# PySDKit: signal decomposition in Python
<div align="center">
[](https://pypi.org/project/PySDKit/)

[](https://www.python.org/)
[](https://pepy.tech/project/pysdkit)

[](https://github.com/psf/black)
A Python library for signal decomposition algorithms 🥳
[Installation](#Installation) |
[Example Script](#Example-Script) |
[Target](#Target) |
[Acknowledgements](#Acknowledgements)
<img src="https://raw.githubusercontent.com/wwhenxuan/PySDKit/main/images/Logo_sd.png" alt="Logo_sd" width="500"/>
</div>
## Installation 🚀 <a id="Installation"></a>
You can install `PySDKit` through pip:
~~~
pip install pysdkit
~~~
We only used [`NumPy`](https://numpy.org/), [`Scipy`](https://scipy.org/) and [`matplotlib`](https://matplotlib.org/) when developing the project.
## Example Script ✨ <a id="Example-Script"></a>
This project integrates simple signal processing methods, signal decomposition and visualization, and builds a general interface similar to [`Scikit-learn`](https://scikit-learn.org/stable/). It is mainly divided into three steps:
1. Import the signal decomposition method;
2. Create an instance for signal decomposition;
3. Use the `fit_transform` method to implement signal decomposition;
4. Visualize and analyze the original signal and the intrinsic mode functions IMFs obtained by decomposition.
~~~python
from pysdkit import EMD
from pysdkit.data import test_emd
from pysdkit.plot import plot_IMFs
t, signal = test_emd()
# create an instance for signal decomposition
emd = EMD()
# implement signal decomposition
IMFs = emd.fit_transform(signal, max_imfs=2)
plot_IMFs(signal, IMFs)
~~~
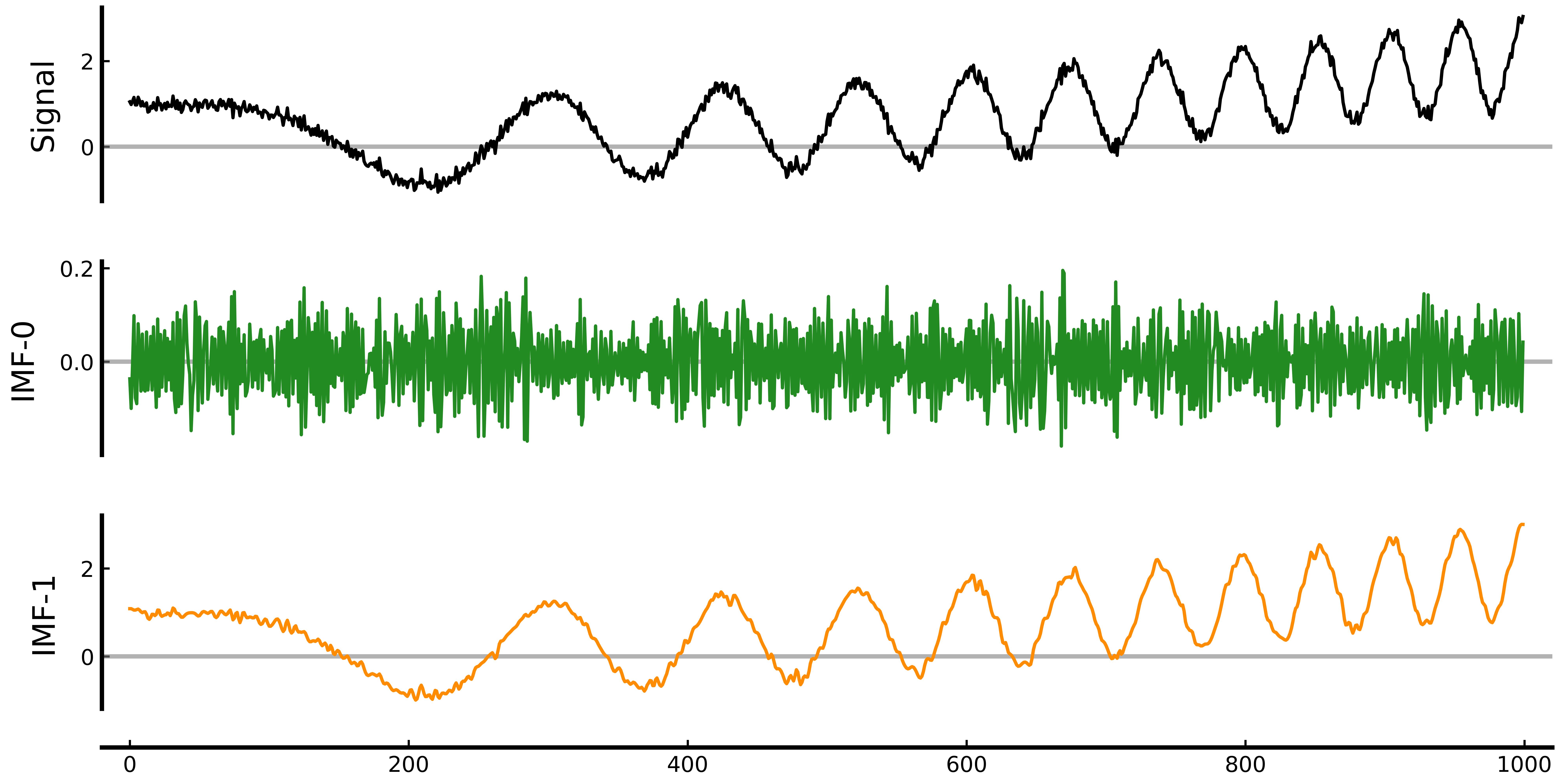
The EMD in the above example is the most classic [`empirical mode decomposition`](https://www.mathworks.com/help/signal/ref/emd.html) algorithm in signal decomposition. For more complex signals, you can try other algorithms such as variational mode decomposition ([`VMD`](https://ieeexplore.ieee.org/abstract/document/6655981)).
~~~python
import numpy as np
from pysdkit import VMD
# load new signal
signal = np.load("./example/example.npy")
# use variational mode decomposition
vmd = VMD(alpha=500, K=3, tau=0.0, tol=1e-9)
IMFs = vmd.fit_transform(signal=signal)
print(IMFs.shape)
vmd.plot_IMFs(save_figure=True)
~~~
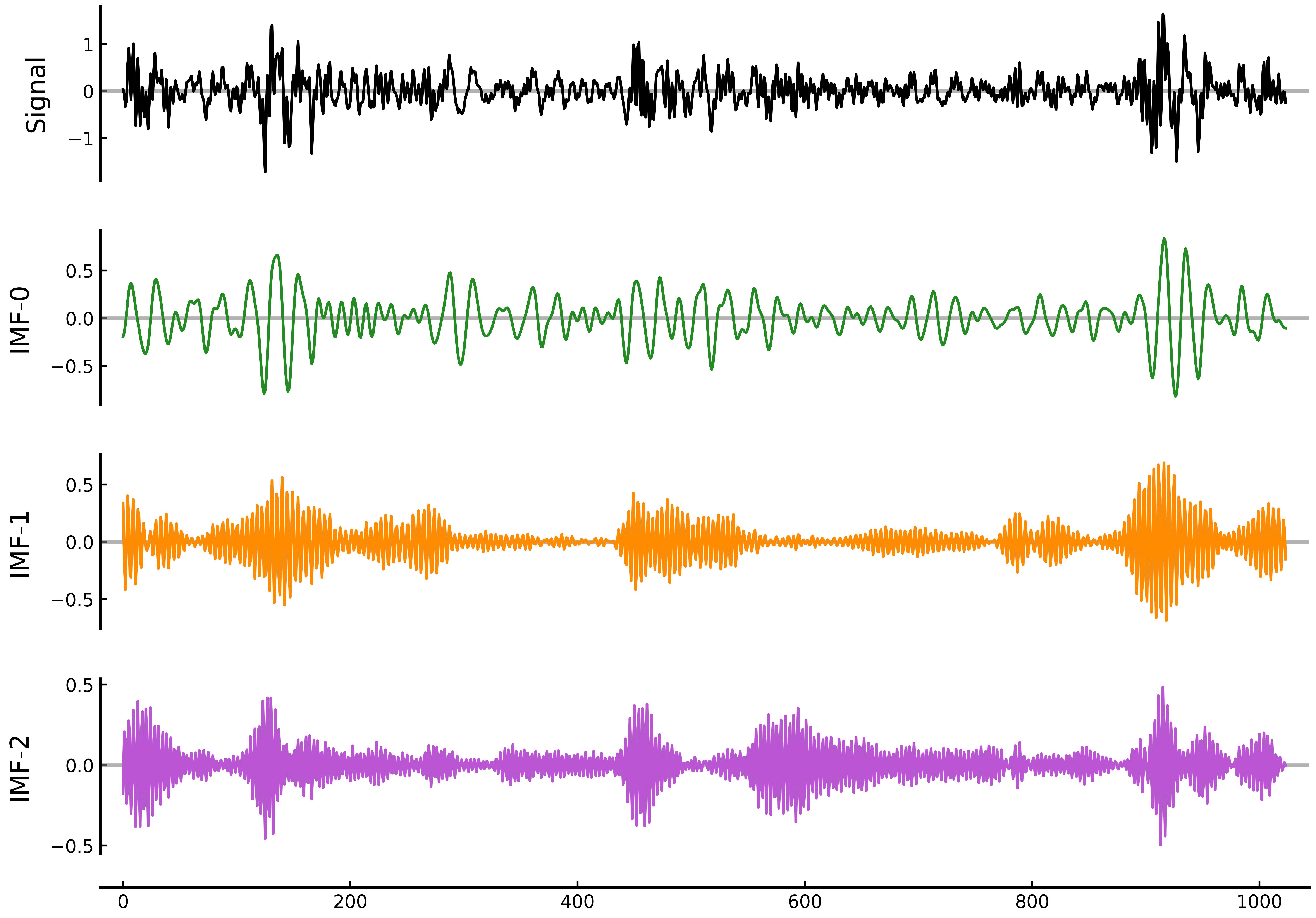
Better observe the characteristics of the decomposed intrinsic mode function in the frequency domain.
~~~python
from pysdkit.plot import plot_IMFs_amplitude_spectra
# frequency domain visualization
plot_IMFs_amplitude_spectra(IMFs, smooth="exp") # use exp smooth
~~~
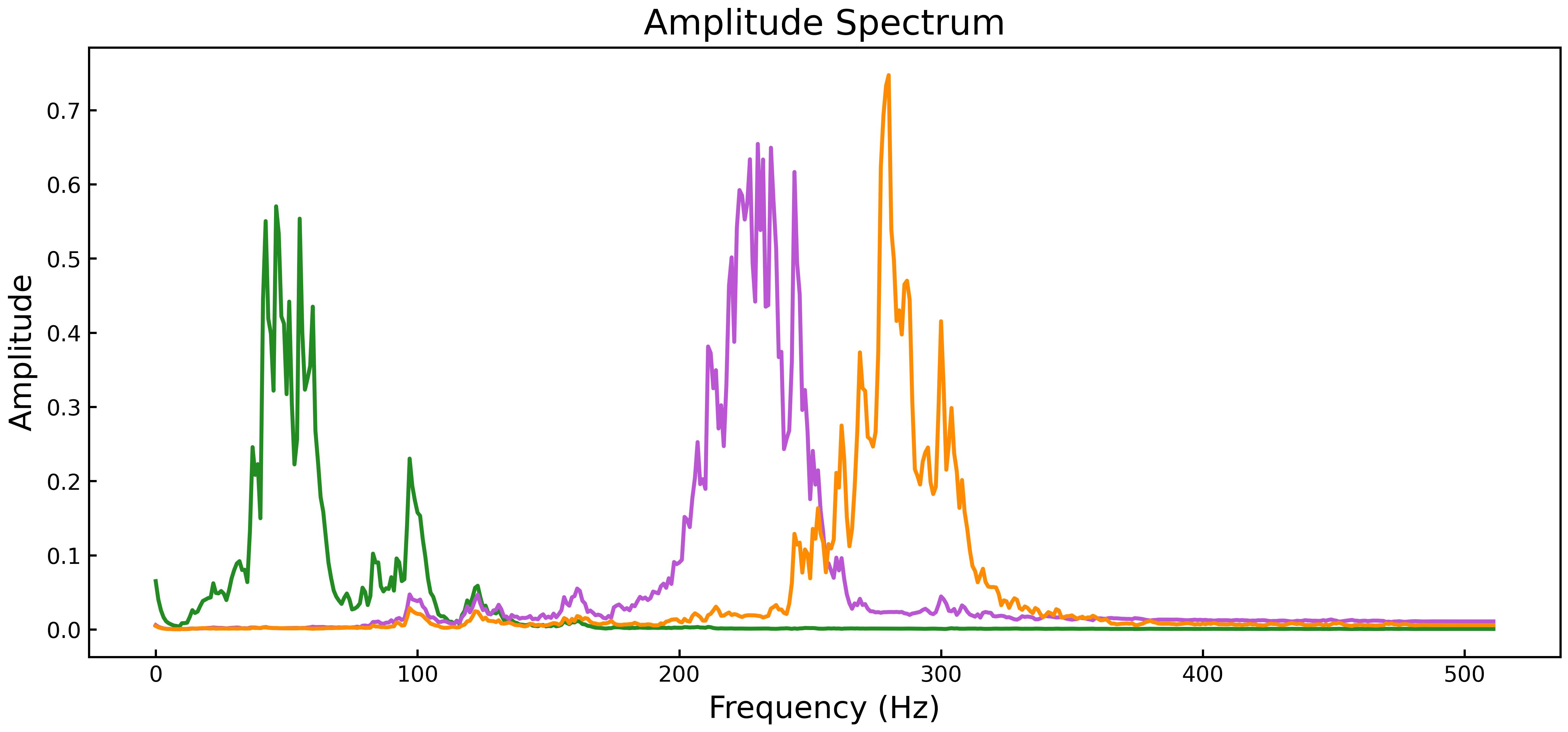
## Target 🎯 <a id="Target"></a>
`PySDKit` is still under development. We are currently working on reproducing the signal decomposition algorithms in the table below, including not only common decomposition algorithms for `univariate signals` such as EMD and VMD, but also decomposition algorithms for `multivariate signals` such as MEMD and MVMD. We will also further reproduce the decomposition algorithms for `two-dimensional images` to make PySDKit not only suitable for signal processing, but also for image analysis and understanding.
| Algorithm | Paper | Code | State |
| :----------------------------------------------------------: | :----------------------------------------------------------: | :----------------------------------------------------------: | :---: |
| [`EMD`](https://github.com/wwhenxuan/PySDKit/blob/main/pysdkit/emd/EMD.py) (Empirical Mode Decomposition) | [[paper]](https://royalsocietypublishing.org/doi/abs/10.1098/rspa.1998.0193) | [[code]](https://www.mathworks.com/help/signal/ref/emd.html) | ✔️ |
| [`MEMD`](https://github.com/wwhenxuan/PySDKit/blob/main/pysdkit/emd/memd.py) (Multivariate Empirical Mode Decomposition) | [[paper]](https://royalsocietypublishing.org/doi/full/10.1098/rspa.2009.0502) | [[code]](https://www.mathworks.com/matlabcentral/fileexchange/71270-fast-and-adaptive-multivariate-and-multidimensional-emd) | ✔️ |
| [`BEMD`](https://github.com/wwhenxuan/PySDKit/blob/main/pysdkit/emd2d/bemd.py) (Bidimensional Empirical Mode Decomposition) | [[paper]](https://www.sciencedirect.com/science/article/abs/pii/S0262885603000945) | [[code]](https://github.com/laszukdawid/PyEMD/blob/master/PyEMD/BEMD.py) | ✖️ |
| [`CEMD`]() (Complex Empirical Mode Decomposition) | [[paper]](https://ieeexplore.ieee.org/abstract/document/4063369) | [[code]]() | ✖️ |
| [`EEMD`](https://github.com/wwhenxuan/PySDKit/blob/main/pysdkit/emd/eemd.py) (Ensemble Empirical Mode Decomposition) | [[paper]](https://www.sciencedirect.com/topics/physics-and-astronomy/ensemble-empirical-mode-decomposition) | [[code]](https://github.com/laszukdawid/PyEMD/blob/master/PyEMD/EEMD.py) | ✔️ |
| [`REMD`](https://github.com/wwhenxuan/PySDKit/blob/main/pysdkit/emd/remd.py) (Robust Empirical Mode Decomposition) | [[paper]](https://www.sciencedirect.com/science/article/pii/S0019057821003785) | [[code]](https://www.mathworks.com/matlabcentral/fileexchange/70032-robust-empirical-mode-decomposition-remd) | ✖️ |
| [`BMEMD`](https://github.com/wwhenxuan/PySDKit/blob/main/pysdkit/emd2d/bmemd.py) (Bidimensional Multivariate Empirical Mode Decomposition) | [[paper]](https://ieeexplore.ieee.org/document/8805082) | [[code]](https://www.mathworks.com/matlabcentral/fileexchange/72343-bidimensional-multivariate-empirical-mode-decomposition?s_tid=FX_rc1_behav) | ✖️ |
| [`CEEMDAN`](https://github.com/wwhenxuan/PySDKit/blob/main/pysdkit/emd/ceemdan.py) (Complete Ensemble EMD with Adaptive Noise) | [[paper]](https://ieeexplore.ieee.org/document/5947265) | [[code]](https://github.com/laszukdawid/PyEMD/blob/master/PyEMD/EEMD.py) | ✔️ |
| [`TVF_EMD`](https://github.com/wwhenxuan/PySDKit/blob/main/pysdkit/tvf_emd/tvf_emd.py) (Time Varying Filter Based EMD) | [[paper]](https://www.sciencedirect.com/science/article/pii/S0165168417301135) | [[code]](https://github.com/stfbnc/pytvfemd/tree/master) | ✔️ |
| [`FAEMD`](https://github.com/wwhenxuan/PySDKit/blob/main/pysdkit/faemd/faemd.py) (Fast and Adaptive EMD) | [[paper]](https://ieeexplore.ieee.org/document/8447300) | [[code]](https://www.mathworks.com/matlabcentral/fileexchange/71270-fast-and-adaptive-multivariate-and-multidimensional-emd) | ✖️ |
| [`MV_FAEMD`]() (Multivariate Fast and Adaptive EMD) | [[paper]](https://ieeexplore.ieee.org/document/8447300) | [[code]](https://www.mathworks.com/matlabcentral/fileexchange/71270-fast-and-adaptive-multivariate-and-multidimensional-emd) | ✖️ |
| [`MD_FAEMD`](https://github.com/wwhenxuan/PySDKit/blob/main/pysdkit/faemd/md_faemd.py) (Multidimensional Fast and Adaptive EMD) | [[paper]](https://ieeexplore.ieee.org/document/8447300) | [[code]](https://www.mathworks.com/matlabcentral/fileexchange/71270-fast-and-adaptive-multivariate-and-multidimensional-emd) | ✖️ |
| [`HVD`](https://github.com/wwhenxuan/PySDKit/blob/main/pysdkit/hvd/hvd.py) (Hilbert Vibration Decomposition) | [[paper]](https://www.sciencedirect.com/science/article/pii/S0022460X06001556) | [[code]](https://www.mathworks.com/matlabcentral/fileexchange/178804-hilbert-vibration-decomposition?s_tid=FX_rc1_behav) | ✔️ |
| [`ITD`](https://github.com/wwhenxuan/PySDKit/blob/main/pysdkit/itd/itd.py) (Intrinsic Time-Scale Decomposition) | [[paper]](https://royalsocietypublishing.org/doi/10.1098/rspa.2006.1761) | [[code]](https://www.mathworks.com/matlabcentral/fileexchange/69380-intrinsic-time-scale-decomposition-itd) | ✔️ |
| [`LMD`](https://github.com/wwhenxuan/PySDKit/blob/main/pysdkit/lmd/lmd.py) (Local Mean Decomposition) | [[paper]](https://www.sciencedirect.com/science/article/abs/pii/S1051200418308133?via%3Dihub) | [[code]](https://github.com/shownlin/PyLMD/blob/master/PyLMD/LMD.py) | ✔️ |
| [`RLMD`](https://github.com/wwhenxuan/PySDKit/blob/main/pysdkit/lmd/rlmd.py) (Robust Local Mean Decomposition) | [[paper]](https://www.sciencedirect.com/science/article/abs/pii/S0888327017301619) | [[code]](https://www.mathworks.com/matlabcentral/fileexchange/66935-robust-local-mean-decomposition-rlmd) | ✖️ |
| [`FMD`](https://github.com/wwhenxuan/PySDKit/blob/main/pysdkit/fmd/fmd.py) (Feature Mode Decomposition) | [[paper]](https://ieeexplore.ieee.org/document/9732251) | [[code]](https://www.mathworks.com/matlabcentral/fileexchange/108099-feature-mode-decomposition-fmd) | ✖️ |
| [`SSA`](https://github.com/wwhenxuan/PySDKit/blob/main/pysdkit/ssa/ssa.py) (Singular Spectral Analysis) | [[paper]](https://orca.cardiff.ac.uk/id/eprint/15208/1/Zhiglavsky_SSA_encyclopedia.pdf) | [[code]](https://www.mathworks.com/matlabcentral/fileexchange/58967-singular-spectrum-analysis-beginners-guide) | ✔️ |
| [`EWT`](https://github.com/wwhenxuan/PySDKit/blob/main/pysdkit/ewt/ewt.py) (Empirical Wavelet Transform) | [[paper]](https://ieeexplore.ieee.org/document/6522142) | [[code]](https://www.mathworks.com/help/wavelet/ug/empirical-wavelet-transform.html) | ✔️ |
| [`EWT2D`](https://github.com/wwhenxuan/PySDKit/blob/main/pysdkit/ewt/ewt2d.py) (2D Empirical Wavelet Transform) | [[paper]](https://arxiv.org/abs/2405.06188) | [[code]](https://github.com/bhurat/EWT-Python) | ✖️ |
| [`VMD`](https://github.com/wwhenxuan/PySDKit/blob/main/pysdkit/vmd/vmd_c.py) (Variational Mode Decomposition) | [[paper]](https://ieeexplore.ieee.org/document/6655981) | [[code]](https://github.com/vrcarva/vmdpy) | ✔️ |
| [`MVMD`](https://github.com/wwhenxuan/PySDKit/blob/main/pysdkit/vmd/mvmd.py) (Multivariate Variational Mode Decomposition) | [[paper]](https://ieeexplore.ieee.org/document/8890883) | [[code]](https://github.com/yunyueye/MVMD) | ✔️ |
| [`VMD2D`](https://github.com/wwhenxuan/PySDKit/blob/main/pysdkit/vmd2d/vmd2d.py) (Two-Dimensional Variational Mode Decomposition) | [[paper]](https://ww3.math.ucla.edu/camreport/cam14-16.pdf) | [[code]](https://www.mathworks.com/matlabcentral/fileexchange/45918-two-dimensional-variational-mode-decomposition?s_tid=srchtitle) | ✔️ |
| [`SVMD`](https://github.com/wwhenxuan/PySDKit/blob/main/pysdkit/vmd/svmd.py) (Successive Variational Mode Decomposition) | [[paper]](https://www.sciencedirect.com/science/article/pii/S0165168420301535) | [[code]](https://www.mathworks.com/matlabcentral/fileexchange/98649-successive-variational-mode-decomposition-svmd-m?s_tid=FX_rc3_behav) | ✖️ |
| [`VNCMD`](https://github.com/wwhenxuan/PySDKit/blob/main/pysdkit/vncmd/vncmd.py) (Variational Nonlinear Chirp Mode Decomposition) | [[paper]](https://ieeexplore.ieee.org/document/7990179) | [[code]](https://www.mathworks.com/matlabcentral/fileexchange/64292-variational-nonlinear-chirp-mode-decomposition) | ✔️ |
| [`MNCMD`](https://github.com/wwhenxuan/PySDKit/blob/main/pysdkit/vncmd/mncmd.py) (Multivariate Nonlinear Chirp Mode Decomposition) | [[paper]](https://www.sciencedirect.com/science/article/abs/pii/S0165168420302103) | [[code]]() | ✖️ |
| [`AVNCMD`](https://github.com/wwhenxuan/PySDKit/blob/main/pysdkit/vncmd/avncmd.py) (Adaptive Variational Nonlinear Chirp Mode Decomposition) | [[paper]](https://ieeexplore.ieee.org/abstract/document/9746147) | [[code]](https://github.com/HauLiang/AVNCMD) | ✖️ |
| [`ACMD`](https://github.com/wwhenxuan/PySDKit/blob/main/pysdkit/vmd/acmd.py) (Adaptive Chirp Mode Decomposition) | [[paper]]() | [[code]](https://www.mathworks.com/matlabcentral/fileexchange/121373-data-driven-adaptive-chirp-mode-decomposition?s_tid=srchtitle) | ✔️ |
| [`BA-ACMD`](https://github.com/wwhenxuan/PySDKit/blob/main/pysdkit/vmd/ba_acmd.py) (Bandwidth-aware adaptive chirp mode decomposition) | [[paper]](https://journals.sagepub.com/doi/abs/10.1177/14759217231174699) | [[code]](https://www.mathworks.com/matlabcentral/fileexchange/132792-bandwidth-aware-adaptive-chirp-mode-decomposition-ba-acmd?s_tid=srchtitle) | ✖️ |
| [`JMD`](https://github.com/wwhenxuan/PySDKit/blob/main/pysdkit/jmd/jmd.py) (Jump Plus AM-FM Mode Decomposition) | [[paper]](https://arxiv.org/abs/2407.07800) | [[code]](https://www.mathworks.com/matlabcentral/fileexchange/169388-jump-plus-am-fm-mode-decomposition-jmd?s_tid=prof_contriblnk) | ✖️ |
| [`MJMD`](https://github.com/wwhenxuan/PySDKit/blob/main/pysdkit/jmd/mjmd.py) (Multivariate Jump Plus AM-FM Mode Decomposition) | [[paper]](https://arxiv.org/abs/2407.07800) | [[code]](https://ms-intl.mathworks.com/matlabcentral/fileexchange/169393-multivariate-jump-plus-am-fm-mode-decomposition-mjmd?s_tid=FX_rc2_behav) | ✖️ |
| [`ESMD`]() (Extreme-Point Symmetric Mode Decomposition) | [[paper]](https://arxiv.org/abs/1303.6540) | [[code]](https://github.com/WuShichao/esmd) | ✖️ |
## Acknowledgements 🎖️ <a id="Acknowledgements"></a>
We would like to thank the researchers in signal processing for providing us with valuable algorithms and promoting the continuous progress in this field. However, since the main programming language used in `signal processing` is `Matlab`, and `Python` is the main battlefield of `machine learning` and `deep learning`, the usage of signal decomposition in machine learning and deep learning is far less extensive than `wavelet transformation`. In order to further promote the organic combination of signal decomposition and machine learning, we developed `PySDKit`. We would like to express our gratitude to [PyEMD](https://github.com/laszukdawid/PyEMD), [Sktime](https://www.sktime.net/en/latest/index.html), [vmdpy](https://github.com/vrcarva/vmdpy), [MEMD-Python-](https://github.com/mariogrune/MEMD-Python-), [ewtpy](https://github.com/vrcarva/ewtpy), [EWT-Python](https://github.com/bhurat/EWT-Python), [PyLMD](https://github.com/shownlin/PyLMD), [pywt](https://github.com/PyWavelets/pywt), [SP_Lib](https://github.com/hustcxl/SP_Lib)and [dsatools](https://github.com/MVRonkin/dsatools).
Raw data
{
"_id": null,
"home_page": "https://github.com/wwhenxuan/PySDKit",
"name": "PySDKit",
"maintainer": null,
"docs_url": null,
"requires_python": ">=3.6",
"maintainer_email": null,
"keywords": "signal decomposition, signal processing, machine learning",
"author": "whenxuan",
"author_email": "wwhenxuan@gmail.com",
"download_url": "https://files.pythonhosted.org/packages/09/fb/ae8cfc9b14beafe9c8670049652921009aaa2c53d8cdf3c834b4eb875477/pysdkit-0.4.12.tar.gz",
"platform": null,
"description": "# PySDKit: signal decomposition in Python\r\n\r\n<div align=\"center\">\r\n\r\n[](https://pypi.org/project/PySDKit/) \r\n\r\n[](https://www.python.org/)\r\n[](https://pepy.tech/project/pysdkit)\r\n\r\n[](https://github.com/psf/black)\r\n\r\nA Python library for signal decomposition algorithms \ud83e\udd73\r\n\r\n[Installation](#Installation) |\r\n[Example Script](#Example-Script) |\r\n[Target](#Target) |\r\n[Acknowledgements](#Acknowledgements)\r\n\r\n<img src=\"https://raw.githubusercontent.com/wwhenxuan/PySDKit/main/images/Logo_sd.png\" alt=\"Logo_sd\" width=\"500\"/>\r\n\r\n</div>\r\n\r\n## Installation \ud83d\ude80 <a id=\"Installation\"></a>\r\n\r\nYou can install `PySDKit` through pip:\r\n\r\n~~~\r\npip install pysdkit\r\n~~~\r\n\r\nWe only used [`NumPy`](https://numpy.org/), [`Scipy`](https://scipy.org/) and [`matplotlib`](https://matplotlib.org/) when developing the project.\r\n\r\n## Example Script \u2728 <a id=\"Example-Script\"></a>\r\n\r\nThis project integrates simple signal processing methods, signal decomposition and visualization, and builds a general interface similar to [`Scikit-learn`](https://scikit-learn.org/stable/). It is mainly divided into three steps:\r\n1. Import the signal decomposition method;\r\n2. Create an instance for signal decomposition;\r\n3. Use the `fit_transform` method to implement signal decomposition;\r\n4. Visualize and analyze the original signal and the intrinsic mode functions IMFs obtained by decomposition.\r\n\r\n~~~python\r\nfrom pysdkit import EMD\r\nfrom pysdkit.data import test_emd\r\nfrom pysdkit.plot import plot_IMFs\r\n\r\nt, signal = test_emd()\r\n\r\n# create an instance for signal decomposition\r\nemd = EMD()\r\n# implement signal decomposition\r\nIMFs = emd.fit_transform(signal, max_imfs=2)\r\nplot_IMFs(signal, IMFs)\r\n~~~\r\n\r\n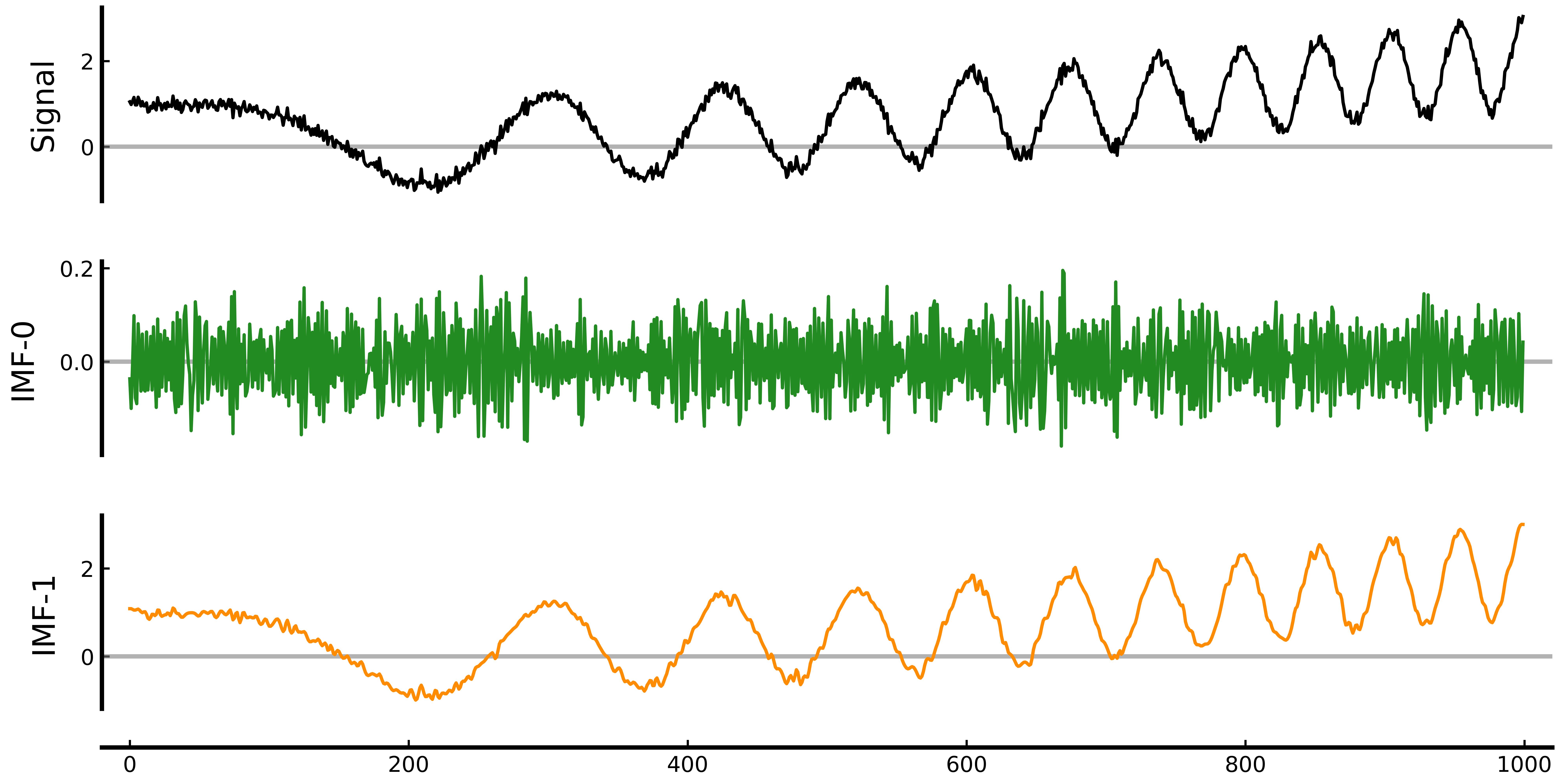\r\n\r\nThe EMD in the above example is the most classic [`empirical mode decomposition`](https://www.mathworks.com/help/signal/ref/emd.html) algorithm in signal decomposition. For more complex signals, you can try other algorithms such as variational mode decomposition ([`VMD`](https://ieeexplore.ieee.org/abstract/document/6655981)).\r\n\r\n~~~python\r\nimport numpy as np\r\nfrom pysdkit import VMD\r\n\r\n# load new signal\r\nsignal = np.load(\"./example/example.npy\")\r\n\r\n# use variational mode decomposition\r\nvmd = VMD(alpha=500, K=3, tau=0.0, tol=1e-9)\r\nIMFs = vmd.fit_transform(signal=signal)\r\nprint(IMFs.shape)\r\n\r\nvmd.plot_IMFs(save_figure=True)\r\n~~~\r\n\r\n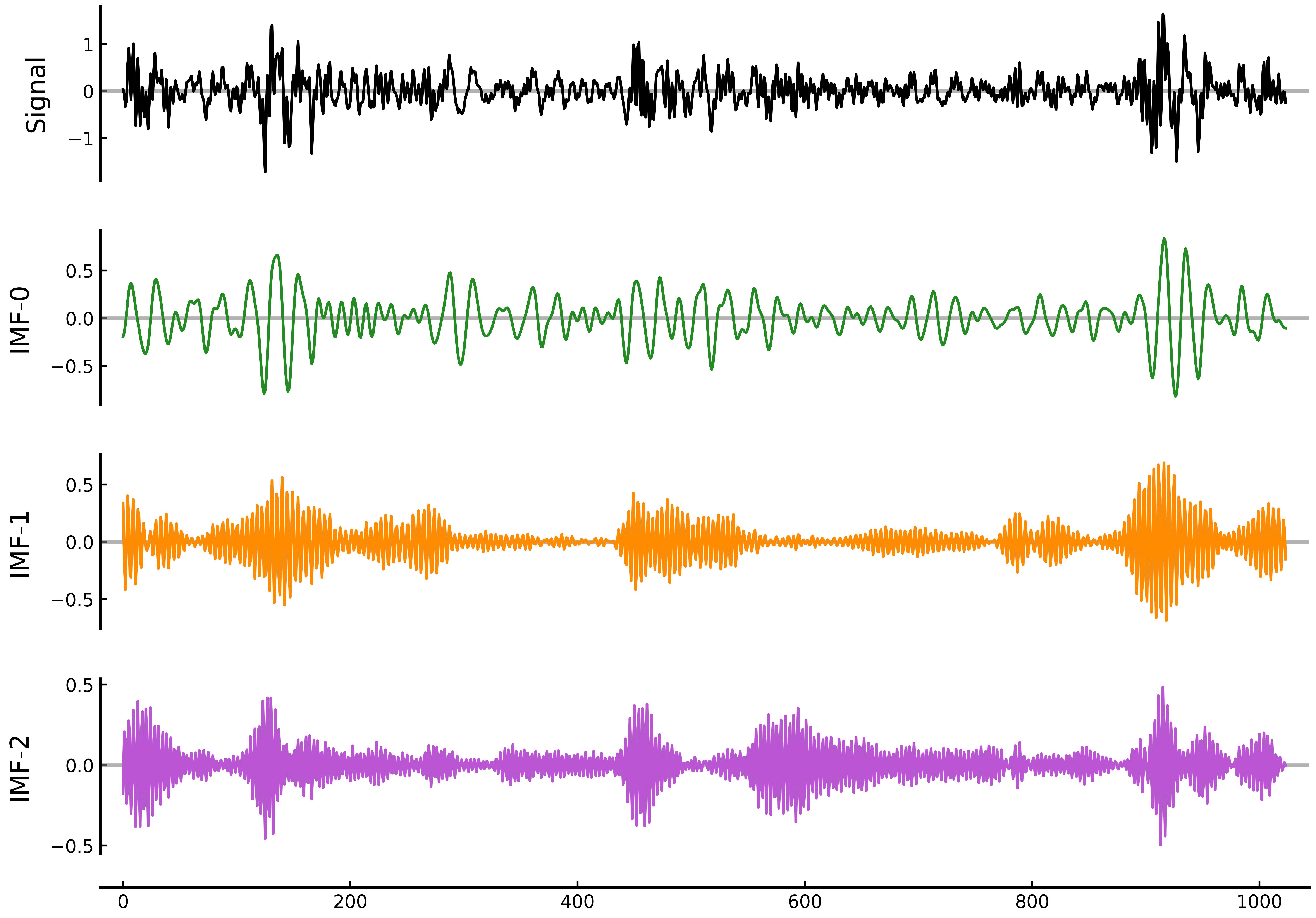\r\n\r\nBetter observe the characteristics of the decomposed intrinsic mode function in the frequency domain.\r\n\r\n~~~python\r\nfrom pysdkit.plot import plot_IMFs_amplitude_spectra\r\n\r\n# frequency domain visualization\r\nplot_IMFs_amplitude_spectra(IMFs, smooth=\"exp\") # use exp smooth\r\n~~~\r\n\r\n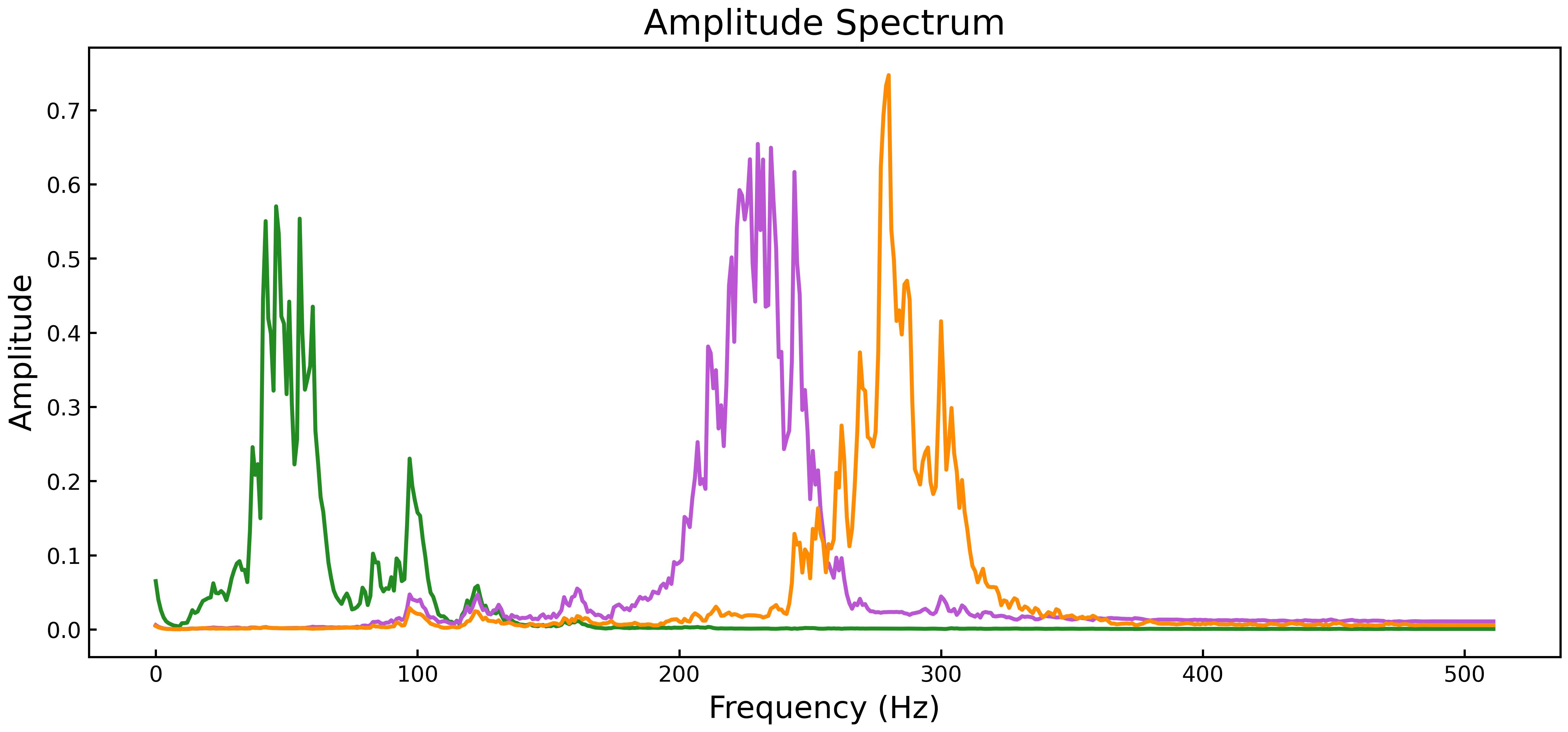\r\n\r\n## Target \ud83c\udfaf <a id=\"Target\"></a>\r\n\r\n`PySDKit` is still under development. We are currently working on reproducing the signal decomposition algorithms in the table below, including not only common decomposition algorithms for `univariate signals` such as EMD and VMD, but also decomposition algorithms for `multivariate signals` such as MEMD and MVMD. We will also further reproduce the decomposition algorithms for `two-dimensional images` to make PySDKit not only suitable for signal processing, but also for image analysis and understanding.\r\n\r\n| Algorithm | Paper | Code | State |\r\n| :----------------------------------------------------------: | :----------------------------------------------------------: | :----------------------------------------------------------: | :---: |\r\n| [`EMD`](https://github.com/wwhenxuan/PySDKit/blob/main/pysdkit/emd/EMD.py) (Empirical Mode Decomposition) | [[paper]](https://royalsocietypublishing.org/doi/abs/10.1098/rspa.1998.0193) | [[code]](https://www.mathworks.com/help/signal/ref/emd.html) | \u2714\ufe0f |\r\n| [`MEMD`](https://github.com/wwhenxuan/PySDKit/blob/main/pysdkit/emd/memd.py) (Multivariate Empirical Mode Decomposition) | [[paper]](https://royalsocietypublishing.org/doi/full/10.1098/rspa.2009.0502) | [[code]](https://www.mathworks.com/matlabcentral/fileexchange/71270-fast-and-adaptive-multivariate-and-multidimensional-emd) | \u2714\ufe0f |\r\n| [`BEMD`](https://github.com/wwhenxuan/PySDKit/blob/main/pysdkit/emd2d/bemd.py) (Bidimensional Empirical Mode Decomposition) | [[paper]](https://www.sciencedirect.com/science/article/abs/pii/S0262885603000945) | [[code]](https://github.com/laszukdawid/PyEMD/blob/master/PyEMD/BEMD.py) | \u2716\ufe0f |\r\n| [`CEMD`]() (Complex Empirical Mode Decomposition) | [[paper]](https://ieeexplore.ieee.org/abstract/document/4063369) | [[code]]() | \u2716\ufe0f |\r\n| [`EEMD`](https://github.com/wwhenxuan/PySDKit/blob/main/pysdkit/emd/eemd.py) (Ensemble Empirical Mode Decomposition) | [[paper]](https://www.sciencedirect.com/topics/physics-and-astronomy/ensemble-empirical-mode-decomposition) | [[code]](https://github.com/laszukdawid/PyEMD/blob/master/PyEMD/EEMD.py) | \u2714\ufe0f |\r\n| [`REMD`](https://github.com/wwhenxuan/PySDKit/blob/main/pysdkit/emd/remd.py) (Robust Empirical Mode Decomposition) | [[paper]](https://www.sciencedirect.com/science/article/pii/S0019057821003785) | [[code]](https://www.mathworks.com/matlabcentral/fileexchange/70032-robust-empirical-mode-decomposition-remd) | \u2716\ufe0f |\r\n| [`BMEMD`](https://github.com/wwhenxuan/PySDKit/blob/main/pysdkit/emd2d/bmemd.py) (Bidimensional Multivariate Empirical Mode Decomposition) | [[paper]](https://ieeexplore.ieee.org/document/8805082) | [[code]](https://www.mathworks.com/matlabcentral/fileexchange/72343-bidimensional-multivariate-empirical-mode-decomposition?s_tid=FX_rc1_behav) | \u2716\ufe0f |\r\n| [`CEEMDAN`](https://github.com/wwhenxuan/PySDKit/blob/main/pysdkit/emd/ceemdan.py) (Complete Ensemble EMD with Adaptive Noise) | [[paper]](https://ieeexplore.ieee.org/document/5947265) | [[code]](https://github.com/laszukdawid/PyEMD/blob/master/PyEMD/EEMD.py) | \u2714\ufe0f |\r\n| [`TVF_EMD`](https://github.com/wwhenxuan/PySDKit/blob/main/pysdkit/tvf_emd/tvf_emd.py) (Time Varying Filter Based EMD) | [[paper]](https://www.sciencedirect.com/science/article/pii/S0165168417301135) | [[code]](https://github.com/stfbnc/pytvfemd/tree/master) | \u2714\ufe0f |\r\n| [`FAEMD`](https://github.com/wwhenxuan/PySDKit/blob/main/pysdkit/faemd/faemd.py) (Fast and Adaptive EMD) | [[paper]](https://ieeexplore.ieee.org/document/8447300) | [[code]](https://www.mathworks.com/matlabcentral/fileexchange/71270-fast-and-adaptive-multivariate-and-multidimensional-emd) | \u2716\ufe0f |\r\n| [`MV_FAEMD`]() (Multivariate Fast and Adaptive EMD) | [[paper]](https://ieeexplore.ieee.org/document/8447300) | [[code]](https://www.mathworks.com/matlabcentral/fileexchange/71270-fast-and-adaptive-multivariate-and-multidimensional-emd) | \u2716\ufe0f |\r\n| [`MD_FAEMD`](https://github.com/wwhenxuan/PySDKit/blob/main/pysdkit/faemd/md_faemd.py) (Multidimensional Fast and Adaptive EMD) | [[paper]](https://ieeexplore.ieee.org/document/8447300) | [[code]](https://www.mathworks.com/matlabcentral/fileexchange/71270-fast-and-adaptive-multivariate-and-multidimensional-emd) | \u2716\ufe0f |\r\n| [`HVD`](https://github.com/wwhenxuan/PySDKit/blob/main/pysdkit/hvd/hvd.py) (Hilbert Vibration Decomposition) | [[paper]](https://www.sciencedirect.com/science/article/pii/S0022460X06001556) | [[code]](https://www.mathworks.com/matlabcentral/fileexchange/178804-hilbert-vibration-decomposition?s_tid=FX_rc1_behav) | \u2714\ufe0f |\r\n| [`ITD`](https://github.com/wwhenxuan/PySDKit/blob/main/pysdkit/itd/itd.py) (Intrinsic Time-Scale Decomposition) | [[paper]](https://royalsocietypublishing.org/doi/10.1098/rspa.2006.1761) | [[code]](https://www.mathworks.com/matlabcentral/fileexchange/69380-intrinsic-time-scale-decomposition-itd) | \u2714\ufe0f |\r\n| [`LMD`](https://github.com/wwhenxuan/PySDKit/blob/main/pysdkit/lmd/lmd.py) (Local Mean Decomposition) | [[paper]](https://www.sciencedirect.com/science/article/abs/pii/S1051200418308133?via%3Dihub) | [[code]](https://github.com/shownlin/PyLMD/blob/master/PyLMD/LMD.py) | \u2714\ufe0f |\r\n| [`RLMD`](https://github.com/wwhenxuan/PySDKit/blob/main/pysdkit/lmd/rlmd.py) (Robust Local Mean Decomposition) | [[paper]](https://www.sciencedirect.com/science/article/abs/pii/S0888327017301619) | [[code]](https://www.mathworks.com/matlabcentral/fileexchange/66935-robust-local-mean-decomposition-rlmd) | \u2716\ufe0f |\r\n| [`FMD`](https://github.com/wwhenxuan/PySDKit/blob/main/pysdkit/fmd/fmd.py) (Feature Mode Decomposition) | [[paper]](https://ieeexplore.ieee.org/document/9732251) | [[code]](https://www.mathworks.com/matlabcentral/fileexchange/108099-feature-mode-decomposition-fmd) | \u2716\ufe0f |\r\n| [`SSA`](https://github.com/wwhenxuan/PySDKit/blob/main/pysdkit/ssa/ssa.py) (Singular Spectral Analysis) | [[paper]](https://orca.cardiff.ac.uk/id/eprint/15208/1/Zhiglavsky_SSA_encyclopedia.pdf) | [[code]](https://www.mathworks.com/matlabcentral/fileexchange/58967-singular-spectrum-analysis-beginners-guide) | \u2714\ufe0f |\r\n| [`EWT`](https://github.com/wwhenxuan/PySDKit/blob/main/pysdkit/ewt/ewt.py) (Empirical Wavelet Transform) | [[paper]](https://ieeexplore.ieee.org/document/6522142) | [[code]](https://www.mathworks.com/help/wavelet/ug/empirical-wavelet-transform.html) | \u2714\ufe0f |\r\n| [`EWT2D`](https://github.com/wwhenxuan/PySDKit/blob/main/pysdkit/ewt/ewt2d.py) (2D Empirical Wavelet Transform) | [[paper]](https://arxiv.org/abs/2405.06188) | [[code]](https://github.com/bhurat/EWT-Python) | \u2716\ufe0f |\r\n| [`VMD`](https://github.com/wwhenxuan/PySDKit/blob/main/pysdkit/vmd/vmd_c.py) (Variational Mode Decomposition) | [[paper]](https://ieeexplore.ieee.org/document/6655981) | [[code]](https://github.com/vrcarva/vmdpy) | \u2714\ufe0f |\r\n| [`MVMD`](https://github.com/wwhenxuan/PySDKit/blob/main/pysdkit/vmd/mvmd.py) (Multivariate Variational Mode Decomposition) | [[paper]](https://ieeexplore.ieee.org/document/8890883) | [[code]](https://github.com/yunyueye/MVMD) | \u2714\ufe0f |\r\n| [`VMD2D`](https://github.com/wwhenxuan/PySDKit/blob/main/pysdkit/vmd2d/vmd2d.py) (Two-Dimensional Variational Mode Decomposition) | [[paper]](https://ww3.math.ucla.edu/camreport/cam14-16.pdf) | [[code]](https://www.mathworks.com/matlabcentral/fileexchange/45918-two-dimensional-variational-mode-decomposition?s_tid=srchtitle) | \u2714\ufe0f |\r\n| [`SVMD`](https://github.com/wwhenxuan/PySDKit/blob/main/pysdkit/vmd/svmd.py) (Successive Variational Mode Decomposition) | [[paper]](https://www.sciencedirect.com/science/article/pii/S0165168420301535) | [[code]](https://www.mathworks.com/matlabcentral/fileexchange/98649-successive-variational-mode-decomposition-svmd-m?s_tid=FX_rc3_behav) | \u2716\ufe0f |\r\n| [`VNCMD`](https://github.com/wwhenxuan/PySDKit/blob/main/pysdkit/vncmd/vncmd.py) (Variational Nonlinear Chirp Mode Decomposition) | [[paper]](https://ieeexplore.ieee.org/document/7990179) | [[code]](https://www.mathworks.com/matlabcentral/fileexchange/64292-variational-nonlinear-chirp-mode-decomposition) | \u2714\ufe0f |\r\n| [`MNCMD`](https://github.com/wwhenxuan/PySDKit/blob/main/pysdkit/vncmd/mncmd.py) (Multivariate Nonlinear Chirp Mode Decomposition) | [[paper]](https://www.sciencedirect.com/science/article/abs/pii/S0165168420302103) | [[code]]() | \u2716\ufe0f |\r\n| [`AVNCMD`](https://github.com/wwhenxuan/PySDKit/blob/main/pysdkit/vncmd/avncmd.py) (Adaptive Variational Nonlinear Chirp Mode Decomposition) | [[paper]](https://ieeexplore.ieee.org/abstract/document/9746147) | [[code]](https://github.com/HauLiang/AVNCMD) | \u2716\ufe0f |\r\n| [`ACMD`](https://github.com/wwhenxuan/PySDKit/blob/main/pysdkit/vmd/acmd.py) (Adaptive Chirp Mode Decomposition) | [[paper]]() | [[code]](https://www.mathworks.com/matlabcentral/fileexchange/121373-data-driven-adaptive-chirp-mode-decomposition?s_tid=srchtitle) | \u2714\ufe0f |\r\n| [`BA-ACMD`](https://github.com/wwhenxuan/PySDKit/blob/main/pysdkit/vmd/ba_acmd.py) (Bandwidth-aware adaptive chirp mode decomposition) | [[paper]](https://journals.sagepub.com/doi/abs/10.1177/14759217231174699) | [[code]](https://www.mathworks.com/matlabcentral/fileexchange/132792-bandwidth-aware-adaptive-chirp-mode-decomposition-ba-acmd?s_tid=srchtitle) | \u2716\ufe0f |\r\n| [`JMD`](https://github.com/wwhenxuan/PySDKit/blob/main/pysdkit/jmd/jmd.py) (Jump Plus AM-FM Mode Decomposition) | [[paper]](https://arxiv.org/abs/2407.07800) | [[code]](https://www.mathworks.com/matlabcentral/fileexchange/169388-jump-plus-am-fm-mode-decomposition-jmd?s_tid=prof_contriblnk) | \u2716\ufe0f |\r\n| [`MJMD`](https://github.com/wwhenxuan/PySDKit/blob/main/pysdkit/jmd/mjmd.py) (Multivariate Jump Plus AM-FM Mode Decomposition) | [[paper]](https://arxiv.org/abs/2407.07800) | [[code]](https://ms-intl.mathworks.com/matlabcentral/fileexchange/169393-multivariate-jump-plus-am-fm-mode-decomposition-mjmd?s_tid=FX_rc2_behav) | \u2716\ufe0f |\r\n| [`ESMD`]() (Extreme-Point Symmetric Mode Decomposition) | [[paper]](https://arxiv.org/abs/1303.6540) | [[code]](https://github.com/WuShichao/esmd) | \u2716\ufe0f |\r\n\r\n## Acknowledgements \ud83c\udf96\ufe0f <a id=\"Acknowledgements\"></a>\r\n\r\nWe would like to thank the researchers in signal processing for providing us with valuable algorithms and promoting the continuous progress in this field. However, since the main programming language used in `signal processing` is `Matlab`, and `Python` is the main battlefield of `machine learning` and `deep learning`, the usage of signal decomposition in machine learning and deep learning is far less extensive than `wavelet transformation`. In order to further promote the organic combination of signal decomposition and machine learning, we developed `PySDKit`. We would like to express our gratitude to [PyEMD](https://github.com/laszukdawid/PyEMD), [Sktime](https://www.sktime.net/en/latest/index.html), [vmdpy](https://github.com/vrcarva/vmdpy), [MEMD-Python-](https://github.com/mariogrune/MEMD-Python-), [ewtpy](https://github.com/vrcarva/ewtpy), [EWT-Python](https://github.com/bhurat/EWT-Python), [PyLMD](https://github.com/shownlin/PyLMD), [pywt](https://github.com/PyWavelets/pywt), [SP_Lib](https://github.com/hustcxl/SP_Lib)and [dsatools](https://github.com/MVRonkin/dsatools).\r\n",
"bugtrack_url": null,
"license": null,
"summary": "A Python library for signal decomposition algorithms with a unified interface.",
"version": "0.4.12",
"project_urls": {
"Homepage": "https://github.com/wwhenxuan/PySDKit"
},
"split_keywords": [
"signal decomposition",
" signal processing",
" machine learning"
],
"urls": [
{
"comment_text": null,
"digests": {
"blake2b_256": "bc09fedd7d4e27992ac17895ad3536e15b72de6e478916c1a71fcd24c96d23f5",
"md5": "90de1cd51b2970087de19e06e9cfee84",
"sha256": "dcf73e15b1bc676d16d52ce25396804e0432a2e4da3baed02641beb640586932"
},
"downloads": -1,
"filename": "PySDKit-0.4.12-py3-none-any.whl",
"has_sig": false,
"md5_digest": "90de1cd51b2970087de19e06e9cfee84",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.6",
"size": 670652,
"upload_time": "2025-02-09T05:04:53",
"upload_time_iso_8601": "2025-02-09T05:04:53.850328Z",
"url": "https://files.pythonhosted.org/packages/bc/09/fedd7d4e27992ac17895ad3536e15b72de6e478916c1a71fcd24c96d23f5/PySDKit-0.4.12-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "09fbae8cfc9b14beafe9c8670049652921009aaa2c53d8cdf3c834b4eb875477",
"md5": "388f0fcb5b39656ed77a7230c41a7ad5",
"sha256": "180cfea5a91397dcee64446aa8a352a6087d4fa1c0323ca7f6546c7dc878eb3c"
},
"downloads": -1,
"filename": "pysdkit-0.4.12.tar.gz",
"has_sig": false,
"md5_digest": "388f0fcb5b39656ed77a7230c41a7ad5",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.6",
"size": 633970,
"upload_time": "2025-02-09T05:04:55",
"upload_time_iso_8601": "2025-02-09T05:04:55.472668Z",
"url": "https://files.pythonhosted.org/packages/09/fb/ae8cfc9b14beafe9c8670049652921009aaa2c53d8cdf3c834b4eb875477/pysdkit-0.4.12.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2025-02-09 05:04:55",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "wwhenxuan",
"github_project": "PySDKit",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"requirements": [
{
"name": "numpy",
"specs": [
[
">=",
"1.24.3"
]
]
},
{
"name": "scipy",
"specs": [
[
">=",
"1.11.1"
]
]
},
{
"name": "matplotlib",
"specs": [
[
">=",
"3.7.2"
]
]
},
{
"name": "tqdm",
"specs": [
[
">=",
"4.66.5"
]
]
},
{
"name": "requests",
"specs": [
[
">=",
"2.32.3"
]
]
}
],
"lcname": "pysdkit"
}