# CyberChipped
[](https://pypi.org/project/cyberchipped/)
[](https://github.com/bevanhunt/cyberchipped/actions)
[](https://app.codecov.io/gh/bevanhunt/cyberchipped)
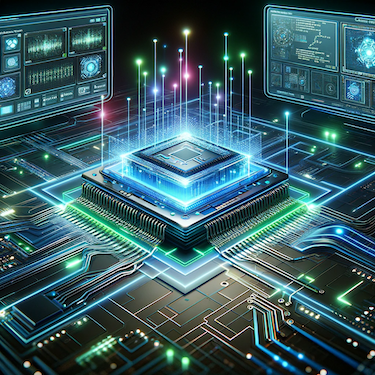
## Introduction
CyberChipped enables building powerful AI apps fast by providing three core abstractions.
These abstractions are the OpenAI Assistant, AI Function, and AI Model.
The key selling point of this library is to build an OpenAI Assistant in two lines of code!
CyberChipped powers the most feature-rich AI Companion - [CometHeart](https://cometheart.com)!
## Install
```bash
pip install cyberchipped
```
## Setup
Create a .env file in your project root with this key in it:
```bash
OPENAI_API_KEY=YOUR_OPENAI_API_KEY
```
## Abstractions
### OpenAI Assistant
```python
from cyberchipped.assistants import Assistant
with Assistant() as ai:
print(ai.say("Hello World!"))
# prints: "Hello there! How can I assist you today?"
```
### AI Function
```python
from cyberchipped import ai_fn
@ai_fn
def echo(text: str) -> str:
"""You return `text`."""
print(echo("Hello World!"))
# prints: "Hello World!"
```
### AI Model
```python
from cyberchipped import ai_model
from pydantic import BaseModel, Field
@ai_model
class Planet(BaseModel):
"""Planet Attributes"""
name: str = Field(..., description="The name of the planet.")
planet = Planet("Mars is a great place to visit!")
print(planet.name)
# prints: "Mars"
```
## Database
CyberChipped requires a database to track and manage OpenAI Assistant threads across runs.
### SQLite (default)
Will be saved in your local working directory (where you code is located) and be called `cyberchipped.db`. Unless you use MongoDB.
### MongoDB
Set the following env vars in your .env file:
```bash
MONGO_URL=YOUR_MONGO_URL
MONGO_DB=YOUR_DATABASE_NAME
MONGO_COLLECTION=YOUR_COLLECTION_NAME
```
## Source
This is a hard fork of [Marvin](https://askmarvin.ai) pre-release
## Platform Support
Mac and Linux
## Requirements
Python >= 3.11
Raw data
{
"_id": null,
"home_page": "https://cyberchipped.com",
"name": "cyberchipped",
"maintainer": "",
"docs_url": null,
"requires_python": ">=3.11",
"maintainer_email": "",
"keywords": "ai,openai",
"author": "Bevan Hunt",
"author_email": "bevan@bevanhunt.com",
"download_url": "https://files.pythonhosted.org/packages/3f/90/55751b82ab150c77ea0e32ba8e5707257ba3200bcf8a63b368054da29716/cyberchipped-0.8.0.tar.gz",
"platform": null,
"description": "# CyberChipped\n\n[](https://pypi.org/project/cyberchipped/)\n[](https://github.com/bevanhunt/cyberchipped/actions)\n[](https://app.codecov.io/gh/bevanhunt/cyberchipped)\n\n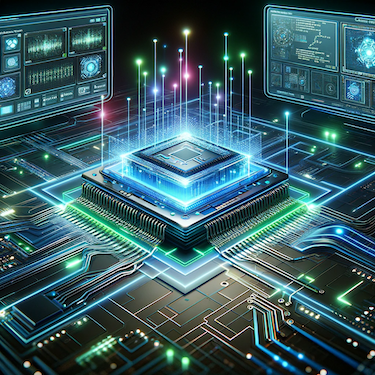\n\n## Introduction\nCyberChipped enables building powerful AI apps fast by providing three core abstractions.\n\nThese abstractions are the OpenAI Assistant, AI Function, and AI Model.\n\nThe key selling point of this library is to build an OpenAI Assistant in two lines of code!\n\nCyberChipped powers the most feature-rich AI Companion - [CometHeart](https://cometheart.com)!\n\n## Install\n\n```bash\npip install cyberchipped\n```\n\n## Setup\nCreate a .env file in your project root with this key in it:\n```bash\nOPENAI_API_KEY=YOUR_OPENAI_API_KEY\n```\n\n## Abstractions\n\n### OpenAI Assistant\n```python\nfrom cyberchipped.assistants import Assistant\n\n\nwith Assistant() as ai:\n print(ai.say(\"Hello World!\"))\n # prints: \"Hello there! How can I assist you today?\"\n```\n\n### AI Function\n```python\nfrom cyberchipped import ai_fn\n\n@ai_fn\ndef echo(text: str) -> str:\n \"\"\"You return `text`.\"\"\"\n\nprint(echo(\"Hello World!\"))\n# prints: \"Hello World!\"\n\n```\n\n### AI Model\n```python\nfrom cyberchipped import ai_model\nfrom pydantic import BaseModel, Field\n\n@ai_model\nclass Planet(BaseModel):\n \"\"\"Planet Attributes\"\"\"\n name: str = Field(..., description=\"The name of the planet.\")\n\nplanet = Planet(\"Mars is a great place to visit!\")\nprint(planet.name)\n# prints: \"Mars\"\n```\n\n## Database\nCyberChipped requires a database to track and manage OpenAI Assistant threads across runs.\n\n\n### SQLite (default)\nWill be saved in your local working directory (where you code is located) and be called `cyberchipped.db`. Unless you use MongoDB.\n\n### MongoDB\nSet the following env vars in your .env file:\n```bash\nMONGO_URL=YOUR_MONGO_URL\nMONGO_DB=YOUR_DATABASE_NAME\nMONGO_COLLECTION=YOUR_COLLECTION_NAME\n```\n\n## Source\nThis is a hard fork of [Marvin](https://askmarvin.ai) pre-release\n\n## Platform Support\nMac and Linux\n\n## Requirements\nPython >= 3.11\n\n",
"bugtrack_url": null,
"license": "Apache-2.0",
"summary": "Build AI Apps Fast",
"version": "0.8.0",
"project_urls": {
"Homepage": "https://cyberchipped.com",
"Repository": "https://github.com/bevanhunt/cyberchipped"
},
"split_keywords": [
"ai",
"openai"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "6df3f278203009f04d0974871911b0abe40681419fce76092cb274ada810edf7",
"md5": "e4b628f4c9df635d775742c68bbc0e33",
"sha256": "e854ab5ac9b40eeb209e5e23e07fcf19086b0b7e8af72e9b4773ba26be719539"
},
"downloads": -1,
"filename": "cyberchipped-0.8.0-py3-none-any.whl",
"has_sig": false,
"md5_digest": "e4b628f4c9df635d775742c68bbc0e33",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.11",
"size": 33891,
"upload_time": "2024-01-08T09:54:34",
"upload_time_iso_8601": "2024-01-08T09:54:34.823000Z",
"url": "https://files.pythonhosted.org/packages/6d/f3/f278203009f04d0974871911b0abe40681419fce76092cb274ada810edf7/cyberchipped-0.8.0-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "3f9055751b82ab150c77ea0e32ba8e5707257ba3200bcf8a63b368054da29716",
"md5": "500c3d5da3b11b621f6c5dda77ae3f7d",
"sha256": "3a91d0e78f84a99b303ef23c7e17b3feae39e958d12601cf4beb3c6b9d157459"
},
"downloads": -1,
"filename": "cyberchipped-0.8.0.tar.gz",
"has_sig": false,
"md5_digest": "500c3d5da3b11b621f6c5dda77ae3f7d",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.11",
"size": 24796,
"upload_time": "2024-01-08T09:54:36",
"upload_time_iso_8601": "2024-01-08T09:54:36.611967Z",
"url": "https://files.pythonhosted.org/packages/3f/90/55751b82ab150c77ea0e32ba8e5707257ba3200bcf8a63b368054da29716/cyberchipped-0.8.0.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-01-08 09:54:36",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "bevanhunt",
"github_project": "cyberchipped",
"travis_ci": false,
"coveralls": true,
"github_actions": true,
"lcname": "cyberchipped"
}