
[๐ซฐ Installation](https://github.com/state-alchemists/zrb/blob/main/docs/installation.md) | [๐ Documentation](https://github.com/state-alchemists/zrb/blob/main/docs/README.md) | [๐ Getting Started](https://github.com/state-alchemists/zrb/blob/main/docs/getting-started.md) | [๐ Common Mistakes](https://github.com/state-alchemists/zrb/blob/main/docs/oops-i-did-it-again/README.md) | [โ FAQ](https://github.com/state-alchemists/zrb/blob/main/docs/faq/README.md)
# ๐ค Zrb: A Framework to Enhance Your Workflow
Zrb is a [CLI-based](https://en.wikipedia.org/wiki/Command-line_interface) automation [tool](https://en.wikipedia.org/wiki/Programming_tool) and [low-code](https://en.wikipedia.org/wiki/Low-code_development_platform) platform. Zrb can help you to:
- __Automate__ day-to-day tasks.
- __Generate__ projects or applications.
- __Prepare__, __run__, and __deploy__ your applications with a single command.
- Etc.
You can also write custom task definitions in [Python](https://www.python.org/), enhancing Zrb's base capabilities. Defining your tasks in Zrb gives you several advantages because:
- Every task has a __retry mechanism__.
- Zrb handles your __task dependencies__ automatically.
- Zrb runs your task dependencies __concurrently__.
# ๐ซฐ Installing Zrb
You can install Zrb as a pip package by invoking the following command:
```bash
pip install zrb
```
Alternatively, you can also use our installation script to install Zrb along with some prerequisites:
```bash
source <(curl -s https://raw.githubusercontent.com/state-alchemists/zrb/main/install.sh)
```
Check our [installation guide](https://github.com/state-alchemists/zrb/blob/main/docs/installation.md) for more information about the installation methods, including installation as a docker container.
# โ๏ธ Zrb as A Task-Automation Tool
At the very core, Zrb is a task automation tool. It helps you to automate some tedious jobs so that you can focus on what matters.
Let's say you want to be able to describe the statistics property of any public CSV dataset. To do this, you need to perform three tasks like the following:
- Install pandas.
- Download the CSV dataset (at the same time).
- Show statistics properties of the CSV dataset.
```
๐ผ
Install Pandas โโโโโโ ๐
โโโโบ Show Statistics
Download Datasets โโโ
โฌ๏ธ
```
You can create a file named `zrb_init.py` and define the tasks as follows:
```python
# File name: zrb_init.py
from zrb import runner, Parallel, CmdTask, python_task, StrInput
DEFAULT_URL = 'https://raw.githubusercontent.com/state-alchemists/datasets/main/iris.csv'
# ๐ผ Define a task named `install-pandas` to install pandas.
# If this task failed, we want Zrb to retry it again 4 times at most.
install_pandas = CmdTask(
name='install-pandas',
cmd='pip install pandas',
retry=4
)
# โฌ๏ธ Define a task named `download-dataset` to download dataset.
# This task has an input named `url`.
# The input will be accessible by using Jinja template: `{{input.url}}`
# If this task failed, we want Zrb to retry it again 4 times at most
download_dataset = CmdTask(
name='download-dataset',
inputs=[
StrInput(name='url', default=DEFAULT_URL)
],
cmd='wget -O dataset.csv {{input.url}}',
retry=4
)
# ๐ Define a task named `show-stat` to show the statistics properties of the dataset.
# @python_task` decorator turns a function into a Zrb Task (i.e., `show_stat` is now a Zrb Task).
# If this task failed, we don't want to retry
@python_task(
name='show-stats',
retry=0
)
def show_stats(*args, **kwargs):
import pandas as pd
df = pd.read_csv('dataset.csv')
return df.describe()
# Define dependencies: `show_stat` depends on both, `download_dataset` and `install_pandas`
Parallel(download_dataset, install_pandas) >> show_stats
# Register the tasks so that they are accessbie from the CLI
runner.register(install_pandas, download_dataset, show_stats)
```
> __๐ NOTE:__ It is possible (although less readable) to define `show_stat` as `CmdTask`:
> <details>
> <summary>Show code</summary>
>
> ```python
> show_stats = CmdTask(
> name='show-stats',
> cmd='python -c "import pandas as pd; df=pd.read_csv(\'dataset.csv\'); print(df.describe())"',
> retry=0
> )
> ```
> </details>
Once you write the definitions, Zrb will automatically load your `zrb_init.py` so that you can invoke your registered task:
```bash
zrb show-stat
```
The command will give you the statistics property of the dataset:
```
sepal_length sepal_width petal_length petal_width
count 150.000000 150.000000 150.000000 150.000000
mean 5.843333 3.054000 3.758667 1.198667
std 0.828066 0.433594 1.764420 0.763161
min 4.300000 2.000000 1.000000 0.100000
25% 5.100000 2.800000 1.600000 0.300000
50% 5.800000 3.000000 4.350000 1.300000
75% 6.400000 3.300000 5.100000 1.800000
max 7.900000 4.400000 6.900000 2.500000
```
<details>
<summary>See the full output</summary>
```
Url [https://raw.githubusercontent.com/state-alchemists/datasets/main/iris.csv]:
๐ค โ โท โ 43598 โ 1/3 ๐ฎ zrb project install-pandas โข Run script: pip install pandas
๐ค โ โท โ 43598 โ 1/3 ๐ฎ zrb project install-pandas โข Working directory: /home/gofrendi/playground/my-project
๐ค โ โท โ 43598 โ 1/3 ๐ zrb project download-dataset โข Run script: wget -O dataset.csv https://raw.githubusercontent.com/state-alchemists/datasets/main/iris.csv
๐ค โ โท โ 43598 โ 1/3 ๐ zrb project download-dataset โข Working directory: /home/gofrendi/playground/my-project
๐ค โณ โท โ 43603 โ 1/3 ๐ zrb project download-dataset โข --2023-11-12 09:45:12-- https://raw.githubusercontent.com/state-alchemists/datasets/main/iris.csv
๐ค โณ โท โ 43603 โ 1/3 ๐ zrb project download-dataset โข Resolving raw.githubusercontent.com (raw.githubusercontent.com)... 185.199.111.133, 185.199.109.133, 185.199.110.133, ...
๐ค โณ โท โ 43603 โ 1/3 ๐ zrb project download-dataset โข Connecting to raw.githubusercontent.com (raw.githubusercontent.com)|185.199.111.133|:443... connected.
๐ค โณ โท โ 43603 โ 1/3 ๐ zrb project download-dataset โข HTTP request sent, awaiting response... 200 OK
๐ค โณ โท โ 43603 โ 1/3 ๐ zrb project download-dataset โข Length: 4606 (4.5K) [text/plain]
๐ค โณ โท โ 43603 โ 1/3 ๐ zrb project download-dataset โข Saving to: โdataset.csvโ
๐ค โณ โท โ 43603 โ 1/3 ๐ zrb project download-dataset โข
๐ค โณ โท โ 43603 โ 1/3 ๐ zrb project download-dataset โข 0K .... 100% 1.39M=0.003s
๐ค โณ โท โ 43603 โ 1/3 ๐ zrb project download-dataset โข
๐ค โณ โท โ 43603 โ 1/3 ๐ zrb project download-dataset โข 2023-11-12 09:45:12 (1.39 MB/s) - โdataset.csvโ saved [4606/4606]
๐ค โณ โท โ 43603 โ 1/3 ๐ zrb project download-dataset โข
๐ค โ โท โ 43601 โ 1/3 ๐ฎ zrb project install-pandas โข Requirement already satisfied: pandas in /home/gofrendi/zrb/.venv/lib/python3.10/site-packages (2.1.3)
๐ค โ โท โ 43601 โ 1/3 ๐ฎ zrb project install-pandas โข Requirement already satisfied: numpy<2,>=1.22.4 in /home/gofrendi/zrb/.venv/lib/python3.10/site-packages (from pandas) (1.26.1)
๐ค โ โท โ 43601 โ 1/3 ๐ฎ zrb project install-pandas โข Requirement already satisfied: python-dateutil>=2.8.2 in /home/gofrendi/zrb/.venv/lib/python3.10/site-packages (from pandas) (2.8.2)
๐ค โ โท โ 43601 โ 1/3 ๐ฎ zrb project install-pandas โข Requirement already satisfied: pytz>=2020.1 in /home/gofrendi/zrb/.venv/lib/python3.10/site-packages (from pandas) (2023.3.post1)
๐ค โ โท โ 43601 โ 1/3 ๐ฎ zrb project install-pandas โข Requirement already satisfied: tzdata>=2022.1 in /home/gofrendi/zrb/.venv/lib/python3.10/site-packages (from pandas) (2023.3)
๐ค โ โท โ 43601 โ 1/3 ๐ฎ zrb project install-pandas โข Requirement already satisfied: six>=1.5 in /home/gofrendi/zrb/.venv/lib/python3.10/site-packages (from python-dateutil>=2.8.2->pandas) (1.16.0)
Support zrb growth and development!
โ Donate at: https://stalchmst.com/donation
๐ Submit issues/PR at: https://github.com/state-alchemists/zrb
๐ค Follow us at: https://twitter.com/zarubastalchmst
๐ค โ โท 2023-11-12 09:45:14.366 โ 43598 โ 1/3 ๐ zrb project show-stats โข Completed in 2.2365798950195312 seconds
sepal_length sepal_width petal_length petal_width
count 150.000000 150.000000 150.000000 150.000000
mean 5.843333 3.054000 3.758667 1.198667
std 0.828066 0.433594 1.764420 0.763161
min 4.300000 2.000000 1.000000 0.100000
25% 5.100000 2.800000 1.600000 0.300000
50% 5.800000 3.000000 4.350000 1.300000
75% 6.400000 3.300000 5.100000 1.800000
max 7.900000 4.400000 6.900000 2.500000
To run again: zrb project show-stats --url "https://raw.githubusercontent.com/state-alchemists/datasets/main/iris.csv"
```
</details>
Since you have registered `install_pandas` and `download_dataset` (i.e., `runner.register(install_pandas, download_dataset)`), then you can also execute those tasks as well:
```bash
zrb install-pandas
zrb download-dataset
```
> __๐ NOTE:__ When executing a task, you can also provide the parameter directly, for example you want to provide the `url`
>
> ```bash
> zrb show-stat --url https://raw.githubusercontent.com/state-alchemists/datasets/main/iris.csv
> ```
>
> When you provide the parameter directly, Zrb will not prompt you to supply the URL.
> Another way to disable the prompt is by set `ZRB_SHOW_PROMPT` to `0` or `false`.
You can also run a Docker compose file, start a Web server, generate a CRUD application, or set up multiple servers simultaneously.
See [our getting started guide](https://github.com/state-alchemists/zrb/blob/main/docs/getting-started.md) and [tutorials](https://github.com/state-alchemists/zrb/blob/main/docs/tutorials/README.md) to learn more about the details.
# โ๏ธ Zrb as A Low-Code Framework
Zrb has some built-in tasks that allow you to create and run a [CRUD](https://en.wikipedia.org/wiki/Create,_read,_update_and_delete) application.
Let's see the following example.
```bash
# Create a project
zrb project create --project-dir my-project --project-name "My Project"
cd my-project
# Create a Fastapp
zrb project add fastapp app --project-dir . --app-name "fastapp" --http-port 3000
# Add library module to fastapp
zrb project add fastapp module --project-dir . --app-name "fastapp" --module-name "library"
# Add entity named "books"
zrb project add fastapp crud --project-dir . --app-name "fastapp" --module-name "library" \
--entity-name "book" --plural-entity-name "books" --column-name "code"
# Add column to the entity
zrb project add fastapp field --project-dir . --app-name "fastapp" --module-name "library" \
--entity-name "book" --column-name "title" --column-type "str"
# Run Fastapp as monolith
zrb project fastapp monolith start
```
Once you invoke the commands, you will be able to access the CRUD application by pointing your browser to [http://localhost:3000](http://localhost:3000)
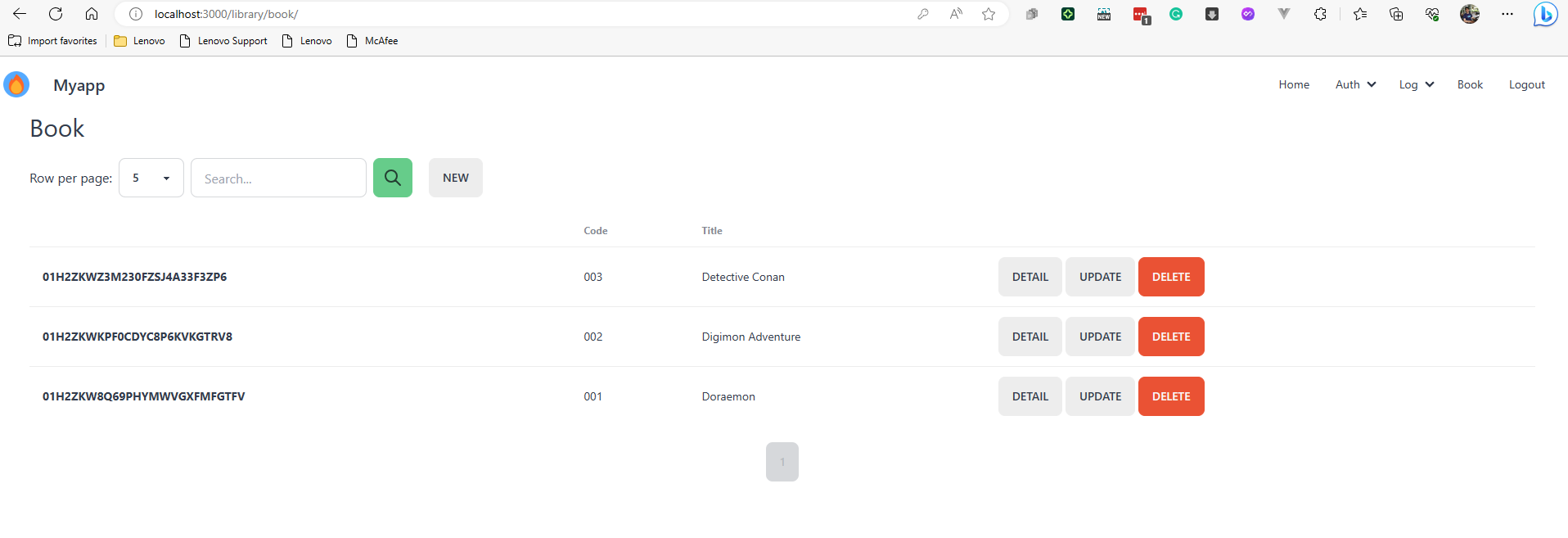
Furthermore, you can also split your application into `microservices`, run them as `docker containers`, and even deploy them to your `kubernetes cluster`.
```bash
# Run Fastapp as microservices
zrb project fastapp microservices start
# Run Fastapp as container
zrb project fastapp container microservices start
zrb project fastapp container stop
# Deploy fastapp and all it's dependencies to kubernetes
docker login
zrb project fastapp microservices deploy
```
Visit [our tutorials](https://github.com/state-alchemists/zrb/blob/main/docs/tutorials/README.md) to see more cool tricks.
# ๐ Documentation
- [๐ซฐ Installation](https://github.com/state-alchemists/zrb/blob/main/docs/installation.md)
- [๐ Getting Started](https://github.com/state-alchemists/zrb/blob/main/docs/getting-started.md)
- [๐ Documentation](https://github.com/state-alchemists/zrb/blob/main/docs/README.md)
- [๐ Common Mistakes](https://github.com/state-alchemists/zrb/blob/main/docs/oops-i-did-it-again/README.md)
- [โ FAQ](https://github.com/state-alchemists/zrb/blob/main/docs/faq/README.md)
# ๐ Bug Report + Feature Request
You can submit bug report and feature request by creating a new [issue](https://github.com/state-alchemists/zrb/issues) on Zrb Github Repositories. When reporting a bug or requesting a feature, please be sure to:
- Include the version of Zrb you are using (i.e., `zrb version`)
- Tell us what you have try
- Tell us what you expect
- Tell us what you get
We will also welcome your [pull requests and contributions](https://github.com/state-alchemists/zrb/pulls).
# โ Donation
Help Red Skull to click the donation button:
[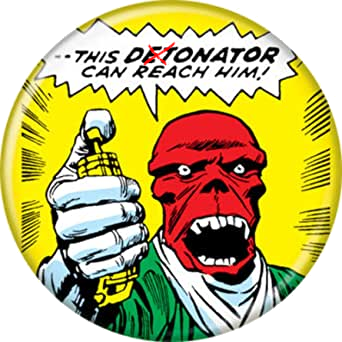](https://stalchmst.com/donation)
# ๐ Fun Fact
> Madou Ring Zaruba (้ญๅฐ่ผชใถใซใ, Madลrin Zaruba) is a Madougu which supports bearers of the Garo Armor. [(Garo Wiki | Fandom)](https://garo.fandom.com/wiki/Zaruba)
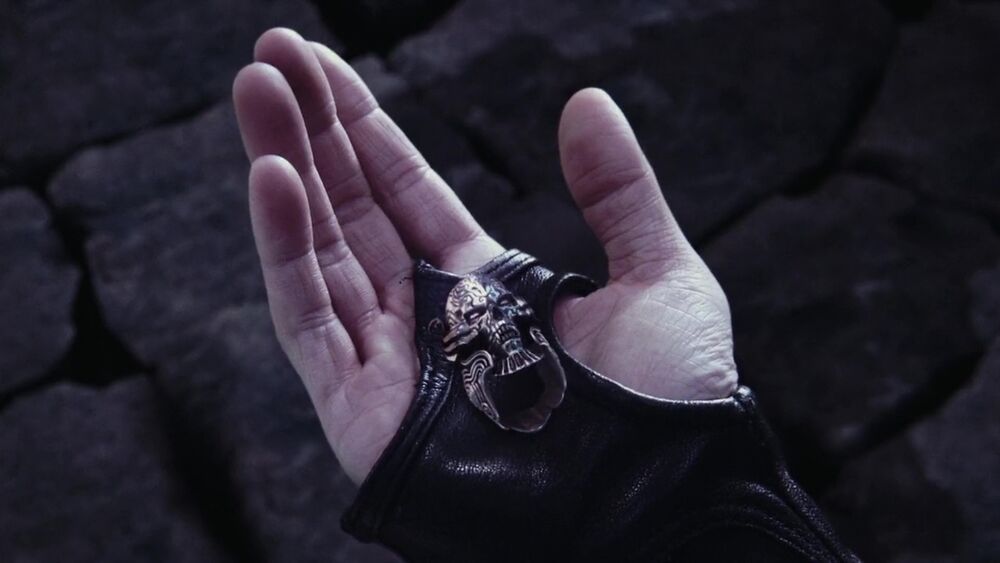
# ๐ Credits
We are thankful for the following libraries and services. They accelerate Zrb development processes and make things more fun.
- Libraries
- [Beartype](https://pypi.org/project/beartype/): Catch invalid typing earlier during runtime.
- [Click](https://pypi.org/project/click/): Creating a beautiful CLI interface.
- [Termcolor](https://pypi.org/project/termcolor/): Make the terminal interface more colorful.
- [Jinja](https://pypi.org/project/Jinja2): A robust templating engine.
- [Ruamel.yaml](https://pypi.org/project/ruamel.yaml/): Parse YAML effortlessly.
- [Jsons](https://pypi.org.project/jsons/): Parse JSON. This package should be part of the standard library.
- [Libcst](https://pypi.org/project/libcst/): Turn Python code into a Concrete Syntax Tree.
- [Croniter](https://pypi.org/project/croniter/): Parse cron pattern.
- [Flit](https://pypi.org/project/flit), [Twine](https://pypi.org/project/twine/), and many more. See the complete list of Zrb's requirements.txt
- Services
- [asciiflow.com](https://asciiflow.com/): Creates beautiful ASCII-based diagrams.
- [emojipedia.org](https://emojipedia.org/): Find emoji.
- [emoji.aranja.com](https://emoji.aranja.com/): Turns emoji into images
- [favicon.io](https://favicon.io/): Turns pictures and texts (including emoji) into favicon.
Raw data
{
"_id": null,
"home_page": "https://github.com/state-alchemists/zrb",
"name": "zrb",
"maintainer": null,
"docs_url": null,
"requires_python": "<4.0.0,>=3.10.0",
"maintainer_email": null,
"keywords": "Automation, Task Runner, Code Generator, Low Code",
"author": "Go Frendi Gunawan",
"author_email": "gofrendiasgard@gmail.com",
"download_url": "https://files.pythonhosted.org/packages/f6/d6/719eea1b7c5de975850a2a5d347f776a08863f069210756c4f1f76e53544/zrb-0.15.1.tar.gz",
"platform": null,
"description": "\n\n\n[\ud83e\udef0 Installation](https://github.com/state-alchemists/zrb/blob/main/docs/installation.md) | [\ud83d\udcd6 Documentation](https://github.com/state-alchemists/zrb/blob/main/docs/README.md) | [\ud83c\udfc1 Getting Started](https://github.com/state-alchemists/zrb/blob/main/docs/getting-started.md) | [\ud83d\udc83 Common Mistakes](https://github.com/state-alchemists/zrb/blob/main/docs/oops-i-did-it-again/README.md) | [\u2753 FAQ](https://github.com/state-alchemists/zrb/blob/main/docs/faq/README.md)\n\n\n# \ud83e\udd16 Zrb: A Framework to Enhance Your Workflow\n\nZrb is a [CLI-based](https://en.wikipedia.org/wiki/Command-line_interface) automation [tool](https://en.wikipedia.org/wiki/Programming_tool) and [low-code](https://en.wikipedia.org/wiki/Low-code_development_platform) platform. Zrb can help you to:\n\n- __Automate__ day-to-day tasks.\n- __Generate__ projects or applications.\n- __Prepare__, __run__, and __deploy__ your applications with a single command.\n- Etc.\n\nYou can also write custom task definitions in [Python](https://www.python.org/), enhancing Zrb's base capabilities. Defining your tasks in Zrb gives you several advantages because:\n\n- Every task has a __retry mechanism__.\n- Zrb handles your __task dependencies__ automatically.\n- Zrb runs your task dependencies __concurrently__.\n\n# \ud83e\udef0 Installing Zrb\n\nYou can install Zrb as a pip package by invoking the following command:\n\n```bash\npip install zrb\n```\n\nAlternatively, you can also use our installation script to install Zrb along with some prerequisites:\n\n```bash\nsource <(curl -s https://raw.githubusercontent.com/state-alchemists/zrb/main/install.sh)\n```\n\nCheck our [installation guide](https://github.com/state-alchemists/zrb/blob/main/docs/installation.md) for more information about the installation methods, including installation as a docker container.\n\n# \u2699\ufe0f Zrb as A Task-Automation Tool\n\nAt the very core, Zrb is a task automation tool. It helps you to automate some tedious jobs so that you can focus on what matters.\n\nLet's say you want to be able to describe the statistics property of any public CSV dataset. To do this, you need to perform three tasks like the following:\n\n- Install pandas.\n- Download the CSV dataset (at the same time).\n- Show statistics properties of the CSV dataset.\n\n```\n \ud83d\udc3c\nInstall Pandas \u2500\u2500\u2500\u2500\u2500\u2510 \ud83d\udcca\n \u251c\u2500\u2500\u25ba Show Statistics\nDownload Datasets \u2500\u2500\u2518\n \u2b07\ufe0f\n```\n\nYou can create a file named `zrb_init.py` and define the tasks as follows:\n\n```python\n# File name: zrb_init.py\nfrom zrb import runner, Parallel, CmdTask, python_task, StrInput\n\nDEFAULT_URL = 'https://raw.githubusercontent.com/state-alchemists/datasets/main/iris.csv'\n\n# \ud83d\udc3c Define a task named `install-pandas` to install pandas.\n# If this task failed, we want Zrb to retry it again 4 times at most.\ninstall_pandas = CmdTask(\n name='install-pandas',\n cmd='pip install pandas',\n retry=4\n)\n\n# \u2b07\ufe0f Define a task named `download-dataset` to download dataset.\n# This task has an input named `url`.\n# The input will be accessible by using Jinja template: `{{input.url}}`\n# If this task failed, we want Zrb to retry it again 4 times at most\ndownload_dataset = CmdTask(\n name='download-dataset',\n inputs=[\n StrInput(name='url', default=DEFAULT_URL)\n ],\n cmd='wget -O dataset.csv {{input.url}}',\n retry=4\n)\n\n# \ud83d\udcca Define a task named `show-stat` to show the statistics properties of the dataset.\n# @python_task` decorator turns a function into a Zrb Task (i.e., `show_stat` is now a Zrb Task).\n# If this task failed, we don't want to retry\n@python_task(\n name='show-stats',\n retry=0\n)\ndef show_stats(*args, **kwargs):\n import pandas as pd\n df = pd.read_csv('dataset.csv')\n return df.describe()\n\n# Define dependencies: `show_stat` depends on both, `download_dataset` and `install_pandas`\nParallel(download_dataset, install_pandas) >> show_stats\n\n# Register the tasks so that they are accessbie from the CLI\nrunner.register(install_pandas, download_dataset, show_stats)\n```\n\n> __\ud83d\udcdd NOTE:__ It is possible (although less readable) to define `show_stat` as `CmdTask`:\n> <details>\n> <summary>Show code</summary>\n>\n> ```python\n> show_stats = CmdTask(\n> name='show-stats',\n> cmd='python -c \"import pandas as pd; df=pd.read_csv(\\'dataset.csv\\'); print(df.describe())\"',\n> retry=0\n> )\n> ```\n> </details>\n\n\nOnce you write the definitions, Zrb will automatically load your `zrb_init.py` so that you can invoke your registered task:\n\n```bash\nzrb show-stat\n```\n\nThe command will give you the statistics property of the dataset:\n\n```\n sepal_length sepal_width petal_length petal_width\ncount 150.000000 150.000000 150.000000 150.000000\nmean 5.843333 3.054000 3.758667 1.198667\nstd 0.828066 0.433594 1.764420 0.763161\nmin 4.300000 2.000000 1.000000 0.100000\n25% 5.100000 2.800000 1.600000 0.300000\n50% 5.800000 3.000000 4.350000 1.300000\n75% 6.400000 3.300000 5.100000 1.800000\nmax 7.900000 4.400000 6.900000 2.500000\n```\n\n<details>\n<summary>See the full output</summary>\n\n```\nUrl [https://raw.githubusercontent.com/state-alchemists/datasets/main/iris.csv]:\n\ud83e\udd16 \u25cb \u25f7 \u2741 43598 \u2192 1/3 \ud83d\udc2e zrb project install-pandas \u2022 Run script: pip install pandas\n\ud83e\udd16 \u25cb \u25f7 \u2741 43598 \u2192 1/3 \ud83d\udc2e zrb project install-pandas \u2022 Working directory: /home/gofrendi/playground/my-project\n\ud83e\udd16 \u25cb \u25f7 \u2741 43598 \u2192 1/3 \ud83c\udf53 zrb project download-dataset \u2022 Run script: wget -O dataset.csv https://raw.githubusercontent.com/state-alchemists/datasets/main/iris.csv\n\ud83e\udd16 \u25cb \u25f7 \u2741 43598 \u2192 1/3 \ud83c\udf53 zrb project download-dataset \u2022 Working directory: /home/gofrendi/playground/my-project\n\ud83e\udd16 \u25b3 \u25f7 \u2741 43603 \u2192 1/3 \ud83c\udf53 zrb project download-dataset \u2022 --2023-11-12 09:45:12-- https://raw.githubusercontent.com/state-alchemists/datasets/main/iris.csv\n\ud83e\udd16 \u25b3 \u25f7 \u2741 43603 \u2192 1/3 \ud83c\udf53 zrb project download-dataset \u2022 Resolving raw.githubusercontent.com (raw.githubusercontent.com)... 185.199.111.133, 185.199.109.133, 185.199.110.133, ...\n\ud83e\udd16 \u25b3 \u25f7 \u2741 43603 \u2192 1/3 \ud83c\udf53 zrb project download-dataset \u2022 Connecting to raw.githubusercontent.com (raw.githubusercontent.com)|185.199.111.133|:443... connected.\n\ud83e\udd16 \u25b3 \u25f7 \u2741 43603 \u2192 1/3 \ud83c\udf53 zrb project download-dataset \u2022 HTTP request sent, awaiting response... 200 OK\n\ud83e\udd16 \u25b3 \u25f7 \u2741 43603 \u2192 1/3 \ud83c\udf53 zrb project download-dataset \u2022 Length: 4606 (4.5K) [text/plain]\n\ud83e\udd16 \u25b3 \u25f7 \u2741 43603 \u2192 1/3 \ud83c\udf53 zrb project download-dataset \u2022 Saving to: \u2018dataset.csv\u2019\n\ud83e\udd16 \u25b3 \u25f7 \u2741 43603 \u2192 1/3 \ud83c\udf53 zrb project download-dataset \u2022\n\ud83e\udd16 \u25b3 \u25f7 \u2741 43603 \u2192 1/3 \ud83c\udf53 zrb project download-dataset \u2022 0K .... 100% 1.39M=0.003s\n\ud83e\udd16 \u25b3 \u25f7 \u2741 43603 \u2192 1/3 \ud83c\udf53 zrb project download-dataset \u2022\n\ud83e\udd16 \u25b3 \u25f7 \u2741 43603 \u2192 1/3 \ud83c\udf53 zrb project download-dataset \u2022 2023-11-12 09:45:12 (1.39 MB/s) - \u2018dataset.csv\u2019 saved [4606/4606]\n\ud83e\udd16 \u25b3 \u25f7 \u2741 43603 \u2192 1/3 \ud83c\udf53 zrb project download-dataset \u2022\n\ud83e\udd16 \u25cb \u25f7 \u2741 43601 \u2192 1/3 \ud83d\udc2e zrb project install-pandas \u2022 Requirement already satisfied: pandas in /home/gofrendi/zrb/.venv/lib/python3.10/site-packages (2.1.3)\n\ud83e\udd16 \u25cb \u25f7 \u2741 43601 \u2192 1/3 \ud83d\udc2e zrb project install-pandas \u2022 Requirement already satisfied: numpy<2,>=1.22.4 in /home/gofrendi/zrb/.venv/lib/python3.10/site-packages (from pandas) (1.26.1)\n\ud83e\udd16 \u25cb \u25f7 \u2741 43601 \u2192 1/3 \ud83d\udc2e zrb project install-pandas \u2022 Requirement already satisfied: python-dateutil>=2.8.2 in /home/gofrendi/zrb/.venv/lib/python3.10/site-packages (from pandas) (2.8.2)\n\ud83e\udd16 \u25cb \u25f7 \u2741 43601 \u2192 1/3 \ud83d\udc2e zrb project install-pandas \u2022 Requirement already satisfied: pytz>=2020.1 in /home/gofrendi/zrb/.venv/lib/python3.10/site-packages (from pandas) (2023.3.post1)\n\ud83e\udd16 \u25cb \u25f7 \u2741 43601 \u2192 1/3 \ud83d\udc2e zrb project install-pandas \u2022 Requirement already satisfied: tzdata>=2022.1 in /home/gofrendi/zrb/.venv/lib/python3.10/site-packages (from pandas) (2023.3)\n\ud83e\udd16 \u25cb \u25f7 \u2741 43601 \u2192 1/3 \ud83d\udc2e zrb project install-pandas \u2022 Requirement already satisfied: six>=1.5 in /home/gofrendi/zrb/.venv/lib/python3.10/site-packages (from python-dateutil>=2.8.2->pandas) (1.16.0)\nSupport zrb growth and development!\n\u2615 Donate at: https://stalchmst.com/donation\n\ud83d\udc19 Submit issues/PR at: https://github.com/state-alchemists/zrb\n\ud83d\udc24 Follow us at: https://twitter.com/zarubastalchmst\n\ud83e\udd16 \u25cb \u25f7 2023-11-12 09:45:14.366 \u2741 43598 \u2192 1/3 \ud83c\udf4e zrb project show-stats \u2022 Completed in 2.2365798950195312 seconds\n sepal_length sepal_width petal_length petal_width\ncount 150.000000 150.000000 150.000000 150.000000\nmean 5.843333 3.054000 3.758667 1.198667\nstd 0.828066 0.433594 1.764420 0.763161\nmin 4.300000 2.000000 1.000000 0.100000\n25% 5.100000 2.800000 1.600000 0.300000\n50% 5.800000 3.000000 4.350000 1.300000\n75% 6.400000 3.300000 5.100000 1.800000\nmax 7.900000 4.400000 6.900000 2.500000\nTo run again: zrb project show-stats --url \"https://raw.githubusercontent.com/state-alchemists/datasets/main/iris.csv\"\n```\n</details>\n\nSince you have registered `install_pandas` and `download_dataset` (i.e., `runner.register(install_pandas, download_dataset)`), then you can also execute those tasks as well:\n\n```bash\nzrb install-pandas\nzrb download-dataset\n```\n\n> __\ud83d\udcdd NOTE:__ When executing a task, you can also provide the parameter directly, for example you want to provide the `url`\n>\n> ```bash\n> zrb show-stat --url https://raw.githubusercontent.com/state-alchemists/datasets/main/iris.csv\n> ```\n>\n> When you provide the parameter directly, Zrb will not prompt you to supply the URL.\n> Another way to disable the prompt is by set `ZRB_SHOW_PROMPT` to `0` or `false`.\n\nYou can also run a Docker compose file, start a Web server, generate a CRUD application, or set up multiple servers simultaneously.\n\nSee [our getting started guide](https://github.com/state-alchemists/zrb/blob/main/docs/getting-started.md) and [tutorials](https://github.com/state-alchemists/zrb/blob/main/docs/tutorials/README.md) to learn more about the details.\n\n# \u2702\ufe0f Zrb as A Low-Code Framework\n\nZrb has some built-in tasks that allow you to create and run a [CRUD](https://en.wikipedia.org/wiki/Create,_read,_update_and_delete) application.\n\nLet's see the following example.\n\n```bash\n# Create a project\nzrb project create --project-dir my-project --project-name \"My Project\"\ncd my-project\n\n# Create a Fastapp\nzrb project add fastapp app --project-dir . --app-name \"fastapp\" --http-port 3000\n\n# Add library module to fastapp\nzrb project add fastapp module --project-dir . --app-name \"fastapp\" --module-name \"library\"\n\n# Add entity named \"books\"\nzrb project add fastapp crud --project-dir . --app-name \"fastapp\" --module-name \"library\" \\\n --entity-name \"book\" --plural-entity-name \"books\" --column-name \"code\"\n\n# Add column to the entity\nzrb project add fastapp field --project-dir . --app-name \"fastapp\" --module-name \"library\" \\\n --entity-name \"book\" --column-name \"title\" --column-type \"str\"\n\n# Run Fastapp as monolith\nzrb project fastapp monolith start\n```\n\nOnce you invoke the commands, you will be able to access the CRUD application by pointing your browser to [http://localhost:3000](http://localhost:3000)\n\n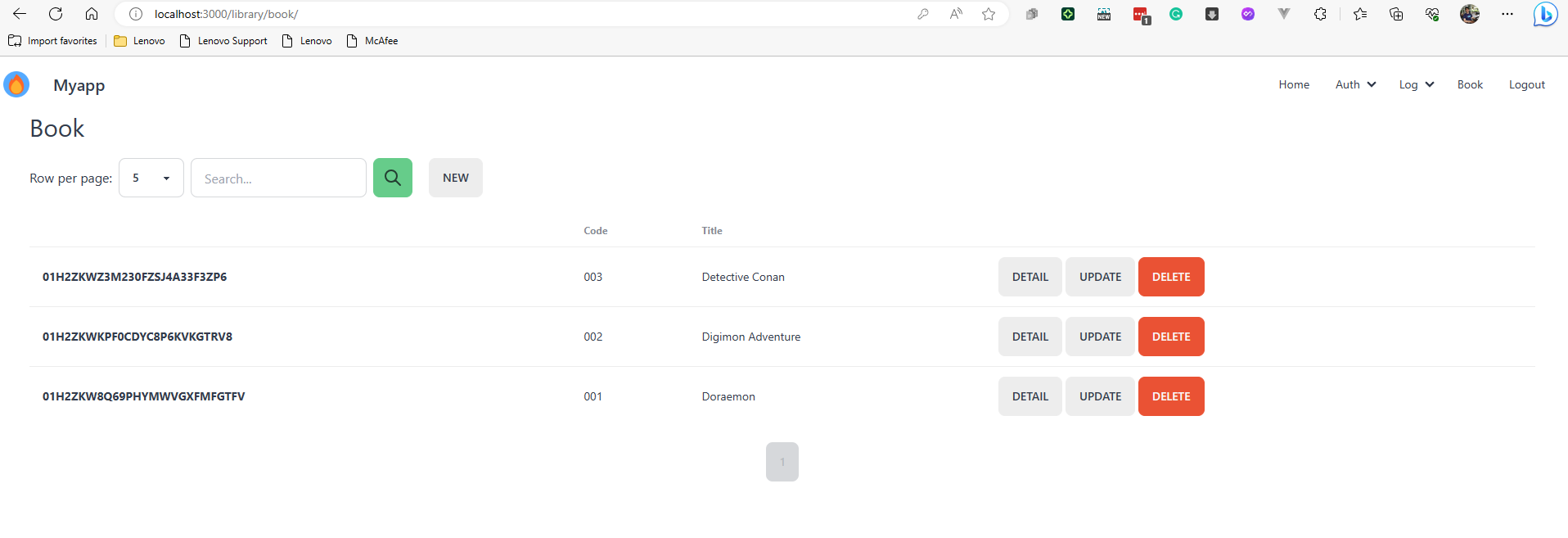\n\nFurthermore, you can also split your application into `microservices`, run them as `docker containers`, and even deploy them to your `kubernetes cluster`.\n\n```bash\n# Run Fastapp as microservices\nzrb project fastapp microservices start\n\n# Run Fastapp as container\nzrb project fastapp container microservices start\nzrb project fastapp container stop\n\n# Deploy fastapp and all it's dependencies to kubernetes\ndocker login\nzrb project fastapp microservices deploy\n```\n\nVisit [our tutorials](https://github.com/state-alchemists/zrb/blob/main/docs/tutorials/README.md) to see more cool tricks.\n\n# \ud83d\udcd6 Documentation\n\n- [\ud83e\udef0 Installation](https://github.com/state-alchemists/zrb/blob/main/docs/installation.md)\n- [\ud83c\udfc1 Getting Started](https://github.com/state-alchemists/zrb/blob/main/docs/getting-started.md)\n- [\ud83d\udcd6 Documentation](https://github.com/state-alchemists/zrb/blob/main/docs/README.md)\n- [\ud83d\udc83 Common Mistakes](https://github.com/state-alchemists/zrb/blob/main/docs/oops-i-did-it-again/README.md)\n- [\u2753 FAQ](https://github.com/state-alchemists/zrb/blob/main/docs/faq/README.md)\n\n# \ud83d\udc1e Bug Report + Feature Request\n\nYou can submit bug report and feature request by creating a new [issue](https://github.com/state-alchemists/zrb/issues) on Zrb Github Repositories. When reporting a bug or requesting a feature, please be sure to:\n\n- Include the version of Zrb you are using (i.e., `zrb version`)\n- Tell us what you have try\n- Tell us what you expect\n- Tell us what you get\n\nWe will also welcome your [pull requests and contributions](https://github.com/state-alchemists/zrb/pulls).\n\n\n# \u2615 Donation\n\nHelp Red Skull to click the donation button:\n\n[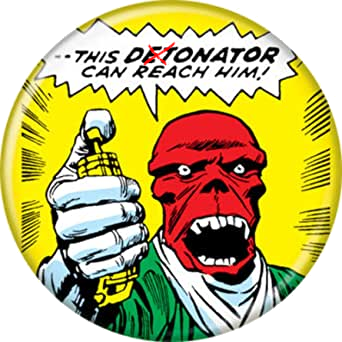](https://stalchmst.com/donation)\n\n# \ud83c\udf89 Fun Fact\n\n> Madou Ring Zaruba (\u9b54\u5c0e\u8f2a\u30b6\u30eb\u30d0, Mad\u014drin Zaruba) is a Madougu which supports bearers of the Garo Armor. [(Garo Wiki | Fandom)](https://garo.fandom.com/wiki/Zaruba)\n\n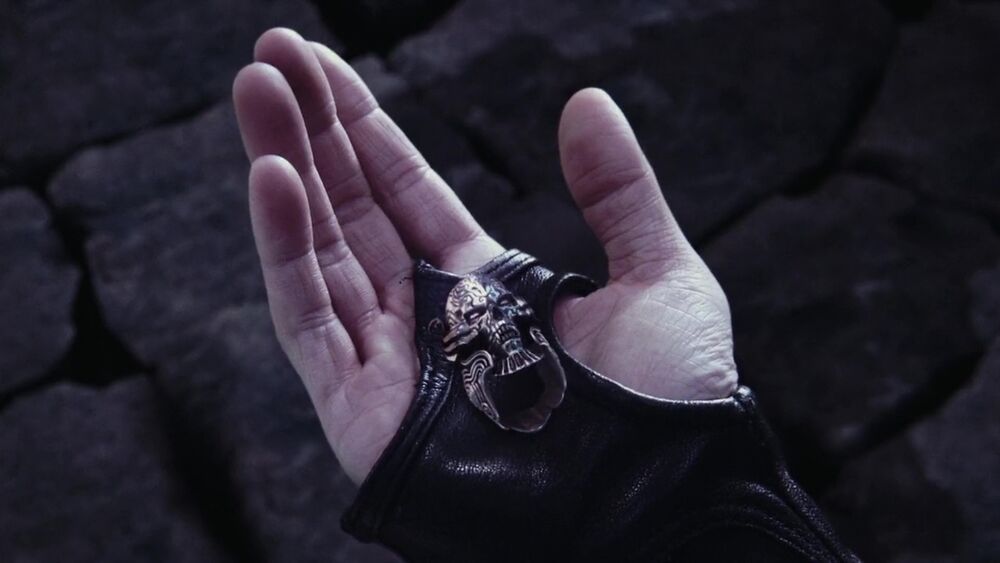\n\n# \ud83d\ude47 Credits\n\nWe are thankful for the following libraries and services. They accelerate Zrb development processes and make things more fun.\n\n- Libraries\n - [Beartype](https://pypi.org/project/beartype/): Catch invalid typing earlier during runtime.\n - [Click](https://pypi.org/project/click/): Creating a beautiful CLI interface.\n - [Termcolor](https://pypi.org/project/termcolor/): Make the terminal interface more colorful.\n - [Jinja](https://pypi.org/project/Jinja2): A robust templating engine.\n - [Ruamel.yaml](https://pypi.org/project/ruamel.yaml/): Parse YAML effortlessly.\n - [Jsons](https://pypi.org.project/jsons/): Parse JSON. This package should be part of the standard library.\n - [Libcst](https://pypi.org/project/libcst/): Turn Python code into a Concrete Syntax Tree.\n - [Croniter](https://pypi.org/project/croniter/): Parse cron pattern.\n - [Flit](https://pypi.org/project/flit), [Twine](https://pypi.org/project/twine/), and many more. See the complete list of Zrb's requirements.txt\n- Services\n - [asciiflow.com](https://asciiflow.com/): Creates beautiful ASCII-based diagrams.\n - [emojipedia.org](https://emojipedia.org/): Find emoji.\n - [emoji.aranja.com](https://emoji.aranja.com/): Turns emoji into images\n - [favicon.io](https://favicon.io/): Turns pictures and texts (including emoji) into favicon.\n",
"bugtrack_url": null,
"license": "AGPL-3.0-or-later",
"summary": "A Framework to Enhance Your Workflow",
"version": "0.15.1",
"project_urls": {
"Documentation": "https://github.com/state-alchemists/zrb",
"Homepage": "https://github.com/state-alchemists/zrb",
"Repository": "https://github.com/state-alchemists/zrb"
},
"split_keywords": [
"automation",
" task runner",
" code generator",
" low code"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "6e2a661f0d584465fb2a1e4c3202c3363a7121286378de7c0e56964de5cfbaf2",
"md5": "fd87d7c95cb6fed2f6addb4d604cc92f",
"sha256": "32f624526d3bb3c4a7a10b81b28b45992f44bcacd587b1c672d231dc07224768"
},
"downloads": -1,
"filename": "zrb-0.15.1-py3-none-any.whl",
"has_sig": false,
"md5_digest": "fd87d7c95cb6fed2f6addb4d604cc92f",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": "<4.0.0,>=3.10.0",
"size": 2931426,
"upload_time": "2024-05-01T09:07:40",
"upload_time_iso_8601": "2024-05-01T09:07:40.151376Z",
"url": "https://files.pythonhosted.org/packages/6e/2a/661f0d584465fb2a1e4c3202c3363a7121286378de7c0e56964de5cfbaf2/zrb-0.15.1-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "f6d6719eea1b7c5de975850a2a5d347f776a08863f069210756c4f1f76e53544",
"md5": "1f129a8d6258db388a11c0826130dfce",
"sha256": "e663182d32d9c35b37b368121fc4878697bcab4c7ece0bf0663f70702d6adb4a"
},
"downloads": -1,
"filename": "zrb-0.15.1.tar.gz",
"has_sig": false,
"md5_digest": "1f129a8d6258db388a11c0826130dfce",
"packagetype": "sdist",
"python_version": "source",
"requires_python": "<4.0.0,>=3.10.0",
"size": 2181151,
"upload_time": "2024-05-01T09:07:44",
"upload_time_iso_8601": "2024-05-01T09:07:44.386132Z",
"url": "https://files.pythonhosted.org/packages/f6/d6/719eea1b7c5de975850a2a5d347f776a08863f069210756c4f1f76e53544/zrb-0.15.1.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-05-01 09:07:44",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "state-alchemists",
"github_project": "zrb",
"travis_ci": false,
"coveralls": true,
"github_actions": true,
"lcname": "zrb"
}