Name | zipline-tej JSON |
Version |
2.1.0
JSON |
| download |
home_page | None |
Summary | A Pythonic backtester for trading algorithms |
upload_time | 2024-10-30 01:15:53 |
maintainer | tej api Development Team |
docs_url | None |
author | tej |
requires_python | >=3.8 |
license | Apache-2.0 |
keywords |
|
VCS |
 |
bugtrack_url |
|
requirements |
No requirements were recorded.
|
Travis-CI |
No Travis.
|
coveralls test coverage |
No coveralls.
|
# Installation
## Used packages and environment
* Main package: Zipline
* Python 3.8 or above (currently support up to 3.11)
* Microsoft Windows OS or macOS or Linux
* Other Python dependency packages: Pandas, Numpy, Logbook, Exchange-calendars, etc.
## How to install Zipline Reloaded modified by TEJ
* We're going to illustrate under anaconda environment, so we suggest using [Anaconda](https://www.anaconda.com/data-science-platform) as development environment.
* Download dependency packages.
1. Windows [(zipline-tej.yml)](https://minhaskamal.github.io/DownGit/#/home?url=https://github.com/tejtw/zipline-tej/blob/main/zipline-tej.yml)
2. Mac [(zipline-tej_mac.yml)](https://minhaskamal.github.io/DownGit/#/home?url=https://github.com/tejtw/zipline-tej/blob/main/zipline-tej_mac.yml)
* Start an Anaconda (base) prompt, create an virtual environment and install the appropriate versions of packages:
(We **strongly** recommand using virtual environment to keep every project independent.) [(reason)](https://csguide.cs.princeton.edu/software/virtualenv#definition)
```
Windows Users
# change directionary to the folder exists zipline-tej.yml
$ cd <C:\Users\username\Downloads>
# create virtual env
$ conda env create -f zipline-tej.yml
# activate virtual env
$ conda activate zipline-tej
Mac Users
# change directionary to the folder exists zipline-tej_mac.yml
$ cd <C:\Users\username\Downloads>
# create virtual env
$ conda env create -f zipline-tej_mac.yml
# activate virtual env
$ conda activate zipline-tej
```
Also, if you are familiar with Python enough, you can create a virtual environment without zipline-tej.yml and here's the sample :
```
# create virtual env
$ conda create -n <env_name> python=3.10
# activate virtual env
$ conda activate <env_name>
# download dependency packages
$ pip install zipline-tej
```
While encountering environment problems, we provided a consistent and stable environment on [Docker hub](https://hub.docker.com/).
For users that using docker, we briefly introduce how to download and use it.
First of all, please download and install [docker-desktop](https://www.docker.com/products/docker-desktop/).
```
1. Start docker-desktop. (Registration is not must.)
2. Select the "images" on the leftside and search "tej87681088/tquant" and click "Pull".
3. After the image was downloaded, click the "run" icon the enter the optional settings.
3-1. Contaner-name: whatever you want.
3-2. Ports: the port to connect, "8888" is recommended.
3-3. Volumes: the place to store files. (You can create volume first on the left side.)
e.g. created a volume named "data", host path enter "data", container path "/app" is recommended.
4. Select the "Containers" leftside, the click the one which its image name is tej87681088/tquant
5. In its "Logs" would show an url like
http://127.0.0.1:8888/tree?token=XXXXXXXXXXXXXXXXXXXXXXXXXXXXXX
6. Go to your browser and enter "http://127.0.0.1:<port_you_set_in_step_3-2>/tree?token=XXXXXXXXXXXXXXXXXXXXXXXXXXXXXX"
6-1. If your port is 8888, you can just click the hyperlink.
7. Start develop your strategy!
NOTICE: Next time, we just need to reproduce step4 to step6.
```
# Quick start
## CLI Interface
The following code implements a simple buy-and-hold trading algorithm.
```python
from zipline.api import order, record, symbol
def initialize(context):
context.asset = symbol("2330")
def handle_data(context, data):
order(context.asset, 10)
record(TSMC=data.current(context.asset, "price"))
def analyze(context=None, results=None):
import matplotlib.pyplot as plt
# Plot the portfolio and asset data.
ax1 = plt.subplot(211)
results.portfolio_value.plot(ax=ax1)
ax1.set_ylabel("Portfolio value (TWD)")
ax2 = plt.subplot(212, sharex=ax1)
results.TSMC.plot(ax=ax2)
ax2.set_ylabel("TSMC price (TWD)")
# Show the plot.
plt.gcf().set_size_inches(18, 8)
plt.show()
```
You can then run this algorithm using the Zipline CLI. But first, you need to download some market data with historical prices and trading volumes:
* Before ingesting data, you have to set some environment variables as follow:
```
# setting TEJAPI_KEY to get permissions loading data
$ set TEJAPI_KEY=<your_key>
$ set TEJAPI_BASE=https://api.tej.com.tw
# setting download ticker
$ set ticker=2330 2317
# setting backtest period
$ set mdate=20200101 20220101
```
* Ingest and run backtesting algorithm
```
$ zipline ingest -b tquant
$ zipline run -f buy_and_hold.py --start 20200101 --end 20220101 -o bah.pickle --no-benchmark --no-treasury
```
Then, the resulting performance DataFrame is saved as bah.pickle, which you can load and analyze from Python.
### More useful zipline commands
Before calling **zipline** in CLI, be sure that TEJAPI_KEY and TEJAPI_BASE were set.
Use **zipline --help** to get more information.
For example :
We want to know how to use **zipline run**, we can run as follow:
```
zipline run --help
```
```
Usage: zipline run [OPTIONS]
Run a backtest for the given algorithm.
Options:
-f, --algofile FILENAME The file that contains the algorithm to run.
-t, --algotext TEXT The algorithm script to run.
-D, --define TEXT Define a name to be bound in the namespace
before executing the algotext. For example
'-Dname=value'. The value may be any python
expression. These are evaluated in order so
they may refer to previously defined names.
--data-frequency [daily|minute]
The data frequency of the simulation.
[default: daily]
--capital-base FLOAT The starting capital for the simulation.
[default: 10000000.0]
-b, --bundle BUNDLE-NAME The data bundle to use for the simulation.
[default: tquant]
--bundle-timestamp TIMESTAMP The date to lookup data on or before.
[default: <current-time>]
-bf, --benchmark-file FILE The csv file that contains the benchmark
returns
--benchmark-symbol TEXT The symbol of the instrument to be used as a
benchmark (should exist in the ingested
bundle)
--benchmark-sid INTEGER The sid of the instrument to be used as a
benchmark (should exist in the ingested
bundle)
--no-benchmark If passed, use a benchmark of zero returns.
-bf, --treasury-file FILE The csv file that contains the treasury
returns
--treasury-symbol TEXT The symbol of the instrument to be used as a
treasury (should exist in the ingested
bundle)
--treasury-sid INTEGER The sid of the instrument to be used as a
treasury (should exist in the ingested
bundle)
--no-treasury If passed, use a treasury of zero returns.
-s, --start DATE The start date of the simulation.
-e, --end DATE The end date of the simulation.
-o, --output FILENAME The location to write the perf data. If this
is '-' the perf will be written to stdout.
[default: -]
--trading-calendar TRADING-CALENDAR
The calendar you want to use e.g. TEJ_XTAI.
TEJ_XTAI is the default.
--print-algo / --no-print-algo Print the algorithm to stdout.
--metrics-set TEXT The metrics set to use. New metrics sets may
be registered in your extension.py.
--blotter TEXT The blotter to use. [default: default]
--help Show this message and exit.
```
### Difference of tquant and fundamentals
* Basically, `tquant` is the one that only contain OHLCV and cash dividend date. And `fundamentals` is the data that exclude from OHLCV, like EPS, gross margin, operating income, etc.
* So in both fundamentals and tquant, we can add tickers as follow :
#### Add tickers
```
$ zipline add -t "<ticker_wants_to_add>"
```
If tickers are more than 1 ticker, split them apart by " " or "," or ";".
#### [fundamentals only] Add fields
```
$ zipline add -f "<field_wants_to_add>"
```
* NOTICE that you `CAN'T` add field and ticker simultaneously.
For more detail use **zipline add --help** .
#### Display bundle-info
```
$ zipline bundle-info
```
To show what the tickers are there in newest bundle.
For more detail use **zipline bundle-info --help** .
#### Switch bundle
Before using switch, use **zipline bundles** to get the timestamp of each folder.
```
$ zipline switch -t "<The_timestamp_of_the_folder_want_to_use>"
```
Due to zipline only using the newest folder, switch can make previous folder become newest.
For more detail use **zipline switch --help** .
#### Update bundle
* You can either update `tquant` or `fundamentals` by using -b to select which one you want to update the bundle information to newest date.[DEFAULT:tquant]
```
$ zipline update -b tquant
$ zipline update -b fundamentals
```
For more detail use **zipline update --help** .
## Jupyter Notebook
### Change Anaconda kernel
* Since we've downloaded package "nb_conda_kernels", we should be able to change kernel in jupyter notebook.
#### How to new a notebook using specific kernel
(1) Open anaconda prompt
(2) Enter the command as follow :
```
# First one can be ignore if already in environment of zipline-tej
$ conda activate zipline-tej
# start a jupyter notebook
$ jupyter notebook
```
(3) Start a notebook and select Python[conda env:zipline-tej]
(4)(Optional) If you have already written a notebook, you can open it and change kernel by clicking the "Kernel" in menu and "Change kernel" to select the specfic kernel.
### Set environment variables TEJAPI_KEY, ticker and mdate
\* ticker would be your target ticker symbol, and it should be a string. If there're more than one ticker needed, use " ", "," or ";" to split them apart.
\* mdate refers the begin date and end date, use " ", "," or ";" to split them apart.
```python
In[1]:
import os
os.environ['TEJAPI_KEY'] = <your_key>
os.environ['ticker'] ='2330 2317'
os.environ['mdate'] ='20200101 20220101'
```
### Call ingest to download data to ~\\\.zipline
```python
In[2]:
!zipline ingest -b tquant
[Out]:
Merging daily equity files:
[YYYY-MM-DD HH:mm:ss.ssssss] INFO: zipline.data.bundles.core: Ingesting tquant.
```
### Design the backtesting strategy
```python
In[3]:
from zipline.api import order, record, symbol
def initialize(context):
context.asset = symbol("2330")
def handle_data(context, data):
order(context.asset, 10)
record(TSMC=data.current(context.asset, "price"))
def analyze(context=None, results=None):
import matplotlib.pyplot as plt
# Plot the portfolio and asset data.
ax1 = plt.subplot(211)
results.portfolio_value.plot(ax=ax1)
ax1.set_ylabel("Portfolio value (TWD)")
ax2 = plt.subplot(212, sharex=ax1)
results.TSMC.plot(ax=ax2)
ax2.set_ylabel("TSMC price (TWD)")
# Show the plot.
plt.gcf().set_size_inches(18, 8)
plt.show()
```
### Run backtesting algorithm and plot
```python
In[4]:
from zipline import run_algorithm
import pandas as pd
from zipline.utils.calendar_utils import get_calendar
trading_calendar = get_calendar('TEJ_XTAI')
start = pd.Timestamp('20200103', tz ='utc' )
end = pd.Timestamp('20211230', tz='utc')
result = run_algorithm(start=start,
end=end,
initialize=initialize,
capital_base=1000000,
handle_data=handle_data,
bundle='tquant',
trading_calendar=trading_calendar,
analyze=analyze,
data_frequency='daily'
)
[Out]:
```
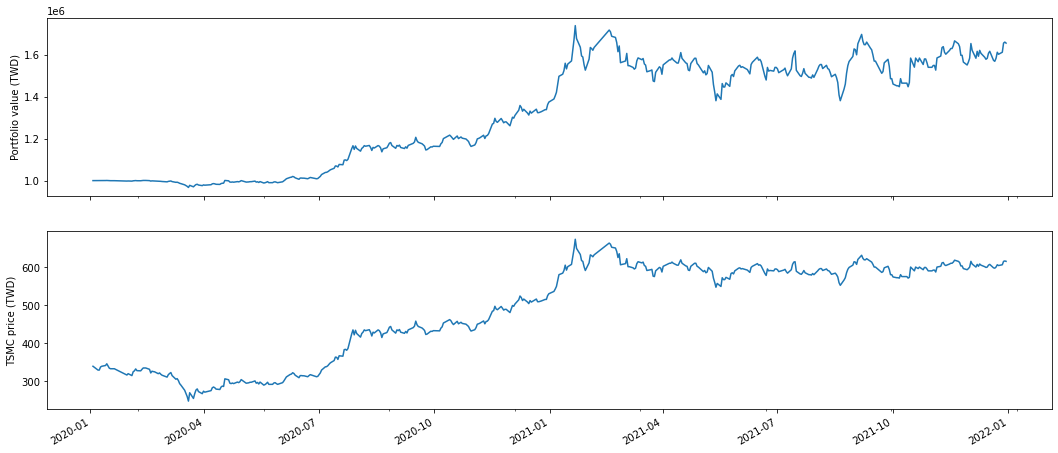
### Show trading process
```python
In[5]:
result
[Out]:
```
<div>
<table border="1" class="dataframe">
<thead>
<tr style="text-align: right;">
<th></th>
<th>period_open</th>
<th>period_close</th>
<th>starting_value</th>
<th>ending_value</th>
<th>starting_cash</th>
<th>ending_cash</th>
<th>portfolio_value</th>
<th>longs_count</th>
<th>shorts_count</th>
<th>long_value</th>
<th>...</th>
<th>treasury_period_return</th>
<th>trading_days</th>
<th>period_label</th>
<th>algo_volatility</th>
<th>benchmark_period_return</th>
<th>benchmark_volatility</th>
<th>algorithm_period_return</th>
<th>alpha</th>
<th>beta</th>
<th>sharpe</th>
</tr>
</thead>
<tbody>
<tr>
<th>2020-01-03 05:30:00+00:00</th>
<td>2020-01-03 01:01:00+00:00</td>
<td>2020-01-03 05:30:00+00:00</td>
<td>0.0</td>
<td>0.0</td>
<td>1.000000e+06</td>
<td>1.000000e+06</td>
<td>1.000000e+06</td>
<td>0</td>
<td>0</td>
<td>0.0</td>
<td>...</td>
<td>0.0</td>
<td>1</td>
<td>2020-01</td>
<td>NaN</td>
<td>0.0</td>
<td>NaN</td>
<td>0.000000</td>
<td>None</td>
<td>None</td>
<td>NaN</td>
</tr>
<tr>
<th>2020-01-06 05:30:00+00:00</th>
<td>2020-01-06 01:01:00+00:00</td>
<td>2020-01-06 05:30:00+00:00</td>
<td>0.0</td>
<td>3320.0</td>
<td>1.000000e+06</td>
<td>9.966783e+05</td>
<td>9.999983e+05</td>
<td>1</td>
<td>0</td>
<td>3320.0</td>
<td>...</td>
<td>0.0</td>
<td>2</td>
<td>2020-01</td>
<td>0.000019</td>
<td>0.0</td>
<td>0.0</td>
<td>-0.000002</td>
<td>None</td>
<td>None</td>
<td>-11.224972</td>
</tr>
<tr>
<th>2020-01-07 05:30:00+00:00</th>
<td>2020-01-07 01:01:00+00:00</td>
<td>2020-01-07 05:30:00+00:00</td>
<td>3320.0</td>
<td>6590.0</td>
<td>9.966783e+05</td>
<td>9.933817e+05</td>
<td>9.999717e+05</td>
<td>1</td>
<td>0</td>
<td>6590.0</td>
<td>...</td>
<td>0.0</td>
<td>3</td>
<td>2020-01</td>
<td>0.000237</td>
<td>0.0</td>
<td>0.0</td>
<td>-0.000028</td>
<td>None</td>
<td>None</td>
<td>-10.038514</td>
</tr>
<tr>
<th>2020-01-08 05:30:00+00:00</th>
<td>2020-01-08 01:01:00+00:00</td>
<td>2020-01-08 05:30:00+00:00</td>
<td>6590.0</td>
<td>9885.0</td>
<td>9.933817e+05</td>
<td>9.900850e+05</td>
<td>9.999700e+05</td>
<td>1</td>
<td>0</td>
<td>9885.0</td>
<td>...</td>
<td>0.0</td>
<td>4</td>
<td>2020-01</td>
<td>0.000203</td>
<td>0.0</td>
<td>0.0</td>
<td>-0.000030</td>
<td>None</td>
<td>None</td>
<td>-9.298128</td>
</tr>
<tr>
<th>2020-01-09 05:30:00+00:00</th>
<td>2020-01-09 01:01:00+00:00</td>
<td>2020-01-09 05:30:00+00:00</td>
<td>9885.0</td>
<td>13500.0</td>
<td>9.900850e+05</td>
<td>9.867083e+05</td>
<td>1.000208e+06</td>
<td>1</td>
<td>0</td>
<td>13500.0</td>
<td>...</td>
<td>0.0</td>
<td>5</td>
<td>2020-01</td>
<td>0.001754</td>
<td>0.0</td>
<td>0.0</td>
<td>0.000208</td>
<td>None</td>
<td>None</td>
<td>5.986418</td>
</tr>
<tr>
<th>...</th>
<td>...</td>
<td>...</td>
<td>...</td>
<td>...</td>
<td>...</td>
<td>...</td>
<td>...</td>
<td>...</td>
<td>...</td>
<td>...</td>
<td>...</td>
<td>...</td>
<td>...</td>
<td>...</td>
<td>...</td>
<td>...</td>
<td>...</td>
<td>...</td>
<td>...</td>
<td>...</td>
<td>...</td>
</tr>
<tr>
<th>2021-12-24 05:30:00+00:00</th>
<td>2021-12-24 01:01:00+00:00</td>
<td>2021-12-24 05:30:00+00:00</td>
<td>2920920.0</td>
<td>2917320.0</td>
<td>-1.308854e+06</td>
<td>-1.314897e+06</td>
<td>1.602423e+06</td>
<td>1</td>
<td>0</td>
<td>2917320.0</td>
<td>...</td>
<td>0.0</td>
<td>484</td>
<td>2021-12</td>
<td>0.232791</td>
<td>0.0</td>
<td>0.0</td>
<td>0.602423</td>
<td>None</td>
<td>None</td>
<td>1.170743</td>
</tr>
<tr>
<th>2021-12-27 05:30:00+00:00</th>
<td>2021-12-27 01:01:00+00:00</td>
<td>2021-12-27 05:30:00+00:00</td>
<td>2917320.0</td>
<td>2933040.0</td>
<td>-1.314897e+06</td>
<td>-1.320960e+06</td>
<td>1.612080e+06</td>
<td>1</td>
<td>0</td>
<td>2933040.0</td>
<td>...</td>
<td>0.0</td>
<td>485</td>
<td>2021-12</td>
<td>0.232577</td>
<td>0.0</td>
<td>0.0</td>
<td>0.612080</td>
<td>None</td>
<td>None</td>
<td>1.182864</td>
</tr>
<tr>
<th>2021-12-28 05:30:00+00:00</th>
<td>2021-12-28 01:01:00+00:00</td>
<td>2021-12-28 05:30:00+00:00</td>
<td>2933040.0</td>
<td>2982750.0</td>
<td>-1.320960e+06</td>
<td>-1.327113e+06</td>
<td>1.655637e+06</td>
<td>1</td>
<td>0</td>
<td>2982750.0</td>
<td>...</td>
<td>0.0</td>
<td>486</td>
<td>2021-12</td>
<td>0.233086</td>
<td>0.0</td>
<td>0.0</td>
<td>0.655637</td>
<td>None</td>
<td>None</td>
<td>1.237958</td>
</tr>
<tr>
<th>2021-12-29 05:30:00+00:00</th>
<td>2021-12-29 01:01:00+00:00</td>
<td>2021-12-29 05:30:00+00:00</td>
<td>2982750.0</td>
<td>2993760.0</td>
<td>-1.327113e+06</td>
<td>-1.333276e+06</td>
<td>1.660484e+06</td>
<td>1</td>
<td>0</td>
<td>2993760.0</td>
<td>...</td>
<td>0.0</td>
<td>487</td>
<td>2021-12</td>
<td>0.232850</td>
<td>0.0</td>
<td>0.0</td>
<td>0.660484</td>
<td>None</td>
<td>None</td>
<td>1.243176</td>
</tr>
<tr>
<th>2021-12-30 05:30:00+00:00</th>
<td>2021-12-30 01:01:00+00:00</td>
<td>2021-12-30 05:30:00+00:00</td>
<td>2993760.0</td>
<td>2995050.0</td>
<td>-1.333276e+06</td>
<td>-1.339430e+06</td>
<td>1.655620e+06</td>
<td>1</td>
<td>0</td>
<td>2995050.0</td>
<td>...</td>
<td>0.0</td>
<td>488</td>
<td>2021-12</td>
<td>0.232629</td>
<td>0.0</td>
<td>0.0</td>
<td>0.655620</td>
<td>None</td>
<td>None</td>
<td>1.235305</td>
</tr>
</tbody>
</table>
<p>488 rows × 38 columns</p>
</div>
---
# More Zipline Tutorials
* For more [tutorials](https://github.com/tejtw/TQuant-Lab)
# Suggestions
* To get TEJAPI_KEY [(link)](https://api.tej.com.tw/trial.html)
* [TEJ Official Website](https://www.tej.com.tw/)
Raw data
{
"_id": null,
"home_page": null,
"name": "zipline-tej",
"maintainer": "tej api Development Team",
"docs_url": null,
"requires_python": ">=3.8",
"maintainer_email": "tej@tej.com.tw",
"keywords": null,
"author": "tej",
"author_email": "tej@tej.com.tw",
"download_url": null,
"platform": null,
"description": "# Installation\n\n## Used packages and environment\n* Main package: Zipline\n* Python 3.8 or above (currently support up to 3.11)\n* Microsoft Windows OS or macOS or Linux\n* Other Python dependency packages: Pandas, Numpy, Logbook, Exchange-calendars, etc.\n\n## How to install Zipline Reloaded modified by TEJ\n\n* We're going to illustrate under anaconda environment, so we suggest using [Anaconda](https://www.anaconda.com/data-science-platform) as development environment.\n\n* Download dependency packages.\n\n1. Windows [(zipline-tej.yml)](https://minhaskamal.github.io/DownGit/#/home?url=https://github.com/tejtw/zipline-tej/blob/main/zipline-tej.yml)\n\n2. Mac [(zipline-tej_mac.yml)](https://minhaskamal.github.io/DownGit/#/home?url=https://github.com/tejtw/zipline-tej/blob/main/zipline-tej_mac.yml)\n\n* Start an Anaconda (base) prompt, create an virtual environment and install the appropriate versions of packages:\n(We **strongly** recommand using virtual environment to keep every project independent.) [(reason)](https://csguide.cs.princeton.edu/software/virtualenv#definition)\n\n```\nWindows Users\n# change directionary to the folder exists zipline-tej.yml\n$ cd <C:\\Users\\username\\Downloads>\n\n# create virtual env\n$ conda env create -f zipline-tej.yml\n\n# activate virtual env\n$ conda activate zipline-tej\nMac Users\n# change directionary to the folder exists zipline-tej_mac.yml\n$ cd <C:\\Users\\username\\Downloads>\n\n# create virtual env\n$ conda env create -f zipline-tej_mac.yml\n\n# activate virtual env\n$ conda activate zipline-tej\n\n```\n\nAlso, if you are familiar with Python enough, you can create a virtual environment without zipline-tej.yml and here's the sample :\n\n```\n# create virtual env\n$ conda create -n <env_name> python=3.10\n\n# activate virtual env\n$ conda activate <env_name>\n\n# download dependency packages\n$ pip install zipline-tej\n\n```\n\nWhile encountering environment problems, we provided a consistent and stable environment on [Docker hub](https://hub.docker.com/).\n\nFor users that using docker, we briefly introduce how to download and use it.\n\nFirst of all, please download and install [docker-desktop](https://www.docker.com/products/docker-desktop/).\n\n```\n\n1. Start docker-desktop. (Registration is not must.)\n\n2. Select the \"images\" on the leftside and search \"tej87681088/tquant\" and click \"Pull\".\n\n3. After the image was downloaded, click the \"run\" icon the enter the optional settings.\n\n3-1. Contaner-name: whatever you want.\n\n3-2. Ports: the port to connect, \"8888\" is recommended.\n\n3-3. Volumes: the place to store files. (You can create volume first on the left side.)\n\ne.g. created a volume named \"data\", host path enter \"data\", container path \"/app\" is recommended.\n\n4. Select the \"Containers\" leftside, the click the one which its image name is tej87681088/tquant\n\n5. In its \"Logs\" would show an url like \nhttp://127.0.0.1:8888/tree?token=XXXXXXXXXXXXXXXXXXXXXXXXXXXXXX\n\n6. Go to your browser and enter \"http://127.0.0.1:<port_you_set_in_step_3-2>/tree?token=XXXXXXXXXXXXXXXXXXXXXXXXXXXXXX\"\n\n6-1. If your port is 8888, you can just click the hyperlink.\n\n7. Start develop your strategy!\n\nNOTICE: Next time, we just need to reproduce step4 to step6.\n```\n\n# Quick start\n\n## CLI Interface\n\nThe following code implements a simple buy-and-hold trading algorithm.\n\n```python\nfrom zipline.api import order, record, symbol\n\ndef initialize(context):\n context.asset = symbol(\"2330\")\n \ndef handle_data(context, data):\n order(context.asset, 10)\n record(TSMC=data.current(context.asset, \"price\"))\n \ndef analyze(context=None, results=None):\n import matplotlib.pyplot as plt\n\n # Plot the portfolio and asset data.\n ax1 = plt.subplot(211)\n results.portfolio_value.plot(ax=ax1)\n ax1.set_ylabel(\"Portfolio value (TWD)\")\n ax2 = plt.subplot(212, sharex=ax1)\n results.TSMC.plot(ax=ax2)\n ax2.set_ylabel(\"TSMC price (TWD)\")\n\n # Show the plot.\n plt.gcf().set_size_inches(18, 8)\n plt.show()\n\n```\nYou can then run this algorithm using the Zipline CLI. But first, you need to download some market data with historical prices and trading volumes:\n* Before ingesting data, you have to set some environment variables as follow:\n \n```\n# setting TEJAPI_KEY to get permissions loading data\n$ set TEJAPI_KEY=<your_key>\n$ set TEJAPI_BASE=https://api.tej.com.tw\n\n# setting download ticker\n$ set ticker=2330 2317\n\n# setting backtest period\n$ set mdate=20200101 20220101\n\n```\n* Ingest and run backtesting algorithm\n\n```\n$ zipline ingest -b tquant\n$ zipline run -f buy_and_hold.py --start 20200101 --end 20220101 -o bah.pickle --no-benchmark --no-treasury \n```\nThen, the resulting performance DataFrame is saved as bah.pickle, which you can load and analyze from Python.\n\n### More useful zipline commands\n\nBefore calling **zipline** in CLI, be sure that TEJAPI_KEY and TEJAPI_BASE were set.\nUse **zipline --help** to get more information.\n\nFor example :\nWe want to know how to use **zipline run**, we can run as follow:\n\n```\nzipline run --help\n```\n\n```\n\nUsage: zipline run [OPTIONS]\n Run a backtest for the given algorithm.\n\nOptions:\n -f, --algofile FILENAME The file that contains the algorithm to run.\n -t, --algotext TEXT The algorithm script to run.\n -D, --define TEXT Define a name to be bound in the namespace\n before executing the algotext. For example\n '-Dname=value'. The value may be any python\n expression. These are evaluated in order so\n they may refer to previously defined names.\n --data-frequency [daily|minute]\n The data frequency of the simulation.\n [default: daily]\n --capital-base FLOAT The starting capital for the simulation.\n [default: 10000000.0]\n -b, --bundle BUNDLE-NAME The data bundle to use for the simulation.\n [default: tquant]\n --bundle-timestamp TIMESTAMP The date to lookup data on or before.\n [default: <current-time>]\n -bf, --benchmark-file FILE The csv file that contains the benchmark\n returns\n --benchmark-symbol TEXT The symbol of the instrument to be used as a\n benchmark (should exist in the ingested\n bundle)\n --benchmark-sid INTEGER The sid of the instrument to be used as a\n benchmark (should exist in the ingested\n bundle)\n --no-benchmark If passed, use a benchmark of zero returns.\n -bf, --treasury-file FILE The csv file that contains the treasury\n returns\n --treasury-symbol TEXT The symbol of the instrument to be used as a\n treasury (should exist in the ingested\n bundle)\n --treasury-sid INTEGER The sid of the instrument to be used as a\n treasury (should exist in the ingested\n bundle)\n --no-treasury If passed, use a treasury of zero returns.\n -s, --start DATE The start date of the simulation.\n -e, --end DATE The end date of the simulation.\n -o, --output FILENAME The location to write the perf data. If this\n is '-' the perf will be written to stdout.\n [default: -]\n --trading-calendar TRADING-CALENDAR\n The calendar you want to use e.g. TEJ_XTAI.\n TEJ_XTAI is the default.\n --print-algo / --no-print-algo Print the algorithm to stdout.\n --metrics-set TEXT The metrics set to use. New metrics sets may\n be registered in your extension.py.\n --blotter TEXT The blotter to use. [default: default]\n --help Show this message and exit.\n\n``` \n\n### Difference of tquant and fundamentals\n\n* Basically, `tquant` is the one that only contain OHLCV and cash dividend date. And `fundamentals` is the data that exclude from OHLCV, like EPS, gross margin, operating income, etc.\n\n* So in both fundamentals and tquant, we can add tickers as follow :\n\n#### Add tickers\n\n```\n$ zipline add -t \"<ticker_wants_to_add>\"\n```\nIf tickers are more than 1 ticker, split them apart by \" \" or \",\" or \";\".\n\n#### [fundamentals only] Add fields\n\n```\n$ zipline add -f \"<field_wants_to_add>\"\n```\n\n* NOTICE that you `CAN'T` add field and ticker simultaneously.\n\nFor more detail use **zipline add --help** .\n\n#### Display bundle-info\n```\n$ zipline bundle-info\n```\nTo show what the tickers are there in newest bundle.\n\nFor more detail use **zipline bundle-info --help** .\n\n#### Switch bundle\n\nBefore using switch, use **zipline bundles** to get the timestamp of each folder.\n\n```\n$ zipline switch -t \"<The_timestamp_of_the_folder_want_to_use>\"\n```\n\nDue to zipline only using the newest folder, switch can make previous folder become newest.\n\nFor more detail use **zipline switch --help** .\n\n#### Update bundle\n\n* You can either update `tquant` or `fundamentals` by using -b to select which one you want to update the bundle information to newest date.[DEFAULT:tquant]\n\n```\n$ zipline update -b tquant\n$ zipline update -b fundamentals\n```\n\nFor more detail use **zipline update --help** .\n\n## Jupyter Notebook \n\n### Change Anaconda kernel\n\n* Since we've downloaded package \"nb_conda_kernels\", we should be able to change kernel in jupyter notebook.\n\n#### How to new a notebook using specific kernel \n\n(1) Open anaconda prompt\n\n(2) Enter the command as follow :\n```\n# First one can be ignore if already in environment of zipline-tej\n$ conda activate zipline-tej \n# start a jupyter notebook\n$ jupyter notebook \n```\n(3) Start a notebook and select Python[conda env:zipline-tej]\n\n(4)(Optional) If you have already written a notebook, you can open it and change kernel by clicking the \"Kernel\" in menu and \"Change kernel\" to select the specfic kernel.\n\n\n### Set environment variables TEJAPI_KEY, ticker and mdate\n\n\\* ticker would be your target ticker symbol, and it should be a string. If there're more than one ticker needed, use \" \", \",\" or \";\" to split them apart. \n\n\\* mdate refers the begin date and end date, use \" \", \",\" or \";\" to split them apart.\n\n```python\nIn[1]:\nimport os \nos.environ['TEJAPI_KEY'] = <your_key> \nos.environ['ticker'] ='2330 2317' \nos.environ['mdate'] ='20200101 20220101' \n```\n### Call ingest to download data to ~\\\\\\.zipline\n\n```python\nIn[2]: \n!zipline ingest -b tquant\n[Out]: \nMerging daily equity files:\n[YYYY-MM-DD HH:mm:ss.ssssss] INFO: zipline.data.bundles.core: Ingesting tquant.\n```\n\n\n### Design the backtesting strategy\n\n```python\nIn[3]:\nfrom zipline.api import order, record, symbol\n\ndef initialize(context):\n context.asset = symbol(\"2330\")\n \ndef handle_data(context, data):\n order(context.asset, 10)\n record(TSMC=data.current(context.asset, \"price\"))\n \ndef analyze(context=None, results=None):\n import matplotlib.pyplot as plt\n\n # Plot the portfolio and asset data.\n ax1 = plt.subplot(211)\n results.portfolio_value.plot(ax=ax1)\n ax1.set_ylabel(\"Portfolio value (TWD)\")\n ax2 = plt.subplot(212, sharex=ax1)\n results.TSMC.plot(ax=ax2)\n ax2.set_ylabel(\"TSMC price (TWD)\")\n\n # Show the plot.\n plt.gcf().set_size_inches(18, 8)\n plt.show()\n```\n### Run backtesting algorithm and plot\n\n```python\nIn[4]:\nfrom zipline import run_algorithm\nimport pandas as pd\nfrom zipline.utils.calendar_utils import get_calendar\ntrading_calendar = get_calendar('TEJ_XTAI')\n\nstart = pd.Timestamp('20200103', tz ='utc' )\nend = pd.Timestamp('20211230', tz='utc')\n\nresult = run_algorithm(start=start,\n end=end,\n initialize=initialize,\n capital_base=1000000,\n handle_data=handle_data,\n bundle='tquant',\n trading_calendar=trading_calendar,\n analyze=analyze,\n data_frequency='daily'\n )\n[Out]:\n```\n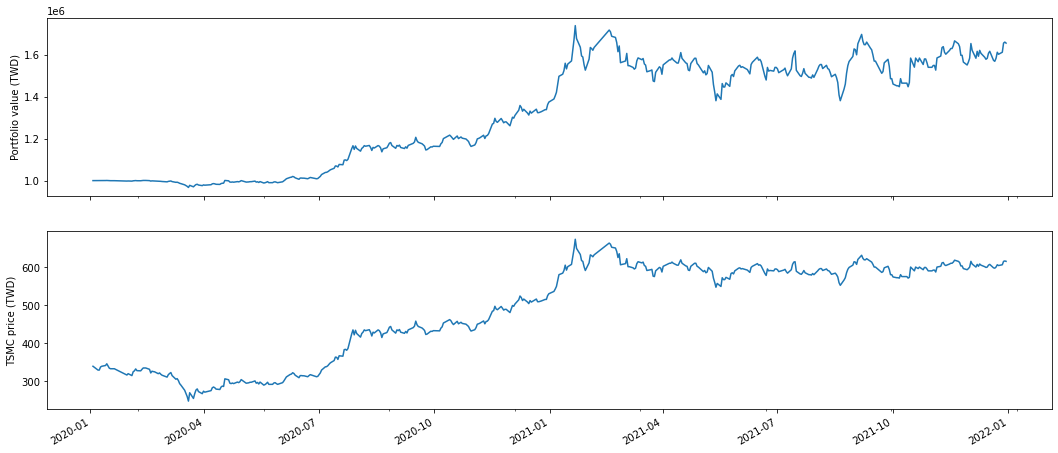\n\n\n### Show trading process\n```python\nIn[5]: \nresult\n[Out]:\n```\n<div>\n<table border=\"1\" class=\"dataframe\">\n <thead>\n <tr style=\"text-align: right;\">\n <th></th>\n <th>period_open</th>\n <th>period_close</th>\n <th>starting_value</th>\n <th>ending_value</th>\n <th>starting_cash</th>\n <th>ending_cash</th>\n <th>portfolio_value</th>\n <th>longs_count</th>\n <th>shorts_count</th>\n <th>long_value</th>\n <th>...</th>\n <th>treasury_period_return</th>\n <th>trading_days</th>\n <th>period_label</th>\n <th>algo_volatility</th>\n <th>benchmark_period_return</th>\n <th>benchmark_volatility</th>\n <th>algorithm_period_return</th>\n <th>alpha</th>\n <th>beta</th>\n <th>sharpe</th>\n </tr>\n </thead>\n <tbody>\n <tr>\n <th>2020-01-03 05:30:00+00:00</th>\n <td>2020-01-03 01:01:00+00:00</td>\n <td>2020-01-03 05:30:00+00:00</td>\n <td>0.0</td>\n <td>0.0</td>\n <td>1.000000e+06</td>\n <td>1.000000e+06</td>\n <td>1.000000e+06</td>\n <td>0</td>\n <td>0</td>\n <td>0.0</td>\n <td>...</td>\n <td>0.0</td>\n <td>1</td>\n <td>2020-01</td>\n <td>NaN</td>\n <td>0.0</td>\n <td>NaN</td>\n <td>0.000000</td>\n <td>None</td>\n <td>None</td>\n <td>NaN</td>\n </tr>\n <tr>\n <th>2020-01-06 05:30:00+00:00</th>\n <td>2020-01-06 01:01:00+00:00</td>\n <td>2020-01-06 05:30:00+00:00</td>\n <td>0.0</td>\n <td>3320.0</td>\n <td>1.000000e+06</td>\n <td>9.966783e+05</td>\n <td>9.999983e+05</td>\n <td>1</td>\n <td>0</td>\n <td>3320.0</td>\n <td>...</td>\n <td>0.0</td>\n <td>2</td>\n <td>2020-01</td>\n <td>0.000019</td>\n <td>0.0</td>\n <td>0.0</td>\n <td>-0.000002</td>\n <td>None</td>\n <td>None</td>\n <td>-11.224972</td>\n </tr>\n <tr>\n <th>2020-01-07 05:30:00+00:00</th>\n <td>2020-01-07 01:01:00+00:00</td>\n <td>2020-01-07 05:30:00+00:00</td>\n <td>3320.0</td>\n <td>6590.0</td>\n <td>9.966783e+05</td>\n <td>9.933817e+05</td>\n <td>9.999717e+05</td>\n <td>1</td>\n <td>0</td>\n <td>6590.0</td>\n <td>...</td>\n <td>0.0</td>\n <td>3</td>\n <td>2020-01</td>\n <td>0.000237</td>\n <td>0.0</td>\n <td>0.0</td>\n <td>-0.000028</td>\n <td>None</td>\n <td>None</td>\n <td>-10.038514</td>\n </tr>\n <tr>\n <th>2020-01-08 05:30:00+00:00</th>\n <td>2020-01-08 01:01:00+00:00</td>\n <td>2020-01-08 05:30:00+00:00</td>\n <td>6590.0</td>\n <td>9885.0</td>\n <td>9.933817e+05</td>\n <td>9.900850e+05</td>\n <td>9.999700e+05</td>\n <td>1</td>\n <td>0</td>\n <td>9885.0</td>\n <td>...</td>\n <td>0.0</td>\n <td>4</td>\n <td>2020-01</td>\n <td>0.000203</td>\n <td>0.0</td>\n <td>0.0</td>\n <td>-0.000030</td>\n <td>None</td>\n <td>None</td>\n <td>-9.298128</td>\n </tr>\n <tr>\n <th>2020-01-09 05:30:00+00:00</th>\n <td>2020-01-09 01:01:00+00:00</td>\n <td>2020-01-09 05:30:00+00:00</td>\n <td>9885.0</td>\n <td>13500.0</td>\n <td>9.900850e+05</td>\n <td>9.867083e+05</td>\n <td>1.000208e+06</td>\n <td>1</td>\n <td>0</td>\n <td>13500.0</td>\n <td>...</td>\n <td>0.0</td>\n <td>5</td>\n <td>2020-01</td>\n <td>0.001754</td>\n <td>0.0</td>\n <td>0.0</td>\n <td>0.000208</td>\n <td>None</td>\n <td>None</td>\n <td>5.986418</td>\n </tr>\n <tr>\n <th>...</th>\n <td>...</td>\n <td>...</td>\n <td>...</td>\n <td>...</td>\n <td>...</td>\n <td>...</td>\n <td>...</td>\n <td>...</td>\n <td>...</td>\n <td>...</td>\n <td>...</td>\n <td>...</td>\n <td>...</td>\n <td>...</td>\n <td>...</td>\n <td>...</td>\n <td>...</td>\n <td>...</td>\n <td>...</td>\n <td>...</td>\n <td>...</td>\n </tr>\n <tr>\n <th>2021-12-24 05:30:00+00:00</th>\n <td>2021-12-24 01:01:00+00:00</td>\n <td>2021-12-24 05:30:00+00:00</td>\n <td>2920920.0</td>\n <td>2917320.0</td>\n <td>-1.308854e+06</td>\n <td>-1.314897e+06</td>\n <td>1.602423e+06</td>\n <td>1</td>\n <td>0</td>\n <td>2917320.0</td>\n <td>...</td>\n <td>0.0</td>\n <td>484</td>\n <td>2021-12</td>\n <td>0.232791</td>\n <td>0.0</td>\n <td>0.0</td>\n <td>0.602423</td>\n <td>None</td>\n <td>None</td>\n <td>1.170743</td>\n </tr>\n <tr>\n <th>2021-12-27 05:30:00+00:00</th>\n <td>2021-12-27 01:01:00+00:00</td>\n <td>2021-12-27 05:30:00+00:00</td>\n <td>2917320.0</td>\n <td>2933040.0</td>\n <td>-1.314897e+06</td>\n <td>-1.320960e+06</td>\n <td>1.612080e+06</td>\n <td>1</td>\n <td>0</td>\n <td>2933040.0</td>\n <td>...</td>\n <td>0.0</td>\n <td>485</td>\n <td>2021-12</td>\n <td>0.232577</td>\n <td>0.0</td>\n <td>0.0</td>\n <td>0.612080</td>\n <td>None</td>\n <td>None</td>\n <td>1.182864</td>\n </tr>\n <tr>\n <th>2021-12-28 05:30:00+00:00</th>\n <td>2021-12-28 01:01:00+00:00</td>\n <td>2021-12-28 05:30:00+00:00</td>\n <td>2933040.0</td>\n <td>2982750.0</td>\n <td>-1.320960e+06</td>\n <td>-1.327113e+06</td>\n <td>1.655637e+06</td>\n <td>1</td>\n <td>0</td>\n <td>2982750.0</td>\n <td>...</td>\n <td>0.0</td>\n <td>486</td>\n <td>2021-12</td>\n <td>0.233086</td>\n <td>0.0</td>\n <td>0.0</td>\n <td>0.655637</td>\n <td>None</td>\n <td>None</td>\n <td>1.237958</td>\n </tr>\n <tr>\n <th>2021-12-29 05:30:00+00:00</th>\n <td>2021-12-29 01:01:00+00:00</td>\n <td>2021-12-29 05:30:00+00:00</td>\n <td>2982750.0</td>\n <td>2993760.0</td>\n <td>-1.327113e+06</td>\n <td>-1.333276e+06</td>\n <td>1.660484e+06</td>\n <td>1</td>\n <td>0</td>\n <td>2993760.0</td>\n <td>...</td>\n <td>0.0</td>\n <td>487</td>\n <td>2021-12</td>\n <td>0.232850</td>\n <td>0.0</td>\n <td>0.0</td>\n <td>0.660484</td>\n <td>None</td>\n <td>None</td>\n <td>1.243176</td>\n </tr>\n <tr>\n <th>2021-12-30 05:30:00+00:00</th>\n <td>2021-12-30 01:01:00+00:00</td>\n <td>2021-12-30 05:30:00+00:00</td>\n <td>2993760.0</td>\n <td>2995050.0</td>\n <td>-1.333276e+06</td>\n <td>-1.339430e+06</td>\n <td>1.655620e+06</td>\n <td>1</td>\n <td>0</td>\n <td>2995050.0</td>\n <td>...</td>\n <td>0.0</td>\n <td>488</td>\n <td>2021-12</td>\n <td>0.232629</td>\n <td>0.0</td>\n <td>0.0</td>\n <td>0.655620</td>\n <td>None</td>\n <td>None</td>\n <td>1.235305</td>\n </tr>\n </tbody>\n</table>\n<p>488 rows \u00d7 38 columns</p>\n</div>\n\n---\n\n# More Zipline Tutorials\n\n* For more [tutorials](https://github.com/tejtw/TQuant-Lab)\n\n# Suggestions\n* To get TEJAPI_KEY [(link)](https://api.tej.com.tw/trial.html)\n* [TEJ Official Website](https://www.tej.com.tw/)\n",
"bugtrack_url": null,
"license": "Apache-2.0",
"summary": "A Pythonic backtester for trading algorithms",
"version": "2.1.0",
"project_urls": {
"homepage": "https://tquant.tejwin.com/",
"repository": "https://github.com/tejtw/zipline-tej"
},
"split_keywords": [],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "c79303b3e188a882b205e1fdd21eae3e5456ec024ca13c7f33bdb177ce4fa04a",
"md5": "2e7c14074e44c824694b02d69d361124",
"sha256": "4f3e316f27ffffca15e55e89142695bd7592baf658aca0120939bbbb2bfffa1b"
},
"downloads": -1,
"filename": "zipline_tej-2.1.0-cp310-cp310-macosx_10_15_x86_64.whl",
"has_sig": false,
"md5_digest": "2e7c14074e44c824694b02d69d361124",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": ">=3.8",
"size": 4318586,
"upload_time": "2024-10-30T01:15:53",
"upload_time_iso_8601": "2024-10-30T01:15:53.332755Z",
"url": "https://files.pythonhosted.org/packages/c7/93/03b3e188a882b205e1fdd21eae3e5456ec024ca13c7f33bdb177ce4fa04a/zipline_tej-2.1.0-cp310-cp310-macosx_10_15_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "7b1517277de2dcfb7658a96c89249839a32268b08cf8c8e982a3b19a61f6ef1f",
"md5": "fcfd6b3930443dca8eebc08368661052",
"sha256": "5b22be3fd8652baaa31145b6e095c96d7e882527b65d86fb1619838ffb4be01d"
},
"downloads": -1,
"filename": "zipline_tej-2.1.0-cp310-cp310-macosx_11_0_arm64.whl",
"has_sig": false,
"md5_digest": "fcfd6b3930443dca8eebc08368661052",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": ">=3.8",
"size": 4186475,
"upload_time": "2024-10-30T01:15:55",
"upload_time_iso_8601": "2024-10-30T01:15:55.609888Z",
"url": "https://files.pythonhosted.org/packages/7b/15/17277de2dcfb7658a96c89249839a32268b08cf8c8e982a3b19a61f6ef1f/zipline_tej-2.1.0-cp310-cp310-macosx_11_0_arm64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "10a9ae9db0faecdf5ac9bd0316788444eafce52769eed4d75ac6915f40759dc5",
"md5": "6a74852b2fb05f459716a67d2abc62e0",
"sha256": "f07152a9766fd165be92705640ba1aa60a365e3d1de13680684e67287291d4d9"
},
"downloads": -1,
"filename": "zipline_tej-2.1.0-cp310-cp310-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"has_sig": false,
"md5_digest": "6a74852b2fb05f459716a67d2abc62e0",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": ">=3.8",
"size": 9209812,
"upload_time": "2024-11-07T14:19:05",
"upload_time_iso_8601": "2024-11-07T14:19:05.560000Z",
"url": "https://files.pythonhosted.org/packages/10/a9/ae9db0faecdf5ac9bd0316788444eafce52769eed4d75ac6915f40759dc5/zipline_tej-2.1.0-cp310-cp310-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "d361d584eb1e69baf63a53e79ce2dd36896bb6140aa737487eda59ee2a8c377a",
"md5": "badff041fc8b170b4657aeaa2a672661",
"sha256": "c6c434170970abd837782da79b44404f48571ee87b330ef497c6f3db180506ee"
},
"downloads": -1,
"filename": "zipline_tej-2.1.0-cp310-cp310-win_amd64.whl",
"has_sig": false,
"md5_digest": "badff041fc8b170b4657aeaa2a672661",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": ">=3.8",
"size": 4127629,
"upload_time": "2024-10-30T01:15:57",
"upload_time_iso_8601": "2024-10-30T01:15:57.676325Z",
"url": "https://files.pythonhosted.org/packages/d3/61/d584eb1e69baf63a53e79ce2dd36896bb6140aa737487eda59ee2a8c377a/zipline_tej-2.1.0-cp310-cp310-win_amd64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "a807c8bd123463ff09416b2bb42d7c6372f5f35306b1c47aa0d97fb59bc391d2",
"md5": "b2b122075fb014128aff8d6ea184e237",
"sha256": "3c221454c3f0a4a0d274940c0fc47d0559d0fae0765559393de061d075906429"
},
"downloads": -1,
"filename": "zipline_tej-2.1.0-cp311-cp311-macosx_10_15_x86_64.whl",
"has_sig": false,
"md5_digest": "b2b122075fb014128aff8d6ea184e237",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": ">=3.8",
"size": 4304555,
"upload_time": "2024-10-30T01:15:59",
"upload_time_iso_8601": "2024-10-30T01:15:59.621243Z",
"url": "https://files.pythonhosted.org/packages/a8/07/c8bd123463ff09416b2bb42d7c6372f5f35306b1c47aa0d97fb59bc391d2/zipline_tej-2.1.0-cp311-cp311-macosx_10_15_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "7d62cce155412c8f8a86378e510d816fcc6336cf82317aa43969f61bc79c8f16",
"md5": "b94569ca64b4c180de14489e9c19a001",
"sha256": "8732e62c1d242591a479aabbfb024ee0dec621ccf90ec91e4f4fc7201c963eee"
},
"downloads": -1,
"filename": "zipline_tej-2.1.0-cp311-cp311-macosx_11_0_arm64.whl",
"has_sig": false,
"md5_digest": "b94569ca64b4c180de14489e9c19a001",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": ">=3.8",
"size": 4171554,
"upload_time": "2024-10-30T01:16:01",
"upload_time_iso_8601": "2024-10-30T01:16:01.690685Z",
"url": "https://files.pythonhosted.org/packages/7d/62/cce155412c8f8a86378e510d816fcc6336cf82317aa43969f61bc79c8f16/zipline_tej-2.1.0-cp311-cp311-macosx_11_0_arm64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "4ecca649755f253bc23e5bdb88999ecdc7b576b0457da12c9cd948433d34e00d",
"md5": "f18862a30a2f4b16a2a08409784c176b",
"sha256": "8d8d160a3dd105e4066783965f32e41c7efe8f058dfb3fc14ddaafcb9424df1f"
},
"downloads": -1,
"filename": "zipline_tej-2.1.0-cp311-cp311-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"has_sig": false,
"md5_digest": "f18862a30a2f4b16a2a08409784c176b",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": ">=3.8",
"size": 9407637,
"upload_time": "2024-11-07T14:19:27",
"upload_time_iso_8601": "2024-11-07T14:19:27.618478Z",
"url": "https://files.pythonhosted.org/packages/4e/cc/a649755f253bc23e5bdb88999ecdc7b576b0457da12c9cd948433d34e00d/zipline_tej-2.1.0-cp311-cp311-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "6415b791210f47e9161dcb538011d9790c425488ad1a6ad342a05fce01f3442a",
"md5": "95232ac95991dc0eaad3e107a30eff10",
"sha256": "287c5221089d91a22a9ddc0d6e8be9e3f551dcb77c5eb26e8a81e92acef31072"
},
"downloads": -1,
"filename": "zipline_tej-2.1.0-cp311-cp311-win_amd64.whl",
"has_sig": false,
"md5_digest": "95232ac95991dc0eaad3e107a30eff10",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": ">=3.8",
"size": 4135917,
"upload_time": "2024-10-30T01:16:03",
"upload_time_iso_8601": "2024-10-30T01:16:03.416436Z",
"url": "https://files.pythonhosted.org/packages/64/15/b791210f47e9161dcb538011d9790c425488ad1a6ad342a05fce01f3442a/zipline_tej-2.1.0-cp311-cp311-win_amd64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "acb14c1e5da0ae9dd2e5afe1ec19c904d1b76c614195253a8eb703d82e3d558c",
"md5": "5cfc7fa0f2723ab1a37e9fee908dba4c",
"sha256": "2d84d399126bcf8c714db70b4c17f9626e7a6e8fc78b0ac43de30fbd654540b5"
},
"downloads": -1,
"filename": "zipline_tej-2.1.0-cp38-cp38-macosx_10_15_x86_64.whl",
"has_sig": false,
"md5_digest": "5cfc7fa0f2723ab1a37e9fee908dba4c",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": ">=3.8",
"size": 4458540,
"upload_time": "2024-10-30T01:16:04",
"upload_time_iso_8601": "2024-10-30T01:16:04.905773Z",
"url": "https://files.pythonhosted.org/packages/ac/b1/4c1e5da0ae9dd2e5afe1ec19c904d1b76c614195253a8eb703d82e3d558c/zipline_tej-2.1.0-cp38-cp38-macosx_10_15_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "83d5348f27a64f1dc69cfce0bc7dfd6619601ffcc1c7c297101ec7fe94b272c1",
"md5": "471341dc1a3f69d1c220cf2b58eb1d6d",
"sha256": "b597dc44eed0a1e632fa270d2dbc783d30869e4ef909d1c5a98973c6033708ef"
},
"downloads": -1,
"filename": "zipline_tej-2.1.0-cp38-cp38-macosx_11_0_arm64.whl",
"has_sig": false,
"md5_digest": "471341dc1a3f69d1c220cf2b58eb1d6d",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": ">=3.8",
"size": 4330796,
"upload_time": "2024-10-30T01:16:06",
"upload_time_iso_8601": "2024-10-30T01:16:06.284376Z",
"url": "https://files.pythonhosted.org/packages/83/d5/348f27a64f1dc69cfce0bc7dfd6619601ffcc1c7c297101ec7fe94b272c1/zipline_tej-2.1.0-cp38-cp38-macosx_11_0_arm64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "7fedd1c4317d2ff572dce4a5b889352841c885f005c39372bb0b07bfee8e9774",
"md5": "fe1f61db156c6ef9bdbf6bd88f8b4a90",
"sha256": "84105d0d9775e2edeb7fd46863bc309ccb90c07f97562b7239fd5e6067663da1"
},
"downloads": -1,
"filename": "zipline_tej-2.1.0-cp38-cp38-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"has_sig": false,
"md5_digest": "fe1f61db156c6ef9bdbf6bd88f8b4a90",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": ">=3.8",
"size": 9437053,
"upload_time": "2024-11-07T14:19:49",
"upload_time_iso_8601": "2024-11-07T14:19:49.087398Z",
"url": "https://files.pythonhosted.org/packages/7f/ed/d1c4317d2ff572dce4a5b889352841c885f005c39372bb0b07bfee8e9774/zipline_tej-2.1.0-cp38-cp38-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "82a294c70dcd78fc5d84836183061cb0aa2e074df4c375a4afd0fd2081f7acc3",
"md5": "73a3b50f36c1ed01dda4c37d66948dc0",
"sha256": "c884cb4470bc17f9e71af39b8ca181c888c974c90f4b7d28f1810ea787ada08d"
},
"downloads": -1,
"filename": "zipline_tej-2.1.0-cp38-cp38-win_amd64.whl",
"has_sig": false,
"md5_digest": "73a3b50f36c1ed01dda4c37d66948dc0",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": ">=3.8",
"size": 4288293,
"upload_time": "2024-10-30T01:16:08",
"upload_time_iso_8601": "2024-10-30T01:16:08.386794Z",
"url": "https://files.pythonhosted.org/packages/82/a2/94c70dcd78fc5d84836183061cb0aa2e074df4c375a4afd0fd2081f7acc3/zipline_tej-2.1.0-cp38-cp38-win_amd64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "0ac68f6f0f23991c9c739804098b45ffd608284ac76c0cd035cd09524962d713",
"md5": "b7623c3fd3378960b1e9c08c95d7f078",
"sha256": "48fd80744f7a30cf1b52a85620ed2bc45334a2318b01017a9aa562c08cc57ef3"
},
"downloads": -1,
"filename": "zipline_tej-2.1.0-cp39-cp39-macosx_10_15_x86_64.whl",
"has_sig": false,
"md5_digest": "b7623c3fd3378960b1e9c08c95d7f078",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": ">=3.8",
"size": 4325691,
"upload_time": "2024-10-30T01:16:09",
"upload_time_iso_8601": "2024-10-30T01:16:09.775138Z",
"url": "https://files.pythonhosted.org/packages/0a/c6/8f6f0f23991c9c739804098b45ffd608284ac76c0cd035cd09524962d713/zipline_tej-2.1.0-cp39-cp39-macosx_10_15_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "579889f09ba65b2d73108e36f7b427f75d0e7c5d215df7801c0e47a0cea93ce8",
"md5": "56f314e70c6d15ed5fdbbf506aaf532a",
"sha256": "6cbae79a0f830c644d3b14671d1e2f045a1f56b10ea4844dea6fb31a6d32aeeb"
},
"downloads": -1,
"filename": "zipline_tej-2.1.0-cp39-cp39-macosx_11_0_arm64.whl",
"has_sig": false,
"md5_digest": "56f314e70c6d15ed5fdbbf506aaf532a",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": ">=3.8",
"size": 4194356,
"upload_time": "2024-10-30T01:16:11",
"upload_time_iso_8601": "2024-10-30T01:16:11.466711Z",
"url": "https://files.pythonhosted.org/packages/57/98/89f09ba65b2d73108e36f7b427f75d0e7c5d215df7801c0e47a0cea93ce8/zipline_tej-2.1.0-cp39-cp39-macosx_11_0_arm64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "5164a2be2a43be268d24de9f3224bad37297a5591539f307a26714de4d2dc84a",
"md5": "de1b4f8239914be1b185423acb222b2b",
"sha256": "1eab57a09fb0d19878f8473490ae86aa8a72a24bfc5fcdc5a429f17fc5c57a48"
},
"downloads": -1,
"filename": "zipline_tej-2.1.0-cp39-cp39-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"has_sig": false,
"md5_digest": "de1b4f8239914be1b185423acb222b2b",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": ">=3.8",
"size": 9230966,
"upload_time": "2024-11-07T14:20:12",
"upload_time_iso_8601": "2024-11-07T14:20:12.597032Z",
"url": "https://files.pythonhosted.org/packages/51/64/a2be2a43be268d24de9f3224bad37297a5591539f307a26714de4d2dc84a/zipline_tej-2.1.0-cp39-cp39-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "51536c6dabff19307e569e1c8f9a197d5e1518191cc3124b89337fe99a7f1d26",
"md5": "34c992b04282fba922baa47040e59c15",
"sha256": "37d60cd80b0c1dfbe58d2dc981af87959523a762956df819ea2fd97131c8e87f"
},
"downloads": -1,
"filename": "zipline_tej-2.1.0-cp39-cp39-win_amd64.whl",
"has_sig": false,
"md5_digest": "34c992b04282fba922baa47040e59c15",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": ">=3.8",
"size": 4131888,
"upload_time": "2024-10-30T01:16:14",
"upload_time_iso_8601": "2024-10-30T01:16:14.885560Z",
"url": "https://files.pythonhosted.org/packages/51/53/6c6dabff19307e569e1c8f9a197d5e1518191cc3124b89337fe99a7f1d26/zipline_tej-2.1.0-cp39-cp39-win_amd64.whl",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-10-30 01:15:53",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "tejtw",
"github_project": "zipline-tej",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "zipline-tej"
}