Name | brep-part-finder JSON |
Version |
0.5.2
JSON |
| download |
home_page | |
Summary | A Python package to identify the part ID number in Brep format CAD files |
upload_time | 2022-12-07 14:20:39 |
maintainer | |
docs_url | None |
author | |
requires_python | >=3.8 |
license | MIT License Copyright (c) 2021-2022 Fusion Energy Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. |
keywords |
brep
geometry
part
find
identify
volume
|
VCS |
|
bugtrack_url |
|
requirements |
No requirements were recorded.
|
Travis-CI |
No Travis.
|
coveralls test coverage |
No coveralls.
|
[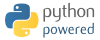](https://www.python.org)
[](https://github.com/fusion-energy/brep_part_finder/actions/workflows/ci_with_install.yml)
[](https://github.com/fusion-energy/brep_part_finder/actions/workflows/python-publish.yml)
[](https://github.com/fusion-energy/brep_part_finder/actions/workflows/anaconda-publish.yml)
[](https://anaconda.org/fusion-energy/brep_part_finder)
[](https://pypi.org/project/brep_part_finder/)
Brep-part-finder is able to search within a Brep file for parts that match user
specified properties such as volume, center of mass and bounding box. The
matching ID number of the part will be returned if found.
This is useful because the order or parts changes when exporting to Brep files.
This part finder package helps keep track of meta data within a workflow.
The [neutronics workflow](https://github.com/fusion-energy/neutronics-workshop)
makes use of this package to help correctly identify materials when making
[DAGMC](https://github.com/svalinn/DAGMC/) h5m files from
[Paramak](https://github.com/fusion-energy/paramak) geometries for neutronics
simulations.
# Installation (Conda)
The installation instructions below create a new conda enviroment and install
```bash
conda create --name bpf_env
conda activate bpf_env
conda install -c cadquery -c fusion-energy -c conda-forge brep_part_finder
```
# Installation (Conda + Pip)
The installation instructions below create a new conda enviroment, install CadQuery and install this package.
The master branch of CadQuery is currently required as latest features are required.
When CadQuery version 2.2 is released then install can target a stable version.
```bash
conda create --name bpf_env
conda activate bpf_env
conda install -c cadquery -c conda-forge cadquery=master
pip install brep_part_finder
```
# Usage
To view the properties of the parts in the Brep file the first stage is to import the package and make use of the ```get_brep_part_properties``` function.
```
import brep_part_finder as bpf
my_brep_part_properties = bpf.get_brep_part_properties('my_brep_file.brep')
print(my_brep_part_properties)
>>>{
1: {
'Center.x': 0, 'Center.y': 0, 'Center.z': 0,
'Volume': 10,
'BoundingBox.xmin': -20, 'BoundingBox.ymin': -20, 'BoundingBox.zmin': -20,
'BoundingBox.xmax': 20, 'BoundingBox.ymax': 20, 'BoundingBox.zmax': 20
},
2: {
'Center.x': 5, 'Center.y': 6, 'Center.z': 7,
'Volume': 10,
'BoundingBox.xmin': -40.0, 'BoundingBox.ymin': -40.0, 'BoundingBox.zmin': -40.0,
'BoundingBox.xmax': 40.0, 'BoundingBox.ymax': 40.0, 'BoundingBox.zmax': 40.0
},
3: {
'Center.x': 0, 'Center.y': 0, 'Center.z': 0,
'Volume': 10,
'BoundingBox.xmin': -10, 'BoundingBox.ymin': -10, 'BoundingBox.zmin': -10,
'BoundingBox.xmax': 10, 'BoundingBox.ymax': 10, 'BoundingBox.zmax': 10
}
}
```
From the above dictionary it is possible to identify parts from their central of mass (x,y,z coordinate), volume and bounding box. This can be done manually or one can pass the required properties into the ```find_part_id``` or ```find_part_ids``` functions to identify the part numbers of solids automatically.
A minimal example that finds the part id numbers with matching volumes
```python
import brep_part_finder as bpf
my_brep_part_properties = bpf.get_brep_part_properties('my_brep_file.brep')
part_id = bpf.find_part_id(
brep_part_properties=my_brep_part_properties,
volume=10,
)
print(part_id)
>> [1, 3, 4]
```
The above example found 3 part ids with matching volumes.
The follow example also specifies the center of mass which helps narrow down the part ids to just to matching parts.
```python
import brep_part_finder as bpf
my_brep_part_properties = bpf.get_brep_part_properties('my_brep_file.brep')
part_id = bpf.find_part_id(
brep_part_properties=my_brep_part_properties,
volume=10,
center_of_mass=(0,0,0),
)
print(part_id)
>> [1, 3]
```
In the this example the bounding box of the part has also been specified and these three pieces of information are enough to find one part that matches all three criteria.
```python
import brep_part_finder as bpf
my_brep_part_properties = bpf.get_brep_part_properties('my_brep_file.brep')
part_id = bpf.find_part_id(
brep_part_properties=my_brep_part_properties,
volume=10,
center_of_mass=(0,0,0),
bounding_box = [[10,10,10], [-10,-10,10]]
)
print(part_id)
>> [3]
```
For more usage examples see the [examples](https://github.com/fusion-energy/brep_part_finder/tree/main/examples) folder in this repository
# Combining with Paramak
When reactor models made with Paramak are exported to Brep files it is likely that the order of parts in the Brep file does not match the order of parts within the Paramak object. Therefore this program is useful when identifying parts in the Brep file. See the [paramak_example](https://github.com/fusion-energy/brep_part_finder/blob/main/examples/paramak_example.py) file in the examples folder of this repository.
Raw data
{
"_id": null,
"home_page": "",
"name": "brep-part-finder",
"maintainer": "",
"docs_url": null,
"requires_python": ">=3.8",
"maintainer_email": "",
"keywords": "brep,geometry,part,find,identify,volume",
"author": "",
"author_email": "Jonathan Shimwell <mail@jshimwell.com>",
"download_url": "https://files.pythonhosted.org/packages/f2/46/f8001047e1da149af51a1bf144fcaa89257d128134e32e7077940df806f6/brep_part_finder-0.5.2.tar.gz",
"platform": null,
"description": "[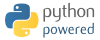](https://www.python.org)\n\n[](https://github.com/fusion-energy/brep_part_finder/actions/workflows/ci_with_install.yml)\n\n[](https://github.com/fusion-energy/brep_part_finder/actions/workflows/python-publish.yml)\n[](https://github.com/fusion-energy/brep_part_finder/actions/workflows/anaconda-publish.yml)\n\n[](https://anaconda.org/fusion-energy/brep_part_finder)\n[](https://pypi.org/project/brep_part_finder/)\n\nBrep-part-finder is able to search within a Brep file for parts that match user\nspecified properties such as volume, center of mass and bounding box. The\nmatching ID number of the part will be returned if found.\n\nThis is useful because the order or parts changes when exporting to Brep files.\nThis part finder package helps keep track of meta data within a workflow.\nThe [neutronics workflow](https://github.com/fusion-energy/neutronics-workshop)\nmakes use of this package to help correctly identify materials when making\n[DAGMC](https://github.com/svalinn/DAGMC/) h5m files from \n[Paramak](https://github.com/fusion-energy/paramak) geometries for neutronics\nsimulations.\n\n# Installation (Conda)\n\nThe installation instructions below create a new conda enviroment and install \n\n```bash\nconda create --name bpf_env\nconda activate bpf_env\nconda install -c cadquery -c fusion-energy -c conda-forge brep_part_finder\n```\n\n# Installation (Conda + Pip)\n\nThe installation instructions below create a new conda enviroment, install CadQuery and install this package.\nThe master branch of CadQuery is currently required as latest features are required.\nWhen CadQuery version 2.2 is released then install can target a stable version.\n\n```bash\nconda create --name bpf_env\nconda activate bpf_env\nconda install -c cadquery -c conda-forge cadquery=master\npip install brep_part_finder\n```\n\n# Usage\n\nTo view the properties of the parts in the Brep file the first stage is to import the package and make use of the ```get_brep_part_properties``` function.\n\n```\nimport brep_part_finder as bpf\n\nmy_brep_part_properties = bpf.get_brep_part_properties('my_brep_file.brep')\n\nprint(my_brep_part_properties)\n>>>{\n 1: {\n 'Center.x': 0, 'Center.y': 0, 'Center.z': 0,\n 'Volume': 10,\n 'BoundingBox.xmin': -20, 'BoundingBox.ymin': -20, 'BoundingBox.zmin': -20,\n 'BoundingBox.xmax': 20, 'BoundingBox.ymax': 20, 'BoundingBox.zmax': 20\n },\n 2: {\n 'Center.x': 5, 'Center.y': 6, 'Center.z': 7,\n 'Volume': 10,\n 'BoundingBox.xmin': -40.0, 'BoundingBox.ymin': -40.0, 'BoundingBox.zmin': -40.0,\n 'BoundingBox.xmax': 40.0, 'BoundingBox.ymax': 40.0, 'BoundingBox.zmax': 40.0\n },\n 3: {\n 'Center.x': 0, 'Center.y': 0, 'Center.z': 0,\n 'Volume': 10,\n 'BoundingBox.xmin': -10, 'BoundingBox.ymin': -10, 'BoundingBox.zmin': -10,\n 'BoundingBox.xmax': 10, 'BoundingBox.ymax': 10, 'BoundingBox.zmax': 10\n } \n }\n```\n\nFrom the above dictionary it is possible to identify parts from their central of mass (x,y,z coordinate), volume and bounding box. This can be done manually or one can pass the required properties into the ```find_part_id``` or ```find_part_ids``` functions to identify the part numbers of solids automatically.\n\nA minimal example that finds the part id numbers with matching volumes\n```python\nimport brep_part_finder as bpf\n\nmy_brep_part_properties = bpf.get_brep_part_properties('my_brep_file.brep')\npart_id = bpf.find_part_id(\n brep_part_properties=my_brep_part_properties,\n volume=10,\n\n)\n\nprint(part_id)\n>> [1, 3, 4]\n```\n\nThe above example found 3 part ids with matching volumes.\n\nThe follow example also specifies the center of mass which helps narrow down the part ids to just to matching parts.\n```python\nimport brep_part_finder as bpf\n\nmy_brep_part_properties = bpf.get_brep_part_properties('my_brep_file.brep')\npart_id = bpf.find_part_id(\n brep_part_properties=my_brep_part_properties,\n volume=10,\n center_of_mass=(0,0,0),\n)\n\nprint(part_id)\n>> [1, 3]\n```\n\nIn the this example the bounding box of the part has also been specified and these three pieces of information are enough to find one part that matches all three criteria.\n```python\nimport brep_part_finder as bpf\n\nmy_brep_part_properties = bpf.get_brep_part_properties('my_brep_file.brep')\npart_id = bpf.find_part_id(\n brep_part_properties=my_brep_part_properties,\n volume=10,\n center_of_mass=(0,0,0),\n bounding_box = [[10,10,10], [-10,-10,10]]\n)\n\nprint(part_id)\n>> [3]\n```\n\nFor more usage examples see the [examples](https://github.com/fusion-energy/brep_part_finder/tree/main/examples) folder in this repository\n\n# Combining with Paramak\n\nWhen reactor models made with Paramak are exported to Brep files it is likely that the order of parts in the Brep file does not match the order of parts within the Paramak object. Therefore this program is useful when identifying parts in the Brep file. See the [paramak_example](https://github.com/fusion-energy/brep_part_finder/blob/main/examples/paramak_example.py) file in the examples folder of this repository.\n",
"bugtrack_url": null,
"license": "MIT License Copyright (c) 2021-2022 Fusion Energy Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the \"Software\"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED \"AS IS\", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. ",
"summary": "A Python package to identify the part ID number in Brep format CAD files",
"version": "0.5.2",
"split_keywords": [
"brep",
"geometry",
"part",
"find",
"identify",
"volume"
],
"urls": [
{
"comment_text": "",
"digests": {
"md5": "0bdb2c9177b2136452ef0fd069e6a6e2",
"sha256": "314a18f634487c8e507188342a5d943bf67ddb492eab57af6eb80ce9e54e773f"
},
"downloads": -1,
"filename": "brep_part_finder-0.5.2-py3-none-any.whl",
"has_sig": false,
"md5_digest": "0bdb2c9177b2136452ef0fd069e6a6e2",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.8",
"size": 8085,
"upload_time": "2022-12-07T14:20:37",
"upload_time_iso_8601": "2022-12-07T14:20:37.413356Z",
"url": "https://files.pythonhosted.org/packages/30/fe/f773a5a7d41698f1b5bbd1622b658ee2c171ae08c85ba3458ed384d8f64e/brep_part_finder-0.5.2-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"md5": "b5507f5b192587159d3e3a0e3e2b1350",
"sha256": "b42e9efb066727eb8deac485ee7f258e0760c893ff53db41bab3ab4baf4198d5"
},
"downloads": -1,
"filename": "brep_part_finder-0.5.2.tar.gz",
"has_sig": false,
"md5_digest": "b5507f5b192587159d3e3a0e3e2b1350",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.8",
"size": 80107,
"upload_time": "2022-12-07T14:20:39",
"upload_time_iso_8601": "2022-12-07T14:20:39.087515Z",
"url": "https://files.pythonhosted.org/packages/f2/46/f8001047e1da149af51a1bf144fcaa89257d128134e32e7077940df806f6/brep_part_finder-0.5.2.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2022-12-07 14:20:39",
"github": false,
"gitlab": false,
"bitbucket": false,
"lcname": "brep-part-finder"
}