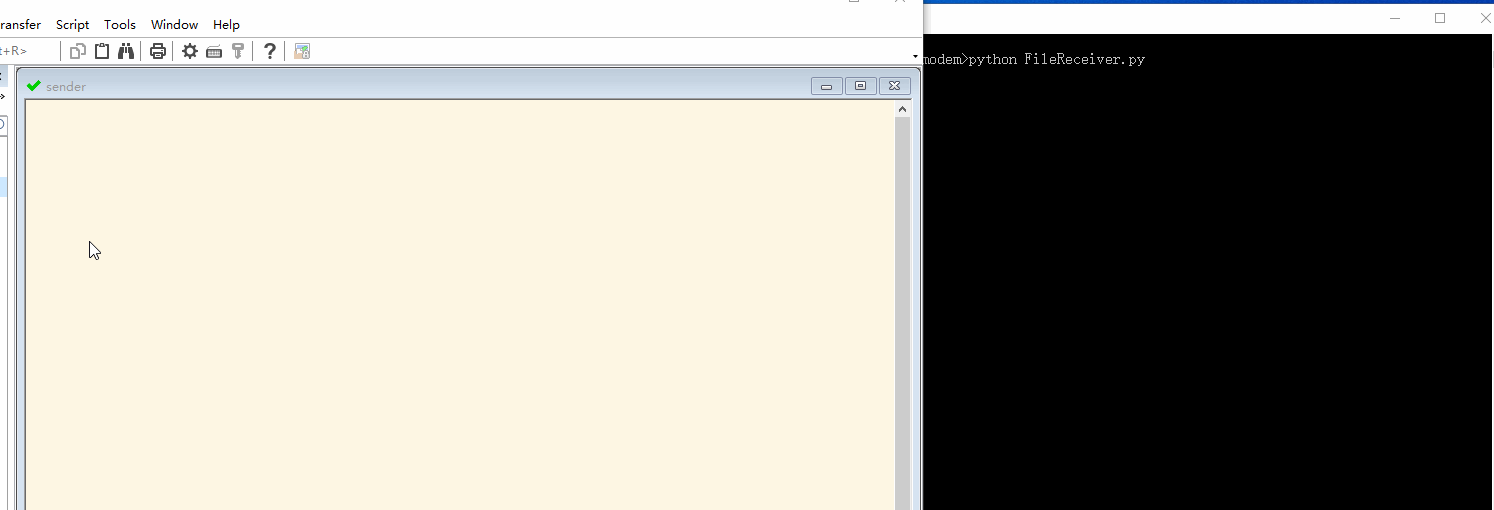
## Installation
```Bash
pip install ymodem
```
## Usage
### CLI TOOL
```Bash
# To get help
ymodem -h
# or
python -m ymodem -h
```
#### Sending a batch of files
```Bash
ymodem send ./file.bin ./file2.bin -p COM4 -b 115200
# or
python -m ymodem send ./file.bin ./file2.bin -p COM4 -b 115200
```
#### Receive a file
```Bash
ymodem recv ./ -p COM4 -b 115200
# or
python -m ymodem recv ./ -p COM4 -b 115200
```
### Source Code
```python
from ymodem.Socket import ModemSocket
# define read
def read(size, timeout = 3):
# implementation
# define write
def write(data, timeout = 3):
# implementation
# create socket
cli = ModemSocket(read, write)
# send multi files
cli.send([file_path1, file_path2, file_path3 ...])
# receive multi files
cli.recv(folder_path)
```
For more detailed usage, please refer to __main__.py.
### API
#### Create MODEM Object
```python
def __init__(self,
read: Callable[[int, Optional[float]], Any],
write: Callable[[Union[bytes, bytearray], Optional[float]], Any],
protocol_type: int = ProtocolType.YMODEM,
protocol_type_options: List[str] = [],
packet_size: int = 1024,
style_id: int = _psm.get_available_styles()[0]):
```
- protocol_type: Protocol type, see Protocol.py
- protocol_type_options: such as g representing the YMODEM-G in the YMODEM protocol.
- packet_size: The size of a single packet, 128/1024 bytes, may be adjusted depending on the protocol style
- style_id: Protocol style, different styles have different support for functional features
#### Send files
```python
def send(self,
paths: List[str],
callback: Optional[Callable[[int, str, int, int], None]] = None
) -> bool:
```
- callback: callback function. see below.
Parameter | Description
-|-
task index | index of current task
task (file) name | name of the file
total packets | number of packets plan to send
success packets | number of packets successfully sent
#### Receive files
```python
def recv(self,
path: str,
callback: Optional[Callable[[int, str, int, int], None]] = None
) -> bool:
```
- path: folder path for storing the target file
- callback: callback function. Same as the callback of send().
#### ATTENTION
Depending on different communication environments, developers may need to manually adjust timeout parameters in _read_and_wait or _write_and_wait.
## Debug
If you want to output debugging information, set the log level to DEBUG.
```python
logging.basicConfig(level=logging.DEBUG, format='%(message)s')
```
## Changelog
### v1.5 (2024/02/03)
- Added cli tool to iteract with YMODEM via Serial bus
### v1.5 (2023/05/20 11:00 +00:00)
- Rewritten send() and recv()
- Support YMODEM-G.
The success rate of YMODEM-G based on pyserial depends on the user's OS, and after testing, the success rate is very low without any delay.
## License
[MIT License](https://opensource.org/licenses/MIT)
Raw data
{
"_id": null,
"home_page": null,
"name": "ymodem",
"maintainer": null,
"docs_url": null,
"requires_python": ">=3.7",
"maintainer_email": null,
"keywords": "pyserial, serial, xmodem, ymodem, python, python3",
"author": null,
"author_email": null,
"download_url": "https://files.pythonhosted.org/packages/df/db/d96a2c259933916bc5062b8f8a297493fcbe44bd9a0f42579cc5f532f9b2/ymodem-1.5.1.tar.gz",
"platform": null,
"description": "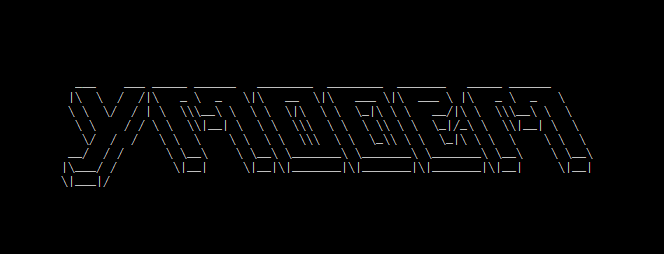\n\nThe YMODEM project is based on XMODEM implementation written by tehmaze. It is also compatible with XMODEM mode.\n\n[](https://opensource.org/licenses/MIT)\n\n\nREADME: [ENGLISH](https://github.com/alexwoo1900/ymodem/blob/master/README.md) | [\u7b80\u4f53\u4e2d\u6587](https://github.com/alexwoo1900/ymodem/blob/master/README_CN.md)\n\n- [**Installation**](#installation)\n- [**Usage**](#usage)\n - [CLI TOOL](#cli-tool) \n - [Sending a batch of files](#sending-a-batch-of-files)\n - [Receive a file](#receive-a-file)\n - [Source Code](#source-code)\n - [API](#api)\n - [Create MODEM Object](#create-modem-object)\n - [Send files](#send-files)\n - [Receive files](#receive-files)\n- [Debug](#debug)\n- [Changelog](#changelog)\n- [License](#license)\n\n## Demo\n\n### Test the sending and receiving functions\n\n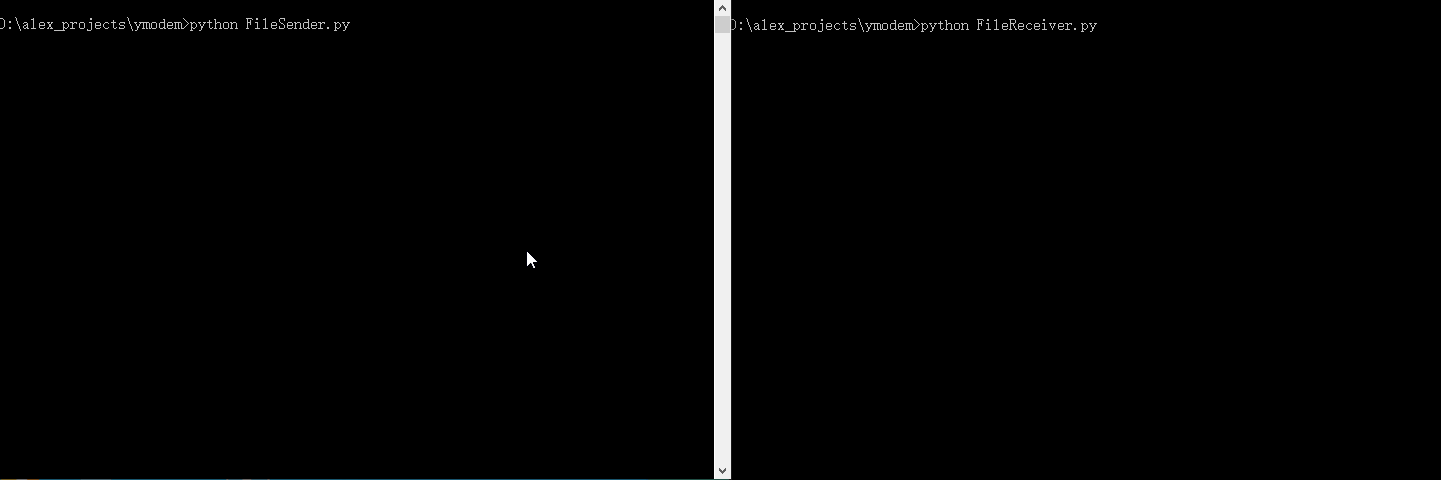\n\n### Interact with SecureCRT\n\nInteract with SecureCRT as sender\n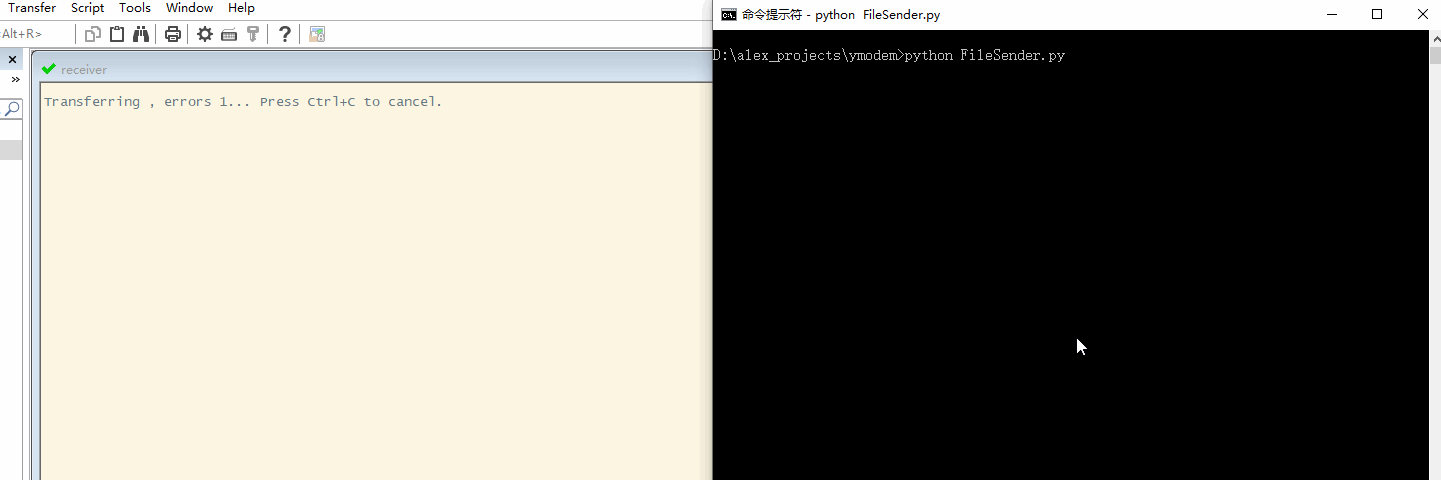\n\nInteract with SecureCRT as Finder\n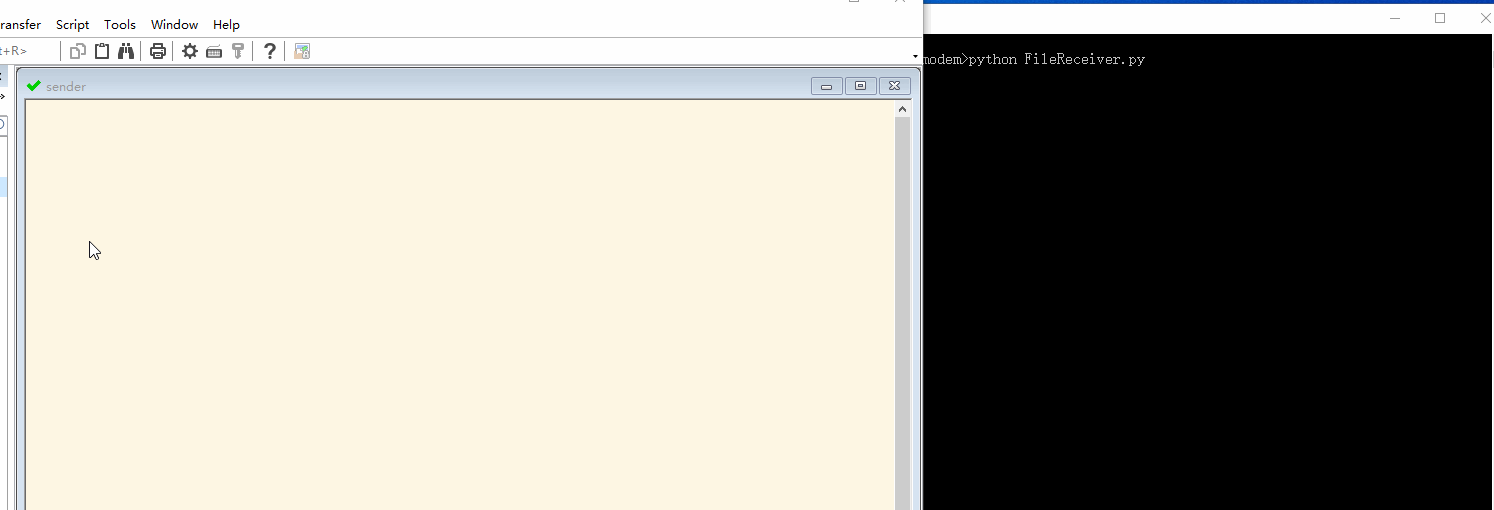\n\n## Installation\n```Bash\npip install ymodem\n```\n\n## Usage\n\n### CLI TOOL\n\n```Bash\n# To get help\nymodem -h\n# or\npython -m ymodem -h\n```\n\n#### Sending a batch of files\n```Bash\nymodem send ./file.bin ./file2.bin -p COM4 -b 115200\n# or\npython -m ymodem send ./file.bin ./file2.bin -p COM4 -b 115200\n```\n\n#### Receive a file\n```Bash\nymodem recv ./ -p COM4 -b 115200\n# or\npython -m ymodem recv ./ -p COM4 -b 115200\n```\n\n### Source Code\n\n```python\nfrom ymodem.Socket import ModemSocket\n\n# define read\ndef read(size, timeout = 3):\n # implementation\n\n# define write\ndef write(data, timeout = 3):\n # implementation\n\n# create socket\ncli = ModemSocket(read, write)\n\n# send multi files\ncli.send([file_path1, file_path2, file_path3 ...])\n\n# receive multi files\ncli.recv(folder_path)\n```\n\nFor more detailed usage, please refer to __main__.py.\n\n\n### API\n\n#### Create MODEM Object\n\n```python\ndef __init__(self, \n read: Callable[[int, Optional[float]], Any], \n write: Callable[[Union[bytes, bytearray], Optional[float]], Any], \n protocol_type: int = ProtocolType.YMODEM, \n protocol_type_options: List[str] = [],\n packet_size: int = 1024,\n style_id: int = _psm.get_available_styles()[0]):\n```\n- protocol_type: Protocol type, see Protocol.py\n- protocol_type_options: such as g representing the YMODEM-G in the YMODEM protocol.\n- packet_size: The size of a single packet, 128/1024 bytes, may be adjusted depending on the protocol style\n- style_id: Protocol style, different styles have different support for functional features\n\n#### Send files\n\n```python\ndef send(self, \n paths: List[str], \n callback: Optional[Callable[[int, str, int, int], None]] = None\n ) -> bool:\n```\n\n- callback: callback function. see below.\n\n Parameter | Description\n -|-\n task index | index of current task\n task (file) name | name of the file\n total packets | number of packets plan to send\n success packets | number of packets successfully sent\n\n#### Receive files\n\n```python\ndef recv(self, \n path: str, \n callback: Optional[Callable[[int, str, int, int], None]] = None\n ) -> bool:\n```\n- path: folder path for storing the target file\n- callback: callback function. Same as the callback of send().\n\n#### ATTENTION\n\nDepending on different communication environments, developers may need to manually adjust timeout parameters in _read_and_wait or _write_and_wait.\n\n## Debug\n\nIf you want to output debugging information, set the log level to DEBUG.\n\n```python\nlogging.basicConfig(level=logging.DEBUG, format='%(message)s')\n```\n\n## Changelog\n\n### v1.5 (2024/02/03)\n\n- Added cli tool to iteract with YMODEM via Serial bus\n\n\n### v1.5 (2023/05/20 11:00 +00:00)\n\n- Rewritten send() and recv()\n- Support YMODEM-G. \n The success rate of YMODEM-G based on pyserial depends on the user's OS, and after testing, the success rate is very low without any delay.\n\n## License\n[MIT License](https://opensource.org/licenses/MIT)\n",
"bugtrack_url": null,
"license": null,
"summary": "Ymodem Python3 implementation",
"version": "1.5.1",
"project_urls": {
"Bug Reports": "https://github.com/alexwoo1900/ymodem/issues",
"Homepage": "https://github.com/alexwoo1900/ymodem",
"Source": "https://github.com/alexwoo1900/ymodem"
},
"split_keywords": [
"pyserial",
" serial",
" xmodem",
" ymodem",
" python",
" python3"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "90449f09d0efaddcc253b4b35fbe26bf419aa40c15f0554df3fbffdf5f5404a3",
"md5": "b758206a73f8fa92ba5bcba1d7cd0964",
"sha256": "55fccfe7243e35bc96d70d6c8778f4ddd1a23f61ea5404cf30470cc5ffe900b3"
},
"downloads": -1,
"filename": "ymodem-1.5.1-py3-none-any.whl",
"has_sig": false,
"md5_digest": "b758206a73f8fa92ba5bcba1d7cd0964",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.7",
"size": 21417,
"upload_time": "2024-05-15T07:29:47",
"upload_time_iso_8601": "2024-05-15T07:29:47.755019Z",
"url": "https://files.pythonhosted.org/packages/90/44/9f09d0efaddcc253b4b35fbe26bf419aa40c15f0554df3fbffdf5f5404a3/ymodem-1.5.1-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "dfdbd96a2c259933916bc5062b8f8a297493fcbe44bd9a0f42579cc5f532f9b2",
"md5": "643e809558a4393936564ac6594ee9d6",
"sha256": "e4373cd6c8d29629495dbffdd9ed187b07f27381231d55d3a137025320d5ce67"
},
"downloads": -1,
"filename": "ymodem-1.5.1.tar.gz",
"has_sig": false,
"md5_digest": "643e809558a4393936564ac6594ee9d6",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.7",
"size": 22149,
"upload_time": "2024-05-15T07:29:48",
"upload_time_iso_8601": "2024-05-15T07:29:48.887664Z",
"url": "https://files.pythonhosted.org/packages/df/db/d96a2c259933916bc5062b8f8a297493fcbe44bd9a0f42579cc5f532f9b2/ymodem-1.5.1.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-05-15 07:29:48",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "alexwoo1900",
"github_project": "ymodem",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"lcname": "ymodem"
}